A Step-by-Step Guide to Building RESTful APIs in Node.js: Dive into the world of backend development and unlock the power of Node.js to create robust and scalable RESTful APIs. This guide will walk you through the entire process, from setting up your environment to deploying your finished product. Get ready to build amazing things!
We’ll cover everything from understanding REST principles and choosing the right database to implementing authentication, handling errors gracefully, and deploying your API to the cloud. Whether you’re a seasoned developer or just starting your journey, this guide will equip you with the knowledge and skills to build powerful APIs that power modern web applications.
Introduction to RESTful APIs and Node.js: A Step-by-Step Guide To Building RESTful APIs In Node.js
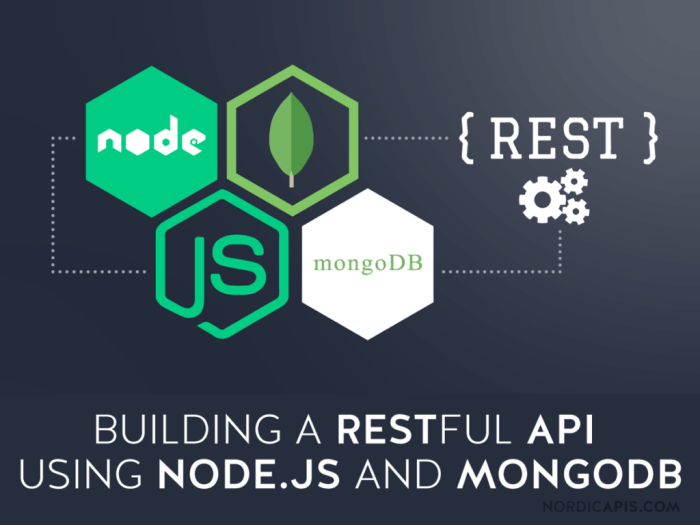
Source: nordicapis.com
Building a modern web application often involves intricate communication between different parts. This is where RESTful APIs shine, acting as the elegant messengers that facilitate this dialogue. This guide will walk you through building these APIs using the powerful Node.js framework.
RESTful APIs, or Representational State Transfer Application Programming Interfaces, are a standardized way for applications to communicate over the internet. They rely on a client-server architecture, using standard HTTP methods to perform actions on resources. Key constraints include a stateless architecture (each request contains all necessary information), client-server separation, and the use of standard HTTP methods for CRUD (Create, Read, Update, Delete) operations.
Advantages of Node.js for Building RESTful APIs
Node.js, built on Chrome’s V8 JavaScript engine, offers several compelling advantages for API development. Its non-blocking, event-driven architecture allows it to handle many concurrent requests efficiently, making it ideal for high-traffic applications. This asynchronous nature prevents a single slow request from blocking others, leading to improved performance and responsiveness. Furthermore, the vast JavaScript ecosystem provides a rich collection of readily available modules and libraries specifically designed for API development, simplifying the development process and speeding up time to market. This “JavaScript everywhere” approach also means developers can potentially reuse their front-end JavaScript skills on the backend, streamlining the development workflow. Finally, Node.js’s lightweight nature contributes to lower server resource consumption compared to some alternative technologies.
Comparison of Node.js with Other Backend Technologies
Node.js isn’t the only game in town when it comes to building APIs. Other popular choices include Python (with frameworks like Django and Flask), Java (with Spring Boot), and Ruby on Rails. Each technology has its strengths and weaknesses. Python, for example, is known for its readability and extensive libraries for data science and machine learning, making it suitable for API projects with data-heavy workloads. Java offers robust scalability and enterprise-level features but can be more resource-intensive. Ruby on Rails prioritizes developer happiness and rapid prototyping but might not be as performant as Node.js under heavy load. The choice ultimately depends on project requirements, team expertise, and scalability needs. For projects demanding high concurrency and real-time features, Node.js often emerges as a strong contender.
Core HTTP Methods in RESTful APIs
Understanding the core HTTP methods is crucial for designing effective RESTful APIs. These methods define the type of operation performed on a resource.
Method | Description | Example Endpoint | Typical Use Case |
---|---|---|---|
GET | Retrieves a resource. | /users/123 | Fetch user details. |
POST | Creates a new resource. | /users | Create a new user account. |
PUT | Updates an existing resource. | /users/123 | Modify user details. |
DELETE | Deletes a resource. | /users/123 | Delete a user account. |
Setting up the Development Environment
Building a RESTful API in Node.js starts with a solid foundation – your development environment. Getting this right ensures a smooth coding experience and prevents frustrating hiccups down the line. We’ll cover everything from installing the necessary tools to setting up your project structure, making sure you’re ready to unleash your API-building superpowers.
Setting up your development environment involves installing Node.js, npm (or yarn – your package manager of choice), creating your project, and installing the Express.js framework. Think of it as prepping your kitchen before you start cooking – you need the right ingredients and tools to create a delicious meal (your API!).
Node.js and npm Installation
Installing Node.js is the first step in your journey. Node.js is a JavaScript runtime environment that allows you to run JavaScript code outside a web browser. You can download the installer directly from the official Node.js website. The installer usually bundles npm (Node Package Manager), which is crucial for managing project dependencies. Alternatively, some users prefer Yarn, a faster and more reliable package manager. Both achieve the same goal: installing and managing the packages your project needs. After installation, verify the installation by opening your terminal and typing `node -v` and `npm -v` (or `yarn -v`). You should see the versions of Node.js and npm (or Yarn) printed to the console. If you encounter issues, refer to the official Node.js documentation for troubleshooting steps.
Creating a New Node.js Project and Initializing package.json
Once Node.js and npm are installed, you can create a new project. Create a new folder for your project (e.g., `my-api`). Navigate to this folder in your terminal. Then, initialize your project using the command `npm init -y`. This creates a `package.json` file, which acts as a manifest for your project, listing its name, version, dependencies, and other metadata. The `-y` flag automatically accepts the default values, making the process quicker. You can later edit the `package.json` file to customize these settings. Think of `package.json` as your project’s identity card.
Installing Express.js
Express.js is a minimalist and flexible Node.js web application framework that provides a robust set of features for building APIs. To install Express.js, use the command `npm install express`. This command downloads Express.js and adds it to your project’s dependencies, listed in the `package.json` file. After installation, you’re ready to start building your API’s routes and functionalities. Express.js provides the foundation upon which you’ll build your API’s structure.
Project Structure
A well-organized project structure is crucial for maintainability and scalability. Here’s a suggested structure:
A simple yet effective project structure could be:
my-api/
├── src/
│ ├── routes/
│ │ └── api.js
│ └── index.js
└── package.json
This structure separates your API routes (`routes/api.js`) from your main application file (`src/index.js`). This separation keeps your code organized and easy to navigate, making development and maintenance a breeze. You can further expand this structure as your API grows.
Building the API with Express.js
Alright, buckle up, coding cowboys and cowgirls! We’ve laid the groundwork, now it’s time to build the actual API using the awesome Express.js framework. Think of Express.js as the scaffolding for your API – it provides the structure and tools to handle incoming requests and send back responses. We’ll be crafting a robust, RESTful API that’s both efficient and elegant.
Express.js simplifies the process of creating a server and defining routes, which are essentially the URLs that trigger specific actions within your API. We’ll cover the essential HTTP methods (GET, POST, PUT, DELETE) and how to handle various request parameters. Let’s get this party started!
Creating a Basic Express.js Server
Setting up a basic Express.js server is remarkably straightforward. You’ll need to install Express.js using npm (Node Package Manager): `npm install express`. Then, you can create a server like this:
“`javascript
const express = require(‘express’);
const app = express();
const port = 3000;
app.listen(port, () =>
console.log(`Server listening on port $port`);
);
“`
This code snippet imports Express, creates an Express application instance, and starts listening on port 3000. The `console.log` statement confirms that the server is running. Simple, yet powerful!
Defining API Routes
Now, let’s define some routes. Routes are the specific URLs that your API will respond to. We’ll use Express.js methods like `app.get`, `app.post`, `app.put`, and `app.delete` to handle different HTTP methods for a single resource, let’s say, `/users`.
Handling Different HTTP Methods
Each HTTP method represents a different type of action:
GET: Retrieves data.
POST: Creates new data.
PUT: Updates existing data.
DELETE: Deletes data.
Let’s illustrate with code:
“`javascript
app.get(‘/users’, (req, res) =>
res.send(‘Get all users’);
);
app.post(‘/users’, (req, res) =>
res.send(‘Create a new user’);
);
app.put(‘/users/:id’, (req, res) =>
res.send(`Update user with ID $req.params.id`);
);
app.delete(‘/users/:id’, (req, res) =>
res.send(`Delete user with ID $req.params.id`);
);
“`
This code defines four routes, each handling a different HTTP method for the `/users` resource. Notice how `:id` in the PUT and DELETE routes allows us to specify a particular user based on their ID. The `req` object contains information about the request, while `res` is used to send the response.
Handling Request Parameters and Query Strings
Request parameters and query strings provide additional ways to customize API requests. Request parameters are part of the URL path (like the `:id` above), while query strings are appended to the URL using a question mark (e.g., `/users?name=John`).
Here’s how to access them:
“`javascript
app.get(‘/users/:id’, (req, res) =>
const userId = req.params.id;
res.send(`User ID: $userId`);
);
app.get(‘/users’, (req, res) =>
const name = req.query.name;
res.send(`Query parameter name: $name`);
);
“`
This code shows how to access a request parameter (`userId`) and a query string parameter (`name`). The `req.params` object contains the route parameters, and `req.query` contains the query string parameters. This flexibility allows for highly targeted API calls.
Data Handling and Databases
Building a robust RESTful API in Node.js isn’t just about crafting elegant routes; it’s about how you manage and interact with your data. Choosing the right database and understanding how to integrate it seamlessly with your API is crucial for scalability and performance. This section dives into the world of database options and provides practical examples to get you started.
The choice of database significantly impacts your API’s architecture and performance. Different databases excel in different scenarios. For instance, NoSQL databases like MongoDB are great for flexible schemas and rapid prototyping, while relational databases like PostgreSQL offer robust data integrity and ACID properties, ideal for applications requiring transactional consistency.
Database Options for Node.js APIs
Several database systems pair well with Node.js, each with its strengths and weaknesses. Selecting the appropriate database depends heavily on your application’s specific requirements and scalability needs. Let’s examine two popular choices: MongoDB and PostgreSQL.
- MongoDB: A NoSQL, document-oriented database. It’s known for its flexibility, scalability, and ease of use, making it a popular choice for rapid development. Data is stored in JSON-like documents, offering a natural fit for JavaScript-based applications. However, maintaining data integrity can be more challenging compared to relational databases.
- PostgreSQL: A powerful, open-source relational database. It offers robust features like ACID compliance, ensuring data consistency and reliability. Its mature ecosystem and extensive tooling make it a solid choice for applications requiring high data integrity and complex queries. The learning curve might be steeper than MongoDB, especially for developers unfamiliar with relational database concepts.
Connecting Node.js to a Database
Connecting your Node.js API to a database involves using a database driver. These drivers provide a JavaScript interface for interacting with the database. Let’s illustrate connecting to MongoDB using the mongoose
ODM (Object Data Modeling) library:
First, install mongoose
:
npm install mongoose
Then, establish a connection:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', useNewUrlParser: true, useUnifiedTopology: true)
.then(() => console.log('Connected to MongoDB!'))
.catch(err => console.error('Error connecting to MongoDB:', err));
Replace 'mongodb://localhost:27017/mydatabase'
with your MongoDB connection string. Similar steps apply to other databases, using their respective drivers (e.g., pg
for PostgreSQL).
Designing Database Schemas
A well-designed schema is fundamental to a functional database. Let’s design schemas for a simple “users” and “products” collection for a hypothetical e-commerce API. We’ll use MongoDB’s schema-less nature to illustrate flexibility.
User Schema (MongoDB)
This schema represents a user in our e-commerce application.
const userSchema = new mongoose.Schema(
firstName: type: String, required: true ,
lastName: type: String, required: true ,
email: type: String, required: true, unique: true ,
password: type: String, required: true ,
// ... other user-related fields
);
const User = mongoose.model('User', userSchema);
Product Schema (MongoDB)
This schema describes a product offered in our e-commerce store.
const productSchema = new mongoose.Schema(
name: type: String, required: true ,
description: type: String, required: true ,
price: type: Number, required: true ,
imageUrl: type: String ,
// ... other product-related fields
);
const Product = mongoose.model('Product', productSchema);
CRUD Operations
CRUD (Create, Read, Update, Delete) operations are the fundamental interactions with your database. Below are examples of these operations using the mongoose
library and the previously defined schemas.
Creating a User:
const newUser = new User( firstName: 'John', lastName: 'Doe', email: '[email protected]', password: 'securepassword' );
newUser.save()
.then(user => console.log('User created:', user))
.catch(err => console.error('Error creating user:', err));
Reading a User:
User.findById('userId')
.then(user => console.log('User found:', user))
.catch(err => console.error('Error finding user:', err));
Updating a User:
User.findByIdAndUpdate('userId', lastName: 'Smith' , new: true )
.then(user => console.log('User updated:', user))
.catch(err => console.error('Error updating user:', err));
Deleting a User:
User.findByIdAndDelete('userId')
.then(() => console.log('User deleted'))
.catch(err => console.error('Error deleting user:', err));
Similar CRUD operations can be performed on the Product
model, adapting the code to the specific fields and functionalities.
Implementing Data Validation and Error Handling
Building a robust RESTful API isn’t just about serving data; it’s about doing so safely and reliably. Data validation and comprehensive error handling are crucial for preventing unexpected behavior, securing your application, and providing a positive user experience. Without these safeguards, your API becomes vulnerable to malicious inputs and unpredictable crashes, leading to frustrating experiences for anyone using it.
Data validation ensures that the data received by your API conforms to the expected format and constraints. This prevents corrupted data from entering your system, leading to database inconsistencies or application errors. Effective error handling provides informative responses to clients when issues arise, allowing developers to debug and resolve problems quickly. This improves the overall maintainability and reliability of your API.
Mastering RESTful APIs in Node.js? Our step-by-step guide’s got you covered, from setting up your environment to deploying your app. But before you scale your tech team, remember the importance of employee well-being; check out this insightful article on Why You Should Consider Offering Health Insurance Benefits to Your Employees to attract and retain top talent.
Then, get back to building those killer APIs!
Middleware for Data Validation
Middleware functions are a powerful tool in Express.js for intercepting requests and performing actions before they reach the route handler. They’re perfect for implementing data validation. We can use packages like `express-validator` to streamline this process. This package provides a set of middleware functions to validate various data types, ensuring data integrity before it reaches the database or application logic. For instance, you can validate if a field is required, has a specific format (like an email address), or falls within a certain range.
Consider a scenario where a user is updating their profile. The API endpoint expects a JSON payload with fields like ‘name’ and ’email’. Using middleware, we can check if the ‘name’ field is present and isn’t empty, and if the ’email’ field is a valid email address. If any validation fails, the middleware can send an appropriate error response to the client, preventing the update from proceeding with invalid data.
Handling Different Error Types
Different errors require different handling strategies. Database errors, for example, might stem from connection issues or constraint violations. Validation errors occur when the input data doesn’t meet the predefined criteria. Authentication errors happen when a user lacks the necessary permissions.
A consistent approach is crucial. For instance, database errors might trigger a 500 Internal Server Error response, while validation errors result in a 400 Bad Request response. Authentication failures would typically lead to a 401 Unauthorized response. Each response should include a clear error message explaining the issue to the client.
Designing a Consistent Error Response Format
Consistency in your error responses is key to making your API easy to use and debug. A standardized format helps clients easily parse and handle errors. A common approach is to return a JSON object with fields like ‘status’, ‘message’, and optionally ‘details’.
Example:
“status”: 400,
“message”: “Validation Error”,
“details”: [
“field”: “email”, “error”: “Invalid email format”,
“field”: “password”, “error”: “Password must be at least 8 characters”
]
This structured approach makes error handling significantly easier for developers consuming your API. The consistent format allows them to anticipate the structure of the error response and handle different error scenarios accordingly. The inclusion of detailed error messages, when appropriate, aids in debugging and troubleshooting.
Implementing Authentication and Authorization
Securing your RESTful API is paramount. Without proper authentication and authorization, your meticulously crafted API becomes vulnerable to unauthorized access and data breaches. This section delves into the crucial aspects of securing your Node.js API, focusing on authentication methods and implementing robust authorization mechanisms. We’ll explore different approaches and provide a practical example using JSON Web Tokens (JWT) for authentication and role-based access control for authorization.
Authentication verifies the identity of a user, while authorization determines what resources a user is allowed to access. These are distinct but interconnected processes that work together to safeguard your API. Choosing the right authentication method depends on your specific needs and security requirements. Factors like scalability, complexity, and security considerations all play a role.
JWT Authentication
JSON Web Tokens (JWTs) are a compact and self-contained way to transmit information between parties as a JSON object. This information is digitally signed, ensuring its integrity and authenticity. In the context of API authentication, a JWT typically contains a user’s ID and other relevant claims. Upon successful authentication, the server issues a JWT to the client, which then includes this token in subsequent requests to access protected resources. The server verifies the token’s signature and payload to authorize access. This approach offers a stateless and scalable solution, making it well-suited for modern APIs.
Implementing JWT Authentication in Node.js
Implementing JWT authentication involves several steps. First, you’ll need to choose a JWT library for Node.js, such as `jsonwebtoken`. Then, you’ll create a route for user login, where you verify user credentials (e.g., username and password) against your database. Upon successful verification, you generate a JWT containing the user’s ID and other claims, and send it back to the client. Subsequent requests to protected routes should include the JWT in the `Authorization` header (e.g., `Bearer
Authorization Middleware
Authorization middleware acts as a gatekeeper, intercepting requests to protected routes and verifying if the user has the necessary permissions to access them. This middleware typically checks the user’s role or permissions against the required access level for the specific route. If the user lacks the necessary permissions, the middleware will respond with an appropriate error message (e.g., 403 Forbidden).
Role-Based Access Control (RBAC)
Role-based access control is a common authorization model where users are assigned to roles (e.g., administrator, editor, viewer), and each role has a set of permissions. This simplifies authorization management by grouping users with similar access rights. In a Node.js API, you can implement RBAC by storing user roles in your database and using the authorization middleware to check if the user’s role has the required permissions for a given route.
Code Example: Role-Based Access Control
“`javascript
const express = require(‘express’);
const jwt = require(‘jsonwebtoken’);
const app = express();
// Sample user data (in a real application, this would come from a database)
const users =
admin: id: 1, role: ‘admin’ ,
editor: id: 2, role: ‘editor’ ,
viewer: id: 3, role: ‘viewer’ ,
;
// Authentication middleware
const authenticateJWT = (req, res, next) =>
const authHeader = req.headers.authorization;
if (authHeader)
const token = authHeader.split(‘ ‘)[1];
jwt.verify(token, ‘your-secret-key’, (err, user) =>
if (err)
return res.sendStatus(403);
req.user = user;
next();
);
else
res.sendStatus(401);
;
// Authorization middleware
const authorize = (role) => (req, res, next) =>
if (req.user.role === role)
next();
else
res.sendStatus(403);
;
// Protected routes
app.get(‘/admin’, authenticateJWT, authorize(‘admin’), (req, res) =>
res.send(‘Admin page’);
);
app.get(‘/editor’, authenticateJWT, authorize(‘editor’), (req, res) =>
res.send(‘Editor page’);
);
app.get(‘/viewer’, authenticateJWT, authorize(‘viewer’), (req, res) =>
res.send(‘Viewer page’);
);
app.listen(3000, () => console.log(‘Server listening on port 3000’));
“`
API Documentation and Testing
Building a robust and maintainable RESTful API isn’t just about writing code; it’s about ensuring others (and your future self!) can understand and use it effectively. This involves comprehensive documentation and rigorous testing. Well-documented APIs are easier to integrate, debug, and evolve, while thorough testing guarantees reliability and prevents unexpected issues.
API documentation acts as a blueprint for your API, providing a clear and concise description of its functionality. This includes details on endpoints, request parameters, response formats, and error handling. Comprehensive testing, on the other hand, validates the functionality of each API endpoint, ensuring it behaves as expected under various conditions.
OpenAPI/Swagger for API Documentation
OpenAPI (formerly Swagger) is a widely adopted specification for defining RESTful APIs. It uses a standardized format (typically YAML or JSON) to describe the API’s structure, allowing for automated generation of interactive documentation and client SDKs. Tools like Swagger UI render this OpenAPI specification into an easily navigable and interactive web page, complete with examples and the ability to test API calls directly within the browser. This significantly improves developer experience and reduces integration time. For instance, an OpenAPI specification might detail an endpoint for retrieving user data, specifying the request method (GET), the URL path (`/users/userId`), request parameters (like `userId`), response codes (200 OK, 404 Not Found), and the structure of the JSON response.
Generating API Documentation with Swagger UI
Integrating Swagger UI into a Node.js project is straightforward. After defining your API using the OpenAPI specification, you can use a library like `swagger-ui-express` to serve the interactive documentation. This library takes the OpenAPI specification file as input and generates a user-friendly interface that allows developers to explore the API’s endpoints, view request/response examples, and even execute test calls. The process typically involves installing the library (`npm install swagger-ui-express`), configuring it to point to your OpenAPI specification, and mounting it as middleware in your Express.js application. A simple configuration might involve loading your specification from a file and then using the middleware to serve the UI.
Unit and Integration Testing of API Endpoints
Unit tests focus on individual components (e.g., a specific function or route handler), verifying their behavior in isolation. Integration tests, on the other hand, test the interaction between different components, ensuring they work together correctly. For example, a unit test might check that a function correctly validates user input, while an integration test might verify that a POST request to a user creation endpoint correctly creates a new user in the database and returns the appropriate response.
Using Jest for API Testing
Jest is a popular JavaScript testing framework known for its simplicity and ease of use. It offers features like built-in mocking, code coverage reports, and a powerful assertion library. A typical Jest test for an API endpoint might involve mocking database interactions, making a request to the endpoint using a tool like `supertest`, and asserting that the response status code and body match the expected values. For example, to test a GET endpoint that retrieves a list of users, you might mock the database query to return a sample user list and then assert that the response contains the expected data. This allows for testing API functionality without needing a running database during the test phase. Jest’s `expect` function allows for clear and concise assertions. For instance, `expect(response.status).toBe(200);` checks if the response status is 200 OK.
Example: Jest Test for a GET Endpoint
“`javascript
const request = require(‘supertest’);
const app = require(‘../app’); // Your Express app
describe(‘GET /users’, () =>
it(‘should return a list of users’, async () =>
const response = await request(app).get(‘/users’);
expect(response.status).toBe(200);
expect(response.body).toEqual([id:1, name: ‘Test User’, id:2, name: ‘Another User’]); //Example Data
);
);
“`
Deployment and Scaling
So, you’ve built your awesome RESTful API in Node.js. Congratulations! But the real work begins now: getting it live and ready to handle users. Deployment and scaling are crucial steps that often get overlooked, but mastering them is key to a successful API. Let’s dive into the practicalities of getting your API out there and ready for the world.
Deploying your Node.js API involves choosing a platform, preparing your code, and configuring your server. Scaling, on the other hand, is about ensuring your API can handle a growing number of requests without crashing or slowing down. We’ll cover both aspects, providing you with the knowledge and tools to make your API robust and scalable.
Deployment Platforms
Choosing the right deployment platform depends on factors like your budget, technical expertise, and the scale of your application. Popular options include Heroku, AWS (Amazon Web Services), and Google Cloud Platform (GCP). Each offers different strengths and weaknesses. Heroku provides a simple, easy-to-use platform-as-a-service (PaaS) ideal for smaller applications and rapid prototyping. AWS and GCP, on the other hand, offer a wider range of services and more control, suitable for larger, more complex applications requiring fine-grained customization. They also offer various pricing tiers, allowing you to scale your resources as needed.
Deploying to Heroku
Heroku simplifies deployment with its Git integration. After creating a Heroku account and installing the Heroku CLI, you’ll need a `Procfile` in your project’s root directory specifying the startup command for your Node.js application (e.g., `web: node index.js`). Then, you can push your code to Heroku using Git commands. Heroku automatically detects the application type and provisions the necessary resources. Monitoring is done through the Heroku dashboard, providing insights into your application’s performance and resource usage. Automatic scaling is available, adjusting resources based on traffic demand.
Deploying to AWS
Deploying to AWS typically involves using services like Elastic Beanstalk or Elastic Container Service (ECS). Elastic Beanstalk automates much of the deployment process, simplifying the setup and management of your application servers. ECS offers more control and allows you to deploy your API as containers, enhancing portability and scalability. AWS offers a wide array of services for monitoring and logging, providing comprehensive insights into your application’s health and performance. Scaling is managed through auto-scaling groups, dynamically adjusting the number of instances based on predefined metrics.
Scaling Strategies
As your API gains popularity, you’ll need to scale it to handle increased traffic. Vertical scaling involves upgrading your server’s resources (CPU, memory, etc.). While simple, it has limitations. Horizontal scaling, on the other hand, involves adding more servers to distribute the load. This is more scalable and often involves load balancers to distribute traffic evenly across multiple servers. Caching mechanisms, such as Redis, can significantly improve performance by storing frequently accessed data in memory. Database optimization and using connection pools are also vital for handling increased database load.
Deployment and Monitoring Checklist, A Step-by-Step Guide to Building RESTful APIs in Node.js
Before deploying to production, ensure you have a robust testing strategy in place. Thoroughly test your API under various load conditions. A checklist for deployment and monitoring might include:
- Code review and testing
- Deployment automation (CI/CD pipeline)
- Monitoring tools setup (e.g., Datadog, New Relic)
- Logging and error handling implementation
- Performance testing and optimization
- Security hardening and vulnerability scanning
- Rollback plan in case of issues
- Regular monitoring and maintenance
A well-defined checklist ensures a smooth deployment and helps maintain a healthy and performant API in production. Remember, proactive monitoring and regular maintenance are essential for long-term success.
Concluding Remarks
Building RESTful APIs with Node.js is a journey, not a sprint. But with this step-by-step guide, you’ve armed yourself with the knowledge to tackle any challenge. Remember, practice makes perfect. So go forth, build your API, and watch your creations power the next generation of web applications. The possibilities are endless!