Introduction to Mobile Development with Swift: Dive headfirst into the electrifying world of iOS app creation! This isn’t your grandpappy’s coding; we’re talking sleek interfaces, powerful functionality, and the satisfying crunch of perfectly executed code. Get ready to unlock the secrets of Swift, the language that’s powering millions of apps, and learn how to build your own from scratch. We’ll cover everything from setting up your development environment to deploying your masterpiece to the App Store.
This guide will walk you through the essentials of Swift, comparing it to other mobile development languages and showcasing its unique advantages. We’ll explore the fundamentals of Swift syntax, object-oriented programming, and user interface design with SwiftUI. Prepare to master data handling, integrate third-party APIs, and tackle the challenges of bringing your app ideas to life. Think of it as your all-access pass to the exciting world of mobile app development.
What is Swift and its relevance to mobile development?
Swift is Apple’s powerful and intuitive programming language, specifically designed for building apps for iOS, macOS, watchOS, and tvOS. Think of it as the modern, streamlined successor to Objective-C, offering a cleaner syntax and a more enjoyable development experience. Its relevance to mobile development, particularly within the Apple ecosystem, is undeniable, given its widespread adoption and the massive user base of Apple devices.
Swift’s rise to prominence is largely due to its focus on safety and performance. It incorporates features that help developers write cleaner, more maintainable code, reducing the likelihood of common errors and leading to faster, more efficient apps. This makes it a compelling choice for developers of all skill levels, from seasoned professionals to enthusiastic beginners.
Advantages of Swift for iOS App Development
Swift boasts several key advantages that make it a top contender in the mobile development landscape. Its concise syntax allows developers to express complex ideas with less code, leading to faster development cycles and reduced maintenance overhead. The language’s focus on safety features like automatic memory management (via Automatic Reference Counting or ARC) significantly minimizes the risk of memory leaks and crashes, common pitfalls in other languages. Furthermore, Swift’s open-source nature fosters a vibrant community, resulting in abundant resources, libraries, and support for developers. Finally, Swift’s performance is exceptional, rivaling even the performance of languages traditionally considered speed demons. Apps built with Swift tend to be responsive and efficient, leading to a better user experience.
Comparison of Swift with Other Mobile Development Languages
While Swift reigns supreme in the Apple ecosystem, it’s crucial to compare it with other popular mobile development languages. Java and Kotlin are dominant players in the Android world. Java, while mature and widely used, can be verbose and less concise than Swift. Kotlin, a more modern language, addresses some of Java’s shortcomings with a cleaner syntax and improved features, but still primarily targets the Android platform. React Native, a framework using JavaScript, allows cross-platform development, targeting both iOS and Android with a single codebase. However, React Native apps often compromise on native performance and may require more effort to achieve a truly native look and feel compared to apps built natively with Swift (or Kotlin/Java). The choice depends on the project’s specific needs and priorities; for a pure iOS focus, Swift’s advantages are hard to ignore.
Swift Development Ecosystem and Tools
The Swift ecosystem is robust and supportive, offering developers a comprehensive suite of tools and resources. Xcode, Apple’s integrated development environment (IDE), provides a powerful and user-friendly environment for writing, debugging, and testing Swift code. It integrates seamlessly with Swift Package Manager (SPM), simplifying dependency management and library integration. A large and active community contributes to a vast collection of open-source libraries and frameworks, extending Swift’s capabilities and speeding up development. This rich ecosystem, combined with Apple’s extensive documentation and support, ensures developers have all the tools they need to succeed.
Comparison of Swift and Objective-C
Feature | Swift | Objective-C |
---|---|---|
Syntax | Modern, concise, and readable | Verbose and complex |
Memory Management | Automatic Reference Counting (ARC) | Manual memory management (prone to leaks) |
Error Handling | Modern error handling with do-catch blocks |
Older, less intuitive error handling |
Safety | Strong emphasis on type safety and null safety | Less emphasis on type safety, prone to null pointer exceptions |
Setting up the Development Environment: Introduction To Mobile Development With Swift
So, you’re ready to dive into the world of iOS app development? Awesome! Before you can start crafting the next killer app, you need to set up your development environment. Think of it as prepping your kitchen before you start baking that amazing cake – you wouldn’t start without the right ingredients and tools, right? This involves installing Xcode, Apple’s integrated development environment (IDE), and familiarizing yourself with some essential tools.
Xcode is your one-stop shop for everything iOS development. It’s where you’ll write your code, design your user interface, test your app, and prepare it for submission to the App Store. Getting it right is crucial for a smooth development journey.
Installing Xcode
Downloading and installing Xcode is straightforward. Head over to the Mac App Store and search for “Xcode.” It’s a large download (several gigabytes), so make sure you have a decent internet connection and plenty of free hard drive space. Once downloaded, the installation process is largely automated. You’ll likely be prompted to agree to Apple’s terms and conditions and choose where to install Xcode on your system. After installation, launch Xcode to ensure everything is working correctly. You might see a welcome screen with options to create a new project or explore existing templates.
Configuring Necessary Tools
Xcode comes bundled with most of the tools you’ll need. However, you might need to install additional components depending on your project’s requirements. For example, if you’re working with specific frameworks or libraries, you might need to download and integrate them into your project. Xcode’s interface provides clear guidance on managing these dependencies.
Organizing Project Files and Folders
A well-organized project is a happy project (and a developer!). Think of your project folder as your digital workspace. A messy workspace leads to frustration and lost time. Keep things neat and tidy from the start. A common approach is to separate code files (Swift files), assets (images, icons), and resource files (like storyboards and property lists) into distinct folders. This makes finding specific files a breeze.
The Importance of Version Control (Git)
Git is your best friend in mobile development. It’s a version control system that lets you track changes to your code, revert to previous versions if needed, and collaborate seamlessly with others. Think of it as an undo button on steroids. Using Git, whether through platforms like GitHub, GitLab, or Bitbucket, is essential for managing your project effectively and protecting your work. It allows for easy collaboration, rollback capabilities, and overall improved workflow.
Sample Project Folder Structure, Introduction to Mobile Development with Swift
Let’s say you’re building a simple to-do list app. A clean folder structure might look like this:
ToDoListApp/
├── ToDoListApp/ // Your main project folder
│ ├── AppDelegate.swift // Application delegate
│ ├── SceneDelegate.swift // Scene delegate
│ ├── ViewController.swift // Main view controller
│ ├── Assets.xcassets/ // Images, icons
│ │ ├── appIcon.png
│ │ └── otherImage.png
│ ├── Resources/ // Other resources
│ │ └── Localizable.strings // Localizations
│ └── ToDoList.swift // Model for the todo list
This structure clearly separates the different parts of your application, making navigation and maintenance much easier. Remember, consistency is key!
Fundamental Swift Concepts
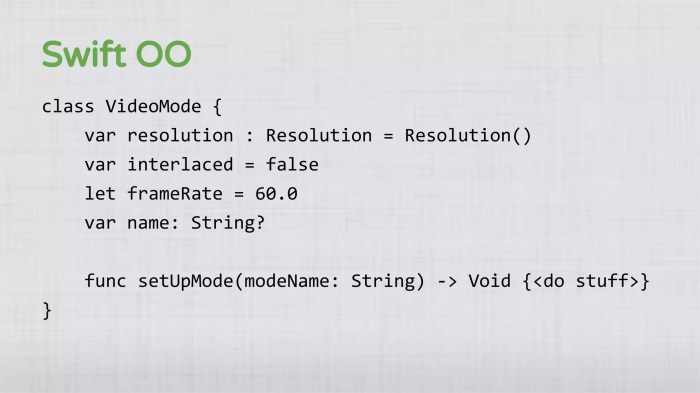
Source: slidesharecdn.com
Swift, Apple’s powerful programming language, forms the bedrock of iOS and macOS app development. Understanding its core features is crucial for building robust and efficient mobile applications. This section dives into the essential building blocks of Swift, providing a solid foundation for your mobile development journey.
Swift boasts a clean syntax and a rich type system, making it relatively easy to learn and use. Its focus on safety and performance helps developers create high-quality apps with fewer errors. Let’s explore some key aspects.
So you’re diving into the exciting world of mobile development with Swift? Building your app is a serious undertaking, and just like protecting your biggest investment, it’s smart to think about the bigger picture. That’s why understanding the importance of financial security is key; check out this article on Why Insurance is Critical for Protecting Your Home and Property for a helpful perspective.
Then, get back to coding that killer app!
Basic Syntax and Data Types
Swift’s syntax is designed for readability and clarity. Variables are declared using the `var` for mutable variables and `let` for constants. Data types are inferred automatically, but you can explicitly specify them for better code understanding. Common data types include `Int` (integers), `Double` (double-precision floating-point numbers), `String` (text), `Bool` (Boolean values – true or false), and more.
For example, declaring a variable named `age` and assigning it an integer value looks like this: var age: Int = 30
. A constant named `name` holding a string would be: let name: String = "John Doe"
. The type annotations (`: Int`, `: String`) are optional but highly recommended for clarity.
Control Flow Statements
Control flow statements dictate the order in which code executes. Swift provides standard `if-else` statements for conditional execution and various loop constructs for repetitive tasks.
The `if-else` statement allows code blocks to be executed based on a condition. For instance:
if age >= 18
print("Adult")
else
print("Minor")
Loops include `for` loops for iterating over a range or collection, and `while` loops for repeating a block as long as a condition is true. `for-in` loops are particularly useful for working with arrays and dictionaries.
for i in 1...5 //Closed Range
print(i)
var numbers = [1, 2, 3, 4, 5]
for number in numbers
print(number)
Object-Oriented Programming Concepts
Swift supports object-oriented programming (OOP) principles, enabling developers to structure code using classes and structures.
Classes are reference types, meaning multiple variables can point to the same instance. Structures, on the other hand, are value types; copying a structure creates a completely independent copy. Inheritance allows classes to inherit properties and methods from a parent class, promoting code reusability. Polymorphism allows objects of different classes to be treated as objects of a common type.
Here’s a simple example illustrating a class:
class Dog
var name: String
init(name: String)
self.name = name
func bark()
print("Woof!")
let myDog = Dog(name: "Buddy")
myDog.bark() // Output: Woof!
Closures and Higher-Order Functions
Closures are self-contained blocks of code that can be passed around and executed later. Higher-order functions accept other functions as arguments or return functions as results. This enables powerful functional programming techniques.
Consider this example of a higher-order function that takes a closure as an argument:
func operate(a: Int, b: Int, operation: (Int, Int) -> Int) -> Int
return operation(a, b)
let sum = operate(a: 5, b: 3, operation: (x, y) in x + y )
let difference = operate(a: 5, b: 3, operation: $0 - $1 ) //Shorthand syntax
print("Sum: \(sum), Difference: \(difference)") //Output: Sum: 8, Difference: 2
User Interface Design with SwiftUI
SwiftUI is Apple’s modern framework for building user interfaces across all Apple platforms. It’s a declarative approach, meaning you describe *what* you want your UI to look like, and SwiftUI handles the *how*. This drastically simplifies UI development, making it faster and less error-prone compared to imperative approaches. Forget wrestling with complex layouts – SwiftUI lets you focus on the user experience.
SwiftUI’s declarative nature means you define your UI using a series of Swift code statements that describe the desired visual hierarchy and data flow. This is in contrast to imperative UI frameworks where you explicitly manipulate UI elements through numerous function calls. The benefits are numerous: cleaner code, easier maintenance, and a more streamlined development process.
Declarative UI and its Benefits
Declarative UI in SwiftUI simplifies development by allowing developers to focus on describing the desired state of the UI rather than the steps to achieve that state. This approach results in code that is more concise, readable, and easier to maintain. Changes to the underlying data automatically update the UI, eliminating the need for manual updates. For example, if you update a variable holding the text for a label, the label automatically reflects that change. This eliminates a significant source of bugs found in imperative UI programming. Imagine building a complex form with dozens of fields; with SwiftUI’s data binding, updating the model automatically updates the UI, a huge time-saver.
Data Binding in SwiftUI
Data binding in SwiftUI connects UI elements to data models. Any change to the data automatically updates the corresponding UI element, and vice-versa. This two-way binding simplifies the process of updating the UI based on user interactions. Consider a simple text field where a user enters their name. With data binding, the entered name is automatically stored in a variable, which is then used elsewhere in your application. If that variable changes from another part of the app, the text field also updates.
Creating Different Types of Views and Layouts
Let’s build a simple user interface with a text field for a username, a button to submit the username, and a label to display a welcome message.
“`swift
import SwiftUI
struct ContentView: View
@State private var username = “”
@State private var welcomeMessage = “”
var body: some View
VStack
TextField(“Enter username”, text: $username)
.padding()
Button(“Submit”)
welcomeMessage = “Welcome, \(username)!”
.padding()
Text(welcomeMessage)
.padding()
“`
This code creates a vertical stack (`VStack`) containing a text field, a button, and a label. The `@State` property wrapper ensures that changes to `username` and `welcomeMessage` automatically update the UI. The `$username` in the `TextField` initializer creates the data binding, directly linking the text field’s content to the `username` state variable. The button’s action updates the `welcomeMessage` variable, triggering an automatic UI update. This simple example showcases the power and elegance of SwiftUI’s declarative approach and data binding. More complex layouts can be built using `HStack` (horizontal stack), `ZStack` (overlapping views), and other layout containers, all with the same ease and efficiency.
Working with Data
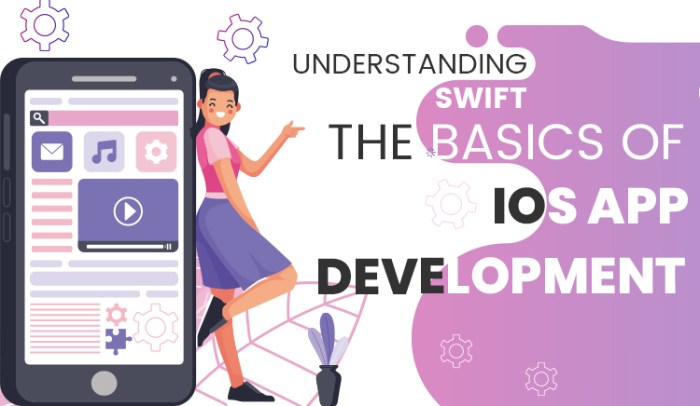
Source: 42works.net
Building amazing mobile apps isn’t just about pretty interfaces; it’s about how smoothly they handle information. This section dives into the nitty-gritty of data management in your Swift projects, covering everything from storing user preferences to fetching data from the internet. Get ready to become a data ninja!
Data Persistence with Core Data and UserDefaults
Core Data and UserDefaults offer distinct approaches to data persistence, each suited for different needs. UserDefaults is ideal for storing small amounts of simple data like user settings or preferences. Think of it as your app’s quick-access memory. It’s easy to use, but not designed for large datasets or complex relationships between data points. Core Data, on the other hand, is a powerful framework for managing larger, more complex datasets. It provides features like data modeling, relationships, and efficient data storage. Imagine Core Data as your app’s robust filing cabinet, perfect for organizing and managing significant amounts of information. Choosing between the two depends on your app’s specific requirements.
Working with JSON Data
JSON (JavaScript Object Notation) is the lingua franca of data exchange on the web. It’s a lightweight, text-based format that’s easy for both humans and machines to read and parse. In Swift, you can easily decode JSON data into Swift structures using the `Codable` protocol. This allows you to seamlessly translate the structured data from a JSON response into easily manageable Swift objects. For example, imagine receiving a JSON response representing a list of products from an e-commerce API. Using `Codable`, you can map this JSON data directly to a Swift `struct` representing a product, complete with properties like name, price, and description. This simplifies data handling significantly.
Data Modeling
Effective data modeling is crucial for creating well-structured and maintainable apps. This involves defining the structure and relationships between different data points within your application. In Swift, you can model your data using `structs` or `classes`. `Structs` are generally preferred for simpler data structures due to their value-type semantics, while `classes` are more suitable for complex data with reference-type behavior and inheritance. Consider a social media app: you might model a “User” with properties like username, profile picture, and posts. You might also model a “Post” with properties like text content, timestamp, and images. Careful consideration of these data models is key to creating an efficient and scalable app.
Fetching and Displaying Data from a Remote API
Fetching data from a remote API is a common task in mobile development. This typically involves making a network request (usually using `URLSession`) to a server endpoint, receiving a response (often in JSON format), parsing the response, and then displaying the data in your app’s user interface. For instance, a weather app might fetch weather data from a weather API. The process involves creating a URL request, handling the response (checking for errors, handling different HTTP status codes), parsing the JSON data into Swift objects (using `Codable`), and then updating the UI with the retrieved information (e.g., displaying temperature, humidity, and weather conditions). Error handling is crucial here; you need to gracefully handle network errors and data parsing errors to prevent your app from crashing. Implementing proper error handling and loading indicators is key to a smooth user experience.
Advanced Topics in Mobile Development
So, you’ve mastered the basics of Swift and SwiftUI. You’re building functional apps, but now it’s time to level up your game. This section dives into the more advanced aspects of mobile development, transforming your apps from good to great. We’ll cover crucial areas that often separate hobby projects from polished, professional applications.
Location Services Implementation
Integrating location services adds a whole new dimension to your apps, enabling features like location-based reminders, nearby searches, and real-time tracking. This involves using Core Location framework, which provides access to the device’s GPS and other location-related data. You’ll need to request user permission to access their location, handle authorization status changes gracefully, and understand the implications of battery consumption. Proper error handling is key, as location services can be unreliable at times. For example, an app like a ride-sharing service heavily relies on accurate and timely location data for both the driver and the passenger. Implementing location services involves carefully managing permissions, choosing appropriate accuracy levels, and handling potential errors like network outages or GPS signal loss.
Integrating Third-Party Libraries and APIs
Leveraging existing libraries and APIs is a cornerstone of efficient development. Instead of reinventing the wheel, you can tap into pre-built functionalities for tasks like payment processing, social media integration, or map displays. Swift Package Manager (SPM) simplifies the process of incorporating external code into your projects. However, careful consideration must be given to the library’s reputation, security, and licensing terms. For instance, integrating a payment gateway like Stripe allows you to seamlessly add in-app purchase capabilities without having to build your own complex payment processing system. Similarly, using the MapKit framework saves you the trouble of building your own mapping functionality.
Common Challenges and Solutions
Mobile development is not without its hurdles. Performance optimization is crucial, especially on resource-constrained devices. Memory management is a key aspect of avoiding crashes and ensuring smooth operation. Debugging can be tricky, requiring a systematic approach and the use of debugging tools. Handling asynchronous operations effectively is also vital, as many tasks, like network requests, happen in the background. For example, an app might experience slowdowns due to inefficient image loading. Optimizing image sizes and using caching mechanisms can significantly improve performance. Another common challenge is managing app size; minimizing unnecessary assets and using compression techniques can keep your app download size manageable.
Deploying to the App Store
Getting your app onto the App Store involves several steps. You’ll need an Apple Developer account, and your app must adhere to Apple’s strict guidelines. The process includes creating an App Store Connect listing, configuring your app’s metadata, managing app versions, and submitting your app for review. Thorough testing is essential to avoid rejection due to bugs or compatibility issues. Apple’s review process can take time, so patience is key. A well-prepared submission, with clear screenshots and a comprehensive description, increases the likelihood of a smooth and swift approval. Successfully navigating this process requires attention to detail and adherence to Apple’s guidelines.
Illustrative Examples
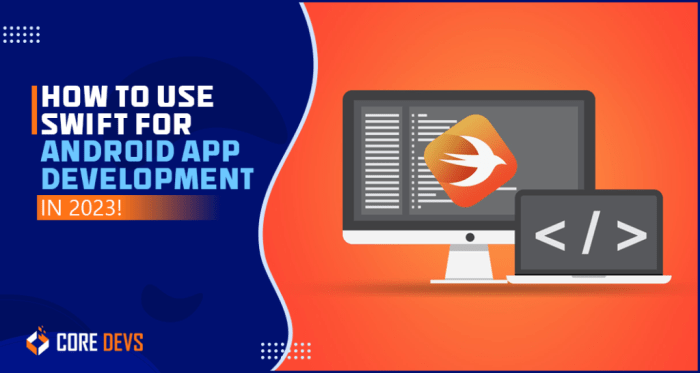
Source: coredevsltd.com
Let’s bring our Swift knowledge to life with some concrete examples. We’ll explore the visual design of two common app types: a calculator and a to-do list. These examples will highlight how design choices impact user experience and how those choices can be implemented using SwiftUI.
This section details the visual aspects, focusing on the user interface (UI) elements and their arrangement. Remember, this is just a design overview; the actual implementation would involve Swift code to handle functionality.
Calculator App Visual Design
Imagine a simple calculator app on a phone screen. The layout is primarily numerical, featuring a grid of buttons. The numbers 0-9 are arranged in a 3×3 grid, occupying the lower portion of the screen. Each number button is square, with a clean sans-serif font like Helvetica Neue in a size large enough for easy tapping. The background of these buttons is a muted grey (#D3D3D3), providing contrast against the white numbers. Above the number buttons, a row of operator buttons (+, -, *, /, =) spans the width of the screen, also using the same muted grey background and white font. The equal sign (=) button is slightly wider than the other operator buttons. At the very top of the screen, a display area shows the current calculation and the result, using a larger, bolder font (perhaps Helvetica Neue Bold) in black on a light grey (#F0F0F0) background. The overall color scheme is minimalist and clean, promoting readability and ease of use. Rounded corners are used on all buttons for a softer look.
To-Do List App Visual Design
A to-do list app presents tasks in a clear, organized manner. Imagine a vertical list of tasks, each displayed as a single row. Incomplete tasks are shown with a checkbox that’s empty (unchecked) next to the task description. The task description uses a regular weight sans-serif font, like Open Sans. Completed tasks have a filled checkbox (checked), and the text is displayed in a lighter grey (#A9A9A9) to visually indicate completion. The background is a clean white, providing good contrast. Each task row has a subtle bottom border (a light grey line) to separate it from the next task. A button at the bottom allows users to add new tasks, clearly labeled “Add Task” with a slightly bolder font weight than the task descriptions. The app uses a consistent, simple layout, making it easy to manage and review the list of to-do items.
Concluding Remarks
Building your first iOS app might seem daunting, but with a solid understanding of Swift and the right tools, you’ll be amazed at what you can create. This introduction to mobile development with Swift has equipped you with the foundational knowledge and practical skills to embark on your coding journey. Remember, practice makes perfect – so start building, iterate, and most importantly, have fun!