Understanding the MVC Design Pattern in Web Development: Ever wondered how your favorite websites manage to keep their data, presentation, and user interactions neatly separated? The secret sauce is often the Model-View-Controller (MVC) design pattern. This architectural marvel streamlines web development, making applications easier to build, maintain, and scale. We’ll unravel the mysteries of MVC, exploring its core components, benefits, and practical implementation in web applications.
From understanding the roles of the Model, View, and Controller to exploring popular MVC frameworks like Ruby on Rails and Laravel, this guide offers a comprehensive overview. We’ll walk you through building a simple MVC application, tackling common challenges, and even delving into advanced concepts like RESTful APIs and security considerations. Get ready to level up your web development game!
Introduction to MVC
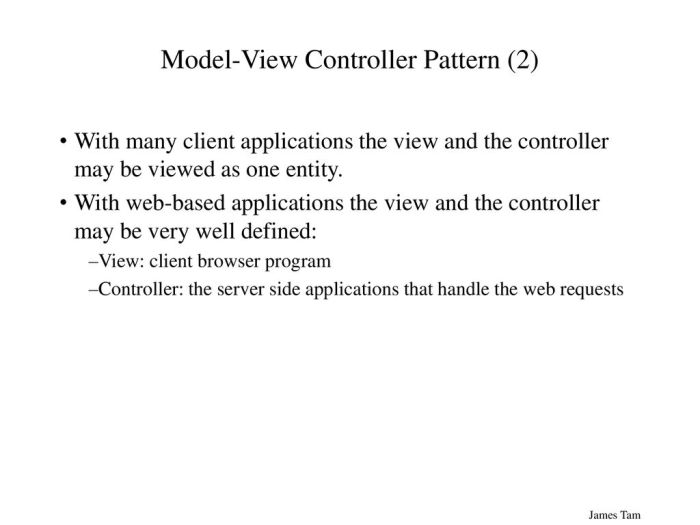
Source: slideplayer.com
Mastering the MVC design pattern in web development is crucial for building scalable and maintainable applications. Think of it like building a solid financial foundation; you wouldn’t skip crucial elements like Why You Should Include Disability Insurance in Your Financial Plan , right? Similarly, a well-structured MVC architecture protects your project from future headaches, ensuring a smooth and efficient development process.
Just like a robust financial plan, a solid MVC structure is an investment in long-term success.
So, you’re diving into the world of web development and you’ve heard whispers of MVC – the Model-View-Controller design pattern. Sounds intimidating, right? Don’t worry, it’s less complicated than it sounds. Think of it as a super-organized way to build websites and web applications, keeping everything neat and tidy, making your code easier to understand, maintain, and scale.
MVC is all about separating concerns. Instead of having all your code in one big, messy pile, you neatly organize it into three distinct parts: the Model, the View, and the Controller. This separation makes development more efficient and collaborative. Imagine trying to build a house without separating the tasks of the architect (design), the construction workers (building), and the interior designer (decor). Chaos, right? MVC provides that same organizational structure for your web applications.
The Core Principles of MVC
The Model handles the data – think of it as the brains of the operation. It’s responsible for fetching, storing, and manipulating data from a database or other sources. The View is what the user sees – the visual presentation of the data. It’s like the pretty face of your application. Finally, the Controller acts as the mediator, receiving user input, updating the Model, and selecting the appropriate View to display. It’s the glue that holds everything together.
A Simple Analogy: The Restaurant
Let’s use a restaurant analogy. The Model is the kitchen – where the food (data) is prepared. The View is the dining room – where the customers (users) see and enjoy the food. The Controller is the waiter – taking orders (user input), relaying them to the kitchen, and bringing the finished food to the table.
A Brief History of MVC
The MVC pattern has a surprisingly long history. Its roots trace back to the 1970s with the Smalltalk language. It wasn’t initially designed for web development, but its principles proved remarkably adaptable. As web development evolved, MVC became increasingly popular, finding its place in various frameworks like Ruby on Rails, ASP.NET MVC, and many others. Its evolution has been marked by improvements in efficiency and integration with modern web technologies. The core principles remain consistent, but the implementation details have evolved to accommodate the changing landscape of web development.
Components of MVC
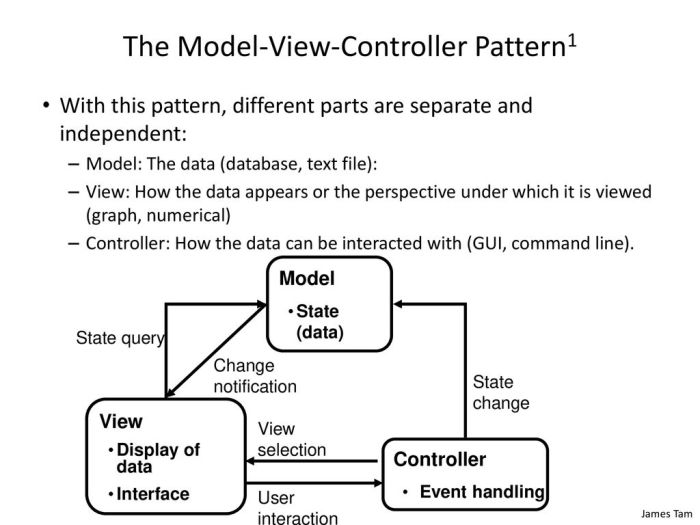
Source: slideplayer.com
So, you’ve grasped the basic concept of MVC – Model-View-Controller. Now let’s dive into the nitty-gritty details of each component and how they work together like a well-oiled machine (or, you know, a really efficient web application). Understanding their individual roles is key to building robust and maintainable web apps.
Model Responsibilities
The Model is the brains of the operation, handling all the data logic. Think of it as the backstage area where all the heavy lifting happens. It’s responsible for interacting with the database, fetching data, performing calculations, and generally managing the application’s data. It doesn’t care about how the data is presented; it just focuses on getting the job done.
For example, imagine a simple e-commerce application. The Model would handle tasks like fetching product information from a database, updating product stock levels after a sale, or calculating the total price of an order. Let’s say we have a database table called `products` with columns like `id`, `name`, `price`, and `stock`. A Model method might look something like this (using Python with a hypothetical database interaction):
“`python
class ProductModel:
def get_product(self, product_id):
# Hypothetical database interaction – replace with your actual database library
query = “SELECT * FROM products WHERE id = %s”
# Execute the query and return the product data
# … database interaction logic …
return product_data
“`
View Responsibilities
The View is all about presentation. It takes the data provided by the Model and displays it in a user-friendly format. It’s the front-end face of your application, responsible for creating the user interface (UI). Think of it as the stylish storefront that showcases your products. The View is purely presentational; it doesn’t contain any business logic or data manipulation.
Let’s illustrate this with a simple HTML example displaying a product from our e-commerce application:
“`html
Name: product.name
Price: $ product.price
Stock: product.stock
“`
This HTML snippet showcases product information. The ` product.name `, ` product.price `, and ` product.stock ` parts would be dynamically populated by the Controller using data fetched from the Model.
Controller Responsibilities
The Controller acts as the intermediary between the Model and the View. It receives user input, updates the Model accordingly, and then selects the appropriate View to display the updated data. It’s the stage manager, orchestrating the interaction between the actors (Model and View).
Let’s look at a simple Python example demonstrating controller logic for adding a new product:
“`python
class ProductController:
def add_product(self, request):
# Get product data from the request (e.g., from a form submission)
name = request.get(‘name’)
price = request.get(‘price’)
stock = request.get(‘stock’)
# Create a new product using the Model
product = ProductModel().create_product(name, price, stock)
# Redirect to a view displaying the newly added product
return redirect(‘/products/’ + str(product.id))
“`
Simple To-Do List MVC Structure, Understanding the MVC Design Pattern in Web Development
Here’s a simple MVC structure for a basic to-do list application:
Component | Responsibility | Example Code Snippet (Python) | Interaction with other Components |
---|---|---|---|
Model | Manages to-do items (add, remove, mark as complete). Interacts with a database (or in-memory storage). | class ToDoModel: def add_item(self, item): ... def remove_item(self, item_id): ... |
Provides data to the Controller; receives updates from the Controller. |
View | Displays the to-do list to the user, showing items and their status. |
|
Receives data from the Controller and displays it. |
Controller | Handles user actions (adding, removing, marking items). Updates the Model and selects the appropriate View. | class ToDoController: def add_item(self, request): item = request.get('item'); self.model.add_item(item); return self.view.render_list() |
Receives user input, updates the Model, and chooses the View. |
Advantages of using MVC
So, you’ve grasped the basics of MVC – Model-View-Controller. But why bother? Why choose this architectural pattern over others? The answer lies in its inherent advantages, particularly when it comes to building robust, scalable, and maintainable web applications. Let’s dive into the key benefits.
MVC offers a significant boost in terms of maintainability and scalability. This means easier updates, less downtime, and the ability to handle increasing user traffic and data volumes without significant performance degradation. The separation of concerns is the magic ingredient here, allowing developers to work on different parts of the application independently, reducing conflicts and speeding up development cycles.
Maintainability and Scalability
The core strength of MVC lies in its separation of concerns. The model handles data, the view displays it, and the controller manages the interaction. This modular design makes it incredibly easy to maintain and update the application. Imagine needing to change the database structure – with MVC, you primarily focus on updating the model, leaving the view and controller largely untouched. Similarly, scaling is simplified; you can independently scale the database server (related to the model) or the web servers handling the views and controllers, optimizing resource allocation for peak performance. This modularity also promotes code reusability, saving time and effort in the long run. For instance, a well-structured model can be easily integrated into different views or even used in other projects.
Comparison with MVP and MVVM
MVC isn’t the only architectural pattern in town. MVP (Model-View-Presenter) and MVVM (Model-View-ViewModel) are popular alternatives. While they share similarities, key differences exist. In MVP, the presenter acts as an intermediary between the model and the view, handling all logic and updates. This results in a more tightly coupled system compared to MVC. MVVM introduces a ViewModel that acts as a data transformation layer between the model and the view, often using data binding techniques for enhanced efficiency. MVVM is particularly well-suited for applications with complex user interfaces. The choice between these patterns depends on the specific needs of the project; MVC’s simplicity and broad applicability make it a solid choice for many web development scenarios.
Improved Code Organization and Reduced Complexity
MVC’s clear separation of concerns translates directly into cleaner, more organized code. Each component has a specific responsibility, making the codebase easier to understand, navigate, and debug. This reduction in complexity leads to faster development, easier collaboration among developers, and a lower likelihood of introducing bugs. The independent testability of each component is another significant advantage. You can unit test the model, the view, and the controller separately, ensuring the reliability of each part and the overall application. This streamlined approach contributes to a more maintainable and scalable application over its lifespan.
MVC Frameworks and Libraries
So, you’ve grasped the core concepts of MVC – Model, View, Controller. That’s awesome! But building a web application from scratch using only the raw MVC principles? Let’s just say it’s a marathon, not a sprint. That’s where MVC frameworks and libraries swoop in to save the day, offering pre-built components and structures to streamline development. Think of them as the scaffolding for your MVC masterpiece.
Ready to explore the world of pre-built MVC solutions? Let’s dive into some popular options and weigh their pros and cons.
Popular MVC Frameworks
Choosing the right MVC framework can significantly impact your project’s success. Several robust frameworks offer distinct advantages depending on your needs and preferences. Here are a few prominent examples:
- Ruby on Rails: Known for its elegance and developer-friendly approach, Rails emphasizes convention over configuration, speeding up development significantly. It’s particularly popular for rapid prototyping and building web applications quickly. Its large community provides ample support and resources.
- Laravel: A PHP framework renowned for its clean syntax and expressive features. Laravel offers a robust ecosystem of tools and packages, simplifying complex tasks like routing, database interactions, and templating. Its elegant design and ease of use make it a favorite among PHP developers.
- ASP.NET MVC: Microsoft’s contribution to the MVC world, this framework is deeply integrated with the .NET ecosystem. It provides a structured approach to building web applications using C# and offers excellent tooling support within Visual Studio. It’s a strong choice for enterprise-level applications.
- Spring MVC: A Java-based framework that’s part of the larger Spring ecosystem. It offers a flexible and highly configurable approach to building web applications, making it suitable for a wide range of projects. Its mature ecosystem and strong community support make it a reliable choice for large-scale applications.
Advantages of Using an MVC Framework
Using an established MVC framework offers several compelling advantages:
- Increased Development Speed: Frameworks provide pre-built components and structures, reducing the amount of code you need to write from scratch. This leads to faster development cycles and quicker time to market.
- Improved Code Organization and Maintainability: The structured nature of MVC frameworks promotes cleaner, more organized code, making it easier to maintain and update your application over time. This is crucial for long-term projects.
- Enhanced Security: Many frameworks incorporate built-in security features, helping to protect your application from common vulnerabilities. This reduces the risk of security breaches and protects user data.
- Large Community Support: Popular frameworks boast large and active communities, providing ample resources, tutorials, and support when you encounter issues.
Disadvantages of Using an MVC Framework
While MVC frameworks offer numerous benefits, it’s important to acknowledge potential drawbacks:
- Learning Curve: Mastering a new framework requires time and effort. The initial learning curve can be steep, especially for developers new to MVC or the specific framework.
- Less Control: Using a framework means you have less direct control over the underlying architecture and implementation details. This can be limiting in certain situations.
- Overhead: Frameworks often introduce some overhead, potentially impacting performance in resource-constrained environments. This is generally negligible for most applications, but it’s a factor to consider.
- Vendor Lock-in: Switching frameworks later can be challenging and time-consuming, potentially leading to vendor lock-in.
Choosing the Right MVC Framework
Selecting the appropriate framework depends on several key factors:
- Project Requirements: Consider the scale, complexity, and specific needs of your project. A simple application might not require the power of a heavyweight framework.
- Team Expertise: Choose a framework your team is familiar with or can easily learn. This minimizes the learning curve and speeds up development.
- Community Support: A large and active community ensures readily available resources and support when you need it.
- Long-Term Maintainability: Consider the framework’s maturity and long-term support. A well-maintained framework ensures your application will remain functional and up-to-date for years to come.
Implementing MVC in a Web Application
So, you’ve grasped the MVC concept – congrats! Now, let’s get our hands dirty and see how it all comes together in a real-world web application. Building even a simple app will solidify your understanding and show you the power of this design pattern. We’ll walk through a basic blog application, but the principles apply broadly.
Implementing MVC isn’t about complex coding; it’s about structuring your code for maintainability and scalability. Think of it like building with LEGOs – individual, well-defined pieces that snap together neatly. This makes it easier to build bigger, more complex projects without things falling apart.
A Step-by-Step Guide to Implementing a Simple MVC Application
Let’s build a tiny blog application. We’ll keep it super basic to illustrate the core MVC principles. This guide assumes you have a basic understanding of a server-side language (like PHP, Python, or Node.js) and HTML.
- Model: Create a class (or object) representing a blog post. This class will handle data storage and retrieval (e.g., from a database or a simple text file). It might have properties like `title`, `content`, `author`, and `date`. Methods would include functions to create, read, update, and delete posts.
- View: Design the user interface. This is your HTML, CSS, and potentially JavaScript. The view receives data from the controller and displays it to the user. Think of a simple template for displaying a single blog post, with placeholders for the title, content, author, and date. Multiple views could exist (e.g., a list of posts, a single post view, a comment form).
- Controller: This acts as the intermediary. It receives user requests (e.g., a request to view a specific blog post), interacts with the model to fetch the necessary data, and then selects the appropriate view to display the data to the user. For our example, a controller might handle requests for viewing a blog post list or a single post. It would fetch data from the model and pass it to the relevant view.
- Putting it Together: When a user requests a blog post, the controller gets the request, calls the model to fetch the post data, and then renders the data using the view template. The user sees the nicely formatted blog post.
Designing a User Interface for a Basic Blog Application
Imagine a simple blog layout. The homepage displays a list of blog post titles with short excerpts and dates. Each title is a link to the individual post’s page. Clicking a title takes the user to a page displaying the full post content, author, and date. This simple design neatly demonstrates the separation of concerns: the model handles data, the view displays it, and the controller manages the flow.
The data flow would be: User clicks a link -> Controller receives the request -> Controller fetches the post data from the Model -> Controller sends the data to the View -> View renders the post details in HTML -> User sees the complete blog post.
Common Challenges in Implementing MVC and Their Solutions
Even with its advantages, MVC implementation isn’t always a walk in the park. Here are some common hurdles and how to navigate them:
- Overly Complex Models: A model that tries to do too much can become unwieldy. The solution? Break down large models into smaller, more manageable ones. Each model should focus on a specific aspect of your data.
- Fat Controllers: Controllers that handle too much logic can become difficult to maintain. The solution? Delegate tasks to the model (data access) or create helper classes to handle specific functionalities. Keep controllers lean and focused on routing and data flow.
- Tight Coupling: If your components are too interdependent, changes in one area might necessitate changes in many others. The solution? Favor loose coupling. Use interfaces and dependency injection to reduce direct dependencies between components. This allows for greater flexibility and easier testing.
- Testing Difficulties: Thorough testing is crucial, but tightly coupled components make testing harder. The solution? Focus on creating testable components. Use mocking and unit testing frameworks to isolate and test individual parts of your application.
Advanced MVC Concepts
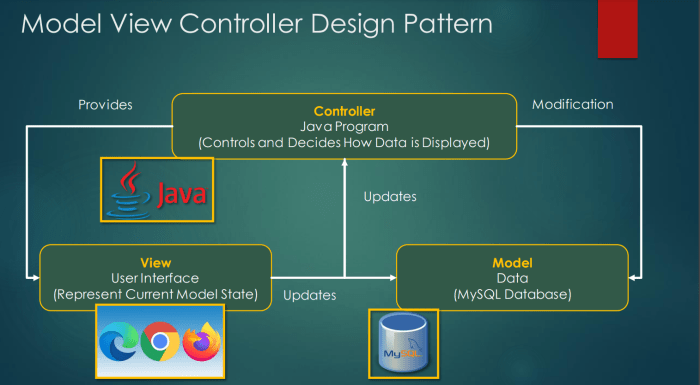
Source: cheggcdn.com
So, you’ve grasped the basics of MVC—congratulations! But the real power of this architectural pattern unfolds when you delve into its more advanced features. This section explores crucial aspects that elevate your MVC applications from simple to sophisticated, paving the way for building robust and scalable web solutions. We’ll be examining how routing, model binding, data validation, and RESTful APIs work together to create a truly powerful and efficient web application.
Routing and URL Mapping
Routing is the process of mapping incoming URLs to specific controller actions. It’s the backbone of how your application responds to user requests. Efficient routing ensures clean URLs, improves , and makes your application more maintainable. Different frameworks handle routing differently. For example, in Ruby on Rails, routes are defined in a `config/routes.rb` file using a Domain Specific Language (DSL). A simple route might look like this: `get ‘/products/:id’, to: ‘products#show’`. This maps a GET request to `/products/123` to the `show` action in the `ProductsController`, passing `123` as the `id` parameter. In contrast, frameworks like ASP.NET MVC utilize attributes on controller actions to define routes, offering a more code-centric approach. A similar route in ASP.NET MVC might be defined using the `[Route(“products/id”)]` attribute on the `Show` action within the `ProductsController`. This flexibility allows developers to choose the method best suited to their project’s needs and scale.
Model Binding and Data Validation
Model binding is the automatic conversion of incoming HTTP requests (like form submissions) into strongly typed objects (your models). This eliminates the tedious manual parsing of request parameters, significantly reducing boilerplate code and improving maintainability. For instance, if a user submits a form with fields for `Name` and `Email`, the framework automatically populates a corresponding `User` model object with these values. Data validation is crucial to ensure the integrity of your application’s data. This involves checking that the data received from the user meets specific criteria (e.g., email format, length restrictions, required fields). Many frameworks provide built-in validation attributes or mechanisms. In ASP.NET MVC, you might use data annotations like `[Required]`, `[EmailAddress]`, and `[StringLength]` directly on your model properties. Rails offers similar capabilities through validations defined within the model itself. Robust validation prevents invalid data from entering your database, safeguarding data integrity and improving the overall user experience.
RESTful APIs within an MVC Architecture
RESTful APIs are a prevalent architectural style for building web services. They leverage standard HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources. Within an MVC architecture, controllers often act as the interface for these APIs. A RESTful API endpoint might look like `/products/123`, where a GET request retrieves details for product with ID 123, a PUT request updates it, and a DELETE request removes it. The model handles the data persistence, ensuring data consistency and separation of concerns. Many modern MVC frameworks seamlessly integrate with RESTful API development, providing features like automatic JSON serialization and deserialization, simplifying the process of building and consuming these APIs. For example, a typical response from a RESTful API in JSON format might look like this: `”id”: 123, “name”: “Example Product”, “price”: 29.99`. This structured format is easily consumed by other applications and services.
Security Considerations in MVC: Understanding The MVC Design Pattern In Web Development
Building a web application using the MVC pattern is awesome – it’s structured, maintainable, and generally a breeze to work with. But don’t get too comfy! Even the most elegant MVC architecture can be vulnerable to security threats if you’re not careful. Let’s dive into the crucial security aspects you need to nail down to protect your application and your users.
MVC applications, while architecturally sound, inherit the security vulnerabilities of any web application. The separation of concerns, a core strength of MVC, doesn’t magically eliminate security risks; it simply helps organize the defense against them. A robust security strategy is crucial, and this requires proactive measures throughout the development lifecycle, from design to deployment and beyond.
Common MVC Vulnerabilities and Mitigation Strategies
Several common vulnerabilities can plague MVC applications. Understanding these threats and implementing appropriate countermeasures is paramount to building secure systems. Ignoring these risks can lead to serious consequences, including data breaches, financial losses, and reputational damage.
- SQL Injection: This classic attack involves injecting malicious SQL code into input fields to manipulate database queries. Mitigation: Always use parameterized queries or prepared statements. Never directly concatenate user input into SQL queries.
- Cross-Site Scripting (XSS): XSS attacks allow attackers to inject malicious scripts into web pages viewed by other users. Mitigation: Implement robust input validation and output encoding (escaping) to neutralize potentially harmful scripts. Use a Content Security Policy (CSP) to further restrict the execution of scripts.
- Cross-Site Request Forgery (CSRF): CSRF attacks trick users into performing unwanted actions on a website they’re already authenticated to. Mitigation: Use CSRF tokens (unique, unpredictable values) embedded in forms and verified on the server-side before processing requests.
- Session Hijacking: Attackers steal a user’s session ID to impersonate them. Mitigation: Use secure cookies (HTTPS only, HttpOnly flag), regenerate session IDs regularly, and implement robust authentication mechanisms, such as multi-factor authentication (MFA).
Input Validation and Sanitization
Input validation and sanitization are fundamental to preventing many common web application vulnerabilities. By carefully examining and cleaning user-supplied data before it reaches your application’s core logic, you can significantly reduce the risk of attacks.
Validation ensures that the data conforms to expected formats and constraints. Sanitization removes or neutralizes potentially harmful characters. For instance, before storing user-provided data in a database, you should validate that it’s within the allowed length, data type, and format. Then, sanitize the data to prevent injection attacks. This two-pronged approach forms the bedrock of secure input handling in MVC applications.
Secure Session Management
Secure session management is critical for protecting user data and maintaining the integrity of your application. Sessions store sensitive information about authenticated users, and compromising them can have severe consequences.
Employing HTTPS for all communication is essential. The use of HttpOnly cookies prevents client-side JavaScript from accessing session IDs, mitigating some XSS attacks. Regular session ID regeneration adds another layer of protection by invalidating old sessions and making it harder for attackers to exploit stolen IDs. Finally, setting appropriate session timeouts helps limit the window of vulnerability if a session is compromised.
Outcome Summary
Mastering the MVC design pattern is a game-changer for any web developer. By understanding the separation of concerns and leveraging the power of MVC frameworks, you can build more robust, maintainable, and scalable web applications. From simple to-do lists to complex e-commerce platforms, the principles of MVC provide a solid foundation for success. So, dive in, experiment, and watch your web development skills soar to new heights!