The Fundamentals of Web Development: A Complete Guide – sounds kinda boring, right? Wrong. Building websites isn’t just about lines of code; it’s about crafting digital experiences. This guide dives deep into the heart of web development, from the foundational HTML, CSS, and JavaScript to the server-side magic of databases and APIs. We’ll unravel the mysteries of front-end and back-end development, showing you how to build responsive, interactive, and secure websites. Get ready to level up your tech game.
We’ll explore the evolution of web technologies, the roles of different developers, and the tools that make the whole process tick. Think of it as your ultimate cheat sheet to becoming a web development ninja. We’ll cover everything from setting up your first server to deploying your masterpiece to the wild world of the internet. No prior experience? No problem. We’ll walk you through it step-by-step.
Introduction to Web Development
Web development, at its core, is the process of building and maintaining websites and web applications. It’s a multifaceted field encompassing a broad range of skills and technologies, all working together to create the interactive and dynamic online experiences we’ve come to expect. This involves everything from designing the visual layout and user interface to writing the code that powers the site’s functionality and manages data. Understanding the fundamental concepts is crucial for anyone looking to enter this exciting and ever-evolving field.
Web development isn’t a monolithic entity; it’s a collaborative effort involving various specialists. Different roles require different skill sets, and a successful project relies on the effective interaction between these roles.
Types of Web Developers and Their Roles, The Fundamentals of Web Development: A Complete Guide
The web development world is populated by diverse specialists, each contributing unique expertise. Front-end developers focus on the user interface, ensuring a visually appealing and user-friendly experience. Back-end developers handle the server-side logic, databases, and application programming interfaces (APIs). Full-stack developers possess proficiency in both front-end and back-end technologies, bridging the gap between the user interface and the server. Database administrators manage and maintain the databases that store website data, while UX/UI designers concentrate on user experience and interface design, focusing on the overall user journey and interaction. Finally, DevOps engineers bridge the gap between development and operations, automating deployment processes and ensuring system reliability.
A Brief History of Web Development Technologies
The evolution of web development technologies has been rapid and transformative. The early web, characterized by static HTML pages, has given way to dynamic, interactive applications powered by sophisticated frameworks and languages. The initial years saw the dominance of simple HTML, CSS, and JavaScript, forming the foundation of the web. The emergence of server-side scripting languages like PHP, ASP, and Python brought about dynamic content generation. The rise of databases like MySQL and PostgreSQL enabled efficient data management. More recently, the introduction of JavaScript frameworks like React, Angular, and Vue.js has revolutionized front-end development, enabling the creation of complex and responsive user interfaces. The development of cloud computing platforms like AWS, Azure, and Google Cloud has further impacted the way web applications are built and deployed. This continuous evolution reflects the constant push for enhanced performance, scalability, and user experience.
Comparison of Front-End and Back-End Development
The distinction between front-end and back-end development is crucial for understanding the overall architecture of a web application. Front-end development focuses on what the user sees and interacts with, while back-end development handles the underlying logic and data management.
Technology | Description | Front-end/Back-end | Example |
---|---|---|---|
HTML | Defines the structure and content of a web page. | Front-end |
|
CSS | Styles the visual presentation of a web page. | Front-end | body background-color: #f0f0f0; |
JavaScript | Adds interactivity and dynamic behavior to web pages. | Front-end (primarily), also Back-end (Node.js) | alert("Hello, world!"); |
Python | A versatile programming language used for back-end development. | Back-end | (Server-side code example omitted for brevity) |
PHP | A server-side scripting language commonly used for web development. | Back-end | (Server-side code example omitted for brevity) |
SQL | A language used for managing and manipulating databases. | Back-end | SELECT * FROM users; |
React | A JavaScript library for building user interfaces. | Front-end | (JSX code example omitted for brevity) |
Node.js | A JavaScript runtime environment for building server-side applications. | Back-end | (Server-side code example omitted for brevity) |
Front-End Development Fundamentals
Building awesome websites isn’t just about having cool ideas; it’s about bringing those ideas to life using the right tools. Front-end development is the art and science of making those websites look good and work seamlessly. This section dives into the fundamental building blocks: HTML, CSS, and JavaScript.
HTML: The Foundation of Your Webpage
HTML, or HyperText Markup Language, is the backbone of every website. Think of it as the skeleton – it provides the structure and organization for your content. It uses tags (like
for headings and
for paragraphs) to define elements on a page, arranging text, images, videos, and other content in a logical way. Without HTML, you’d just have a jumbled mess of code. It dictates the order and relationship between different parts of your webpage, ensuring a clear and understandable structure for both users and search engines.
CSS: Styling Your Webpage
While HTML provides the structure, CSS, or Cascading Style Sheets, is where the visual magic happens. CSS allows you to control the appearance of your webpage: colors, fonts, layouts, and more. Imagine it as the clothing – it dresses up the skeleton, making it visually appealing and consistent with your brand. You can style individual elements or apply styles across your entire website, ensuring a cohesive and professional look. Think of it as your website’s fashion designer.
JavaScript: Adding Interactivity and Dynamic Behavior
JavaScript brings your website to life by adding interactivity and dynamic behavior. It’s the brain – it allows you to create elements that respond to user actions, like clicking buttons, filling out forms, or scrolling through content. JavaScript allows for features such as animations, dynamic content updates, and interactive maps. Without JavaScript, your website would be static and less engaging. It’s the key ingredient to creating a truly immersive user experience.
A Simple Webpage Example
Let’s create a simple webpage using HTML, CSS, and JavaScript. This example will display a heading, a paragraph, and a button that changes the text of the paragraph when clicked.
“`html
Welcome to My Webpage!
This is a paragraph.
“`
Responsive Web Design Techniques
Responsive web design ensures your website looks great on all devices, from desktops to tablets to smartphones. This is crucial in today’s multi-device world. Here’s a snippet demonstrating the use of viewport meta tag and CSS media queries, essential tools for responsive design.
“`html
This is a responsive heading
This is a paragraph of text.
“`
This example shows how different styles can be applied based on screen size. The `@media` queries allow you to adjust the font sizes (and any other style) depending on the device’s width. The viewport meta tag ensures proper scaling on mobile devices. For instance, a website designed without responsiveness might display poorly on a small phone screen, while this approach ensures readability and usability regardless of the screen size.
Back-End Development Fundamentals
So, you’ve conquered the front-end – the flashy, user-facing part of the web. Now it’s time to dive into the engine room: the back-end. This is where the magic happens, the data is stored, and the logic that makes your website tick is executed. Think of it as the unseen architect behind the beautiful façade you built earlier. Let’s explore the core components.
The back-end is a complex ecosystem, but understanding its fundamental building blocks will empower you to create robust and dynamic web applications. This section will cover essential concepts and technologies that form the backbone of any successful back-end system.
Popular Server-Side Programming Languages
Server-side languages are the brains of the operation, processing data and interacting with databases. Python, PHP, and Node.js are among the most popular choices, each with its own strengths and weaknesses. Python, known for its readability and versatility, is often used for data science and machine learning integrations. PHP, a long-standing veteran, powers a significant portion of the web, known for its ease of use and large community support. Node.js, built on JavaScript, allows developers to use the same language on both the front-end and back-end, promoting efficiency and code reuse. The best choice depends heavily on project requirements and developer preference.
Database Systems: A Comparison
Databases are the heart of most back-end systems, storing and managing the application’s data. MySQL and PostgreSQL are relational databases, organizing data into tables with rows and columns, offering strong data integrity and well-established tools. MongoDB, on the other hand, is a NoSQL database, offering more flexibility and scalability for handling unstructured or semi-structured data. The choice between relational and NoSQL databases often depends on the nature of the data and the application’s specific needs. For instance, a social media platform might benefit from MongoDB’s flexibility in handling user-generated content, while an e-commerce site might prefer the structured approach of MySQL for managing product catalogs and inventory.
APIs: The Bridge Between Front-End and Back-End
APIs, or Application Programming Interfaces, are the communication channels between the front-end and back-end. They act as messengers, allowing the front-end to request data and functionality from the back-end and receive responses. For example, when you log into a website, the front-end sends your credentials via an API to the back-end, which then verifies your information and sends back a response indicating success or failure. APIs are crucial for creating modular and scalable web applications.
Common Back-End Frameworks
Frameworks provide pre-built structures and tools to streamline back-end development. Popular examples include Django (Python), Laravel (PHP), and Express.js (Node.js). These frameworks offer features like routing, database interaction, and security, significantly reducing development time and effort. Choosing the right framework depends on the chosen server-side language and project specifics. For instance, Django’s “batteries-included” approach is ideal for rapid development, while Express.js provides maximum flexibility for custom solutions.
Setting Up a Simple Back-End Server: A Step-by-Step Guide
Setting up a basic back-end server involves several steps: First, choose a server-side language (e.g., Node.js) and a framework (e.g., Express.js). Next, install the necessary dependencies using a package manager like npm or yarn. Then, create a simple server script that listens for requests on a specific port. Finally, test the server by sending requests using tools like Postman or curl. This process involves configuring the server to handle different HTTP methods (GET, POST, etc.) and responding accordingly. For example, a simple server might respond to a GET request with a “Hello, world!” message. More complex servers would interact with databases and other services to process data and fulfill user requests. This simple setup demonstrates the fundamental principles of back-end development, laying the groundwork for more complex applications.
Web Development Tools and Technologies: The Fundamentals Of Web Development: A Complete Guide
Building a website is like constructing a house – you need the right tools and materials. This section dives into the essential tools and technologies that power modern web development, from the code editors you use to the frameworks that structure your projects. Mastering these tools is crucial for efficiency and creating high-quality websites.
Code Editors and IDEs
Choosing the right code editor or Integrated Development Environment (IDE) is a personal preference, but certain features are universally beneficial. Code editors offer basic text editing with syntax highlighting, while IDEs provide more advanced features like debugging tools, version control integration, and intelligent code completion. Popular choices include VS Code (known for its extensive extensions and customization), Sublime Text (lightweight and fast), Atom (highly customizable and open-source), and WebStorm (a powerful IDE specifically designed for web development). Each offers a unique set of features catering to different workflows and preferences. The best choice depends on individual needs and project complexity.
Version Control Systems: Git
Git is the industry standard for version control. It allows developers to track changes to their code, collaborate effectively, and revert to previous versions if needed. Think of it as a time machine for your codebase. Using Git involves creating repositories, committing changes, branching for parallel development, and merging code from different branches. Popular platforms like GitHub, GitLab, and Bitbucket provide hosting for Git repositories, enabling collaboration and code sharing among developers. Mastering Git is essential for any serious web developer, ensuring efficient teamwork and a robust development process.
Debugging Tools and Techniques
Bugs are inevitable in software development. Debugging tools help identify and fix these errors. Browsers like Chrome and Firefox have built-in developer tools with powerful debugging capabilities, allowing you to step through code, inspect variables, and identify the source of errors. Techniques like console logging (printing messages to the browser’s console) and using breakpoints (pausing code execution at specific points) are invaluable for effective debugging. Learning effective debugging strategies saves time and prevents the release of buggy applications.
Web Development Frameworks and Libraries
Frameworks and libraries provide pre-built components and structures that streamline web development. Frameworks like React, Angular, and Vue.js (for front-end development) and Node.js, Django, and Ruby on Rails (for back-end development) offer structured approaches to building complex applications. Libraries like jQuery (for DOM manipulation) and Bootstrap (for responsive design) provide ready-made functionalities, accelerating development. Choosing the right framework or library depends on project requirements, team expertise, and performance considerations. Each offers unique advantages and trade-offs.
Testing Methodologies
Thorough testing is critical to ensure the quality and reliability of a web application. Different testing methodologies address various aspects of software quality.
Methodology | Description | Advantages | Disadvantages |
---|---|---|---|
Unit Testing | Testing individual components or modules of code in isolation. | Easy to implement, identifies bugs early. | Can miss integration issues. |
Integration Testing | Testing the interaction between different modules or components. | Reveals integration-related bugs. | Can be more complex to set up. |
System Testing | Testing the entire system as a whole. | Comprehensive testing of the final product. | Can be time-consuming and expensive. |
User Acceptance Testing (UAT) | Testing by end-users to ensure the system meets their requirements. | Validates user experience and requirements. | Relies on user availability and feedback. |
Web Development Workflow and Best Practices
Building a website isn’t just about slapping code together; it’s a carefully orchestrated process demanding planning, execution, and a healthy dose of best practices. Think of it like building a house – you wouldn’t start laying bricks without blueprints, would you? This section dives into the typical workflow and essential strategies for creating robust, efficient, and secure web applications.
A typical web development project unfolds in several key stages. Each phase is crucial, and skipping steps often leads to headaches down the line. From initial conception to deployment and maintenance, a well-defined workflow ensures a smoother, more successful project.
Project Planning and Design
Effective planning is paramount. This stage involves defining the project’s scope, identifying target users, and outlining the website’s functionality and features. Wireframing, a low-fidelity visual representation of the website’s layout, is a crucial tool here. Imagine sketching out the basic structure of each page – where the navigation bar goes, the placement of content blocks, and the overall flow. This helps to visualize the user experience before diving into coding. Following this, mockups, higher-fidelity visual representations, provide a more detailed look at the website’s design, incorporating visual elements and branding. A comprehensive project plan also includes timelines, resource allocation, and a clear definition of success metrics. For example, a project plan for an e-commerce website might include metrics like conversion rates and average order value, allowing for the measurement of project success beyond just completion.
Clean and Efficient Code
Writing clean, efficient, and maintainable code is essential for long-term success. This involves adhering to coding standards, using meaningful variable names, and employing consistent formatting. Proper code commenting, explaining the purpose of different code sections, is also vital for maintainability and collaboration. Imagine a future developer needing to understand and modify your code – clear comments act as signposts, guiding them through the codebase. Techniques like modularity, breaking down code into reusable components, and version control, using systems like Git to track changes and collaborate effectively, are also crucial. A real-world example would be a large e-commerce platform; maintaining and updating thousands of lines of code becomes nearly impossible without clean, well-documented code and version control.
Web Development Security Best Practices
Security should be a primary concern from the outset. Common vulnerabilities like SQL injection and cross-site scripting (XSS) can have devastating consequences. Employing secure coding practices, such as input validation and output encoding, is essential. Regular security audits and penetration testing can help identify and address potential weaknesses. Choosing strong, regularly updated passwords, using HTTPS to encrypt data transmitted between the browser and server, and implementing robust authentication and authorization mechanisms are all critical for building a secure web application. For instance, a banking website must prioritize security above all else to protect user financial data.
Common Web Development Challenges and Solutions
Web development isn’t always smooth sailing. Common challenges include debugging complex code, managing large projects effectively, and adapting to evolving technologies. Debugging tools, integrated development environments (IDEs), and collaborative platforms can help mitigate these issues. Effective project management methodologies, like Agile, break down projects into smaller, manageable sprints, facilitating better organization and adaptability. Staying updated on the latest technologies and best practices through continuous learning is also essential for addressing emerging challenges. For example, the rapid evolution of JavaScript frameworks requires developers to constantly adapt and learn new tools and techniques to stay competitive and build cutting-edge applications.
Deployment and Hosting
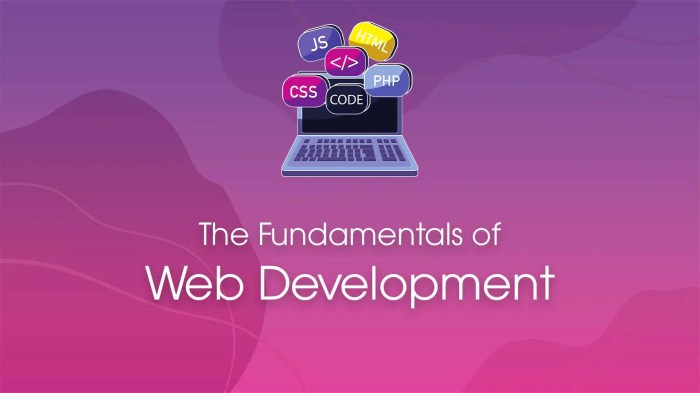
Source: theiku.com
So, you’ve built your awesome website! Now comes the exciting (and sometimes slightly terrifying) part: getting it live on the internet. This involves choosing a hosting provider and deploying your code – essentially, making your website accessible to the world. Let’s break down the key aspects of this crucial final step.
Web Hosting Options
Choosing the right web hosting plan is a bit like choosing a house – you need to consider your budget, needs, and future growth. Shared hosting is like living in an apartment building; you share resources with others, making it affordable but potentially less powerful. VPS (Virtual Private Server) is more like a townhouse – you have your own dedicated space but still share some infrastructure. Cloud hosting is the mansion – scalable, flexible, and powerful, but it comes with a higher price tag. The best option depends on your website’s traffic, complexity, and your technical expertise. A simple blog might thrive on shared hosting, while a high-traffic e-commerce site would need the robustness of cloud hosting or a VPS.
Deploying a Simple Website: A Step-by-Step Guide
Let’s assume you’ve built a basic static website using HTML, CSS, and JavaScript. Deploying this is relatively straightforward. First, you’ll need to choose a hosting provider (let’s say, for simplicity, a shared hosting provider). Then, you’ll typically use an FTP client (like FileZilla) to upload your website’s files (HTML, CSS, JavaScript, images, etc.) to the server’s designated directory. This directory is usually provided by your hosting provider during the account setup process. Once uploaded, your website should be accessible via your domain name (more on that below). Many hosting providers also offer one-click deployment options or intuitive control panels that simplify this process.
Choosing a Domain Name
Your domain name is your website’s address on the internet (e.g., www.example.com). Choosing a good one is crucial for branding and user experience. Keep it short, memorable, relevant to your website’s content, and easy to spell. Check for availability using domain registrars like GoDaddy or Namecheap. Consider using relevant s to improve search engine optimization (). Also, ensure the domain extension (.com, .org, .net, etc.) aligns with your website’s purpose. For example, a blog might use .com, while a non-profit organization might prefer .org.
Setting Up SSL Certificates
SSL (Secure Sockets Layer) certificates are essential for securing your website, especially if you handle sensitive information like user logins or credit card details. An SSL certificate encrypts the communication between your website and users, preventing eavesdropping and data breaches. Most hosting providers offer free SSL certificates through Let’s Encrypt, a non-profit certification authority. Setting up an SSL certificate usually involves installing it through your hosting provider’s control panel or using command-line tools. Once installed, your website’s address will change from “http://” to the more secure “https://”.
Website Deployment Checklist
Before launching your website, use this checklist to ensure a smooth process:
- Back up your website files and database.
- Test your website thoroughly on different devices and browsers.
- Choose a reliable hosting provider and plan.
- Obtain a domain name and point it to your hosting server.
- Install an SSL certificate to secure your website.
- Configure your website’s DNS records correctly.
- Monitor your website’s performance after launch.
Following this checklist minimizes potential problems and ensures a successful website launch. Remember, thorough testing is key to a successful deployment.
Advanced Web Development Concepts
So, you’ve mastered the basics of web development – congrats! But the world of web development is constantly evolving, and to truly build cutting-edge applications, you need to understand some more advanced concepts. This section dives into the exciting world of serverless architectures, containers, microservices, modern JavaScript frameworks, and the crucial roles of accessibility and . Get ready to level up your web dev game!
Serverless Architectures: A Paradigm Shift
Serverless computing is a cloud-based execution model where the cloud provider dynamically manages the allocation of computing resources. Instead of managing servers directly, developers focus on writing and deploying code as functions, triggered by events. This approach offers significant advantages, such as reduced operational overhead, improved scalability, and cost optimization. Imagine a website’s image upload feature: with serverless, the function only runs when an image is uploaded, eliminating the need to keep a server running constantly. This results in cost savings and efficient resource utilization. Major cloud providers like AWS (Lambda), Google Cloud (Cloud Functions), and Azure (Functions) offer robust serverless platforms.
Containerization with Docker
Docker is a leading containerization platform that packages applications and their dependencies into standardized units called containers. This ensures consistent execution across different environments (development, testing, production), simplifying deployment and reducing the “it works on my machine” problem. A Docker container bundles the application code, libraries, runtime, system tools, and settings, creating a self-contained unit that can be easily moved and deployed. This simplifies collaboration among developers and speeds up the deployment process. For instance, a developer working on a Node.js application can package the application and all its dependencies into a Docker container, ensuring that the application runs consistently on any machine with Docker installed.
Microservices Architecture
Microservices break down a large application into smaller, independent services that communicate with each other. Instead of a monolithic application, you have many smaller, specialized services working together. This modular approach enhances scalability, maintainability, and fault tolerance. If one service fails, the others can continue operating, ensuring application resilience. Netflix is a prime example; its massive platform is built on a microservices architecture, allowing for independent scaling and updates of individual components. Each microservice focuses on a specific business function, simplifying development and deployment.
Modern JavaScript Frameworks
The JavaScript landscape is dynamic, with several powerful frameworks simplifying front-end development. React, Angular, and Vue.js are prominent examples. React, known for its component-based architecture and virtual DOM, allows for efficient updates and rendering of user interfaces. Angular, a comprehensive framework, offers a structured approach to building complex applications. Vue.js, known for its ease of learning and flexibility, is a great option for both small and large projects. Choosing the right framework depends on project requirements and team expertise. Each framework offers a unique set of features and approaches to building user interfaces.
Accessibility and Best Practices
Accessibility and are critical for any successful website. Accessibility ensures that websites are usable by people with disabilities, adhering to guidelines like WCAG (Web Content Accessibility Guidelines). This includes providing alternative text for images, using appropriate heading structures, and ensuring sufficient color contrast. (Search Engine Optimization) involves optimizing websites to rank higher in search engine results. This includes using relevant s, optimizing website structure, and building high-quality content. Both accessibility and improve the user experience and broaden the reach of your website. Failing to address these aspects can significantly limit your website’s potential.
Closure
So, you’ve conquered the fundamentals. You’ve learned the languages, mastered the frameworks, and even tamed the dreaded debugging process. Now you’re armed with the knowledge to build amazing things. Remember, web development is a journey, not a destination. Keep exploring, keep learning, and most importantly, keep building. The internet awaits your creations! Go forth and code!