Creating Your First Mobile App with React Native: Dive into the exciting world of mobile app development! This guide walks you through building your first app using React Native, a powerful framework that lets you build native-quality apps for both iOS and Android using JavaScript. We’ll cover everything from setting up your development environment to deploying your finished app to the app stores. Get ready to transform your coding skills and unleash your inner app developer!
We’ll explore the core concepts of React Native, including components, JSX, and state management, and show you how to build a user interface (UI), handle user input, manage data, and integrate with APIs. We’ll also touch upon crucial aspects like app deployment, testing, and even some advanced concepts to help you build robust and high-performing apps. So, are you ready to build something amazing?
Introduction to React Native
So, you’re ready to dive into the world of mobile app development? Forget the endless headaches of learning Swift or Kotlin – React Native is here to save the day (and your sanity). This framework lets you build truly native-feeling apps for both iOS and Android using just JavaScript. It’s like getting two birds stoned with one JavaScript-powered slingshot.
React Native offers a compelling blend of speed, efficiency, and cross-platform compatibility. Imagine building one codebase and deploying it to both the App Store and Google Play – less work, double the reach. That’s the power of React Native.
Benefits of Using React Native
React Native’s popularity isn’t just hype. It boasts several key advantages that make it a top choice for developers. Its “learn once, write everywhere” philosophy dramatically reduces development time and costs, making it a particularly attractive option for startups and smaller teams. Plus, the large and active community provides ample support and resources. The ability to reuse components across platforms also streamlines the development process and ensures consistency in the user experience. Finally, React Native allows for faster iteration and easier debugging, speeding up the overall development cycle.
Core Concepts of React Native
React Native leverages React, a popular JavaScript library for building user interfaces. Understanding its core concepts is crucial for effective app development.
Components are the fundamental building blocks of React Native apps. They’re reusable pieces of code that encapsulate specific UI elements and their logic. Think of them as LEGO bricks – you combine them to create complex structures. For example, a button, a text input field, or an image would each be a component.
JSX (JavaScript XML) is a syntax extension that allows you to write HTML-like code within your JavaScript. This makes it easier to structure and visualize your UI. Instead of writing complex JavaScript functions to create elements, you can use JSX to write something more intuitive and readable. For instance, `
State management is crucial for handling data changes in your app. React Native apps are reactive – they automatically update the UI whenever the state changes. This ensures your app always displays the most current information. Popular state management solutions include Redux and Context API, offering different approaches to managing and updating data throughout your application.
Setting Up the React Native Development Environment
Ready to get your hands dirty? Setting up your environment is the first step. This involves installing Node.js and npm (or yarn), followed by the React Native CLI (command-line interface).
First, download and install Node.js from the official website. This installation usually includes npm, the Node Package Manager, which you’ll need to install React Native and other dependencies. Alternatively, you can use Yarn, a faster package manager.
Next, install the React Native CLI globally using npm or yarn: `npm install -g react-native-cli` or `yarn global add react-native-cli`.
Once the CLI is installed, you can create a new project using the command: `react-native init MyFirstApp`. This will generate a basic React Native project named “MyFirstApp”. You’ll need to have Android Studio (with the Android SDK) or Xcode (for iOS development) installed, depending on the platform you want to target. After the project is created, you can navigate to the project directory and run it on an emulator or a physical device using the appropriate commands for Android (`npx react-native run-android`) or iOS (`npx react-native run-ios`). Remember to follow the detailed instructions provided by the React Native documentation for a smooth installation process. Troubleshooting might be necessary, especially if you encounter platform-specific issues.
Building the User Interface (UI)
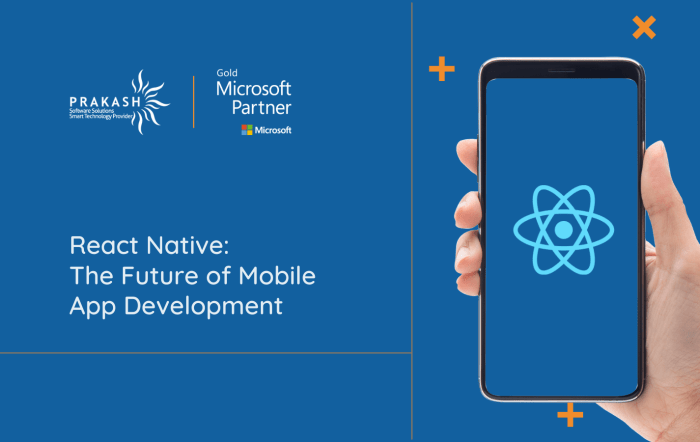
Source: prakashinfotech.com
Building your first mobile app with React Native? Awesome! But before you launch your landlord app (yes, you’re thinking big!), make sure you’ve got the right protection. Check out this guide on How to Choose Insurance Coverage for Your Rental Property to avoid future headaches. Then, get back to coding that killer React Native UI – you got this!
So, you’ve got the basics of React Native down. Now it’s time to get your hands dirty and build something visually appealing! This section will guide you through crafting a user interface, from laying out components to managing the ever-changing state of your app. Think of it as decorating your digital apartment – you need a plan and the right tools.
Simple UI Layout with React Native Components
Let’s design a simple to-do list app. Understanding component hierarchy is crucial for building scalable and maintainable UIs. Think of it like building with LEGOs – you start with individual bricks (components) and assemble them into larger structures. Below is a table illustrating the component structure of our basic to-do app.
Component Name | Description | Props Used | Example Code Snippet |
---|---|---|---|
View | The fundamental building block for UI layout. Think of it as a container. | style | <View style=styles.container>...</View> |
Text | Displays text. | style, children | <Text style=styles.title>My To-Do List</Text> |
TextInput | Allows users to input text. | style, onChangeText, value | <TextInput style=styles.input onChangeText=setText value=text /> |
Button | Triggers an action when pressed. | style, onPress, title | <Button title="Add Task" onPress=addTask /> |
FlatList | Displays a scrollable list of items. | data, renderItem, keyExtractor | <FlatList data=todos renderItem=renderItem keyExtractor=(item) => item.id /> |
Styling Components with Inline Styles and StyleSheet
React Native offers two primary ways to style components: inline styles and `StyleSheet`. Inline styles are great for quick adjustments, while `StyleSheet` promotes code reusability and maintainability, especially for larger projects. Imagine inline styles as quickly applying a single coat of paint, while `StyleSheet` is like using a pre-mixed paint can for consistency across your entire project.
Here’s how you’d use both methods:
Inline Styles:
<Text style=fontSize: 20, color: 'blue'>Hello</Text>
StyleSheet:
const styles = StyleSheet.create(
container:
flex: 1,
justifyContent: 'center',
alignItems: 'center',
,
title:
fontSize: 24,
fontWeight: 'bold',
,
);
<View style=styles.container>
<Text style=styles.title>My App</Text>
</View>
Managing UI State with useState and useReducer
Managing the UI state is fundamental to creating dynamic and interactive apps. React Native provides `useState` and `useReducer` hooks for this purpose. `useState` is perfect for simple state management, while `useReducer` shines when dealing with complex state logic and multiple state updates. Think of `useState` as a simple on/off switch, while `useReducer` is a more sophisticated control panel.
`useState` Example:
const [count, setCount] = useState(0);
<Button title="Increment" onPress=() => setCount(count + 1) />
<Text>Count: count</Text>
`useReducer` Example:
const initialState = count: 0;
const reducer = (state, action) =>
switch (action.type)
case 'increment':
return count: state.count + 1;
case 'decrement':
return count: state.count - 1;
default:
return state;
;
const [state, dispatch] = useReducer(reducer, initialState);
<Button title="Increment" onPress=() => dispatch(type: 'increment') />
<Button title="Decrement" onPress=() => dispatch(type: 'decrement') />
<Text>Count: state.count</Text>
Handling User Input and Interactions: Creating Your First Mobile App With React Native
So, you’ve got your basic React Native app looking pretty slick. But a static screen is about as exciting as watching paint dry. To make your app truly interactive and user-friendly, you need to handle user input. This means making your app respond to things like button presses, text entry, and even swipes. Let’s dive into the juicy details of making your app react to its users.
Text Input Handling
Capturing user text is fundamental. React Native provides the `TextInput` component for this. It’s super straightforward. You’ll typically use the `onChangeText` prop to handle changes in the input field. This prop takes a function that receives the new text as an argument.
Here’s a simple example:
“`javascript
import React, useState from ‘react’;
import View, TextInput, Text from ‘react-native’;
const MyTextInput = () =>
const [text, setText] = useState(”);
return (
value=text
/>
);
;
export default MyTextInput;
“`
This code creates a text input field. As the user types, the `onChangeText` function updates the `text` state variable, which is then displayed below the input field. Simple, right?
Button Interactions
Buttons are the workhorses of interaction. In React Native, the `TouchableOpacity` component (or `Button` for a simpler approach) lets users trigger actions. The `onPress` prop takes a function that executes when the button is tapped.
Let’s create a button that displays an alert:
“`javascript
import React from ‘react’;
import TouchableOpacity, Text, Alert from ‘react-native’;
const MyButton = () =>
const handlePress = () =>
Alert.alert(‘Button Pressed!’, ‘You tapped the button!’);
;
return (
);
;
export default MyButton;
“`
This code creates a button that, when pressed, displays a simple alert box. You can replace `Alert.alert` with any function you want to execute, making it a powerful tool for controlling app behavior.
Touch Events
Beyond buttons, you can handle more general touch events using the `PanResponder` API. This allows for gestures like swiping and dragging. While more complex than buttons, it opens up a world of possibilities for more interactive interfaces. Think of creating a draggable element or a gesture-based control.
Designing an Interactive Form
Let’s build a simple registration form. This will combine text inputs and buttons to showcase a more complex interaction.
“`javascript
import React, useState from ‘react’;
import View, TextInput, Button, Text from ‘react-native’;
const RegistrationForm = () =>
const [name, setName] = useState(”);
const [email, setEmail] = useState(”);
const [password, setPassword] = useState(”);
const handleSubmit = () =>
// Handle form submission here (e.g., API call)
console.log(‘Name:’, name);
console.log(‘Email:’, email);
console.log(‘Password:’, password);
;
return (
);
;
export default RegistrationForm;
“`
This form includes fields for name, email, and password. The `handleSubmit` function (currently a placeholder) would typically handle sending the data to a server or performing other actions. The `secureTextEntry` prop ensures the password field masks the input.
Screen Navigation with React Navigation
To move between different parts of your app, you’ll need navigation. React Navigation is a popular library for this. It allows you to define different screens and handle transitions between them.
Here’s a basic example of navigating between two screens:
“`javascript
//Screen1.js
import React from ‘react’;
import View, Text, Button from ‘react-native’;
import useNavigation from ‘@react-navigation/native’;
const Screen1 = () =>
const navigation = useNavigation();
return (
);
;
export default Screen1;
//Screen2.js
import React from ‘react’;
import View, Text from ‘react-native’;
const Screen2 = () =>
return (
);
;
export default Screen2;
“`
This requires setting up React Navigation in your app (instructions are readily available in the React Navigation documentation). This example shows how to use `useNavigation` hook to navigate to another screen using its name.
Data Management and APIs
So, you’ve built the beautiful UI of your React Native app, but where’s the data going to come from? This is where data management and APIs step in – the unsung heroes that bring your app to life. We’ll explore different ways to store and retrieve data, transforming your static app into a dynamic, data-driven experience.
Local Data Storage with AsyncStorage, Creating Your First Mobile App with React Native
AsyncStorage is a simple, asynchronous, persistent, key-value storage system in React Native. Think of it as your app’s personal, onboard storage locker. It’s perfect for storing small amounts of data that need to be readily available, even when the app is offline. For larger datasets or more complex data structures, consider other options (like Realm or SQLite).
Here’s how you can save and retrieve data using AsyncStorage:
“`javascript
import AsyncStorage from ‘@react-native-async-storage/async-storage’;
// Saving data
const saveData = async (key, value) =>
try
await AsyncStorage.setItem(key, value);
catch (e)
console.error(‘Failed to save the data:’, e);
;
// Retrieving data
const getData = async (key) =>
try
const value = await AsyncStorage.getItem(key);
return value;
catch (e)
console.error(‘Failed to retrieve the data:’, e);
;
// Example usage:
saveData(‘userName’, ‘JohnDoe’);
getData(‘userName’).then(value => console.log(‘User Name:’, value));
“`
Fetching Data from a Remote API using Fetch
Now, let’s connect your app to the outside world! Fetching data from a remote API is crucial for most modern apps. The `fetch` API is a built-in JavaScript feature that makes this process relatively straightforward. We’ll use a simple example to illustrate how to retrieve data and handle potential errors.
“`javascript
const fetchData = async () =>
try
const response = await fetch(‘https://api.example.com/data’);
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
const data = await response.json();
return data;
catch (error)
console.error(‘Error fetching data:’, error);
return null; // Or throw the error, depending on your error handling strategy
;
fetchData().then(data => console.log(‘Fetched Data:’, data));
“`
This code snippet fetches data from a hypothetical API endpoint. It checks the response status and parses the JSON response. Crucially, it includes error handling to gracefully manage potential network issues or API errors. Remember to replace `’https://api.example.com/data’` with your actual API endpoint.
Displaying API Data in a List View
Once you’ve fetched data, you need a way to present it to the user. A list view, using components like `FlatList` or `SectionList` in React Native, is a common and efficient way to display collections of data.
Imagine you’ve fetched an array of objects, each representing a news article with a title and description. You could render this data in a `FlatList` like so:
“`javascript
import FlatList, Text, View from ‘react-native’;
const NewsList = ( newsData ) =>
const renderItem = ( item ) => (
);
return (
/>
);
;
// Example usage (assuming ‘newsData’ is fetched from an API):
fetchData().then(data =>
“`
This creates a scrollable list displaying the title and description of each news article. Remember to replace placeholder styles with your own custom styles. The `keyExtractor` function is crucial for performance and efficient updates of the list. It ensures React Native can uniquely identify each item in the list.
App Deployment
So, you’ve built your killer React Native app. Congrats! Now comes the exciting (and sometimes slightly terrifying) part: getting it into the hands of your users. Deploying to both iOS and Android involves a slightly different process, but the core principles remain the same: build a release version, prepare your store listings, and then submit! Let’s break it down.
Deploying your React Native app involves building optimized versions for each platform and then submitting them to their respective app stores. This process ensures your app is ready for the scrutiny of users and meets the standards of each platform.
Building and Deploying to iOS and Android
Building a release version of your app requires using the React Native CLI. For iOS, you’ll need a Mac and Xcode. The process involves running commands like `npx react-native run-ios –configuration Release`. This will create an .ipa file, which is the package you’ll upload to the Apple App Store. Android is slightly simpler; you can build an APK (Android Package Kit) file using commands like `npx react-native run-android –configuration Release`. This file will then be uploaded to the Google Play Store. Remember, you’ll need to configure your app’s signing keys for both platforms to ensure security and proper identification. These keys are crucial for maintaining the integrity and authenticity of your app, preventing unauthorized modifications or distribution.
Creating and Configuring App Store Accounts
Before you can deploy, you’ll need accounts with both the Apple App Store and the Google Play Store. Apple’s App Store Connect requires a paid developer program membership. You’ll need to provide information about your app, including screenshots, descriptions, and a privacy policy. The process involves creating a detailed app listing that showcases your app’s functionality and benefits to potential users. Google Play Console is similar, requiring you to create a developer account and provide details about your app. You’ll need to upload your APK, provide screenshots, and fill out various forms. Both platforms have strict guidelines, so carefully review their requirements before submitting your app. Failing to adhere to these guidelines can result in app rejection. For example, Apple is particularly strict about UI guidelines and performance standards, while Google focuses on security and malware prevention.
Testing Strategies for App Stability and Functionality
Thorough testing is paramount before launching your app. This prevents embarrassing bugs and ensures a positive user experience. Consider these strategies:
- Unit Testing: Test individual components of your app in isolation. This helps identify issues early on.
- Integration Testing: Test how different components interact with each other. This reveals potential problems in the flow of your app.
- End-to-End Testing: Test the entire app flow from start to finish, simulating real-world user scenarios. This ensures that all aspects of your app function as intended.
- UI Testing: Automated tests that verify the visual elements of your app, ensuring consistency and correctness across different devices and screen sizes.
- User Acceptance Testing (UAT): Have a group of real users test your app and provide feedback. This provides invaluable insights into the user experience.
Testing is not just about finding bugs; it’s about ensuring a smooth, enjoyable experience for your users. A well-tested app builds trust and encourages positive reviews. Consider utilizing both automated and manual testing techniques for a comprehensive approach. For instance, automated UI testing can catch regressions quickly, while manual testing allows for more nuanced evaluation of the user experience.
Advanced Concepts (Optional)
Leveling up your React Native game involves diving into more advanced techniques that significantly impact app performance, scalability, and overall user experience. This section explores crucial concepts to take your app from good to great. We’ll touch upon efficient state management, performance optimization strategies, and common pitfalls to avoid.
State Management with Redux and MobX
Managing application state effectively becomes increasingly important as your React Native app grows in complexity. Uncontrolled state management can lead to bugs and make code difficult to maintain. Libraries like Redux and MobX provide structured approaches to handle state, making your application more predictable and easier to debug. Redux follows a unidirectional data flow, employing a single source of truth (the store) to manage the application’s state. MobX, on the other hand, uses observable objects and reactions to automatically update components when data changes. This makes it more concise and less verbose than Redux for smaller to medium-sized applications.
Consider a simple to-do list application. With Redux, you’d define actions to add, remove, and toggle tasks. These actions would update the store, triggering re-renders of components displaying the to-do list. MobX would involve making the to-do list an observable array. Adding or removing items would automatically update the components displaying the list. The choice between Redux and MobX depends on project size and complexity, personal preference, and team familiarity.
Performance Optimization Techniques
A high-performing app is a happy app. React Native offers various techniques to optimize your app’s speed and responsiveness. These include optimizing images (using appropriate sizes and formats), minimizing the number of components, using `FlatList` or `SectionList` instead of `ScrollView` for large lists, and leveraging asynchronous operations to avoid blocking the main thread. Code splitting, which involves breaking down your app into smaller bundles, can significantly reduce initial load times. Profiling your app with tools like React Native Debugger can help identify performance bottlenecks and guide optimization efforts. For example, imagine an e-commerce app displaying hundreds of products. Using `FlatList` instead of `ScrollView` will drastically improve scrolling performance, preventing lag and enhancing user experience.
Common Challenges and Solutions
Developing React Native apps is not always smooth sailing. Common issues include debugging complexities, platform-specific inconsistencies, and managing dependencies. Debugging can be more challenging than with native development, requiring familiarity with tools like React Native Debugger and knowledge of JavaScript debugging techniques. Platform-specific inconsistencies might necessitate conditional rendering or platform-specific code, which adds complexity. Effective dependency management is critical to avoid conflicts and ensure smooth updates. Using tools like Expo and carefully managing package versions minimizes these issues. For example, a common challenge is handling different screen sizes and orientations across various devices. Using responsive design principles and utilizing flexbox ensures consistent layout across different devices.
Concluding Remarks
Building your first mobile app with React Native is a journey, not a sprint. From mastering the fundamentals of React Native components and state management to deploying your app on the App Store and Google Play, you’ve gained valuable skills. Remember, continuous learning and practice are key to becoming a proficient mobile app developer. So, go forth, build, iterate, and watch your app idea come to life! The world awaits your next app innovation.