How to Work with JSON Data in JavaScript? It’s a question every web developer wrestles with sooner or later. JSON, that ubiquitous data format, is the backbone of countless web applications, powering everything from dynamic content updates to complex data visualizations. This guide will walk you through everything you need to know, from parsing and accessing data to handling asynchronous requests and even tackling those monstrous JSON files that seem to defy logic. Get ready to master JSON manipulation in JavaScript!
We’ll cover the fundamentals of JSON syntax and its relationship with JavaScript, showing you how to use `JSON.parse()` and `JSON.stringify()`, and mastering techniques like dot and bracket notation for accessing data within nested objects and arrays. We’ll explore efficient looping strategies for handling JSON arrays, and dive into the power of the `fetch` API for asynchronous data retrieval, including promises and `async/await`. Along the way, we’ll address common pitfalls and offer practical best practices for working with JSON data effectively, even at scale.
Introduction to JSON and JavaScript
JSON (JavaScript Object Notation) and JavaScript are like two peas in a pod, working seamlessly together in the world of web development. Understanding their relationship is key to mastering data handling on the web. JSON is a lightweight data-interchange format, while JavaScript is a programming language that allows you to manipulate and utilize that data. This means JSON provides the structure, and JavaScript provides the tools to interact with it.
JSON Data Structures
JSON structures data in a human-readable format based on key-value pairs. These pairs are organized into objects (enclosed in curly braces “) and arrays (enclosed in square brackets `[]`). Objects represent collections of properties, while arrays represent ordered lists of values. Nesting is allowed, meaning objects can contain other objects and arrays, and vice versa, creating complex data structures. This hierarchical structure allows for the representation of intricate data models. Consider a simple example representing a person: `”name”: “Alice”, “age”: 30, “city”: “New York”`. Here, `”name”`, `”age”`, and `”city”` are keys, and `”Alice”`, `30`, and `”New York”` are their corresponding values.
The Relationship Between JSON and JavaScript
JavaScript’s inherent ability to parse and manipulate JSON data makes it an ideal language for working with this format. The `JSON.parse()` method converts a JSON string into a JavaScript object, making it easily accessible and usable within your code. Conversely, `JSON.stringify()` converts a JavaScript object back into a JSON string, useful for sending data to a server or storing it persistently. This built-in compatibility streamlines the process of data exchange and manipulation. Because JSON syntax is largely derived from JavaScript object literal notation, the transition between the two is very smooth.
Valid and Invalid JSON Syntax
Valid JSON adheres to strict rules. Here’s what makes JSON valid:
- Data is in key-value pairs.
- Keys are enclosed in double quotes.
- Values can be primitive data types (strings, numbers, booleans, null) or other JSON objects or arrays.
- There must be a single top-level JSON object or array.
Invalid JSON often violates these rules. For example, `”name”: ‘Alice’` is invalid because keys must be double-quoted. Similarly, `”name”: Alice` is invalid for the same reason. Unclosed brackets or braces also invalidate JSON. Strict adherence to syntax is crucial for successful parsing.
JSON and JavaScript Data Type Equivalents
The following table shows the correspondence between JSON data types and their JavaScript equivalents:
JSON Data Type | JavaScript Equivalent | Example | Description |
---|---|---|---|
String | String | “Hello, world!” | Text enclosed in double quotes. |
Number | Number | 123, 3.14 | Integer or floating-point number. |
Boolean | Boolean | true, false | Logical value representing true or false. |
Null | null | null | Represents the absence of a value. |
Array | Array | [1, 2, 3] | Ordered list of values. |
Object | Object | “name”: “Alice”, “age”: 30 | Collection of key-value pairs. |
Parsing JSON Data
Okay, so you’ve got this JSON string – a bunch of curly braces and square brackets holding your precious data. But it’s just text, right? Your JavaScript can’t directly access those names and values. That’s where parsing comes in – transforming that raw JSON text into a JavaScript object you can actually *use*.
Think of it like this: you’ve got a delicious cake (your JSON data), but you can’t eat it until you take it out of the box (parse it). JavaScript’s `JSON.parse()` method is your cake-unboxing tool.
JSON.parse() Method
The `JSON.parse()` method is your go-to for turning JSON strings into usable JavaScript objects. It takes the JSON string as an argument and returns a JavaScript object representing that data. It’s super straightforward. For example:
let jsonString = '"name": "Hipwee", "yearFounded": 2014';
let jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Outputs: Hipwee
console.log(jsonObject.yearFounded); // Outputs: 2014
This code snippet first defines a JSON string. Then, `JSON.parse()` converts it into a JavaScript object. Finally, we can access the object’s properties like `name` and `yearFounded`. Easy peasy, lemon squeezy.
Error Handling During JSON Parsing
But what happens if your JSON string is malformed? A single missing comma or a misplaced bracket can throw a wrench in the works. That’s where error handling comes in. A poorly formatted JSON string will cause `JSON.parse()` to throw a `SyntaxError`. To prevent your script from crashing, you should wrap your `JSON.parse()` call within a `try…catch` block.
Try…Catch Blocks for Robust Parsing
The `try…catch` block is your safety net. The code inside the `try` block is where you attempt the potentially problematic operation (in this case, `JSON.parse()`). If an error occurs, the code jumps to the `catch` block, where you can handle the error gracefully.
let jsonString = '"name": "Hipwee", "yearFounded": 2014';
try
let jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name);
catch (error)
console.error("Error parsing JSON:", error);
This improved example handles potential errors. If `JSON.parse()` fails, the `catch` block logs an error message to the console, preventing a complete application crash. Always remember to handle potential errors; it’s good coding practice and prevents unexpected behavior.
Best Practices for Parsing Large JSON Files
For smaller JSON strings, `JSON.parse()` is perfectly fine. But when dealing with massive JSON files, things can get slow. Streaming JSON parsers might be necessary to avoid loading the entire file into memory at once. These advanced techniques are outside the scope of this simple guide, but it’s something to keep in mind for larger datasets. For smaller files, though, sticking with `JSON.parse()` is perfectly acceptable. Remember to optimize for your specific needs.
Accessing JSON Data
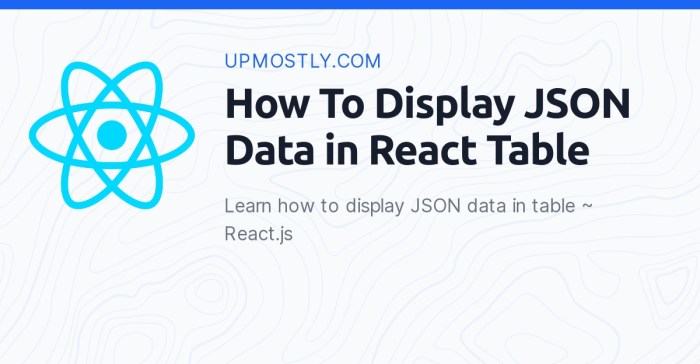
Source: upmostly.com
So, you’ve parsed your JSON data – congrats! Now the real fun begins: getting at that juicy information. Think of your JSON as a well-organized filing cabinet, and accessing the data is like finding the specific file you need. We’ll explore two primary methods: dot notation and bracket notation. Both are equally valid, but one might be more convenient depending on the situation.
Accessing JSON data involves navigating through its nested structure to retrieve specific values. This is done using either dot notation (.
) or bracket notation ([]
), both offering unique advantages and disadvantages.
Dot Notation
Dot notation provides a clean and readable way to access properties of a JSON object, particularly when you know the exact property names beforehand. It’s like directly addressing a file by its name in our filing cabinet analogy. You simply chain property names together using dots.
Let’s say we have a JSON object representing a person:
let person =
"firstName": "John",
"lastName": "Doe",
"age": 30,
"address":
"street": "123 Main St",
"city": "Anytown"
;
To access the person’s first name, we use:
let firstName = person.firstName; // firstName will be "John"
To access the city from the nested address object:
let city = person.address.city; // city will be "Anytown"
Bracket Notation
Bracket notation offers more flexibility, especially when dealing with dynamic property names or nested structures where property names might not be immediately known. Think of this as using a catalog number to find a file instead of its name.
Using the same person
object, we can access the first name using:
let firstName = person["firstName"]; // firstName will be "John"
The key difference here is that the property name is enclosed in square brackets. This is particularly useful when the property name is stored in a variable:
let propertyName = "lastName";
let lastName = person[propertyName]; // lastName will be "Doe"
Accessing nested properties is also straightforward:
let street = person["address"]["street"]; // street will be "123 Main St"
Comparing Dot and Bracket Notation
Dot notation is generally preferred for its readability when property names are known and static. However, bracket notation provides the flexibility needed when dealing with dynamic property names or accessing properties through loops or conditional statements. For nested objects, both notations work equally well, though bracket notation might be slightly more verbose for deeply nested structures.
Extracting Data from a Complex JSON Structure
Let’s consider a more complex scenario: Imagine a JSON object representing a product catalog:
let productCatalog =
"products": [
"id": 1,
"name": "Widget A",
"price": 19.99,
"category": "Electronics"
,
"id": 2,
"name": "Widget B",
"price": 29.99,
"category": "Tools"
]
;
To extract the name of the first product, we could use:
let productName = productCatalog.products[0].name; // productName will be "Widget A"
This demonstrates a combination of dot and bracket notation to access data within arrays and nested objects. We use bracket notation to access the first element of the products
array (index 0) and then dot notation to access the name
property.
Parsing JSON in JavaScript? Piece of cake, once you get the hang of `JSON.parse()`. But life throws curveballs; planning for the unexpected is key, which is why understanding The Importance of Disability Insurance for Your Long-Term Financial Security is just as crucial as mastering your coding skills. After all, reliable income ensures you can keep those JSON projects flowing, even when things get bumpy.
For a more robust solution, especially if you need to iterate through multiple products, you’d likely use a loop. This approach avoids hardcoding indices and makes the code more scalable and maintainable.
Working with JSON Arrays
So, you’ve conquered the basics of JSON in JavaScript – parsing, accessing individual bits of data? Awesome! Now let’s level up and tackle JSON arrays. Think of them as neatly organized lists of JSON objects, each potentially holding a treasure trove of information. Mastering arrays unlocks the power to handle larger, more complex datasets efficiently.
JSON arrays are incredibly common in web APIs, often used to represent collections of items, like a list of products, a feed of social media posts, or even the results of a database query. Knowing how to navigate and manipulate these arrays is key to building dynamic and responsive web applications.
Iterating Over JSON Arrays with Loops
JavaScript offers several ways to loop through a JSON array’s elements. Choosing the right loop depends on your needs and coding style. Each approach offers a slightly different way to access and work with the data. Let’s explore the most popular options: the `for` loop, the `for…of` loop, and the `forEach` method.
Using the `for` Loop
The traditional `for` loop provides precise control over iteration. You specify the starting point, ending condition, and increment step. This is great for when you need to access the index of each element or perform specific actions based on the index.
let jsonArray = ["name":"Apple", "price":1.00, "name":"Banana", "price":0.50, "name":"Orange", "price":0.75];
for (let i = 0; i < jsonArray.length; i++)
console.log("Fruit: " + jsonArray[i].name + ", Price: $" + jsonArray[i].price);
This loop iterates through each object in the `jsonArray`, accessing its properties by index (`jsonArray[i]`) and printing the fruit name and price.
Using the `for...of` Loop
The `for...of` loop offers a cleaner syntax for iterating over iterable objects, including arrays. It directly accesses the value of each element without needing to deal with indices explicitly. This simplifies the code and improves readability.
let jsonArray = ["name":"Apple", "price":1.00, "name":"Banana", "price":0.50, "name":"Orange", "price":0.75];
for (const fruit of jsonArray)
console.log("Fruit: " + fruit.name + ", Price: $" + fruit.price);
Here, the loop directly accesses each fruit object in the `jsonArray`, making the code more concise.
Using the `forEach` Method, How to Work with JSON Data in JavaScript
The `forEach` method is a powerful array method that provides a callback function executed for each element in the array. This approach is often preferred for its readability and conciseness, especially when performing simple operations on each element.
let jsonArray = ["name":"Apple", "price":1.00, "name":"Banana", "price":0.50, "name":"Orange", "price":0.75];
jsonArray.forEach(fruit =>
console.log("Fruit: " + fruit.name + ", Price: $" + fruit.price);
);
The `forEach` method neatly handles the iteration, and the arrow function (`fruit => ...`) provides a compact way to access and process each fruit object.
Manipulating Data Within JSON Arrays
Once you can iterate, you can modify! You might need to update prices, add new items, or remove outdated entries. Directly modifying the array elements is straightforward.
let jsonArray = ["name":"Apple", "price":1.00, "name":"Banana", "price":0.50, "name":"Orange", "price":0.75];
jsonArray[0].price = 1.25; // Update Apple's price
jsonArray.push("name":"Grape", "price":0.60); // Add a new fruit
console.log(jsonArray);
Filtering and Sorting JSON Arrays
Often, you'll need to filter a JSON array to select only specific elements or sort it based on a particular property. JavaScript provides powerful methods to achieve this efficiently.
let jsonArray = [
"name":"Apple", "price":1.00, "color":"red",
"name":"Banana", "price":0.50, "color":"yellow",
"name":"Orange", "price":0.75, "color":"orange",
"name":"Grape", "price":0.60, "color":"purple"
];
//Filter for fruits with price less than $0.75
let filteredArray = jsonArray.filter(fruit => fruit.price < 0.75);
//Sort by price (ascending)
let sortedArray = jsonArray.sort((a, b) => a.price - b.price);
console.log("Filtered array:", filteredArray);
console.log("Sorted array:", sortedArray);
The `filter` method creates a new array containing only the elements that satisfy the given condition. The `sort` method sorts the array in place based on the comparison function provided. Remember, `a.price - b.price` is a crucial part of sorting numerically.
Creating JSON Data
So, you've learned to parse and access JSON data in JavaScript – awesome! Now let's flip the script and learn how to *create* it. Knowing how to generate JSON is crucial for building dynamic web applications that interact with APIs and databases. This section will cover crafting JSON objects and arrays directly within your JavaScript code.
Creating JSON data in JavaScript involves constructing JavaScript objects and arrays, then converting them into JSON strings using the JSON.stringify()
method. This process is straightforward, but understanding the nuances of JSON structure is key to creating valid and easily usable data.
JSON Object Creation
Creating JSON objects in JavaScript is as simple as creating standard JavaScript objects. You define key-value pairs, where keys are strings (usually enclosed in double quotes) and values can be various data types like strings, numbers, booleans, other objects, or arrays. Let's illustrate:
const myJSONObject =
"name": "Hipwee",
"category": "Technology Blog",
"active": true,
"posts": 1000
;
This JavaScript object perfectly mirrors a JSON object. To convert it into a JSON string, use JSON.stringify()
:
const myJSONString = JSON.stringify(myJSONObject);
console.log(myJSONString); // Output: "name":"Hipwee","category":"Technology Blog","active":true,"posts":1000
JSON Array Creation
JSON arrays are simply ordered lists of values, and in JavaScript, these are represented as arrays. Each element in the array can be any valid JSON data type – numbers, strings, booleans, other objects, or even nested arrays.
const myJSONArray = [
"id": 1, "title": "Article 1" ,
"id": 2, "title": "Article 2" ,
"id": 3, "title": "Article 3"
];
Similar to objects, we use JSON.stringify()
to transform this JavaScript array into a JSON array string:
const myJSONArrayString = JSON.stringify(myJSONArray);
console.log(myJSONArrayString);
// Output: ["id":1,"title":"Article 1","id":2,"title":"Article 2","id":3,"title":"Article 3"]
Formatting JSON Data for Readability
While the above JSON strings are technically valid, they're not very readable. For better readability, especially when dealing with complex JSON structures, you can use a second argument in JSON.stringify()
to control the formatting:
const formattedJSONString = JSON.stringify(myJSONObject, null, 2);
console.log(formattedJSONString);
/* Output:
"name": "Hipwee",
"category": "Technology Blog",
"active": true,
"posts": 1000
*/
The second argument, 2
, specifies the number of spaces for indentation, making the JSON much easier to read and understand. You can adjust this value to suit your preference. Using this formatting consistently will improve the maintainability and collaboration on your projects.
Handling Asynchronous JSON Data (AJAX)
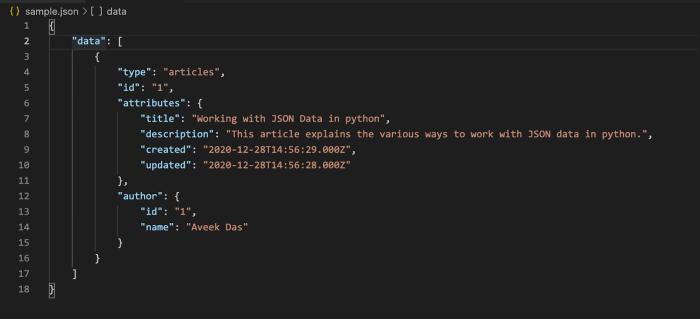
Source: website-files.com
Fetching JSON data from a server is a crucial aspect of modern web development. Often, the data you need isn't readily available on the page itself; it's stored remotely and needs to be retrieved dynamically. This is where AJAX (Asynchronous JavaScript and XML) comes in, allowing your JavaScript code to communicate with a server without interrupting the user experience. While the name includes "XML," JSON has become the dominant data format for this purpose due to its lightweight and human-readable nature.
The `fetch` API provides a clean and modern way to handle these asynchronous requests. It's built into most modern browsers and offers a promise-based approach that simplifies error handling and improves code readability compared to older AJAX methods. Understanding promises and their handling is key to using `fetch` effectively. The `async/await` syntax further streamlines the process, making asynchronous code look and behave more like synchronous code.
The Fetch API and Promises
The `fetch` API sends a request to a specified URL and returns a promise. This promise resolves to a `Response` object, which contains the response from the server. To access the actual JSON data, you then need to call the `json()` method on the `Response` object, which also returns a promise that resolves to the parsed JSON data. If the request fails (e.g., due to a network error or a server error), the promise will reject, and you need to handle that rejection appropriately to avoid crashing your application. Proper error handling is paramount for robust applications. A typical `fetch` call might look like this:
fetch('https://api.example.com/data')
.then(response =>
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
return response.json();
)
.then(data =>
// Process the JSON data here
console.log(data);
)
.catch(error =>
// Handle errors here
console.error('There has been a problem with your fetch operation:', error);
);
Async/Await for Simplified Asynchronous Operations
The `async/await` syntax simplifies the handling of promises by making asynchronous code appear synchronous. The `async` makes a function return a promise, and the `await` pauses execution until a promise resolves. This significantly improves code readability and maintainability. Let's rewrite the previous example using `async/await`:
async function fetchData()
try
const response = await fetch('https://api.example.com/data');
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
const data = await response.json();
// Process the JSON data here
console.log(data);
catch (error)
// Handle errors here
console.error('There has been a problem with your fetch operation:', error);
fetchData();
This version is much cleaner and easier to follow. The `try...catch` block neatly handles potential errors. Remember to replace `'https://api.example.com/data'` with a valid URL providing JSON data.
Example: Fetching and Displaying JSON Data
Let's create a simple example that fetches JSON data from a hypothetical API endpoint and displays it on a webpage. Assume the API returns an array of objects, each representing a product with a name and price. We'll create an unordered list to display this information. Note that this requires a functioning API endpoint at the specified URL. If you don't have one, replace the URL with a publicly available JSON API for testing.
async function displayProducts()
try
const response = await fetch('https://api.example.com/products');
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
const products = await response.json();
const productList = document.getElementById('productList');
productList.innerHTML = ''; // Clear previous content
products.forEach(product =>
const listItem = document.createElement('li');
listItem.textContent = `$product.name - $$product.price`;
productList.appendChild(listItem);
);
catch (error)
console.error('Error fetching products:', error);
const productList = document.getElementById('productList');
productList.innerHTML = '
';
displayProducts();
This code fetches the product data, iterates through it, and dynamically creates list items to display the product name and price on a page element with the ID "productList". The `try...catch` block handles potential errors gracefully, displaying an error message to the user if something goes wrong. Remember to include a `
` element in your HTML.
Advanced JSON Techniques: How To Work With JSON Data In JavaScript
So you've mastered the basics of JSON in JavaScript – congrats! But the real power of JSON unlocks when you delve into more advanced techniques. This section explores strategies for handling complex scenarios and maximizing efficiency when working with JSON data, transforming you from a JSON novice to a JSON ninja.
Beyond simple parsing and accessing, advanced JSON manipulation involves tackling large datasets, ensuring data integrity, and optimizing performance within the browser. Let's explore some key strategies.
JSON Schema Validation
JSON Schema provides a powerful mechanism for validating the structure and content of your JSON data. It defines a schema – a blueprint – that specifies the expected data types, properties, and constraints. By validating against a schema, you can catch errors early, ensuring data consistency and preventing unexpected behavior in your application. For instance, a schema might dictate that a "user" object must have a "name" string and an "age" integer, preventing invalid data from entering your system. This validation can be implemented using libraries like Ajv or others, streamlining the process of ensuring data quality. Schema validation is particularly crucial when dealing with data from external sources, where you have less control over the data's integrity.
Handling Large JSON Datasets
Working with massive JSON files can be a performance bottleneck. Strategies for efficient handling include streaming the data instead of loading it all at once. Libraries exist to help process JSON incrementally, reducing memory consumption and improving responsiveness. Another approach is to use optimized data structures within JavaScript, such as Map or Set, for faster lookups and manipulation if appropriate to your data's organization. Consider pre-processing large datasets on the server-side to reduce the amount of data transferred to the client. For example, you could filter or aggregate data before sending it to the browser, reducing the size of the JSON payload significantly.
Common Challenges and Solutions in Real-World Applications
Real-world JSON handling often presents unique hurdles. One common challenge is dealing with inconsistent or malformed JSON data from external APIs. Robust error handling is crucial, including mechanisms to gracefully handle missing fields, unexpected data types, or parsing errors. Another common issue is managing nested JSON structures, requiring careful navigation and potentially recursive functions to access deeply embedded data. Consider using libraries that offer more advanced JSON path traversal capabilities. Finally, security is paramount; always sanitize and validate JSON data received from untrusted sources to prevent vulnerabilities like cross-site scripting (XSS) attacks.
Efficient JSON Handling in Browser Environments
Optimizing JSON handling in browsers focuses on minimizing performance overhead. Loading and parsing large JSON files can block the main thread, leading to a sluggish user experience. Asynchronous operations are vital; use `fetch` or `XMLHttpRequest` to load JSON data asynchronously, preventing the UI from freezing. Consider using Web Workers to offload computationally intensive JSON processing tasks to separate threads, improving responsiveness. Efficient data structures within JavaScript can further boost performance, and techniques like memoization can reduce redundant computations when working with frequently accessed JSON data. Minimizing unnecessary DOM manipulation related to JSON updates also contributes to a smoother user experience.
Illustrative Example: A Simple JSON Application
Let's build a mini-weather app to solidify our understanding of JSON manipulation in JavaScript. This app will fetch weather data from a sample JSON endpoint (we'll use a placeholder here for simplicity; a real-world app would use a proper API key and endpoint), parse the data, and display it neatly on the webpage.
This example demonstrates a typical workflow: fetching data, handling potential errors, and presenting the information to the user in a user-friendly manner. We'll break down the process step-by-step.
Fetching JSON Data
This section details the process of retrieving JSON data from a specified URL using the `fetch` API. This is a standard method for making asynchronous requests in modern JavaScript.
First, we define the URL of our sample JSON weather data:
const weatherAPI = 'https://example-weather-api.com/weather'; // Replace with a real API endpoint if available
Then, we use the `fetch` API to retrieve the data. The `fetch` function returns a Promise, which we handle using `.then()` to process the successful response and `.catch()` to handle any errors:
fetch(weatherAPI)
.then(response =>
if (!response.ok)
throw new Error(`HTTP error! status: $response.status`);
return response.json();
)
.then(weatherData => displayWeather(weatherData))
.catch(error => console.error('There has been a problem with your fetch operation:', error));
Processing and Displaying Weather Data
This section explains how to process the fetched JSON data and display it to the user. We'll assume our JSON data contains properties like `city`, `temperature`, and `condition`.
The `displayWeather` function takes the parsed JSON data as input and updates the HTML to display the weather information. For simplicity, we'll directly manipulate the DOM:
function displayWeather(weatherData)
const cityElement = document.getElementById('city');
const tempElement = document.getElementById('temperature');
const conditionElement = document.getElementById('condition');
cityElement.textContent = weatherData.city;
tempElement.textContent = `$weatherData.temperature°C`;
conditionElement.textContent = weatherData.condition;
This assumes that we have elements with the IDs 'city', 'temperature', and 'condition' in our HTML where we want to display the data. For instance, our HTML might look like this:
<div>
<p>City: <span id="city"></span></p>
<p>Temperature: <span id="temperature"></span></p>
<p>Condition: <span id="condition"></span></p>
</div>
Error Handling
The example includes error handling to gracefully manage situations where the data fetch fails. The `.catch()` block logs errors to the console, preventing the application from crashing. A more robust application would present a more user-friendly error message to the user. For example, it might display an alert or update the UI to indicate a problem retrieving the data.
Ultimate Conclusion
So there you have it – a comprehensive journey into the world of JSON and JavaScript. You've learned how to parse, access, manipulate, and even create JSON data, handling both synchronous and asynchronous operations with grace. Remember, the key is understanding the structure and utilizing the right tools. Whether you're building a simple application or a complex web service, mastering JSON manipulation in JavaScript is a crucial skill that will elevate your development game. Now go forth and conquer those JSON files!