Understanding the Basics of Java Programming isn’t just about lines of code; it’s about unlocking a world of possibilities. From crafting sleek mobile apps to building robust enterprise systems, Java’s versatility is undeniable. This guide dives into the core concepts, equipping you with the foundational knowledge to embark on your Java coding journey. We’ll cover everything from setting up your development environment to mastering object-oriented programming, ensuring you’re ready to build your first project in no time.
We’ll break down complex topics into digestible chunks, using clear explanations and practical examples. Get ready to explore the power of Java, understand its architecture, and learn how to write clean, efficient code. Whether you’re a complete beginner or looking to solidify your understanding, this guide provides a solid stepping stone to becoming a proficient Java developer.
Introduction to Java
Java, a ubiquitous programming language, has profoundly shaped the digital landscape. Its journey, from humble beginnings to widespread adoption, reflects its adaptability and power. Understanding its history and core features is crucial for anyone venturing into the world of programming.
Java’s History and Evolution
Java’s story began in the early 1990s at Sun Microsystems (later acquired by Oracle). Initially conceived as “Oak,” intended for interactive television, it quickly evolved to become a platform-independent language ideal for the burgeoning World Wide Web. The “write once, run anywhere” (WORA) philosophy, a cornerstone of Java, revolutionized software development. Major milestones include the release of JDK 1.0 in 1996, the introduction of Java 2 (J2SE, J2EE, J2ME) in the late 1990s, and the continuous evolution through numerous updates and releases, each bringing performance enhancements, new features, and security improvements. The open-source nature of Java, particularly with the advent of OpenJDK, further fueled its growth and community involvement.
Key Features and Benefits of Java
Java boasts several compelling features that have contributed to its enduring popularity. Its object-oriented nature promotes modularity, reusability, and maintainability of code. The platform independence achieved through the Java Virtual Machine (JVM) allows Java applications to run seamlessly across different operating systems without modification. Robust exception handling mechanisms enhance the reliability of applications, while automatic garbage collection simplifies memory management. Java’s extensive libraries and frameworks provide developers with a rich ecosystem of tools and resources, accelerating development cycles. Finally, a large and active community ensures readily available support, tutorials, and third-party libraries.
Real-World Applications of Java
Java’s versatility is evident in its widespread adoption across diverse industries. Android app development heavily relies on Java (and now Kotlin), powering billions of mobile devices worldwide. Many enterprise-level applications, from banking systems to e-commerce platforms, leverage Java’s scalability and security features. Big data technologies like Hadoop and Spark utilize Java for their core components. Furthermore, numerous desktop applications, scientific simulations, and embedded systems utilize Java’s power and flexibility. The sheer breadth of Java’s applications underscores its enduring relevance in the modern technological landscape.
Comparison of Java with Other Popular Programming Languages
Choosing the right programming language depends heavily on the project’s specific requirements. Here’s a comparison of Java with Python and C++:
Language | Paradigm | Strengths | Weaknesses |
---|---|---|---|
Java | Object-Oriented | Platform independence, robust, scalable, large community, extensive libraries | Can be verbose, slower performance compared to C++, steeper learning curve for beginners |
Python | Multi-paradigm (Object-Oriented, Procedural, Functional) | Easy to learn, readable syntax, large community, extensive libraries (especially for data science), rapid prototyping | Generally slower than Java and C++, global interpreter lock (GIL) can limit performance in multi-threaded applications |
C++ | Multi-paradigm (Object-Oriented, Procedural) | High performance, fine-grained control over system resources, widely used in game development and high-performance computing | Steeper learning curve, memory management can be complex, platform-dependent (though cross-compilation exists) |
Setting up the Java Development Environment
So, you’re ready to dive into the world of Java? Awesome! Before you can start crafting amazing applications, you need to set up your development environment. Think of it like prepping your kitchen before baking a cake – you need the right tools and ingredients. This section will guide you through installing the JDK, setting up an IDE, and configuring your system for smooth Java coding.
Installing the Java Development Kit (JDK)
The Java Development Kit (JDK) is the foundation of your Java journey. It contains the Java compiler, runtime environment, and other essential tools. Downloading and installing the JDK is pretty straightforward. First, head to the official Oracle website (or a reputable alternative like Adoptium Temurin) and locate the JDK download page. Choose the version appropriate for your operating system (Windows, macOS, or Linux). Once downloaded, run the installer and follow the on-screen instructions. You’ll likely need to accept the license agreement and choose an installation directory. Remember the installation path, as you’ll need it later.
Setting up an Integrated Development Environment (IDE)
While you *can* write Java code in a simple text editor, using an Integrated Development Environment (IDE) significantly boosts your productivity. IDEs provide features like code completion, debugging tools, and project management capabilities. Popular choices include Eclipse and IntelliJ IDEA (IntelliJ has a free Community Edition). Download the IDE of your choice, run the installer, and follow the instructions. During the installation, you might be asked to specify the JDK installation directory – this is where you’ll use the path you remembered earlier.
Configuring Environment Variables
To ensure your system can easily find the Java compiler and other tools, you need to set up environment variables. This is essentially telling your operating system where to look for Java. The specific steps vary slightly depending on your operating system, but generally, you’ll need to add the `JAVA_HOME` variable, pointing to your JDK installation directory, and add the `%JAVA_HOME%\bin` (or equivalent for your OS) to your `PATH` variable. This allows you to run Java commands from any directory in your terminal or command prompt. A quick Google search for “setting Java environment variables [your OS]” will provide detailed instructions specific to your system.
Compiling and Running a “Hello, World!” Program
Let’s create and run a classic “Hello, World!” program. This is the quintessential first program for any new programmer. Create a new file named `HelloWorld.java` and paste the following code:
public class HelloWorld
public static void main(String[] args)
System.out.println("Hello, World!");
Now, open your command prompt or terminal, navigate to the directory containing `HelloWorld.java`, and type the following commands:
javac HelloWorld.java
java HelloWorld
The first command compiles your code, creating a `HelloWorld.class` file. The second command runs the compiled code, printing “Hello, World!” to your console. Congratulations, you’ve just written and run your first Java program! This simple process showcases the power of the JDK and the ease of using the command line for basic Java operations. Now you’re ready to tackle more complex projects!
Core Java Concepts
Okay, so you’ve got your Java environment set up – congrats! Now it’s time to dive into the heart of Java: its core concepts. This section will unpack the magic behind object-oriented programming (OOP) and show you how it all works in Java. Think of it as learning the building blocks for creating awesome Java applications.
Object-Oriented Programming (OOP) Principles in Java
Java is an object-oriented programming language, which means it revolves around the concept of “objects.” These objects are like real-world entities – a car, a dog, a user account – represented in code. OOP is built on four fundamental principles: encapsulation, inheritance, polymorphism, and abstraction. Let’s break them down. Encapsulation bundles data (attributes) and methods (actions) that operate on that data within a class, protecting the internal state. Inheritance allows creating new classes (child classes) based on existing ones (parent classes), inheriting their attributes and methods. Polymorphism enables objects of different classes to be treated as objects of a common type, allowing for flexible code. Abstraction simplifies complex systems by modeling only essential features, hiding unnecessary details.
Classes and Objects: Attributes and Methods
In Java, a class is a blueprint for creating objects. It defines the attributes (data) and methods (behavior) that objects of that class will have. An object is an instance of a class; it’s a concrete realization of that blueprint.
For example, consider a `Car` class:
“`java
public class Car
String model;
String color;
int year;
public void start()
System.out.println(“Car started!”);
public void accelerate()
System.out.println(“Car accelerating!”);
“`
Here, `model`, `color`, and `year` are attributes, while `start()` and `accelerate()` are methods. To create a `Car` object:
“`java
Car myCar = new Car();
myCar.model = “Toyota Camry”;
myCar.color = “Silver”;
myCar.year = 2023;
myCar.start();
“`
This creates a `Car` object named `myCar` and sets its attributes. Then, it calls the `start()` method.
Inheritance, Polymorphism, and Encapsulation
Let’s see these OOP principles in action. Inheritance lets us create a `SportsCar` class that inherits from the `Car` class, adding features specific to sports cars:
“`java
public class SportsCar extends Car
boolean turbocharged;
public void drift()
System.out.println(“Sports car drifting!”);
“`
`SportsCar` inherits all attributes and methods from `Car` and adds its own (`turbocharged` and `drift()`). Polymorphism allows us to treat both `Car` and `SportsCar` objects as `Car` objects, even though `SportsCar` has extra functionality.
Encapsulation protects the internal state of a class. We can make attributes private, accessing them only through methods (getters and setters):
“`java
public class Car
private String model; // Private attribute
public String getModel() // Getter
return model;
public void setModel(String model) // Setter
this.model = model;
// … other attributes and methods
“`
This prevents direct modification of the `model` attribute, ensuring data integrity.
Designing a Simple Class: The Student Class
Let’s create a `Student` class representing a real-world entity:
“`java
public class Student
String name;
int studentID;
String major;
public Student(String name, int studentID, String major)
this.name = name;
this.studentID = studentID;
this.major = major;
public void printInfo()
System.out.println(“Name: ” + name);
System.out.println(“Student ID: ” + studentID);
System.out.println(“Major: ” + major);
“`
This class has attributes for name, student ID, and major, and a `printInfo()` method to display student details. You can create `Student` objects and use the `printInfo()` method to access their information. For example, creating a `Student` object and printing its information:
“`java
Student student1 = new Student(“Alice”, 12345, “Computer Science”);
student1.printInfo();
“`
Data Types and Variables
Java, like any other programming language, needs a way to store and manipulate information. This is where data types and variables come into play. They’re the fundamental building blocks that allow you to represent and work with different kinds of data within your Java programs. Think of them as containers holding specific types of information, each with its own set of rules and characteristics.
Fundamental Data Types in Java
Java offers a range of built-in data types to handle various kinds of information. These are categorized based on the type of values they can hold. Understanding these types is crucial for writing efficient and error-free code. Choosing the right data type for a specific task optimizes memory usage and prevents unexpected behavior.
int
: Represents integers (whole numbers) such as -2, 0, 10, 1000. It’s commonly used for counting, indexing, and representing quantities.float
: Represents single-precision floating-point numbers (numbers with decimal points). For example, 3.14, -2.5, 0.0. It’s suitable for situations where fractional precision is needed but high accuracy isn’t paramount.double
: Represents double-precision floating-point numbers, offering higher precision thanfloat
. Examples include 3.14159265359, -2.50001, 0.0001. It’s preferred for scientific calculations or when high accuracy is required.boolean
: Represents boolean values, which can only betrue
orfalse
. Used for logical operations and conditional statements.char
: Represents single characters, such as ‘A’, ‘b’, ‘!’, ‘5’. It’s used for handling text characters individually.
Variable Declaration and Initialization
Variables are named storage locations in memory where you can store data. Before using a variable, you must declare it, specifying its data type and name. Initialization means assigning a value to the variable. For example:
int age = 30;
This line declares an integer variable named age
and initializes it with the value 30. The data type (int
) determines the kind of value the variable can hold. Failing to initialize a variable can lead to unpredictable behavior, so it’s a best practice to always initialize variables when you declare them.
Primitive and Reference Data Types
Java distinguishes between primitive and reference data types. Primitive types directly hold the data value (like int
, float
, etc.), while reference types hold a memory address that points to where the data is stored (objects, arrays).
- Primitive Data Types: These are the fundamental data types we’ve already discussed (
int
,float
,boolean
,char
, etc.). They are stored directly in memory. - Reference Data Types: These represent objects and other complex data structures. They store a memory address pointing to the object’s location in memory. Examples include
String
,Arrays
, and custom-defined classes.
Examples of Data Type Usage
Let’s illustrate with some practical examples:
int numberOfStudents = 25; // Integer representing the number of students
double averageGrade = 87.5; // Double representing an average grade
boolean isEnrolled = true; // Boolean indicating enrollment status
char initial = 'J'; // Character representing an initial
String studentName = "John Doe"; // String representing a student's name
These examples show how different data types are used to represent various kinds of information in a program, from numerical values to textual data and logical states. The choice of data type is crucial for accuracy and efficiency.
Operators and Control Flow
Java’s power lies not just in its ability to store and manipulate data, but also in its capacity to control the flow of execution within a program. This control is achieved through operators and control flow statements, allowing you to create dynamic and responsive applications. Understanding these fundamental building blocks is crucial for any aspiring Java developer.
Arithmetic Operators
Arithmetic operators perform mathematical calculations. These include addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). The modulus operator returns the remainder after division. For example, 10 % 3 would result in 1. Java also supports increment (++) and decrement (–) operators, which increase or decrease a variable’s value by one. These operators can be used before (pre-increment/decrement) or after (post-increment/decrement) the variable. The difference lies in when the value is incremented or decremented relative to its use in the expression.
Comparison Operators
Comparison operators compare two values and return a boolean result (true or false). These operators include equals to (==), not equals to (!=), greater than (>), less than (<), greater than or equals to (>=), and less than or equals to (<=). These are fundamental for decision-making within your programs. For instance, if (age >= 18) …
checks if the variable ‘age’ is greater than or equal to 18.
Logical Operators
Logical operators combine boolean expressions. The AND operator (&&) returns true only if both operands are true. The OR operator (||) returns true if at least one operand is true. The NOT operator (!) inverts the boolean value of its operand. For example, if (temperature > 25 && humidity < 50) ...
checks if both temperature is above 25 and humidity is below 50.
Conditional Statements: if-else
The if-else
statement allows you to execute different blocks of code based on a condition. The if
block executes if the condition is true; otherwise, the else
block executes. You can also chain multiple if-else if
blocks to handle multiple conditions. Consider a simple example determining if a number is positive, negative, or zero:
int number = 10;
if (number > 0)
System.out.println("Positive");
else if (number < 0)
System.out.println("Negative");
else
System.out.println("Zero");
Conditional Statements: switch
The switch
statement provides a more concise way to handle multiple conditions based on the value of an integer or enumerated type. It checks the value of an expression against a series of cases. If a match is found, the corresponding block of code is executed. The default
case is optional and executes if no other case matches.
int day = 3;
switch (day)
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Other day");
Looping Constructs: for
The for
loop is used for iterating a specific number of times. It consists of three parts: initialization, condition, and increment/decrement. The loop continues as long as the condition is true.
for (int i = 0; i < 10; i++)
System.out.println(i);
This loop prints numbers from 0 to 9.
Looping Constructs: while
The while
loop repeats a block of code as long as a condition is true. The condition is checked before each iteration.
int count = 0;
while (count < 5)
System.out.println(count);
count++;
This loop also prints numbers from 0 to 4.
Looping Constructs: do-while
The do-while
loop is similar to the while
loop, but the condition is checked at the end of each iteration. This ensures the code block executes at least once.
int count = 0;
do
System.out.println(count);
count++;
while (count < 5);
This loop, again, prints numbers from 0 to 4.
Java Operators Summary
Operator | Symbol | Precedence | Functionality |
---|---|---|---|
Arithmetic | +,-,*,/,%,++,-- | High | Mathematical operations, increment, decrement |
Comparison | ==, !=, >, <, >=, <= | Medium | Compares two values, returns boolean |
Logical | &&, ||, ! | Low | Combines boolean expressions |
Assignment | = | Very Low | Assigns a value to a variable |
Arrays and Collections
Java's power lies not just in its syntax but also in how efficiently it handles data. Understanding arrays and collections is crucial for building robust and scalable applications. These structures provide different ways to organize and access data, each with its own strengths and weaknesses. Choosing the right one depends entirely on your specific needs.
Arrays in Java
Arrays are fundamental data structures in Java, providing a way to store a fixed-size sequence of elements of the same data type. They are declared using square brackets `[]` and initialized with values enclosed in curly braces ``. For example, `int[] numbers = new int[]1, 2, 3, 4, 5;` declares and initializes an integer array named `numbers`. Accessing elements is straightforward using their index (starting from 0). For instance, `numbers[0]` would return 1. The size of an array is fixed at the time of creation; you cannot add or remove elements after initialization. This fixed-size nature is both an advantage (predictable memory usage) and a disadvantage (potential for wasted space or insufficient capacity).
ArrayList in Java
The `ArrayList` class, part of Java's Collections Framework, offers a dynamic array implementation. Unlike standard arrays, `ArrayLists` can grow or shrink as needed. This flexibility makes them ideal when you don't know the exact number of elements beforehand. Elements are accessed using their index, similar to arrays, but the size adjusts automatically as elements are added or removed. Creating an `ArrayList` is simple: `ArrayList
LinkedList in Java
The `LinkedList` class is another important member of the Collections Framework. Unlike `ArrayList`, which stores elements contiguously in memory, `LinkedList` uses a doubly linked list structure. Each element points to the previous and next element, allowing for efficient insertion and deletion of elements at any position. However, accessing elements by index is slower compared to `ArrayList` because the list must be traversed. `LinkedLists` excel in scenarios where frequent insertions or deletions are required, such as managing a queue or stack. Declaration is similar to `ArrayList`: `LinkedList
HashMap in Java
`HashMap` is a key-value data structure that implements the `Map` interface. It uses a hash table to store data, providing fast access to elements using their keys. Each key is unique, and it maps to a specific value. This is extremely useful for representing relationships between data. For example, a `HashMap` could store student names (keys) and their corresponding grades (values). Creating a `HashMap` looks like this: `HashMap
Arrays vs. Collections: A Comparison
Feature | Arrays | Collections (e.g., ArrayList, LinkedList, HashMap) |
---|---|---|
Size | Fixed | Dynamic |
Data Type | Homogeneous (same type) | Homogeneous or Heterogeneous (using wrapper classes) |
Performance (access) | Fast random access | ArrayList: Fast random access; LinkedList: Slow random access |
Performance (insertion/deletion) | Slow (requires shifting elements) | ArrayList: Slow in the middle; LinkedList: Fast |
Flexibility | Low | High |
Example: Using Arrays and Collections
Let's say we need to store a list of student names. Using an array, we might run into size limitations. An `ArrayList`, however, dynamically adjusts to accommodate any number of students. Similarly, if we want to store student names and their grades, a `HashMap` is the perfect choice, allowing for quick retrieval of a student's grade using their name as the key. The choice between arrays and collections depends heavily on the application's requirements and the trade-offs between performance and flexibility.
Input/Output Operations
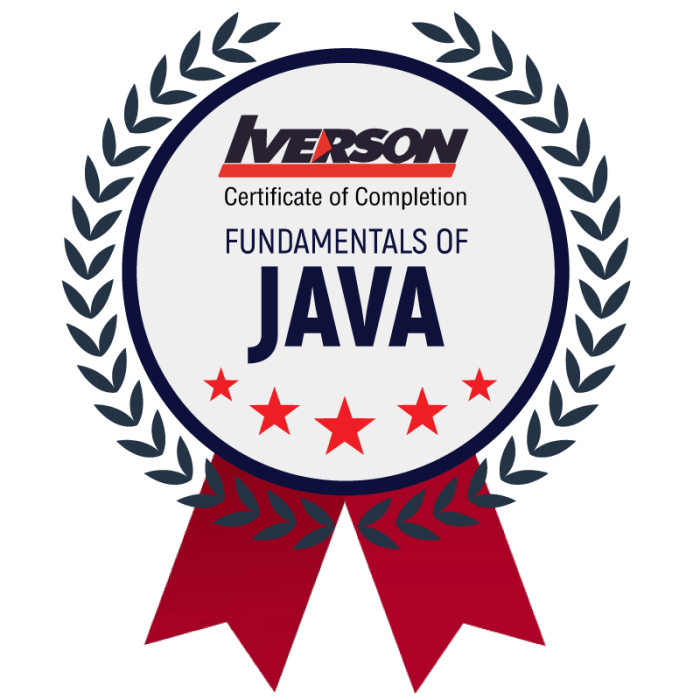
Source: credly.com
Java's power extends beyond internal calculations; it excels at interacting with the outside world through input and output (I/O) operations. This involves getting data from users or files and sending processed data back to the user or storing it persistently. Mastering I/O is crucial for building interactive and data-driven applications.
This section delves into the fundamental techniques for handling I/O in Java, focusing on console input using the Scanner
class and file I/O using FileWriter
. We'll also explore exception handling, a critical aspect of robust I/O programming.
So you're diving into the world of Java programming, learning about objects and methods? That's awesome! But remember, securing your data is just as crucial, especially if you're building applications that handle sensitive info. Check out this article on How to Protect Your Business from Data Breaches with Insurance to understand the importance of robust security measures.
Then, you can confidently build secure and efficient Java applications.
Console Input with Scanner
The Scanner
class provides a straightforward way to read data from the console (standard input). It allows you to retrieve various data types, such as integers, floating-point numbers, and strings. The process typically involves creating a Scanner
object linked to System.in
, then using methods like nextInt()
, nextDouble()
, and nextLine()
to read the corresponding data types.
For example, to read an integer from the user:
Scanner scanner = new Scanner(System.in);
System.out.print("Enter an integer: ");
int number = scanner.nextInt();
System.out.println("You entered: " + number);
scanner.close();
Remember to close the Scanner
using scanner.close()
to release system resources.
File Output with FileWriter, Understanding the Basics of Java Programming
The FileWriter
class facilitates writing data to files. It allows you to create new files or overwrite existing ones. The process involves creating a FileWriter
object, specifying the file path, and using the write()
method to write data to the file. Finally, remember to close the FileWriter
using close()
to ensure data is flushed to disk and resources are released.
Here's an example of writing a string to a file:
FileWriter writer = new FileWriter("output.txt");
writer.write("This is some text to be written to the file.");
writer.close();
Error handling is crucial; if the file doesn't exist or there are permission issues, an exception will be thrown. Proper exception handling prevents program crashes.
Exception Handling during File I/O
File I/O operations are prone to exceptions, such as IOException
(for general I/O errors), FileNotFoundException
(if the specified file doesn't exist), and SecurityException
(if there are permission issues). Using try-catch
blocks is essential for handling these exceptions gracefully.
Consider this example demonstrating exception handling:
try
FileWriter writer = new FileWriter("output.txt");
writer.write("This is some text.");
writer.close();
catch (IOException e)
System.err.println("An error occurred: " + e.getMessage());
This code attempts to write to "output.txt". If any IOException
occurs (e.g., the file is unwritable), the catch
block handles the error, preventing the program from terminating abruptly.
Reading and Writing Data to Files
Reading and writing data to files involves a combination of techniques. For writing, FileWriter
(or its buffered counterpart, BufferedWriter
for improved performance) is used. For reading, classes like FileReader
(or BufferedReader
) are employed. The choice depends on the data type and the desired level of efficiency. For structured data, consider using object serialization or data formats like JSON or XML.
For instance, to read the contents of a file and print them to the console:
try (BufferedReader reader = new BufferedReader(new FileReader("input.txt")))
String line;
while ((line = reader.readLine()) != null)
System.out.println(line);
catch (IOException e)
System.err.println("An error occurred: " + e.getMessage());
This code reads "input.txt" line by line and prints each line. The try-with-resources
statement ensures the reader
is automatically closed, even if exceptions occur.
Methods and Functions
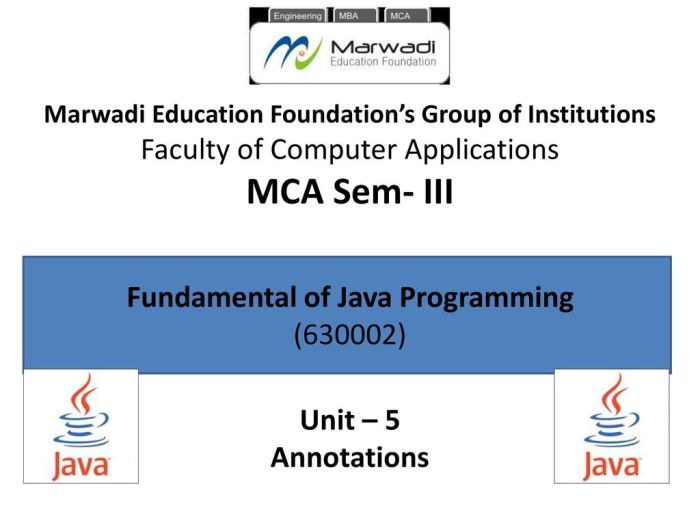
Source: slideplayer.com
Java methods, also known as functions, are blocks of code designed to perform specific tasks. They are fundamental to writing clean, efficient, and reusable Java programs. Think of them as mini-programs within your larger program, promoting modularity and making your code easier to understand and maintain. This modular approach allows you to break down complex problems into smaller, manageable pieces.
Methods are incredibly important because they help you avoid repeating code. Instead of writing the same sequence of instructions multiple times, you write it once as a method and call it whenever needed. This reduces redundancy, making your code shorter, easier to debug, and less prone to errors. It also improves readability, making it easier for others (and your future self!) to understand what your code does.
Defining and Calling Methods
Defining a method involves specifying its name, return type (the type of data it sends back), parameters (input values), and the code it executes. The syntax is straightforward:
public static returnType methodName(parameterType parameter1, parameterType parameter2, ...)
// Method body: code to be executed
return returnValue; //If the method has a return type
Calling a method involves using its name, followed by parentheses containing any necessary arguments (values passed to the parameters). For example:
public static int add(int a, int b)
return a + b;
public static void main(String[] args)
int sum = add(5, 3); // Calling the add method
System.out.println(sum); // Output: 8
In this example, `add` is a method that takes two integers as input and returns their sum. The `main` method calls `add`, passing 5 and 3 as arguments. The returned value (8) is then stored in the `sum` variable and printed to the console. Methods without a return type (like `void`) simply execute their code and don't return any value.
Method Overloading and Overriding
Method overloading allows you to define multiple methods with the same name but different parameters. The compiler distinguishes between them based on the number and types of parameters. This is useful for creating methods that perform similar tasks with varying inputs.
public static int add(int a, int b) return a + b;
public static double add(double a, double b) return a + b;
Here, we have two `add` methods: one for integers and one for doubles. The compiler will choose the correct method based on the types of arguments used when calling it.
Method overriding, on the other hand, occurs in inheritance. A subclass can provide a specific implementation for a method that is already defined in its superclass. This allows you to customize the behavior of inherited methods.
A Program Demonstrating Method Use
Let's create a program that uses methods to calculate the area and perimeter of a rectangle:
public class Rectangle
public static double calculateArea(double length, double width)
return length * width;
public static double calculatePerimeter(double length, double width)
return 2 * (length + width);
public static void main(String[] args)
double length = 5.0;
double width = 3.0;
double area = calculateArea(length, width);
double perimeter = calculatePerimeter(length, width);
System.out.println("Area: " + area); // Output: Area: 15.0
System.out.println("Perimeter: " + perimeter); // Output: Perimeter: 16.0
This program defines two methods, `calculateArea` and `calculatePerimeter`, which are called from the `main` method to calculate and display the area and perimeter of a rectangle. This clearly demonstrates how methods promote code reusability and organization.
Exception Handling: Understanding The Basics Of Java Programming
So, you've learned to write Java code that does amazing things – calculates rocket trajectories, manages complex databases, even builds a virtual pet hamster (if that's your thing). But what happens when things go wrong? A user enters incorrect data, a file is missing, or your network connection drops? That's where exception handling comes in – it's the superhero of your Java program, swooping in to save the day when errors occur.
Exception handling is all about gracefully managing errors in your code without causing your entire program to crash. Instead of a sudden, unexpected stop, you can catch errors, handle them appropriately, and continue execution. This makes your applications more robust and user-friendly.
Try-Catch Blocks: The Exception Handling Duo
The core of Java's exception handling mechanism is the `try-catch` block. The `try` block encloses the code that might throw an exception. If an exception occurs within the `try` block, the program jumps to the corresponding `catch` block that handles that specific type of exception. Think of it like this: you `try` to do something, and if it fails (`catch`), you have a plan B.
Here's a simple example:
```java
try
int result = 10 / 0; // This will throw an ArithmeticException
System.out.println("Result: " + result);
catch (ArithmeticException e)
System.out.println("Error: Cannot divide by zero!");
```
In this example, dividing by zero throws an `ArithmeticException`. The `catch` block intercepts this exception, preventing the program from crashing and printing an informative error message instead.
Types of Exceptions in Java
Java has a rich hierarchy of exception classes. Understanding the different types is crucial for effective exception handling. They're broadly categorized into checked and unchecked exceptions.
Checked exceptions are exceptions that the compiler forces you to handle. These often represent situations that can reasonably be anticipated, like file I/O errors or network problems. You must either catch them using a `try-catch` block or declare them using the `throws` in your method signature.
Unchecked exceptions, also known as runtime exceptions, are not checked by the compiler. These often represent programming errors, like trying to access an array element outside its bounds or using a null pointer. While you can handle them with `try-catch`, it's often more effective to prevent them through careful coding practices.
The Finally Block: Ensuring Cleanup
The `finally` block is an optional part of `try-catch` that guarantees code execution regardless of whether an exception occurred or was caught. It's commonly used for cleanup tasks, like closing files or releasing resources. This ensures that resources are always properly released, preventing resource leaks and improving the stability of your program.
Example:
```java
try
// Code that might throw an exception
FileInputStream fileInputStream = new FileInputStream("myFile.txt");
// ... process the file ...
catch (FileNotFoundException e)
System.out.println("File not found: " + e.getMessage());
finally
// This code ALWAYS executes
System.out.println("Closing file..."); // Simulates closing the file
```
Here, the "Closing file..." message will be printed whether or not a `FileNotFoundException` occurs.
Exception Handling Scenarios
Let's explore a couple of practical scenarios:
Scenario 1: Handling File I/O Errors
When reading or writing files, `IOExceptions` can occur if the file doesn't exist, is inaccessible, or there are other disk errors. Robust file handling involves wrapping file operations in `try-catch` blocks that handle these exceptions.
Scenario 2: User Input Validation
When accepting user input, you should validate it to prevent errors. For example, if your program expects a number, and the user enters text, you might catch a `NumberFormatException`.
Wrap-Up
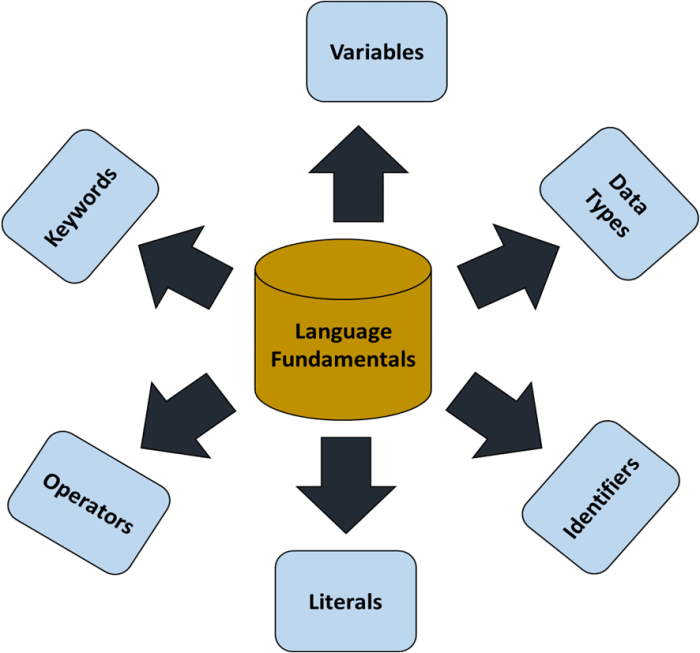
Source: intellipaat.com
So, you've journeyed through the fundamentals of Java programming. You've wrestled with objects, tamed variables, and conquered control flow. Now, armed with this knowledge, the possibilities are endless. Remember, practice is key. Experiment, build projects, and don't be afraid to break things – that's where real learning happens. The world of Java is vast and exciting; this is just the beginning of your coding adventure. Happy coding!