Getting Started with Android App Development: Dive headfirst into the exciting world of mobile app creation! This isn’t your grandpappy’s coding; we’re talking sleek interfaces, intuitive designs, and the power to bring your app ideas to life. From setting up your Android Studio to mastering UI design and conquering the Google Play Store, we’ll navigate the entire journey, demystifying each step along the way. Prepare for a coding adventure that’s as rewarding as it is challenging.
We’ll cover everything from choosing the right development environment and understanding core Android concepts to mastering UI design, handling data, and deploying your app to the world. Think of this as your ultimate cheat sheet for building your first killer Android app—no prior experience necessary (though a healthy dose of curiosity definitely helps!). We’ll break down complex topics into manageable chunks, providing practical examples and clear explanations to ensure you’re confident every step of the way.
Choosing Your Development Environment
So, you’re ready to dive into the world of Android app development? Awesome! But before you start coding up a storm, you need to pick the right tools for the job. Think of it like choosing the perfect paintbrush – the wrong one can make even the most talented artist struggle. Your development environment is your digital canvas, so choosing wisely is key.
Android Studio is the official IDE (Integrated Development Environment) from Google, and it’s generally the recommended choice for most developers. While other IDEs exist, Android Studio offers a streamlined workflow specifically designed for Android development, including features like intelligent code completion, built-in debugging tools, and seamless integration with the Android SDK. Using a different IDE might mean more manual configuration and less efficient development.
Android Studio Setup
Setting up Android Studio involves a few steps, but don’t worry, it’s not rocket science. First, download the latest stable version of Android Studio from the official Google website. The installer will guide you through the process, but you’ll need to choose a few options. You’ll be prompted to select components like the Android SDK (Software Development Kit), which contains all the necessary tools and libraries to build Android apps. Make sure you select everything you need; you can always add more later if necessary. The SDK includes things like the Android emulator (more on that later), different Android versions (APIs), and build tools. After installation, Android Studio will likely prompt you to download necessary SDK components. This is a crucial step that ensures you have the required tools for building and running your apps.
Creating a New Android Project
Once Android Studio is installed and the SDK is configured, creating your first project is surprisingly straightforward. Upon launching, you’ll be greeted with the “Welcome to Android Studio” screen. Click on “New Project.” You’ll then be guided through a series of steps: Choose the type of project (e.g., Empty Activity, Basic Activity, etc.), give your app a name, select a language (Kotlin or Java are common choices), and specify a minimum SDK version (this determines which Android versions your app will support). Android Studio will then generate the basic project structure for you, including essential files like the `MainActivity.java` (or `MainActivity.kt` if you chose Kotlin) and the `activity_main.xml` layout file.
Android Emulators: A Comparison
Testing your app on a physical device is ideal, but emulators provide a convenient alternative, especially during development. The Android emulator bundled with Android Studio is a robust option, allowing you to simulate various Android devices and versions. However, it can be resource-intensive and sometimes slow. Other emulators, such as Genymotion or Xamarin Android Emulator, offer potentially faster performance and additional features, but may require separate installation and configuration. The choice often comes down to balancing performance needs with the convenience of integration with Android Studio. For beginners, the built-in emulator is a great starting point. As you become more experienced and your projects become more complex, exploring alternative emulators might be beneficial.
Understanding Core Android Concepts
So, you’ve chosen your development environment – congrats! Now let’s dive into the nitty-gritty of Android development. Understanding the core concepts is crucial for building even the simplest app, and mastering them will lay the foundation for more complex projects down the line. Think of it like learning your ABCs before writing a novel. This section will cover the essential building blocks: the Activity lifecycle, Intents, and XML layouts.
Activity Lifecycle and its Various States
The Activity is the fundamental building block of an Android app’s user interface. Think of it as a single screen in your app. The Activity lifecycle manages the creation, pausing, resuming, and destruction of these screens. Understanding this lifecycle is essential for managing resources efficiently and preventing crashes. An activity goes through several states, including:
- onCreate(): This is where the activity is first created. It’s your chance to initialize essential components, set up the layout, and perform any one-time setup tasks.
- onStart(): The activity becomes visible to the user.
- onResume(): The activity is in the foreground and interacting with the user. This is where you might start animations or begin listening for sensor data.
- onPause(): The activity is losing focus, perhaps because another activity is starting. Use this to save data, stop animations, and release resources that aren’t critical.
- onStop(): The activity is no longer visible to the user. This is a good time to release more resources, such as network connections.
- onDestroy(): The activity is being destroyed. Perform any final cleanup tasks here, such as releasing remaining resources.
Mastering the activity lifecycle ensures your app handles transitions smoothly and avoids memory leaks. For instance, imagine a game: `onPause()` would pause the game, while `onResume()` would resume it.
The Role of Intents in Android App Navigation
Intents are the messengers of the Android world. They’re how different components of your app, or even different apps, communicate and trigger actions. Think of them as little envelopes carrying instructions. Intents are used for starting activities, broadcasting events, and delivering data between components.
A simple example: Imagine a button that opens a map to show a specific location. An intent would be used to launch the map application and pass the location coordinates as data. There are two main types of intents: explicit and implicit. Explicit intents specify the exact component to be started, while implicit intents describe the action to be performed, allowing the system to choose the appropriate component (like choosing a map app from several installed ones).
Designing a Simple User Interface (UI) using XML Layouts
Android uses XML to define the structure and layout of your app’s user interface. This separation of concerns – separating UI design from app logic – makes your code cleaner and easier to maintain. XML layouts use a hierarchy of views, with each view representing a UI element. Best practices include using meaningful names for your views, organizing your layout logically, and leveraging Android’s layout managers (like LinearLayout, RelativeLayout, and ConstraintLayout) to position elements efficiently.
For instance, a simple login screen might have a `LinearLayout` containing two `EditText` fields for username and password, and a `Button` for login. The XML would define the placement and attributes of these elements.
Examples of Different UI Components and Their Usage
Android provides a rich set of UI components to build visually appealing and interactive apps. Here are a few common examples:
- TextView: Displays text. You can customize its font, size, color, and more.
- EditText: Allows users to input text. It’s commonly used for forms and search bars.
- Button: Triggers an action when clicked. It’s the workhorse of user interaction.
- ImageView: Displays images. You can load images from resources or from the network.
- CheckBox: Allows users to select one or more options.
- RadioButton: Allows users to select only one option from a group.
These components are the building blocks for creating complex and engaging user interfaces. For example, a to-do list app would use `TextViews` to display tasks, `CheckBoxes` to mark them as complete, and potentially an `EditText` to add new tasks.
Working with Layouts and UI Design
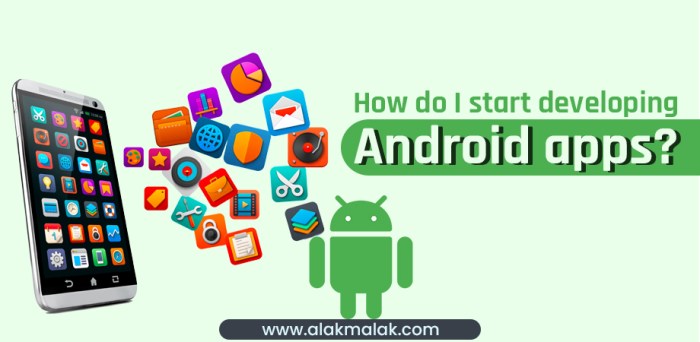
Source: alakmalak.com
Building a killer Android app isn’t just about coding; it’s about crafting a user experience that’s both beautiful and functional. This means mastering the art of layouts and UI design, ensuring your app looks great on any device, from a tiny phone to a massive tablet. We’ll explore the tools and techniques that’ll transform your app from a functional mess into a polished masterpiece.
Android offers a variety of layout managers to help you arrange UI elements effectively. Choosing the right layout is crucial for creating a responsive and visually appealing interface. We’ll delve into the most popular options, highlighting their strengths and weaknesses to help you make informed decisions.
ConstraintLayout: Building Responsive Layouts
ConstraintLayout is the go-to layout manager for modern Android development. Its power lies in its ability to create complex layouts with minimal nesting, leading to improved performance and easier maintenance. Constraints define the relationships between UI elements, allowing them to adapt dynamically to different screen sizes and orientations. For example, you can easily constrain a button to be centered horizontally and vertically within its parent container, ensuring it always looks good, regardless of screen dimensions. Imagine a button that always stays perfectly centered, no matter how the user rotates their phone – that’s the magic of ConstraintLayout. It uses a visual editor within Android Studio, making it incredibly intuitive to create complex relationships between widgets. You define constraints by dragging and dropping lines between UI elements, specifying relationships like “left-of,” “right-of,” “above,” “below,” and more.
LinearLayout and RelativeLayout: Classic Layout Managers
While ConstraintLayout is the modern favorite, understanding LinearLayout and RelativeLayout is still valuable. LinearLayout arranges elements in a single row (horizontal) or column (vertical), while RelativeLayout positions elements relative to each other or their parent. LinearLayout is straightforward for simple layouts but can become unwieldy with complex designs, leading to nested layouts and potential performance issues. RelativeLayout provides more flexibility but can be harder to manage for very intricate interfaces. Think of LinearLayout as a well-organized bookshelf – everything is neatly stacked, but adding new items can be tricky if the space is limited. RelativeLayout, on the other hand, is like a freeform wall display – more flexible but requires careful planning to avoid visual chaos.
Implementing Themes and Styles
Themes and styles provide a powerful way to control the look and feel of your app without modifying individual UI elements. A theme defines the overall appearance, such as colors, fonts, and shapes, while styles target specific UI components. Using themes and styles ensures consistency throughout your app and makes it easy to update the visual design in one central location. Imagine updating your app’s color scheme – with themes, you change it in one place, and the entire app updates instantly, saving you hours of tedious work. This also allows for easy creation of light and dark modes for enhanced user accessibility and preference.
Creating Custom Views and Extending Existing Components, Getting Started with Android App Development
For truly unique designs, you can create custom views by extending existing components or building them from scratch. This allows you to create highly specialized UI elements tailored to your app’s specific needs. Consider a custom progress bar with a unique animation or a custom button with a non-standard shape – custom views provide the freedom to implement these functionalities. Extending existing components offers a more efficient approach when you only need minor modifications, allowing you to leverage the functionality of existing widgets while customizing their appearance. This approach minimizes development time and ensures you maintain a consistent visual style within your app.
Data Handling and Storage
Building an Android app often involves managing user data, preferences, and other information. Efficient data handling is crucial for a smooth user experience and app performance. This section explores various methods for storing data within your Android application, highlighting their strengths and weaknesses. Choosing the right approach depends heavily on the type and volume of data you need to store, as well as the level of persistence required.
Shared Preferences
Shared Preferences are ideal for storing simple key-value pairs, such as user settings or preferences. They are easy to use and offer quick access to small amounts of data. Data is stored in an XML file within the app’s internal storage. This method is best suited for settings that don’t require complex data structures or large amounts of information. For instance, you might store a user’s preferred theme, language, or notification settings using Shared Preferences. Retrieving and saving data is straightforward using the `SharedPreferences` API.
Internal Storage
Internal storage provides a way to store larger amounts of data directly within the app’s private directory. This approach is suitable for files that should not be accessible by other apps. Access is restricted to your app only. Data stored here is automatically deleted when the app is uninstalled. You can use this for storing files, images, or other data that doesn’t need a structured database approach. The following code snippet demonstrates reading and writing a simple text file to internal storage:
“`java
// Writing to internal storage
try (FileOutputStream fos = openFileOutput(“my_file.txt”, Context.MODE_PRIVATE))
String data = “This is some sample data.”;
fos.write(data.getBytes());
catch (IOException e)
e.printStackTrace();
// Reading from internal storage
try (FileInputStream fis = openFileInput(“my_file.txt”))
int size = fis.available();
byte[] buffer = new byte[size];
fis.read(buffer);
String data = new String(buffer);
// Process the data
catch (IOException e)
e.printStackTrace();
“`
SQLite Databases
For more complex data structures and larger datasets, SQLite databases offer a robust and efficient solution. SQLite is a lightweight embedded database engine that’s included with Android. It allows you to store data in tables with defined columns and relationships, providing a structured approach to data management. This is particularly useful for applications that require persistent storage of structured information, such as a contact list or a to-do list.
Database Schema for User Information
A simple database schema for storing user information might include a table named `users` with columns like `id` (INTEGER PRIMARY KEY), `username` (TEXT UNIQUE), `email` (TEXT), and `password` (TEXT). This structure allows for efficient storage and retrieval of user details. You would use SQL queries to interact with this database, inserting, updating, and querying data as needed.
Comparison of Data Storage Methods
The following table compares the different data storage methods discussed:
Method | Pros | Cons | Best Use Cases |
---|---|---|---|
Shared Preferences | Simple, easy to use, quick access | Limited storage capacity, suitable only for small amounts of data | App settings, user preferences |
Internal Storage | Larger storage capacity, private to the app | Data deleted on app uninstall, not suitable for structured data | Storing files, images, or other non-structured data |
SQLite Databases | Robust, efficient for large datasets, structured data storage | More complex to implement than other methods | Storing structured data, such as user profiles, contacts, or inventory |
Networking and APIs
So, you’ve mastered the basics of Android development – congrats! Now it’s time to connect your app to the real world, and that means learning about networking and APIs. APIs, or Application Programming Interfaces, are essentially messengers that allow your app to communicate with servers and retrieve data. This opens up a world of possibilities, from fetching weather updates to displaying social media feeds. Let’s dive into how to make this happen.
This section will guide you through making network requests, parsing JSON responses, handling errors, and running network operations in the background. We’ll use practical examples to illustrate each step, making this process as smooth as possible.
Diving into Android app development? Remember, building a successful app means more than just coding; it’s about protecting your venture. Consider the legal landscape – check out this article on How Insurance Helps Protect Your Business from Lawsuits to understand the importance of safeguarding your work. Getting your app out there involves risk, so smart planning is key from day one.
Making Network Requests with HTTP Libraries
Android provides several libraries for making HTTP requests, but Retrofit and Volley are popular choices. Retrofit, known for its clean and concise syntax, simplifies the process of making network calls. It uses annotations to map HTTP requests to methods in your code. Volley, on the other hand, is optimized for handling multiple network requests concurrently, which is beneficial for apps that require frequent data updates. Below is a simplified example using Retrofit:
First, you’ll need to add the Retrofit dependency to your `build.gradle` file. Then, you can create an interface defining your API endpoints and use Retrofit to create an instance of this interface. This instance will handle the actual network calls. The response will be in JSON format, ready for parsing.
Parsing JSON Data
Once you’ve made a successful network request, you’ll receive data in JSON format. This structured data needs to be parsed into usable objects within your app. Libraries like Gson or Moshi simplify this process by automatically converting JSON to Java objects. You define classes that mirror the structure of your JSON data, and these libraries handle the mapping. Error handling is crucial here; ensure you handle potential exceptions during parsing.
Handling Network Errors and Exceptions
Network requests are inherently unreliable. Issues like network outages, server errors, or timeouts can occur. Robust error handling is essential for a smooth user experience. You should wrap network calls in `try-catch` blocks to catch potential exceptions like `IOException` or `SocketTimeoutException`. Inform the user of the error gracefully, perhaps displaying a message or retrying the request after a delay.
Consider implementing exponential backoff for retrying failed requests. This strategy increases the delay between retries, reducing the load on the server and improving the chances of success.
Implementing Background Tasks for Network Operations
Performing network operations on the main thread can freeze your app’s UI, leading to a poor user experience. Therefore, it’s crucial to perform network operations in the background. You can use tools like Kotlin Coroutines or RxJava to manage background threads effectively. These tools provide mechanisms for asynchronous programming, ensuring that network operations don’t block the main thread.
Coroutines offer a more modern and efficient approach to background tasks compared to older methods like AsyncTask. They are simpler to use and manage, and better integrate with the Android lifecycle.
App Testing and Debugging
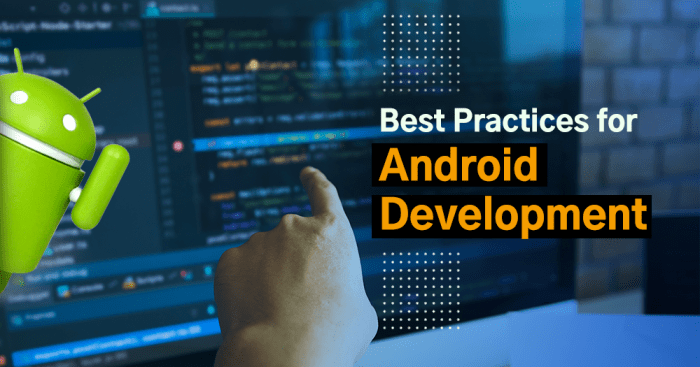
Source: mobisoftinfotech.com
Building an awesome Android app isn’t just about coding; it’s about making sure it works flawlessly. Think of testing and debugging as the final polish that transforms your app from a rough draft into a polished masterpiece ready for the world. This crucial phase ensures a smooth user experience and prevents embarrassing crashes. Let’s dive into the nitty-gritty.
Unit Testing in Android
Unit tests are your first line of defense. They focus on individual components of your app, like a single function or class, to verify they behave as expected. Imagine testing each brick before building a wall – you want to ensure each brick is strong and perfectly shaped before constructing the entire structure. Android provides tools like JUnit and Mockito to help you write these tests. A typical unit test might check if a function correctly calculates a value or if a class properly handles specific inputs. Writing comprehensive unit tests is a crucial part of building robust and reliable apps. They save you countless hours of debugging later by catching errors early in the development cycle.
Using the Android Debug Bridge (adb)
The Android Debug Bridge (adb) is your secret weapon for debugging. This command-line tool lets you interact directly with your Android device or emulator. You can use adb to install and uninstall apps, view logs (essential for finding the source of crashes), and even control your device remotely. For instance, `adb logcat` displays real-time logs from your app, revealing errors, warnings, and other important information. `adb install
Types of Android App Testing
Different testing types target different aspects of your app. Unit tests, as discussed earlier, verify individual components. Integration tests check how multiple components work together, like how your database interacts with your UI. UI tests automate user interactions, verifying that buttons work correctly, screens load properly, and data displays as intended. Each type is important for comprehensive testing: unit tests for individual component correctness, integration tests for component interactions, and UI tests for end-to-end functionality verification. Think of it like a quality control process where each stage catches different types of errors.
Best Practices for Testable Code
Writing clean, maintainable code is paramount for effective testing. Follow these guidelines:
- Keep functions small and focused: Small, single-purpose functions are much easier to test than large, complex ones.
- Follow the Single Responsibility Principle: Each class should have only one responsibility. This makes it easier to isolate and test individual parts of your app.
- Use Dependency Injection: This pattern helps decouple your classes, making them more easily testable.
- Write clear and concise code: Well-documented code is easier to understand and test.
By adhering to these principles, you significantly reduce the complexity of testing your app, leading to faster development cycles and higher quality software.
Deployment and Publishing: Getting Started With Android App Development
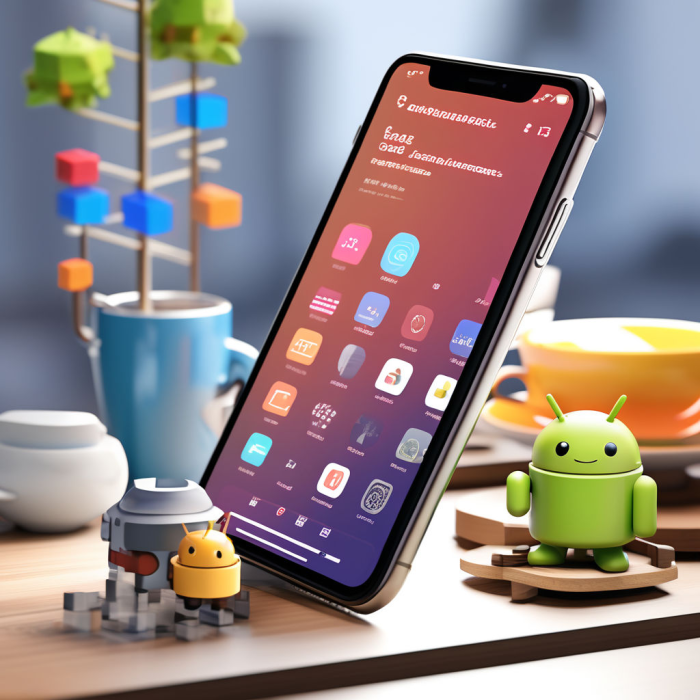
Source: medium.com
So, you’ve built your killer Android app. Congrats! Now comes the exciting (and sometimes slightly terrifying) part: getting it into the hands of users. Publishing your app to the Google Play Store isn’t just about uploading a file; it’s about navigating a process, meeting specific requirements, and preparing your app for a real-world audience. Let’s break down the steps involved.
The journey from your development environment to the Google Play Store involves several key stages, each requiring attention to detail. From creating a release-ready APK to navigating the Play Console’s requirements, a well-executed deployment strategy is crucial for a successful app launch. Ignoring these steps can lead to delays, rejections, and ultimately, a frustrated developer.
Creating a Release-Ready APK
Building a release-ready APK (Android Package Kit) is different from creating a debug APK used during development. Release APKs are optimized for size and performance, undergo rigorous testing, and are signed with a release keystore. This keystore acts as a digital signature, verifying your app’s authenticity and allowing Google Play to manage updates effectively. Failing to use a correctly configured keystore will prevent your app from being published. The process typically involves using Android Studio’s build options to generate a signed APK, selecting the release build type, and ensuring all necessary code obfuscation and optimization steps are completed. A properly configured build process is essential to minimize the app’s size and enhance its performance on various Android devices.
Google Play Store Requirements and Guidelines
Before you even think about uploading, familiarize yourself with the Google Play Store’s policies. These policies cover everything from app functionality and content to user privacy and security. They’re designed to maintain a safe and positive experience for all users. Violating these policies can lead to your app being rejected, sometimes without warning. Key areas to focus on include:
- Content Rating: Accurately rating your app’s content (e.g., age appropriateness, violence, etc.) is crucial. This helps Google categorize and recommend your app to the right audience. An incorrect rating can result in immediate rejection.
- Privacy Policy: You must have a clear and easily accessible privacy policy explaining how your app collects, uses, and protects user data. This is non-negotiable; failure to comply will result in rejection.
- App Functionality: Your app must function correctly and be free of bugs and crashes. Thorough testing is essential before submission.
- Marketing Materials: Accurate and compelling screenshots, app descriptions, and promotional materials are essential to attract users. Misleading or inaccurate information will negatively impact your app’s success.
Publishing to the Google Play Store: A Step-by-Step Guide
Publishing involves several distinct phases. First, you’ll need a Google Play Developer account. Once that’s set up, follow these steps:
- Create a new app release: In the Google Play Console, you’ll create a new release and upload your signed APK.
- Fill out the store listing: This includes the app title, description, screenshots, icon, and other marketing materials. Make sure everything is accurate and appealing.
- Set pricing and distribution: Decide whether your app will be free or paid, and select your target regions.
- Review and submit: Carefully review all information before submitting your app for review. Google will review your app, which can take a few days or longer.
- Respond to feedback (if any): If Google identifies issues, you’ll need to address them and resubmit your app.
- Publish your app: Once approved, you can finally publish your app and make it available to users.
Potential Challenges and Solutions
The publishing process isn’t always smooth sailing. Here are some common challenges and how to tackle them:
- App Rejection: Carefully review Google’s rejection reasons and address them promptly. This often involves fixing bugs, improving app descriptions, or clarifying privacy policies.
- Slow Review Times: Google’s review process can vary. Submitting a well-documented and polished app can help expedite the process.
- Technical Issues: Thorough testing before submission helps prevent technical problems. If issues arise after publishing, promptly address them with updates.
- Marketing Challenges: A successful app launch requires more than just a great app. Develop a solid marketing strategy to reach your target audience.
Advanced Topics (Optional)
Level up your Android app development skills with these advanced features. This section delves into more complex concepts that can significantly enhance your app’s functionality and user experience. While not strictly necessary for basic app creation, mastering these techniques will allow you to build truly powerful and sophisticated applications.
Background Services and Notifications
Background services allow your app to perform tasks even when it’s not actively in the foreground. This is crucial for things like syncing data, playing music, or tracking location. Notifications, on the other hand, are the primary way to alert users to important events happening in the background, even if the app isn’t open. A well-designed notification system is key to maintaining user engagement and providing timely updates. For example, a fitness app might use a background service to track steps and send a notification when a daily goal is reached. Properly managing background processes is essential to avoid draining the device’s battery and maintaining a positive user experience.
Fragments and UI Component Management
Fragments are reusable UI components that can be embedded within an Activity. Think of them as modular building blocks for your app’s interface. They help to manage complex layouts, improve code organization, and enable dynamic UI updates. Using fragments allows you to create adaptable interfaces that can respond to different screen sizes and orientations. For instance, a news app might use fragments to display different sections (top stories, sports, etc.) within a single Activity, making the UI more manageable and efficient. This modular approach also facilitates easier testing and maintenance of individual UI elements.
Location Services and GPS Data
Integrating location services allows your app to leverage the device’s GPS capabilities, enabling location-based features. This opens up possibilities for apps like navigation tools, ride-sharing services, or location-based games. It’s important to handle location data responsibly, respecting user privacy and adhering to relevant permissions. For example, a weather app uses location data to provide localized weather forecasts, while a social media app might use it to show nearby friends or events. Remember to clearly inform users about how their location data will be used and provide options to control these permissions.
Architectural Patterns: MVVM and MVP
Choosing the right architectural pattern is crucial for building maintainable and scalable Android applications. Two popular patterns are Model-View-ViewModel (MVVM) and Model-View-Presenter (MVP). MVVM uses a ViewModel to separate the UI logic from the data, making testing and maintenance easier. MVP separates the presentation logic (Presenter) from the UI (View), leading to a more organized and testable codebase. Both patterns promote a cleaner separation of concerns, resulting in more robust and easily maintainable apps. The choice between MVVM and MVP often depends on the project’s complexity and developer preference; both have proven effective in large-scale Android projects.
Wrap-Up
Building your first Android app is a journey, not a sprint. But armed with the right knowledge and a dash of determination, you’ll be amazed at what you can create. Remember, the most important thing is to start. Experiment, learn from your mistakes, and celebrate every small victory. Before you know it, you’ll be showcasing your app to the world, and that feeling? Pure, unadulterated awesomeness. So, what are you waiting for? Let’s build something amazing!