How to Implement Unit Testing in Your Code? Sounds boring, right? Wrong! Unit testing isn’t just for coding nerds; it’s your secret weapon against buggy code and sleepless nights. This guide dives deep into the world of unit tests, showing you how to write them, why they matter, and how to make them your new best friend. Get ready to level up your coding game.
We’ll cover everything from setting up your testing environment and choosing the right framework for your language (Python’s pytest? Java’s JUnit? We’ve got you covered!) to mastering advanced techniques like mocking and boosting your test coverage. We’ll even tackle the dreaded question of how to test complex logic and handle those pesky external dependencies. By the end, you’ll be writing cleaner, more reliable code – and sleeping soundly knowing your software is rock-solid.
Introduction to Unit Testing
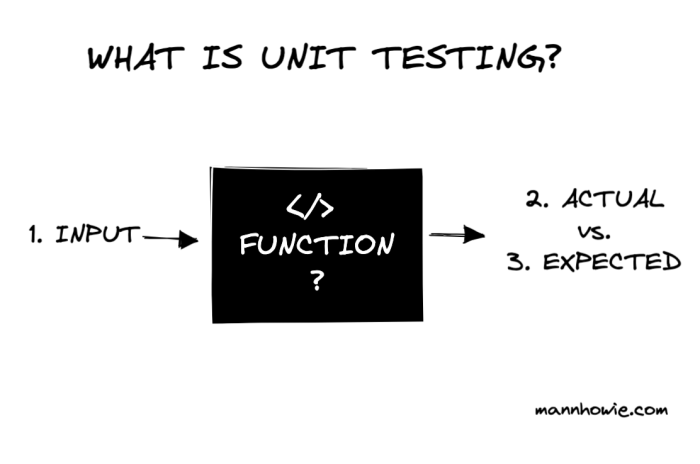
Source: ctfassets.net
Solid unit testing ensures your code behaves as expected, minimizing future headaches. Think of it like securing your financial future – planning for the unexpected is key, much like learning How to Maximize Your Life Insurance Policy’s Benefits to protect your loved ones. Just as a well-structured policy offers peace of mind, thorough unit tests give you confidence in your code’s reliability.
Unit testing is like giving your code a mini-checkup before it goes live. It’s a crucial part of software development that helps you catch bugs early and ensure your code works as expected. Think of it as preventative maintenance for your software – much easier (and cheaper!) to fix a small problem in a single unit than to debug a massive, tangled mess later on.
Unit testing involves writing small, isolated tests for individual units of code (typically functions or methods). These tests verify that each unit behaves correctly in isolation, ensuring that the building blocks of your application are sound. This approach significantly reduces the risk of unexpected behavior and makes debugging significantly easier.
Benefits of Unit Testing
Unit testing offers a plethora of benefits throughout the software development lifecycle. It improves code quality by identifying and resolving defects early, reducing the overall cost of development and maintenance. Furthermore, it facilitates better code design through the encouragement of modularity and separation of concerns. By writing tests first (test-driven development), developers are encouraged to design cleaner, more maintainable code. Finally, unit tests serve as living documentation, illustrating how different parts of the code are intended to function. This is incredibly helpful for onboarding new developers or revisiting code after a period of inactivity.
Types of Unit Tests
While the core concept remains the same, there are nuances in how unit tests are approached. Functional unit tests verify the functionality of a single unit in isolation, ensuring that given a specific input, it produces the expected output. Integration tests, on the other hand, test the interaction between multiple units or modules to ensure they work together seamlessly. The line between unit and integration tests can sometimes be blurry, and the choice often depends on the complexity of the system and the specific testing goals.
Common Unit Testing Frameworks
Several excellent frameworks simplify the process of writing and running unit tests. These frameworks provide tools for writing assertions (verifying expected outcomes), organizing tests, and generating reports. The choice of framework depends largely on the programming language used in your project.
Language | Framework | Description | Example Code Snippet |
---|---|---|---|
Java | JUnit | A widely used framework for writing unit tests in Java. Provides annotations for defining test methods and assertions for verifying results. | @Test |
Python | pytest | A popular and versatile testing framework for Python, known for its simplicity and extensibility. | def test_add(): |
C# (.NET) | NUnit | A widely adopted unit testing framework for .NET applications, offering a rich set of features and integrations. | [Test] |
JavaScript | Jest | A JavaScript testing framework built by Facebook, particularly well-suited for React applications. It’s known for its ease of use and comprehensive features. | test('adds 1 + 2 to equal 3', () => |
Setting up a Unit Testing Environment: How To Implement Unit Testing In Your Code
Setting up a solid unit testing environment is crucial for writing robust and maintainable code. It’s like building a strong foundation for your house – you wouldn’t skip the groundwork, would you? The right environment makes writing, running, and interpreting your tests a breeze. This section will guide you through setting up this crucial foundation, regardless of your chosen programming language.
Choosing the right tools and structuring your tests effectively are key components of a successful unit testing strategy. Let’s dive into the practical aspects.
Test Runner Selection and Configuration
Selecting the appropriate test runner is the first step. Popular choices include Jest (JavaScript), pytest (Python), JUnit (Java), and NUnit (.NET). Each runner offers a unique set of features and integrates seamlessly with various IDEs and build systems. For instance, Jest boasts its own mocking capabilities and a simple API, making it a favorite among JavaScript developers. Pytest, on the other hand, is known for its flexibility and extensive plugin ecosystem, catering to diverse Python project needs. The configuration process usually involves installing the runner via a package manager (like npm, pip, or Maven) and potentially configuring it within your project’s build system (like Gradle or Make). Proper configuration ensures tests run smoothly within your development workflow.
Organizing Unit Test Code
Effective organization is paramount for maintainable test suites. Think of it as keeping your toolbox tidy – you’ll find the right wrench much faster! A common practice is to place test files alongside the code they test, using a naming convention like `filename_test.py` (Python) or `filename.spec.js` (JavaScript). This keeps related code close, improving readability and maintainability. Furthermore, grouping tests by functionality or module helps in isolating and debugging failures. Consider using descriptive names for your test functions, clearly indicating the tested behavior. For example, instead of `test_1()`, use `test_calculate_total_price_with_discount()`.
Dependency Injection in Unit Testing
Dependency injection is a game-changer for writing effective unit tests. It allows you to isolate units of code and test them independently, without relying on external services or databases. Imagine testing a function that sends an email; without dependency injection, you’d need a real email server for every test, which is slow and unreliable. Instead, you inject a mock email service that simulates sending emails without actually connecting to an external server. This significantly speeds up testing and prevents test failures due to external dependencies.
Illustrative Diagram of Dependency Injection, How to Implement Unit Testing in Your Code
Consider a simple function that calculates the total price of an order, including tax. This function relies on a `TaxCalculator` class.
“`
+—————–+ +—————–+ +—————–+
| OrderProcessor |—->| TaxCalculator |—->| Database |
+—————–+ +—————–+ +—————–+
^ |
| |
+——————————————-+
“`
In a unit test, we would inject a mock `TaxCalculator` instead of the real one:
“`
+—————–+ +—————–+
| OrderProcessor |—->| MockTaxCalculator|
+—————–+ +—————–+
“`
The `MockTaxCalculator` would return predefined tax values, allowing us to test the `OrderProcessor` in isolation, regardless of the `Database` or the actual tax calculation logic. This makes our tests faster, more reliable, and easier to maintain.
Writing Effective Unit Tests
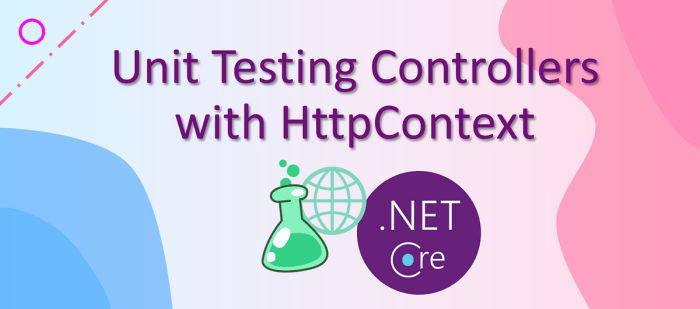
Source: medium.com
Writing clean, concise, and maintainable unit tests is crucial for building robust and reliable software. Effective unit tests act as a safety net, catching bugs early in the development process and preventing regressions as your code evolves. They also improve code design by encouraging you to write smaller, more modular functions that are easier to test. Think of them as tiny, focused experiments that verify each part of your application works as expected.
The key to writing effective unit tests lies in adhering to the FIRST principles: Fast, Independent, Repeatable, Self-Validating, and Thorough. Fast tests run quickly, allowing for rapid feedback during development. Independent tests don’t rely on each other, ensuring that a failure in one test doesn’t cascade into others. Repeatable tests produce the same results every time they are run. Self-validating tests automatically determine whether they passed or failed without manual intervention. And thorough tests cover all aspects of the code’s functionality.
Good and Bad Unit Test Examples
Let’s illustrate the difference between good and bad unit tests with a simple example. Suppose we have a function that calculates the sum of two numbers:
Bad Test:
function testSum()
if (sum(2, 3) == 5)
echo "Test passed!";
else
echo "Test failed!";
This test lacks structure and doesn’t provide much information in case of failure. It also mixes testing logic with output, making it harder to maintain and integrate into a testing framework.
Good Test (using PHPUnit style):
assertEquals(5, sum(2, 3));
public function testSumNegativeNumbers()
$this->assertEquals(-1, sum(-2, 1));
public function testSumZero()
$this->assertEquals(0, sum(0, 0));
?>
This example demonstrates better structure, using a testing framework (PHPUnit in this case) for clear assertion and reporting. It also includes multiple test cases covering different scenarios, making the test suite more comprehensive.
Test-Driven Development (TDD)
Test-driven development is a powerful approach where you write the tests *before* you write the code. This forces you to think carefully about the design and functionality of your code from the perspective of the user or the system that will interact with it. The TDD cycle typically involves these steps:
- Write a failing test: First, write a test that defines a specific piece of functionality you want to implement. This test will initially fail because the code doesn’t exist yet.
- Write the minimal code to pass the test: Next, write the simplest possible code that makes the test pass. Avoid over-engineering or adding extra features at this stage.
- Refactor the code: Once the test passes, refactor the code to improve its design, readability, and maintainability. Ensure that the refactoring doesn’t break the existing tests.
Let’s illustrate this with a simple example. Let’s say we need a function to check if a number is even:
Step 1: Failing Test
assertTrue(isEven(2)); // This will fail initially
?>
Step 2: Minimal Code to Pass the Test
Step 3: Refactoring (in this case, no significant refactoring needed).
By following the TDD cycle, you ensure that your code is thoroughly tested and that new features don’t break existing functionality. It promotes cleaner, more maintainable code and leads to fewer bugs.
Testing Different Code Structures
Unit testing isn’t a one-size-fits-all affair. The approach you take significantly depends on the structure of the code you’re testing. Whether you’re dealing with simple functions or complex class hierarchies, understanding how to tailor your tests is crucial for effective and comprehensive coverage. This section will explore best practices for testing various code structures and offer strategies for tackling complex logic.
Testing different code structures requires a nuanced approach. The complexity of your code dictates the testing strategy. Simple functions might require straightforward input-output checks, while complex classes need a more modular and systematic testing approach.
Testing Functions
Functions, the building blocks of many programs, are relatively straightforward to test. The primary focus is on verifying the function’s output for various valid and invalid inputs. This often involves creating multiple test cases, each covering different scenarios, including edge cases and boundary conditions. For example, a function calculating the area of a circle should be tested with positive radii, zero radius, and negative radii (expecting an error or specific handling). The use of parameterized tests, where the same test function is run with multiple sets of inputs, greatly simplifies this process. Mocking dependencies (if any) is also essential for isolating the function’s behavior.
Testing Classes and Methods
Testing classes and their methods requires a more organized approach. You need to consider the interactions between different methods within the class and how they affect the class’s overall state. This often involves testing individual methods in isolation (similar to function testing) and then testing the interactions between methods through integration tests within the class. Consider using test-driven development (TDD) to design and implement your tests alongside the class, ensuring that each method is designed with testability in mind. For example, a class managing a user account might have methods for creating, updating, and deleting accounts. Each method should be tested independently, and then integration tests should verify the correct interaction between these methods (e.g., attempting to delete a non-existent account).
Testing Complex Logic
Complex logic, often involving multiple conditional statements, loops, and interactions with external systems, poses unique challenges. Breaking down the complex logic into smaller, more manageable units is essential. This might involve creating helper functions or refactoring the code to improve testability. Consider using techniques like state-based testing, where you test the system’s behavior as it transitions between different states. Another useful approach is property-based testing, where you define properties that the system should always satisfy, and the testing framework generates random inputs to check these properties. For example, a sorting algorithm’s correctness can be tested by checking if the output is always sorted and contains all the elements of the input. This approach helps uncover edge cases that might be missed with traditional test case design.
Common Testing Scenarios and Approaches
Understanding common scenarios and associated testing approaches is vital for efficient and comprehensive unit testing.
Below is a list of common testing scenarios and their associated unit test approaches:
- Scenario: Input validation. Approach: Provide invalid inputs (null, empty, wrong data type) and verify that the function/method handles them correctly (e.g., throws an exception, returns an error code).
- Scenario: Boundary conditions. Approach: Test the function/method with inputs at the limits of its valid range (e.g., minimum and maximum values, empty collections).
- Scenario: Error handling. Approach: Simulate error conditions (e.g., file not found, network error) and verify that the function/method handles them gracefully.
- Scenario: Interactions between classes. Approach: Use mocking to isolate the interaction between classes and test the behavior of one class when interacting with mocked dependencies.
- Scenario: Complex algorithms. Approach: Break down the algorithm into smaller, testable units and test each unit independently. Consider property-based testing.
Handling External Dependencies
Unit testing thrives on isolation. But what happens when your code needs to talk to a database, an external API, or any other external system? Suddenly, your nice, predictable unit tests become flaky and unreliable, dependent on the whims of external services. This is where strategies for managing external dependencies become crucial for robust and maintainable unit tests.
Testing code that interacts with external systems requires a shift in approach. You can’t simply let your tests directly access these systems; doing so introduces unpredictable delays, reliance on network connectivity, and potential data corruption issues. Instead, you need to simulate or “mock” the behavior of these external systems.
Mocking and Stubbing
Mocking and stubbing are techniques used to replace real external dependencies with controlled substitutes during testing. A mock simulates the behavior of an object, allowing you to verify interactions with it (did it get called with the right arguments?), while a stub provides canned responses to method calls, focusing on controlling the output of the dependency. Think of a mock as a spy, watching interactions, and a stub as an actor, providing predetermined lines.
Consider a scenario where you have a user service that fetches user data from a database. Directly testing this service would require a running database, making the test slow, fragile, and dependent on database availability. Instead, we can mock the database interaction.
Example: Mocking a Database Interaction
Let’s say we have a Python class `UserService` that retrieves user data from a database:
“`python
import sqlite3
class UserService:
def __init__(self, db_path):
self.conn = sqlite3.connect(db_path)
def get_user(self, user_id):
cursor = self.conn.cursor()
cursor.execute(“SELECT * FROM users WHERE id = ?”, (user_id,))
user = cursor.fetchone()
self.conn.close()
return user
“`
Testing this directly is problematic. Instead, we can use the `unittest.mock` library to mock the `sqlite3.connect` function:
“`python
import unittest
from unittest.mock import patch
from your_module import UserService # Assuming UserService is in your_module.py
class TestUserService(unittest.TestCase):
@patch(‘sqlite3.connect’)
def test_get_user(self, mock_connect):
# Mock the database connection
mock_cursor = mock_connect.return_value.cursor.return_value
mock_cursor.fetchone.return_value = (‘John Doe’, 123) # Stubbed return value
# Create UserService instance using the mock connection
user_service = UserService(‘path/to/db’)
# Call the method being tested
user = user_service.get_user(1)
# Assertions
self.assertEqual(user, (‘John Doe’, 123))
mock_connect.assert_called_once() # Verify the connection was called once
mock_cursor.execute.assert_called_once_with(“SELECT * FROM users WHERE id = ?”, (1,)) #Verify the correct query was executed.
if __name__ == ‘__main__’:
unittest.main()
“`
In this example, `@patch(‘sqlite3.connect’)` replaces the real `sqlite3.connect` function with a mock object. We then configure the mock to return a cursor with a predefined `fetchone` result, simulating a successful database query. This isolates the `get_user` method from the actual database, making the test fast, reliable, and independent of external factors. The `assert_called_once()` methods verify that the database connection and query were called as expected. This ensures that not only does the function return the correct value but also that it interacts with the database correctly.
Advanced Unit Testing Techniques
So you’ve mastered the basics of unit testing—congrats! But the world of testing is vast, and there’s a whole universe of advanced techniques to make your tests even better. This section dives into strategies for maximizing test coverage, reliability, and efficiency, especially when dealing with larger codebases.
Moving beyond simple test cases requires a strategic approach. Think of it like upgrading from a basic bicycle to a high-performance racing bike – you’ll need to understand the nuances of each component to truly optimize performance. This section covers key techniques that will elevate your testing game.
Improving Test Coverage and Reliability
Comprehensive test coverage is crucial for ensuring software quality. It’s not just about the number of tests, but also the quality and effectiveness of those tests. Aiming for 100% line coverage isn’t always realistic or necessary, but striving for high coverage in critical sections of your code is paramount. This involves strategically designing tests that cover various scenarios, including edge cases, boundary conditions, and error handling. Using techniques like mutation testing can help identify weaknesses in your test suite by subtly altering your code and seeing if your tests still catch the errors.
Code Coverage Tools
Code coverage tools provide invaluable insights into which parts of your code are actually exercised by your tests. These tools instrument your code, tracking which lines, branches, and paths are executed during test runs. Popular tools include SonarQube, JaCoCo (for Java), and Istanbul (for JavaScript). The reports generated by these tools visually represent your code coverage, highlighting areas that need more attention. For example, JaCoCo might show a visual representation of your code with different colors indicating the percentage of lines covered by your tests. Uncovered lines are usually marked in red, indicating that those sections of the code haven’t been tested. This allows developers to quickly identify gaps in their testing strategy and write additional tests to improve coverage.
Managing and Running Large Test Suites
As your project grows, so does your test suite. Managing and running hundreds or thousands of tests efficiently becomes a significant challenge. Effective strategies include: parallelization (running tests concurrently), test categorization (grouping tests by functionality or module), and utilizing test runners with robust reporting features. Test runners like Jest (JavaScript) or pytest (Python) offer features like parallel execution and detailed reporting, allowing for faster feedback cycles and easier identification of failing tests. Imagine having 1000 unit tests. Running them sequentially could take a considerable amount of time. However, with parallelization, you can divide these tests across multiple processors, significantly reducing the overall execution time.
Integrating Unit Tests into the Development Workflow
Seamlessly weaving unit testing into your development process isn’t just about writing tests; it’s about making them an integral part of your daily routine. This ensures consistent code quality and reduces the likelihood of bugs creeping in. Think of it as building quality control directly into your coding workflow, rather than as a separate, afterthought task.
Integrating unit tests effectively requires a shift in mindset – from viewing testing as an extra step to viewing it as a crucial component of development itself. This proactive approach leads to more robust, reliable software in the long run. The benefits are numerous, from early bug detection to faster debugging times.
Continuous Integration and Continuous Delivery with Unit Tests
Implementing CI/CD pipelines with unit tests is key to automating the testing process and ensuring code quality across all deployments. A well-structured CI/CD pipeline automatically runs unit tests whenever new code is pushed, providing immediate feedback on the impact of changes. This allows for quick identification and resolution of issues before they reach production. Imagine a scenario where a developer commits code that introduces a bug. With CI/CD and unit tests, the pipeline will immediately flag the failure, alerting the team to the problem. This prevents the faulty code from reaching the production environment, saving time and resources.
Using Unit Test Results to Improve Code Quality
Unit test results provide invaluable insights into the quality and stability of your codebase. High test coverage, coupled with consistently passing tests, indicates robust and well-tested code. Conversely, a high failure rate highlights areas needing attention. Detailed test reports can pinpoint the specific lines of code causing issues, making debugging significantly easier and faster. For example, if a significant portion of tests related to a specific module consistently fail, it indicates that the module requires refactoring or more thorough testing. Analyzing test results also allows developers to identify patterns in bugs, leading to proactive improvements in coding practices and reduced future errors. This data-driven approach to code quality is crucial for maintaining a healthy and reliable software project.
Integrating Unit Tests into the SDLC
Unit testing should be integrated into each phase of the SDLC, starting from the design phase. During the design phase, consider the testability of your code. During the development phase, write unit tests alongside the code, following a test-driven development (TDD) approach whenever possible. In the testing phase, the unit tests form the foundation of the testing strategy. Finally, in the deployment and maintenance phases, unit tests ensure that changes don’t introduce regressions. This consistent integration ensures that unit testing remains a continuous and effective process throughout the entire lifecycle of the software project. By adopting this strategy, teams can significantly reduce the risk of introducing bugs and improve the overall quality of their software.
Last Point
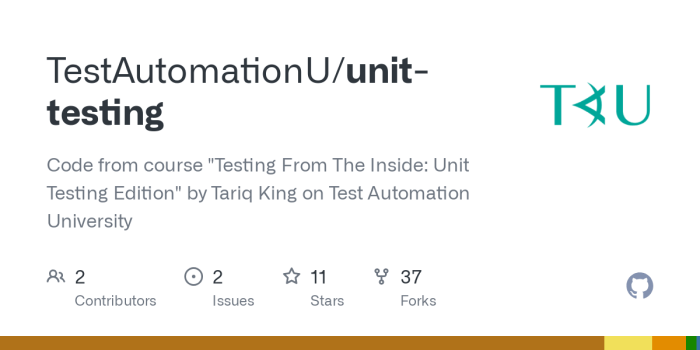
Source: githubassets.com
So, there you have it – your crash course in unit testing. Remember, writing effective unit tests isn’t just about catching bugs; it’s about building a foundation of robust, maintainable code. Embrace the power of TDD (Test-Driven Development), and watch your coding skills soar to new heights. Happy testing!