How to Manage Asynchronous Code in JavaScript with Promises? Sounds intimidating, right? But fear not, fellow coder! This isn’t some arcane JavaScript wizardry; it’s the key to unlocking smooth, responsive web applications. We’ll unravel the mysteries of asynchronous operations, conquer the complexities of Promises, and emerge victorious, wielding the power of `.then()`, `.catch()`, and `async/await` like seasoned pros. Prepare for a deep dive into the world of non-blocking code, where your JavaScript dreams become reality.
This guide navigates the landscape of asynchronous JavaScript, starting with the fundamentals of Promises and their states. We’ll explore chaining Promises for elegant sequential operations, and harness the power of `Promise.all` and `Promise.race` for concurrent tasks. Then, we’ll conquer the cleaner syntax of `async/await`, mastering error handling along the way. Finally, we’ll briefly touch upon alternatives, ensuring you have a well-rounded understanding of managing asynchronous code in JavaScript.
Introduction to Asynchronous JavaScript
JavaScript, at its core, is a single-threaded language. This means it executes one task at a time. However, the web is inherently asynchronous; users interact, data loads from servers, and animations play concurrently. This creates a need for JavaScript to handle multiple operations seemingly simultaneously without blocking the main thread and freezing the user interface. Understanding this asynchronous nature is crucial for building responsive and efficient web applications.
Asynchronous operations are those that don’t block the execution of other code while they’re running. Instead of waiting for a lengthy process (like fetching data from a server) to complete before moving on, asynchronous code allows other tasks to proceed. This prevents the browser from becoming unresponsive, ensuring a smooth user experience.
Examples of Asynchronous Operations
Many common web development tasks rely on asynchronous programming. Consider fetching data from an API: if the request took a long time, a synchronous approach would freeze the entire page until the response arrived. This is unacceptable. Asynchronous requests, however, allow the user to continue interacting with the page while the data is being retrieved in the background. Other examples include: handling user inputs (clicks, form submissions), manipulating the DOM (Document Object Model) after animations complete, and setting timers or intervals.
Challenges of Managing Asynchronous Operations Without Promises
Before the introduction of Promises, managing asynchronous operations in JavaScript was notoriously difficult. The primary tool was callbacks, which, when nested deeply (often referred to as “callback hell”), became incredibly hard to read, maintain, and debug. Imagine a scenario where you need to perform three sequential asynchronous operations: fetching data, processing it, and then updating the UI. With callbacks, this would result in a pyramid of nested functions, making it extremely challenging to track the flow of execution and handle errors effectively. For instance, consider a simple example: an API call to fetch user data, followed by another call to fetch their posts, and finally updating a user profile display. The nesting of callbacks would make error handling complex and the code almost unreadable. This lack of structure and readability led to significant challenges in managing complexity and ensuring the reliability of asynchronous JavaScript code.
Understanding JavaScript Promises
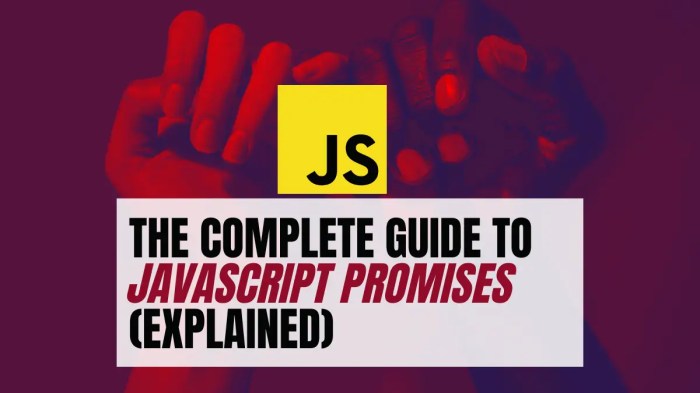
Source: becomebetterprogrammer.com
Mastering asynchronous JavaScript with Promises is crucial for building robust web apps, ensuring smooth user experiences. But even the best code can face unexpected hiccups; that’s where a solid risk management strategy comes in, like understanding the importance of The Role of Insurance in Protecting Your Online Business , especially for those relying on online transactions. Proper insurance can help mitigate potential financial losses, letting you focus on perfecting your Promise-based JavaScript and delivering a killer product.
Promises are the unsung heroes of asynchronous JavaScript, smoothing out the bumpy ride of dealing with operations that take time to complete, like fetching data from a server or processing large files. They provide a cleaner, more manageable way to handle these asynchronous tasks compared to older callback-based approaches. Think of them as IOUs – a promise that a value will eventually be delivered (or that an error occurred).
At its core, a JavaScript Promise is an object representing the eventual completion (or failure) of an asynchronous operation. It exists in one of three states: pending, fulfilled, or rejected. The pending state is the initial state; the promise hasn’t yet completed or failed. Fulfilled indicates the operation completed successfully, and a result value is available. Rejected means the operation failed, and a reason for the failure (usually an error object) is available.
Creating Promises with the Promise Constructor
Creating a Promise involves using the Promise
constructor, which takes a single argument: an executor function. This executor function receives two arguments: resolve
and reject
. resolve
is called when the asynchronous operation is successful, passing the result value. reject
is called if the operation fails, passing the reason for failure.
Here’s an example of creating a promise that simulates fetching data after a 2-second delay:
const myPromise = new Promise((resolve, reject) =>
setTimeout(() =>
const data = message: 'Data fetched successfully!' ;
resolve(data); // Resolve the promise with the data
, 2000);
);
myPromise.then(data => console.log(data.message));
In this example, if the setTimeout
function completes without errors, the resolve
function is called, passing the data
object. If an error occurs within the setTimeout
(though unlikely in this simple example), the reject
function would be called instead.
Using .then(), .catch(), and .finally(), How to Manage Asynchronous Code in JavaScript with Promises
Once a promise is created, you use the .then()
, .catch()
, and .finally()
methods to handle its eventual resolution or rejection.
The .then()
method takes a callback function that is executed when the promise is fulfilled. This callback receives the resolved value as an argument. The .catch()
method takes a callback function that is executed when the promise is rejected. This callback receives the rejection reason as an argument. Finally, .finally()
executes a callback regardless of whether the promise is fulfilled or rejected; it’s often used for cleanup tasks.
myPromise
.then(data =>
console.log('Success:', data.message);
return fetchMoreData(); //Chaining promises
)
.then(moreData => console.log('More data:', moreData))
.catch(error => console.error('Error:', error))
.finally(() => console.log('Promise execution complete'));
This example demonstrates chaining promises using multiple .then()
calls. The .catch()
method handles any errors that occur in either .then()
block, and .finally()
ensures a cleanup message is logged regardless of success or failure.
Chaining Promises
So you’ve grasped the basics of Promises – fantastic! Now let’s level up your asynchronous JavaScript game by learning how to chain them together. Chaining allows you to execute multiple asynchronous operations sequentially, making your code cleaner and easier to follow, especially when dealing with complex workflows. Think of it like an assembly line for your asynchronous tasks.
Chaining promises is all about using the `.then()` method to link one promise to the next. Each `.then()` handles the result of the previous promise, passing it on as input to the next. This creates a sequence where the next task only starts once the previous one is complete. It’s a beautiful dance of asynchronous elegance.
Promise Chaining with .then()
The `.then()` method takes a function as an argument. This function receives the resolved value of the preceding promise and returns a new promise (or a value, which implicitly becomes a resolved promise). This allows you to create a chain of promises, where the result of one promise feeds into the next. Let’s illustrate with a simple example:
“`javascript
const promise1 = new Promise((resolve, reject) =>
setTimeout(() => resolve(“Step 1 complete”), 1000);
);
promise1
.then(result =>
console.log(result); // Outputs: “Step 1 complete”
return new Promise((resolve, reject) =>
setTimeout(() => resolve(“Step 2 complete”), 1000);
);
)
.then(result =>
console.log(result); // Outputs: “Step 2 complete”
);
“`
In this example, `promise1` resolves after a second, passing its result to the first `.then()`. This `.then()` then returns another promise that resolves after another second, and its result is handled by the second `.then()`. See how smoothly they flow into each other?
Error Handling in Chained Promises with .catch()
Even the best-laid plans can go awry. That’s why error handling is crucial in asynchronous operations. The `.catch()` method acts as a safety net for your promise chain. It catches any errors that occur in any of the promises in the chain and handles them gracefully, preventing your entire application from crashing.
“`javascript
const promise1 = new Promise((resolve, reject) =>
setTimeout(() => reject(“Something went wrong!”), 1000);
);
promise1
.then(result =>
console.log(result);
)
.catch(error =>
console.error(“Error:”, error); // Outputs: “Error: Something went wrong!”
);
“`
Here, `promise1` rejects. The `.catch()` method at the end of the chain intercepts the error, preventing it from propagating further. This is crucial for robust asynchronous programming. You can even place `.catch()` at various points in the chain for more granular error handling.
Complex Asynchronous Operation with Chained Promises
Let’s visualize a more involved scenario: fetching user data, then their posts, and finally displaying a summary. This involves multiple asynchronous steps, perfectly suited for promise chaining.
Step | Action | Promise State | Result |
---|---|---|---|
1 | Fetch User Data | Resolved | userId: 1, userName: “HipweeUser” |
2 | Fetch User Posts (using userId from Step 1) | Resolved | [postId: 1, title: “Promise Chain Magic”, postId: 2, title: “Async Awaits are Cool Too”] |
3 | Display Summary (userName and post titles) | Resolved | HipweeUser’s posts: Promise Chain Magic, Async Awaits are Cool Too |
4 | (Error Handling) Network Issue during Post Fetch | Rejected | Error: Network request failed |
This table illustrates a typical flow. Step 4 shows how a `.catch()` could handle a network error during the post fetching stage. This prevents the entire operation from failing; instead, it allows for graceful degradation or user notification. The power of chaining is clear: it orchestrates a complex series of asynchronous tasks into a well-managed, error-resistant sequence.
Promise.all and Promise.race
Let’s dive into two powerful methods that significantly enhance your asynchronous JavaScript prowess: `Promise.all` and `Promise.race`. These methods allow you to orchestrate multiple promises, handling them either concurrently or competitively, depending on your needs. Understanding their differences and applications is crucial for building efficient and robust asynchronous applications.
These methods offer distinct approaches to managing multiple promises. `Promise.all` executes all provided promises concurrently and resolves only when *all* promises have resolved successfully. `Promise.race`, on the other hand, resolves or rejects as soon as the *first* promise in the iterable resolves or rejects, ignoring the rest.
Promise.all: Concurrent Promise Execution
`Promise.all` takes an iterable (like an array) of promises as input. It returns a single promise that resolves when all the input promises resolve. The resolved value is an array containing the resolved values of the input promises in the same order. If any of the input promises reject, the `Promise.all` promise rejects immediately, and the rejection reason is the rejection reason of the first rejected promise. This makes it ideal for scenarios where you need all operations to complete successfully before proceeding.
Imagine you’re fetching data from multiple APIs simultaneously. Using `Promise.all`, you can fetch them concurrently and then process the combined results once all requests have returned successfully.
const apiUrls = [
'https://api.example.com/data1',
'https://api.example.com/data2',
'https://api.example.com/data3'
];
const fetchData = url => fetch(url).then(response => response.json());
Promise.all(apiUrls.map(fetchData))
.then(results =>
console.log('All data fetched successfully:', results);
// Process the combined results from all APIs
)
.catch(error =>
console.error('Error fetching data:', error);
);
This code snippet fetches data from three different API endpoints concurrently. The `Promise.all` method ensures that the code inside the `.then` block only executes after all three fetches are complete and successful. Any error during any of the fetches will trigger the `.catch` block.
Promise.race: Selecting the Fastest Promise
`Promise.race` is designed for situations where you need the result of the fastest-completing promise. It takes an iterable of promises as input and returns a single promise that resolves or rejects as soon as the first input promise resolves or rejects. The resolved or rejected value is the resolved or rejected value of the first settled promise.
Consider a scenario where you have multiple backup servers and need to connect to the first available one as quickly as possible. `Promise.race` provides an elegant solution.
const serverUrls = [
'https://server1.example.com',
'https://server2.example.com',
'https://server3.example.com'
];
const connectToServer = url =>
return new Promise((resolve, reject) =>
setTimeout(() =>
// Simulate a connection attempt with varying success and latency
const success = Math.random() > 0.2; // 80% chance of success
if (success)
resolve(`Connected to $url`);
else
reject(`Failed to connect to $url`);
, Math.random() * 2000); // Simulate latency between 0 and 2 seconds
);
;
Promise.race(serverUrls.map(connectToServer))
.then(result => console.log(result))
.catch(error => console.error(error));
This example simulates connecting to three servers with varying connection times and success rates. `Promise.race` ensures that the application connects to the first server that responds successfully, even if others are still attempting to connect or fail. This minimizes latency and improves responsiveness.
Async/Await Syntax
Async/await is JavaScript’s syntactic sugar for working with promises, making asynchronous code look and behave a bit more like synchronous code. It’s a cleaner, more readable alternative to the `.then()` chaining method we’ve previously explored, especially when dealing with multiple asynchronous operations. Think of it as a more elegant way to manage the complexities of promises without sacrificing the power and flexibility they offer.
Async/await builds directly upon the promise foundation. The `async` transforms a function into an asynchronous function that implicitly returns a promise. The `await` can only be used inside an `async` function; it pauses execution until a promise resolves (or rejects), returning the resolved value or throwing an error. This allows you to write asynchronous code that reads like synchronous code, improving readability and maintainability significantly.
Async Function Declaration and Usage
Declaring an `async` function is straightforward: simply prefix the `function` with `async`. The function then implicitly returns a promise, whether you explicitly use `return` or not. If the function doesn’t explicitly return a value, it implicitly returns a resolved promise with `undefined`. The `await` , used within the `async` function, pauses execution until the promised value is available. This makes handling multiple asynchronous operations much simpler and easier to follow. Consider this example:
“`javascript
async function fetchData()
try
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
return data;
catch (error)
console.error(‘Error fetching data:’, error);
return null; // or throw error; See best practices below.
fetchData().then(data =>
if (data)
console.log(‘Data received:’, data);
else
console.log(‘Failed to retrieve data.’);
);
“`
This example fetches data from a hypothetical API. The `await` ensures that `response.json()` only executes after the `fetch` promise resolves. The `try…catch` block handles potential errors during the fetch process.
Error Handling with Async/Await
Effective error handling is crucial in asynchronous programming. Async/await simplifies this with the familiar `try…catch` block. However, best practices should be followed for robust error management.
Best Practices for Error Handling in Async/Await: Always wrap your asynchronous operations within a `try…catch` block. Handle errors gracefully, providing informative error messages to the user (or logging them for debugging). Consider whether to re-throw the error or return a default value depending on the context and desired behavior. Avoid swallowing exceptions completely – always log them, at least, for debugging purposes. For critical errors, re-throwing allows for higher-level error handling mechanisms to take over.
This example demonstrates a more robust approach to error handling, specifically highlighting the importance of logging errors for debugging purposes, even if a default value is returned to prevent application crashes. In a real-world application, you would likely replace `console.error` with more sophisticated logging mechanisms.
Handling Errors in Asynchronous Code: How To Manage Asynchronous Code In JavaScript With Promises
Asynchronous JavaScript, while powerful, introduces complexities in error handling. Unlike synchronous code where errors halt execution immediately, asynchronous operations can leave errors bubbling silently, potentially leading to unexpected behavior and application crashes. Understanding how to effectively manage these errors is crucial for building robust and reliable JavaScript applications.
Errors in asynchronous JavaScript typically arise from network requests failing, database operations encountering issues, or problems within the asynchronous function itself. These can manifest as rejected promises, exceptions thrown within async functions, or even unhandled rejections that silently crash your application. Proper error handling ensures graceful degradation, providing informative feedback to users and preventing catastrophic failures.
Using try…catch Blocks with Async/Await
The `try…catch` block is a familiar construct for handling synchronous errors, but it also plays a crucial role in managing exceptions within asynchronous functions using `async/await`. The `try` block encloses the asynchronous operation, and any `throw` statements or rejected promises within this block will be caught by the `catch` block. This allows for centralized error handling and prevents the unhandled rejection from silently crashing the application.
Consider a scenario where you’re fetching data from an API. If the API request fails (e.g., due to a network error or a server-side problem), the promise will reject. Wrapping the API call within a `try…catch` block ensures that you can handle this rejection gracefully, potentially displaying an error message to the user or retrying the request.
async function fetchData()
try
const response = await fetch('/api/data');
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
const data = await response.json();
return data;
catch (error)
console.error('Error fetching data:', error);
// Handle the error, e.g., display an error message to the user
return null; // or throw the error to be handled further up the call stack
Utilizing Promise.catch()
The `.catch()` method is a specific way to handle rejected promises. It provides a clean and concise way to handle errors that originate from a promise chain. Unlike `try…catch`, which catches any error within its scope, `.catch()` specifically targets errors stemming from a rejected promise. This method is particularly useful when dealing with multiple asynchronous operations linked together through a promise chain.
If a promise in the chain rejects, the execution jumps directly to the `.catch()` block, preventing further execution down the chain. This simplifies error handling in complex asynchronous flows, ensuring that errors don’t propagate unchecked.
fetch('/api/data')
.then(response => response.json())
.then(data =>
// Process the data
)
.catch(error =>
console.error('Error:', error);
// Handle the error
);
Best Practices for Robust Error Handling
Implementing robust error handling requires a proactive approach. It’s not just about catching errors but also about preventing them and providing meaningful feedback.
- Always handle rejected promises: Never let a promise reject silently. Use `.catch()` to handle any potential errors.
- Use specific error types: Throw custom error objects with descriptive messages to aid in debugging.
- Centralized error logging: Implement a centralized logging mechanism to track errors across your application.
- Provide informative error messages to users: Avoid cryptic error messages; instead, provide user-friendly explanations.
- Implement retry mechanisms: For transient errors (e.g., network issues), consider implementing retry logic with exponential backoff.
- Graceful degradation: Design your application to gracefully handle errors without crashing, potentially providing fallback mechanisms.
Advanced Promise Techniques
So you’ve mastered the basics of Promises in JavaScript? Fantastic! Now let’s dive into some more advanced techniques that will elevate your asynchronous coding game to the next level. We’ll explore powerful tools that provide greater control and flexibility when working with asynchronous operations.
These advanced techniques allow for more elegant and efficient handling of asynchronous tasks, improving code readability and maintainability. Understanding these concepts will make you a true JavaScript ninja when it comes to managing asynchronous code.
Promise.resolve and Promise.reject
Promise.resolve
and Promise.reject
are factory methods that allow you to create resolved or rejected Promises directly, without the need for a constructor. This is particularly useful when you need to create a Promise from a synchronous value or want to immediately resolve or reject a Promise based on a condition. Promise.resolve(value)
creates a Promise that is immediately resolved with the given value
, while Promise.reject(reason)
creates a Promise that is immediately rejected with the given reason
.
For example, Promise.resolve(10)
creates a resolved Promise with the value 10, and Promise.reject("Error occurred")
creates a rejected Promise with the reason “Error occurred”. This simplifies handling scenarios where you already have a value or error condition and want to seamlessly integrate it into the Promise chain.
Creating Custom Promise-based APIs
Building custom Promise-based APIs allows you to encapsulate asynchronous operations within a clean and consistent interface. This improves code organization and makes your asynchronous functions easier to use and understand. By returning a Promise from your custom function, you can chain subsequent operations using `.then()` and `.catch()`.
Consider a function that fetches user data from an API. Instead of handling the complex network request directly in the calling code, you can create a function that returns a Promise. The Promise will resolve with the user data if the request is successful, or reject with an error if something goes wrong. This abstracts away the implementation details and provides a simple, consistent interface for the calling code.
Example:
function fetchUserData(userId)
return new Promise((resolve, reject) =>
// Simulate an API call
setTimeout(() =>
const userData = id: userId, name: 'John Doe' ;
resolve(userData); // Resolve with user data
, 1000);
);
This fetchUserData
function returns a Promise. The calling code can then use `.then()` to handle the resolved data and `.catch()` to handle any errors.
Real-World Scenario: User Authentication
Let’s illustrate the power of Promises with a common real-world application: user authentication. Imagine a login system where a user submits their credentials. The system needs to verify these credentials against a database or an external authentication service. This process is inherently asynchronous.
A Promise-based approach allows you to elegantly handle the asynchronous nature of this operation. You could create a function, authenticateUser(username, password)
, that returns a Promise. This Promise resolves with the user’s data if authentication is successful, and rejects with an error message otherwise. The calling code can then chain `.then()` to handle successful login (e.g., redirecting the user to their profile page) and `.catch()` to handle authentication failures (e.g., displaying an error message to the user).
The beauty of this approach lies in its clean separation of concerns. The authentication logic is encapsulated within the authenticateUser
function, while the calling code simply handles the success or failure cases using the Promise’s API. This makes the code more modular, readable, and maintainable.
Alternatives to Promises (Brief Overview)
While Promises are a powerful tool for managing asynchronous JavaScript, they aren’t the only game in town. Understanding alternative approaches helps you choose the best tool for the job, especially in legacy codebases or situations where Promises might feel a bit overkill. This section briefly explores callbacks and async iterators, comparing them to Promises to highlight their strengths and weaknesses.
Before diving into the specifics, it’s important to remember that the choice between Promises, callbacks, and async iterators often depends on the complexity of your asynchronous operations and the overall structure of your application. Simplicity and maintainability are key considerations.
Callbacks
Callbacks, the oldest method for handling asynchronous operations in JavaScript, are functions passed as arguments to other functions, executed after an asynchronous operation completes. They’re simple to understand and implement, but their main drawback is the notorious “callback hell” – deeply nested callbacks that make code hard to read and maintain. Imagine a situation where you need to perform three sequential asynchronous operations: fetching data, processing it, and then displaying it. With callbacks, this would lead to a pyramid of nested functions, making debugging and modification a nightmare.
Async Iterators
Async iterators provide a more elegant way to handle asynchronous sequences of data. They are particularly useful when dealing with streams of data, such as reading large files or processing data from a network connection. Unlike Promises, which resolve to a single value, async iterators allow you to iterate over a sequence of values, one at a time, asynchronously. This approach excels in scenarios where you need to process a large number of items sequentially without blocking the main thread. However, they might not be the best choice for simple asynchronous operations.
Promises vs. Callbacks vs. Async Iterators
The choice between Promises, callbacks, and async iterators depends heavily on context. Callbacks are best suited for simple, single asynchronous operations, but quickly become unwieldy for more complex scenarios. Promises offer a more structured and manageable way to handle multiple asynchronous operations, especially when chaining operations. Async iterators are ideal for processing streams of data, offering a cleaner approach than managing multiple Promises in a loop. Choosing the right tool depends on the project’s specific needs, balancing complexity with readability and maintainability. For most modern JavaScript projects, Promises offer a superior balance of power and readability, but understanding callbacks and async iterators allows you to appreciate the full spectrum of options available.
Closure
Mastering asynchronous JavaScript with Promises isn’t just about writing cleaner code; it’s about building faster, more responsive applications that users will love. By understanding the core concepts, from basic Promise handling to the elegance of `async/await`, you’ll be equipped to tackle even the most complex asynchronous operations. So ditch the callback hell and embrace the power of Promises – your users (and your sanity) will thank you for it. Now go forth and build amazing things!