The Power of Automation: Using Scripts to Simplify Tasks – sounds kinda geeky, right? But imagine this: waking up to a perfectly organized inbox, reports generated automatically, or even your social media posts scheduled in advance. That’s the magic of automation scripts. We’re diving deep into how you can harness the power of code to conquer those tedious, repetitive tasks, freeing up your time for things that actually matter (like, maybe, finally finishing that novel you’ve been meaning to write).
This isn’t about becoming a coding ninja overnight. We’ll break down the basics of scripting, explore different languages, and show you how to build your own simple automation scripts. From there, we’ll level up to more advanced techniques, tackling larger datasets and complex workflows. Think of it as a superpower for your productivity – one line of code at a time.
Introduction
In today’s fast-paced digital world, efficiency is king. We’re constantly bombarded with tasks, big and small, that eat into our precious time. But what if there was a way to reclaim those hours, to automate the mundane and focus on what truly matters? Enter the world of scripting and task automation. Essentially, automation involves using scripts – sets of instructions written in a programming language – to perform repetitive tasks automatically. This frees you up to concentrate on more complex and creative endeavors.
Task automation using scripts isn’t some futuristic fantasy; it’s a practical tool accessible to everyone, regardless of their coding expertise. By leveraging readily available tools and pre-built scripts, even beginners can experience the transformative power of automation.
Examples of Automating Everyday Tasks
Many everyday tasks can be significantly streamlined through automation. Imagine automatically backing up your important files every night, ensuring you never lose precious data. Or consider the time saved by automatically downloading and organizing your favorite podcasts, freeing up your mornings for more productive activities. Automated email responses can handle routine inquiries, while scripts can manage social media posts, ensuring consistent engagement with your audience. Beyond these personal examples, businesses utilize automation extensively for processes like data entry, report generation, and customer service interactions.
Advantages of Using Scripts for Task Simplification
The benefits of automating tasks using scripts are numerous and far-reaching. First and foremost, automation drastically reduces the time spent on repetitive tasks. This translates directly into increased productivity and efficiency. Moreover, automation minimizes human error. Scripts follow instructions precisely, eliminating the risk of mistakes associated with manual execution. This leads to improved accuracy and reliability in your work. Finally, automation allows for scalability. Once a script is created, it can be easily used to automate the same task multiple times, across different datasets or platforms, without requiring additional manual effort.
Comparison: Manual vs. Automated Task Completion
The following table illustrates the significant time savings and efficiency gains achieved through task automation. Note that these times are illustrative and can vary based on task complexity and script efficiency.
Task | Manual Time | Automated Time | Efficiency Gain |
---|---|---|---|
Downloading and organizing 100 files | 30 minutes | 2 minutes | 93.3% |
Sending 50 personalized emails | 2 hours | 15 minutes | 92.5% |
Backing up 50GB of data | 1 hour | 5 minutes | 95% |
Generating a monthly sales report | 1 day | 15 minutes | 98.75% |
Types of Automation Scripts
So, you’re ready to dive into the world of automation? Awesome! But before you start slinging code, you need to know which weapon to wield. Choosing the right scripting language is crucial for efficient and effective automation. Think of it like choosing the right tool for the job – a hammer isn’t ideal for screwing in a screw, right?
The scripting language you select will depend heavily on what you’re trying to automate. Each language has its strengths and weaknesses, making them better suited for specific tasks. Let’s explore some popular options and see how they stack up.
Popular Scripting Languages for Automation
Python, Bash, and PowerShell are three heavy hitters in the automation world. Each offers a unique set of capabilities and is best suited for different scenarios. Understanding their strengths and weaknesses is key to picking the right tool for your automation project.
Python, with its readability and vast libraries, shines in complex automation tasks requiring data manipulation or interaction with other systems. Bash, a command-line interpreter, excels at system administration and tasks involving the Linux/Unix environment. PowerShell, Microsoft’s answer to Bash, reigns supreme in Windows environments, allowing powerful control over the operating system.
Python: The Versatile All-Rounder
Python’s clean syntax and extensive libraries make it a favorite for automation tasks ranging from web scraping to data analysis. Its cross-platform compatibility is a huge plus, allowing scripts to run on various operating systems without significant modification. However, Python can be slower than compiled languages for computationally intensive tasks.
* Strengths: Readable syntax, vast libraries (e.g., `requests`, `BeautifulSoup`, `selenium`), cross-platform compatibility.
* Weaknesses: Can be slower than compiled languages for computationally intensive tasks.
* Applications: Web scraping, data processing, automating software testing, interacting with APIs, controlling hardware.
Bash: The Linux/Unix Maestro, The Power of Automation: Using Scripts to Simplify Tasks
Bash, the Bourne Again Shell, is a powerful command-line interpreter integral to Linux and macOS systems. Its strength lies in its ability to seamlessly integrate with the Unix-like operating system, making it ideal for system administration tasks and automating repetitive command-line operations. However, its syntax can be less intuitive for beginners compared to Python.
* Strengths: Tight integration with Linux/Unix systems, powerful command-line capabilities, efficient for system administration tasks.
* Weaknesses: Can be less intuitive for beginners, less portable across operating systems.
* Applications: System administration, automating backups, managing files and directories, scripting server deployments.
PowerShell: The Windows Powerhouse
PowerShell, developed by Microsoft, provides robust control over Windows systems. It excels at managing Active Directory, configuring applications, and automating administrative tasks within the Windows environment. While powerful, its syntax can be challenging for those unfamiliar with the .NET framework.
* Strengths: Deep integration with Windows, powerful for managing Active Directory and other Windows components.
* Weaknesses: Primarily Windows-based, steeper learning curve for beginners compared to Python.
* Applications: Active Directory management, Windows system administration, automating software deployments, managing network configurations.
Examples of Automation Scripts and Their Use Cases
Choosing the right scripting language is only half the battle. Understanding how to apply these languages to real-world scenarios is just as crucial.
Here are a few examples illustrating the diversity of automation scripts and their practical applications:
- Python script for automating social media posting: A Python script could be used to schedule and automatically post updates to various social media platforms at pre-defined times, saving you valuable time and ensuring consistent engagement.
- Bash script for automating server backups: A Bash script can automate the process of backing up server data to a remote location on a regular schedule, minimizing the risk of data loss.
- PowerShell script for automating user account creation: A PowerShell script can automate the creation of new user accounts in Active Directory, streamlining the onboarding process for new employees.
- Python script for web scraping product prices: A Python script, utilizing libraries like `requests` and `BeautifulSoup`, can scrape product prices from e-commerce websites, allowing for price comparison and automated alerts.
- Bash script for monitoring system resources: A Bash script can continuously monitor system resources like CPU usage, memory, and disk space, sending alerts if thresholds are exceeded.
Building Basic Automation Scripts
Let’s ditch the repetitive drudgery and dive into the exciting world of building your own automation scripts! We’ll craft a simple script to handle a common task, showing you the power of automation firsthand. Think of it as your first step towards becoming a scripting ninja.
This section will walk you through creating a basic script, explaining each line of code in detail, demonstrating its execution, and showing how to handle potential errors. We’ll use Python, a versatile and beginner-friendly language, perfect for getting started with automation.
File Renaming Script
This script will rename all `.txt` files in a specified directory, adding a prefix to each filename. This is a perfect example of a task easily automated to save time and prevent manual errors.
We’ll assume you have a directory containing several `.txt` files. The script will add the prefix “Auto_” to each filename. For instance, `mydocument.txt` will become `Auto_mydocument.txt`.
“`python
import os
def rename_files(directory, prefix):
for filename in os.listdir(directory):
if filename.endswith(“.txt”):
base, ext = os.path.splitext(filename)
new_filename = prefix + base + ext
os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))
# Specify the directory and prefix
directory_path = “/path/to/your/files” # Replace with your directory
prefix_to_add = “Auto_”
# Execute the script
try:
rename_files(directory_path, prefix_to_add)
print(“Files renamed successfully!”)
except FileNotFoundError:
print(f”Error: Directory ‘directory_path’ not found.”)
except OSError as e:
print(f”An error occurred: e”)
“`
Let’s break down the code line by line:
* `import os`: This line imports the `os` module, which provides functions for interacting with the operating system, including file system operations.
* `def rename_files(directory, prefix):`: This defines a function called `rename_files` that takes the directory path and the prefix as input.
* `for filename in os.listdir(directory):`: This loop iterates through all files and directories in the specified directory.
* `if filename.endswith(“.txt”):`: This condition checks if the filename ends with “.txt”.
* `base, ext = os.path.splitext(filename):`: This line splits the filename into its base name and extension.
* `new_filename = prefix + base + ext`: This creates the new filename by adding the prefix to the base name.
* `os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))`: This line renames the file using the `os.rename()` function. `os.path.join()` ensures correct path construction across different operating systems.
* The `try…except` block handles potential errors: `FileNotFoundError` if the directory doesn’t exist, and a generic `OSError` for other file system errors. This is crucial for robust script execution.
To execute this script, replace `/path/to/your/files` with the actual path to your directory containing the `.txt` files. Then, run the script using a Python interpreter. The output will indicate success or any errors encountered. For example, if the script runs successfully, you’ll see “Files renamed successfully!” printed to the console. If the directory is not found, you’ll get an appropriate error message. This error handling makes the script more user-friendly and less prone to unexpected crashes.
Advanced Automation Techniques: The Power Of Automation: Using Scripts To Simplify Tasks
Stepping up your automation game means mastering more sophisticated techniques. Beyond basic scripts, the real power lies in leveraging loops, conditional logic, and seamless integration with other applications. This unlocks the potential to automate complex tasks and manage vast amounts of data with ease and efficiency.
Looping and Conditional Statements
Loops and conditional statements are the backbone of powerful automation. Loops allow you to repeat a block of code multiple times, while conditional statements (like “if-then-else” structures) allow your script to make decisions based on certain conditions. For instance, a loop could process each file in a directory, while a conditional statement might check if a file exists before attempting to process it. This combination enables scripts to handle dynamic situations and adapt to changing data. Consider a script that needs to process a thousand spreadsheets: a loop iterates through each spreadsheet, and a conditional statement within the loop could check for errors in each sheet before moving on to the next. Efficient error handling and robust data processing are key benefits.
Interacting with External Applications and APIs
The ability to interact with other applications and APIs (Application Programming Interfaces) opens a whole new world of automation possibilities. APIs are essentially messengers that allow different software programs to communicate and exchange data. Your automation script can use APIs to interact with web services, databases, or other software, enabling tasks like automatically uploading data to a cloud service, fetching data from a website, or controlling other applications. For example, a script might use the Twitter API to automatically post tweets, or a Google Sheets API to update spreadsheets with data from a sensor. This interoperability is essential for building truly integrated and powerful automation workflows.
Handling Large Datasets and Complex Workflows
Automation truly shines when handling large datasets and complex workflows. Imagine needing to process terabytes of data or coordinate dozens of individual steps. Without automation, this would be a nightmare. However, by breaking down the tasks into smaller, manageable units and using loops and conditional statements to control the flow, you can build robust and efficient scripts. Consider a script that processes a large CSV file: the script might read the file in chunks, process each chunk individually, and then combine the results. Error handling is critical in such scenarios, as a single error could bring the entire process to a halt. Well-structured code, robust error handling, and efficient data management techniques are key to success in these situations.
Building a Complex Automation Script: A Step-by-Step Guide
Let’s build a script that automates a slightly more involved process: Imagine you have many image files, and you need to resize them all to a specific dimension and then rename them sequentially.
- Define Requirements: Clearly Artikel the steps involved. We need to iterate through all image files in a directory, resize each image, and rename it with a sequential number.
- Choose Your Tools: Select appropriate libraries or modules. Python, with libraries like Pillow (for image manipulation) and os (for file system operations), is a good choice.
- Write the Code: This involves using loops to iterate through the files, conditional statements to handle potential errors (e.g., non-image files), and functions to encapsulate the resizing and renaming logic.
- Test Thoroughly: Test your script on a small sample of images before running it on the entire dataset. This helps catch errors early and ensures the script works as expected.
- Optimize and Refine: Once tested, refine the script for efficiency and robustness. Consider adding error logging and handling to make it more resilient.
A simplified Python example (error handling omitted for brevity):
“`python
from PIL import Image
import osdef resize_and_rename(input_dir, output_dir, new_width, new_height):
i = 1
for filename in os.listdir(input_dir):
if filename.endswith((‘.jpg’, ‘.jpeg’, ‘.png’)):
img_path = os.path.join(input_dir, filename)
img = Image.open(img_path)
img = img.resize((new_width, new_height))
new_filename = f”i:04d.jpg” # 0001.jpg, 0002.jpg, etc.
new_img_path = os.path.join(output_dir, new_filename)
img.save(new_img_path)
i += 1resize_and_rename(“input_images”, “output_images”, 640, 480)
“`
This example demonstrates the core concepts: iterating through files, image processing, and sequential renaming. A real-world implementation would require more robust error handling and potentially more sophisticated image processing techniques.
Security and Best Practices
Automating tasks is awesome—it saves time and boosts efficiency. But like any powerful tool, automation scripts can introduce security risks if not handled carefully. Think of it like this: you’re giving your computer a set of instructions; if those instructions are flawed, things can go sideways quickly. Let’s explore how to build secure and reliable automation scripts to avoid those potential pitfalls.
Security in automation isn’t just about preventing malicious attacks; it’s also about preventing accidental damage from poorly written scripts. A script that accidentally deletes important files or sends sensitive data to the wrong place can be just as damaging as a deliberate attack. Therefore, a robust approach to security involves both preventative measures and careful error handling.
Potential Security Risks
Automation scripts, if poorly designed or implemented, can open doors to various security vulnerabilities. For example, scripts accessing sensitive data without proper authentication could expose confidential information. Similarly, scripts with hardcoded credentials are extremely vulnerable to compromise. Another common risk is insufficient input validation, allowing malicious users to inject harmful commands into the script. Finally, scripts lacking proper error handling can crash unexpectedly, leaving systems in an unstable state.
Best Practices for Secure Scripting
Building secure automation scripts requires a multi-layered approach. First, always employ the principle of least privilege. This means granting your scripts only the minimum necessary permissions to perform their tasks. Avoid running scripts with administrator privileges unless absolutely essential. Second, never hardcode sensitive information like passwords or API keys directly into your scripts. Instead, use environment variables or secure configuration files to store this information separately. Third, rigorously validate all user inputs to prevent injection attacks. This involves checking data types, lengths, and formats to ensure they conform to expected values. Finally, regularly review and update your scripts to address any known vulnerabilities or security patches.
Error Handling and Logging
Robust error handling is crucial for preventing unexpected script failures and ensuring system stability. Implement comprehensive try-except blocks to catch and handle potential errors gracefully. Instead of crashing, your scripts should log the error, attempt recovery if possible, and notify the user or administrator. Thorough logging is equally important. Record all significant events, including successful operations, errors, and warnings, with timestamps and relevant context. This detailed logging helps in debugging, auditing, and identifying potential security breaches. Consider using structured logging formats like JSON to facilitate easier analysis and integration with monitoring systems.
Version Control for Automation Scripts
Implementing version control is essential for managing changes to your automation scripts over time. Using a system like Git allows you to track modifications, revert to previous versions if needed, and collaborate with others on script development. This not only simplifies maintenance but also provides a valuable audit trail, aiding in security investigations and ensuring accountability. Regular commits with clear, descriptive messages are key to maintaining a well-organized and understandable version history. Branching strategies can further enhance collaboration and help isolate experimental changes from the main codebase.
Real-World Applications
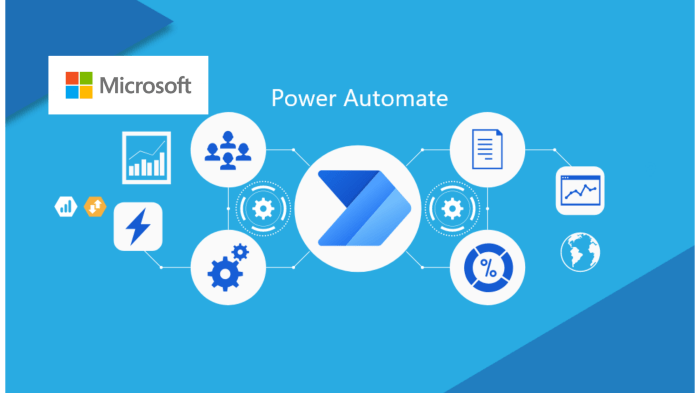
Source: gizmeon.com
Automating tedious tasks is a game-changer, freeing up time for what truly matters. Think about managing chronic illnesses – the paperwork, appointments, and medication tracking can be overwhelming. Understanding the intricacies of your health coverage, as detailed in this insightful article on The Role of Health Insurance in Managing Chronic Illnesses , is crucial. By automating reminders and record-keeping, you reclaim control and simplify the complexities of healthcare management.
Automation scripts aren’t just a futuristic fantasy; they’re quietly revolutionizing industries worldwide. From streamlining mundane tasks to enabling complex analyses, their impact is undeniable, boosting efficiency and freeing up human capital for more strategic endeavors. Let’s dive into some real-world examples showcasing the power of automation.
The benefits of automation extend far beyond simple time-saving. By automating repetitive processes, businesses reduce errors, improve consistency, and free up employees to focus on higher-value work that requires creativity and critical thinking. This ultimately leads to increased productivity, cost savings, and a significant competitive advantage in today’s fast-paced market.
Examples of Automation Across Industries
Automation scripts are transforming various sectors, each benefiting from tailored solutions. In IT, scripts automate server maintenance, software deployments, and network monitoring. Finance leverages scripts for high-frequency trading, risk assessment, and fraud detection. Marketing teams utilize scripts for email campaigns, social media management, and data analysis. These are just a few examples; the possibilities are practically limitless.
Case Studies: Successful Automation Implementations
Here are some compelling examples of how automation scripts have yielded significant results across different industries:
Industry | Task Automated | Scripting Language | Benefits Achieved |
---|---|---|---|
IT | Daily server backups and log analysis | Python | Reduced downtime by 75%, improved data security, freed up IT staff for more complex projects. |
Finance | Automated report generation and data reconciliation | R | Improved accuracy of financial reporting by 90%, reduced processing time by 80%, minimized human error. |
Marketing | Automated email marketing campaigns and social media posting | JavaScript (Node.js) | Increased engagement rates by 40%, improved customer segmentation, optimized campaign scheduling, allowing marketers to focus on creative content. |
E-commerce | Order processing and inventory management | PHP | Reduced order fulfillment time by 50%, minimized stockouts, improved customer satisfaction through faster delivery. |
Future Trends in Automation
The world of automation scripting is constantly evolving, driven by rapid advancements in technology. We’re moving beyond simple, rule-based scripts towards intelligent systems capable of learning, adapting, and even predicting user needs. This shift promises to revolutionize how we approach automation, opening up exciting new possibilities while also presenting unique challenges.
The integration of Artificial Intelligence (AI) and Machine Learning (ML) is arguably the most significant trend shaping the future of automation. These technologies empower scripts to go beyond pre-programmed instructions, enabling them to analyze data, identify patterns, and make decisions autonomously. This means scripts can become more efficient, adaptable, and capable of handling complex tasks previously requiring human intervention.
AI and Machine Learning’s Impact on Automation Scripting
AI and ML are fundamentally altering the landscape of automation scripting. Instead of relying solely on explicit instructions, scripts can now learn from data, improving their performance over time. This “learning” aspect allows for the creation of self-improving scripts that require less human intervention for maintenance and updates. For instance, a script designed to categorize customer emails could initially rely on predefined rules. However, with ML, it can learn to identify new categories and improve its accuracy based on the analysis of a large dataset of past emails. This results in more accurate and efficient email sorting over time, reducing manual effort and improving overall efficiency. This dynamic adaptation makes scripts more robust and adaptable to changing environments.
Challenges and Opportunities in Automated Scripting
The increasing sophistication of automation presents both challenges and opportunities. One key challenge is ensuring the security and reliability of AI-powered scripts. As scripts become more autonomous, the potential for errors or malicious use increases. Robust security measures and rigorous testing protocols are crucial to mitigate these risks. On the other hand, the opportunities are immense. The ability to automate complex, data-intensive tasks can significantly improve productivity across various industries, from finance and healthcare to manufacturing and logistics. Furthermore, the development of user-friendly tools and platforms for creating and managing AI-powered scripts will democratize access to automation technology, empowering a wider range of users.
Visual Representation of Automation Scripting Evolution
Imagine a timeline. At the beginning (perhaps the 1980s), we see simple batch scripts – rudimentary, linear sequences of commands. These are represented by a single, straight line. Then, in the 1990s and early 2000s, we see the rise of more sophisticated scripting languages like Python and Perl, branching out from the initial line – representing increased complexity and functionality. These branches represent different scripting paradigms and applications. Fast forward to the present day, and the timeline explodes into a complex network of interconnected nodes and paths. This intricate web symbolizes the integration of AI and ML, where scripts are no longer just executing pre-defined tasks but actively learning, adapting, and making decisions based on data analysis. The future extends this network further, with even more intricate connections and unforeseen paths, signifying the ever-expanding possibilities of intelligent automation.
Concluding Remarks
So, there you have it: a glimpse into the world of automation scripting. While the initial learning curve might seem steep, the long-term benefits are undeniable. Imagine a world where mundane tasks are handled effortlessly, freeing you up to focus on creativity, innovation, and maybe even a little bit of well-deserved relaxation. Embrace the power of automation – your future self will thank you.