Understanding the Benefits of Test-Driven Development (TDD): Think writing code is just about getting things to work? Think again. TDD flips the script, building a safety net of tests *before* you even write the actual code. Sounds counterintuitive? It’s not. This approach, while initially seeming slower, ultimately boosts efficiency, reduces bugs, and produces cleaner, more maintainable code. Prepare to have your coding paradigm shifted.
This deep dive explores the core principles of TDD, walking you through its famous “Red-Green-Refactor” cycle. We’ll unpack how TDD leads to improved code quality, enhanced design, increased productivity, and better collaboration. We’ll even tackle common TDD challenges and offer practical solutions to help you navigate the process. Get ready to level up your coding game.
Defining Test-Driven Development (TDD)
Test-Driven Development (TDD) might sound intimidating, like some coding ninja technique, but it’s actually a surprisingly straightforward approach to building software that’s both robust and reliable. Think of it as meticulously planning your code *before* you even write a single line. It’s all about writing tests *first*, then writing the code to pass those tests, and finally, refining that code to be clean and efficient. This iterative process ensures you’re building exactly what you need, and nothing more.
TDD isn’t just about catching bugs; it’s about designing better software from the ground up. By focusing on the desired functionality first (through tests), you’re forced to think more clearly about the problem you’re trying to solve, leading to more modular, maintainable, and ultimately, less buggy code. It’s a proactive approach to quality assurance, saving you headaches (and potentially, your job) down the line.
So, you’re diving into Test-Driven Development (TDD)? Smart move! Just like proactively protecting your bottom line is crucial, understanding the benefits of TDD is equally important for long-term project success. Think of it like risk mitigation; preventing bugs early saves major headaches later, much like securing your business with a robust insurance policy, as detailed in this helpful resource: The Benefits of Insuring Your Business Against Employee Injuries.
Ultimately, both TDD and smart insurance choices are about minimizing unforeseen costs and maximizing long-term gains.
Core Principles of TDD
The core of TDD rests on three fundamental principles: First, you write a failing test that defines a specific piece of functionality. Then, you write the simplest possible code to make that test pass. Finally, you refactor your code to improve its design and readability without changing its functionality. This cycle is repeated for every new feature or piece of functionality. It’s a relentless cycle of testing, coding, and refining.
The TDD Cycle: Red-Green-Refactor
The TDD process is often described using the “Red-Green-Refactor” cycle:
Red: This stage involves writing a failing test. This test should clearly define a specific requirement or piece of functionality. Seeing that red failure message is your confirmation that you’re actually testing something! It’s a crucial first step.
Green: Next, you write the minimal amount of code necessary to make the failing test pass. Don’t worry about elegance or perfection at this stage; just get the test to pass. Think of it as a quick and dirty solution.
Refactor: Once the test is green, you can focus on improving the code’s design, readability, and efficiency. This stage is all about making your code cleaner and more maintainable without altering its functionality (ensuring your tests still pass!).
Implementing TDD: A Step-by-Step Guide
Let’s illustrate TDD with a simple example: writing a function to add two numbers. We’ll use Python and its built-in testing framework, `unittest`.
Step | Action | Code Example | Expected Outcome |
---|---|---|---|
1 | Write a failing test |
“`python import unittest class TestAddition(unittest.TestCase): if __name__ == ‘__main__’: |
Test fails because the `add` function doesn’t exist yet. |
2 | Write the simplest code to pass the test |
“`python def add(x, y): return x + y “` |
Test passes. |
3 | Refactor (in this case, there’s not much to refactor for such a simple function) | No changes needed. | Test still passes. Code remains clean and efficient. |
Benefits of TDD: Understanding The Benefits Of Test-Driven Development (TDD)
Test-Driven Development (TDD) isn’t just a trendy coding practice; it’s a powerful technique that fundamentally alters how you approach software development. By writing tests *before* writing the actual code, you’re setting yourself up for success in terms of code quality, maintainability, and overall project health. Let’s dive into how TDD dramatically improves the quality of your code.
Improved Code Quality through TDD is a game-changer. It’s like having a meticulous architect meticulously plan every detail of a building before a single brick is laid. The result? A structurally sound, elegant, and easily adaptable design. This same principle applies to your codebase.
Cleaner and More Maintainable Code
TDD inherently fosters cleaner and more maintainable code. The act of writing a test first forces you to think deeply about the function or feature’s purpose and expected behavior. This focused approach results in smaller, more modular functions, each with a clearly defined responsibility. This modularity makes the code easier to understand, modify, and debug. Imagine trying to untangle a massive ball of yarn versus working with neatly organized strands – the difference is night and day. With TDD, you’re working with the organized strands.
Reduced Bugs and Errors
The most obvious benefit of TDD is the significant reduction in bugs and errors. Because you’re testing each piece of code as you build it, you catch errors early in the development cycle, when they are much easier and cheaper to fix. Think of it as quality control built directly into your development process. Debugging becomes less of a frantic search for elusive errors and more of a methodical process of verifying the code against pre-defined expectations. The cost of fixing a bug found during testing is far less than fixing a bug found in production.
Codebase Comparison: TDD vs. Non-TDD
Let’s compare codebases developed with and without TDD to illustrate the differences in complexity and readability.
- Code Complexity: A TDD-driven codebase exhibits lower cyclomatic complexity (a measure of code complexity). Functions are smaller, more focused, and easier to understand. A non-TDD codebase, on the other hand, often results in larger, more complex functions that are harder to comprehend and maintain.
- Readability: TDD promotes highly readable code. Each function has a clear purpose, reflected in its name and implementation. Tests themselves act as living documentation, illustrating how the code is intended to be used. Conversely, code written without TDD can become convoluted and difficult to read, making future modifications challenging.
- Maintainability: Changes in a TDD-driven codebase are less likely to introduce new bugs because of the comprehensive test suite. The tests act as a safety net, ensuring that modifications don’t break existing functionality. In contrast, making changes to a non-TDD codebase can be risky, with a higher probability of introducing unintended consequences.
- Testability: Code written with TDD in mind is inherently more testable. It’s designed to be modular and have well-defined interfaces, making it easier to write unit tests. Code written without TDD is often tightly coupled and difficult to test effectively.
Benefits of TDD: Understanding The Benefits Of Test-Driven Development (TDD)
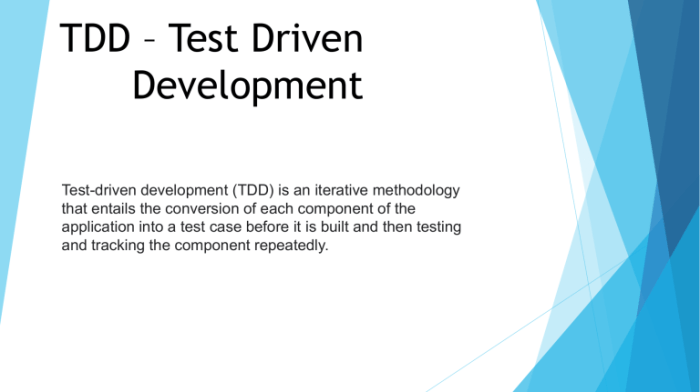
Source: studylib.net
Test-Driven Development (TDD) isn’t just about writing tests; it’s a powerful design philosophy that fundamentally reshapes how you approach building software. By writing tests *before* writing the code, you’re essentially outlining the desired functionality and behavior first. This seemingly simple shift leads to significant improvements in the overall design and architecture of your application.
TDD acts as a constant design feedback loop. Each test represents a small, well-defined piece of functionality, forcing you to think carefully about how different parts of your system interact. This granular approach naturally leads to a more modular and maintainable codebase. Instead of building a monolithic, hard-to-understand system, you’re constructing a collection of independent, testable components that work together seamlessly.
Improved Software Design through TDD
TDD promotes a cleaner, more elegant design by encouraging the creation of small, focused units of code. Because you’re writing tests first, you’re forced to consider the interface and functionality of each component before diving into implementation details. This leads to better separation of concerns, resulting in code that is easier to understand, maintain, and extend. Imagine building a house—with TDD, you’d carefully plan each room (unit of code) before starting construction, ensuring everything fits together perfectly. Without TDD, you might end up with a chaotic structure, difficult to modify or expand.
Modular and Flexible Architecture with TDD
The modular nature of code produced through TDD makes the architecture inherently more flexible. Each module is self-contained and easily replaceable or modifiable without affecting other parts of the system. This allows for easier adaptation to changing requirements and simplifies the integration of new features. Think of Lego bricks: each brick is a self-contained unit, and you can easily rearrange them to create different structures. Similarly, TDD-driven modules can be easily combined and recombined to build different functionalities.
Early Detection of Design Flaws with TDD
One of the most significant advantages of TDD is its ability to identify design flaws early in the development process. By writing tests before writing the code, you’re essentially performing a design review at a granular level. If you find it difficult to write a test for a particular piece of functionality, it often indicates a design problem that needs to be addressed. This proactive approach saves time and resources by preventing costly rework later in the development cycle. For example, if you’re struggling to test a complex function, it might suggest that the function is trying to do too much and needs to be broken down into smaller, more manageable units. This early identification prevents the accumulation of technical debt and improves the overall quality of the software.
Benefits of TDD: Understanding The Benefits Of Test-Driven Development (TDD)
Test-Driven Development (TDD) isn’t just a trendy buzzword; it’s a practical approach that can significantly boost your development team’s efficiency and save your company serious cash. By shifting the focus to writing tests *before* writing code, TDD subtly reshapes the development process, leading to a surprisingly positive impact on productivity and the bottom line. Let’s dive into the specifics.
Reduced Debugging Time and Effort
Debugging is the bane of many a programmer’s existence. Hours, sometimes days, can be lost tracking down elusive bugs, leading to project delays and frustrated developers. TDD dramatically minimizes this time sink. Since you’re writing tests first, you’re essentially outlining the expected behavior of your code *before* you even write it. This upfront planning acts as a safety net, catching potential errors early in the development cycle—before they snowball into larger, more complex problems. The result? Less time spent wrestling with cryptic error messages and more time building features. Imagine the scenario: a developer spends two days debugging a complex issue in a large codebase. With TDD, that same issue might have been caught and fixed within minutes, saving valuable time and resources.
Cost Savings from Fewer Bugs and Easier Maintenance
The cost of fixing bugs increases exponentially the later they are discovered. A bug found during testing is far cheaper to fix than one discovered after release, potentially requiring costly patches, customer support calls, and even legal action. TDD, by its very nature, dramatically reduces the number of bugs that make it to production. This translates directly into significant cost savings. Moreover, the well-structured, modular code that results from TDD is easier to maintain and update. This ease of maintenance further reduces long-term costs, preventing the accumulation of technical debt and simplifying future development efforts.
Faster Development Cycles
TDD might seem counterintuitive at first—writing tests *before* the code itself. However, this upfront investment pays off handsomely in the long run. The clarity and precision demanded by TDD lead to a more focused and efficient development process. Developers are less likely to wander off on tangents or implement unnecessary features. The well-defined test cases provide a clear roadmap, guiding the development process and preventing scope creep. This streamlined approach contributes to faster development cycles and quicker time-to-market.
- Scenario 1: Reduced Rework: A project initially estimated to require 100 hours of debugging without TDD is completed with only 20 hours of debugging using TDD. This translates to a saving of 80 hours of developer time, representing significant cost reduction.
- Scenario 2: Avoided Production Bugs: A bug discovered after release might cost $5,000 to fix, including customer support, updates, and potential legal fees. TDD helps avoid such expensive post-release fixes.
- Scenario 3: Enhanced Maintainability: A project requiring 50 hours of maintenance annually without TDD might only require 20 hours with TDD, saving 30 hours annually and reducing long-term maintenance costs.
Benefits of TDD: Understanding The Benefits Of Test-Driven Development (TDD)
Test-Driven Development (TDD) isn’t just about writing better code; it’s about fostering a healthier, more collaborative development environment. By shifting the focus to defining expected behavior before writing the actual code, TDD inadvertently improves communication and collaboration throughout the entire software development lifecycle. This leads to fewer misunderstandings, quicker resolutions to conflicts, and ultimately, a smoother, more efficient project.
Improved collaboration and communication are significant, often overlooked, advantages of embracing TDD. The structured approach inherent in TDD promotes a shared understanding of project goals and functionality, minimizing the chances of misinterpretations and integration issues.
Improved Developer Collaboration
TDD acts as a shared language among developers. When everyone works from a common set of tests, disagreements about functionality become less frequent. The tests serve as a concrete, unambiguous specification of what the code *should* do. This reduces ambiguity and promotes a consistent understanding of the project requirements. For instance, imagine a team working on an e-commerce platform. With TDD, a developer working on the shopping cart might write a test that verifies the correct calculation of the total price, including taxes and discounts. Another developer working on the payment gateway can then use this same test as a reference to ensure their integration doesn’t break the existing functionality. This shared understanding, built upon a foundation of testable units, minimizes the risk of integration conflicts and significantly streamlines the development process. Instead of lengthy discussions and guesswork, developers can simply refer to the tests to understand the expected behavior and ensure their code integrates seamlessly.
Improved Communication Between Developers and Stakeholders
Well-defined tests serve as living documentation, bridging the communication gap between technical teams and non-technical stakeholders. Stakeholders, such as product managers or clients, may not understand complex code, but they can easily grasp the meaning of a test that verifies a specific feature, like “the login form should reject invalid passwords.” This translates complex technical details into readily understandable statements of functionality. Furthermore, the iterative nature of TDD allows for frequent demonstrations of working software, fueled by the passing tests. This fosters transparency and provides stakeholders with a clear picture of progress and allows for early feedback, preventing costly rework later in the development cycle. For example, a test that verifies the correct display of product images can be easily shown to a client, confirming that their specific requirements are being met. This visual representation and demonstrable progress significantly improve communication and build trust between developers and stakeholders.
Tests as Code Documentation
Comprehensive test suites act as a dynamic and always-up-to-date form of documentation. Unlike static documentation, which can quickly become outdated, tests reflect the current functionality of the code. Each test describes a specific aspect of the system’s behavior, providing a practical example of how a particular feature is intended to work. This “executable documentation” is far more reliable and less prone to errors than traditional documentation methods. For instance, a test case that checks the functionality of a user registration form, covering scenarios like successful registration, password validation, and error handling, offers a clear and concise illustration of how the form should behave. This detailed, functional documentation, directly embedded within the codebase, ensures that developers always have a clear and accurate understanding of the system’s intended functionality, significantly reducing the time spent deciphering complex code or searching through outdated documents.
Addressing Common TDD Challenges
Embracing Test-Driven Development (TDD) can feel like a leap of faith, especially for teams accustomed to traditional development workflows. The initial learning curve and potential for increased development time can be daunting. However, understanding and proactively addressing common challenges is key to successfully integrating TDD into your project. This section will explore some typical hurdles and offer practical solutions to navigate them effectively.
Misconceptions about TDD
Many developers harbor misconceptions about TDD, hindering its effective implementation. One common myth is that TDD significantly increases development time. While initially, writing tests *before* code might seem slower, the long-term benefits of reduced debugging and improved code quality often outweigh this initial overhead. Another misconception is that TDD is only suitable for small projects. This is untrue; well-structured TDD practices, including careful test design and modularity, scale effectively to larger projects. Finally, some believe TDD is solely about unit testing. While unit testing is a core component, TDD also encourages integration and system-level testing to ensure comprehensive code coverage.
Strategies for Overcoming TDD Challenges
Overcoming the challenges of TDD requires a strategic approach. Firstly, start small. Begin by applying TDD to a small, well-defined module or feature within your project. This allows your team to gain experience and confidence before tackling larger components. Secondly, embrace incremental development. Write small, focused tests, then implement the minimal amount of code necessary to pass those tests. Avoid over-engineering at this stage. Thirdly, foster a collaborative environment. Regular code reviews and pair programming can help ensure consistency in testing practices and identify potential issues early on. Finally, invest in training and mentorship. Providing your team with proper TDD training and ongoing support is crucial for successful adoption.
Managing Test Complexity in Large Projects, Understanding the Benefits of Test-Driven Development (TDD)
Scaling TDD to large projects requires careful planning and execution. The sheer volume of tests can become overwhelming, leading to maintenance nightmares and slowing down the development process. Effective strategies include employing robust testing frameworks, implementing continuous integration and continuous delivery (CI/CD) pipelines, and focusing on modular design. Furthermore, prioritizing tests based on risk and criticality can help manage the testing workload efficiently.
Challenge | Solution |
---|---|
Excessive Test Run Times | Employ parallel testing, optimize test suite structure, and utilize caching mechanisms. |
Difficult Test Maintenance | Follow clean code principles when writing tests, use descriptive test names, and refactor tests regularly. |
Fragile Tests | Isolate tests from external dependencies (e.g., databases, APIs), use mocking and stubbing effectively. |
Overly Complex Tests | Break down complex tests into smaller, more focused units. Adhere to the principle of “one test, one assertion”. |
Lack of Test Coverage | Prioritize testing critical paths and high-risk areas. Implement code coverage analysis tools to track progress. |
Illustrative Examples of TDD in Action
Test-Driven Development (TDD) isn’t just theoretical; it’s a practical approach that dramatically improves software development. Let’s dive into some real-world scenarios to see how TDD shines. We’ll explore a simple application, then show how TDD helps navigate a bug fix and improves long-term code maintainability.
Imagine you’re building a simple to-do list application. This app allows users to add tasks, mark them as complete, and delete them. The core functionality revolves around managing a list of tasks, each with a description and a completion status. Using TDD, you would start by writing a test for the most basic functionality: adding a task. This test would fail initially because the code to add a task doesn’t exist yet. You would then write the minimal amount of code necessary to pass the test. Next, you’d write a test for marking a task as complete, then write the code to make that test pass. You’d repeat this cycle for deleting tasks and any other features. This iterative process ensures that each piece of functionality is thoroughly tested from the start, preventing major issues down the line.
A Hypothetical Bug Fix with TDD
Let’s say, during development of our to-do list app, a bug is discovered: completed tasks are still appearing in the main task list. Without TDD, finding the root cause could be a time-consuming process involving extensive debugging. With TDD in place, however, locating and fixing the bug becomes significantly easier. The existing tests for marking a task as complete and displaying the task list would immediately fail, pinpointing the exact location of the error. The developer can then focus their efforts on correcting the specific code segment that failed the test, ensuring a quick and efficient fix. The comprehensive test suite ensures that the fix doesn’t introduce new bugs.
TDD’s Impact on Code Maintainability
Consider a simple function in our to-do list application responsible for calculating the total number of incomplete tasks. Without TDD, this function might be written without much consideration for future changes or extensibility. Over time, as the application grows and new features are added, maintaining and modifying this function becomes a nightmare. With TDD, however, this function would be accompanied by a set of comprehensive tests. These tests act as a safety net, allowing developers to confidently refactor the code or add new functionality without fear of breaking existing behavior. For instance, if the application needs to handle different task priorities in the future, the developer can modify the function to include priority in the calculation. The existing TDD tests will immediately highlight any unintended consequences of these changes, ensuring the integrity of the application.
Ultimate Conclusion
So, is Test-Driven Development worth the hype? Absolutely. While there’s a learning curve, the long-term benefits of cleaner code, fewer bugs, and faster development cycles far outweigh the initial investment. By embracing TDD, you’re not just writing code; you’re crafting a robust, maintainable, and ultimately, more successful application. Ready to ditch the debugging marathons and embrace a more efficient workflow? Dive into TDD today.