Building Your First Game with Pygame in Python: Level up your coding skills and unleash your inner game designer! This guide takes you from zero to hero, walking you through setting up your environment, mastering Pygame’s core functionalities, and building a fully playable Pong clone. We’ll cover everything from handling user input and drawing shapes to implementing game mechanics and adding sound effects – all with clear, concise explanations and plenty of code examples. Get ready to transform lines of code into pixelated fun!
We’ll break down the process into manageable steps, ensuring you understand each concept before moving on. You’ll learn how to create game loops, manage game time, handle collisions, and even add some snazzy sound effects. By the end, you’ll not only have a working Pong game, but a solid foundation in Pygame development that you can use to build even more ambitious projects. So grab your keyboard, and let’s get started!
Setting Up Your Development Environment
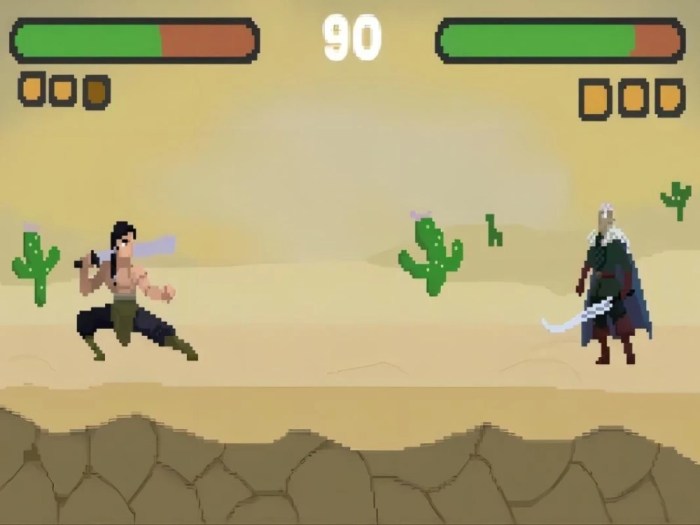
Source: cloudinary.com
So you’re ready to dive into the world of game development with Pygame? Awesome! Before we start crafting pixel-perfect masterpieces, let’s make sure your computer is properly equipped. This involves installing the necessary software and setting up a well-organized project structure. Think of it as building the foundation for your game empire.
Getting your development environment ready is crucial. A smooth setup ensures a hassle-free coding experience, preventing frustrating roadblocks later on. A well-organized project structure also improves code readability and maintainability, particularly as your game grows in complexity.
Python Installation
Installing Python is the first step. Head over to the official Python website (python.org) and download the latest version suitable for your operating system (Windows, macOS, or Linux). During installation, make sure to check the box that adds Python to your system’s PATH environment variable. This allows you to run Python commands from any directory in your terminal or command prompt. The installer will guide you through the process; just follow the on-screen instructions. Once installed, open your terminal or command prompt and type `python –version` or `python3 –version`. You should see the installed Python version displayed, confirming a successful installation.
Pygame Installation
With Python installed, it’s time to bring in Pygame. Open your terminal or command prompt and use the pip package installer (usually included with Python) to install Pygame. Type the command `pip install pygame` and press Enter. Pip will download and install Pygame, along with any necessary dependencies. You might need administrator privileges (running the command prompt as administrator) depending on your system. A successful installation will display a message indicating that Pygame was installed successfully.
Verifying Pygame Installation
To confirm everything’s working as expected, let’s create a simple Pygame program. Create a new file (e.g., `test_pygame.py`) and paste the following code:
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Pygame Test")
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.display.flip()
pygame.quit()
Save the file and run it from your terminal using the command `python test_pygame.py`. If a window titled “Pygame Test” appears and closes without errors, congratulations! Pygame is successfully installed and ready to be used. If you encounter errors, double-check your installation steps and ensure Python and pip are correctly configured.
Project File Organization
Organizing your project files effectively is crucial for larger projects. A well-structured project makes it easier to find, manage, and maintain your code. This is especially important when collaborating with others or working on complex games.
A suggested project structure:
mygame/
├── main.py # Main game file
├── assets/ # Images, sounds, etc.
│ ├── images/
│ │ └── player.png
│ └── sounds/
│ └── jump.wav
├── modules/ # Custom game modules
│ └── player.py
└── documentation/ # Game design documents, etc.
This structure keeps assets separate from code, making it easy to manage and update resources. The `modules` directory allows for better code organization as your game grows in complexity. The `documentation` folder helps keep track of design decisions and game mechanics. Adapting this structure to your specific needs is encouraged. The key is consistency and logical separation of concerns.
Understanding Pygame Basics: Building Your First Game With Pygame In Python
So, you’ve got your Python environment set up and you’re ready to dive into the world of game development. Pygame, a fantastic library, is your trusty sidekick on this adventure. Let’s unpack its core components and get you building your first game in no time. Think of Pygame as a toolbox filled with pre-built functions designed to make game creation easier.
Pygame’s core functionality revolves around a few key modules. These modules handle everything from displaying graphics on the screen to responding to user input. Mastering these will be crucial to your Pygame journey.
Pygame Initialization
Before you can use any of Pygame’s features, you need to initialize it. This process sets up the necessary resources for Pygame to function correctly. It’s like plugging in your game console before you can start playing. The initialization is done using the `pygame.init()` function. If successful, it returns a tuple containing the number of initialized modules. Failure to initialize correctly usually means a dependency isn’t met or a Pygame installation issue.
“`python
import pygame
pygame.init()
# Check for successful initialization
print(pygame.init()) # Output: (6, 0) (example output, numbers may vary)
“`
Creating and Managing Game Windows
Once Pygame is initialized, you can create the game window—the canvas where your game’s action unfolds. This involves setting the window’s size, title, and other display properties. The `pygame.display.set_mode()` function handles this. The function takes a tuple representing the window dimensions as its argument.
“`python
# Set the window dimensions
window_width = 800
window_height = 600
screen = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption(“My First Pygame Game”) # Set the window title
“`
This code creates a window 800 pixels wide and 600 pixels tall, titled “My First Pygame Game”. The `screen` variable is a surface object representing the window’s drawing area.
Handling User Input
Interacting with your game is key, and Pygame makes handling keyboard and mouse input straightforward. The `pygame.event.get()` function retrieves a list of events, such as key presses, mouse clicks, and mouse movements. You then process these events to control your game’s behavior.
“`python
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
print(“Left arrow key pressed”)
if event.type == pygame.MOUSEBUTTONDOWN:
print(“Mouse button clicked”)
pygame.display.flip() # Updates the entire display surface to the screen
pygame.quit() # Quit Pygame
“`
This example shows a basic game loop that continuously checks for events. If the user closes the window (`pygame.QUIT`), the loop ends. Key presses and mouse clicks are also detected and printed to the console. `pygame.display.flip()` updates the entire screen, crucial for displaying changes made to the game window. `pygame.quit()` cleans up Pygame resources after the game ends.
Game Loop Fundamentals
Building a game in Pygame, or any game engine for that matter, hinges on a crucial concept: the game loop. Think of it as the heart of your game, constantly beating, updating, and rendering everything you see on screen. Without a well-structured game loop, your game simply won’t function.
The game loop is essentially a continuous cycle that manages and updates all aspects of your game. It handles user input, updates game logic, and redraws the screen, creating the illusion of movement and interaction. Understanding its structure is fundamental to creating any interactive experience.
The Game Loop’s While Loop
The core of the Pygame game loop is a `while` loop. This loop runs continuously until the game is explicitly closed. Inside this loop, all the necessary game operations are performed. The loop’s condition is usually tied to a variable that becomes `False` when the player quits the game (e.g., by closing the window). A simple structure might look like this:
running = True
while running:
# Handle events
# Update game state
# Draw everything
# Limit frame rate
# Check for quit events
This structure ensures that the game continues to run until the `running` variable is set to `False`.
Managing Game Time and Frame Rate
Maintaining a consistent frame rate is vital for smooth gameplay. A fluctuating frame rate can lead to jerky movements and an unpleasant user experience. Pygame provides tools to control this. The `pygame.time.Clock` class is your best friend here. It allows you to track time elapsed and limit the frame rate to a desired value.
clock = pygame.time.Clock()
FPS = 60 # Frames per secondwhile running:
# ... other game logic ...
clock.tick(FPS) # Limits the frame rate to FPS
The `clock.tick(FPS)` function ensures that the loop doesn’t run faster than the specified FPS. If the game logic takes less time than 1/FPS seconds, it will pause to maintain the desired frame rate. This prevents the game from consuming excessive CPU resources and ensures smoother gameplay.
Handling Events Within the Game Loop
The game loop is where you respond to player actions and other events. Pygame’s `pygame.event.get()` function retrieves a list of events that have occurred since the last call. These events could be keyboard presses, mouse clicks, window resizing, or even joystick movements. The loop iterates through these events, processing them accordingly.
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# Handle left arrow key press
elif event.key == pygame.K_RIGHT:
# Handle right arrow key press
# ... handle other event types ...
This code snippet demonstrates how to handle `QUIT` and `KEYDOWN` events. Similar logic can be applied to handle other event types, allowing for diverse player interactions. Each event type carries specific attributes, allowing you to extract detailed information about the event (like which key was pressed). Efficient event handling is crucial for creating responsive and engaging games.
Drawing Shapes and Images
So you’ve got your Pygame environment set up and understand the game loop – fantastic! Now it’s time to bring your game to life by adding visuals. This section covers drawing basic shapes and loading images, setting the stage for more complex game elements. We’ll explore how to precisely place and scale these visual components to create a polished look.
Pygame provides straightforward functions for drawing shapes directly onto the screen, and it’s equally easy to load and manipulate images. Mastering these techniques is fundamental to building any visually appealing game.
Drawing Basic Shapes
Pygame offers functions to draw various shapes. For rectangles and circles, you use pygame.draw.rect()
and pygame.draw.circle()
respectively. These functions require you to specify the color, position, and dimensions of the shape. For example, to draw a red rectangle at position (50, 50) with width 100 and height 50, you’d use:
pygame.draw.rect(screen, (255, 0, 0), (50, 50, 100, 50))
Similarly, a blue circle with a radius of 30 centered at (200, 100) would be:
pygame.draw.circle(screen, (0, 0, 255), (200, 100), 30)
Remember that the color is represented as an RGB tuple (Red, Green, Blue), each value ranging from 0 to 255.
Loading and Displaying Images, Building Your First Game with Pygame in Python
Loading images involves using pygame.image.load()
, specifying the file path to your image. It’s crucial to convert the loaded image to a suitable format using .convert()
or .convert_alpha()
for better performance, especially with transparency. After loading, the image can be blitted (drawn) onto the screen using screen.blit()
, specifying the image and its position.
For instance, if you have an image named ‘player.png’ in the same directory as your script:
player_image = pygame.image.load('player.png').convert_alpha()
screen.blit(player_image, (100, 150))
This code loads ‘player.png’, handles potential transparency, and draws it at (100, 150).
Scaling and Positioning Images
Precise control over image placement and size is vital. Pygame doesn’t directly resize images; you need to use pygame.transform.scale()
. This function takes the image and the desired width and height as input. Consider a scenario where you need to scale ‘player.png’ to half its original size:
scaled_player = pygame.transform.scale(player_image, (player_image.get_width() // 2, player_image.get_height() // 2))
screen.blit(scaled_player, (100,150))
This scales the image and then blitts it to the screen. The position (100, 150) defines the top-left corner of the scaled image.
Creating a Simple Sprite Function
To encapsulate the process of drawing a sprite (an image representing a game character or object), create a function. This promotes code reusability and organization. The function should take the image, position, and screen as arguments and handle blitting.
def draw_sprite(image, x, y, screen):
screen.blit(image, (x, y))
This function neatly handles the process, allowing you to easily draw the sprite at various positions. For instance, `draw_sprite(player_image, 200, 200, screen)` would draw the player image at (200,200).
Implementing Game Mechanics
So you’ve got your Pygame window up and running, shapes are bouncing around, maybe even a sprite or two. Now for the fun part: making your game actually *do* something! This is where we inject some life into our digital creations, adding rules, interactions, and that satisfying sense of accomplishment (or frustration, depending on the player’s skill!). We’ll tackle collision detection, object movement, basic physics, and managing the game’s overall state. Let’s get coding!
Implementing game mechanics involves bringing your game’s elements to life through interactions and rules. This section covers crucial aspects like collision detection, object manipulation, basic physics integration, and managing the different stages of your game.
Collision Detection
Collision detection is the process of determining whether two or more game objects are overlapping. In Pygame, this often involves checking the bounding rectangles of sprites or shapes. A simple approach uses the `colliderect()` method. For example, if you have two rectangles, `rect1` and `rect2`, you can check for a collision with: `if rect1.colliderect(rect2): # Collision occurred!`. More complex collision detection might involve pixel-perfect checks for smoother interactions, but `colliderect()` is a great starting point for simple games. Consider a scenario where a player-controlled character (represented by a rectangle) needs to collect coins (also rectangles). The game would check if the player’s rectangle intersects with any coin rectangle using `colliderect()`. If a collision is detected, the coin is removed, and the player’s score is updated.
Creating and Moving Game Objects
Creating game objects in Pygame often involves defining classes to represent them. Each class would hold relevant attributes like position, size, image, and speed. Moving objects is typically achieved by updating their position within the game loop. For example, you might add a velocity vector to an object’s position each frame. Let’s say you have a class called `Player` with attributes `x` and `y` representing its position. Inside the game loop, you might update the position like this: `player.x += player.speed_x; player.y += player.speed_y`. This simple addition will move the player smoothly across the screen. Remember to account for boundary conditions to prevent the object from leaving the game screen.
Implementing Basic Game Physics
Basic game physics often involves simulating gravity and movement. Gravity can be implemented by adding a constant downward acceleration to the vertical velocity of objects. For example, you could add a `gravity` variable to your object class and increment the vertical velocity (`speed_y`) by `gravity` each frame. This creates a falling effect. Movement can be controlled by user input, which would adjust the object’s velocity. For instance, pressing the right arrow key might increase `speed_x`, simulating movement to the right. A classic example is a ball falling under gravity. The game would apply a constant downward acceleration to the ball’s vertical velocity, simulating its descent. User interaction (e.g., pressing spacebar) could add an upward impulse, affecting the ball’s vertical velocity.
Managing Game State
Game state refers to the current situation of the game. This includes things like the player’s score, level, lives, whether the game is running or paused, and what screen is currently displayed (e.g., main menu, game over screen). Managing game state often involves using variables or a more structured approach, such as a state machine. A simple approach involves using flags or boolean variables. For example, a variable `game_over` set to `True` would indicate the game is over. More complex games might use a dictionary or class to manage a larger number of game state variables. This dictionary could contain key-value pairs such as `’score’: 100, ‘level’: 2, ‘lives’: 3, ‘game_state’: ‘playing’`.
Adding Sound Effects and Music
Level up your Pygame game from silent pixels to an immersive auditory experience! Adding sound effects and music dramatically enhances gameplay, creating a more engaging and memorable experience for players. We’ll explore how to seamlessly integrate audio into your Pygame projects, transforming them from simple visuals to fully realized interactive worlds.
Pygame provides straightforward methods for loading and playing both sound effects and background music. The key is using the pygame.mixer
module, which handles all the audio processing. Remember to initialize the mixer before using any of its functions. This ensures that Pygame is ready to handle audio playback.
Loading and Playing Sound Effects
Sound effects add punch and realism to game events, like explosions, jumps, or button clicks. Loading and playing these is a simple two-step process. First, you load the sound file using pygame.mixer.Sound()
. Then, you play it using the play()
method. Let’s say you have a sound file named “jump.wav” in your game’s directory:
import pygame
pygame.mixer.init() # Initialize the mixer
jump_sound = pygame.mixer.Sound("jump.wav")
# ... later in your game loop, when the player jumps ...
jump_sound.play()
You can control aspects of sound playback, such as looping, with additional methods. For instance, jump_sound.play(loops=-1)
would play the sound repeatedly until explicitly stopped using jump_sound.stop()
.
Level up your coding skills by building your first game with Pygame in Python – it’s surprisingly easier than you think! But before you hit the virtual road, remember real-world responsibility: if you’re a teen driver, check out this article on Why You Should Consider Adding Car Insurance for Teenage Drivers to stay safe and legal. Then, get back to those Pygame sprites and conquer the game dev world!
Implementing Background Music
Background music sets the mood and atmosphere of your game. Unlike sound effects, background music typically plays continuously. Pygame handles this using pygame.mixer.music
. To play a music track, for instance, “background.mp3”, you would use the following:
pygame.mixer.music.load("background.mp3")
pygame.mixer.music.play(-1) # -1 plays the music on a loop
Remember to handle potential errors, such as the file not being found, using try-except blocks for robust error handling. This prevents your game from crashing if a sound file is missing.
Sound Design’s Importance in Games
Sound design is often underestimated but is crucial for creating a compelling game experience. Well-chosen sound effects and music can significantly enhance immersion, guide the player, and emphasize key moments. For example, a tense, suspenseful soundtrack during a boss battle heightens the drama, while cheerful music during exploration creates a lighter mood. Poor sound design, on the other hand, can detract from the overall experience, making the game feel cheap or unprofessional.
Managing Sound Effects Volume
Giving players control over the volume of sound effects adds to the game’s user-friendliness. This can be achieved by adjusting the volume of the pygame.mixer.Sound
object. The volume is set as a float between 0.0 (muted) and 1.0 (full volume). Here’s how you can create a function to manage sound effect volume:
def adjust_sound_volume(sound, volume):
if 0.0 <= volume <= 1.0:
sound.set_volume(volume)
else:
print("Volume must be between 0.0 and 1.0")
# Example usage:
jump_sound.set_volume(0.5) # Sets the volume to 50%
adjust_sound_volume(jump_sound, 0.8) # Sets the volume to 80%
This function ensures the volume remains within the acceptable range, preventing unexpected behavior or errors.
Creating a Simple Game Example
Let's dive into the satisfying world of game development by building a classic: Pong! This seemingly simple game provides a perfect platform to solidify your Pygame skills and understand core game development concepts. We'll craft a functional Pong clone, focusing on clean code organization, event handling, and collision detection.
We'll break down the process into manageable chunks, starting with the game's structure and moving on to the implementation of its key features. Remember, building a game is an iterative process; start simple, test frequently, and gradually add complexity.
Game Structure and Initialization
Before we start bouncing balls, let's set up the foundation. We'll define our game window, initialize Pygame, and create variables to store our game's essential data. This structured approach ensures our code remains organized and easy to understand, even as the game grows more complex.
Paddle and Ball Creation
Next, we define our game objects: the paddles and the ball. We'll use Pygame's rectangle objects to represent these elements, making collision detection straightforward. Each object will have properties like position, size, and speed. The initial positions and speeds are crucial for setting up the game's starting state.
Event Handling and Player Input
Pygame's event handling system is our bridge to player interaction. We'll listen for keyboard events (typically the 'W' and 'S' keys for one paddle, and the up and down arrow keys for the other) to control the vertical movement of the paddles. We'll use a simple update mechanism to adjust the paddle positions based on these inputs, keeping them within the bounds of the game screen.
Ball Movement and Collision Detection
The ball's movement is governed by its x and y velocity. We'll update its position in each game loop iteration based on these velocities. Collision detection is where things get interesting. We'll check for collisions between the ball and the paddles, and between the ball and the top and bottom edges of the screen. Upon collision with a paddle, the ball's x velocity will reverse, simulating a bounce. Collisions with the top and bottom will reverse the y velocity.
Scoring and Game Over
To make our Pong game truly complete, we'll add a scoring system. Each time the ball passes beyond a paddle's reach, we'll increment the score for the opposing player. We can display the score on the screen using Pygame's font rendering capabilities. A game over condition can be triggered when one player reaches a predefined score.
Key Game Variables
Here's a table summarizing the key variables used in our Pong game:
Variable Name | Data Type | Description | Value (Example) |
---|---|---|---|
window_width |
int |
Width of the game window | 800 |
window_height |
int |
Height of the game window | 600 |
paddle_width |
int |
Width of the paddles | 10 |
paddle_height |
int |
Height of the paddles | 100 |
ball_size |
int |
Diameter of the ball | 15 |
ball_x_speed |
int |
Horizontal speed of the ball | 5 |
ball_y_speed |
int |
Vertical speed of the ball | 5 |
paddle_speed |
int |
Speed of the paddles | 7 |
player1_score |
int |
Score of player 1 | 0 |
player2_score |
int |
Score of player 2 | 0 |
Advanced Techniques (Optional)
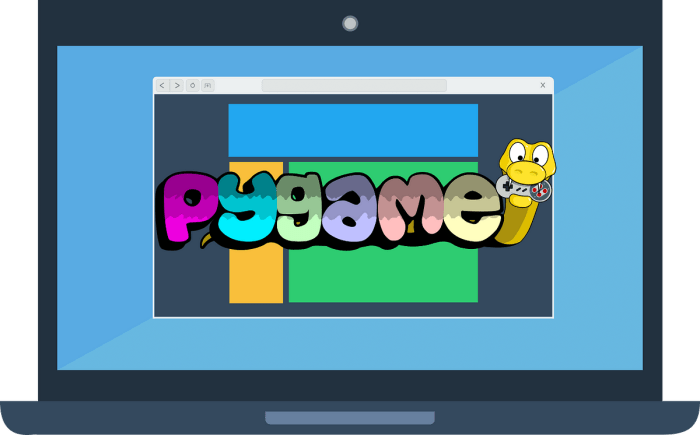
Source: medium.com
Level up your Pygame game development skills with these advanced techniques. Taking your project beyond the basics involves optimizing performance, implementing sophisticated mechanics, and adding polished visual elements. These additions significantly enhance the player experience and transform your game from a simple project into something truly impressive.
This section dives into some optional but highly recommended techniques that can elevate your Pygame game to the next level. We'll explore efficient animation methods, strategies for creating intelligent opponents, performance optimization strategies, and the creation of user-friendly menus.
Sprite Sheets for Animation
Using sprite sheets offers a highly efficient method for animating characters and objects in your game. Instead of loading multiple individual image files, a sprite sheet combines all frames of an animation into a single image. Your code then extracts the appropriate frame from the sheet based on the animation's current state. This significantly reduces the number of files your game needs to load, leading to faster loading times and improved performance. For instance, a character walking animation might have 8 frames; all 8 frames would be arranged sequentially in a single image file, making it easy to access the individual frames using slicing techniques within Pygame. This approach improves performance by reducing I/O operations. Imagine a character walking animation, where each frame is a separate image. Loading eight separate images takes longer and consumes more memory than loading a single sprite sheet containing all eight frames.
Implementing Complex Game Mechanics: AI Opponents
Creating believable and challenging AI opponents adds depth and replayability to your game. Simple AI can be implemented using finite state machines, where the opponent switches between different behaviors based on pre-defined conditions. More advanced AI can leverage pathfinding algorithms (like A*) to navigate the game world, or utilize machine learning techniques for more dynamic and unpredictable behavior. Consider a simple game of Pong: a basic AI opponent might simply move towards the ball's vertical position, while a more sophisticated AI could predict the ball's trajectory and intercept it more effectively. This level of AI complexity dramatically increases the challenge and engagement.
Improving Game Performance and Optimization
Optimizing your game's performance is crucial, especially for larger or more complex projects. Techniques include minimizing the number of objects drawn each frame, using efficient data structures, and leveraging Pygame's optimization features. Profiling your code to identify bottlenecks is also invaluable. For example, drawing many small images individually can be slower than drawing a single, larger image containing all the small images. Techniques like blitting (directly copying pixel data to the screen) are more efficient than drawing shapes repeatedly. Proper use of Pygame's double buffering (drawing to an off-screen surface before updating the display) also dramatically smooths animations.
Designing a Simple Menu System
A well-designed menu system enhances user experience. You can create menus using Pygame's drawing functions to display text and buttons. Event handling allows players to interact with the menu, selecting options and navigating through different screens. Consider using a state machine to manage different menu states (main menu, options, etc.). A typical menu might consist of buttons for "Start Game," "Options," and "Exit," each triggering a specific action. Each button could be represented as a rectangle, with text drawn on top, and event handling to detect mouse clicks within the rectangle's boundaries. The visual appeal of the menu can be greatly improved with the use of images and fonts.
Closure
Creating your first game is a rewarding experience, and Pygame makes it surprisingly accessible. You've learned the fundamentals of game development, from setting up your environment to implementing basic game mechanics and adding sound. Remember, this is just the beginning! The possibilities are endless – experiment with different game concepts, explore advanced techniques, and most importantly, have fun. The world of game development awaits; go forth and create!