Understanding the Basics of Python for Beginners? Think less coding bootcamp, more “aha!” moments. This isn’t about becoming a Python ninja overnight; it’s about unlocking the power of this versatile language in a way that’s actually fun and surprisingly simple. We’ll demystify the jargon, ditch the intimidating code walls, and show you the surprisingly straightforward path to Python proficiency. Get ready to ditch the “I’m not a coder” mindset – because you’re about to become one.
We’ll cover everything from setting up your Python environment (seriously, it’s easier than you think) to mastering core concepts like data types, control flow, and functions. We’ll even tackle error handling – because even the pros face those pesky bugs. By the end, you’ll have a solid foundation to build upon, whether you dream of data science, web development, or just want to impress your friends with your newfound coding skills. Let’s dive in!
Introduction to Python
Python, a name that might conjure images of slithering reptiles, actually refers to one of the most popular and beginner-friendly programming languages in the world. It’s a versatile tool used everywhere from building websites and analyzing data to automating tasks and even creating games. Understanding its basics is a gateway to a world of possibilities.
Python’s journey began in the late 1980s, born from a desire for a clear, readable, and efficient language. Guido van Rossum, its creator, aimed to build a language that prioritized programmer happiness and productivity. This focus on readability, along with its extensive libraries and supportive community, has contributed significantly to its widespread adoption. Its primary purpose is to provide a powerful yet accessible tool for a wide range of programming tasks.
Advantages of Learning Python for Beginners
Python’s simplicity and readability make it an ideal starting point for anyone venturing into the world of programming. Unlike some languages that require intricate syntax and complex structures, Python emphasizes clarity. This allows beginners to focus on understanding programming concepts rather than getting bogged down in the intricacies of the language itself. Its vast community support means finding answers to questions and help with problems is relatively easy. Numerous online resources, tutorials, and forums are dedicated to assisting Python learners of all levels. The availability of extensive libraries simplifies complex tasks, allowing beginners to achieve tangible results quickly, boosting their confidence and motivation.
Setting Up a Python Environment
Setting up your Python environment is straightforward. First, download the latest version of Python from the official Python website (python.org). Choose the installer appropriate for your operating system (Windows, macOS, or Linux). During installation, ensure you check the box to add Python to your PATH environment variable. This allows you to run Python from your command line or terminal without navigating to the Python installation directory. After installation, open your command line or terminal and type `python –version` to verify the installation. If Python is correctly installed, you’ll see the version number displayed. For a more integrated development experience, consider using a code editor like VS Code, Sublime Text, or PyCharm. These editors provide features like syntax highlighting, code completion, and debugging tools, making the coding process much smoother.
Resources for Beginners
The internet is a treasure trove of resources for aspiring Python programmers. Websites like Codecademy, Coursera, and edX offer interactive Python courses for beginners. YouTube channels dedicated to programming tutorials provide visual learning experiences. The official Python documentation is a comprehensive resource for in-depth information. Finally, engaging with online communities such as Stack Overflow and Reddit’s r/learnpython can provide valuable support and guidance from experienced programmers. Don’t hesitate to ask questions – the Python community is generally very welcoming and helpful.
Basic Syntax and Data Types
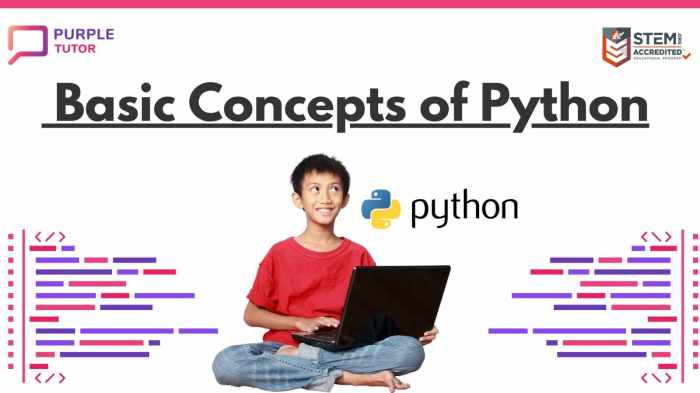
Source: purpletutor.com
Python’s beauty lies in its readability and straightforward syntax. Unlike some languages burdened by complex punctuation, Python uses indentation to define code blocks, making your code cleaner and easier to understand. This, combined with its diverse data types, forms the bedrock of any Python program. Let’s dive in!
Indentation
Python uses indentation (whitespace at the beginning of a line) to define code blocks. This is crucial; unlike languages that use curly braces “, Python relies entirely on consistent indentation to group statements together. Incorrect indentation leads to `IndentationError` – a common mistake for beginners. Generally, four spaces are the recommended and widely accepted standard for indentation. Maintaining consistent indentation throughout your code is paramount for readability and error-free execution.
Common Data Types
Python offers several built-in data types to represent different kinds of information. Understanding these types is fundamental to writing effective Python code.
Data Type | Description | Example |
---|---|---|
Integer (int) | Whole numbers (positive, negative, or zero) without decimal points. | x = 10 |
Float (float) | Numbers with decimal points. | y = 3.14159 |
String (str) | Sequences of characters enclosed in single (‘ ‘) or double (” “) quotes. | name = "Python" |
Boolean (bool) | Represents truth values; either True or False . |
is_active = True |
Variable Assignment and Type Conversion
Variables in Python are used to store data. You assign a value to a variable using the `=` operator. Python is dynamically typed, meaning you don’t need to explicitly declare the data type of a variable; Python infers it automatically. Type conversion (also called type casting) allows you to change a variable from one data type to another.
For example:
age = 30 # Integer
price = 99.99 # Float
message = "Hello, world!" # String
is_valid = False # Boolean
# Type Conversion
age_str = str(age) # Convert integer to string
price_int = int(price) # Convert float to integer (decimal part is truncated)
Basic Arithmetic Operators
Python supports standard arithmetic operations:
Operator | Description | Example |
---|---|---|
+ |
Addition | 5 + 3 # Result: 8 |
- |
Subtraction | 10 - 4 # Result: 6 |
* |
Multiplication | 6 * 7 # Result: 42 |
/ |
Division | 15 / 2 # Result: 7.5 |
// |
Floor Division (integer division) | 15 // 2 # Result: 7 |
% |
Modulo (remainder) | 15 % 2 # Result: 1 |
|
Exponentiation | 2 3 # Result: 8 |
Remember the order of operations (PEMDAS/BODMAS) applies in Python as well: Parentheses, Exponents, Multiplication and Division (from left to right), Addition and Subtraction (from left to right).
Control Flow Statements
So you’ve grasped the basics of Python – variables, data types, the whole shebang. Now it’s time to level up your coding game with control flow statements. These are the building blocks that allow your programs to make decisions and repeat actions, transforming simple scripts into dynamic, powerful tools. Think of them as the director’s chair in your Python movie – they dictate the flow of the action.
Control flow statements determine the order in which your code executes. Without them, your program would simply run line by line, from top to bottom, lacking the flexibility to respond to different situations or repeat tasks. Mastering these is key to writing efficient and sophisticated Python code.
Conditional Statements (if, elif, else)
Conditional statements let your program make decisions based on whether a condition is true or false. The workhorses here are `if`, `elif` (short for “else if”), and `else`. The `if` statement checks a condition; if it’s true, the code block following it executes. `elif` allows you to check additional conditions if the preceding `if` condition is false. Finally, `else` provides a default action if none of the preceding conditions are met.
Let’s say you want to check if a number is positive, negative, or zero:
number = 10
if number > 0:
print("Positive number")
elif number < 0:
print("Negative number")
else:
print("Zero")
This code first checks if `number` is greater than 0. If true, it prints "Positive number". Otherwise, it moves to the `elif` statement, checking if `number` is less than 0. If true, it prints "Negative number". If neither of the first two conditions is true, the `else` block executes, printing "Zero".
Looping Constructs (for, while)
Loops are your go-to tools for repeating a block of code multiple times. Python offers two main types: `for` and `while` loops.
The `for` loop iterates over a sequence (like a list or string) or other iterable object. It's perfect when you know how many times you need to repeat a task.
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop iterates through the `fruits` list, printing each fruit name. Each iteration assigns the next item from the list to the variable `fruit`.
The `while` loop repeats a block of code as long as a specified condition is true. It's ideal when you don't know in advance how many iterations you'll need – the loop continues until the condition becomes false.
count = 0
while count < 5:
print(count)
count += 1
This loop prints numbers from 0 to 4. The loop continues as long as `count` is less than 5. The `count += 1` line is crucial; it increments `count` in each iteration, eventually making the condition false and ending the loop. Without it, you'd have an infinite loop!
So you're diving into the world of Python, learning loops and functions? That's awesome! Just like building a solid Python foundation, securing your home needs careful planning. Check out this article on Why You Should Consider Adding Home Insurance Riders for Extra Protection to ensure you've got all your bases covered, then get back to conquering those Python challenges!
Nested Loops and Conditional Statements
You can combine loops and conditional statements to create more complex logic. Nested loops involve placing one loop inside another. This is useful when you need to iterate over multiple sequences or repeat a task multiple times within each iteration of an outer loop.
Imagine you want to print a multiplication table:
for i in range(1, 11):
for j in range(1, 11):
print(i * j, end="\t") # \t adds a tab for better formatting
print() # New line after each row
This code uses nested `for` loops to iterate through numbers from 1 to 10. The inner loop calculates and prints the product of `i` and `j`, while the outer loop handles the rows of the table.
Program Using Loops and Conditional Statements
Let's build a simple program that checks if a number is even or odd and prints the result for a range of numbers.
for num in range(1, 11):
if num % 2 == 0:
print(f"num is even")
else:
print(f"num is odd")
This program iterates through numbers 1 to 10. The `if` statement checks if the number is even (divisible by 2) using the modulo operator (`%`). If it's even, it prints a message indicating so; otherwise, it prints that the number is odd.
Working with Data Structures
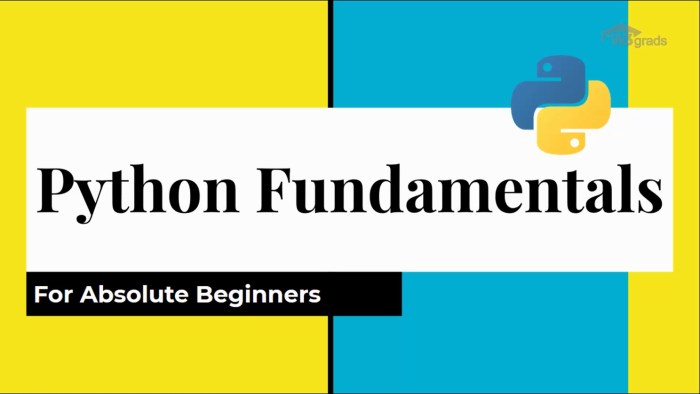
Source: amazonaws.com
Python offers several built-in data structures that are fundamental for organizing and manipulating data. Understanding these structures is crucial for writing efficient and readable code. We'll explore lists, tuples, and dictionaries, highlighting their key differences and practical applications.
Lists, Tuples, and Dictionaries: A Comparison, Understanding the Basics of Python for Beginners
Let's delve into the core characteristics of these versatile data structures. The table below provides a concise overview.
Name | Description | Mutability | Example |
---|---|---|---|
List | An ordered, mutable sequence of items. Allows duplicate elements. | Mutable | my_list = [1, "hello", 3.14, True] |
Tuple | An ordered, immutable sequence of items. Allows duplicate elements. | Immutable | my_tuple = (1, "hello", 3.14, True) |
Dictionary | An unordered collection of key-value pairs. Keys must be unique and immutable. | Mutable | my_dict = "name": "Alice", "age": 30, "city": "New York" |
List Methods
Lists provide a rich set of methods for manipulating their contents. These methods allow for efficient addition, removal, and modification of elements.
Here are some commonly used list methods:
append(item)
: Adds an item to the end of the list.insert(index, item)
: Inserts an item at a specific index.remove(item)
: Removes the first occurrence of an item.pop(index)
: Removes and returns the item at a specific index (defaults to the last item).extend(iterable)
: Adds all items from an iterable to the end of the list.sort()
: Sorts the list in ascending order (in-place).reverse()
: Reverses the order of elements in the list (in-place).
Tuple Methods
Tuples, being immutable, have fewer methods compared to lists. Their immutability ensures data integrity.
While tuples don't offer extensive modification methods, they do provide a few useful ones:
count(item)
: Returns the number of times an item appears in the tuple.index(item)
: Returns the index of the first occurrence of an item.
Dictionary Methods
Dictionaries offer a variety of methods for accessing, adding, and removing key-value pairs.
Effective manipulation of dictionaries relies on these methods:
get(key, default)
: Returns the value associated with a key; returns a default value if the key is not found.items()
: Returns a view object containing key-value pairs as tuples.keys()
: Returns a view object containing all keys.values()
: Returns a view object containing all values.pop(key, default)
: Removes and returns the value associated with a key; returns a default value if the key is not found.update(other_dict)
: Updates the dictionary with key-value pairs from another dictionary.
Use Cases and Iterations
Lists are ideal for storing sequences of items that may need to be modified. Tuples are best suited for representing fixed collections of data where immutability is desired, like coordinates or database records. Dictionaries excel at representing structured data with key-value relationships, such as user profiles or configuration settings.
Iterating through each data structure is straightforward:
Lists:
for item in my_list:
print(item)
Tuples:
for item in my_tuple:
print(item)
Dictionaries:
for key, value in my_dict.items():
print(f"key: value")
Functions and Modules
Python functions are reusable blocks of code that perform specific tasks. They're like mini-programs within your larger program, making your code more organized, readable, and efficient. Using functions avoids repetitive coding and makes debugging easier. Modules, on the other hand, are files containing Python code (functions, classes, variables) that you can import into your programs to extend their functionality. Think of them as pre-built toolkits.
Functions help you break down complex problems into smaller, manageable pieces. This modular approach is a cornerstone of good programming practice, improving code maintainability and collaboration. Modules, in turn, allow you to leverage the work of others and avoid reinventing the wheel. They provide access to a vast library of pre-written code for various tasks, from mathematical calculations to web development.
Defining and Calling Functions
Defining a function involves using the `def` , followed by the function name, parentheses `()`, and a colon `:`. The code block within the function is indented. Parameters are passed within the parentheses, and a `return` statement specifies the output value.
Here’s a simple example of a function that adds two numbers:
```python
def add_numbers(x, y):
"""This function adds two numbers and returns the sum."""
sum = x + y
return sum
result = add_numbers(5, 3)
print(result) # Output: 8
```
This function, `add_numbers`, takes two arguments (`x` and `y`), adds them, and returns the sum. The `"""Docstring"""` explains what the function does. Calling the function involves using its name followed by parentheses containing the arguments.
Importing and Using Modules
Modules are imported using the `import` statement. For example, to use the `math` module (which contains mathematical functions), you would write `import math`. You can then access functions within the module using the dot notation (e.g., `math.sqrt(9)`).
You can also import specific functions from a module using `from math import sqrt`. This allows you to call the function directly without the module name prefix (e.g., `sqrt(9)`).
Let's explore another approach: using `as` to give a module a shorter alias. For instance, `import math as m` allows you to write `m.sqrt(9)` instead of `math.sqrt(9)`. This improves code readability, especially when dealing with lengthy module names.
Program Utilizing Functions and an External Module
This program calculates the area of a circle using a function and the `math` module:
```python
import math
def calculate_circle_area(radius):
"""Calculates the area of a circle given its radius."""
area = math.pi * radius2
return area
radius = float(input("Enter the radius of the circle: "))
area = calculate_circle_area(radius)
print(f"The area of the circle is: area")
```
This program defines a function `calculate_circle_area` that uses `math.pi` from the `math` module to compute the area. The program prompts the user for the radius, calls the function, and displays the result. The `f-string` formatting provides a clean way to incorporate the calculated area into the output message.
Input and Output Operations
Python offers robust tools for interacting with users and files, making it a versatile language for various applications. Understanding input and output operations is crucial for building interactive programs and handling data efficiently. This section will cover the fundamental techniques for obtaining user input, displaying output, and managing file operations.
Getting User Input with input()
The `input()` function is the primary method for obtaining user input in Python. It reads a line of text from the console, converts it to a string, and returns the string value. For example, the code `name = input("Enter your name: ")` prompts the user to enter their name and stores the input in the `name` variable. Any data entered will be treated as a string, even if it represents a number. To use it as a number, you'll need to explicitly convert it using functions like `int()` or `float()`. Consider the following example where the user provides their age:
```python
age_str = input("Enter your age: ")
age_int = int(age_str) #Convert to integer
print(f"You will be age_int + 1 next year.")
```
This code takes the user's age input, converts it to an integer, and then uses it in a calculation. Error handling (e.g., using `try-except` blocks) is recommended to manage potential issues if the user inputs non-numeric data.
Displaying Output to the Console with print()
The `print()` function is used to display output to the console. It's a fundamental tool for providing feedback to the user and displaying the results of program operations. The `print()` function can handle various data types, automatically converting them to string representations before output. For enhanced formatting, f-strings (formatted string literals) offer a concise way to embed variables directly within strings. For example:
```python
name = "Alice"
age = 30
print(f"My name is name and I am age years old.")
```
This will print: "My name is Alice and I am 30 years old." You can also use `print()` to display multiple values separated by spaces or customize the separator using the `sep` argument. For instance, `print("apple", "banana", "cherry", sep=", ")` will print "apple, banana, cherry".
File Handling: Reading and Writing
Python provides built-in functions for working with files. The `open()` function is used to open a file, specifying the file path and the mode ('r' for reading, 'w' for writing, 'a' for appending, etc.). After opening, you can use methods like `read()`, `readline()`, `readlines()`, and `write()` to interact with the file's contents. Remember to close the file using `close()` when you're finished to release system resources. Using a `with` statement is recommended as it automatically handles file closing, even if errors occur:
```python
with open("my_file.txt", "w") as f:
f.write("This is some text.\n")
f.write("This is another line.\n")
with open("my_file.txt", "r") as f:
contents = f.read()
print(contents)
```
This code first writes two lines to "my_file.txt" and then reads and prints the entire content. Error handling (e.g., checking if the file exists before attempting to open it) is crucial for robust file operations.
Processing Data from a File
This example demonstrates reading data from one file, processing it, and writing the results to another. Let's assume we have a file ("numbers.txt") containing a list of numbers, one per line. We'll read these numbers, square each one, and write the squared numbers to a new file ("squared_numbers.txt"):
```python
try:
with open("numbers.txt", "r") as infile, open("squared_numbers.txt", "w") as outfile:
for line in infile:
try:
number = int(line.strip()) # remove whitespace and convert to int
squared = number 2
outfile.write(str(squared) + "\n")
except ValueError:
print(f"Skipping invalid line: line.strip()")
except FileNotFoundError:
print("numbers.txt not found.")
```
This code demonstrates error handling for both file opening and invalid data within the file. It's a robust approach to file processing.
Error Handling and Debugging
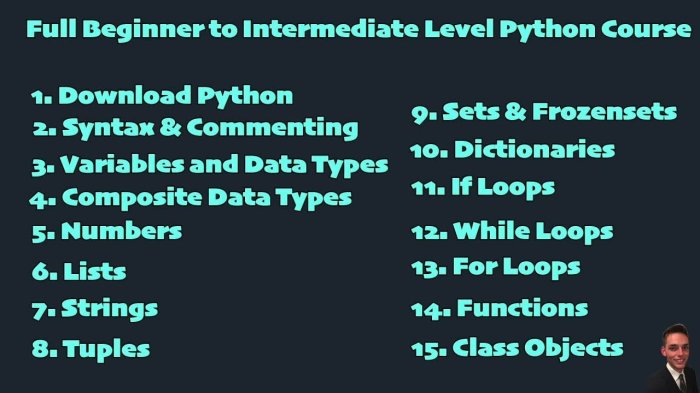
Source: cloudfront.net
Writing clean, efficient Python code is only half the battle. The other half involves anticipating and gracefully handling errors, a crucial skill for any programmer. Debugging, the process of identifying and fixing errors, is an iterative journey that requires patience and a systematic approach. This section will equip you with the tools and knowledge to navigate the sometimes-treacherous waters of Python errors.
Common Python Errors
Python, like any programming language, throws errors when something goes wrong. Understanding these errors is the first step towards effectively debugging your code. Let's examine some frequently encountered error types. A `SyntaxError` occurs when the Python interpreter encounters code that violates the language's grammatical rules. This could be due to typos, missing colons, or incorrect indentation. A `TypeError` arises when an operation is performed on an object of an inappropriate type, such as trying to add a string to an integer. Finally, a `NameError` signals that a variable or function has been used before it has been defined or is misspelled. Recognizing these error messages is key to understanding where your code went astray.
Debugging Techniques
When faced with an error, your first instinct might be to frantically search for the problem. However, a methodical approach is more effective. The simplest debugging technique is the liberal use of `print()` statements. Strategically placed `print()` calls can reveal the values of variables at different points in your code, helping you track down the source of the error. More advanced debugging involves using a Python debugger, such as `pdb` (Python Debugger). `pdb` allows you to step through your code line by line, inspect variables, and set breakpoints. This provides a granular level of control, allowing you to pinpoint the exact location and cause of the error.
Try-Except Blocks for Exception Handling
While debugging helps you find and fix errors, exception handling provides a way to gracefully manage errors that might occur during runtime. `try-except` blocks allow you to anticipate potential errors and execute alternative code if an exception is raised. The `try` block contains the code that might cause an error. If an error occurs, the code within the corresponding `except` block is executed. This prevents your program from crashing and allows you to handle the error in a controlled manner. For example, you can display a user-friendly message or log the error for later analysis.
Example Program with Error Handling
Let's illustrate the power of `try-except` blocks with a simple program that divides two numbers. We'll anticipate the `ZeroDivisionError` that might occur if the denominator is zero.
```python
def divide(x, y):
try:
result = x / y
print("Result:", result)
except ZeroDivisionError:
print("Error: Cannot divide by zero.")
divide(10, 2) # Output: Result: 5.0
divide(10, 0) # Output: Error: Cannot divide by zero.
```
This example shows how a `try-except` block prevents the program from crashing when dividing by zero. Instead, it prints an informative error message, providing a more robust and user-friendly experience. The `try` block attempts the division; if it fails due to a `ZeroDivisionError`, the `except` block's code executes instead.
Illustrative Example: A Simple Number Guessing Game: Understanding The Basics Of Python For Beginners
Let's solidify our Python knowledge by building a simple number guessing game. This program will demonstrate the practical application of concepts like variables, loops, conditional statements, and input/output operations, all covered in the previous sections. It's a fun way to see how these elements work together to create a functional application.
This number guessing game challenges the user to guess a randomly generated number within a specified range. The program provides feedback after each guess, indicating whether the guess is too high or too low. The game continues until the user correctly guesses the number. The program also tracks the number of attempts taken.
Game Functionality and Code
The game begins by importing the `random` module to generate a random number. The program then prompts the user to enter the upper limit of the guessing range. A random number is generated within this range. The core of the game lies within a `while` loop that continues until the user guesses correctly. Inside the loop, the user's guess is compared to the random number, and appropriate feedback is provided. The number of attempts is incremented with each guess. Finally, once the number is guessed, a congratulatory message is displayed along with the number of attempts.
```python
import randomdef number_guessing_game():
"""Plays a number guessing game with the user."""
print("Welcome to the Number Guessing Game!")
try:
upper_limit = int(input("Enter the upper limit for the guessing range: "))
if upper_limit <= 0: print("Please enter a positive integer.") return except ValueError: print("Invalid input. Please enter an integer.") return secret_number = random.randint(1, upper_limit) guesses_taken = 0 guess = 0 while guess != secret_number: try: guess = int(input(f"Take a guess (1-upper_limit): ")) guesses_taken += 1 if guess < secret_number: print("Too low!") elif guess > secret_number:
print("Too high!")
except ValueError:
print("Invalid input. Please enter an integer.")
continueprint(f"Congratulations! You guessed the number in guesses_taken tries.")
if __name__ == "__main__":
number_guessing_game()```
Illustrative Input and Output
Here are a couple of examples illustrating the program's input and output:
Example 1:
Input:
```
Enter the upper limit for the guessing range: 100
Take a guess (1-100): 50
Too low!
Take a guess (1-100): 75
Too high!
Take a guess (1-100): 62
Too low!
Take a guess (1-100): 68
Too high!
Take a guess (1-100): 65
Congratulations! You guessed the number in 5 tries.
```
Example 2:
Input:
```
Enter the upper limit for the guessing range: 20
Take a guess (1-20): 10
Too high!
Take a guess (1-20): 5
Too low!
Take a guess (1-20): 7
Congratulations! You guessed the number in 3 tries.
```
Design Choices
The program utilizes functions to improve code organization and readability. Error handling (using `try-except` blocks) is incorporated to gracefully handle invalid user input, preventing crashes. The use of a `while` loop ensures the game continues until the correct guess is made. Clear and concise prompts guide the user through the game. The inclusion of the `if __name__ == "__main__":` block ensures that the `number_guessing_game()` function is only called when the script is run directly, not when imported as a module. This is a standard Python best practice.
Ending Remarks
So, you've conquered the basics of Python. You've wrestled with loops, tamed data structures, and even outsmarted a few errors. That's a huge accomplishment! Remember, learning to code is a journey, not a sprint. Don't be afraid to experiment, break things (and then fix them!), and most importantly, have fun. The world of Python is vast and exciting – this is just the beginning of your coding adventure. Now go build something amazing!