Getting Started with C++: A Beginner’s Guide – sounds intimidating, right? Wrong! This isn’t some arcane ritual reserved for coding ninjas. We’re peeling back the curtain on the world of C++, revealing its power and elegance in a way that’s actually…fun. Forget the jargon, we’re diving headfirst into the basics, from setting up your coding environment to building your first programs. Think of it as your crash course to becoming a C++ whisperer.
This guide will walk you through the fundamentals of C++, starting with a gentle introduction to its history and capabilities. We’ll compare it to other popular languages like Python and Java, helping you understand where C++ shines (and where it might not be the best fit). Then, we’ll get our hands dirty, setting up your development environment and building that classic “Hello, World!” program. From there, we’ll tackle essential concepts like data types, operators, control flow, and functions, all explained with clear examples and practical applications. By the end, you’ll have a solid foundation to build upon and maybe even impress your friends with your newfound coding skills.
Introduction to C++
C++, a powerful and versatile programming language, has been shaping the technological landscape for decades. Understanding its history, capabilities, and limitations is crucial for any aspiring programmer. This section provides a foundational overview of C++, comparing it to other popular languages and highlighting its strengths and weaknesses.
C++ History and Purpose
Developed by Bjarne Stroustrup in the early 1980s as an extension of the C programming language, C++ aimed to enhance C’s capabilities with object-oriented programming (OOP) features. This addition allowed for more complex program structures, improved code reusability, and easier management of large projects. C++’s original purpose was to improve upon C’s limitations while retaining its efficiency. Its initial applications included system programming, game development, and high-performance computing – areas where its speed and control are highly valuable. Today, its applications span a vast array of domains, from embedded systems to large-scale applications.
Advantages and Disadvantages of C++
C++ offers several compelling advantages. Its performance is exceptionally high due to its close-to-hardware nature and manual memory management, making it ideal for performance-critical applications. Its versatility allows developers to work at various levels of abstraction, from low-level system programming to high-level application development. The extensive standard library and rich ecosystem of third-party libraries further enhance its capabilities. However, C++ also has disadvantages. The complexity of the language, especially its manual memory management, can lead to memory leaks and other errors if not handled carefully. The steeper learning curve compared to some other languages can be a barrier for beginners. The compilation process can also be slower compared to interpreted languages.
Comparison of C++ with Other Programming Languages
C++, Java, and Python represent distinct approaches to software development. C++ prioritizes performance and control, Java emphasizes platform independence and robust object-oriented programming, and Python values ease of use and rapid prototyping. The choice of language depends heavily on the project’s requirements. For example, a high-performance game engine would likely benefit from C++’s speed, while a web application might be better suited to Python’s rapid development capabilities. Java’s platform independence makes it a strong choice for cross-platform applications.
Language | Feature | C++ Implementation | Comparison Notes |
---|---|---|---|
C++ | Memory Management | Manual (using new and delete ) |
Offers fine-grained control but requires careful handling to avoid memory leaks. Java and Python use automatic garbage collection. |
Java | Object-Oriented Programming | Supports OOP principles like encapsulation, inheritance, and polymorphism. | Similar to C++, but with stricter enforcement of OOP concepts. |
Python | Ease of Use | Concise syntax and large standard library simplify development. | Significantly easier to learn and use than C++ but generally slower in execution. |
C++ | Performance | Compiled language, resulting in highly optimized executable code. | Generally faster than Java and Python, especially for computationally intensive tasks. |
Java | Platform Independence | Runs on the Java Virtual Machine (JVM), enabling cross-platform compatibility. | C++ requires separate compilation for different operating systems. Python also offers good cross-platform support. |
Python | Rapid Prototyping | Interpreted language with dynamic typing, enabling faster development cycles. | C++’s compilation process can be time-consuming, making it less suitable for rapid prototyping. |
Setting up the Development Environment
Getting your C++ coding environment ready is the first step on your programming journey. Think of it like setting up your artist’s studio before you start painting – you need the right tools and workspace to create your masterpiece. This section will guide you through installing a compiler and an IDE, making the process straightforward and painless. We’ll then walk through compiling and running a classic “Hello, World!” program to solidify your understanding.
Setting up your C++ development environment involves two key components: a compiler and an Integrated Development Environment (IDE). The compiler translates your human-readable C++ code into machine-readable instructions that your computer can understand and execute. An IDE provides a user-friendly interface for writing, compiling, debugging, and running your code, making the development process much more efficient.
Installing a C++ Compiler (g++)
The GNU Compiler Collection (GCC), specifically the g++ compiler, is a powerful and widely used free and open-source compiler for C++. It’s available for various operating systems, including Windows, macOS, and Linux. The installation process varies slightly depending on your operating system. For example, on Linux distributions like Ubuntu, you can typically install g++ using the system’s package manager (apt). On Windows, you might download the MinGW (Minimalist GNU for Windows) distribution, which includes g++. Once installed, you should be able to open your terminal or command prompt and type `g++ –version` to verify the installation. This command will display the version of g++ installed, confirming its successful setup.
Setting up an Integrated Development Environment (IDE)
An IDE significantly enhances your coding experience by providing a comprehensive environment for writing, compiling, debugging, and running your code. Popular choices include Visual Studio Code (VS Code) and Code::Blocks. Both are free, versatile, and offer extensive support for C++.
VS Code is a lightweight yet powerful editor that, with the right extensions, becomes a fully-fledged IDE. After installing VS Code, you’ll need to install the C++ extension from the marketplace. This extension provides features like IntelliSense (code completion), debugging support, and integration with various compilers.
Code::Blocks is a more traditional IDE, offering a built-in compiler and debugger. It’s a great option if you prefer a complete IDE out-of-the-box without needing to install additional extensions. The installation process for both VS Code and Code::Blocks usually involves downloading the installer from their respective websites and following the on-screen instructions.
Compiling and Running a “Hello, World!” Program
Let’s create a simple “Hello, World!” program to test your newly set up environment. This classic program is a fundamental starting point for any programming language.
First, create a new file named `hello.cpp` (or similar) in your chosen IDE or text editor. Then, add the following code:
#include
int main()
std::cout << "Hello, World!" << std::endl; return 0;
This code includes the iostream library (for input/output), defines a main function (where the program execution begins), prints “Hello, World!” to the console, and returns 0 to indicate successful execution.
To compile and run this program, you’ll typically use the compiler from your terminal or command prompt, or through the IDE’s built-in tools. If using the command line, navigate to the directory containing `hello.cpp` and use the following command:
g++ hello.cpp -o hello
This command compiles `hello.cpp` and creates an executable file named `hello`. To run the program, simply type:
./hello
(or `hello.exe` on Windows). This should print “Hello, World!” to your console. Your IDE will likely have a “build” and “run” option that automates this process.
Setting Up a C++ Development Environment: A Flowchart
Imagine a flowchart with these steps:
1. Start: A rounded rectangle representing the beginning of the process.
2. Choose Compiler: A rectangle representing the decision to select a C++ compiler (e.g., g++).
3. Install Compiler: A rectangle representing the installation process of the chosen compiler.
4. Choose IDE (Optional): A diamond representing a decision point; if an IDE is desired, proceed to the next step; otherwise, skip to step 6.
5. Install IDE: A rectangle representing the installation of the chosen IDE (e.g., VS Code, Code::Blocks).
6. Create a Source Code File: A rectangle representing the creation of a new C++ file (e.g., `hello.cpp`).
7. Write Code: A rectangle representing the process of writing the C++ code.
8. Compile Code: A rectangle representing the compilation of the C++ code using the compiler.
9. Run Executable: A rectangle representing the execution of the compiled code.
10. End: A rounded rectangle representing the end of the process.
The flowchart would visually depict the sequential steps involved in setting up the development environment, making it easier to follow the process. Arrows would connect the different shapes, indicating the flow of the process.
Basic Syntax and Data Types
C++ boasts a powerful and expressive syntax, allowing for intricate program design. Understanding its fundamental building blocks – data types and their manipulation – is crucial for any aspiring C++ programmer. This section will explore the core syntax rules and introduce the essential data types you’ll encounter frequently.
Fundamental Syntax Rules
C++ code follows a structured format. Statements are terminated by semicolons (;). Braces and define code blocks, such as those within functions or loops. Whitespace (spaces, tabs, newlines) generally doesn’t affect the program’s logic, enhancing readability. Comments, using // for single-line and /* … */ for multi-line comments, are essential for explaining code functionality. Proper indentation significantly improves code clarity and maintainability. For example, a simple `Hello, world!` program demonstrates these basics:
“`c++
#include
int main() // Main function block begins
std::cout << "Hello, world!" << std::endl; // Output statement
return 0; // Indicates successful execution
// Main function block ends
```
Data Types
C++ provides various data types to represent different kinds of information. Choosing the appropriate data type is vital for efficient memory management and accurate program behavior. Each type has a specific size and range of values it can hold.
Variables and Constants
Variables are named storage locations that hold data values. They are declared using a data type followed by the variable name. Constants, declared using the `const` , hold values that cannot be changed during program execution.
“`c++
int age = 30; // Integer variable
float price = 99.99; // Floating-point variable
const char initial = ‘J’; // Character constant
bool isAdult = true; // Boolean variable
“`
Data Type Summary
The following table summarizes common C++ data types, their typical sizes (which may vary slightly depending on the system architecture and compiler), and their uses.
Data Type | Size (bytes) | Typical Use |
---|---|---|
int |
4 | Whole numbers (e.g., age, count) |
float |
4 | Single-precision floating-point numbers (e.g., price, temperature) |
double |
8 | Double-precision floating-point numbers (e.g., scientific calculations, high precision) |
char |
1 | Single characters (e.g., initials, letters) |
bool |
1 | Boolean values (true or false) |
Operators and Expressions: Getting Started With C++: A Beginner’s Guide
Operators are the heart of any C++ program, enabling us to manipulate data and perform calculations. Understanding how operators work and their precedence is crucial for writing correct and efficient code. This section delves into the various types of operators and how they function within expressions.
Arithmetic Operators
Arithmetic operators perform standard mathematical calculations. These include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). The modulus operator returns the remainder of a division. For instance, 10 % 3 would result in 1. Be mindful of integer division: dividing two integers results in an integer, truncating any fractional part. For example, 7 / 2 equals 3, not 3.5. To get a floating-point result, at least one operand should be a floating-point number (e.g., 7.0 / 2).
Logical Operators
Logical operators are used to combine or manipulate boolean expressions (expressions that evaluate to true or false). The primary logical operators are AND (&&), OR (||), and NOT (!). The AND operator returns true only if both operands are true. The OR operator returns true if at least one operand is true. The NOT operator inverts the truth value of its operand. Consider this example: bool x = true; bool y = false; bool result = x && y; // result is false
.
Relational Operators
Relational operators compare two values and return a boolean result. These include less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), equal to (==), and not equal to (!=). It’s crucial to distinguish between the assignment operator (=) and the equality operator (==). The assignment operator assigns a value, while the equality operator compares values. Incorrect use of these can lead to subtle but significant bugs. For example, if (x = 5) /* This assigns 5 to x, always true */
versus if (x == 5) /* This compares x to 5 */
Operator Precedence
Operator precedence defines the order in which operators are evaluated in an expression. Operators with higher precedence are evaluated before those with lower precedence. For example, multiplication and division have higher precedence than addition and subtraction. Parentheses can be used to override the default precedence. Consider the expression 10 + 5 * 2
. Multiplication has higher precedence, so it’s evaluated first, resulting in 20. However, (10 + 5) * 2
evaluates to 30 because the parentheses force addition to be performed first. A complete operator precedence table is readily available in most C++ documentation.
Operator Overloading
Operator overloading allows you to redefine the behavior of operators for user-defined types (classes and structs). This lets you use operators in intuitive ways with your own custom data structures. For example, you could overload the + operator to add two objects of your custom class.
class MyComplex
public:
double real;
double imag;
MyComplex operator+(const MyComplex& other) const
return real + other.real, imag + other.imag;
;
int main()
MyComplex c1 = 1.0, 2.0;
MyComplex c2 = 3.0, 4.0;
MyComplex c3 = c1 + c2; //Uses overloaded + operator
return 0;
This code defines a `MyComplex` class and overloads the + operator to add two complex numbers. The overloaded operator takes another `MyComplex` object as input and returns a new `MyComplex` object representing the sum. This allows for a more natural and readable way to work with complex numbers.
Control Flow Statements
Controlling the order in which your C++ code executes is crucial for creating programs that perform complex tasks. Control flow statements allow you to dictate the flow of execution, branching to different parts of your code based on conditions or repeating sections of code. This section explores the fundamental control flow mechanisms in C++.
Conditional Statements: if, else if, and else
Conditional statements determine which block of code is executed based on a boolean condition (true or false). The `if` statement executes a block of code only if the condition is true. The `else if` statement provides a way to check multiple conditions sequentially, and the `else` statement executes a block of code if none of the preceding conditions are true.
#include <iostream>
int main()
int age = 20;
if (age < 18)
std::cout << "You are a minor." << std::endl;
else if (age >= 18 && age < 65)
std::cout << "You are an adult." << std::endl;
else
std::cout << "You are a senior citizen." << std::endl;
return 0;
This code snippet demonstrates a simple age-based conditional check. The output will vary depending on the value of the `age` variable. Note the use of the logical AND operator (`&&`) to combine multiple conditions within the `else if` statement.
Iteration Statements: for, while, and do-while
Iteration statements, or loops, allow you to repeat a block of code multiple times. The `for` loop is typically used when you know the number of iterations in advance. The `while` loop continues as long as a condition is true, and the `do-while` loop is similar to `while` but guarantees at least one execution of the loop body.
The `for` loop is ideal for iterating over arrays or performing a task a specific number of times. The general structure is: for (initialization; condition; increment/decrement) /* code to be executed */
The `while` loop is suitable when the number of iterations isn’t known beforehand. It checks the condition before each iteration. The structure is: while (condition) /* code to be executed */
The `do-while` loop is similar to `while`, but the condition is checked at the end of each iteration, ensuring at least one execution. The structure is: do /* code to be executed */ while (condition);
Switch Statements
The `switch` statement provides a concise way to select one block of code to execute from multiple cases based on the value of an integer expression. It’s particularly useful when you have multiple possible values and want to avoid lengthy `if-else if-else` chains.
#include <iostream>
int main()
int day = 3;
switch (day)
case 1:
std::cout << "Monday" << std::endl;
break;
case 2:
std::cout << "Tuesday" << std::endl;
break;
case 3:
std::cout << "Wednesday" << std::endl;
break;
default:
std::cout << "Other day" << std::endl;
return 0;
This example shows how a `switch` statement can efficiently handle different days of the week. The `break` statement is crucial to prevent fallthrough to subsequent cases. The `default` case handles situations where the expression doesn’t match any of the specified cases.
Nested Loops and Pattern Generation
Nested loops involve placing one loop inside another. This technique is powerful for creating patterns or processing multi-dimensional data structures.
Here’s a program that uses nested loops to generate a simple pattern:
#include <iostream>
int main()
for (int i = 1; i <= 5; ++i)
for (int j = 1; j <= i; ++j)
std::cout << "*";
std::cout << std::endl;
return 0;
This code will produce the following output:
*
*
*
The outer loop controls the number of rows, while the inner loop controls the number of asterisks printed in each row. This demonstrates a basic pattern; more complex patterns can be created using similar nested loop structures with conditional logic.
Functions
Functions are the building blocks of well-structured C++ programs. They allow you to break down complex tasks into smaller, more manageable, and reusable pieces of code. This modular approach improves code readability, maintainability, and efficiency, making your programs easier to understand, debug, and extend. Think of them as mini-programs within your main program.
Functions encapsulate a specific task, promoting code reusability. Instead of writing the same code multiple times, you can define a function once and call it whenever needed. This reduces redundancy and makes your code more concise. Moreover, well-defined functions improve the overall organization and structure of your program, making it easier for others (and your future self!) to understand and modify.
Function Definition and Syntax
A function in C++ is defined using a specific syntax. It consists of a return type, the function name, a parameter list (enclosed in parentheses), and a function body (enclosed in curly braces). The return type specifies the data type of the value the function returns; if the function doesn’t return a value, the return type is `void`. The parameter list declares the variables the function accepts as input. The function body contains the code that performs the function’s task.
Here’s a general structure:
return_type function_name(parameter_type parameter1, parameter_type parameter2, ...)
// Function body: statements to perform the task
return value; // If the return type is not void
For example, a function to add two integers would look like this:
int add(int a, int b)
return a + b;
Function Parameters and Return Values
Function parameters allow you to pass data into the function. These parameters act as local variables within the function’s scope. Return values allow the function to send data back to the part of the program that called it. The return type of a function determines the type of value it can return. A function can return only one value, but this value can be a complex data structure.
For instance, consider a function that calculates the area of a rectangle:
double calculateArea(double length, double width)
return length * width;
This function takes two `double` parameters (length and width) and returns their product (the area) as a `double`.
Example: Calculating the Factorial
The factorial of a non-negative integer n (denoted by n!) is the product of all positive integers less than or equal to n. For example, 5! = 5 * 4 * 3 * 2 * 1 = 120. Let’s create a C++ function to calculate the factorial:
long long factorial(int n)
if (n == 0)
return 1;
else
long long result = 1;
for (int i = 1; i <= n; i++) result *= i; return result;
This function takes an integer `n` as input and returns its factorial as a `long long` to handle larger factorial values. The function handles the base case (n=0) where the factorial is 1. Otherwise, it iteratively calculates the factorial using a loop. Note the use of `long long` to avoid integer overflow for larger values of `n`.
Arrays and Strings
Arrays and strings are fundamental data structures in C++, offering efficient ways to manage collections of data. Understanding how to use them is crucial for building more complex and powerful programs. This section will cover the basics of array and string manipulation in C++, equipping you with the tools to handle various data processing tasks.
Array Declaration and Access
In C++, an array is a contiguous block of memory that stores elements of the same data type. Arrays are declared by specifying the data type, the array name, and the number of elements enclosed in square brackets. For example, `int numbers[5];` declares an array named `numbers` that can hold 5 integers. Accessing individual elements is done using their index, starting from 0. So, `numbers[0]` refers to the first element, `numbers[1]` to the second, and so on. Attempting to access an element outside the defined bounds (e.g., `numbers[5]` in this case) leads to undefined behavior, often resulting in a program crash.
Array Manipulation
Once an array is declared, its elements can be initialized directly or assigned values later in the program. For example:
int scores[3] = 85, 92, 78; // Initialization during declaration
int ages[5];
ages[0] = 25;
ages[1] = 30;
ages[2] = 22;
ages[3] = 28;
ages[4] = 35; // Assigning values individually
Arrays can be used in loops to process their elements efficiently. For instance, to calculate the sum of elements in the `scores` array:
int sum = 0;
for (int i = 0; i < 3; i++)
sum += scores[i];
String Manipulation with Standard Library Functions
Strings in C++ are essentially arrays of characters, but they are often handled using the `
String Manipulation Examples
The following code demonstrates some common string manipulation techniques:
#include
#include
int main()
std::string message = "Hello, world!";
std::string name = "Alice";
std::cout << message << std::endl; // Output: Hello, world! std::cout << message.length() << std::endl; // Output: 13 (length of the string) std::string greeting = message + ", " + name + "!"; // Concatenation std::cout << greeting << std::endl; // Output: Hello, world!, Alice! std::string sub = message.substr(7, 5); // Extract substring "world" std::cout << sub << std::endl; // Output: world size_t pos = message.find("world"); // Find the position of "world" std::cout << pos << std::endl; // Output: 7 (index of "world") return 0;
This example shows how to use `length()`, string concatenation using the `+` operator, `substr()` for substring extraction, and `find()` for searching within a string. These are just a few of the many functions offered by the `std::string` class. Refer to C++ documentation for a complete list.
A Program Demonstrating Array and String Manipulation, Getting Started with C++: A Beginner's Guide
Let's create a program that takes names as input from the user, stores them in an array, and then prints a greeting message for each person.
#include
#include
int main()
const int numPeople = 3;
std::string names[numPeople];
for (int i = 0; i < numPeople; i++) std::cout << "Enter name " << i + 1 << ": "; std::getline(std::cin, names[i]); // Use getline to handle spaces in names for (int i = 0; i < numPeople; i++) std::cout << "Hello, " << names[i] << "!" << std::endl; return 0;
This program demonstrates both array usage (to store names) and string manipulation (to create personalized greetings). The use of `std::getline` is crucial for correctly handling names that contain spaces.
Pointers
Pointers are a fundamental concept in C++ that allows you to work directly with memory addresses. Understanding pointers unlocks a deeper level of control over how your program interacts with data, enabling you to create more efficient and flexible code. Think of a pointer as a variable that holds the memory address of another variable. This seemingly simple idea opens up powerful possibilities, especially when dealing with large datasets or dynamic memory allocation.
Pointers and Memory Addresses
A pointer's core function is to store the memory address where a specific data element resides. Every variable in your program is stored at a particular location in your computer's memory. This location is represented by a unique memory address. A pointer variable holds this address, allowing you to indirectly access and manipulate the data at that location. This indirect access is what makes pointers so versatile and powerful, but also potentially tricky for beginners.
Pointer Declaration and Usage
Declaring a pointer involves specifying the data type of the variable it will point to, followed by an asterisk (*) and the pointer's name. For example, `int *ptr;` declares a pointer named `ptr` that can hold the address of an integer variable. To assign a memory address to a pointer, you use the address-of operator (&). For instance, if `int num = 10;`, then `ptr = #` assigns the memory address of `num` to `ptr`. You can then access the value stored at the address held by `ptr` using the dereference operator (*), like this: `int value = *ptr;`. This will assign the value of `num` (which is 10) to `value`.
Pointers and Arrays
Pointers and arrays are intrinsically linked in C++. An array name, when used in an expression (except when used with the `sizeof` operator or the unary `&` operator), decays into a pointer to its first element. This means that if you have an array `int arr[5] = 1, 2, 3, 4, 5;`, then `arr` acts as a pointer to `arr[0]`. You can access array elements using pointer arithmetic. For example, `*(arr + 1)` accesses the second element (value 2), equivalent to `arr[1]`. This pointer arithmetic allows for efficient traversal and manipulation of array elements.
Dynamic Memory Allocation
Dynamic memory allocation allows you to allocate memory during runtime, as opposed to static allocation where memory is assigned at compile time. This is crucial for situations where you don't know the exact amount of memory needed beforehand. The `new` operator allocates memory from the heap, while `delete` deallocates it. For example, `int *dynArr = new int[10];` allocates space for an array of 10 integers. Remember to always use `delete[] dynArr;` to release the allocated memory when it's no longer needed to prevent memory leaks. Failing to do so can lead to program instability and resource exhaustion. Consider a scenario where you're processing a file of unknown size: dynamic allocation lets you allocate memory as needed, reading the file chunk by chunk. Without it, you'd have to guess the maximum file size, potentially wasting memory or risking a crash if the file exceeds your guess.
Introduction to Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a powerful programming paradigm that organizes code around "objects" rather than functions and logic. This approach makes code more modular, reusable, and easier to maintain, especially for larger and more complex projects. Understanding OOP principles is crucial for writing efficient and scalable C++ applications.
Core Principles of OOP
OOP revolves around three core principles: encapsulation, inheritance, and polymorphism. Encapsulation bundles data and methods that operate on that data within a single unit, called a class. Inheritance allows creating new classes (derived classes) based on existing ones (base classes), inheriting their properties and behaviors. Polymorphism enables objects of different classes to respond to the same method call in their own specific way. These principles work together to create flexible and robust software designs.
Classes and Objects
A class serves as a blueprint for creating objects. It defines the data (member variables) and the functions (member functions or methods) that objects of that class will possess. An object is an instance of a class; it's a concrete realization of the blueprint. For example, consider a `Car` class. It might have member variables like `color`, `model`, and `speed`, and methods like `accelerate()`, `brake()`, and `turn()`. Each individual car you create would be an object of the `Car` class, each with its own specific values for `color`, `model`, and `speed`.
Constructors and Destructors
Constructors are special member functions that are automatically called when an object of a class is created. They initialize the object's member variables. Destructors are also special member functions that are automatically called when an object is destroyed (goes out of scope or is explicitly deleted). They perform cleanup tasks, such as releasing memory allocated by the object. For instance, a `Car` class constructor might initialize the `speed` to 0, while the destructor might handle the release of any dynamically allocated memory used by the car object.
Example: A Simple Class
Let's create a simple `Dog` class to illustrate these concepts.
```c++
#include
#include
class Dog
private:
std::string name;
std::string breed;
int age;
public:
// Constructor
Dog(std::string dogName, std::string dogBreed, int dogAge) : name(dogName), breed(dogBreed), age(dogAge)
// Method to bark
void bark()
std::cout << "Woof!" << std::endl;
// Method to display dog information
void displayInfo()
std::cout << "Name: " << name << ", Breed: " << breed << ", Age: " << age << std::endl;
// Destructor (optional in this simple example, but good practice)
~Dog()
std::cout << "Dog object destroyed." << std::endl;
;
int main()
Dog myDog("Buddy", "Golden Retriever", 3);
myDog.bark();
myDog.displayInfo();
return 0;
```
This code defines a `Dog` class with private member variables for name, breed, and age, and public methods for barking and displaying information. The constructor initializes these variables when a `Dog` object is created. The destructor (although simple in this case) shows a good practice for cleanup. The `main` function creates a `Dog` object and uses its methods. The output clearly demonstrates the object's properties and actions.
Final Wrap-Up
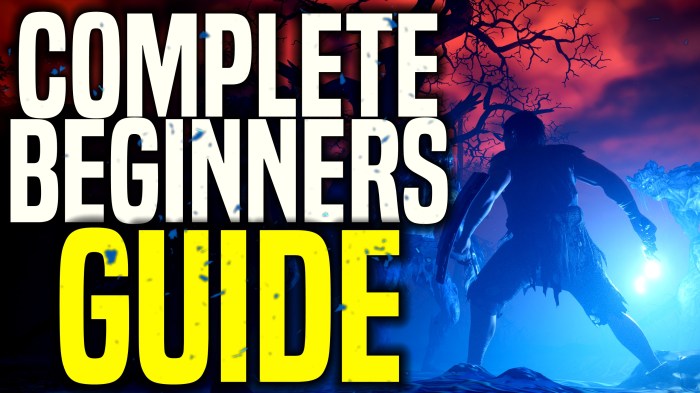
Source: deltiasgaming.com
So, you've conquered the basics of C++. You've wrestled with variables, tamed loops, and even befriended pointers. Congratulations! This journey has just begun. Remember, mastering any programming language takes time and practice. Don't be afraid to experiment, make mistakes (lots of them!), and seek out help when needed. The online C++ community is vast and supportive. The world of software development is now within your reach – go forth and code!
So you're diving into the world of programming with "Getting Started with C++: A Beginner's Guide"? That's awesome! Just like learning to code requires planning and foresight, securing your future requires similar thoughtfulness. Check out this article on The Role of Life Insurance in Providing for Your Family’s Needs to understand how to build a solid financial foundation, then get back to conquering those C++ pointers!