Building Responsive Websites with CSS Grid and Flexbox: Forget clunky, frustrating layouts! This isn’t your grandpappy’s web design. We’re diving deep into the power duo that’s revolutionizing how we build websites that look stunning on every device, from tiny smartphones to massive desktop monitors. Get ready to ditch the headaches and embrace the elegance of fluid, adaptable design.
This guide unravels the mysteries of CSS Grid and Flexbox, showing you how to harness their combined might to create responsive masterpieces. We’ll cover everything from the foundational concepts to advanced techniques, peppered with practical examples and real-world scenarios. Prepare to level up your web development game.
Introduction to Responsive Web Design
Let’s face it: nobody wants to squint at a website on their phone, or deal with a page that overflows off the screen. Responsive web design is the solution, ensuring your website looks great and functions flawlessly on any device, from tiny smartphones to massive desktop monitors. It’s not just about aesthetics; it’s about user experience, accessibility, and ultimately, success in today’s digital landscape.
Responsive web design is all about creating websites that adapt to different screen sizes and orientations. This means text reflows, images resize, and layouts adjust seamlessly to provide an optimal viewing experience regardless of the device used. The core principle is to design for flexibility, letting the content dictate the layout rather than forcing a rigid structure. This flexibility is crucial for reaching a wider audience and ensuring everyone has a positive experience interacting with your website.
The Benefits of CSS Grid and Flexbox for Responsive Layouts
CSS Grid and Flexbox are the powerhouses behind modern responsive design. Before their arrival, developers often relied on cumbersome techniques like media queries and floats, leading to complex and often fragile layouts. Grid and Flexbox provide a streamlined and efficient approach, making responsive design significantly easier and more manageable. Grid excels at creating complex two-dimensional layouts, while Flexbox is perfect for aligning and distributing items within a single dimension. Together, they form a powerful duo, allowing for the creation of incredibly flexible and responsive designs. For example, imagine a website showcasing a product portfolio. Using Grid, you can easily create a grid-based layout for showcasing multiple products. Then, using Flexbox, you can perfectly align the product images and descriptions within each grid cell, ensuring a clean and consistent presentation across all devices.
A Brief History of Responsive Design Techniques
The early days of web design saw websites built primarily for desktop computers. Adapting to smaller screens was a major challenge, often involving creating separate mobile versions of websites. This was inefficient and created maintenance headaches. The advent of media queries allowed developers to apply different styles based on screen size, but this approach still required a significant amount of manual tweaking. The introduction of CSS Grid and Flexbox in recent years revolutionized the field. These powerful tools provide a declarative and intuitive way to create flexible and responsive layouts, simplifying the development process and allowing for more creative and robust designs. The shift from cumbersome workarounds to the elegance of Grid and Flexbox represents a significant leap forward in responsive web design.
Understanding CSS Grid
CSS Grid is like a superhero for web layout. It’s a powerful tool that lets you create complex, responsive designs with ease, ditching the frustration of floats and flexbox limitations for larger layouts. Forget wrestling with divs; Grid gives you a clear, structured way to arrange website elements. Think of it as a master grid controlling the entire page, or sections within it.
CSS Grid uses a two-dimensional system of rows and columns to position items. Unlike Flexbox, which is best for one-dimensional layouts (either rows or columns), Grid excels at managing both simultaneously. This makes it perfect for building complex page layouts efficiently. It’s your secret weapon for creating sophisticated designs without the usual headaches.
Grid Fundamentals: Rows, Columns, and Areas
CSS Grid establishes a grid system through rows and columns, creating a framework for positioning items. These rows and columns form cells, the individual units within the grid. You can then explicitly place elements into specific cells, or let the grid automatically arrange them. The `grid-template-columns` and `grid-template-rows` properties define the structure, specifying the size and number of rows and columns. The `grid-gap` property adds spacing between grid items. Finally, named grid areas allow for more complex and flexible layout control. You can visualize this as a spreadsheet, where each cell is an element, and the rows and columns define the layout.
Creating a Basic Grid Layout
Let’s build a simple layout. First, you’ll need a container element to act as the grid. Then, using CSS, you define the grid structure. For example, to create a grid with three columns of equal width, you would use `grid-template-columns: repeat(3, 1fr);`. The `1fr` unit means “one fraction” – distributing available space equally among the columns. Similarly, `grid-template-rows` controls the row structure. Adding `grid-gap: 10px;` adds 10 pixels of space between the grid items.
Controlling Grid Structure with Properties
The power of CSS Grid lies in its properties. `grid-template-columns` and `grid-template-rows` are fundamental. You can specify column widths using pixel values (e.g., `grid-template-columns: 100px 200px auto;`), fractions (`1fr`, `2fr`), or percentages. Similarly, you can define row heights. `grid-gap` controls spacing between grid items, both horizontally and vertically. It significantly enhances visual organization and readability. Experimenting with these properties is key to mastering CSS Grid’s flexibility. For instance, `grid-template-columns: 100px repeat(2, 1fr);` creates a grid with a 100px wide column followed by two equally sized columns that share the remaining space.
Responsive 2-Column Layout with CSS Grid
Let’s craft a responsive two-column layout. This is where CSS Grid truly shines. We’ll use media queries to adjust the layout based on screen size.
“`html
“`
“`css
.container
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr)); /* Adjust minmax values as needed */
grid-gap: 10px;
.item
background-color: #f0f0f0;
padding: 20px;
@media (max-width: 600px)
.container
grid-template-columns: 1fr; /* Stack columns vertically on smaller screens */
“`
This code creates a grid that adapts to different screen sizes. On larger screens, it displays two columns. On smaller screens (below 600px), it stacks the items vertically, ensuring a seamless user experience on all devices. `repeat(auto-fit, minmax(250px, 1fr))` is crucial here, dynamically adjusting the number of columns based on available space.
Comparison with HTML Tables
While HTML tables might seem like a quick solution for layout, they are semantically incorrect for visual layout. Tables are designed for tabular data, not for arranging website elements. Using tables for layout leads to accessibility issues and makes maintaining the site much harder. CSS Grid provides a clean, semantic approach to creating layouts, improving accessibility and maintainability.
“`html
Item 1 | Item 2 |
“`
This table achieves a similar visual layout, but its semantic meaning is incorrect. It’s not presenting data in a table format; it’s simply arranging content. Using CSS Grid is the correct approach, leading to cleaner, more accessible, and maintainable code.
Mastering CSS Flexbox
Flexbox is your secret weapon for creating responsive layouts with ease. Forget wrestling with floats and positioning; Flexbox offers a streamlined, intuitive approach to arranging items in one dimension (either a row or a column). Think of it as the ultimate tool for aligning and distributing space within a container. Mastering Flexbox will unlock a whole new level of design flexibility for your websites.
At its core, Flexbox involves two key components: the flex container and its flex items. The flex container is the parent element, and you apply the flexbox properties to it. The flex items are the children of the container, automatically inheriting flexbox behavior. This parent-child relationship is crucial for understanding how Flexbox works.
Flexbox Properties: `flex-direction`, `justify-content`, and `align-items`
These three properties are your primary controls for manipulating the layout within a flex container. Understanding how they interact is key to achieving the desired arrangement of your flex items.
flex-direction
determines the direction in which flex items are placed. Common values include row
(default, items arranged horizontally), row-reverse
(items arranged horizontally in reverse order), column
(items arranged vertically), and column-reverse
(items arranged vertically in reverse order). Changing this property instantly alters the overall flow of your layout.
justify-content
controls the alignment of flex items along the main axis (the direction specified by flex-direction
). For example, space-between
distributes items evenly with space between them, center
centers the items, and flex-start
aligns them to the beginning of the container. This property is essential for distributing space effectively within the container.
align-items
controls the alignment of flex items along the cross axis (perpendicular to the main axis). center
vertically centers the items, flex-start
aligns them to the start of the cross axis, and stretch
(the default) stretches items to fill the container’s height. This is crucial for vertical alignment and controlling the height of your flex items.
Creating a Responsive Navigation Bar with Flexbox
Let’s build a simple navigation bar that adapts beautifully to different screen sizes. We’ll use a nav
element as our flex container and a
elements for our navigation links.
By setting display: flex;
on the nav
element, we activate Flexbox. We can then use justify-content: space-around;
to evenly distribute the navigation links. This creates a clean, balanced layout that works well on larger screens. For smaller screens, we might use media queries to adjust the flex-direction
to column
, stacking the links vertically for a more mobile-friendly experience. This ensures the navigation remains usable and visually appealing across various devices.
Comparing CSS Grid and Flexbox
Grid and Flexbox are both powerful layout tools, but they excel in different scenarios.
Choosing the right tool depends on your specific layout needs. Here’s a comparison:
- Flexbox: Ideal for one-dimensional layouts (rows or columns), aligning and distributing items within a single container. It’s excellent for navigation bars, cards, and other components where items need to be arranged along a single axis.
- Grid: Best suited for two-dimensional layouts, where you need to control both rows and columns simultaneously. It shines in complex page layouts, where you want precise control over the placement of multiple elements in a grid-like structure. Think website headers, footers, and main content areas.
For example, a hero section with an image and text overlay might be better suited to Grid, allowing precise control over the positioning of both elements. Conversely, a simple horizontal navigation bar is a perfect use case for Flexbox due to its ease of use in one-dimensional layouts.
Mastering responsive web design with CSS Grid and Flexbox is key to creating seamless user experiences across devices. Think about the scalability needed – it’s similar to how the insurance industry adapts, as highlighted in this insightful article on The Role of Insurance in the Sharing Economy , which addresses the complexities of risk management in dynamic platforms.
Ultimately, both require a flexible, adaptable framework to thrive in a rapidly evolving landscape.
Combining CSS Grid and Flexbox
Think of CSS Grid and Flexbox as a dynamic duo for web layout. While both are powerful tools for arranging elements, they excel in different situations. Using them together unleashes their full potential, creating flexible and responsive designs that adapt seamlessly to various screen sizes. It’s like having a master architect (Grid) planning the overall building structure and a skilled interior designer (Flexbox) meticulously arranging the furniture within each room.
Grid is best for laying out major page sections – think of the overall structure of your website. Flexbox, on the other hand, shines when arranging items *within* those sections – handling smaller, more detailed arrangements. This collaborative approach leads to cleaner, more maintainable code and ultimately, a superior user experience.
Scenarios Benefiting from Combined Grid and Flexbox
Combining Grid and Flexbox is particularly advantageous when building complex layouts requiring both a robust overall structure and precise internal arrangement of elements. For instance, consider a website with a main content area featuring multiple cards, a sidebar with navigation, and a header and footer. Grid excels at positioning the header, main content, and sidebar, while Flexbox expertly aligns and distributes the cards within the main content area and items within the sidebar. Another example is a multi-column layout where each column needs internal flexibility for different content lengths. Grid establishes the columns, and Flexbox ensures that content within each column adapts gracefully to varying lengths.
Example: Grid for Overall Layout, Flexbox for Sections
Let’s imagine a simple website layout with a main content area and a sidebar. We’ll use Grid to define the main structure and Flexbox to manage the arrangement of elements within the content area and sidebar.
HTML Structure for Grid and Flexbox Layout
This table illustrates the basic HTML structure:
Element | CSS Module | Description |
---|---|---|
<header> |
N/A (Simple styling) | Website header |
<main> |
CSS Grid | Contains the main content area and sidebar |
<section class="content"> |
CSS Flexbox | Main content area (e.g., articles, cards) |
<aside> |
CSS Flexbox | Sidebar with navigation or widgets |
<footer> |
N/A (Simple styling) | Website footer |
This setup allows for a clean separation of concerns, making the code easier to understand and maintain. The Grid manages the two main columns (content and sidebar), while Flexbox handles the arrangement of items within each column.
Responsive Card Layout with Grid and Flexbox
Imagine a section displaying a series of product cards. We can use Grid to create the overall layout of the cards (e.g., two cards per row on larger screens, one per row on smaller screens), and Flexbox to control the alignment and spacing of elements *within* each card (like the image, title, and description). This approach ensures that the cards remain neatly arranged and responsive across different screen sizes, providing a visually appealing and user-friendly experience. For example, on a larger screen, the Grid could arrange cards in a 2-column layout. On a smaller screen, it could switch to a 1-column layout, while Flexbox within each card maintains consistent internal alignment.
Handling Different Screen Sizes and Devices
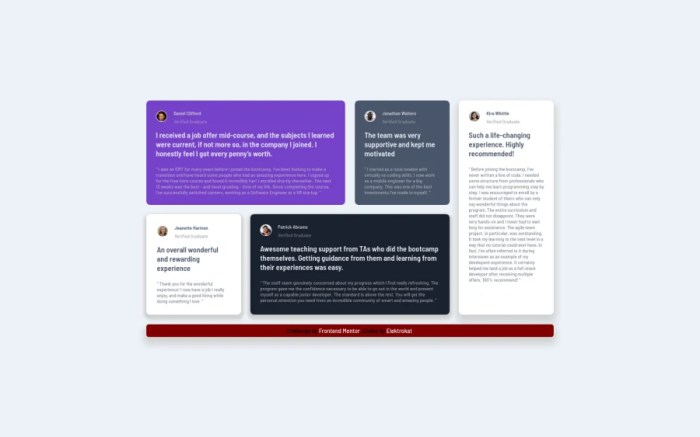
Source: cloudinary.com
Building a truly responsive website means ensuring it looks fantastic on everything from tiny smartphone screens to massive desktop monitors. This isn’t about creating separate websites; it’s about using clever CSS techniques to adapt a single design to fit any viewport. The key player in this responsive magic show? Media queries.
Media queries are like conditional statements for your CSS. They allow you to apply different styles based on the characteristics of the device viewing the page, such as screen width, height, orientation, and even resolution. This gives you complete control over how your layout adjusts to different screen sizes. Think of it as having multiple blueprints for your website, each tailored to a specific device type.
Media Queries for Adaptive Layouts
Media queries work by using the `@media` rule, followed by a condition that specifies the target device characteristics. For example, `@media (max-width: 768px)` applies styles only when the screen width is 768 pixels or less – perfect for targeting tablets and smaller devices. You can combine multiple conditions, such as `@media (min-width: 1024px) and (orientation: landscape)`, to target specific scenarios. Within the media query, you define the CSS rules that should be applied when the condition is met. This allows you to create entirely different layouts for desktops, tablets, and mobile devices without writing separate stylesheets. For instance, you might use a single-column layout on mobile, a two-column layout on tablets, and a three-column layout on desktops, all controlled by different media queries.
Creating Layouts for Different Devices
Let’s imagine a simple three-column layout. On desktop, we want three columns side-by-side. On tablets, we want two columns, and on mobile, a single column. We’d achieve this with a series of media queries:
`/* Desktop: Three columns */`
`@media (min-width: 1024px) `
` .container display: grid; grid-template-columns: repeat(3, 1fr); `
“
`/* Tablet: Two columns */`
`@media (min-width: 768px) and (max-width: 1023px) `
` .container display: grid; grid-template-columns: repeat(2, 1fr); `
“
`/* Mobile: One column */`
`@media (max-width: 767px) `
` .container display: grid; grid-template-columns: 1fr; `
“
This example uses CSS Grid for layout, but you could achieve the same result with Flexbox. The key is the use of media queries to switch between different grid or flexbox configurations based on screen size.
Organizing Media Queries for Responsive Design
A well-structured responsive design utilizes a cascading approach to media queries. Start with styles for the largest screen size (desktop), then progressively add media queries for smaller screen sizes, overriding styles as needed. This ensures that styles are applied in the correct order, avoiding conflicts and maintaining consistency. It’s also good practice to use clear and descriptive breakpoint values, making your CSS easy to understand and maintain. For instance, instead of using arbitrary numbers, use meaningful names like `$desktop`, `$tablet`, and `$mobile`, defined as variables to keep things consistent.
Creating a Responsive Image Gallery
Building a responsive image gallery involves ensuring images scale appropriately without distorting their aspect ratio and adjust their layout to fit the available space.
Here’s how to create one:
- Use the `max-width: 100%` rule: This ensures images never exceed their container’s width, preventing them from overflowing. Combine this with `height: auto` to maintain the aspect ratio.
- Employ CSS Grid or Flexbox: Use a grid or flexbox layout to arrange the images responsively. This allows for flexible arrangements across different screen sizes. You can adjust the number of columns based on screen size using media queries.
- Consider using the `object-fit` property: This allows you to control how images are displayed within their container. For example, `object-fit: cover` will ensure the image covers the entire container, while `object-fit: contain` will maintain the aspect ratio while fitting within the container.
- Implement lazy loading: This technique only loads images when they are visible in the viewport, improving page load times, especially on mobile devices. This is usually achieved with JavaScript libraries or attributes like `loading=”lazy”`.
Advanced Techniques and Best Practices
Level up your responsive web design game by mastering advanced techniques and best practices. This isn’t just about making websites look good on different screens; it’s about building them efficiently, accessibly, and sustainably. We’ll delve into strategies that’ll transform your CSS skills from good to great, ensuring your websites are not only beautiful but also performant and future-proof.
Building truly robust and scalable responsive websites requires more than just a basic understanding of Grid and Flexbox. It involves strategic planning, efficient coding, and a deep appreciation for the underlying principles of web development. This section will equip you with the knowledge to tackle complex layouts and optimize your workflow for maximum efficiency.
Complex Layout Handling
Complex layouts often require a nuanced approach, leveraging the strengths of both CSS Grid and Flexbox in tandem. Imagine designing a website with a three-column layout, where the sidebar is fixed-width, the main content area is flexible, and a right-hand column adjusts to available space. Grid excels at defining the overall structure (three columns), while Flexbox allows for fine-grained control over the distribution of space within each column, ensuring responsiveness across various screen sizes. You might use Grid for the primary layout and then apply Flexbox to individual grid items for more detailed arrangement. For instance, the main content area could use Flexbox to arrange cards or articles in a responsive manner.
Efficient and Maintainable CSS
Writing clean, well-organized CSS is paramount for maintainability. This means adhering to a consistent naming convention, using a CSS methodology like BEM (Block, Element, Modifier), and avoiding overly specific selectors. Employing CSS preprocessors like Sass or Less can enhance organization and readability through features like nesting, variables, and mixins. This will improve your workflow and make it easier to update and maintain your stylesheets as your project evolves. Imagine trying to debug a huge, unorganized CSS file versus a well-structured one – the difference is night and day!
Semantic HTML for Accessibility and
Semantic HTML uses tags that clearly describe the purpose of content, not just its visual appearance. Instead of using divs for everything, leverage tags like `