An Introduction to REST APIs and How They Work: Dive into the world of APIs, those unsung heroes connecting apps and services. We’ll unpack what REST APIs actually *are*, exploring their core components and how they power everything from your favorite social media feed to online banking. Get ready to decode the magic behind seamless online experiences!
This guide breaks down the complexities of REST APIs into digestible chunks. We’ll cover the essential HTTP methods (GET, POST, PUT, DELETE), the importance of status codes, and the structure of requests and responses. You’ll even learn how to design your own simple API, understand authentication, and master error handling. By the end, you’ll be speaking fluent API – or at least, understanding enough to impress your tech-savvy friends.
What are REST APIs?
Imagine you’re ordering food online. You don’t go into the restaurant’s kitchen; you use an app to place your order. That app acts as a messenger, sending your request to the restaurant’s system and bringing back the confirmation and, eventually, your delicious meal. REST APIs are similar – they’re messengers that allow different software systems to talk to each other without needing to know the intricate details of how each system works internally. They’re the behind-the-scenes communication channels that power much of the internet as we know it.
REST APIs, or Representational State Transfer Application Programming Interfaces, are a standardized way for software systems to communicate and exchange data. They use simple, standard HTTP requests (like GET, POST, PUT, DELETE) to interact with data. This makes them incredibly versatile and easy to implement compared to more complex communication protocols.
Core Architectural Constraints of REST
REST APIs adhere to a set of architectural constraints that ensure consistency and interoperability. These constraints aren’t strict rules, but guidelines that, when followed, lead to a more robust and scalable API. These constraints include things like:
- Client-Server Architecture: The client (like your food ordering app) and the server (the restaurant’s system) are independent entities. This allows for flexibility and scalability.
- Statelessness: Each request from the client to the server contains all the information needed to process the request. The server doesn’t store any context about past requests. This simplifies the server’s design and improves its resilience.
- Cacheability: Responses from the server can be cached to improve performance. This reduces the load on the server and speeds up responses for subsequent requests.
- Uniform Interface: REST APIs use standard HTTP methods (GET, POST, PUT, DELETE) to perform different actions on resources. This simplifies the interaction between the client and the server.
- Layered System: The client doesn’t need to know whether it’s directly interacting with the server or an intermediary. This allows for the introduction of load balancers and other infrastructure components without affecting the client.
- Code on Demand (Optional): The server can extend client functionality by transferring executable code to the client. This is less common but can be useful in certain situations.
REST API Use Cases Across Industries
REST APIs are ubiquitous, powering countless applications across various sectors.
- E-commerce: APIs enable integration between shopping carts, payment gateways, and inventory management systems. For example, an e-commerce site might use an API to check inventory levels with a warehouse system before confirming an order.
- Social Media: Platforms like Twitter and Facebook use APIs to allow third-party developers to access and interact with their data. This enables the creation of countless apps and integrations.
- Financial Services: Banks and other financial institutions use APIs to provide access to account information, transaction history, and other financial data. This facilitates the development of personal finance apps and other financial tools.
- Healthcare: APIs are increasingly used to share patient data between healthcare providers, improving coordination of care. Imagine a system where a doctor can access a patient’s medical history from another hospital via an API.
- Mapping and Location Services: Services like Google Maps and Mapbox use APIs to provide location data and mapping functionalities to other applications. This allows developers to integrate map features into their own apps.
Key Components of a REST API
REST APIs, at their core, are all about organized access to data. Think of them as polite waiters in a fancy restaurant – they understand your requests (using specific commands), fetch the right dish (data), and serve it back neatly (in a structured format). To understand how this works, we need to dive into the key components that make a REST API tick.
Resources and URIs
Resources represent the data you want to access – think customer information, product details, or even weather updates. Each resource is uniquely identified by a Uniform Resource Identifier (URI), which is essentially its web address. For example, `/customers/123` might represent the details of customer number 123. The URI acts like the restaurant’s menu, clearly pointing to the specific dish (resource) you’re ordering. The structure of URIs helps organize the API, making it intuitive and easy to navigate. A well-designed URI structure improves API readability and maintainability.
HTTP Methods
REST APIs use standard HTTP methods to communicate. These methods define the type of operation you want to perform on a resource. Think of them as the waiter’s actions – getting a dish, placing an order, updating a dish, or removing it from the menu.
- GET: Retrieves a resource. It’s like asking the waiter, “Can I have the menu?” or “What’s the price of this dish?”. It’s a read-only operation and should not change the server’s state.
- POST: Creates a new resource. Imagine ordering a custom dish – you’re providing the waiter with the ingredients and instructions. This method is used to submit data to the server, often creating a new entry in a database.
- PUT: Updates an existing resource. This is like asking the waiter to modify a dish – perhaps changing the ingredients or the name. It replaces the entire resource with the data provided.
- DELETE: Deletes a resource. This is like telling the waiter to remove a dish from the menu. It removes the specified resource from the server.
HTTP Status Codes
Every time you interact with a REST API, you receive a response. This response includes an HTTP status code, which indicates the outcome of your request. These codes are crucial for debugging and understanding if your request was successful or not. Think of them as the waiter’s feedback – a thumbs up (success) or a slight frown (failure).
Code | Description | Success/Failure | Example |
---|---|---|---|
200 OK | The request was successful. | Success | A successful GET request retrieving customer data. |
201 Created | The request was successful, and a new resource was created. | Success | A successful POST request creating a new customer account. |
400 Bad Request | The server could not understand the request due to malformed syntax. | Failure | Sending a request with missing required parameters. |
404 Not Found | The requested resource could not be found. | Failure | Trying to access a customer with an ID that doesn’t exist. |
500 Internal Server Error | The server encountered an unexpected condition that prevented it from fulfilling the request. | Failure | A server-side error preventing the processing of the request. |
Understanding API Requests and Responses
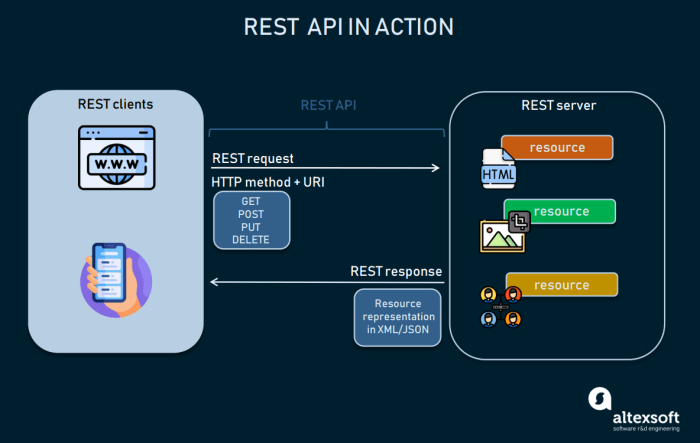
Source: altexsoft.com
Think of REST APIs as waiters in a fancy restaurant. You (the client) send a request (your order) to the waiter (the API), and they fetch the information (your meal) from the kitchen (the server) and bring it back to you. This “order” and “meal” are structured in specific ways, and understanding this structure is key to working with APIs.
API requests and responses follow a pretty standard format, making communication between different systems smooth and predictable. Let’s break down the key parts.
API Request Structure
An API request is essentially a message sent to a server to retrieve or manipulate data. It’s composed of several parts: the method (like GET, POST, PUT, DELETE), the URL (the address of the resource), headers (additional information about the request), and sometimes a body (the data you’re sending).
For example, imagine you’re building an app that needs to fetch a user’s profile from a social media platform. Your request might look like this (simplified):
Method: GET
URL: `https://api.example.com/users/123`
Headers: `Authorization: Bearer your_access_token` (This tells the server you’re authorized)
Body: (Usually empty for a GET request, as you’re just retrieving data)
Data Formats in API Communication
APIs use standardized formats to exchange data, the most common being JSON (JavaScript Object Notation) and XML (Extensible Markup Language). JSON is generally preferred for its simplicity and readability.
JSON Example
A JSON request body might look like this if you were sending data to create a new user:
“`json
“username”: “johndoe”,
“email”: “[email protected]”,
“password”: “securepassword”
“`
This is a simple object with key-value pairs. The keys are strings, and the values can be strings, numbers, booleans, or even nested objects.
XML Example
The same data in XML would look more verbose:
“`xml
“`
XML uses tags to structure the data, making it more complex to parse but offering more flexibility in terms of data representation. JSON’s simplicity makes it the champion in most API interactions.
API Response Structure
After sending a request, the server responds with a message containing the requested data (or an error message). This response includes a status code indicating the outcome of the request and a data payload.
Status Codes
Status codes are three-digit numbers that communicate the result of the request. For example:
* `200 OK`: The request was successful.
* `404 Not Found`: The requested resource wasn’t found.
* `500 Internal Server Error`: Something went wrong on the server side.
JSON Response Example
Let’s say you successfully fetched a user’s profile. A JSON response might look like this:
“`json
“status”: “success”,
“data”:
“id”: 123,
“username”: “johndoe”,
“email”: “[email protected]”,
“profile_picture”: “url_to_picture.jpg”
“`
This response includes a “status” field indicating success and a “data” field containing the user’s profile information. This structured format makes it easy for your app to extract the necessary information. The structure is consistent and easily parsed by various programming languages.
Designing RESTful APIs
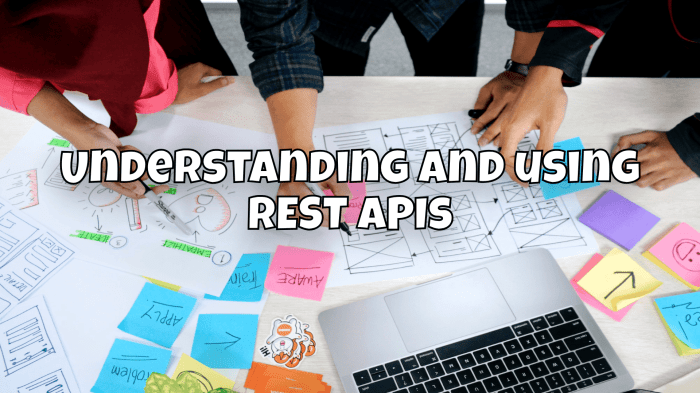
Source: disane.dev
Crafting a stellar REST API isn’t just about making it work; it’s about making it a joy to use. A well-designed API is intuitive, efficient, and scalable, leading to happy developers and robust applications. This section dives into the best practices for building APIs that are both user-friendly and performant.
Designing a RESTful API involves careful consideration of several key aspects. The goal is to create a consistent and predictable interface that’s easy to understand and integrate with. This includes thoughtful resource naming, a clear URL structure, and the appropriate use of HTTP methods. Let’s explore these crucial elements in detail.
Resource Naming and URL Structure, An Introduction to REST APIs and How They Work
Proper resource naming and URL structure are fundamental to a well-designed REST API. Think of resources as the core entities within your application, such as users, products, or blog posts. URLs should clearly reflect the location and manipulation of these resources. Avoid using verbs in your URLs; instead, focus on nouns that represent the resources themselves. For example, `/users` is better than `/getUsers`. Consistent naming conventions, such as using plural nouns for collections of resources, enhance readability and predictability. Deeply nested URLs should be avoided as they can become cumbersome and difficult to manage. A well-structured URL is concise and self-, immediately conveying the resource being accessed. Consider using hyphens to improve readability in longer URLs.
Designing a REST API for a To-Do List Application
Let’s illustrate these principles by designing a simple REST API for a to-do list application. This example will highlight the key components – resources, endpoints, and HTTP methods – demonstrating how a clean and effective API is structured.
The following bullet points Artikel the design of a REST API for a to-do list application, showcasing best practices for resource naming and URL structure.
Understanding REST APIs is key to modern app development; they’re the behind-the-scenes magic making everything connect. But just like choosing the right API, you need to be equally savvy about your personal insurance, ensuring it’s perfectly tailored to your life – check out this guide on How to Make Sure Your Insurance Policy Meets Your Specific Needs to learn more.
Back to APIs: mastering their intricacies unlocks a world of seamless digital experiences.
- Resource: Tasks. This represents the core entity of the application.
- Endpoint:
/tasks
. This endpoint represents the collection of all tasks. - HTTP Methods:
GET /tasks
: Retrieves a list of all tasks.POST /tasks
: Creates a new task. The request body would contain the task details (e.g., title, description, due date).
- Endpoint:
/tasks/id
. This endpoint represents a specific task identified by its unique ID. - HTTP Methods:
GET /tasks/id
: Retrieves a specific task.PUT /tasks/id
: Updates a specific task. The request body would contain the updated task details.DELETE /tasks/id
: Deletes a specific task.
This simple design showcases the effective use of standard HTTP methods and clear, concise URLs, resulting in an intuitive and efficient API. Each endpoint clearly indicates the resource being accessed and the action being performed, adhering to RESTful principles.
Authentication and Authorization in REST APIs
So you’ve built your awesome REST API, but what’s stopping everyone and their dog from accessing it? Security, my friend! That’s where authentication and authorization come in. Think of it like this: authentication is proving who you are, while authorization is proving what you’re allowed to do. Without these, your API is a wide-open door, inviting unwanted guests to your digital party.
Authentication mechanisms are the gatekeepers, verifying the identity of the client trying to access your API. Authorization, on the other hand, acts as the bouncer, deciding which parts of the club (your API) each guest can access. Let’s dive into the specifics.
API Keys
API keys are like simple passwords for your API. They’re unique strings of characters that clients use to identify themselves. It’s a straightforward approach, often used for simpler APIs or internal applications. A client includes the API key in the request header, and the server verifies it against a list of valid keys. While easy to implement, API keys have limitations. Revoking a compromised key can be tricky, requiring updating all client applications. They also offer limited control over what actions a client can perform.
OAuth 2.0
OAuth 2.0 is a much more sophisticated authentication protocol, particularly useful for applications needing access to user data. Instead of sharing passwords directly, it uses access tokens. Imagine it as a temporary ID card that grants specific permissions. A client requests an access token from an authorization server, typically after the user authenticates with their existing credentials (like a Google or Facebook login). This token is then used to make API requests. OAuth 2.0 offers granular control over permissions, making it ideal for situations where you need fine-grained access control, like allowing an app to only access a user’s profile picture, not their entire data. The strength lies in its flexibility and the ability to manage access effectively. However, its complexity makes implementation more challenging.
Authorization Methods
Securing your API isn’t just about knowing *who* is accessing it; it’s about knowing *what* they can do. Authorization mechanisms determine the level of access granted to authenticated clients. This often involves checking roles, permissions, or access control lists (ACLs).
Role-Based Access Control (RBAC)
RBAC is a popular approach. Users are assigned to roles (e.g., “admin,” “editor,” “viewer”), and each role is associated with specific permissions. For example, an “admin” might have full access, while a “viewer” can only read data. This simplifies authorization management, but can become complex with many roles and permissions.
Attribute-Based Access Control (ABAC)
ABAC takes a more granular approach, considering various attributes of the user, the resource, and the environment. For instance, access could be granted based on the user’s department, the resource’s sensitivity level, and the time of day. ABAC offers great flexibility, but its complexity requires a sophisticated implementation.
Comparison of Authorization Methods
Method | Strengths | Weaknesses |
---|---|---|
RBAC | Simple to implement and understand; easy to manage roles. | Can become complex with many roles and permissions; less granular than ABAC. |
ABAC | Highly granular control; adaptable to diverse scenarios. | Complex to implement and manage; requires a sophisticated infrastructure. |
Error Handling and Best Practices: An Introduction To REST APIs And How They Work
Building robust and user-friendly REST APIs requires careful consideration of error handling. A well-designed error handling strategy not only improves the user experience but also aids in debugging and maintaining the API’s health. Ignoring errors can lead to frustrating experiences for developers integrating your API and ultimately impact the success of your application.
Effective error handling involves providing informative error messages, consistent response formats, and implementing proper logging and monitoring. This ensures that errors are easily identified, understood, and addressed, leading to a more stable and reliable API.
Informative Error Responses
Providing clear and concise error messages is crucial for effective debugging. Instead of generic error codes, REST APIs should return detailed error responses that include a descriptive error message, an appropriate HTTP status code, and potentially a unique error code for easier tracking. For instance, a 404 (Not Found) error should specify exactly what resource was not found, and a 400 (Bad Request) error should detail the specific input validation issue. Consider using a standardized error response format like JSON, with fields like “status”, “message”, “code”, and “details”. An example of a well-structured error response might look like this:
"status": "error",
"message": "Invalid input: Username must be at least 6 characters long.",
"code": "VALIDATION_ERROR",
"details": ["username field failed validation"]
HTTP Status Codes
Proper use of HTTP status codes is paramount. Each status code conveys a specific meaning, enabling clients to understand the nature of the response without needing to parse the entire response body. Using the correct status code is not just good practice; it’s crucial for interoperability and client-side error handling. For example, a 401 (Unauthorized) status code clearly indicates an authentication failure, while a 500 (Internal Server Error) signals a problem on the server side.
API Logging and Monitoring
Comprehensive logging is essential for tracking API performance, identifying errors, and debugging issues. Logs should include timestamps, request details (URLs, methods, headers, parameters), response details (status codes, response times), and any error messages. Effective monitoring involves using tools to track key metrics like request latency, error rates, and throughput. This allows for proactive identification of performance bottlenecks and potential problems before they impact users. Popular monitoring tools such as Datadog, New Relic, or custom solutions can provide valuable insights into API health. Consider setting up alerts for critical errors or performance degradation.
Rate Limiting and Throttling
To prevent abuse and ensure fair usage, implement rate limiting and throttling mechanisms. Rate limiting restricts the number of requests a client can make within a specific time window. Throttling temporarily reduces the rate of requests when the API is under heavy load, preventing server overload. When rate limits are exceeded, return appropriate HTTP status codes (e.g., 429 Too Many Requests) with clear instructions on how to handle the limitation.
Input Validation
Always validate user inputs to prevent common vulnerabilities like SQL injection or cross-site scripting (XSS). Thoroughly check all data received from clients, ensuring it conforms to expected data types and formats. Reject invalid input with appropriate error messages and status codes. Consider using input validation libraries to simplify the process and ensure comprehensive validation.
Tools and Technologies for Working with REST APIs
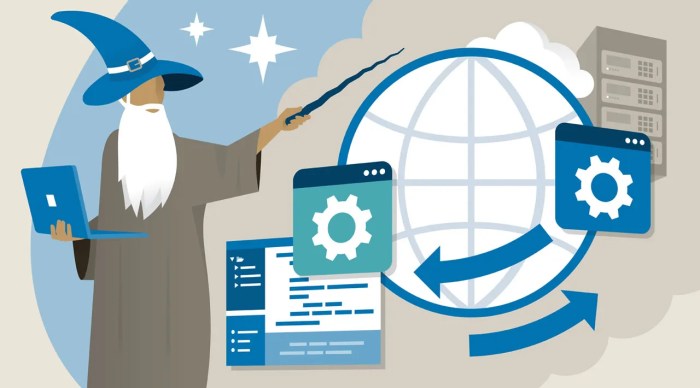
Source: morioh.com
Building and interacting with REST APIs isn’t just about understanding the concepts; you need the right tools to make it all happen smoothly. This section dives into some popular choices for developing, testing, and documenting your APIs, making your API journey a whole lot easier.
From crafting robust APIs to meticulously testing their functionality, the right tools can significantly impact your workflow’s efficiency and the overall quality of your API. Let’s explore some essential players in the REST API ecosystem.
Popular API Development and Testing Tools
Several tools are indispensable for developers working with REST APIs. These range from comprehensive IDE integrations to specialized testing applications. Choosing the right tool often depends on individual preferences and project requirements.
- Postman: A widely-used API development environment that allows you to send requests, view responses, save requests for later use, and even automate testing. Its intuitive interface makes it perfect for both beginners and experienced developers. You can organize requests into collections, add assertions for automated testing, and collaborate with teammates effectively.
- Swagger/OpenAPI: More than just a tool, Swagger (now often referred to as OpenAPI) is a specification and a set of tools for designing, building, documenting, and consuming RESTful web services. It provides a standardized way to describe your API, allowing for automatic generation of documentation and client SDKs. This makes it incredibly easy for others to integrate with your API.
- Insomnia: A powerful and versatile alternative to Postman, Insomnia offers a similar range of features, including request building, response inspection, and environment management. It’s known for its clean interface and robust features for collaboration.
- curl: A command-line tool available on most operating systems. While less visually appealing than graphical tools, `curl` is incredibly versatile and powerful for making API requests directly from the terminal. It’s invaluable for scripting and automation.
The Importance of API Documentation
Thorough API documentation is crucial for successful API adoption. Well-written documentation acts as a bridge between the API and its users, enabling them to understand how to interact with the API effectively. Clear documentation reduces integration time and minimizes errors. It’s a critical part of API design and is often overlooked.
Example API Documentation Snippet
“`markdown
# GET /users/idRetrieves a single user by their ID.
Parameters:
* `id` (integer, required): The ID of the user to retrieve.
Responses:
* 200 OK: Returns the user object.
“`json“id”: 1,
“name”: “John Doe”,
“email”: “[email protected]”“`
* 404 Not Found: The user with the given ID was not found.
“`
Using Postman to Make an API Request
Let’s illustrate a simple API request using Postman. Imagine an API endpoint that retrieves a list of products. We’ll use a hypothetical endpoint for demonstration purposes.
1. Setting up the Request: In Postman, you’d create a new request. Select the GET method and enter the API endpoint URL (e.g., `https://api.example.com/products`).
2. Sending the Request: Click the “Send” button. Postman will send the request to the server.
3. Interpreting the Response: Postman displays the response in various tabs (Headers, Body, etc.). The “Body” tab typically shows the response data in JSON or XML format. For example, you might see a JSON array of product objects, each containing details like product ID, name, and price. The response status code (e.g., 200 OK) indicates whether the request was successful.
For instance, a successful response might look like this:
“`json
[
“id”: 1, “name”: “Product A”, “price”: 19.99,
“id”: 2, “name”: “Product B”, “price”: 29.99
]
“`
Closure
So, there you have it – a whirlwind tour of REST APIs! From understanding the fundamentals to designing your own, we’ve covered the essentials. While the world of APIs is vast and constantly evolving, this introduction provides a solid foundation for further exploration. Now go forth and build amazing things!