How to Master Object-Oriented Programming in Python: Dive into the elegant world of OOP and unlock Python’s true potential. This isn’t your grandma’s programming; we’re talking about building robust, scalable, and downright awesome applications. Forget spaghetti code – learn to craft clean, maintainable structures using classes, objects, and inheritance. We’ll unravel the mysteries of encapsulation, polymorphism, and abstraction, transforming you from a coding novice to a Python OOP ninja.
This guide breaks down complex OOP concepts into digestible chunks, complete with practical examples and real-world applications. We’ll cover everything from the fundamental principles to advanced techniques like metaclasses and decorators. Get ready to level up your Python game and build software that’s not just functional, but truly impressive.
Understanding Core OOP Concepts in Python: How To Master Object-Oriented Programming In Python
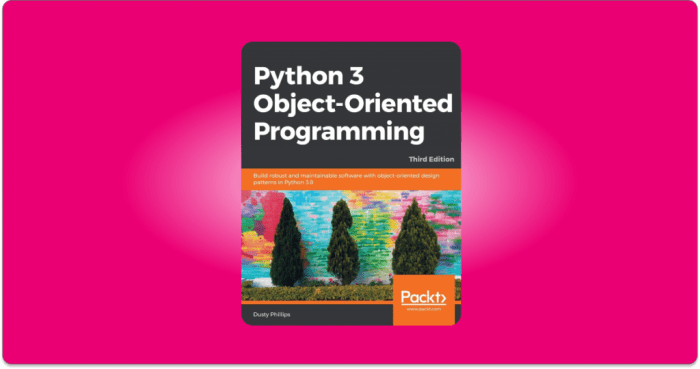
Source: howtolearnmachinelearning.com
Object-Oriented Programming (OOP) is a powerful programming paradigm that organizes code around “objects” rather than functions and logic. Mastering OOP in Python unlocks the ability to write cleaner, more maintainable, and scalable code, especially for complex projects. This section dives into the core concepts that underpin OOP, providing a clear understanding of their practical applications.
The Four Pillars of OOP
Object-oriented programming rests on four fundamental principles: encapsulation, inheritance, polymorphism, and abstraction. Understanding these principles is crucial for effectively utilizing the power of OOP.
Encapsulation bundles data (attributes) and methods (functions) that operate on that data within a single unit, the class. This protects data integrity and simplifies code organization. Think of it as a capsule containing everything needed for a specific task. In Python, we achieve encapsulation using access modifiers like underscores (`_` for protected and `__` for private attributes).
Inheritance allows creating new classes (child classes) based on existing ones (parent classes). Child classes inherit attributes and methods from their parent, promoting code reusability and reducing redundancy. This is like building upon pre-existing blueprints, adding or modifying features as needed.
Polymorphism means “many forms.” It allows objects of different classes to respond to the same method call in their own specific way. This enables flexibility and extensibility in your code. For instance, different animal classes (Dog, Cat) might both have a `makeSound()` method, but each would implement it differently.
Abstraction hides complex implementation details and exposes only essential information to the user. This simplifies interaction with objects and reduces complexity. Think of a car: you interact with the steering wheel, gas pedal, and brakes, without needing to understand the intricate mechanics under the hood.
Python Example Illustrating Encapsulation
Let’s create a simple `BankAccount` class to demonstrate encapsulation:
“`python
class BankAccount:
def __init__(self, account_number, balance):
self._account_number = account_number # Protected attribute
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def withdraw(self, amount):
if self.__balance >= amount:
self.__balance -= amount
else:
print(“Insufficient funds”)
def get_balance(self):
return self.__balance
account = BankAccount(“12345”, 1000)
print(account.get_balance()) # Accessing balance through a method
#print(account.__balance) # This would raise an AttributeError because __balance is private
“`
Here, `_account_number` and `__balance` are protected and private attributes respectively, limiting direct access and enforcing controlled modification through methods.
Procedural vs. Object-Oriented Programming
Procedural programming focuses on procedures or functions, while OOP centers around objects. Procedural programs are often simpler for small tasks, but OOP excels in managing complexity. In procedural programming, data and functions are separate, leading to potential data inconsistencies. OOP, with its encapsulation, maintains data integrity better. OOP’s inheritance and polymorphism promote code reusability and flexibility, advantages absent in procedural approaches.
Practical Scenarios Where OOP Excels
OOP shines in scenarios involving complex data structures and interactions, such as game development (managing characters, items, and game world), graphical user interfaces (GUI) (handling user input and window interactions), and large-scale software projects (modular design and maintainability). In these cases, the organizational structure and code reusability provided by OOP significantly simplify development and maintenance compared to a procedural approach.
Inheritance and Polymorphism
Level up your Python game by understanding inheritance and polymorphism – two powerful OOP concepts that let you build flexible and reusable code. Think of them as superpowers for your classes, allowing you to create a structured and efficient program. We’ll explore how these concepts work, showing you practical examples that will make your coding life significantly easier.
Inheritance: Building Upon Existing Classes
Inheritance is all about creating new classes (child classes) based on existing ones (parent classes). The child class inherits the attributes and methods of the parent, allowing for code reuse and a clear hierarchical structure. This avoids redundant code and promotes better organization. Python supports single, multiple, and multilevel inheritance, providing flexibility in designing class relationships.
Single Inheritance
In single inheritance, a child class inherits from only one parent class. Imagine you have a `Vehicle` class with attributes like `color` and `speed`. You can create a `Car` class that inherits from `Vehicle`, adding car-specific attributes like `num_doors`.
“`python
class Vehicle:
def __init__(self, color, speed):
self.color = color
self.speed = speed
class Car(Vehicle):
def __init__(self, color, speed, num_doors):
super().__init__(color, speed) # Call parent class constructor
self.num_doors = num_doors
my_car = Car(“red”, 60, 4)
print(f”My car is my_car.color, goes my_car.speed mph, and has my_car.num_doors doors.”)
“`
Multiple Inheritance
Multiple inheritance lets a child class inherit from multiple parent classes. This is useful when a class needs functionality from several unrelated classes. For example, a `FlyingCar` could inherit from both `Car` and `Airplane`.
“`python
class Airplane:
def __init__(self, altitude):
self.altitude = altitude
class FlyingCar(Car, Airplane):
def __init__(self, color, speed, num_doors, altitude):
Car.__init__(self, color, speed, num_doors)
Airplane.__init__(self, altitude)
my_flying_car = FlyingCar(“silver”, 100, 2, 10000)
print(f”My flying car is my_flying_car.color, goes my_flying_car.speed mph, has my_flying_car.num_doors doors, and flies at my_flying_car.altitude feet.”)
“`
Multilevel Inheritance
Multilevel inheritance extends the concept further. A child class inherits from a parent class, which itself inherits from another parent class. Think of it as a family tree of classes. We could extend our example by creating an `ElectricCar` that inherits from `Car`.
“`python
class ElectricCar(Car):
def __init__(self, color, speed, num_doors, battery_capacity):
super().__init__(color, speed, num_doors)
self.battery_capacity = battery_capacity
my_electric_car = ElectricCar(“blue”, 50, 4, 75)
print(f”My electric car is my_electric_car.color, goes my_electric_car.speed mph, has my_electric_car.num_doors doors, and has a my_electric_car.battery_capacity kWh battery.”)
“`
A Vehicle Hierarchy
Let’s create a more comprehensive vehicle hierarchy. We’ll start with a base `Vehicle` class and then create subclasses for `Car`, `Truck`, and `Bicycle`.
“`python
class Vehicle:
def __init__(self, color, max_speed):
self.color = color
self.max_speed = max_speed
class Car(Vehicle):
def __init__(self, color, max_speed, num_doors):
super().__init__(color, max_speed)
self.num_doors = num_doors
class Truck(Vehicle):
def __init__(self, color, max_speed, payload_capacity):
super().__init__(color, max_speed)
self.payload_capacity = payload_capacity
class Bicycle(Vehicle):
def __init__(self, color, max_speed, num_gears):
super().__init__(color, max_speed)
self.num_gears = num_gears
“`
Polymorphism: Many Forms, One Interface
Polymorphism allows objects of different classes to be treated as objects of a common type. This means you can use the same method name on different objects, and the specific behavior will depend on the object’s class. This is achieved through method overriding and operator overloading.
Method Overriding
Method overriding allows a child class to provide a specific implementation for a method that is already defined in its parent class. This enables you to customize the behavior inherited from the parent class without modifying the parent’s code.
“`python
class Animal:
def speak(self):
print(“Generic animal sound”)
class Dog(Animal):
def speak(self):
print(“Woof!”)
class Cat(Animal):
def speak(self):
print(“Meow!”)
my_dog = Dog()
my_cat = Cat()
my_dog.speak() # Output: Woof!
my_cat.speak() # Output: Meow!
“`
Operator Overloading, How to Master Object-Oriented Programming in Python
Operator overloading lets you define how operators (like +, -, *, /) work with your custom classes. For example, you could overload the `+` operator to add two `Vector` objects together.
“`python
class Vector:
def __init__(self, x, y):
self.x = x
self.y = y
def __add__(self, other):
return Vector(self.x + other.x, self.y + other.y)
v1 = Vector(1, 2)
v2 = Vector(3, 4)
v3 = v1 + v2
print(f”v3 = (v3.x, v3.y)”) # Output: v3 = (4, 6)
“`
Compile-Time vs. Runtime Polymorphism
Compile-time polymorphism (also known as static polymorphism) is resolved at compile time. A prime example is method overloading (not directly supported in Python), where multiple methods with the same name but different parameters exist within a class. Runtime polymorphism (dynamic polymorphism) is resolved at runtime. Method overriding is a classic example of runtime polymorphism. The correct method is determined based on the object’s actual type. Python primarily supports runtime polymorphism.
Abstraction and Encapsulation
Object-Oriented Programming (OOP) isn’t just about creating classes and objects; it’s about building robust, maintainable, and secure software. This is where abstraction and encapsulation come in – two powerful concepts that work hand-in-hand to achieve these goals. Think of them as the security guards of your code, protecting your data and simplifying its structure.
Abstraction simplifies complex systems by hiding unnecessary details and presenting only essential information to the user. Encapsulation, on the other hand, bundles data and methods that operate on that data within a single unit (like a class), controlling access to the internal workings. This protects data integrity and prevents unintended modifications. Together, they are crucial for creating well-structured and reliable Python programs.
Data Hiding and Security through Encapsulation
Encapsulation is all about protecting your data. Imagine a car: you interact with the steering wheel, accelerator, and brakes, but you don’t need to know the intricate details of the engine’s internal combustion process. Similarly, in OOP, encapsulation hides the internal implementation details of a class, exposing only the necessary interface to the outside world. This reduces complexity, improves code maintainability, and enhances security by preventing direct manipulation of internal data. For example, consider a `BankAccount` class. You might have methods like `deposit()` and `withdraw()`, but the internal representation of the balance (perhaps as a private attribute) is hidden. This prevents accidental or malicious changes to the account balance outside the defined methods.
Abstract Base Classes (ABCs) in Python
Abstract Base Classes (ABCs) are powerful tools for enforcing abstraction. They define a common interface for subclasses, specifying methods that *must* be implemented. This ensures consistency across different implementations and promotes code reusability. Python’s `abc` module provides the tools to create ABCs. By defining abstract methods (methods without implementation), you force subclasses to provide their own specific implementations, ensuring a consistent interface while allowing flexibility in how those methods are implemented. This leads to more organized and predictable code.
Using Abstract Methods and Properties to Enforce Abstraction
Let’s illustrate with an example. Suppose we’re building a system for different types of shapes. We can create an abstract base class `Shape` with abstract methods like `area()` and `perimeter()`. Concrete subclasses like `Circle`, `Rectangle`, and `Triangle` would then *have* to implement these methods according to their specific shapes. This ensures all shapes consistently provide area and perimeter calculations, regardless of their individual characteristics.
Example: Controlling Access to Attributes with Properties
Here’s a Python class demonstrating the use of properties to control access to attributes:
“`python
class Person:
def __init__(self, name, age):
self._name = name # Protected attribute
self._age = age # Protected attribute
@property
def name(self):
return self._name
@name.setter
def name(self, new_name):
if isinstance(new_name, str):
self._name = new_name
else:
raise ValueError(“Name must be a string”)
@property
def age(self):
return self._age
@age.setter
def age(self, new_age):
if isinstance(new_age, int) and new_age > 0:
self._age = new_age
else:
raise ValueError(“Age must be a positive integer”)
person = Person(“Alice”, 30)
print(person.name) # Accessing the name
person.name = “Bob” # Modifying the name
print(person.name)
#person.name = 123 # This will raise a ValueError
“`
This example shows how properties (`@property`) allow controlled access to attributes. The `setter` methods ensure data integrity by validating input before updating the attributes. This is a practical demonstration of encapsulation, protecting the internal state of the `Person` object from invalid modifications.
Advanced OOP Techniques in Python
Python’s object-oriented programming capabilities extend far beyond the basics. Mastering advanced techniques unlocks the potential to create more robust, maintainable, and elegant code. This section delves into powerful tools like composition, metaclasses, and decorators, showing you how to elevate your Python OOP game.
Composition versus Inheritance
Composition and inheritance are both powerful tools for code reuse, but they achieve this in fundamentally different ways. Inheritance establishes an “is-a” relationship, where a subclass inherits attributes and methods from a superclass. Composition, on the other hand, establishes a “has-a” relationship, where a class contains instances of other classes as attributes. This offers greater flexibility and avoids some of the pitfalls of inheritance, like tight coupling and the fragility of the base class problem.
Let’s illustrate with an example. Imagine we’re modeling a car. Using inheritance, we might have a `Vehicle` base class and a `Car` subclass. However, a car is more accurately described as *having* an engine, wheels, and a steering wheel, rather than *being* an engine, wheels, and a steering wheel. Composition better reflects this reality:
“`python
class Engine:
def start(self):
print(“Engine started”)
class Wheels:
def rotate(self):
print(“Wheels rotating”)
class SteeringWheel:
def turn(self):
print(“Steering wheel turning”)
class Car:
def __init__(self):
self.engine = Engine()
self.wheels = Wheels()
self.steering_wheel = SteeringWheel()
def drive(self):
self.engine.start()
self.wheels.rotate()
print(“Car driving”)
my_car = Car()
my_car.drive()
“`
This example demonstrates how composition allows for more modular and flexible designs. Changes to the `Engine` class, for example, won’t directly affect the `Car` class unless explicitly changed within the `Car` class’s methods.
Metaclasses: Customizing Class Creation
Metaclasses are classes whose instances are classes. They provide a powerful mechanism to intercept and modify the class creation process. This allows for centralized control over class attributes, methods, and behavior. A common use case is to automatically add methods or attributes to all classes within a specific module or project.
For example, we could create a metaclass that automatically adds a timestamp to every class:
“`python
import datetime
class TimestampMeta(type):
def __new__(cls, name, bases, attrs):
attrs[‘creation_timestamp’] = datetime.datetime.now()
return super().__new__(cls, name, bases, attrs)
class MyClass(metaclass=TimestampMeta):
pass
print(MyClass.creation_timestamp)
“`
This code demonstrates how a metaclass intercepts the class creation process (`__new__`) to add a timestamp attribute.
Best Practices for Designing Efficient and Well-Structured Classes
Designing efficient and well-structured classes is crucial for maintainable and scalable code. Key principles include:
- Single Responsibility Principle: Each class should have only one reason to change.
- Keep it Simple: Avoid overly complex classes. Break down large classes into smaller, more manageable ones.
- Use descriptive names: Class and method names should clearly indicate their purpose.
- Encapsulation: Protect internal data by using private attributes (using a leading underscore).
- Docstrings: Always include clear and concise docstrings to explain the class and its methods.
Following these best practices results in code that’s easier to understand, test, and maintain.
Decorators: Modifying Class Behavior
Decorators provide a concise way to modify or enhance class methods without directly altering their source code. They’re particularly useful for adding cross-cutting concerns like logging, authentication, or caching.
Consider a decorator that logs method calls:
“`python
import functools
def log_method_call(func):
@functools.wraps(func)
def wrapper(self, *args, kwargs):
print(f”Calling method: func.__name__ with args: args, kwargs: kwargs”)
result = func(self, *args, kwargs)
print(f”Method func.__name__ returned: result”)
return result
return wrapper
class MyClass:
@log_method_call
def my_method(self, a, b):
return a + b
my_instance = MyClass()
my_instance.my_method(2, 3)
“`
This example shows how a decorator cleanly adds logging functionality to the `my_method` without modifying its core logic. This approach promotes cleaner, more organized code.
Exception Handling and OOP
Exception handling is crucial for building robust and reliable object-oriented programs in Python. Without it, unexpected errors can crash your application, leaving users frustrated and data potentially corrupted. Integrating exception handling directly into your class methods ensures that your objects can gracefully handle errors, preventing program crashes and providing informative feedback.
Exception handling within class methods allows for controlled responses to potential issues, making your code more predictable and maintainable. This is achieved primarily through the use of `try`, `except`, and `finally` blocks. By anticipating potential problems and defining how your classes should react, you build more resilient and user-friendly applications.
Handling Exceptions within Class Methods
The `try-except-finally` block is your best friend when it comes to handling exceptions within class methods. The `try` block contains the code that might raise an exception. The `except` block catches specific exceptions and executes alternative code to handle the error. The `finally` block, which is optional, contains code that always executes, regardless of whether an exception occurred. This is useful for cleanup tasks, such as closing files or releasing resources.
Here’s an example:
“`python
class FileProcessor:
def process_file(self, filename):
try:
with open(filename, ‘r’) as f:
data = f.read()
# Process the data
result = self.process_data(data)
return result
except FileNotFoundError:
return “Error: File not found.”
except Exception as e:
return f”An unexpected error occurred: e”
finally:
print(“File processing complete.”)
def process_data(self, data):
#Some data processing logic
return data.upper()
processor = FileProcessor()
print(processor.process_file(“my_file.txt”)) #Assumes my_file.txt exists
print(processor.process_file(“nonexistent_file.txt”))
“`
This example demonstrates how to handle `FileNotFoundError` specifically and provides a generic error message for other exceptions. The `finally` block ensures that the “File processing complete” message is always printed.
Creating Custom Exception Classes
Sometimes, the built-in Python exceptions aren’t sufficient to capture the specific error conditions within your application. This is where custom exception classes come in handy. Creating custom exceptions allows you to provide more context-specific error messages and improve the clarity of your error handling. Custom exceptions are defined by inheriting from the base `Exception` class or one of its subclasses.
Here’s how to create a custom exception:
“`python
class InvalidInputError(Exception):
def __init__(self, message, value):
super().__init__(message)
self.value = value
class DataValidator:
def validate_data(self, value):
if value < 0:
raise InvalidInputError("Value must be non-negative.", value)
return value
validator = DataValidator()
try:
result = validator.validate_data(-5)
print(result)
except InvalidInputError as e:
print(f"Error: e")
print(f"Invalid value: e.value")
```
This example defines `InvalidInputError`, which provides both an error message and the invalid value itself. This offers more detail to the user or developer when handling the error.
Improving Robustness with Exception Handling
By strategically incorporating exception handling into your class methods, you significantly enhance the robustness of your object-oriented programs. This approach prevents unexpected crashes, improves error reporting, and facilitates easier debugging. Instead of the program abruptly halting, it can handle errors gracefully, potentially recovering from minor issues or providing helpful feedback to the user. This leads to a more reliable and user-friendly application. The key is to anticipate potential problems and design your classes to respond appropriately, making them more resilient to unexpected inputs or conditions.
Working with Modules and Packages
Python’s power lies not just in its elegant syntax but also in its robust module and package system. This allows you to organize your code efficiently, promoting reusability and maintainability. By breaking down large projects into smaller, manageable units, you can improve collaboration and reduce code duplication. Mastering modules and packages is crucial for building sophisticated and scalable applications.
Modules are simply Python files containing functions, classes, and variables. Packages, on the other hand, are a way to structure collections of modules into a hierarchical directory structure. This organization helps manage the complexity of larger projects, making them easier to navigate and understand. Let’s delve into the practical aspects of creating, using, and managing these essential building blocks of Python programming.
Conquering object-oriented programming in Python? It’s all about mastering classes and inheritance. Think of it like building a robust system; you need to protect your digital assets just as you’d protect your real-world ones, which is why understanding How to Choose the Right Insurance Coverage for Your High-Net-Worth Assets is surprisingly relevant. Back to Python: effective encapsulation is key to building clean, maintainable code – just like a well-structured insurance portfolio.
Creating a Python Module
A Python module is nothing more than a `.py` file. Let’s say we’re building a simple geometry library. We can create a module named `geometry.py` containing classes for different geometric shapes. This module could include classes like `Circle`, `Rectangle`, and `Triangle`, each with methods for calculating area, perimeter, and other relevant properties. For instance, the `Circle` class might have a constructor that takes the radius as input and methods to calculate the area (πr²) and circumference (2πr). The `Rectangle` class would similarly have methods for calculating area (length * width) and perimeter (2 * (length + width)).
Organizing Modules into a Package
To organize multiple modules, we create a package. A package is essentially a directory containing one or more modules, plus a special file named `__init__.py`. This `__init__.py` file can be empty, or it can contain initialization code that runs when the package is imported. Let’s assume we want to create a package named `shapes`. Inside the `shapes` directory, we would place our `geometry.py` module (and any other related modules) along with the `__init__.py` file. This structure clearly groups related modules, enhancing code organization. For example, we could have another module in the `shapes` package, perhaps `threeDShapes.py`, containing classes for cubes, spheres, etc.
Importing and Using Classes from Modules and Packages
Once you’ve created your modules and packages, you can import and use the classes they contain. Importing a class from a module is straightforward:
from geometry import Circle
my_circle = Circle(5)
area = my_circle.calculate_area()
This code snippet imports the `Circle` class from the `geometry` module and creates an instance of it. Similarly, importing from a package involves specifying the package and module:
from shapes.geometry import Rectangle
my_rectangle = Rectangle(4, 6)
perimeter = my_rectangle.calculate_perimeter()
This imports the `Rectangle` class from the `geometry` module within the `shapes` package. The `__init__.py` file in the package plays a role in how the package is imported; it can contain code that runs during import, potentially setting up variables or functions for use across the package’s modules.
Best Practices for Structuring Code
Effective module and package structuring is crucial for large projects. Here are some key best practices:
Properly structuring your code into modules and packages is essential for maintainability and scalability. By following these best practices, you can create well-organized, reusable, and easily understandable Python projects.
Illustrative Examples
Object-oriented programming (OOP) isn’t just an abstract concept; it’s the backbone of countless applications we use daily. Understanding how OOP principles are applied in real-world scenarios solidifies your grasp of the subject and reveals its immense practical value. Let’s dive into a concrete example to illustrate this.
We’ll explore a simplified version of a popular e-commerce platform, focusing on how OOP structures the core components. This example will highlight how classes and objects interact, showcasing the power of inheritance, polymorphism, and encapsulation.
E-commerce Platform Structure
Imagine an online store selling various products. Using OOP, we can model this system effectively. The key is to identify the core entities and their relationships.
The following Artikels the classes and objects involved, demonstrating how OOP principles facilitate efficient code organization and maintainability:
- Product Class: This is a base class representing a generic product.
- Attributes:
product_id
(integer),name
(string),description
(string),price
(float),stock_quantity
(integer) - Methods:
get_price()
,update_stock()
,display_details()
- Attributes:
- Clothing Class: This inherits from the
Product
class, adding clothing-specific attributes.- Attributes:
size
(string),color
(string),material
(string) - Methods:
get_size()
- Attributes:
- Electronics Class: Another subclass of
Product
, adding attributes specific to electronics.- Attributes:
manufacturer
(string),warranty_period
(integer),model_number
(string) - Methods:
get_warranty()
- Attributes:
- ShoppingCart Class: This class manages the customer’s shopping cart.
- Attributes:
items
(list ofProduct
objects),total_price
(float) - Methods:
add_item()
,remove_item()
,calculate_total()
,checkout()
- Attributes:
- Customer Class: Represents a customer with their details.
- Attributes:
customer_id
(integer),name
(string),email
(string),address
(string),shopping_cart
(ShoppingCart
object) - Methods:
view_cart()
,place_order()
- Attributes:
In this structure, inheritance allows us to avoid code duplication by extending the Product
class. Polymorphism is demonstrated through the various Product
subclasses, each having its own unique attributes and methods. Encapsulation protects internal data by using methods to access and modify attributes, ensuring data integrity. For example, the update_stock()
method in the Product
class ensures that stock quantities are updated correctly and consistently.
Concluding Remarks
Mastering object-oriented programming in Python isn’t just about writing code; it’s about building a framework for cleaner, more efficient, and scalable software. By understanding the core principles of encapsulation, inheritance, polymorphism, and abstraction, you’ll unlock a new level of coding prowess. This guide has equipped you with the tools to not only understand OOP but to apply it effectively in your projects. So, go forth and build amazing things!