How to Create Animations with CSS and JavaScript? Let’s ditch the boring static websites and dive into the vibrant world of web animation! This guide will walk you through creating everything from subtle fades to complex, interactive effects using the power of CSS and JavaScript. We’ll cover the basics, explore advanced techniques, and even troubleshoot those pesky animation glitches. Get ready to level up your web design game!
We’ll explore the strengths of both CSS and JavaScript for animation, showing you when to use each and how to combine them for maximum impact. Think smooth, efficient animations that will wow your users – no more clunky, jarring transitions. We’ll cover key concepts like `@keyframes`, `requestAnimationFrame`, and easing functions, providing practical examples and clear explanations along the way. Prepare to unleash your inner animation wizard!
Introduction to CSS Animations
CSS animations? Think of them as the secret sauce that transforms static websites into dynamic, engaging experiences. No need for JavaScript acrobatics for simple movements – CSS handles it gracefully, making your website feel alive and interactive. We’re diving into the core concepts, showing you how to bring your web elements to life with smooth, stylish transitions.
At its heart, CSS animation lets you control the visual changes of an element over a specified duration. You define the start and end states, and CSS smoothly transitions between them. It’s a powerful tool for creating everything from subtle hover effects to complex, attention-grabbing sequences. Forget clunky JavaScript; CSS animations are often simpler, more efficient, and easier to maintain.
Mastering CSS animations and JavaScript opens up a world of creative possibilities for web design. But amidst the hustle of coding, remember the importance of securing your future; check out this article on The Benefits of Having a Comprehensive Insurance Plan for Your Family for peace of mind. Then, get back to those slick animations – because a solid financial foundation fuels creative freedom!
The @keyframes Rule
The `@keyframes` rule is the engine of CSS animations. It defines the animation’s different stages, each marked by a percentage from 0% to 100%. You specify the styles for each percentage point, and CSS interpolates between them to create the animation. For instance, 0% represents the animation’s starting point, and 100% represents its ending point. Intermediate percentages define transitional states.
Consider this example: You want a box to fade in. At 0%, it’s completely transparent; at 100%, it’s fully opaque. `@keyframes` allows you to define these states, and CSS handles the smooth transition between them. This eliminates the need for complex JavaScript code to manage the opacity change over time.
Examples of Simple CSS Animations
Let’s illustrate with some practical examples. These are fundamental building blocks you can combine for more complex animations.
Fading
Imagine a button that subtly fades in when a user hovers over it. This creates a responsive, visually appealing interaction. The `@keyframes` rule would define the opacity at different stages of the hover, starting at 0% opacity and ending at 100%.
Scaling
Scaling is another common animation. Think of a loading icon that grows and shrinks rhythmically. This involves changing the `transform: scale()` property within the `@keyframes` rule, smoothly transitioning between different scale values over time. This creates a visually engaging effect.
Moving Elements
Moving elements is also possible, using the `transform: translate()` property. For example, you could create a simple animation where an element slides from left to right across the screen. This is achieved by modifying the `translate()` values within the `@keyframes` rule to gradually shift the element’s position.
CSS Animation Properties Comparison
Several properties fine-tune your animations. Understanding them is crucial for crafting polished effects.
Property | Description | Example | Notes |
---|---|---|---|
animation-duration |
Specifies the animation’s length. | animation-duration: 2s; |
Value is in seconds or milliseconds. |
animation-timing-function |
Defines the animation’s pacing (ease, linear, ease-in-out, etc.). | animation-timing-function: ease-in-out; |
Controls the speed curve of the animation. |
animation-iteration-count |
Specifies how many times the animation should play. | animation-iteration-count: infinite; |
Use infinite for continuous looping. |
animation-delay |
Specifies a delay before the animation starts. | animation-delay: 1s; |
Value is in seconds or milliseconds. |
Introduction to JavaScript Animations
Okay, so you’ve mastered the art of CSS animations, creating smooth transitions and captivating effects. But what if you need more control? What if you want animations that react to user interactions, change dynamically based on data, or achieve levels of complexity that CSS alone can’t handle? That’s where JavaScript steps in, boosting your animation game to the next level. Think of CSS animations as the foundation, and JavaScript as the architect who builds the truly impressive structures on top.
JavaScript offers unparalleled flexibility and power when it comes to animating elements. You can manipulate any CSS property, respond to events in real-time, and create intricate, data-driven animations that are impossible to achieve solely with CSS. This means your animations can become interactive, responsive, and far more engaging for your users.
Using `requestAnimationFrame` for Smooth Animations, How to Create Animations with CSS and JavaScript
`requestAnimationFrame` is your secret weapon for creating buttery-smooth animations in JavaScript. Unlike `setTimeout` or `setInterval`, which rely on fixed time intervals that can lead to jerky animations, `requestAnimationFrame` synchronizes the animation with the browser’s refresh rate. This ensures that your animations are rendered at the optimal time, resulting in a significantly smoother and more visually appealing experience. It essentially asks the browser, “Hey, when’s the next best time to redraw this?” and then the browser responds accordingly, ensuring the animation is tied to the screen’s refresh rate. This makes the animation more efficient and less likely to strain the user’s device. The function takes a callback function as an argument, which is executed before the next repaint. This callback function usually updates the animation’s state and requests the next frame.
Manipulating CSS Properties with JavaScript
JavaScript allows you to directly manipulate an element’s CSS properties using its `style` property or by leveraging methods like `classList.add()` and `classList.remove()`. For example, you can change an element’s position, size, color, opacity, and virtually any other CSS property dynamically within your JavaScript code. This provides a powerful way to control and modify your animations in real-time, based on user actions or other events.
Consider the example of changing the `left` property to animate an element horizontally. You could increment the value within a `requestAnimationFrame` loop to create a smooth movement. The flexibility extends to all aspects of CSS styling, allowing for intricate and complex animation sequences.
Animating an Element’s Position Using JavaScript: A Step-by-Step Guide
Let’s animate a simple square across the screen. We’ll use `requestAnimationFrame` for smooth animation and directly manipulate the element’s `left` and `top` properties.
First, select your element (let’s assume it has the ID “mySquare”). Then, initialize variables to track the element’s position and animation speed. Inside a `requestAnimationFrame` loop, update the `left` and `top` properties based on the speed and desired direction. The loop continues until a specific condition is met, such as reaching a target position.
Here’s a simplified representation of the process:
“`javascript
const square = document.getElementById(‘mySquare’);
let x = 0;
let y = 0;
let speed = 5;
function animate()
x += speed;
y += speed; // You can adjust this to control vertical movement.
square.style.left = x + ‘px’;
square.style.top = y + ‘px’;
if (x < 500 && y < 500) //Example ending condition. Adjust as needed. requestAnimationFrame(animate); animate(); ``` This code snippet shows the basic principle. You'd need to style your `#mySquare` element with `position: absolute;` to allow for absolute positioning and ensure the `left` and `top` properties have an effect. Remember that error handling and more sophisticated movement patterns (e.g., curves, bouncing) would require more advanced techniques. This example simply provides a basic framework for understanding the core concept.
Combining CSS and JavaScript Animations
Harnessing the power of both CSS and JavaScript for animations unlocks a world of creative possibilities. But like any dynamic duo, understanding their strengths and weaknesses is key to creating smooth, efficient, and visually stunning animations. This section dives into the art of combining these two animation powerhouses, exploring when to use each, and how their performance stacks up.
The synergy between CSS and JavaScript animations lies in their complementary capabilities. CSS excels at simple, declarative animations, while JavaScript offers unparalleled control and complexity for intricate, data-driven effects. By strategically combining them, developers can create sophisticated animations that are both performant and visually captivating.
CSS Animation Advantages and Disadvantages When Used With JavaScript
CSS animations shine when dealing with straightforward visual transformations. Their declarative nature makes them easy to implement and maintain, often requiring less code than their JavaScript counterparts. However, their limitations become apparent when handling complex interactions or dynamic data manipulation—areas where JavaScript excels. Using CSS for the foundational animation and JavaScript for intricate control provides a balanced approach. For instance, CSS might handle the core animation of an element, while JavaScript dynamically adjusts its properties based on user input or data updates.
Scenarios Favoring CSS Animations
CSS animations are ideally suited for simple, repeatable animations that don’t require significant runtime manipulation. Think subtle hover effects, loading indicators, or transitions between different states of a UI element. These animations can be easily defined in CSS, leading to cleaner code and potentially better performance compared to JavaScript-based alternatives. For example, a simple fade-in effect on an image when it loads is perfectly handled by CSS’s `transition` property. No JavaScript needed!
Scenarios Favoring JavaScript Animations
JavaScript animations come into their own when dealing with complex, dynamic, or data-driven scenarios. Imagine an animation where the movement of an element is dictated by real-time data from a sensor or a user’s interaction. Or consider a complex animation involving multiple elements interacting in a non-linear fashion. JavaScript provides the flexibility and control to manage these scenarios efficiently. A prime example would be a game where character movement is controlled by user input and physics calculations – CSS simply can’t handle that level of dynamic interaction.
Performance Comparison of CSS and JavaScript Animations
Generally, CSS animations tend to be more performant than their JavaScript counterparts, especially for simpler animations. The browser’s rendering engine is highly optimized for handling CSS animations, often leveraging hardware acceleration. JavaScript animations, on the other hand, require more processing power, as they involve manipulating the DOM (Document Object Model) directly. However, modern JavaScript engines and animation libraries have significantly improved performance, minimizing the gap. The performance difference is most noticeable in resource-constrained environments or when dealing with many simultaneous animations. In such cases, a strategic mix of CSS and JavaScript, prioritizing CSS where possible, is vital for maintaining a smooth user experience.
Advanced Animation Techniques
Level up your animation game! We’ve covered the basics, but now it’s time to dive into the more complex and powerful techniques that will transform your animations from simple to stunning. This section explores creating intricate animation sequences, mastering easing functions, handling user interactions, and orchestrating complex multi-element animations.
Creating intricate and engaging animations requires a deeper understanding of how to sequence actions and control the flow of movement. By combining CSS and JavaScript, you gain the power to create truly dynamic and responsive experiences.
CSS and JavaScript Animation Sequences
Animating elements sequentially involves carefully coordinating the timing and execution of different animation steps. This can be achieved using CSS animation properties like `animation-delay` and `animation-iteration-count` in conjunction with JavaScript event listeners. For example, you could animate an element to move across the screen, then change color, and finally disappear, all triggered in a specific order. JavaScript provides the precision to control the exact timing and conditions for each step. Consider a loading animation: a circle could expand, then a checkmark appears after a delay, finally fading out the circle. This sequence relies on precise timing controlled by both CSS and JavaScript to ensure a smooth, visually appealing animation.
Easing Functions
Easing functions control the speed and pacing of animations, adding a sense of realism and fluidity. Instead of a simple linear animation, easing functions allow for acceleration, deceleration, and other nuanced transitions. CSS provides built-in easing functions like `ease`, `ease-in`, `ease-out`, and `ease-in-out`, while JavaScript libraries like GSAP (GreenSock Animation Platform) offer a far wider range of custom easing functions, enabling incredibly fine-grained control over animation curves. Imagine a bouncing ball animation; without easing, it would appear unnatural. Easing functions create the realistic deceleration as the ball reaches its peak and the acceleration as it falls back down.
Handling Events and User Interaction
Interactive animations respond to user actions, creating dynamic and engaging experiences. JavaScript event listeners are crucial here. For example, you could trigger an animation when a user hovers over an element, clicks a button, or scrolls down the page. Consider a button that expands on hover, showcasing more information, or a progress bar that animates as a user uploads a file. This responsiveness is key to creating user interfaces that feel intuitive and rewarding.
Complex Multi-Element Animations
Orchestrating animations involving multiple elements interacting with each other unlocks a whole new level of visual complexity. This requires careful planning and coordination using JavaScript to manage the timing and dependencies between different animation sequences. Consider a game where multiple characters move across the screen, colliding and interacting with each other. This requires intricate JavaScript logic to handle collisions, changes in movement based on interactions, and the smooth animation of each element in response to these interactions. Another example is a responsive navigation menu that animates its sub-menus based on user selection. Each element’s animation is carefully choreographed to provide a seamless and intuitive user experience.
Practical Examples and Illustrations
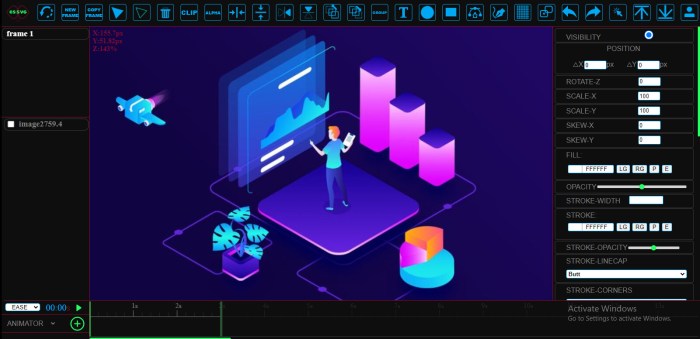
Source: discourse-cdn.com
Let’s dive into some concrete examples to solidify your understanding of CSS and JavaScript animations. We’ll build three common animation types: a bouncing ball, a loading spinner, and a progress bar. These examples will demonstrate how to combine CSS and JavaScript for powerful and dynamic effects.
Bouncing Ball Animation
This animation simulates a ball bouncing repeatedly within a defined area. We’ll use CSS for styling and basic animation properties, and JavaScript to control the ball’s movement and bouncing behavior. The key is to manipulate the ball’s position using JavaScript’s `setInterval` function and CSS’s `transform: translate()` property.
Code Section | Description | Code Snippet |
---|---|---|
HTML | Sets up a simple div to represent the ball. | <div id="ball"></div> |
CSS | Styles the ball and sets up initial position. We use `border-radius` for a circular shape. | #ball width: 50px; height: 50px; background-color: red; border-radius: 50%; position: absolute; left: 50px; top: 50px; |
JavaScript | This code uses `setInterval` to update the ball’s position repeatedly, simulating the bounce. The `xSpeed` and `ySpeed` variables control the direction and speed. We use `getBoundingClientRect()` to get the ball’s position and dimensions relative to the viewport. |
|
Loading Spinner Animation
A loading spinner provides visual feedback while an operation is in progress. This example uses CSS animations for a smooth, rotating effect. We can achieve this by using the `@keyframes` rule in CSS to define the animation and applying it to a suitable element.
The spinner’s visual appearance could be a circle, several dots, or even a more complex shape. The key is to rotate the element continuously using the `rotate` transform within the `@keyframes` definition.
Progress Bar Animation
A progress bar visually represents the completion status of a task. This example uses CSS to style the bar and JavaScript to dynamically update its length based on the progress percentage.
The visual representation is a rectangular bar that fills up from left to right. We can use a `div` element with a background color to represent the progress bar itself and a nested `div` with a different background color to represent the filled portion. JavaScript updates the width of the inner `div` based on the progress percentage.
Various Animation Effects Achievable with CSS and JavaScript
The combination of CSS and JavaScript offers a vast array of animation possibilities. Here are some examples:
These examples demonstrate just a fraction of what’s possible. The power lies in combining the ease of CSS animations with the dynamic control offered by JavaScript.
- Fades: Smoothly appearing and disappearing elements using `opacity`.
- Transitions: Changing element properties (e.g., size, color) smoothly over time.
- Transforms: Rotating, scaling, and moving elements with CSS transforms and JavaScript manipulation.
- Keyframes Animations: Complex animations defined using CSS `@keyframes`.
- Parallax Scrolling: Creating depth and movement effects as the user scrolls.
- Custom Cursors: Changing the cursor appearance based on user interaction.
- Interactive Animations: Animations triggered by user events (clicks, mouseovers).
Troubleshooting and Optimization
So you’ve dived headfirst into the world of CSS and JavaScript animations, crafting mesmerizing visual effects. But sometimes, even the most meticulously planned animation can hit a snag. This section tackles the common pitfalls and provides solutions to ensure your animations run smoothly, efficiently, and across all browsers and devices. We’ll cover practical strategies for debugging and optimization, transforming your animation frustrations into triumphs.
Optimizing animations isn’t just about making things look pretty; it’s about ensuring a smooth user experience, especially on less powerful devices. A poorly optimized animation can lead to choppy playback, dropped frames, and even browser crashes. This is where understanding common issues and employing optimization techniques becomes crucial.
Common Animation Problems
Several issues frequently crop up during animation development. These range from browser-specific rendering quirks to performance bottlenecks caused by inefficient code. Understanding these problems is the first step towards solving them.
- Janky Animations: Animations appearing jerky or stuttering, often due to insufficient browser resources or inefficient animation code.
- Browser Compatibility Issues: Animations rendering differently or not at all across various browsers (Chrome, Firefox, Safari, Edge).
- Performance Bottlenecks: Animations causing slowdowns or freezes, particularly on low-powered devices or when multiple animations run concurrently.
- Unexpected Behavior: Animations behaving unexpectedly due to unforeseen interactions between CSS and JavaScript, or conflicts with other website elements.
Performance Optimization Techniques
Optimizing animation performance involves a multifaceted approach. It’s not a one-size-fits-all solution, but rather a combination of techniques tailored to the specific animation and target environment.
- Hardware Acceleration: Utilize the GPU for rendering animations wherever possible. This significantly reduces the load on the CPU, resulting in smoother performance. This can often be achieved by using CSS transforms (
translate
,scale
,rotate
) instead of manipulating properties liketop
,left
, orwidth
directly. - Reduce Animation Complexity: Simplify animations by reducing the number of elements involved, minimizing the number of properties being animated, and using simpler animation curves. Avoid complex calculations within animation loops.
- Use Efficient Animation Libraries: Consider using established animation libraries like GSAP (GreenSock Animation Platform) or Anime.js, which are optimized for performance and cross-browser compatibility. These libraries often handle many of the low-level optimization details for you.
- RequestAnimationFrame: Use
requestAnimationFrame
for smoother animations. This function synchronizes animation updates with the browser’s repaint cycle, preventing unnecessary redraws and improving efficiency. For example, instead of usingsetInterval
, userequestAnimationFrame
to update your animation loop.
Debugging Animation Issues
Debugging animations can be tricky, but utilizing browser developer tools is crucial. These tools provide insights into performance bottlenecks, rendering issues, and more.
- Browser Developer Tools: Use the performance profiler to identify areas of slowdowns. The timeline tool can help pinpoint specific frames where issues occur. The inspector allows examination of CSS and JavaScript code directly within the context of the animation.
- Console Logging: Strategically placed
console.log
statements can help track the values of variables and the flow of execution within animation code. This helps pinpoint errors or unexpected behavior. - Step-Through Debugging: Use the debugger in your browser’s developer tools to step through the animation code line by line, examining variable values and identifying the exact point where an error occurs.
Cross-Browser Compatibility
Ensuring your animations work consistently across different browsers requires careful planning and testing. Certain CSS properties and JavaScript APIs might behave differently across browsers, necessitating workarounds or alternative approaches.
- Prefixes: While less necessary now than in the past, be mindful of CSS vendor prefixes (e.g.,
-webkit-
,-moz-
) for older browsers. Modern CSS is generally well-supported, but testing is still crucial. - Feature Detection: Use feature detection techniques to check for browser support of specific CSS properties or JavaScript APIs before using them in your animations. This prevents errors in browsers lacking the required functionality.
- Polyfills: For features not supported by all browsers, consider using polyfills – code snippets that add missing functionality. This ensures a consistent experience across a wider range of browsers.
- Thorough Testing: Test your animations on different browsers and devices (desktops, tablets, mobiles) to ensure consistent behavior. Consider using browser testing services or virtual machines for efficient testing across various browser versions and operating systems.
Last Word: How To Create Animations With CSS And JavaScript
So, you’ve learned the secrets to crafting stunning animations with CSS and JavaScript. From simple transitions to complex, interactive effects, the possibilities are endless. Remember to leverage the strengths of both languages, optimize for performance, and most importantly, have fun experimenting! Go forth and animate!