A Guide to Version Control with Git and GitHub: Ever wished you could magically undo coding mistakes or effortlessly collaborate on projects? Version control is your superpower, and Git and GitHub are the tools to wield it. This guide dives into the world of managing code changes, making collaboration a breeze, and preventing coding chaos. Get ready to level up your dev game!
From setting up your first Git repository to mastering branching strategies and navigating the intricacies of GitHub, we’ll cover everything you need to know. We’ll demystify complex commands, offer practical examples, and equip you with the skills to confidently manage your projects. Prepare for a smooth journey into the heart of collaborative coding.
Introduction to Version Control and Git
Version control is like having a superpower for your projects. It lets you track every single change you make, rewind to previous versions if needed, and collaborate seamlessly with others. Think of it as a detailed history book for your code, documents, or any other files you’re working on. Without it, you’re essentially working blindfolded, making it hard to manage updates and potentially losing hours of work to accidental deletions or overwrites.
Version control systems (VCS) are the tools that make this magic happen. They record changes to files over time, allowing you to recall specific versions and understand who made which edits. This is invaluable for teamwork, especially in software development, where multiple developers work simultaneously on the same project.
Benefits of Using Git for Software Development
Git offers a plethora of advantages for software developers. Its distributed nature means every developer has a complete copy of the project’s history, enabling offline work and faster branching and merging. This flexibility significantly reduces bottlenecks and streamlines the development process. Git’s speed and efficiency are unmatched by many other systems, allowing for rapid iteration and deployment. Furthermore, its robust branching model facilitates experimentation with new features without disrupting the main codebase. The extensive community support and abundance of readily available resources make learning and using Git relatively straightforward.
Comparison of Git with Other Version Control Systems
While other version control systems like Subversion (SVN) exist, Git’s distributed nature sets it apart. SVN is a centralized system, meaning the project history resides on a central server. This creates a single point of failure and can be slower for larger projects. Git, in contrast, allows developers to work independently and then merge their changes, promoting greater collaboration and resilience. The branching and merging capabilities in Git are also far more flexible and efficient than in SVN, allowing for a more agile development workflow. The speed and efficiency of Git, especially for larger projects, often makes it the preferred choice among developers.
Installing Git on Different Operating Systems
Installing Git is generally a straightforward process, regardless of your operating system.
Git Installation on Windows
1. Download the latest Git for Windows installer from the official Git website.
2. Run the installer and follow the on-screen instructions. You’ll likely be presented with several options; the default settings are usually suitable for most users. Pay attention to the options related to the command-line interface and your preferred text editor.
3. Once the installation completes, open your command prompt or Git Bash to verify the installation by typing `git –version`. This should display the installed Git version.
Git Installation on macOS
1. Download the latest Git for macOS installer from the official Git website.
2. Run the installer and follow the on-screen instructions. Similar to Windows, the default settings are usually sufficient.
3. Verify the installation by opening your terminal and typing `git –version`.
Git Installation on Linux
The installation process on Linux distributions varies depending on your specific distribution (e.g., Ubuntu, Fedora, Debian). Generally, you can use your distribution’s package manager. For example, on Debian/Ubuntu, you would use the command `sudo apt-get update && sudo apt-get install git`. After installation, verify it by typing `git –version` in your terminal.
Basic Git Commands and Workflow
So, you’ve grasped the core concept of version control – fantastic! Now let’s dive into the practical side of things with Git, the undisputed king of version control systems. We’ll cover the essential commands and a typical workflow, making your Git journey smooth sailing. Think of this as your Git initiation ritual – once you’ve completed this section, you’ll be ready to tackle more complex scenarios.
Git operates on a local repository, a hidden folder within your project directory that stores all your project’s version history. Let’s build one and get familiar with the key commands.
Creating a Git Repository
Creating a new Git repository is straightforward. Navigate to your project’s directory using your terminal or command prompt. Then, simply type `git init`. This command creates a hidden `.git` folder in your project’s root directory, initializing the repository. After this, Git starts tracking changes within that directory. Think of it as setting up the stage for your project’s version control journey.
Using Core Git Commands
Now that we’ve initialized our repository, let’s explore some fundamental commands.
git status
: This command is your best friend. It shows you the current state of your repository, highlighting any changes you’ve made that haven’t been staged or committed. It’s like a quick project health check, telling you what needs attention.
Mastering Git and GitHub is like building a solid financial foundation for your code projects. Just as careful planning secures your future, version control safeguards your work. Think of it like securing your investments – you wouldn’t skip it, right? Learn more about securing your financial future with How to Protect Your Investments with Life Insurance , and then get back to building that awesome app, knowing your code is safe and sound with Git.
git add
: After making changes to your files, use `git add
git commit -m "Your descriptive message"
: This command saves your staged changes with a descriptive message. The `-m` flag allows you to include a concise summary of your changes. Think of each commit as a snapshot of your project at a specific point in time. The message acts as a label for that snapshot, making it easy to understand what changes were made.
git log
: This command displays the commit history of your repository, showing each commit’s message, author, date, and hash (a unique identifier). It’s a chronological record of your project’s evolution, allowing you to easily review changes and revert to previous versions if necessary.
Common Git Workflows: Branching and Merging
Imagine you’re working on a new feature while simultaneously fixing a bug. Using Git’s branching capabilities prevents your feature development from disrupting the main codebase. A branch is essentially a parallel version of your project.
To create a new branch, use `git checkout -b
The Importance of Clear Commit Messages
Writing clear and concise commit messages is crucial for maintainability and collaboration. A good commit message should briefly explain *what* changes were made, *why* they were made, and ideally, *how* they were implemented (if relevant). For example, instead of “fix,” use something more descriptive like “Fixed bug in user authentication: corrected password validation regex.” Imagine having to decipher vague commit messages months later – good commit messages save time and headaches down the road.
Branching and Merging in Git
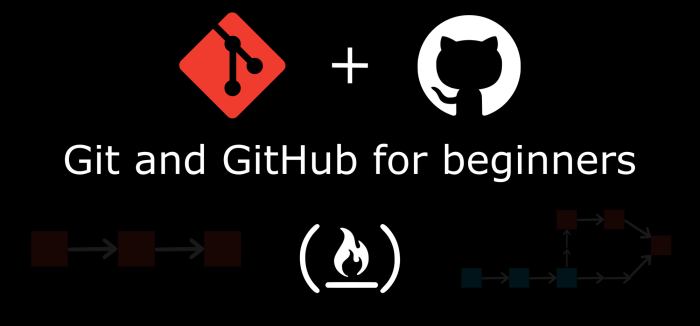
Source: freecodecamp.org
Git’s branching model is its superpower. It’s what lets you work on multiple features simultaneously without messing up your main codebase. Think of branches as parallel universes for your project, each containing its own version of the code. This allows for cleaner development, easier collaboration, and less risk of breaking your production-ready code.
Branching and merging are fundamental Git operations that enable parallel development and code integration. Understanding these concepts is crucial for efficient teamwork and managing complex projects. This section will cover the practical aspects of branching, merging, conflict resolution, and different branching strategies.
Branch Creation, Merging, and Deletion
Creating a new branch is straightforward using the command git branch
. This creates a new branch pointing to the same commit as your current branch. To start working on this new branch, use git checkout
. This switches your working directory to the newly created branch. Now you can make changes, commit them, and the main branch remains untouched. When your work is ready, merge the branch back into the main branch using git merge
. This integrates your changes. Finally, to remove the branch, use git branch -d
(use -D
for forceful deletion if the branch has unmerged changes).
Merge Conflict Resolution
Sometimes, two branches modify the same lines of code. This creates a merge conflict. Git will mark these conflicts in the affected files. You’ll need to manually edit these files, resolving the conflicts by choosing which changes to keep. After resolving the conflicts, stage the changes using git add
and then commit the merge using git commit
. Git will automatically create a merge commit that combines the changes from both branches.
Examples of Git Commands
Let’s say we’re building a website. We have a `main` branch representing the live site. We want to add a new feature: a contact form.
First, we create a new branch:
git branch contact-form
Then, we switch to it:
git checkout contact-form
We develop the contact form, commit our changes:
git add .
git commit -m "Added contact form"
Once the form is ready, we switch back to the `main` branch:
git checkout main
Finally, we merge the `contact-form` branch:
git merge contact-form
If no conflicts arise, the merge is clean. If conflicts occur, Git will highlight them in the affected files, requiring manual resolution before committing the merge.
Rebase is an alternative to merging. Instead of creating a merge commit, rebase rewrites the commit history by applying your commits on top of the target branch. This results in a cleaner, linear history, but should be used cautiously, especially on shared branches.
git rebase
Branching Strategies Comparison
Understanding different branching strategies is crucial for managing the complexity of larger projects and teams. Here’s a comparison:
Branching Strategy | Description | Advantages | Disadvantages |
---|---|---|---|
Gitflow | Uses separate branches for development, features, releases, and hotfixes. | Strict workflow, good for large teams, clear separation of concerns. | Can be complex for smaller projects, adds overhead. |
GitHub Flow | Simpler workflow; features are branched from `main`, reviewed, and merged back. | Simple, easy to understand, good for frequent releases. | Less structure, may lead to less organization in larger projects. |
GitLab Flow | Extends GitHub Flow with support for environments (e.g., staging, production). | Combines simplicity with environment management. | Requires more setup and understanding than GitHub Flow. |
Trunk-Based Development | All developers work directly on the `main` branch, integrating frequently. | Simple, minimizes merge conflicts, promotes frequent integration. | Requires rigorous testing and continuous integration. |
Working with Remote Repositories on GitHub: A Guide To Version Control With Git And GitHub
So you’ve mastered the basics of Git, managing your project files locally. But the real power of Git unlocks when you start collaborating and sharing your code with others – that’s where GitHub comes in. This section will guide you through connecting your local Git repository to a remote repository on GitHub, enabling seamless teamwork and version control across multiple machines.
GitHub is the go-to platform for hosting and managing Git repositories. It’s a social coding environment where developers can share their projects, collaborate on code, and track changes efficiently. Let’s explore how to leverage this powerful tool to enhance your Git workflow.
Creating a GitHub Account and Repository
First things first: you’ll need a GitHub account. Head over to github.com, click “Sign up,” and follow the straightforward steps to create your profile. Once you’re in, creating a new repository is equally simple. Click the “+” button in the top right corner, select “New repository,” and fill in the required information: repository name (choose something descriptive and memorable), a brief description, and whether you want it to be public (anyone can see it) or private (only you and those you explicitly grant access to can see it). You can also choose to initialize the repository with a README file (highly recommended!), a .gitignore file (to specify files Git should ignore), and a license. After you click “Create repository,” GitHub will generate a unique URL for your new repository.
Connecting a Local Git Repository to a Remote Repository on GitHub
Now that you have a remote repository on GitHub, it’s time to link it to your local project. This process involves using the `git remote add` command. Let’s say your local repository is in a folder named “my-project” and your GitHub repository URL is `https://github.com/yourusername/my-project.git`. Open your terminal, navigate to the “my-project” directory, and execute the following command:
git remote add origin https://github.com/yourusername/my-project.git
This command adds a remote named “origin” (a common convention) pointing to your GitHub repository. You can now push your local commits to this remote repository.
Using `git remote`, `git push`, and `git pull`
The `git remote` command allows you to manage your remote repositories. Beyond `add`, you can use `remove` to delete a remote, `rename` to change its name, and `show` to view details about a remote.
The `git push` command uploads your local commits to the remote repository. After making changes and committing them locally, use:
git push origin main
(or `git push origin master` if your main branch is named `master`). This pushes your commits from your local `main` branch to the `main` branch on the remote repository.
`git pull` downloads changes from the remote repository to your local repository. Before making changes, it’s good practice to pull the latest updates to avoid conflicts:
git pull origin main
This fetches and merges changes from the `main` branch on the remote repository into your local `main` branch.
Best Practices for Collaborating on a Git Repository with Multiple Developers
Effective collaboration requires clear communication and established workflows. Here are some best practices:
Before diving into a list of best practices, it’s crucial to understand that consistent communication and well-defined workflows are key to successful collaboration. These practices minimize conflicts and ensure everyone is on the same page.
- Use Feature Branches: Instead of directly committing to the `main` branch, create separate branches for each new feature or bug fix. This isolates changes and prevents disrupting the main codebase.
- Frequent Commits and Push: Commit your changes regularly with descriptive commit messages. Push your feature branches to the remote repository often to share your progress and allow for early feedback.
- Pull Requests (PRs): Use pull requests to propose changes to the main branch. This allows for code review and discussion before merging.
- Clear Communication: Maintain open communication with your team members. Discuss changes, potential conflicts, and any issues you encounter.
- Regularly Update Your Local Repository: Pull the latest changes from the remote repository frequently to ensure you’re working with the most up-to-date code.
Creating a Pull Request on GitHub
Once you’ve pushed your feature branch to the remote repository, you can create a pull request to merge it into the main branch. On GitHub, navigate to your repository. You’ll see a “Pull requests” tab. Click “New pull request.” GitHub will automatically detect your branch and suggest a comparison with the main branch. Review the changes, write a clear and concise description of your work, and click “Create pull request.” Your team can then review your code, provide feedback, and approve the merge. Once approved, you can merge your branch into the main branch.
Git Ignoring Files and Folders
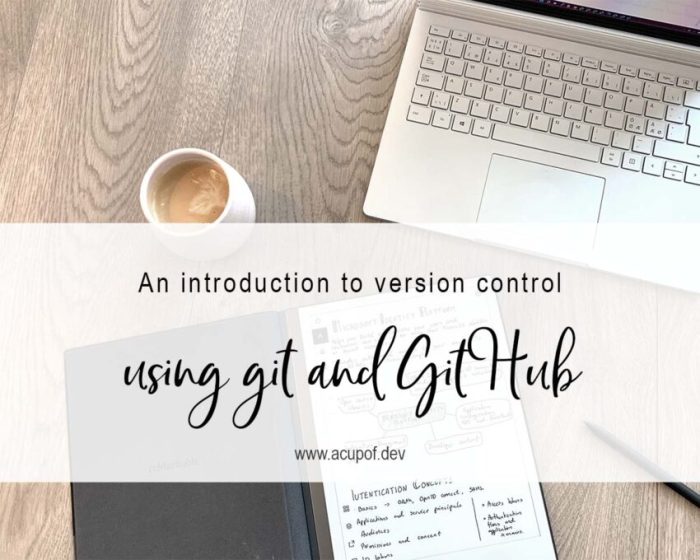
Source: elischei.com
Keeping your Git repository clean and efficient is crucial for a smooth workflow. A cluttered repo can lead to unnecessary commits, merge conflicts, and a generally frustrating experience. That’s where `.gitignore` files come in – your secret weapon for maintaining a tidy and focused version control system. This unsung hero helps you specify files and folders that Git should completely ignore, preventing them from being tracked and cluttering your repository.
Ignoring files is essential for excluding files that are generated during the build process, temporary files, system-specific configurations, or sensitive data like passwords. By strategically using a `.gitignore` file, you can keep your repository focused on the core code and assets that truly matter, enhancing collaboration and reducing potential conflicts.
Files and Folders Typically Ignored
A `.gitignore` file acts as a filter, telling Git which files and folders to ignore. Common candidates for exclusion include:
- Temporary files: Files created during development, like `.tmp` files, `*.log` files, and other temporary data generated by your IDE or build processes.
- System files: Operating system-specific files like `.DS_Store` (macOS), `Thumbs.db` (Windows), or other hidden files that are not part of your project’s source code.
- Build artifacts: Files generated by compilers or build tools, such as `.class` files (Java), `.o` files (C/C++), or output directories containing compiled code.
- Configuration files: Local configuration files that might contain sensitive data or paths specific to your development environment. Examples include `config.ini`, `.env` files containing database credentials or API keys.
- Backup files: Files with extensions like `.bak`, `.swp`, or similar, which are usually backups created by text editors.
Examples of .gitignore Entries
The `.gitignore` file uses simple patterns to specify files and folders to ignore. Here are some examples:
*.log
: Ignores all files ending with `.log`.temp/
: Ignores the entire `temp` directory and its contents.*.class
: Ignores all files ending with `.class`.node_modules/
: Ignores the `node_modules` directory, common in JavaScript projects.*.env
: Ignores all files ending with `.env` (containing sensitive data)./secrets/
: Ignores the entire `secrets` directory (for storing sensitive data).
You can use wildcard characters like `*` (matches any sequence of characters), `?` (matches any single character), and `[]` (matches any character within the brackets) to create more flexible patterns. For instance, `[abc].txt` would match `a.txt`, `b.txt`, or `c.txt`.
Creating and Managing a .gitignore File
Creating a `.gitignore` file is straightforward. Simply create a new file named `.gitignore` in the root directory of your project. Add your desired ignore patterns, one per line. After adding or modifying the `.gitignore` file, you need to stage and commit it using `git add .gitignore` and `git commit -m “Added .gitignore”`. Git will then respect the patterns defined within the file. If you need to ignore files that were already tracked, you may need to remove them from the staging area using `git rm –cached
Understanding Git History and Rewriting History
Navigating the history of your Git repository is crucial for effective collaboration and project management. Understanding how changes have evolved, who made them, and when, allows for better debugging, easier collaboration, and a clearer picture of your project’s development. However, Git’s power extends beyond simply viewing history; it also offers tools to modify that history, a capability that demands careful consideration and understanding.
Git provides a detailed record of every change made to your project. This history, visualized through commands like `git log`, is not just a chronological list; it’s a complex graph reflecting branches, merges, and individual commits. Mastering the ability to interpret this graph is essential for efficient Git usage. Incorrectly manipulating this history, however, can lead to significant problems, especially in collaborative environments.
Git Revert
The `git revert` command creates a new commit that undoes the changes introduced by a specific commit. This is a safe and non-destructive approach to reversing changes, as it preserves the original commit history. It’s particularly valuable when working on shared repositories, as it avoids altering the shared history and potential conflicts with other developers. The process involves creating a new commit that essentially “reverses” the effects of the target commit. For example, `git revert
Git Reset
`git reset` is a more powerful, yet potentially dangerous, command. It alters the project’s history by moving the branch pointer to a different commit. This can be used to undo commits, but it directly modifies the branch’s history. There are three main modes: `–soft`, `–mixed` (the default), and `–hard`. `–soft` only moves the branch pointer, leaving the changes staged; `–mixed` moves the pointer and unstages the changes; and `–hard` moves the pointer and discards the changes entirely. Using `git reset –hard` on a shared branch is strongly discouraged, as it can cause significant issues for collaborators. For example, `git reset –hard HEAD~2` would move the branch pointer two commits back, discarding all changes in those commits.
Risks and Best Practices for Rewriting Git History
Rewriting history, primarily through `git reset` and interactive rebasing (`git rebase -i`), introduces significant risks, especially in collaborative projects. Altering the shared history can lead to confusion, conflicts, and broken workflows for other developers. Furthermore, it can make it difficult to track the evolution of the project accurately. Best practices emphasize avoiding history rewriting on shared branches. If changes need to be undone, `git revert` is the preferred approach. If rewriting is necessary, it should be done on local branches before merging into the shared branch. Thorough communication with collaborators is also crucial to avoid unexpected issues.
Scenarios Where Rewriting History Might Be Useful
Rewriting history can be beneficial in specific circumstances, primarily when dealing with local branches and before sharing code with others. For example, squashing multiple commits into a single, more concise commit before creating a pull request improves readability and reduces noise in the project history. Similarly, amending the most recent commit (`git commit –amend`) allows for correcting small mistakes or adding forgotten files without creating unnecessary commits. These actions are perfectly acceptable as long as they occur on local branches before pushing to remote repositories.
Scenarios Where Rewriting History Should Be Avoided
Rewriting history on shared branches should be strictly avoided. This includes any branch that has been pushed to a remote repository and is accessible to other developers. Modifying the shared history can lead to conflicts, broken workflows, and significant disruption for collaborators. This can result in lost work, confusion, and increased development time as everyone attempts to resolve the inconsistencies. Always prioritize using `git revert` for undoing changes on shared branches.
Advanced Git Techniques
Level up your Git game with these advanced techniques that’ll transform you from a Git novice to a pro. We’ll explore powerful commands, strategies for managing complex projects, and different workflows to streamline your collaboration. Get ready to conquer even the most challenging version control scenarios!
Mastering Git isn’t just about knowing the basics; it’s about wielding its power effectively. This section delves into advanced techniques that will significantly improve your efficiency and collaboration, especially in larger, more complex projects.
Interactive Rebase
Interactive rebasing, using the command `git rebase -i`, allows you to edit your commit history. This is incredibly useful for cleaning up messy commit messages, squashing multiple commits into one, or reordering commits to create a more linear and readable history. The interactive rebase opens a text editor where you can choose actions for each commit, such as “pick,” “reword,” “edit,” “squash,” “fixup,” and “drop.” For instance, you might use “squash” to combine several small, related commits into a single, more descriptive commit. This makes your project history much easier to understand and navigate, particularly for collaborators. Careful consideration should be given before rebasing commits that have already been pushed to a shared repository, as this can cause complications for others working on the project.
Managing Large and Complex Projects
Working with large, complex projects requires a structured approach to Git. One effective strategy is to utilize a modular approach, breaking down the project into smaller, more manageable repositories. This allows for independent development and deployment of modules, reducing the overall complexity of the main repository. Another important aspect is to establish clear branching strategies, perhaps employing a Gitflow workflow (discussed below), to manage different features, releases, and bug fixes concurrently. Regularly using techniques like `git gc` to clean up the repository can help maintain performance and prevent excessive storage usage. Finally, effective communication and collaboration among team members are crucial for success in large-scale projects.
Comparison of Git Workflows
Several Git workflows exist, each with its own advantages and disadvantages. Let’s compare two popular options: Gitflow and GitHub Flow.
Gitflow is a more formal branching model, utilizing branches like `develop`, `master`, `feature`, `release`, and `hotfix`. It’s well-suited for larger projects with a structured release cycle. GitHub Flow, on the other hand, is simpler and focuses on feature branches directly from `master`. It’s ideal for smaller teams or projects with frequent releases. The choice depends on the project’s complexity and team size.
Workflow | Description | Advantages | Disadvantages |
---|---|---|---|
Gitflow | Uses multiple long-lived branches for features, releases, and hotfixes. | Structured, well-suited for large projects with planned releases. | Can be complex to learn and manage. |
GitHub Flow | Uses short-lived feature branches directly from master. | Simple and easy to learn, fast release cycles. | Less structured, might not be suitable for large or complex projects. |
Illustrating a Complex Merge Scenario
Imagine three developers working on a project. Developer A creates a feature branch (`feature/new-login`) and makes several commits. Simultaneously, Developer B works on a bug fix (`bugfix/broken-image`) and merges it into `master`. Developer C starts working on a new feature (`feature/user-profiles`), also branching from `master`. Developer A finishes `feature/new-login` and attempts to merge it into `master`. However, due to the changes made by Developer B and C, a complex merge conflict arises. The visual representation would show three diverging branches from `master`: `feature/new-login`, `bugfix/broken-image`, and `feature/user-profiles`. The merge of `feature/new-login` into `master` would require resolving the conflicts that arise from the changes in `bugfix/broken-image` and `feature/user-profiles`. This illustrates how concurrent development can lead to complex merge situations requiring careful conflict resolution. This scenario highlights the importance of frequent integration and clear communication among developers.
GitHub Features and Collaboration
GitHub is more than just a repository for your code; it’s a powerful platform for collaborative software development. Its features go beyond simple version control, offering robust tools for issue tracking, project management, and code review, fostering efficient teamwork and high-quality software. Let’s dive into how these features enhance the development process.
GitHub’s collaborative features are designed to streamline workflows and improve communication among developers. From tracking bugs and feature requests to managing complex projects and facilitating code reviews, GitHub provides a centralized hub for all aspects of the development lifecycle. The seamless integration of these tools allows teams to work more efficiently and produce better software.
Issue Tracking and Project Management, A Guide to Version Control with Git and GitHub
GitHub’s issue tracker allows developers to report bugs, suggest features, and track the progress of tasks within a project. Issues can be organized into different labels (like “bug,” “enhancement,” “feature”), assigned to specific team members, and prioritized based on urgency or importance. This system provides a clear overview of the project’s current state and allows for efficient task management. Projects can be further organized using GitHub’s project boards, allowing teams to visualize workflows using Kanban or other methods. For instance, a team could use a Kanban board to track the progress of issues through different stages, such as “To Do,” “In Progress,” and “Done,” providing a visual representation of their workflow.
GitHub’s Pull Request Review Process
The pull request is the heart of GitHub’s collaborative workflow. It’s a mechanism for proposing changes to a codebase. A developer creates a branch, makes their changes, and then submits a pull request to merge their branch into the main branch (often called `main` or `master`). Other developers can then review the code, provide feedback, and suggest improvements before the changes are merged. This process ensures code quality, reduces the risk of introducing bugs, and fosters knowledge sharing within the team. A well-written pull request includes a clear description of the changes made, making it easy for reviewers to understand the context and purpose of the modifications.
Step-by-Step Guide to Using GitHub’s Collaboration Tools
- Create an Issue: When a bug is found or a new feature is needed, create an issue describing the problem or desired functionality clearly and concisely. Assign it to a developer and add relevant labels.
- Create a Branch: Before making any code changes, create a new branch from the main branch. This isolates your changes and prevents disrupting the main codebase.
- Make Changes and Commit: Make the necessary code changes, commit them with descriptive messages explaining each change, and push the branch to your GitHub repository.
- Create a Pull Request: Submit a pull request to merge your branch into the main branch. This triggers the code review process.
- Review and Provide Feedback: Other developers review the code, provide feedback, and request changes if necessary. The pull request serves as a central place for discussion and collaboration.
- Merge the Pull Request: Once the code review is complete and all changes are approved, merge the pull request to integrate the changes into the main branch.
Writing Clear and Concise Pull Request Descriptions
A well-written pull request description is crucial for effective code review. It should clearly and concisely explain the purpose of the changes, the problem it solves, and the approach taken. Use bullet points to highlight key changes and link to relevant issues. For example:
Fixes issue #123: Improved performance of the search function by implementing a new caching mechanism. This change reduces the average search time by 50%.
* Implemented a new caching layer using Redis.
* Optimized database queries.
* Added unit tests to verify the functionality.
This structured approach makes it easy for reviewers to quickly understand the changes and focus on the most important aspects.
Final Conclusion
So, there you have it – your passport to the world of seamless code management! Mastering Git and GitHub isn’t just about technical skills; it’s about streamlining your workflow, fostering collaboration, and ultimately, building better software. Embrace the power of version control, and watch your productivity soar. Now go forth and conquer those codebases!