Understanding Recursion and How to Use It in Programming: Ever felt like you’re caught in a never-ending loop, only it’s a beautifully self-referential one? That’s recursion, baby! It’s a programming technique where a function calls itself, kinda like a digital Matryoshka doll. We’ll unravel the magic behind this powerful concept, from its basic principles to advanced applications, showing you how to wield recursion like a coding ninja.
This guide dives deep into the heart of recursive functions, exploring everything from base cases and recursive steps to common patterns like linear, tail, and tree recursion. We’ll compare recursion to its iterative counterpart, highlighting the strengths and weaknesses of each approach. Get ready to conquer complex problems with elegant recursive solutions, master debugging techniques, and even optimize your code for peak performance. We’ll cover practical applications in algorithms, data structures, and more – making you a recursion pro in no time.
Introduction to Recursion: Understanding Recursion And How To Use It In Programming
Recursion, in the world of programming, is like that set of Russian nesting dolls: a function that calls itself within its own definition. It’s a powerful technique that elegantly solves problems that can be broken down into smaller, self-similar subproblems. Think of it as a clever way to tackle complex tasks by repeatedly applying a simpler version of the same solution.
Recursion works by defining a base case—a condition that stops the function from calling itself infinitely—and a recursive step—the part where the function calls itself with a modified input, moving closer to the base case with each call. Without a base case, your function would enter an infinite loop, crashing your program. It’s like the smallest doll in the set—it doesn’t contain another doll, stopping the nesting process.
Recursive Function Structure
A recursive function typically follows a specific structure. It begins by checking the base case. If the base case is met, the function returns a value, ending the recursion. If the base case isn’t met, the function performs some operations and then calls itself with modified input, progressively approaching the base case. This iterative process continues until the base case is finally reached. The function then unwinds, returning values from each recursive call back up the chain to produce the final result. This structured approach ensures that the recursion eventually terminates, avoiding infinite loops.
Analogy: The Tower of Hanoi
Imagine the classic Tower of Hanoi puzzle: you have three pegs and a set of disks of different sizes, stacked on one peg in decreasing order of size. The goal is to move the entire stack to another peg, one disk at a time, with the rule that a larger disk can never be placed on top of a smaller one. Solving this puzzle recursively is intuitive. You can break down the problem into smaller subproblems: to move ‘n’ disks, you first move ‘n-1’ disks to an auxiliary peg, then move the largest disk to the target peg, and finally move the ‘n-1’ disks from the auxiliary peg to the target peg. This self-similar structure is perfectly suited for a recursive solution.
Real-World Applications of Recursion, Understanding Recursion and How to Use It in Programming
Recursion isn’t just a theoretical concept; it’s a practical tool used to solve numerous real-world problems. Consider traversing file systems: finding all files within a directory and its subdirectories can be efficiently accomplished using recursion. Each directory can be seen as a subproblem, mirroring the structure of the entire file system. Similarly, algorithms for processing tree-like data structures, such as parsing XML or HTML documents, often employ recursion to navigate the hierarchical structure. Many sorting algorithms, like merge sort and quicksort, also leverage recursion for their elegant and efficient implementation. Finally, fractal generation, which produces self-similar patterns like the Mandelbrot set, heavily relies on recursive algorithms to create these intricate designs.
Basic Principles of Recursive Functions
Recursion, at its core, is a programming technique where a function calls itself within its own definition. It’s a powerful tool for solving problems that can be broken down into smaller, self-similar subproblems. Think of it like a set of Russian nesting dolls – each doll contains a smaller version of itself, until you reach the smallest doll. Understanding the fundamental principles is key to harnessing its power effectively.
Understanding recursive functions hinges on two critical components: the base case and the recursive step. Without these, your function will endlessly call itself, leading to a dreaded stack overflow error – a situation where your program runs out of memory trying to keep track of all the nested function calls.
Base Case and Recursive Step
The base case is the condition that stops the recursion. It’s the smallest, simplest version of the problem that can be solved directly, without further recursive calls. Think of it as the smallest nesting doll – you don’t need to open it to see what’s inside. The recursive step, on the other hand, is where the function calls itself, but with a slightly modified input, moving closer to the base case with each call. It’s the process of opening each doll to reveal the next smaller one. Without a properly defined base case, the recursive step will continue indefinitely.
Factorial Calculation with Recursion
Let’s illustrate these concepts with a classic example: calculating the factorial of a number. The factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n. For example, 5! = 5 * 4 * 3 * 2 * 1 = 120. Here’s a recursive function in Python to compute the factorial:
def factorial(n):
if n == 0: # Base case: factorial of 0 is 1
return 1
else: # Recursive step: n! = n * (n-1)!
return n * factorial(n-1)
In this function, the base case is when n
equals 0. The recursive step calculates the factorial by multiplying n
with the factorial of n-1
. Each recursive call reduces the input value of n
by 1, eventually reaching the base case.
Tracing Recursive Function Execution
Tracing the execution of a recursive function can be visualized using a stack diagram. Imagine a stack of plates, where each plate represents a function call. When a function calls itself, a new plate is added to the stack. The function executes, and when it reaches a return statement, the plate is removed from the stack. Let’s trace the execution of `factorial(3)`:
1. factorial(3): The function is called with n=3. The base case is not met. The function returns 3 * factorial(2). A new plate is added to the stack.
2. factorial(2): The function is called with n=2. The base case is not met. The function returns 2 * factorial(1). Another plate is added.
3. factorial(1): The function is called with n=1. The base case is not met. The function returns 1 * factorial(0). Another plate is added.
4. factorial(0): The function is called with n=0. The base case (n==0) is met. The function returns 1. This plate is removed from the stack.
5. factorial(1): The function now returns 1 * 1 = 1. This plate is removed.
6. factorial(2): The function now returns 2 * 1 = 2. This plate is removed.
7. factorial(3): The function now returns 3 * 2 = 6. This plate is removed.
The final result, 6, is returned. The stack is now empty. This step-by-step visualization helps understand how the recursive calls unwind and produce the final result.
Common Recursive Patterns
Recursion, while elegant, isn’t a one-size-fits-all solution. Understanding different recursive patterns helps you choose the right approach for your problem, optimizing for efficiency and avoiding common pitfalls like stack overflow errors. Let’s explore some key patterns.
Linear Recursion
Linear recursion is the simplest form. Each recursive call makes only one further recursive call. Think of it like a single line of dominoes falling – one after the other. This pattern is straightforward to understand but can be less efficient for deeply nested calls due to the function call overhead. The time complexity often directly reflects the input size.
Pattern | Description | Example Code (Python) | Time Complexity |
---|---|---|---|
Linear Recursion | A recursive function makes only one recursive call within its body. |
|
O(n) |
Tail Recursion
Tail recursion is a special case of linear recursion where the recursive call is the very last operation performed in the function. This is crucial because compilers and interpreters can often optimize tail-recursive functions into iterative loops, preventing stack overflow errors even with very deep recursion. Think of it like a perfectly choreographed dance where each step leads directly to the next, without any lingering actions.
Pattern | Description | Example Code (Python – Note: Python doesn’t optimize tail recursion) | Time Complexity |
---|---|---|---|
Tail Recursion | The recursive call is the last operation performed; optimizable to iteration in some languages. |
|
O(n) |
Tree Recursion
Tree recursion is where a function makes multiple recursive calls within its body. Imagine a branching tree structure – each node can have multiple children, leading to a potentially large number of recursive calls. This pattern is common when dealing with tree-like data structures or problems that can be broken down into smaller, independent subproblems. The time complexity can vary greatly depending on the branching factor and depth of the tree.
Pattern | Description | Example Code (Python) | Time Complexity |
---|---|---|---|
Tree Recursion | A recursive function makes multiple recursive calls. |
|
O(2n) - Inefficient due to repeated calculations |
Recursion vs. Iteration
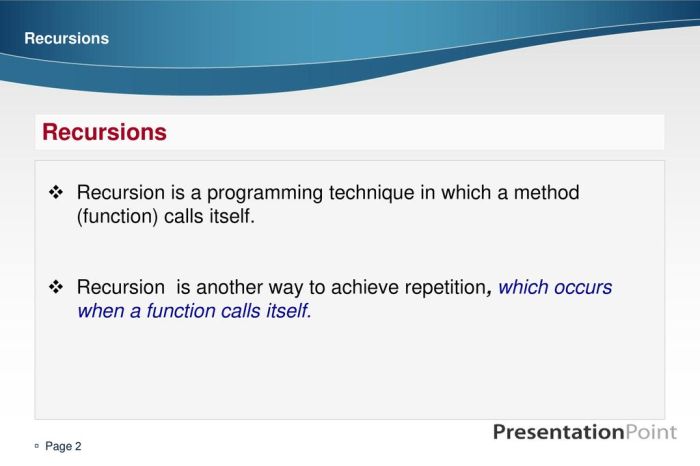
Source: slideplayer.com
Grasping recursion in programming can feel like navigating a maze, each function call a step deeper. But just like understanding the potential risks to your home, planning for the unexpected is key; consider adding earthquake coverage to your insurance policy, as explained in this helpful article: Why You Should Add Earthquake Coverage to Your Home Insurance.
Similarly, recursive functions need a solid base case to prevent infinite loops – a well-defined exit strategy is crucial for both coding and life’s unexpected tremors.
Recursion and iteration are two fundamental programming paradigms for solving repetitive problems. Both achieve similar results, but they differ significantly in their approach, leading to trade-offs in terms of code readability, efficiency, and memory usage. Understanding these differences is crucial for choosing the most appropriate method for a given task.
Comparison of Recursive and Iterative Approaches
Let's illustrate the differences by examining the calculation of the Fibonacci sequence. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. Both recursion and iteration can be used to generate this sequence. A recursive approach defines the Fibonacci number as a function of itself (F(n) = F(n-1) + F(n-2)), while an iterative approach uses a loop to calculate each number sequentially.
Advantages and Disadvantages of Recursion
Recursion offers elegance and simplicity for problems that can be naturally broken down into smaller, self-similar subproblems. The code often mirrors the problem's structure, making it easier to understand and implement. However, recursion can be less efficient due to function call overhead and the potential for stack overflow errors if the recursion depth becomes too large. Deeply nested recursive calls can consume significant memory on the call stack.
Advantages and Disadvantages of Iteration
Iteration, using loops like `for` or `while`, is generally more efficient than recursion because it avoids the overhead of function calls. It's also less prone to stack overflow errors. However, iterative solutions can sometimes be more complex and harder to read, especially for problems that have a naturally recursive structure. The code might become less intuitive and harder to maintain as the complexity increases.
Comparative Table: Recursion vs. Iteration
This table summarizes the key differences between recursive and iterative approaches:
Feature | Recursion | Iteration |
---|---|---|
Readability | Can be more concise and elegant for naturally recursive problems; can be less readable for complex problems. | Can be less concise but generally easier to understand for most problems. |
Efficiency | Generally less efficient due to function call overhead; can be significantly slower for deep recursion. | Generally more efficient; avoids function call overhead. |
Memory Usage | Can consume more memory due to the call stack; risk of stack overflow for deep recursion. | Generally uses less memory; less prone to stack overflow. |
Error Handling | More susceptible to stack overflow errors. | Less susceptible to stack overflow errors. |
Practical Applications of Recursion
Recursion, while conceptually elegant, isn't just a theoretical exercise. It's a powerful tool used extensively in real-world programming scenarios, offering efficient and often elegant solutions to complex problems. Its ability to break down large problems into smaller, self-similar subproblems makes it particularly well-suited for certain data structures and algorithms.
Recursion shines when dealing with data that naturally exhibits a hierarchical or self-similar structure. This inherent recursive nature simplifies problem-solving and leads to concise, readable code. Let's dive into some key areas where recursion proves its worth.
Sorting Algorithms
Merge sort and quicksort are two classic examples of sorting algorithms that leverage the power of recursion. Merge sort recursively divides the unsorted list into smaller sublists until each sublist contains only one element (which is inherently sorted). Then, it recursively merges the sublists to produce new sorted sublists until there is only one sorted list remaining. Quicksort, on the other hand, selects a 'pivot' element and recursively partitions the array into two sub-arrays, one containing elements smaller than the pivot and the other containing elements larger than the pivot. This process continues recursively until all sub-arrays are sorted. The recursive calls elegantly handle the sub-problems, resulting in efficient sorting, especially for large datasets. The efficiency gains stem from the divide-and-conquer approach; tackling smaller, manageable chunks of the problem instead of attempting to sort the entire list at once.
Graph Traversal
Graphs, representing connections between nodes, are another area where recursion shines. Depth-first search (DFS) is a graph traversal algorithm that utilizes recursion to explore a graph's nodes as deeply as possible along each branch before backtracking. Imagine navigating a maze; DFS is like following one path to its end before trying another. This recursive approach is ideal for tasks like finding paths, detecting cycles, or topological sorting. The recursive nature allows the algorithm to naturally explore the graph's branches systematically.
Tree Operations
Trees, hierarchical data structures, are naturally suited for recursive processing. Operations like tree traversal (inorder, preorder, postorder), searching (finding a specific node), and insertion/deletion of nodes are often implemented recursively. The recursive approach mirrors the tree's hierarchical structure, making the code concise and easier to understand. For example, traversing a binary tree recursively involves visiting the left subtree, the root node, and then the right subtree – a naturally recursive process. This recursive structure neatly aligns with the inherent structure of the tree itself.
Recursive Function for Depth-First Search
Let's illustrate recursion with a concrete example: a depth-first search (DFS) on a graph represented as an adjacency list. An adjacency list is a way to represent a graph where each node has a list of its neighbors.
```python
def dfs(graph, node, visited, path):
visited[node] = True
path.append(node)
for neighbor in graph[node]:
if not visited[neighbor]:
dfs(graph, neighbor, visited, path)
return path
# Example graph represented as an adjacency list
graph =
'A': ['B', 'C'],
'B': ['D', 'E'],
'C': ['F'],
'D': [],
'E': ['F'],
'F': []
visited = node: False for node in graph
path = dfs(graph, 'A', visited, [])
print(f"Depth-First Search path: path") # Output will show a path, example: ['A', 'B', 'D', 'E', 'F', 'C']
```
This Python function recursively explores the graph. The `visited` dictionary keeps track of visited nodes, preventing cycles. The `path` list stores the order of visited nodes. The function recursively calls itself for each unvisited neighbor, effectively traversing the graph depth-first.
Linked Lists
Recursion finds application in manipulating linked lists. Operations like traversing a linked list, searching for a specific node, or inserting/deleting a node can be elegantly implemented using recursion. The recursive approach mirrors the linked list's sequential structure, where each node points to the next. Each recursive call processes the next node in the sequence until the end of the list is reached.
Trees
As mentioned earlier, trees are a prime example of recursive data structures. Their hierarchical nature naturally lends itself to recursive algorithms. Traversing a tree (inorder, preorder, postorder), searching for a node, or inserting/deleting a node are all commonly implemented recursively. The recursive calls neatly follow the tree's branching structure, making the code both concise and efficient. The recursive approach directly mirrors the tree's structure, making the implementation intuitive and easier to understand.
Debugging Recursive Functions
Recursive functions, while elegant and powerful, can be notoriously tricky to debug. The nested calls and potential for infinite loops make tracking down errors more challenging than with iterative functions. Understanding common pitfalls and employing effective debugging strategies is crucial for mastering recursion.
Debugging recursive functions often involves carefully tracing the function's execution through each recursive call. This requires a methodical approach and a keen eye for detail, especially when dealing with complex recursive structures. The most common problems stem from incorrect base cases or faulty recursive steps, both of which can lead to unexpected behavior or program crashes.
Infinite Recursion and Stack Overflow
Infinite recursion occurs when a recursive function never reaches its base case, resulting in an endless chain of calls. This quickly consumes system resources, eventually leading to a stack overflow error – a dreaded crash caused by exceeding the program's memory allocated for function calls. Imagine a child's set of Russian nesting dolls, infinitely nested. The program tries to create a new doll (function call) for each level, but runs out of space. This is the essence of a stack overflow in the context of recursion. To prevent this, always meticulously verify that your base case is correctly defined and that the recursive step moves closer to the base case with each call.
Strategies for Debugging Recursive Functions
Effective debugging involves a combination of careful code review, strategic print statements, and the use of a debugger. Begin by thoroughly examining your base case and recursive step. Are they correctly defined? Does the recursive step consistently progress towards the base case? A simple, yet effective technique is to add print statements at strategic points within the function to track the values of key variables during each recursive call. This allows you to visually follow the flow of execution and identify where things go wrong. For instance, printing the input value and the return value at the beginning and end of the function can be invaluable.
Using a Debugger to Trace Recursive Function Execution
Debuggers provide a powerful tool for stepping through the execution of a recursive function, inspecting variables at each level of recursion. Most IDEs (Integrated Development Environments) include built-in debuggers. Using a debugger, you can set breakpoints at various points in your code, allowing you to pause execution and inspect the call stack. The call stack shows the sequence of function calls, enabling you to visualize the progression of the recursion. By stepping through the code line by line, you can observe the values of variables and understand the flow of execution at each level. This detailed view helps pinpoint errors in base cases, recursive steps, or other logical flaws that might be hard to detect through simple print statements. A visual representation of the call stack, showing the function's parameters and return values at each level, is crucial for understanding the recursion's progression.
Optimizing Recursive Functions
Recursive functions, while elegant and often mirroring the problem's structure beautifully, can sometimes stumble into performance pitfalls. Unoptimized recursion can lead to excessive function calls and potentially stack overflow errors, especially when dealing with large input sizes. Fortunately, several techniques can significantly boost the efficiency of your recursive algorithms.
The key to optimizing recursive functions lies in reducing redundant computations and managing the call stack effectively. This involves strategic approaches like memoization and tail call optimization, which we'll explore in detail. Understanding these techniques can transform a slow, inefficient recursive function into a highly performant one.
Memoization
Memoization is a powerful optimization technique that stores the results of expensive function calls and reuses them when the same inputs occur again. This avoids redundant calculations, significantly speeding up the function, especially for recursive functions that repeatedly compute the same values. Imagine calculating the Fibonacci sequence recursively without memoization – each Fibonacci number requires recalculating previously computed numbers. Memoization prevents this repetition.
Consider a recursive function to calculate the nth Fibonacci number:
```python
def fibonacci_no_memo(n):
if n <= 1:
return n
else:
return fibonacci_no_memo(n-1) + fibonacci_no_memo(n-2)
def fibonacci_memo(n, memo=):
if n in memo:
return memo[n]
if n <= 1:
return n
else:
result = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
memo[n] = result
return result
```
The `fibonacci_memo` function uses a dictionary `memo` to store previously calculated Fibonacci numbers. Before making a recursive call, it checks if the result is already in `memo`. If so, it returns the stored value; otherwise, it calculates the value, stores it in `memo`, and returns it. For larger values of `n`, the difference in performance between `fibonacci_no_memo` and `fibonacci_memo` becomes dramatic. `fibonacci_no_memo` exhibits exponential time complexity, while `fibonacci_memo` achieves linear time complexity thanks to memoization.
Tail Call Optimization
Tail call optimization (TCO) is a compiler optimization technique that transforms a recursive function call into a loop. This prevents the accumulation of function calls on the call stack, avoiding potential stack overflow errors. However, TCO is not universally supported by all programming languages and compilers. Languages like Scheme and some functional programming languages explicitly support TCO. In languages like Python, TCO is generally not available.
A tail-recursive function is one where the recursive call is the very last operation performed in the function. For example:
```python
# This is NOT tail-recursive because addition happens after the recursive call
def factorial_not_tail_recursive(n, acc=1):
if n == 0:
return acc
else:
return acc * factorial_not_tail_recursive(n-1, acc)
#This is tail-recursive (although Python won't optimize it)
def factorial_tail_recursive(n, acc=1):
if n == 0:
return acc
else:
return factorial_tail_recursive(n-1, n * acc)
```
In the `factorial_tail_recursive` example, the recursive call is the last operation. If a compiler supports TCO, it would transform this into an iterative loop, significantly improving performance and preventing stack overflow for large values of `n`. However, Python's interpreter doesn't perform TCO, so even though the function is tail-recursive, it will still use the call stack.
Advanced Recursion Techniques
Recursion, while seemingly simple at its core, can reach impressive levels of sophistication. Mastering these advanced techniques unlocks the ability to solve complex problems elegantly and efficiently, often in ways that iterative approaches struggle to match. This section delves into the more nuanced aspects of recursion, revealing its power beyond basic examples.
Beyond the standard recursive function calls, more advanced scenarios require a deeper understanding of how recursion interacts with other programming concepts. This often involves leveraging the power of higher-order functions and exploring the fascinating world of mutual recursion.
Mutual Recursion
Mutual recursion occurs when two or more functions call each other in a cyclical manner. This creates a feedback loop where each function's execution depends on the output of another, ultimately leading to a desired result. It's a powerful technique for modelling systems with interdependent components, often seen in situations where a clear hierarchical structure isn't readily apparent.
Consider a scenario where you're parsing a complex grammar. One function might handle noun phrases, while another handles verb phrases. The noun phrase function might call the verb phrase function to check for certain grammatical structures, and vice-versa. This interdependency is naturally expressed through mutual recursion. The functions recursively call each other until a base case is reached, successfully parsing the sentence.
Recursion with Higher-Order Functions
Higher-order functions, functions that take other functions as arguments or return functions as results, can significantly enhance the power and flexibility of recursion. Combining these two concepts allows for concise and elegant solutions to complex problems.
Imagine a scenario where you need to process a list of data, applying a recursive function to each element. A higher-order function can encapsulate the iteration, making the code cleaner and easier to understand. For example, a function could take a recursive function as an argument and apply it to each element of a list using a map operation. This approach simplifies the logic and makes the code more modular and reusable.
Scenarios Requiring Advanced Recursion Techniques
Advanced recursion techniques aren't always necessary, but they become invaluable in specific contexts. These scenarios often involve intricate data structures or complex logical relationships that are difficult to manage with simple iterative approaches.
One prime example is compiler design. Compilers need to parse complex grammar rules, and mutual recursion is often used to handle the recursive nature of these rules. Another example is artificial intelligence, particularly in algorithms that use tree-like structures such as game-playing AI or natural language processing. In these cases, the inherent recursive structure of the problem lends itself perfectly to recursive solutions enhanced by higher-order functions for greater efficiency and code clarity.
Final Conclusion
So, you've conquered the art of recursion! From understanding its core principles to mastering advanced techniques, you've unlocked a powerful tool in your programming arsenal. Remember, recursion is not just about writing elegant code; it's about thinking recursively – breaking down complex problems into smaller, self-similar subproblems. With practice and a keen eye for detail, you'll find yourself effortlessly crafting efficient and elegant solutions to problems that once seemed insurmountable. Now go forth and recursively conquer!