How to Optimize Your Code for Better Performance? Let’s be real, nobody wants laggy code. It’s like that one friend who’s always late – annoying and frustrating. This guide dives deep into the nitty-gritty of code optimization, from understanding bottlenecks to mastering advanced techniques. Get ready to ditch the slowdowns and unleash the power of efficient coding!
We’ll explore everything from choosing the right algorithms and data structures to conquering memory management and leveraging parallel programming. Think of it as a performance upgrade for your code – smoother, faster, and ready to handle anything you throw at it. We’ll even cover code style, because, let’s face it, clean code is happy code (and often faster too!).
Understanding Code Performance Bottlenecks
Writing efficient code isn’t just about making your program work; it’s about making it work *well*. Slow code can lead to frustrated users, wasted resources, and even system instability. Understanding the sources of these performance bottlenecks is crucial for building robust and responsive applications. This section dives into the common culprits that can drag down your code’s speed.
Inefficient Algorithms
The algorithm you choose fundamentally dictates how your code operates. An inefficient algorithm, even with perfectly optimized data structures, will always be a performance drag. For example, a naive search algorithm might have to check every element in a large dataset, leading to O(n) time complexity. In contrast, a binary search on a sorted dataset achieves O(log n) complexity, a significant improvement for large datasets. The choice between these algorithms dramatically affects performance, especially as the input size grows. Consider the difference: searching a million items with a linear search takes significantly longer than using a binary search. This difference becomes exponentially more pronounced with even larger datasets.
Data Structures and Their Impact
Data structures are the fundamental building blocks for organizing and storing data within your program. Choosing the right data structure is paramount for performance. For instance, using a linked list to search for a specific element is far less efficient than using a hash table or a binary search tree for the same task. The choice depends heavily on the specific operations you’ll be performing. If you need frequent lookups, a hash table might be optimal, offering near-constant time complexity (O(1)). However, if you need to maintain order and perform frequent insertions and deletions, a balanced binary search tree might be a better choice.
Comparison of Data Structures
Let’s compare a few common data structures and their suitability for different tasks:
Data Structure | Best Use Case | Time Complexity (Search) | Time Complexity (Insertion) |
---|---|---|---|
Array | Storing and accessing elements by index | O(1) | O(n) (in the worst case, depending on implementation) |
Linked List | Frequent insertions and deletions | O(n) | O(1) |
Hash Table | Fast lookups, insertions, and deletions | O(1) (average case) | O(1) (average case) |
Binary Search Tree | Ordered data, efficient search, insertion, and deletion | O(log n) (average case) | O(log n) (average case) |
Common Performance Bottlenecks
Identifying bottlenecks requires careful analysis and profiling. Here’s a table summarizing some common bottlenecks, their causes, and potential solutions:
Bottleneck | Cause | Solution | Example |
---|---|---|---|
Slow Database Queries | Inefficient queries, lack of indexing, large datasets | Optimize queries, add indexes, database normalization | Using a `SELECT *` query instead of selecting only necessary columns. |
I/O Operations | Frequent file access, network requests | Caching, asynchronous operations, optimize file access patterns | Repeatedly reading large files without caching. |
Inefficient Algorithms | Using algorithms with poor time complexity | Replace with more efficient algorithms | Using a bubble sort on a large dataset instead of quicksort or mergesort. |
Memory Leaks | Unreleased memory allocations | Proper memory management, garbage collection | Failing to release dynamically allocated memory. |
Algorithm Optimization Techniques: How To Optimize Your Code For Better Performance
Choosing the right algorithm is fundamental to writing efficient code. A poorly chosen algorithm can lead to significant performance bottlenecks, even with perfectly optimized code elsewhere. Understanding the strengths and weaknesses of different algorithmic approaches is crucial for creating high-performing applications. This section explores several key techniques for optimizing your algorithms.
Choosing the Right Algorithm
Selecting the appropriate algorithm depends heavily on the specific problem and the characteristics of the input data. For instance, sorting a small list might be efficiently done with a simple algorithm like bubble sort, while sorting a massive dataset necessitates a more sophisticated approach like merge sort or quicksort, which boast better time complexities (O(n log n) versus O(n^2)). The trade-off often lies between simplicity and efficiency; sometimes, a slightly less efficient algorithm might be preferred for its readability and maintainability, especially if the performance difference is negligible for the expected input size. Consider the size of your input data, the frequency of operations, and the overall complexity of the algorithm when making your selection.
Dynamic Programming
Dynamic programming is a powerful technique that solves complex problems by breaking them down into smaller, overlapping subproblems. It avoids redundant computations by storing and reusing the solutions to these subproblems. This is particularly useful for problems exhibiting optimal substructure, where the optimal solution to the overall problem can be constructed from optimal solutions to its subproblems. A classic example is the Fibonacci sequence calculation. A naive recursive approach leads to exponential time complexity, whereas a dynamic programming solution achieves linear time complexity by storing previously computed Fibonacci numbers.
Divide-and-Conquer vs. Greedy Algorithms
Divide-and-conquer algorithms work by recursively breaking down a problem into smaller subproblems, solving them independently, and then combining their solutions to obtain the overall solution. Mergesort and quicksort are prime examples. Greedy algorithms, on the other hand, make locally optimal choices at each step, hoping to find a global optimum. While often simpler to implement, greedy algorithms don’t always guarantee the best solution. For example, Dijkstra’s algorithm for finding the shortest path in a graph is a greedy algorithm, but it only works correctly for graphs with non-negative edge weights. The choice between divide-and-conquer and greedy algorithms depends on the problem’s structure and the need for an optimal versus an approximate solution.
Memoization for Recursive Algorithms
Memoization is a specific optimization technique used to improve the performance of recursive algorithms. It works by storing the results of expensive function calls and returning the cached result when the same inputs occur again. This avoids redundant computations and significantly reduces the overall execution time, especially for recursive functions with overlapping subproblems. Imagine calculating the nth Fibonacci number recursively without memoization; many subproblems are recalculated repeatedly. Memoization elegantly addresses this by storing and reusing previously calculated Fibonacci numbers.
Code Optimization Examples, How to Optimize Your Code for Better Performance
The following examples illustrate how different algorithmic approaches can impact code performance:
- Calculating Fibonacci Numbers: A naive recursive approach has exponential time complexity. A dynamic programming solution using an array to store previously calculated values reduces this to linear time complexity. A further optimization would be to only store the last two Fibonacci numbers, reducing space complexity to O(1).
- Searching for an element in a sorted array: A linear search has O(n) time complexity. Binary search, a divide-and-conquer approach, reduces this to O(log n), significantly improving performance for large arrays.
- Finding the shortest path in a graph: Dijkstra’s algorithm (a greedy approach) efficiently finds the shortest path in a graph with non-negative edge weights. For graphs with negative edge weights, the Bellman-Ford algorithm (a dynamic programming approach) is needed to handle potential negative cycles.
Data Structure Selection and Optimization
Choosing the right data structure is crucial for writing efficient code. The performance of your application can dramatically improve—or suffer—based on this single decision. Understanding the strengths and weaknesses of different data structures allows you to tailor your code for optimal speed and resource usage. This section dives into the key players and how to select the best fit for your needs.
Data structures are fundamental building blocks in programming. They determine how data is organized and accessed, directly impacting the efficiency of algorithms that operate on that data. Poor data structure choices can lead to sluggish performance, especially when dealing with large datasets or complex operations. Conversely, a well-chosen data structure can significantly accelerate your code.
Array Performance Characteristics
Arrays provide contiguous memory allocation, leading to fast element access using their index. The time complexity for accessing an element is O(1), making them ideal for scenarios requiring frequent lookups. However, inserting or deleting elements in the middle of an array can be slow (O(n)) because it necessitates shifting subsequent elements. Arrays excel in situations where you need quick access to elements by their position and modifications are infrequent or happen at the end. Consider using arrays when you’re working with a fixed-size collection of elements where random access is paramount. For instance, storing a list of student scores where you frequently need to access individual scores by student ID would be well-suited for an array.
Linked List Performance Characteristics
Unlike arrays, linked lists store elements in nodes, each pointing to the next. This allows for efficient insertion and deletion (O(1) if you have a pointer to the node before the insertion/deletion point, otherwise O(n) to find the node) anywhere in the list, but accessing a specific element requires traversing the list from the head (O(n)). Linked lists are advantageous when frequent insertions and deletions are expected, especially in the middle of the sequence. A classic example is managing a queue where elements are added and removed from opposite ends. Consider linked lists when you need a dynamic data structure that can grow or shrink efficiently, and where random access isn’t a critical requirement.
Hash Table Performance Characteristics
Hash tables utilize a hash function to map keys to indices in an array, enabling very fast average-case lookups, insertions, and deletions (O(1)). However, collisions (multiple keys mapping to the same index) can degrade performance to O(n) in the worst case. Hash tables are perfect for scenarios needing quick key-value lookups, like dictionaries or symbol tables. Imagine a system storing user profiles; a hash table would allow for incredibly fast retrieval of user data using the username as the key.
Trees and Graphs for Complex Data Manipulation
Trees and graphs are hierarchical structures ideal for representing relationships between data. Trees are particularly useful for organizing data in a hierarchical manner, such as file systems or organizational charts. Their efficiency depends on the type of tree (e.g., binary search trees offer O(log n) average-case search, insertion, and deletion). Graphs, on the other hand, are powerful for modeling networks and connections, such as social networks or transportation routes. Graph algorithms like Dijkstra’s algorithm can efficiently find the shortest paths within a graph. The performance of tree and graph operations varies greatly depending on the specific algorithm and data structure used.
Optimizing Database Queries and Data Retrieval
Database optimization is a multifaceted area. Efficient query design is paramount. Using appropriate indexes, optimizing joins, and minimizing data retrieval are key strategies. Consider using techniques like query caching and connection pooling to further enhance performance. Analyzing query execution plans can reveal bottlenecks and guide optimization efforts. For instance, adding an index to frequently queried columns can significantly speed up data retrieval.
Data Structure Comparison
Data Structure | Average Time Complexity (Search/Insert/Delete) | Suitable Use Cases |
---|---|---|
Array | O(1)/O(n)/O(n) | Storing and accessing elements by index, fixed-size collections |
Linked List | O(n)/O(1)/O(1) (with pointer to node) | Dynamic collections with frequent insertions/deletions, queues |
Hash Table | O(1)/O(1)/O(1) (average case) | Key-value stores, dictionaries, symbol tables |
Binary Search Tree | O(log n)/O(log n)/O(log n) (average case) | Ordered data, efficient search, insertion, and deletion |
Graph | Varies depending on algorithm | Modeling networks, relationships, shortest path finding |
Memory Management and Optimization
Optimizing your code for speed isn’t just about clever algorithms; it’s also about how efficiently your program handles memory. Memory leaks and inefficient memory usage can significantly slow down your application, leading to crashes, sluggish performance, and frustrated users. This section dives into the crucial aspects of memory management and how you can fine-tune it for peak performance.
Memory Leaks and Their Performance Impact
Memory leaks occur when your program allocates memory but fails to release it when it’s no longer needed. Over time, this accumulation of unused memory fragments can lead to a variety of problems. The most obvious is a gradual increase in memory consumption, eventually exhausting available system resources. This can manifest as slowdowns, application crashes, or even system instability. The impact is particularly noticeable in long-running applications or those handling large datasets, where the gradual accumulation of leaked memory can quickly become overwhelming. For example, a web server constantly creating new connections without properly closing them could experience a significant performance degradation as the number of leaked connections grows.
Memory Management Techniques in Different Programming Languages
Efficient memory management varies across programming languages. In languages like C and C++, developers have direct control over memory allocation and deallocation using functions like `malloc` and `free`. Careful pairing of allocation and deallocation is crucial; forgetting to `free` allocated memory results in a leak. In contrast, languages like Java and Python employ garbage collection, automating the process of reclaiming unused memory. However, even with garbage collection, inefficient coding practices can still lead to performance issues, such as excessive object creation or holding onto large, unnecessary objects for extended periods. For instance, in Python, creating numerous large lists without releasing them can quickly consume significant memory. Languages like C# provide features like the `using` statement, which ensures that resources, including memory, are released even if exceptions occur.
Garbage Collection and its Role in Memory Optimization
Garbage collection (GC) is a crucial feature in many modern programming languages. GC automatically identifies and reclaims memory occupied by objects that are no longer referenced by the program. While this simplifies memory management, it’s not a magic bullet. The GC process itself consumes CPU cycles, and inefficient algorithms can lead to frequent and lengthy garbage collection pauses, impacting application responsiveness. Different GC algorithms exist, each with its own trade-offs between performance and memory usage. Understanding your language’s GC and its behavior is vital for optimizing performance. For example, choosing appropriate data structures can significantly impact the GC’s workload. Using immutable data structures can reduce the need for frequent garbage collection, while poorly designed object hierarchies can lead to longer garbage collection cycles.
Common Memory Management Issues and Their Solutions
Several common issues can negatively affect memory management. One prevalent problem is the use of global variables that hold large amounts of data. These variables persist throughout the application’s lifetime, consuming memory even when not actively needed. Another common issue is the creation of unnecessary copies of large objects. This can be addressed by using techniques like pass-by-reference instead of pass-by-value. Finally, inefficient caching mechanisms can lead to excessive memory consumption. Reviewing and optimizing caching strategies is crucial for reducing memory footprint. Solutions involve careful refactoring to reduce global variable usage, employing efficient data structures to minimize object copying, and implementing intelligent caching mechanisms with well-defined expiration policies.
Best Practices for Minimizing Memory Usage in Large Applications
Minimizing memory usage in large applications requires a proactive approach.
- Use efficient data structures: Choose data structures appropriate for the task. Arrays are generally more memory-efficient than linked lists for sequential access. Hash tables offer fast lookups but may consume more memory than sorted arrays.
- Avoid unnecessary object creation: Reuse objects whenever possible to reduce the load on the garbage collector.
- Implement object pooling: Pre-allocate a pool of objects to be reused, reducing the overhead of creating new objects.
- Optimize string manipulation: String concatenation in loops can be inefficient. Use `StringBuilder` (or equivalent) to reduce memory allocations.
- Use memory profiling tools: Tools like Valgrind (for C/C++) or Java VisualVM can help identify memory leaks and other memory-related issues.
- Release resources promptly: Explicitly close files, network connections, and other resources as soon as they are no longer needed.
Code Profiling and Analysis
Optimizing code isn’t just about guessing; it’s about knowing exactly where your program spends its time and resources. This is where code profiling comes in – a crucial step in transforming sluggish code into a lean, mean, performance machine. Profiling tools provide the data-driven insights you need to make informed optimization decisions, moving beyond guesswork and into the realm of precise, targeted improvements.
The Importance of Code Profiling Tools
Code profiling tools are indispensable for identifying performance bottlenecks in your applications. They act like detective tools, meticulously tracking the execution of your code, pinpointing precisely which functions or code sections consume the most processing power or memory. Without profiling, optimization efforts can be haphazard and ineffective, like trying to fix a leaky faucet without knowing which pipe is leaking. Profiling offers a clear, quantifiable view of your code’s behavior, guiding you toward the most impactful optimizations. This targeted approach saves valuable development time and ensures your efforts yield significant performance gains.
Identifying Performance Bottlenecks Using Profiling Tools
Profiling tools work by instrumenting your code, collecting data on various aspects of its execution. This data might include the time spent in each function, the number of times functions are called, memory allocation patterns, and more. By analyzing this data, you can quickly identify the “hot spots” in your code – the areas responsible for the majority of the execution time or memory consumption. These hot spots represent the performance bottlenecks you need to address. The process often involves running your code with the profiler enabled, reviewing the generated reports, and focusing your optimization efforts on the identified bottlenecks. This iterative approach ensures you tackle the most significant performance issues first.
Different Types of Profiling Techniques
Profiling isn’t a one-size-fits-all solution; different techniques target different aspects of code performance.
- CPU Profiling: This focuses on identifying the parts of your code consuming the most CPU time. It helps pinpoint computationally intensive functions or loops that are slowing down your program. The results often show a breakdown of execution time spent in different parts of the code, allowing for precise identification of performance bottlenecks. For example, a CPU profile might reveal that a specific sorting algorithm is taking up 80% of the total execution time, highlighting it as a prime candidate for optimization.
- Memory Profiling: This technique tracks memory allocation and deallocation, helping to identify memory leaks or inefficient memory usage. It’s particularly useful for identifying large memory allocations, excessive memory fragmentation, or areas where memory is not being released properly. A memory profile might, for instance, show a steady increase in memory usage over time, indicating a potential memory leak that needs to be addressed.
Examples of Using Profiling Tools to Analyze Code Performance
Let’s illustrate with a few hypothetical scenarios.
- Scenario 1: A CPU profile reveals that a nested loop within a specific function is responsible for 90% of the execution time. This points to an opportunity to optimize the algorithm used within the loop, potentially by using a more efficient algorithm or reducing the number of iterations.
- Scenario 2: A memory profile shows that a particular data structure is causing excessive memory allocation. This suggests the need to switch to a more memory-efficient data structure, or to optimize the way data is managed within the existing structure.
Step-by-Step Guide on Using a Profiling Tool
Let’s consider a simple Python example and walk through a profiling process. Assume we have a function that calculates the factorial of a number using recursion:
“`python
def factorial_recursive(n):
if n == 0:
return 1
else:
return n * factorial_recursive(n-1)
factorial_recursive(5)
“`
To profile this, we’ll use the `cProfile` module, a built-in Python profiler.
- Import the `cProfile` module:
import cProfile
- Profile the function:
cProfile.run('factorial_recursive(5)')
- Analyze the output: The output will show a detailed breakdown of the function calls, including the number of calls, execution time, and cumulative time for each function. This allows you to identify the parts of the code that consume the most time. The output might show that the recursive calls dominate the execution time, suggesting that an iterative approach might be more efficient.
This simple example demonstrates how profiling can highlight performance issues and guide optimization strategies. More sophisticated profilers offer even more detailed insights and visualization capabilities.
Parallel and Concurrent Programming for Performance Improvement
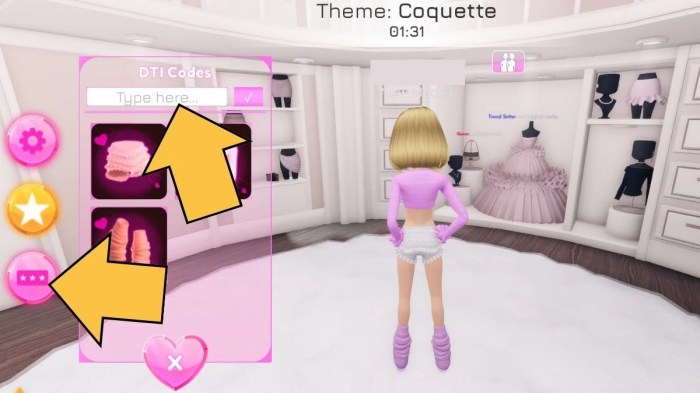
Source: progameguides.com
Unlocking the true potential of your code often involves harnessing the power of multiple processors or cores. Parallel and concurrent programming offer a pathway to significantly boost performance, especially when dealing with computationally intensive tasks or large datasets. By cleverly dividing the workload, you can achieve execution speeds far exceeding what’s possible with traditional single-threaded approaches.
Parallel and concurrent programming techniques allow you to execute multiple parts of your program simultaneously, dramatically reducing overall runtime. This is particularly beneficial for applications where independent tasks can be performed concurrently, like processing large images, analyzing massive datasets, or rendering complex 3D graphics. However, it’s important to understand the nuances of both parallel and concurrent programming, as they differ in their approaches and present unique challenges.
Multithreading and Multiprocessing
Multithreading and multiprocessing represent two primary approaches to parallel programming. Multithreading involves creating multiple threads within a single process, all sharing the same memory space. This offers a relatively lightweight approach to parallelism, ideal for tasks with frequent communication between threads. Multiprocessing, on the other hand, creates multiple independent processes, each with its own memory space. This provides greater isolation between tasks but involves higher overhead due to inter-process communication.
The choice between multithreading and multiprocessing depends on the specific application. For CPU-bound tasks where computation dominates, multiprocessing often yields better performance because it can fully utilize multiple cores. For I/O-bound tasks where the program spends a significant amount of time waiting for external resources (like network requests or disk I/O), multithreading can be more efficient, as one thread can perform I/O while others continue computation.
Streamlining your code isn’t just about faster load times; it’s about resilience. A well-optimized system is less vulnerable to exploits, which is why understanding the importance of robust security is key. Check out this article on The Benefits of Insuring Your Business Against Cybersecurity Risks to protect your digital assets. Ultimately, efficient code minimizes attack surfaces, leading to better performance and peace of mind.
Challenges of Concurrent Programming and Mitigation Strategies
Concurrent programming, while offering performance advantages, introduces complexities like race conditions, deadlocks, and data inconsistencies. A race condition occurs when multiple threads access and modify shared data simultaneously, leading to unpredictable results. A deadlock arises when two or more threads block each other indefinitely, waiting for resources held by each other. Data inconsistencies can occur when threads modify shared data without proper synchronization mechanisms.
Effective strategies to address these challenges include using synchronization primitives like mutexes (mutual exclusion locks) to protect shared resources, semaphores to control access to a limited number of resources, and condition variables to coordinate thread execution based on specific conditions. Careful design of data structures and algorithms is also crucial. For example, using thread-safe data structures can significantly reduce the risk of race conditions.
Comparison of Concurrency Models
Several concurrency models exist, each with its strengths and weaknesses. The shared-memory model, used in multithreading, relies on threads sharing the same memory space. This simplifies communication but necessitates careful synchronization. The message-passing model, often used in multiprocessing, involves processes communicating through explicit message exchanges. This provides greater isolation but requires more complex communication mechanisms.
Actor models, another popular approach, represent concurrency as a network of independent actors that communicate asynchronously through message passing. This model is particularly well-suited for distributed systems and fault tolerance. The choice of concurrency model depends on the application’s requirements and the trade-offs between performance, complexity, and scalability.
Performance Improvement with Parallel Programming: A Case Study
Consider the task of processing a large image. A single-threaded approach might involve iterating through each pixel sequentially, applying a filter or transformation. However, by dividing the image into smaller blocks and processing each block in parallel using multithreading or multiprocessing, the processing time can be drastically reduced. For instance, if we divide an image into four equal parts and process them concurrently on a four-core processor, we could theoretically achieve a four-fold speedup. In practice, the speedup might be slightly less due to overhead, but the improvement would still be substantial. This example demonstrates how parallel programming can significantly accelerate computationally intensive tasks.
Code Style and Readability for Optimization
While you might think code style is purely an aesthetic concern, its impact on performance is surprisingly significant, albeit indirect. Clean, well-structured code is easier to understand, maintain, and optimize. Conversely, messy, poorly written code can lead to hidden bugs, increased debugging time, and ultimately, slower execution. This section explores how improving your code style directly contributes to better performance.
Code style and readability influence performance through their effect on developer productivity and maintainability. Clean code is easier to debug and optimize, leading to fewer errors and faster execution. Conversely, poorly written code makes these tasks far more difficult, potentially leading to performance bottlenecks that could have been easily avoided.
Best Practices for Writing Clean and Maintainable Code
Writing clean code involves adhering to consistent formatting, using meaningful variable and function names, and structuring code logically. This improves readability and reduces the likelihood of errors. Consistency is key; use a style guide (like PEP 8 for Python) and stick to it. Meaningful names make your code self-documenting, reducing the need for excessive comments. Modular design, breaking down complex tasks into smaller, manageable functions, enhances code organization and reusability.
The Importance of Code Comments and Documentation
Comprehensive comments and documentation are crucial for understanding and maintaining code. They explain the purpose of code sections, clarify complex algorithms, and provide context for future modifications. Comments should be concise and accurate, avoiding redundant explanations of obvious code. Good documentation goes beyond comments; it includes clear descriptions of functions, classes, and modules, making the codebase easier to navigate and understand. Think of it as creating a roadmap for yourself and other developers.
Well-Structured Code and Debugging/Optimization
Well-structured code simplifies debugging and optimization by making it easier to identify performance bottlenecks. Modular design allows you to isolate problem areas and test individual components. Consistent naming conventions help you quickly locate specific functions or variables. Clear code reduces the time spent understanding the code’s logic before you can begin optimizing it. Imagine trying to optimize a spaghetti code mess versus a neatly organized, well-commented function – the difference is night and day.
Examples of Good and Bad Code Styles
Let’s compare two code snippets that accomplish the same task – calculating the sum of numbers in a list.
Bad Code Style:
a=[1,2,3,4,5];s=0;for(i=0;i
This is cramped, uses unclear variable names, and lacks comments. It's difficult to understand and maintain.
Good Code Style:
function sumNumbers(numbers) // Initialize the sum to 0 let sum = 0; // Iterate over the numbers array and add each number to the sum for (let i = 0; i < numbers.length; i++) sum += numbers[i]; // Return the calculated sum return sum; let numbers = [1, 2, 3, 4, 5]; let total = sumNumbers(numbers); console.log(total); // Output: 15
This example is well-formatted, uses descriptive variable names, includes comments, and is broken down into a reusable function. It is significantly easier to understand, debug, and optimize.
The difference in readability directly impacts the time spent understanding and modifying the code. The bad example could easily harbor subtle bugs, making optimization more challenging and potentially less efficient than the clean, well-structured alternative.
Advanced Optimization Techniques
Pushing your code's performance to the absolute limit often requires venturing beyond the basics. This section delves into advanced optimization strategies that can significantly impact your application's speed and efficiency, particularly in demanding scenarios. While these techniques can be powerful, they also demand a deeper understanding of your system's architecture and potential trade-offs.
Compiler Optimizations and Their Impact
Compilers aren't just translators; they're sophisticated tools capable of significantly improving your code's performance. Modern compilers employ a range of optimization techniques, including loop unrolling, inlining, constant folding, and dead code elimination. Loop unrolling reduces the overhead of loop iterations by replicating the loop body. Inlining replaces function calls with the function's code directly, avoiding the function call overhead. Constant folding evaluates constant expressions at compile time, eliminating runtime calculations. Dead code elimination removes code that has no effect on the program's output. The level of optimization applied is often configurable through compiler flags, allowing you to fine-tune the performance versus code size trade-off. For example, using the `-O3` flag in GCC often results in aggressive optimizations, but might increase compilation time. Understanding these optimizations and how to effectively utilize compiler flags is crucial for maximizing performance without manual code tweaking.
Assembly Language for Critical Performance Sections
For truly performance-critical sections of code, assembly language can provide unparalleled control. While less portable and more difficult to maintain than higher-level languages, assembly allows for direct manipulation of CPU registers and instructions, bypassing the limitations of compilers. This is particularly useful for highly optimized algorithms or low-level operations where every cycle counts. Consider a scenario where you're implementing a highly optimized cryptographic function. Using assembly language might allow you to leverage specialized CPU instructions for cryptographic operations, resulting in a substantial performance boost compared to a C++ implementation. However, the expertise required to write and maintain efficient assembly code is high, and it drastically reduces code readability and maintainability. This approach should be reserved for only the most critical sections of code where the performance gains significantly outweigh the development and maintenance costs.
Optimizing I/O Operations
Input/output (I/O) operations, such as reading from disk or network, are often significant bottlenecks in application performance. Optimizing I/O involves techniques like asynchronous I/O, buffering, and minimizing I/O operations. Asynchronous I/O allows the program to continue executing while I/O operations are performed in the background, preventing blocking. Buffering involves grouping multiple I/O requests into larger blocks, reducing the overhead of individual requests. Minimizing I/O operations often involves careful data structure design and algorithmic choices to reduce the frequency of disk or network access. For example, a database application might benefit from caching frequently accessed data in memory to reduce disk reads. Efficient I/O management can dramatically improve the responsiveness and throughput of your application, especially in data-intensive scenarios.
Scenarios Where Code Optimization is Unnecessary or Counterproductive
It's crucial to understand that not all code requires optimization. Premature optimization, focusing on optimizing code that doesn't represent a performance bottleneck, can be counterproductive, leading to more complex, less readable, and potentially buggy code. Optimization should be data-driven; profile your application to identify actual bottlenecks before investing time in optimizing code that has a negligible impact on overall performance. In many cases, choosing the right algorithm or data structure will yield far greater performance improvements than micro-optimizations. Furthermore, excessive optimization might lead to code that is difficult to understand, maintain, and debug, ultimately negating the performance gains.
Advanced Optimization Techniques Summary
Technique | Application | Potential Drawbacks |
---|---|---|
Compiler Optimizations | Improve performance automatically; fine-tuning via compiler flags. | Can increase compilation time; might not always be sufficient for critical sections. |
Assembly Language | Maximum control over CPU instructions for critical sections. | Reduced portability, maintainability, and readability; requires specialized expertise. |
I/O Optimization | Improve performance of input/output operations; asynchronous I/O, buffering, minimizing I/O calls. | Increased complexity; potential for errors in asynchronous operations; requires careful design. |
Final Summary
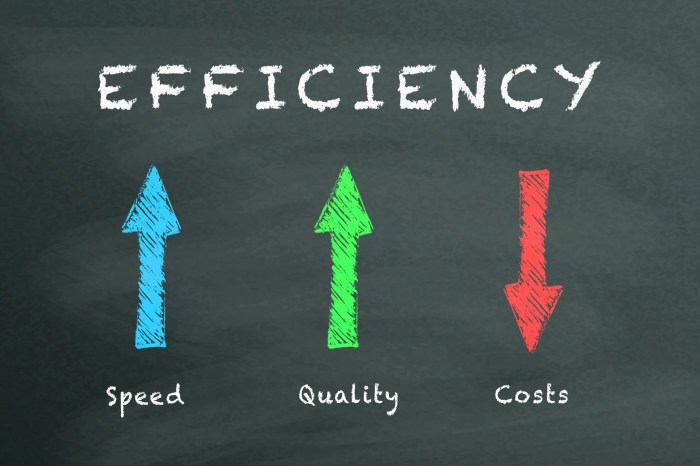
Source: cloudinary.com
So, there you have it – a comprehensive toolkit for boosting your code's performance. Remember, optimization is an ongoing process, not a one-time fix. By consistently applying these strategies and staying curious about new techniques, you'll create code that's not just functional but downright elegant and efficient. Now go forth and conquer those performance bottlenecks!