A Deep Dive into Object-Oriented Design Patterns: Forget boring textbooks – let’s crack the code on building elegant, scalable software. This isn’t your grandpappy’s programming; we’re diving headfirst into the world of design patterns, those reusable solutions to common software design problems. We’ll unravel the mysteries of creational, structural, and behavioral patterns, showing you how to choose the right tool for the job and avoid those pesky anti-patterns that can turn your clean code into a tangled mess. Get ready to level up your coding game!
From the fundamental principles of OOD – encapsulation, inheritance, polymorphism, and abstraction – to the intricacies of patterns like Singleton, Factory, and Observer, we’ll explore practical examples and real-world applications. We’ll compare and contrast different patterns, highlighting their strengths and weaknesses, and equip you with the knowledge to make informed decisions when designing your next project. Think of this as your ultimate guide to mastering the art of object-oriented design.
Introduction to Object-Oriented Design (OOD)
Object-Oriented Design (OOD) is a powerful programming paradigm that organizes software design around data, or objects, rather than functions and logic. This approach allows for creating more modular, flexible, and maintainable code, especially for complex applications. Understanding its core principles is crucial for any aspiring software developer.
Fundamental Principles of OOD
OOD rests on four fundamental pillars: encapsulation, inheritance, polymorphism, and abstraction. These principles work together to create robust and reusable software components. Encapsulation bundles data and the methods that operate on that data within a single unit, protecting the internal state from outside interference. Inheritance allows creating new classes (objects) based on existing ones, inheriting their properties and behaviors, promoting code reuse and reducing redundancy. Polymorphism enables objects of different classes to respond to the same method call in their own specific way, enhancing flexibility and extensibility. Finally, abstraction focuses on essential characteristics while hiding unnecessary details, simplifying complex systems and improving code readability.
Comparison of Procedural and Object-Oriented Programming
Procedural programming focuses on procedures or functions that operate on data. It’s simpler for smaller programs but can become unwieldy as complexity increases. Object-oriented programming, conversely, structures code around objects that encapsulate both data and methods. This modularity makes OOD better suited for large, complex projects, facilitating easier maintenance, scalability, and collaboration among developers. Consider a simple task like managing a library: a procedural approach might involve separate functions for adding books, searching for books, and checking out books, operating on a global data structure. An OOD approach would define a “Book” object with its own methods for adding details, searching, and managing checkout status, making the code more organized and easier to understand.
Real-World Applications of OOD
OOD finds extensive application in diverse fields. Consider the development of video games, where characters, items, and environments are naturally represented as objects with their own properties and behaviors. In banking systems, accounts, transactions, and customers are all modeled as objects, ensuring data integrity and security. Similarly, in e-commerce platforms, products, orders, and user accounts are represented as objects, managing complex interactions efficiently. Even operating systems leverage OOD principles extensively, with processes, files, and hardware devices often modeled as objects.
A Simple Class Diagram Illustrating OOD Concepts
Imagine a simple “Car” class. This class would encapsulate data like model, color, and speed, along with methods like accelerate(), brake(), and turn(). We could then create a “SportsCar” class that inherits from the “Car” class, adding properties like horsepower and topSpeed, while inheriting the basic car functionality. Polymorphism could be implemented by having both “Car” and “SportsCar” classes respond to an “accelerate()” method, but with different implementations based on their specific characteristics. This illustrates encapsulation (data and methods bundled together), inheritance (SportsCar inheriting from Car), and polymorphism (different acceleration behavior). The abstraction lies in the fact that we don’t need to know the intricate details of the engine to use the car; we simply interact with the higher-level methods. A class diagram would visually represent this hierarchy, showing the “Car” class as a parent class with the “SportsCar” class extending from it. The diagram would also show the attributes (data) and methods associated with each class.
Creational Design Patterns
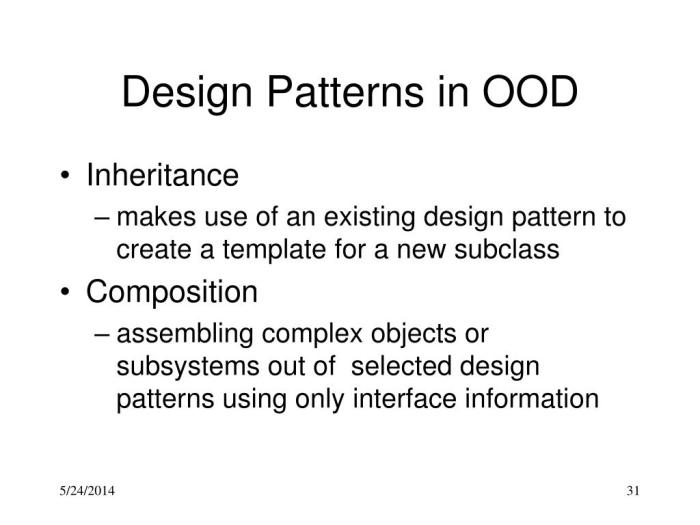
Source: slideserve.com
Creational design patterns are like the blueprints of object creation. They provide elegant solutions for instantiating objects in a flexible and controlled manner, making your code more maintainable, reusable, and less prone to errors. They’re all about managing object creation mechanisms, shielding the client code from the complexities of how objects are created and put together. Think of them as sophisticated object factories, each with its own specialty.
These patterns enhance code flexibility by decoupling object creation from their usage. This means you can change the way objects are created without altering the code that uses them. This is particularly useful when dealing with complex object hierarchies or when the creation process itself involves multiple steps or dependencies.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is useful for managing resources that should only exist once, such as a database connection or a logging service. Overuse can lead to tight coupling, however.
Here’s a Java example:
public class Singleton
private static final Singleton INSTANCE = new Singleton();
private Singleton()
public static Singleton getInstance()
return INSTANCE;
public void doSomething()
System.out.println("Singleton doing something!");
Factory Pattern
The Factory pattern defines an interface for creating objects but lets subclasses decide which class to instantiate. This promotes loose coupling and allows for easy addition of new object types without modifying existing code.
A Python example:
class Dog:
def speak(self):
return "Woof!"
class Cat:
def speak(self):
return "Meow!"
class AnimalFactory:
def create_animal(self, animal_type):
if animal_type == "dog":
return Dog()
elif animal_type == "cat":
return Cat()
else:
return None
Abstract Factory Pattern
The Abstract Factory pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes. This is useful when you need to create multiple related objects and want to keep the creation process consistent.
Consider a UI toolkit where you might need to create buttons, text fields, and other components. An abstract factory could handle the creation of these components for different platforms (e.g., Windows, macOS).
Builder Pattern
The Builder pattern separates the construction of a complex object from its representation, allowing the same construction process to create various representations. This is useful for objects with many optional parameters.
A Java example illustrating a house builder:
public class HouseBuilder
private String foundation;
private String walls;
// ... other house components
public HouseBuilder setFoundation(String foundation)
this.foundation = foundation;
return this;
// ... other setters
public House build()
return new House(foundation, walls, ...);
Prototype Pattern
The Prototype pattern specifies the kinds of objects to create using a prototypical instance, and create new objects by copying this prototype. This is effective when the cost of creating an object is high, or when you need to create many similar objects.
Imagine cloning a complex game character object instead of recreating it from scratch every time.
Singleton vs. Factory Pattern Comparison
The Singleton pattern guarantees only one instance, while the Factory pattern focuses on creating objects of various types. Singletons can lead to tight coupling and testing difficulties, whereas Factories promote flexibility and loose coupling. The choice depends on the specific needs of the application.
Pattern | Purpose | Use Cases | Complexity |
---|---|---|---|
Singleton | Ensure a class has only one instance | Database connection, logging service | Low |
Factory | Create objects without specifying the exact class | Creating objects with varying implementations | Medium |
Abstract Factory | Create families of related objects | UI toolkits, document creation | High |
Builder | Construct complex objects step-by-step | Building complex objects with many attributes | Medium |
Prototype | Create objects by copying a prototype | Cloning complex objects, object pooling | Medium |
Structural Design Patterns
Structural design patterns are all about composing classes and objects to form larger structures. They focus on how classes and objects are organized and interact to create more complex systems, often addressing issues of flexibility, maintainability, and code reusability. Think of them as the architects of your object-oriented world, shaping the overall structure and relationships between different components.
Unlike creational patterns that deal with object creation, structural patterns are concerned with how these created objects are assembled and organized. This involves establishing relationships between objects, providing a clean and efficient way to manage complexity, and ensuring that changes in one part of the system don’t unnecessarily ripple through the rest.
Understanding object-oriented design patterns is crucial for building robust and scalable systems. But even the most meticulously crafted software architecture can’t completely eliminate risk; that’s where the practical side comes in. Consider reading this article on why cyber insurance is becoming essential for modern businesses: Why Cyber Insurance is Becoming Essential for Modern Businesses , because protecting your digital assets is just as vital as the design itself.
Ultimately, solid design and smart risk management go hand-in-hand.
Adapter Pattern
The Adapter pattern is your go-to solution when you need to make two incompatible interfaces work together. Imagine you have a square peg and a round hole – the adapter acts as a translator, allowing the square peg to fit into the round hole without modifying either the peg or the hole. This is particularly useful when integrating legacy systems or third-party libraries with your existing codebase.
Consider a scenario where you have an old library that uses a specific interface, and a new component that uses a different one. Instead of rewriting either, you create an adapter that converts the calls from the new component to the format understood by the old library, acting as a bridge between the two.
// Old Library Interface
interface OldLibrary
void oldMethod();
// New Component Interface
interface NewComponent
void newMethod();
// Adapter Class
class Adapter implements OldLibrary
private NewComponent newComponent;
public Adapter(NewComponent newComponent)
this.newComponent = newComponent;
@Override
public void oldMethod()
newComponent.newMethod(); // Adapts the new method to the old interface
// Client Code
public class Client
public static void main(String[] args)
NewComponent newComp = new NewComponentImpl();
OldLibrary adapter = new Adapter(newComp);
adapter.oldMethod(); // Uses the adapter to call the new component through the old interface
// Implementation of NewComponent
class NewComponentImpl implements NewComponent
@Override
public void newMethod()
System.out.println("New method called!");
Bridge Pattern
The Bridge pattern decouples an abstraction from its implementation, allowing both to vary independently. This is incredibly useful when you have a complex system with many possible implementations for a single abstraction. It prevents a tight coupling between the abstraction and its implementation, promoting flexibility and maintainability.
Imagine designing a drawing application. You might have different types of shapes (abstraction) and different rendering engines (implementation). The Bridge pattern allows you to switch rendering engines without affecting the shape classes, and vice-versa, making your application highly adaptable.
Composite Pattern
The Composite pattern lets you compose objects into tree structures to represent part-whole hierarchies. This allows you to treat individual objects and compositions of objects uniformly. Think of it as building a tree structure where leaves are individual objects and branches are groups of objects.
A classic example is a file system. Files are individual objects, while directories are composite objects containing other files and directories. You can treat both files and directories using the same interface (e.g., `getSize()`), regardless of whether it’s a single file or a complex directory structure.
Decorator Pattern
The Decorator pattern dynamically adds responsibilities to an object without altering its structure. This is achieved by wrapping the object with another object that provides the additional functionality. It’s a flexible alternative to subclassing when you need to add features on the fly.
Consider adding features to a coffee order. You can start with a basic coffee and then add milk, sugar, whipped cream, etc., each adding a decorator that wraps the previous one. This approach avoids creating a huge number of subclasses for every possible combination of add-ons.
Facade Pattern
The Facade pattern provides a simplified interface to a complex subsystem. It hides the complexity behind a single, easy-to-use interface. This is useful for making complex systems easier to use and understand, especially for clients who don’t need to know the intricate details of the underlying implementation.
Think of a stereo system. Instead of interacting with individual components (amplifier, tuner, CD player), you use a single remote control (the facade) to control the entire system.
Flyweight Pattern
The Flyweight pattern uses sharing to support large numbers of fine-grained objects efficiently. It minimizes memory usage by sharing common parts of objects. This is particularly useful when dealing with a huge number of similar objects, reducing the overall memory footprint.
A common example is a text editor. Instead of creating a separate object for each character, the Flyweight pattern shares character objects, reducing memory consumption significantly, especially when dealing with large documents.
Proxy Pattern
The Proxy pattern provides a surrogate or placeholder for another object to control access to it. This is useful for controlling access, adding additional functionality (e.g., logging, caching), or delaying object creation until it’s actually needed.
Imagine loading a large image. Instead of loading the entire image immediately, a proxy can load a smaller thumbnail first, and then load the full-size image only when needed. This improves performance by delaying the loading of resource-intensive objects.
Behavioral Design Patterns
Behavioral design patterns address communication between objects and how they manage their responsibilities. They’re crucial for building flexible, maintainable, and scalable applications by defining clear communication channels and responsibilities, leading to cleaner code and easier debugging. They essentially dictate *how* objects interact, unlike creational patterns (which focus on *how* objects are created) or structural patterns (which focus on *how* objects are composed).
Chain of Responsibility
The Chain of Responsibility pattern lets multiple objects have a chance to handle a request. This avoids coupling the sender of the request to its specific receiver. The chain of handlers is created, and each handler can either process the request or pass it to the next handler in the chain. This is incredibly useful for scenarios where you don’t know in advance which object should handle a specific request.
“`java
// Example in Java
interface Handler
void handleRequest(Request request);
class ConcreteHandler1 implements Handler
@Override
public void handleRequest(Request request)
if (request.getAmount() < 10)
System.out.println("ConcreteHandler1 handled the request.");
else
System.out.println("ConcreteHandler1 passed the request.");
// ... other ConcreteHandlers ...
class Request
private int amount;
public Request(int amount)
this.amount = amount;
public int getAmount()
return amount;
// Client code
public class Client
public static void main(String[] args)
Handler h1 = new ConcreteHandler1();
Handler h2 = new ConcreteHandler2(); // Assuming ConcreteHandler2 exists
h1.setNext(h2); // Setting up the chain
h1.handleRequest(new Request(5)); // h1 handles
h1.handleRequest(new Request(15)); // h2 handles
```
A flowchart depicting the Chain of Responsibility would show a linear sequence of handlers. Each handler receives the request; if it can process it, it does so and the chain stops. If not, it passes the request to the next handler in the line. The final handler in the chain might represent a default or error handling mechanism.
Interpreter
The Interpreter pattern defines a representation for a grammar along with an interpreter that uses the representation to interpret sentences in the grammar. This is suitable for situations involving parsing and interpreting languages, commands, or expressions. Consider a simple calculator; the interpreter would take an expression like “2 + 3 * 4” and break it down into its constituent parts to calculate the result.
Iterator
The Iterator pattern provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. This pattern decouples the traversal logic from the collection itself, allowing different traversal methods to be used with the same collection. For example, you can iterate through a list using a forward iterator, a reverse iterator, or even a filtered iterator without changing the list’s structure.
Mediator
The Mediator pattern defines an object that encapsulates how a set of objects interact. This promotes loose coupling by keeping objects from referring to each other explicitly, and it makes it easier to vary their interaction independently. It’s like a central communication hub; all objects communicate through the mediator.
Memento
The Memento pattern provides a way to restore an object to its previous state without violating encapsulation. This is achieved by storing the object’s internal state in a Memento object, which is then used to revert the object to its earlier condition. This is valuable in scenarios requiring undo/redo functionality or version control.
Observer
The Observer pattern defines a one-to-many dependency between objects where a state change in one object (the subject) automatically notifies and updates its dependents (the observers). This is widely used in event-driven systems and GUI frameworks.
State
The State pattern allows an object to alter its behavior when its internal state changes. The object appears to change its class. This is useful for managing objects with complex state transitions.
Strategy
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This lets the algorithm vary independently from clients that use it. Think of different sorting algorithms (bubble sort, merge sort, quicksort) – each is a strategy, and you can choose the best one for your needs.
Template Method
The Template Method pattern defines the skeleton of an algorithm in a base class, allowing subclasses to override specific steps without changing the overall algorithm’s structure. This is excellent for enforcing a specific workflow while allowing flexibility in individual steps.
Visitor
The Visitor pattern represents an operation to be performed on the elements of an object structure. It lets you define a new operation without changing the classes of the elements on which it operates. This is useful for adding new functionality to existing class hierarchies without modifying the classes themselves.
Observer vs. Mediator: A Comparison
The Observer and Mediator patterns both manage communication between objects, but they differ significantly in their approach. The Observer pattern is a one-to-many relationship where a subject notifies multiple observers of changes in its state. The Mediator pattern, however, is a many-to-many relationship where objects communicate through a central mediator. In Observer, the communication is unidirectional (subject to observers), whereas in Mediator, it’s multidirectional (objects communicate with each other through the mediator). Choosing between them depends on the specific communication needs of the application. If you need a simple, one-to-many notification mechanism, the Observer pattern is sufficient. If you need more complex, multidirectional communication, the Mediator pattern is more appropriate.
Choosing the Right Design Pattern
Picking the perfect design pattern isn’t about finding the “best” one; it’s about finding the *right* one for your specific project needs. Just like choosing the right tool for a job, selecting the wrong design pattern can lead to messy, inflexible, and hard-to-maintain code. This section will equip you with the knowledge to navigate this crucial decision.
Choosing a design pattern involves understanding your project’s context, identifying recurring problems, and evaluating the trade-offs of each pattern. It’s a balancing act between flexibility, performance, and complexity.
Factors Influencing Pattern Selection
Selecting the appropriate design pattern hinges on several key factors. These factors, when carefully considered, guide developers toward the most suitable solution for their specific project requirements. Ignoring these factors can lead to inefficient and cumbersome code. Let’s delve into these crucial aspects.
- Problem Domain: The nature of the problem you’re solving significantly influences pattern choice. For instance, if you need to create objects in a flexible way, a creational pattern like Factory or Abstract Factory might be ideal. If you need to manage interactions between objects, a behavioral pattern like Observer or Strategy would be more appropriate.
- Scalability and Maintainability: Consider how your system might grow and evolve. Patterns like Singleton, while seemingly simple for managing single instances, can become a bottleneck in larger, more complex applications. Patterns promoting loose coupling, like Facade or Mediator, generally lead to better scalability and easier maintenance.
- Performance Requirements: Some patterns have inherent performance implications. For example, using Decorator patterns can add overhead due to the layered structure. If performance is critical, carefully evaluate the impact of your chosen pattern.
- Team Expertise: The familiarity of your team with different patterns is a critical factor. Choosing a pattern that your team understands well will lead to faster development and easier debugging. Conversely, choosing a complex pattern that the team isn’t proficient in might lead to increased development time and potential errors.
Scenario-Based Pattern Selection
Let’s examine some scenarios where different patterns excel:
- Scenario: You need to create objects of different classes based on a configuration file. Suitable Pattern: Factory Method or Abstract Factory. These patterns abstract the object creation process, allowing for easy extension and modification without altering existing code.
- Scenario: You need to provide a simplified interface to a complex subsystem. Suitable Pattern: Facade. This pattern wraps a complex set of classes into a single, easy-to-use interface.
- Scenario: You need to allow objects to interact without knowing each other’s concrete classes. Suitable Pattern: Observer. This pattern enables loose coupling between objects by allowing one object to notify others of changes in its state.
Trade-offs Associated with Design Patterns
Every design pattern introduces certain trade-offs. Understanding these is vital for informed decision-making.
- Complexity vs. Flexibility: More complex patterns often offer greater flexibility, but at the cost of increased code complexity and potential performance overhead. Simpler patterns are easier to understand and implement, but might limit future flexibility.
- Performance vs. Maintainability: Some patterns, like Decorator, can impact performance due to added layers. Others, like Singleton, can create dependencies that hinder maintainability and testability.
- Coupling vs. Cohesion: Patterns aiming for loose coupling often improve maintainability but might decrease cohesion within a module. The balance needs to be carefully considered.
A Decision Tree for Pattern Selection
A decision tree can help guide you through the process of selecting the appropriate pattern. While a comprehensive tree would be extensive, a simplified version illustrates the basic approach. This would start with identifying the primary problem (creation, structure, behavior), then branching based on secondary factors like scalability needs and existing codebase. For example, if the problem is object creation and scalability is a major concern, the tree might lead to the Factory Method or Abstract Factory patterns. If the problem involves managing interactions between objects and loose coupling is desired, the tree might suggest the Observer or Mediator patterns. A more detailed tree would require further branching based on specific sub-problems and constraints.
Advanced Topics in OOD and Design Patterns
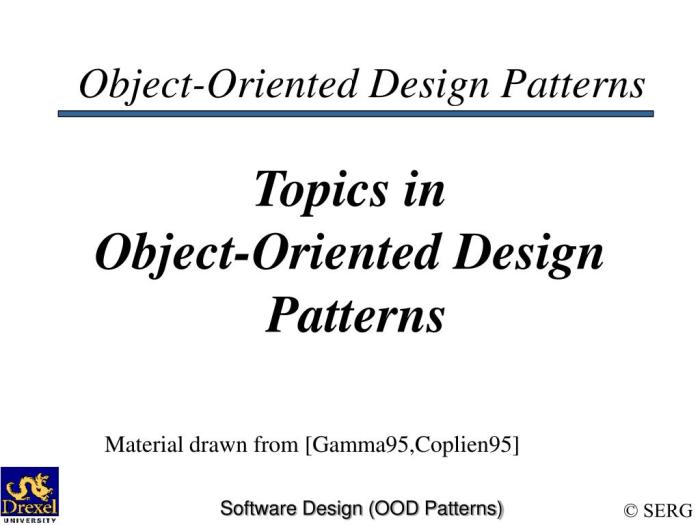
Source: slideserve.com
Stepping beyond the foundational design patterns, we delve into the more nuanced aspects of Object-Oriented Design (OOD), exploring techniques that elevate code quality and project scalability. This section focuses on refining your OOD skills to tackle complex, real-world scenarios.
SOLID Principles and Their Importance in OOD
The SOLID principles—Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion—are not just buzzwords; they’re cornerstones of robust and maintainable object-oriented code. They provide a framework for designing classes and modules that are flexible, adaptable, and easy to understand. Ignoring them often leads to brittle, hard-to-modify codebases. Each principle addresses a specific common problem in software design. For example, the Single Responsibility Principle advocates for each class having only one reason to change, preventing monolithic, unwieldy classes. The Open/Closed Principle encourages designing classes that are open for extension but closed for modification, minimizing the risk of introducing bugs when adding new features. The Liskov Substitution Principle ensures that subtypes can replace their base types without altering the correctness of the program. Interface Segregation Principle promotes creating small, specific interfaces rather than large, general-purpose ones, reducing dependency coupling. Finally, the Dependency Inversion Principle suggests that high-level modules should not depend on low-level modules; both should depend on abstractions. Abstractions should not depend on details; details should depend on abstractions.
Challenges and Best Practices for Implementing Design Patterns in Large-Scale Projects, A Deep Dive into Object-Oriented Design Patterns
Scaling design patterns to large projects introduces complexities. Maintaining consistency across a large codebase requires meticulous planning and a shared understanding of the chosen patterns. Communication and collaboration are paramount. Best practices include thorough documentation, regular code reviews, and the use of consistent naming conventions. Employing a modular design, breaking down the project into smaller, manageable components, helps prevent the system from becoming an unmaintainable monolith. Careful consideration should be given to the trade-offs of each pattern; some patterns might introduce overhead that outweighs their benefits in certain contexts. For instance, using the Decorator pattern excessively can lead to a complex, hard-to-understand class hierarchy.
Improving Code Testability and Maintainability with Design Patterns
Design patterns intrinsically enhance testability and maintainability. Well-structured code, adhering to SOLID principles and employing appropriate patterns, is easier to test because individual components are loosely coupled and have well-defined responsibilities. This allows for isolated unit testing, simplifying the debugging process. Maintainability is improved because changes in one part of the system are less likely to have cascading effects on other parts. For example, the Strategy pattern allows for easy swapping of algorithms without modifying the core logic, simplifying maintenance and updates. The use of factories and abstract factories makes it easier to change the creation process of objects without impacting the rest of the application.
Anti-Patterns and How to Avoid Them
Anti-patterns are common solutions that, while seemingly simple, lead to poor design and maintainability. One common anti-pattern is “God Objects,” where a single class takes on too many responsibilities. This violates the Single Responsibility Principle and makes the class difficult to understand, test, and maintain. Another is “Spaghetti Code,” characterized by convoluted, unstructured code with tangled dependencies. This makes it extremely difficult to understand and modify the code. Avoiding these requires careful planning, adherence to SOLID principles, and a commitment to writing clean, well-documented code. Regular code reviews can help identify potential anti-patterns before they become entrenched in the codebase. Refactoring existing code to eliminate anti-patterns is a continuous process that requires discipline and a willingness to improve code quality over time. For example, breaking down a “God Object” into smaller, more focused classes addresses this anti-pattern directly. Similarly, refactoring tangled dependencies and improving code structure helps alleviate “Spaghetti Code”.
Illustrative Examples: A Deep Dive Into Object-Oriented Design Patterns
Let’s dive into real-world scenarios demonstrating the power and practicality of combining multiple design patterns. Understanding how these patterns interact is crucial for building robust, maintainable, and scalable software systems. We’ll explore a complex e-commerce system and then show how refactoring can improve existing code.
E-commerce System Utilizing Multiple Design Patterns
This example illustrates an e-commerce system incorporating several design patterns to handle product catalog management, order processing, and user authentication. The system needs to be flexible, scalable, and easily maintainable as it grows.
The system uses the following patterns:
- Factory Pattern: Used to create different types of products (e.g., books, electronics, clothing) without specifying their concrete classes. A
ProductFactory
class handles the creation of products based on input parameters. This promotes loose coupling and allows for easy addition of new product types. - Singleton Pattern: Implements a single instance of the
OrderProcessor
class to manage all order processing tasks. This ensures consistent order handling and avoids conflicts. - Strategy Pattern: Used for different payment methods (credit card, PayPal, etc.). Each payment method is a separate strategy class implementing a common interface. This allows for easy addition and switching of payment methods without modifying the core order processing logic.
- Observer Pattern: Used to notify users of order status changes (e.g., order placed, shipped, delivered). The
Order
class acts as the subject, and user accounts act as observers. This provides real-time updates to users. - Decorator Pattern: Used to add extra features to products (e.g., gift wrapping, expedited shipping). Each feature is a decorator class that wraps the product and adds its functionality. This allows for flexible and dynamic addition of product features.
Class Diagram Description
The class diagram shows the relationships between the classes. Product
is an abstract class with subclasses like Book
, Electronic
, and Clothing
. ProductFactory
creates instances of these subclasses. Order
aggregates Product
objects. OrderProcessor
manages orders, interacting with payment strategy classes and notifying observers. Decorator classes like GiftWrapDecorator
and ExpeditedShippingDecorator
extend product functionality. The User
class implements the Observer
interface.
Sequence Diagram Description
The sequence diagram depicts the interaction between objects during order placement. The User
interacts with the system, selecting products and choosing a payment method. The OrderProcessor
interacts with the ProductFactory
to create products, then with the chosen payment strategy to process payment. Upon successful payment, the OrderProcessor
updates the Order
status and notifies the User
(observer) through the Order
object.
Refactoring Existing Code to Incorporate Design Patterns
Consider a legacy system with a monolithic order processing function. This function handles payment, order creation, and user notification within a single large method. This is difficult to maintain and extend.
Refactoring involves:
- Extracting payment processing into separate strategy classes (Strategy Pattern). This isolates payment logic and makes it easier to add new payment methods.
- Creating an
OrderProcessor
class (Singleton Pattern) to manage order creation and status updates. This centralizes order handling logic. - Implementing an observer pattern to notify users of order status changes. This improves the system’s responsiveness and user experience.
This refactoring improves code readability, maintainability, and extensibility by applying design patterns. The system becomes more modular, allowing for easier testing and future development.
Summary
So, you’ve journeyed through the fascinating world of object-oriented design patterns. You’ve learned not just *what* these patterns are, but *why* they matter and *how* to apply them effectively. Remember, mastering OOD isn’t about memorizing every single pattern; it’s about understanding the underlying principles and choosing the right tools to solve your specific problems. Go forth and build amazing software! Now go forth and conquer those coding challenges. The power of elegant, maintainable code is in your hands.