Exploring the Power of Functional Programming in JavaScript sets the stage for a deep dive into a paradigm shift in how we approach JavaScript development. Forget messy, unpredictable code – functional programming offers elegance, efficiency, and a whole lot less debugging. We’ll unravel the core principles, from pure functions and immutability to higher-order functions like `map`, `reduce`, and `filter`. Get ready to unlock a new level of JavaScript mastery.
This journey will take you through the fundamental concepts, demonstrating how immutability enhances predictability, and pure functions boost testability and reusability. We’ll explore powerful techniques like function composition, currying, and partial application, showing you how to write cleaner, more maintainable code. Even the tricky subject of side effects will be tackled, offering strategies for managing them within a functional context. By the end, you’ll be equipped to apply these principles to real-world projects, transforming your JavaScript game.
Introduction to Functional Programming in JavaScript: Exploring The Power Of Functional Programming In JavaScript
So, you’re diving into the world of functional programming in JavaScript? Awesome! It might seem a bit intimidating at first, but trust us, the payoff is huge – cleaner, more predictable, and easier-to-maintain code. Think of it as upgrading your coding kitchen from a chaotic mess to a sleek, well-organized space.
Functional programming is all about treating computation as the evaluation of mathematical functions and avoiding changing-state and mutable data. Instead of relying on loops and side effects, you build your programs by composing pure functions that always produce the same output for the same input. This approach makes code much easier to reason about, test, and debug. Imagine a world where your code behaves exactly as expected, every single time – that’s the magic of functional programming.
Core Principles of Functional Programming
Functional programming rests on three key pillars: pure functions, immutability, and higher-order functions. Pure functions are the workhorses of this paradigm. They always return the same output for a given input and have no side effects – meaning they don’t modify any data outside their own scope. Immutability ensures that data doesn’t change after it’s created, preventing unexpected behavior. Higher-order functions, on the other hand, treat functions as first-class citizens, allowing you to pass them as arguments to other functions or return them as results. This enables powerful abstractions and code reusability.
Pure Functions and Higher-Order Functions in JavaScript
Let’s illustrate with some JavaScript examples. A pure function example:
function add(x, y)
return x + y;
This `add` function always returns the sum of its inputs and doesn’t change anything outside its scope. It’s a perfect example of a pure function. Now, a higher-order function example:
function applyOperation(x, y, operation)
return operation(x, y);let result = applyOperation(5, 3, add); // result will be 8
Here, `applyOperation` takes another function (`add`) as an argument and uses it to perform the calculation. This showcases the power and flexibility of higher-order functions.
A Brief History of Functional Programming and its Influence on JavaScript
Functional programming’s roots trace back to the lambda calculus developed in the 1930s. Languages like Lisp and Haskell were early pioneers, championing this paradigm. While JavaScript isn’t purely functional, it embraces many functional concepts, particularly with the rise of libraries like Lodash and Ramda. This incorporation reflects a broader trend in modern JavaScript development towards more concise, declarative, and maintainable code. The increased adoption of functional programming in JavaScript highlights its growing importance in building robust and scalable applications.
Immutability and its Benefits
Immutability, a cornerstone of functional programming, is the practice of creating data structures that cannot be modified after their creation. Think of it like a perfectly preserved historical artifact – you can examine it, copy it, or use it to create something new, but the original remains untouched. This seemingly simple concept has profound implications for building robust and predictable JavaScript applications.
Immutability significantly improves code predictability and reduces bugs by eliminating side effects. Side effects, those sneaky changes that happen outside the scope of a function, are a major source of headaches in programming. When data is immutable, a function can’t accidentally modify something it shouldn’t, leading to cleaner, more reliable code that’s easier to reason about and debug. This is especially valuable in larger, more complex projects where tracing down the source of a bug can be a time-consuming nightmare.
Maintaining Immutability in JavaScript
Several strategies help maintain immutability in JavaScript. One common approach is to create new data structures instead of modifying existing ones. For example, instead of directly pushing an item onto an array, create a new array with the item added. This ensures the original array remains unchanged. Functional programming provides several helpful tools for this, such as the `map`, `filter`, and `reduce` methods, which all operate on existing data to produce new data without altering the originals. Another crucial technique is to leverage JavaScript’s built-in immutable data structures like `const` for variables and using spread syntax (`…`) to create copies of arrays or objects.
Mutable vs. Immutable Data Structures
The difference between mutable and immutable data structures is fundamental to understanding immutability’s power.
Structure | Mutability | Example (Mutable) | Example (Immutable) |
---|---|---|---|
Array | Mutable | let arr = [1, 2, 3]; arr.push(4); // arr is now [1, 2, 3, 4] |
const arr = [1, 2, 3]; const newArr = [...arr, 4]; // arr is still [1, 2, 3], newArr is [1, 2, 3, 4] |
Object | Mutable | let obj = a: 1 ; obj.a = 2; // obj is now a: 2 |
const obj = a: 1 ; const newObj = ...obj, a: 2 ; // obj is still a: 1 , newObj is a: 2 |
String | Immutable (in most JavaScript engines) | let str = "hello"; str += "!"; //Technically creates a new string |
const str = "hello"; const newStr = str + "!"; // str remains "hello", newStr is "hello!" |
Number | Immutable | let num = 5; num += 1; // num is now 6 (re-assignment, not in-place modification) |
const num = 5; const newNum = num + 1; // num remains 5, newNum is 6 |
Pure Functions and Their Advantages
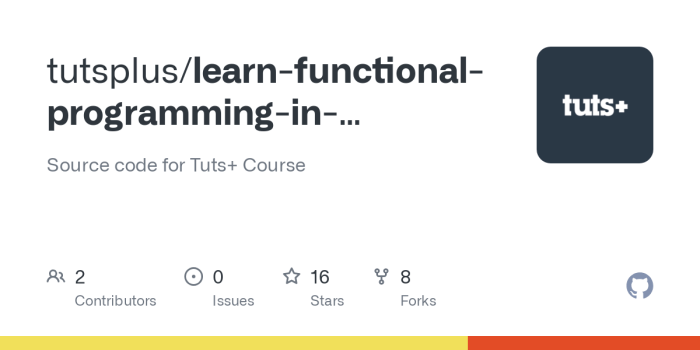
Source: githubassets.com
So, you’ve grasped immutability – fantastic! Now let’s level up your functional programming game with pure functions. These aren’t some mystical coding creatures; they’re a powerful tool for writing cleaner, more predictable JavaScript. Think of them as the reliable, consistent friends you always want on your development team.
Pure functions are the epitome of predictability in programming. They’re essentially functions that always produce the same output for the same input, and they have no side effects. This means they don’t modify any data outside their own scope, nor do they rely on anything external like global variables or network calls. In contrast, impure functions can interact with the outside world, making their behavior less predictable and harder to reason about.
Defining Pure and Impure Functions
A pure function is defined by two key characteristics: given the same input, it *always* returns the same output, and it doesn’t cause any observable side effects. An impure function, on the other hand, might produce different outputs for the same input (perhaps based on a random number generator or the current time), or it might modify variables outside its local scope, changing the state of your application in unpredictable ways.
Let’s illustrate with examples. Consider a function that adds two numbers:
`function add(x, y) return x + y; `
This is a pure function. Given `add(2, 3)`, it will *always* return `5`. It doesn’t change any external state. Now, consider a function that updates a global variable:
`let counter = 0; function incrementCounter() counter++; `
This is an impure function. It modifies the `counter` variable, which exists outside the function’s scope. Each call to `incrementCounter()` will produce a different side effect.
Benefits of Pure Functions
The benefits of embracing purity are substantial. Pure functions are inherently easier to test because their output is solely determined by their input. You can simply feed them test data and verify the results without worrying about external dependencies or state changes. This dramatically simplifies testing and improves code reliability.
Furthermore, pure functions are highly reusable. Because they don’t rely on external context, you can confidently use them in various parts of your application without fear of unexpected interactions or conflicts. This promotes modularity and makes your codebase more maintainable.
Refactoring Impure Functions into Pure Functions
Turning impure functions into their pure counterparts often involves carefully managing state. Let’s take the `incrementCounter()` example. We can refactor it to be pure by explicitly passing the counter as an argument and returning the updated value:
`function incrementCounter(counter) return counter + 1; `
Now, the function doesn’t modify any external state; it simply takes a counter value as input and returns the incremented value. The caller is responsible for managing the counter’s state. This approach makes the function’s behavior completely transparent and predictable. This principle of passing state explicitly is crucial for transforming impure functions into their pure equivalents. By doing so, you gain the significant advantages that pure functions offer, leading to cleaner, more reliable, and easier-to-maintain JavaScript code.
Higher-Order Functions
JavaScript’s functional programming capabilities are significantly boosted by higher-order functions. These aren’t just cool features; they’re powerful tools that let you write cleaner, more efficient, and easier-to-understand code. They treat functions as first-class citizens, meaning you can pass them as arguments to other functions and return them as values. This opens up a world of possibilities for manipulating data and streamlining your workflow. Let’s dive into three essential higher-order functions: `map`, `reduce`, and `filter`.
These functions provide elegant ways to process arrays without resorting to traditional loops like `for` or `while`. They enhance code readability and often lead to more concise solutions. Think of them as specialized tools designed for specific array manipulation tasks, making your code both more efficient and easier to understand.
Map Function
The `map()` function transforms each element of an array using a provided function and returns a new array with the transformed elements. It applies the given function to each element, creating a new array of the same length with the results. This is incredibly useful for tasks like converting data types, applying formatting, or performing calculations on each array item.
For instance, let’s say you have an array of numbers and you want to double each one. With `map()`, you can achieve this with a single, concise line of code:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(number => number * 2); // doubledNumbers will be [2, 4, 6, 8, 10]
Notice how clean and readable this is compared to a traditional `for` loop. The `map()` function handles the iteration implicitly, focusing the code on the transformation logic itself.
Reduce Function
The `reduce()` function is a powerhouse for accumulating results from an array. It takes a callback function and an initial value as arguments. The callback function is executed for each element, accumulating a single result from the entire array. This is perfect for tasks like summing array elements, finding the maximum or minimum value, or concatenating strings.
Let’s say we want to calculate the sum of all numbers in an array:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0); // sum will be 15
Here, the `reduce()` function iterates through the array, adding each `currentValue` to the `accumulator`. The initial value of the `accumulator` is 0. The final result is the sum of all elements.
Filter Function
The `filter()` function creates a new array containing only the elements that pass a certain condition specified by a provided function. It iterates through the array, applying a test to each element. Only elements that satisfy the condition are included in the new array. This is ideal for selecting specific elements based on criteria.
Imagine you have an array of objects representing products, and you want to filter out only the products with a price greater than $10:
const products = [
name: 'Product A', price: 15 ,
name: 'Product B', price: 5 ,
name: 'Product C', price: 20
];
const expensiveProducts = products.filter(product => product.price > 10); // expensiveProducts will contain Product A and Product C
The `filter()` function elegantly selects only the products meeting the specified price condition.
Comparison with Traditional Looping Structures
Using higher-order functions like `map`, `reduce`, and `filter` offers several advantages over traditional looping structures:
- Readability: Higher-order functions often result in more concise and readable code, as they express the intent of the operation more clearly.
- Maintainability: The code is generally easier to maintain and understand because the logic is more focused and less cluttered with loop management details.
- Efficiency: In many cases, higher-order functions can be more efficient than manually written loops, especially for large arrays, because they are optimized for array processing.
- Declarative Style: Higher-order functions encourage a declarative programming style, where you specify *what* you want to do rather than *how* to do it, leading to cleaner and more expressive code.
- Functional Purity (Potentially): When used correctly, these functions can contribute to writing more pure functions, enhancing testability and predictability.
Currying and Partial Application
Level up your JavaScript game with currying and partial application – two functional programming techniques that unlock incredible code flexibility and reusability. These methods transform how you think about functions and arguments, leading to cleaner, more maintainable code. Essentially, they let you break down complex functions into smaller, more manageable pieces.
Currying and partial application are closely related but distinct concepts. Currying transforms a function that takes multiple arguments into a sequence of functions that each take a single argument. Partial application, on the other hand, fixes some of a function’s arguments, creating a new function with fewer arguments. Let’s dive into the specifics.
Currying
Currying is a powerful technique where a function that takes multiple arguments is transformed into a sequence of functions that each take a single argument. This process creates a chain of functions, where each function returns another function until the final result is computed. The advantage? Increased code modularity and reusability.
Consider a function that adds three numbers:
“`javascript
const addThreeNumbers = (a, b, c) => a + b + c;
“`
The curried version would look like this:
“`javascript
const curriedAddThreeNumbers = (a) => (b) => (c) => a + b + c;
“`
Now, we can call it like this:
“`javascript
const add5and10 = curriedAddThreeNumbers(5)(10); // Returns a function that takes one argument
const result = add5and10(3); // result is 18
“`
This approach allows us to partially apply arguments, creating specialized functions. For instance, `curriedAddThreeNumbers(5)(10)` returns a function that adds 15 to any input. This level of flexibility makes your code adaptable to various scenarios.
Partial Application
Partial application involves creating a new function by pre-filling some of the arguments of an existing function. This simplifies function calls and enhances code readability. It’s a more direct way to achieve some of the benefits of currying, without the nested function structure.
Let’s revisit our `addThreeNumbers` function:
“`javascript
const addThreeNumbers = (a, b, c) => a + b + c;
“`
Using partial application with a library like Lodash, we can create a new function that adds 5 and 10 to any input:
“`javascript
const _ = require(‘lodash’);
const add15 = _.partial(addThreeNumbers, 5, 10);
const result = add15(3); // result is 18
“`
Here, `_.partial` takes the original function and the pre-filled arguments, returning a new function that expects only the remaining arguments. This is cleaner than the curried version if you don’t need the intermediate functions.
Advantages of Currying and Partial Application
Both currying and partial application offer significant advantages in functional programming:
Improved Code Readability: Breaking down complex functions into smaller, more focused pieces enhances code clarity and makes it easier to understand the logic.
Increased Code Reusability: Partially applied functions can be reused in different parts of your application, reducing code duplication and improving maintainability.
Enhanced Flexibility: Currying and partial application allow you to create specialized functions tailored to specific needs, boosting code adaptability.
Simplified Testing: Smaller, more focused functions are easier to test individually, leading to more robust and reliable code.
Better Composition: Curried and partially applied functions compose more easily, enabling the creation of sophisticated functionalities by chaining simple functions.
Handling Side Effects
So, you’ve embraced the purity of functional programming in JavaScript – immutability, pure functions, the whole shebang. But the real world is messy. It throws curveballs like network requests, user interactions, and database updates – all things that, let’s face it, cause side effects. Understanding and managing these side effects is crucial for building robust and predictable functional applications. Let’s dive in.
Side effects, in essence, are any action that alters something outside the scope of a function. This can range from modifying global variables to making API calls. They break the beautiful predictability of pure functions, making testing and reasoning about your code significantly harder.
Common Sources of Side Effects in JavaScript
Side effects are often lurking where you least expect them. They’re not always obvious, and identifying them is the first step towards managing them effectively. Here are some common culprits:
- Modifying Global Variables: Directly altering variables outside the function’s local scope introduces unpredictable behavior and makes code harder to maintain.
- Mutating Data Structures: Changing arrays or objects in place (using methods like
push()
,splice()
, or directly assigning new values to object properties) is a major source of side effects. - I/O Operations: Interactions with the outside world, such as network requests (
fetch()
), file system access, or console logging (console.log()
), all involve side effects. - Date and Time: Functions relying on the current date or time can produce different results depending on when they’re executed, making testing difficult.
- DOM Manipulation: Directly altering the Document Object Model (DOM) – think updating the UI – introduces side effects that can complicate your code.
Strategies for Minimizing Side Effects
The ideal scenario is to eliminate side effects entirely. While this isn’t always feasible, we can certainly minimize their impact and contain them effectively.
- Favor Immutability: Stick to immutable data structures. Create new objects or arrays instead of modifying existing ones. This prevents unexpected changes elsewhere in your code.
- Isolate Side Effects: Group side-effecting operations into specific functions or modules. This creates clear boundaries and makes testing easier. Consider using techniques like the Command pattern to encapsulate actions that modify the outside world.
- Use Pure Functions Where Possible: Whenever feasible, design functions that don’t have side effects. These are easier to test, reason about, and reuse.
- Functional Composition: Break down complex operations into smaller, composable pure functions, and only use side-effecting functions at the edges of your application.
The Role of Monads in Handling Side Effects
Monads are a powerful concept in functional programming that provide a structured way to handle side effects. Think of them as containers that encapsulate values along with their associated side effects. They allow you to chain operations involving side effects while maintaining a semblance of functional purity. Common monads used for this purpose include the Maybe monad (for handling potential null or undefined values) and the Either monad (for handling potential errors). While a deep dive into monads is beyond the scope of this discussion, understanding their role in managing side effects is key to writing more robust functional JavaScript code. They essentially help you manage the “flow” of potential errors or exceptional situations without explicitly resorting to non-functional techniques like try-catch blocks. They allow you to express error handling and other side-effects in a compositional way, enabling a functional paradigm even when faced with the inherent non-functional nature of certain operations.
Practical Applications and Case Studies
Functional programming, while initially seeming abstract, offers tangible benefits in real-world JavaScript applications. Its emphasis on immutability, pure functions, and higher-order functions leads to cleaner, more maintainable, and often more performant code. Let’s explore how these principles translate into practical solutions.
Many popular JavaScript libraries and frameworks leverage functional programming concepts extensively. Understanding these principles helps developers write more efficient and predictable code, reducing debugging time and improving overall application quality.
Mastering functional programming in JavaScript unlocks elegant code, but life throws curveballs. Think about securing your financial future; unexpected disability can derail your coding career, which is why understanding the importance of insurance is crucial. Check out this resource on The Role of Insurance in Protecting Your Income from Disability to plan ahead. Then, armed with financial security, you can return to exploring the power and purity of functional paradigms in your JavaScript projects with peace of mind.
React and Functional Components
React, a dominant JavaScript library for building user interfaces, heavily utilizes functional components. These components are pure functions that take props (input) and return JSX (output), representing the user interface. This approach promotes reusability, testability, and simplifies state management. For example, a simple functional component to display a user’s name might look like this:
const UserGreeting = ( name ) => ;
This concise component is pure, predictable, and easy to test because its output depends solely on its input. No side effects are involved. The immutability of props ensures that changes within the component don’t unexpectedly affect other parts of the application.
Data Transformation with Ramda
Libraries like Ramda provide a collection of higher-order functions that simplify complex data manipulations. Imagine you have an array of user objects, and you need to extract only their email addresses. With Ramda, this becomes a one-liner:
const emails = R.map(R.prop('email'), users);
This elegantly demonstrates the power of functional composition. R.map
applies a function (R.prop('email')
) to each element of the array, extracting the email property. This approach is significantly more concise and readable than equivalent imperative code.
Case Study: Improving a Legacy Codebase, Exploring the Power of Functional Programming in JavaScript
A large e-commerce platform faced challenges with its legacy codebase, characterized by mutable state, complex dependencies, and frequent bugs. Refactoring parts of the application to embrace functional programming principles significantly improved maintainability and reduced bugs. Specifically, migrating from imperative loops to functional transformations using map
, filter
, and reduce
resulted in code that was easier to understand, test, and debug. The introduction of pure functions minimized unexpected side effects, and the adoption of immutability made the code more predictable and less prone to errors. The result was a decrease in bug reports and faster development cycles. The project demonstrated that even large, established codebases can benefit from incorporating functional programming techniques. The improvements in maintainability and reduced debugging time yielded a substantial return on investment.
Advanced Functional Programming Concepts (Optional)
So, you’ve grasped the basics of functional programming in JavaScript – congrats! Ready to level up your skills? Let’s dive into some more advanced concepts that can significantly boost your code’s elegance and efficiency. These aren’t strictly necessary for all projects, but understanding them unlocks a deeper appreciation of functional programming’s power.
These advanced concepts help you manage complexity and work with potentially problematic data more effectively, leading to cleaner, more robust code. We’ll explore functors, applicatives, and monoids – powerful tools that provide structure and control when dealing with computations that might involve uncertainty or multiple possible outcomes.
Functors
Functors are a type of structure that represents a value wrapped in a context. Think of it like a container that holds a value, but also provides a way to apply a function to that value *without* directly accessing it. This is done through a special method called `map`. The `map` method applies a function to the value inside the container and returns a new container with the transformed value. This ensures immutability—a core principle of functional programming.
A simple example using an array (which acts as a functor):
“`javascript
const numbers = [1, 2, 3];
const doubledNumbers = numbers.map(x => x * 2); // [2, 4, 6]
“`
Here, `map` applies the doubling function to each element without modifying the original `numbers` array. This is a fundamental characteristic of functors: they allow you to transform the contained value while maintaining the container’s structure.
Applicatives
Applicatives build upon functors. While functors apply a single function to a single value, applicatives allow you to apply a *function contained within a context* to a *value contained within a context*. This is particularly useful when dealing with functions that might themselves be uncertain or involve multiple possibilities. The key method here is `ap` (apply).
Consider a scenario where you have a function and an argument, both wrapped in containers:
“`javascript
const add5 = x => x + 5;
const maybeNumber = value: 10, isPresent: true; //Simulating a context that might not have a value
const add5Result = applicativeApply(maybeNumber, add5); //Result would be value: 15, isPresent: true if correctly implemented, otherwise a default
“`
This `applicativeApply` (a hypothetical function) would apply the function `add5` to the `maybeNumber` value, correctly handling the possibility that `maybeNumber` might not have a value (represented by the `isPresent` property in this simplified example). A real-world example might involve handling asynchronous operations or dealing with potentially missing data.
Monoids
Monoids represent a structure with two key components: a binary operation (often denoted by ⊕) and an identity element (often denoted by ε). The binary operation must be associative (meaning (a ⊕ b) ⊕ c = a ⊕ (b ⊕ c)) and the identity element must satisfy a ⊕ ε = a and ε ⊕ a = a for all elements a. Monoids provide a way to combine values in a consistent and predictable manner.
A simple example is the addition of numbers: the binary operation is addition (+), and the identity element is 0. String concatenation is another example: the binary operation is concatenation, and the identity element is the empty string “”. Monoids are useful for accumulating values or reducing collections.
“`javascript
//Example with string concatenation (a monoid)
const strings = [“Hello”, ” “, “World”];
const combinedString = strings.reduce((acc, str) => acc + str, “”); // “Hello World”
“`
The `reduce` function here showcases the monoid’s properties: it combines the strings using concatenation (the binary operation), starting with an empty string (the identity element).
Ending Remarks
So, there you have it – a glimpse into the world of functional programming in JavaScript. By embracing immutability, mastering pure functions, and utilizing higher-order functions, you can dramatically improve your code’s quality, readability, and maintainability. This isn’t just about writing better code; it’s about unlocking a more efficient and enjoyable development process. Ready to ditch the spaghetti code and embrace the elegance of functional programming? The journey starts now.