Building Dynamic Websites with JavaScript and React: Dive into the electrifying world of interactive web experiences! Forget static pages; we’re talking about websites that breathe, react, and adapt to user input. This isn’t your grandma’s website—we’re building the future of the web, one component at a time. Get ready to unleash your inner web wizard and conjure up dynamic masterpieces.
This guide walks you through the essentials of building dynamic websites using the power of JavaScript and React. From setting up your development environment to mastering advanced concepts like state management and routing, we’ll cover everything you need to know to create truly engaging and interactive web applications. Prepare for a coding adventure filled with practical examples and insightful tips to help you level up your web development game.
Introduction to Dynamic Websites: Building Dynamic Websites With JavaScript And React
Forget those static, unchanging websites of yesteryear. Dynamic websites are the vibrant, interactive online experiences we all know and love. They adapt and change based on user input, server-side data, and even real-time events, creating a personalized and engaging online journey. This interactivity is what sets them apart, offering a far richer user experience than their static counterparts.
Dynamic websites offer a significant advantage over static ones. Static websites, essentially just collections of HTML files, deliver the same content to every visitor. Dynamic websites, on the other hand, leverage server-side scripting and databases to create unique content tailored to each user’s interaction. This results in a more engaging, personalized, and ultimately, more successful online presence. Think about it – personalized recommendations, user accounts, and interactive forms are all hallmarks of the dynamic web.
Examples of Dynamic Websites and Their Functionalities
Many popular websites exemplify the power of dynamic content. Consider Amazon. Its product catalog, user reviews, shopping cart, and personalized recommendations are all dynamically generated. The site isn’t simply displaying a pre-written page; it’s constantly updating and changing based on user actions and available data. Similarly, Facebook’s newsfeed, tailored to each user’s connections and interests, is a prime example of dynamic content delivery. The constant stream of updates, posts, and interactions wouldn’t be possible without dynamic website technologies. Even something as seemingly simple as a contact form on a website is a dynamic element; it processes user input and sends it to an email address.
The Role of JavaScript and React in Building Dynamic Websites
JavaScript is the backbone of dynamic web interactions. It allows for client-side scripting, meaning code runs directly in the user’s web browser, enabling interactive elements like animations, form validation, and real-time updates without requiring a page reload. React, a popular JavaScript library, takes this dynamism a step further. It allows developers to build complex user interfaces (UIs) efficiently and effectively. React’s component-based architecture promotes code reusability and simplifies the management of large and complex web applications. By breaking down the UI into smaller, manageable components, React makes it easier to build, update, and maintain dynamic websites. Imagine building a large website like Amazon without a framework like React; the complexity would be astronomical. React simplifies the process significantly, enabling the creation of highly interactive and responsive web experiences.
Setting up the Development Environment
So you’re ready to dive into the electrifying world of React? Awesome! But before you start crafting those dazzling user interfaces, you’ll need a solid development environment. Think of it as your digital workshop – properly set up, it’ll make your coding journey smooth sailing. A poorly configured environment, on the other hand? Let’s just say it’s like trying to build a skyscraper with a rusty spoon.
Setting up your React development environment involves installing essential tools and configuring them to work together seamlessly. This process might seem daunting at first, but we’ll break it down step-by-step, making it easier than ordering your favorite takeout.
A Step-by-Step Guide to Setting up a React Development Environment
First, ensure you have Node.js and npm (or yarn) installed. Node.js is the runtime environment, and npm (Node Package Manager) or yarn is your package manager – your trusty sidekick for installing and managing all the necessary libraries. Download the latest versions from the official Node.js website. Once installed, open your terminal and type `node -v` and `npm -v` (or `yarn -v`) to verify the installations. Next, you’ll create your React project using Create React App (CRA), a tool that sets up a modern development environment with all the bells and whistles. Open your terminal, navigate to your desired project directory, and run `npx create-react-app my-react-app` (replace `my-react-app` with your project’s name). This command will download and install all the necessary dependencies and create the basic project structure for you. After the installation completes, navigate into the project directory using `cd my-react-app` and start the development server using `npm start`. This will open your project in your default browser, and you’re ready to start coding!
Essential Tools and Libraries for React Development
Choosing the right tools is crucial for efficient development. Here’s a breakdown of the essentials, presented in a handy table:
Tool | Description | Installation | Example Usage |
---|---|---|---|
Node.js | JavaScript runtime environment. | Download from nodejs.org | Runs JavaScript code outside of a browser. |
npm (or yarn) | Package manager for Node.js. | Comes bundled with Node.js (or install separately using your system’s package manager). | npm install react react-dom |
Create React App (CRA) | Simplifies React project setup. | npx create-react-app my-app |
Generates a boilerplate React project. |
Visual Studio Code (VS Code) or similar IDE | Code editor with React support (extensions available). | Download from code.visualstudio.com | Provides syntax highlighting, autocompletion, debugging tools. |
React Developer Tools | Browser extension for inspecting React components. | Available in Chrome Web Store and Firefox Add-ons. | Inspect component hierarchy, props, and state. |
Simple Project Structure for a React Application
A well-organized project structure is key to maintainability. While CRA provides a solid foundation, understanding the structure is essential for future projects. A typical structure includes folders for components, styling (CSS or other styling solutions), and potentially separate folders for utilities or other specific functionalities.
A well-structured project makes scaling and collaboration a breeze.
For example, a simple project might look like this:
“`
my-react-app/
├── public/
│ └── index.html
├── src/
│ ├── App.js
│ ├── components/
│ │ ├── Header.js
│ │ └── Footer.js
│ ├── styles/
│ │ └── App.css
│ └── index.js
└── package.json
“`
This structure keeps things neat and logical, making it easy to find and manage your code as your project grows. Remember, consistency is key!
Core Concepts of React
So, you’ve got your dev environment set up and you’re ready to dive into the wild world of React? Awesome! Before you start building the next Instagram clone, let’s lay down some fundamental concepts. Think of this as your React survival guide – essential knowledge for navigating the React jungle.
React’s magic lies in its component-based architecture. It’s like building with LEGOs, but instead of bricks, you have reusable pieces of code that represent parts of your user interface. This modular approach makes building, maintaining, and scaling complex applications a breeze. We’ll explore the different types of components and how they interact to create dynamic and responsive web experiences. Get ready to become a React component ninja!
Components in React
Components are the fundamental building blocks of any React application. They are independent, reusable pieces of code that encapsulate specific UI elements and their logic. This modularity promotes code reusability, maintainability, and organization. Imagine building a house – you wouldn’t build the entire thing from scratch each time, right? You’d use pre-fabricated components like doors, windows, and walls. React components work similarly, allowing you to build complex UIs from smaller, manageable parts. There are two main types: functional components and class components.
Functional Components
Functional components are the simplest form of React components. They are essentially JavaScript functions that accept props (properties) as input and return a JSX element representing the UI. They are often preferred for their simplicity and readability, especially for smaller, less complex components. For example, a simple functional component to display a greeting might look like this:
“`javascript
function Greeting(props)
return ;
“`
This component takes a `name` prop and renders a greeting message. It’s concise, easy to understand, and highly reusable.
Class Components, Building Dynamic Websites with JavaScript and React
Class components offer more advanced features, such as internal state management and lifecycle methods. They are defined using ES6 classes and extend the `React.Component` class. While functional components with hooks are now the more common approach, understanding class components provides valuable context for understanding React’s evolution. A class component example:
“`javascript
class Counter extends React.Component
constructor(props)
super(props);
this.state = count: 0 ;
render()
return (
You clicked this.state.count times
);
“`
This component maintains an internal state (`count`) and updates it when the button is clicked. This demonstrates the power of class components for managing dynamic UI updates.
JSX Syntax
JSX (JavaScript XML) is a syntax extension to JavaScript that allows you to write HTML-like code within your JavaScript files. It makes writing React components more intuitive and readable. Think of it as a bridge between JavaScript logic and the HTML structure of your UI. JSX isn’t actually JavaScript, but it gets compiled into plain JavaScript before your browser runs it. It allows you to embed JavaScript expressions within your HTML, making dynamic content updates straightforward. For instance:
“`javascript
const name = “Hipwee”;
const element =
Hello, name!
;
“`
This JSX code will render “Hello, Hipwee!” to the screen. The `name` part is a JavaScript expression embedded within the JSX, dynamically injecting the value of the `name` variable. JSX significantly improves the developer experience when building React applications, making it easier to write and maintain complex UI structures.
Handling User Interactions
Making your React website dynamic isn’t just about pretty visuals; it’s about responsiveness. Users expect their actions – clicks, hovers, form submissions – to trigger changes, and that’s where handling user interactions comes in. This involves capturing events, updating your component’s internal state, and reflecting those changes in the user interface. Think of it as the choreography behind a website’s responsiveness.
React provides elegant mechanisms for managing this. We’ll explore how to use event handlers to capture user actions, how to update the component’s state to reflect those actions, and how to leverage this state to dynamically update the UI. The key is to understand the flow: event triggers state change, state change updates the UI.
Event Handling in React Components
React uses a synthetic event system. This means that when a user interacts with a component (e.g., clicks a button), React creates a synthetic event object. You can then use event handlers – functions that are triggered by these events – to capture and process this information. These handlers are attached directly to the JSX elements using attributes like `onClick`, `onMouseOver`, `onSubmit`, etc. For example, an `onClick` handler on a button will execute a function whenever the button is clicked. The function can then perform actions such as updating the component’s state or making API calls. This approach allows for clean, declarative event handling within the component’s structure.
Managing Component State and UI Updates
The `useState` hook is fundamental to managing component state in functional components. It allows you to declare a state variable and a function to update it. Whenever the state changes, React automatically re-renders the component, updating the UI to reflect the new state. The key is to call the state update function (usually named something like `setState`) within your event handlers. This ensures that user interactions directly influence the component’s state and trigger UI updates. For instance, a counter component might increment its state whenever a button is clicked, leading to a visual update of the counter’s value.
A Simple Form Component
Let’s create a simple form to illustrate these concepts. Imagine a form that collects a user’s name. We’ll use a controlled component approach, where the form’s input values are directly managed by the component’s state. This provides a more predictable and manageable way to handle form submissions.
“`javascript
import React, useState from ‘react’;
function NameForm()
const [name, setName] = useState(”);
const handleSubmit = (event) =>
event.preventDefault();
alert(‘A name was submitted: ‘ + name);
;
return (
);
export default NameForm;
“`
This code defines a `NameForm` component that uses `useState` to manage the `name` state. The `onChange` event handler updates the `name` state whenever the input field changes. The `onSubmit` handler prevents the default form submission behavior and alerts the submitted name. This example showcases how to connect user input directly to the component’s state, resulting in a dynamic form experience. The `value` attribute in the input ensures that the input field always reflects the current state of `name`, creating a controlled component.
Data Fetching and Display
So, you’ve built your React app’s foundation – now it’s time to make it *dynamic*. This means bringing in data from the outside world, breathing life into your static components. We’re talking about fetching data from APIs, the lifeblood of modern web apps. Get ready to transform your app from a pretty face into a data-driven powerhouse!
Fetching data from APIs is like ordering your favorite takeout – you send a request, and you get a delicious response (hopefully!). But unlike takeout, you need to handle this asynchronous process carefully. React provides powerful tools to manage this, ensuring your app remains smooth and responsive even while waiting for data to arrive.
Fetching Data with `fetch` and Axios
The `fetch` API is a built-in browser feature that’s become a standard for making network requests. It’s straightforward and easy to use, making it a great starting point. Axios, on the other hand, is a popular third-party library that offers additional features like automatic JSON transformation and request interception. Both accomplish the same goal: retrieving data from a server. The choice often comes down to personal preference and the specific needs of your project. For simpler projects, `fetch` might suffice; for larger, more complex apps, Axios’s extra features can be invaluable.
Handling Asynchronous Operations
Because network requests take time, we need to handle them asynchronously. This means our app doesn’t freeze while waiting for the data. In React, we use asynchronous JavaScript functions (async/await) along with state management to handle this gracefully. The `useState` hook helps manage the loading state, the fetched data, and any potential errors. This way, you can display a loading indicator while fetching data, and handle errors smoothly without crashing the app. Think of it as a polite “please wait” message while your app fetches the necessary information.
Displaying Fetched Data
Once the data arrives, the next step is to display it beautifully in your user interface. React’s component-based architecture makes this easy. You can use the fetched data to dynamically populate your components, creating a truly interactive and responsive experience. Imagine updating a product list in real-time based on new inventory or showing personalized recommendations based on user data – that’s the power of dynamic data display.
Example: Fetching and Displaying Data from a Public API
Let’s build a simple component that fetches data from a public API (like a JSONPlaceholder API) and displays it in an unordered list.
First, we’ll define a component that uses the `useEffect` hook to fetch data when the component mounts. This hook allows us to perform side effects (like API calls) after the component renders. We’ll also use `useState` to manage the loading state and the data itself. Error handling is crucial; we’ll use a `try…catch` block to manage potential network issues.
Inside the `useEffect` hook, we make a `fetch` request to the API endpoint. The response is then converted to JSON using `response.json()`. The fetched data is then updated in the component’s state. If there’s an error, we’ll update the error state appropriately.
Finally, we’ll conditionally render the content based on the loading and error states. If loading, we display a loading message. If an error occurs, we display the error message. Otherwise, we display the fetched data in an unordered list.
Building dynamic websites with JavaScript and React offers incredible flexibility; you can craft interactive interfaces for anything, even niche markets like student health insurance. Check out this report on Exploring the Growing Market for Student Health Insurance to see how data visualization, a key strength of React, could be applied. Ultimately, mastering these frameworks allows developers to build solutions for diverse and evolving needs.
function DataList()
const [data, setData] = useState([]);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() =>
const fetchData = async () =>
try
const response = await fetch('https://jsonplaceholder.typicode.com/todos');
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
const jsonData = await response.json();
setData(jsonData);
catch (err)
setError(err);
finally
setLoading(false);
;
fetchData();
, []);
if (loading)
return
Loading...
;
if (error)
return
Error: error.message
;
return (
-
data.map(item => (
- item.title
))
);
Managing Component State
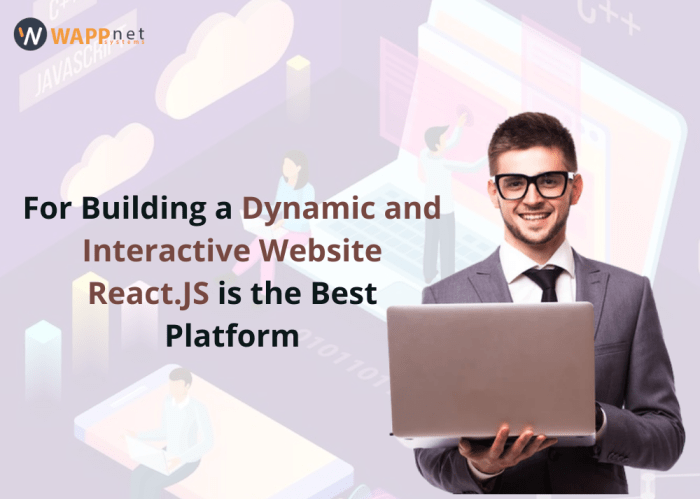
Source: wappnet.com
So, you’ve built some cool React components, but they’re feeling a little… static. Like a website stuck in the 90s. Time to inject some dynamism! Managing component state is the key to building interactive and responsive React applications. It’s about how your components remember and update data, influencing what users see and how they interact with your website. Think of it as the app’s memory, constantly changing based on user actions and external data. Getting this right is crucial for a smooth and enjoyable user experience.
Choosing the right state management solution depends on the complexity of your app. For small projects, a simple solution might suffice, but as your app grows, you might need a more robust approach. Let’s explore the most common options.
React State Management Solutions Comparison
This table compares three popular React state management solutions: `useState`, `useReducer`, and the Context API. Each offers a different approach to handling data, and understanding their strengths and weaknesses will help you pick the best tool for the job.
State Management Solution | Description | Advantages | Disadvantages |
---|---|---|---|
useState |
A Hook that lets you add state to functional components. It’s simple and perfect for managing small amounts of data. It uses a simple array destructuring pattern to access and update state. For example: const [count, setCount] = useState(0); |
Easy to learn and use; ideal for simple state management; minimal boilerplate. | Can become unwieldy for complex state logic; not suitable for sharing state across many components; state updates can be difficult to track in large applications. |
useReducer |
Another Hook, but it’s better suited for managing more complex state logic. It uses a reducer function to update the state, allowing for cleaner state updates and easier debugging. Think of it as a more structured approach to state updates, often used when state updates depend on the previous state. | Improved code organization for complex state; easier to debug; promotes better separation of concerns. | Steeper learning curve than useState ; adds more complexity for simple state scenarios. |
Context API | Provides a way to pass data through the component tree without having to pass props down manually at every level. This is particularly useful for global state that needs to be accessed by many components. | Efficiently shares state across components; avoids prop drilling; simplifies state management in large applications. | Can be overused; might make debugging more challenging if not implemented carefully; adds complexity to the application. |
Routing and Navigation
So, you’ve built some awesome React components, displaying dynamic data and handling user interactions like a pro. But what happens when your app grows beyond a single page? That’s where routing comes in – the secret sauce that lets you create multi-page experiences within a single-page application (SPA). Think of it as the navigational system of your website, seamlessly guiding users between different sections without the need for full page reloads.
Routing in SPAs is crucial because it allows for a much smoother and more responsive user experience. Instead of the jarring page refreshes of traditional websites, users navigate between different views within the same application, making the experience feel much more fluid and modern. This is achieved by dynamically updating the content of the page based on the URL, providing a seamless transition between different parts of the application. This also contributes to improved performance, as only the necessary parts of the application are updated, rather than the entire page.
React Router Implementation
React Router is the go-to library for handling routing in React applications. It provides a declarative way to define routes and map them to specific components. Essentially, you tell React Router which component should be rendered based on the URL. This involves installing the library (`npm install react-router-dom`) and then using its components like `
For example, you might have a route `/home` that renders a `Home` component, a route `/about` that renders an `About` component, and a route `/contact` that renders a `Contact` component. React Router handles the behind-the-scenes magic of updating the view based on the URL, making it easy for developers to manage complex navigation within their applications.
Designing a Simple Navigation System
Let’s craft a basic navigation system. Imagine a website with three pages: Home, About, and Contact. We’ll use React Router to link these pages together.
First, you would wrap your application with `
For instance, a `` component would render a clickable link that, when clicked, updates the URL to `/about` and renders the corresponding `About` component. This allows for a clean and intuitive navigation experience without requiring page reloads. The user experience is enhanced by the seamless transition between pages, creating a more engaging and modern feel. This approach is also beneficial for purposes as search engines can easily crawl and index the content of different routes within the SPA.
Advanced React Concepts
So, you’ve mastered the basics of React—congrats! But the React universe extends far beyond components and state. This section dives into some more advanced techniques that’ll supercharge your React development skills and help you build truly robust and scalable applications. We’ll explore Higher-Order Components (HOCs), Render Props, and the power of React Hooks. Get ready to level up your React game!
These advanced concepts offer powerful ways to reuse logic, manage complexity, and write cleaner, more maintainable React code. They’re essential tools for building large-scale applications where code organization and reusability become paramount.
Higher-Order Components (HOCs) and Render Props
Higher-Order Components are functions that take a component as an argument and return a new enhanced component. Render props, on the other hand, utilize a prop to inject rendering logic into a component. Both patterns offer ways to compose and reuse functionality across multiple components without resorting to inheritance, keeping your codebase DRY (Don’t Repeat Yourself) and easier to maintain.
Imagine you need to add authentication or logging capabilities to several components. Instead of repeating the same logic in each component, you can create an HOC that handles authentication and wraps your existing components. Similarly, a render prop allows you to pass a rendering function to a component, letting that component determine how data is displayed, offering a flexible and powerful alternative to HOCs in certain situations.
Consider a scenario where you have multiple components that need to fetch data from an API. An HOC could handle the fetching logic, providing the fetched data as props to the wrapped component. This keeps the data fetching logic separate and reusable, leading to cleaner and more organized code. Conversely, a render prop could be used to pass a rendering function to a component that handles the API call, allowing for customized rendering based on the fetched data.
React Hooks for Managing State and Side Effects
React Hooks are functions that let you “hook into” React state and lifecycle features from within functional components. Before Hooks, state management in functional components was limited, often requiring class components. Hooks changed that, enabling cleaner and more concise code by providing access to state, side effects, and more directly within functional components.
Hooks like useState
allow you to manage component state within functional components, while useEffect
lets you perform side effects like data fetching or subscriptions. This eliminates the need for class components in many cases, leading to simpler, more readable code. For instance, using useState
, you can easily manage the state of a form or a counter within a functional component, simplifying the code significantly compared to managing state in a class component.
The useEffect
hook allows you to run side effects after a render. This is particularly useful for fetching data, subscribing to events, or cleaning up after a component unmounts. For example, you could use useEffect
to fetch data from an API when a component mounts, and then clean up the subscription when the component unmounts, preventing memory leaks. The use of dependencies array in useEffect
ensures that the effect runs only when specific values change.
Testing React Applications
Building robust and reliable React applications isn’t just about crafting beautiful UIs; it demands a rigorous testing strategy. Think of testing as your safety net, catching bugs before they crash your app and frustrate your users. Without proper testing, you’re essentially building on quicksand – a seemingly stable foundation that could collapse at any moment.
Testing in React development ensures that your components behave as expected, your data flows correctly, and your user experience remains seamless. It’s a crucial step in the development lifecycle, minimizing the risk of unexpected errors and ensuring a high-quality product. This isn’t just about catching minor glitches; thorough testing prevents major headaches down the line, saving you time, money, and a whole lot of frustration.
Unit Testing
Unit testing focuses on the smallest testable parts of your application – individual components or functions. The goal is to verify that each unit works correctly in isolation. This allows you to pinpoint the source of errors quickly and efficiently. For instance, you might test a button component to ensure it correctly updates the application state when clicked, without worrying about how other parts of the application behave. This approach allows for rapid feedback and easier debugging.
Integration Testing
Integration testing takes a step further, examining how different units work together. It verifies that components interact correctly and that data flows smoothly between them. Imagine testing a login form: unit tests might verify individual input fields, while integration tests would check that the entire form submits data correctly to the backend. This ensures that the different parts of your application are compatible and work seamlessly as a whole.
End-to-End Testing
End-to-end (E2E) testing simulates a real user’s journey through your application. It covers the entire application flow, from start to finish, testing the interaction between the front-end, back-end, and any external services. Think of it as a complete system test. For example, an E2E test might verify that a user can successfully register an account, log in, and then make a purchase. This approach ensures that your application functions correctly as a complete system.
Testing Frameworks and Libraries
Several powerful tools are available to streamline the testing process in React. Choosing the right one depends on your project’s needs and complexity.
Commonly used frameworks include:
- Jest: A widely adopted JavaScript testing framework known for its ease of use and speed. It’s often bundled with Create React App, making it a convenient choice for many React developers. Jest offers features like mocking, snapshot testing, and code coverage reporting.
- React Testing Library: This library focuses on testing components from the user’s perspective, making your tests more reliable and less prone to breaking when the implementation details change. It encourages testing against the user interface rather than the internal implementation.
- Enzyme: Another popular testing utility, Enzyme provides helpful utilities for traversing and manipulating the React component tree. While powerful, it’s worth noting that its development has slowed, with React Testing Library often being preferred for its user-centric approach.
Deployment of React Applications
So, you’ve built your awesome React app. Congratulations! Now comes the exciting (and sometimes slightly terrifying) part: getting it live on the internet. Deployment might sound daunting, but with the right tools and a bit of know-how, it’s a manageable process. This section will walk you through several popular options and help you choose the best fit for your project.
Deploying your React application involves transferring your built code to a server where it can be accessed by users. This process typically involves building your application (creating optimized files for production), then uploading these files to a hosting provider. The choice of hosting provider will depend on factors like your budget, technical expertise, and the specific needs of your application.
Deployment Platforms: Netlify, Vercel, and AWS
Choosing the right platform for deploying your React app depends on your priorities. Netlify, Vercel, and AWS Amplify are three popular choices, each with its own strengths and weaknesses.
- Netlify: Known for its ease of use and seamless integration with Git repositories like GitHub and GitLab. Netlify excels at providing a straightforward deployment workflow, often requiring minimal configuration. It’s a great choice for beginners and projects that don’t require extensive server-side customization.
- Vercel: Similar to Netlify in its ease of use and Git integration, Vercel is particularly popular within the React community due to its strong support for Next.js, a popular React framework. Vercel often boasts impressive performance and scalability.
- AWS Amplify: Part of the broader Amazon Web Services ecosystem, Amplify provides a more comprehensive solution for deploying and managing applications. While it offers greater flexibility and control, it comes with a steeper learning curve and potentially higher costs compared to Netlify and Vercel. Amplify is ideal for larger, more complex projects or when you need tight integration with other AWS services.
Comparison of Deployment Options
Here’s a quick comparison to help you decide:
Feature | Netlify | Vercel | AWS Amplify |
---|---|---|---|
Ease of Use | Excellent | Excellent | Good |
Cost | Free tier available, scales up | Free tier available, scales up | Pay-as-you-go, can be expensive |
Scalability | Excellent | Excellent | Excellent |
Integration with Git | Excellent | Excellent | Good |
Serverless Functions | Yes | Yes | Yes |
Deploying to Netlify: A Step-by-Step Guide
Let’s illustrate the deployment process using Netlify. This process is generally similar for other platforms, but the specific steps and interface will vary.
1. Build your React application: Run the `npm run build` command in your project’s terminal. This creates a `build` folder containing optimized files for production.
2. Create a Netlify account: If you don’t already have one, sign up for a free Netlify account.
3. Import your project: Netlify integrates directly with Git repositories. Connect your GitHub, GitLab, or Bitbucket account and select your project repository.
4. Configure the build settings: Netlify will detect your project type and suggest appropriate build commands. Usually, it will automatically detect `npm run build` as the build command and the `build` folder as the publish directory. You might need to adjust these settings if your project uses a different build process.
5. Deploy: Netlify will automatically build and deploy your application. Once the process is complete, you’ll receive a link to your live website.
Remember to consult the official documentation for Netlify (or your chosen platform) for the most up-to-date instructions and specific details related to your project setup. Each platform offers detailed guides and support resources to help you through the process.
End of Discussion
So, you’ve journeyed through the captivating world of building dynamic websites with JavaScript and React. You’ve conquered components, tamed state management, and even navigated the complexities of routing. Now, go forth and create! The web awaits your innovative, interactive creations. Remember, the possibilities are endless—so let your imagination run wild and build something amazing.