How to Write Secure Code in JavaScript: Think your JavaScript code is bulletproof? Think again! From sneaky XSS attacks to the ever-present threat of CSRF, the digital world is a jungle out there. This guide isn’t just for seasoned devs; even beginners need to know the basics of keeping their code—and their users—safe. We’ll unravel the mysteries of input validation, secure data handling, and choosing the right libraries, showing you how to build JavaScript applications that won’t leave you vulnerable.
We’ll cover everything from the fundamentals of secure coding practices to advanced techniques for protecting against sophisticated attacks. Get ready to level up your JavaScript security game and build applications that are as robust as they are innovative. We’ll explore real-world examples, practical tips, and clear explanations, making sure you understand not just *what* to do, but *why* it’s crucial.
Introduction to Secure Coding Practices in JavaScript
JavaScript, the ubiquitous language powering interactive web experiences, also presents significant security challenges. Failing to prioritize secure coding practices can expose your applications and users to devastating consequences, from data breaches and financial losses to reputational damage and legal repercussions. Understanding and implementing robust security measures is therefore not just a best practice, but a necessity for any serious JavaScript developer.
Common JavaScript Vulnerabilities
JavaScript applications are susceptible to a range of vulnerabilities, many stemming from how the language interacts with browsers, servers, and user input. Ignoring these vulnerabilities can lead to serious security breaches. Let’s examine some of the most prevalent threats.
Cross-Site Scripting (XSS) attacks exploit vulnerabilities to inject malicious scripts into websites viewed by other users. Imagine a scenario where a user’s input is directly reflected back on a page without proper sanitization. An attacker could insert JavaScript code into a comment field, which would then execute in the context of other users’ browsers, potentially stealing cookies, redirecting users to phishing sites, or defacing the website.
CSRF (Cross-Site Request Forgery) attacks trick users into performing unwanted actions on a website they’re already authenticated to. This is often done by embedding malicious links or forms within seemingly legitimate websites. For instance, a malicious link might be disguised as a “like” button; when clicked, it could unknowingly perform actions on the user’s behalf, like transferring funds or changing account settings.
SQL Injection attacks target applications that interact with databases. If user input isn’t properly parameterized, an attacker could craft malicious SQL queries that bypass security measures and access, modify, or delete sensitive data. For example, a poorly designed login form could allow an attacker to inject code into the username field, executing arbitrary SQL commands on the database.
Real-World Examples of JavaScript Security Breaches
Numerous high-profile incidents demonstrate the devastating impact of insecure JavaScript code. One notable example involves a vulnerability that allowed attackers to compromise user accounts on a popular social media platform by injecting malicious JavaScript into comments. This breach resulted in the theft of personal information and a significant loss of user trust. Another case involved an e-commerce website where an XSS vulnerability allowed attackers to steal credit card information, causing substantial financial losses for both the business and its customers. These breaches highlight the importance of proactive security measures.
JavaScript Security Vulnerabilities and Mitigation Strategies
Vulnerability Type | Description | Example | Mitigation Strategy |
---|---|---|---|
Cross-Site Scripting (XSS) | Malicious scripts injected into a website. | User input directly reflected without sanitization. | Input validation and sanitization; output encoding; Content Security Policy (CSP). |
Cross-Site Request Forgery (CSRF) | Tricking users into performing unwanted actions. | Malicious link disguised as a legitimate button. | Using anti-CSRF tokens; verifying HTTP Referer header (with caution); implementing double-submit cookie. |
SQL Injection | Manipulating database queries with malicious input. | User input directly used in SQL queries without parameterization. | Parameterized queries (prepared statements); input validation; using ORM (Object-Relational Mapper) frameworks. |
Session Hijacking | Stealing a user’s session ID to impersonate them. | Intercepting session cookies through insecure communication channels. | HTTPS; secure cookie settings (HttpOnly, Secure flags); regular session timeouts. |
Input Validation and Sanitization
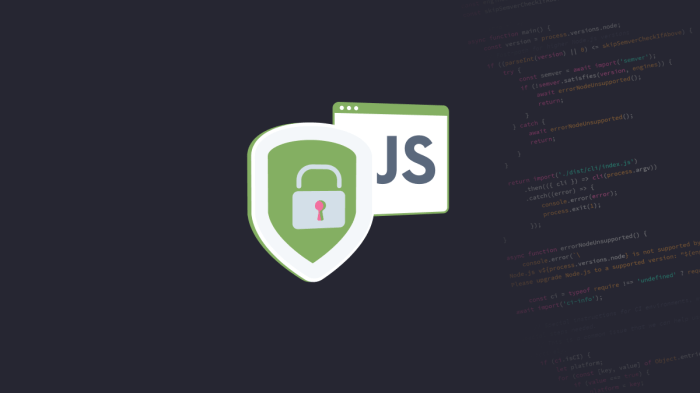
Source: stackdiary.com
Building secure JavaScript applications hinges on effectively handling user input. Untrusted data, if processed carelessly, can open doors to a variety of attacks, from simple display errors to devastating cross-site scripting (XSS) vulnerabilities. This section dives into the crucial practices of input validation and sanitization to protect your application.
Input validation is the process of ensuring that data received from users conforms to the expected format and constraints. Sanitization, on the other hand, is the process of cleaning or transforming data to remove or neutralize potentially harmful elements. Both are essential steps in creating robust security.
Email Address Validation and Injection Prevention
A common scenario involves validating email addresses. A simple regular expression can check for basic formatting, but more robust validation might involve checking the domain’s existence using a DNS lookup (though this adds complexity). The following function provides a basic example:
function isValidEmail(email)
// Basic email validation using a regular expression. This is not exhaustive!
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(email);
This function only checks for a basic structure; a more rigorous approach might use a dedicated email validation library. Crucially, never rely solely on client-side validation. Always perform server-side validation as well to prevent malicious users from bypassing client-side checks. Preventing injection attacks requires careful sanitization, particularly when using user input in database queries or within HTML contexts. Never directly embed user-supplied data into SQL queries or HTML without proper escaping or parameterization.
Data Sanitization Techniques
Several methods exist for sanitizing data depending on its intended use. For HTML contexts, using DOMPurify or similar libraries is recommended to effectively remove or escape potentially harmful HTML tags and attributes. For SQL queries, always use parameterized queries or prepared statements to prevent SQL injection vulnerabilities. This ensures that user input is treated as data, not as executable code. Never directly concatenate user input into SQL strings.
For other contexts, such as handling data in JSON objects or within regular expressions, appropriate escaping or encoding techniques should be used. For example, encoding special characters within JSON objects can prevent issues if the data is later used in a different context.
Best Practices for Handling Untrusted Data
- Principle of Least Privilege: Grant user input only the necessary permissions. Avoid granting excessive access rights.
- Input Validation: Always validate user input against defined rules and constraints, both client-side and server-side.
- Output Encoding: Encode data appropriately based on the context in which it will be used (HTML, SQL, JSON, etc.).
- Sanitization Libraries: Utilize established libraries for sanitization (e.g., DOMPurify for HTML, parameterized queries for SQL).
- Regular Expression Usage: Use regular expressions carefully. Overly complex expressions can be difficult to maintain and may introduce vulnerabilities.
- Defense in Depth: Implement multiple layers of security to mitigate risks. Relying on a single validation or sanitization method is insufficient.
- Security Audits: Regularly conduct security audits and penetration testing to identify and address vulnerabilities.
Remember, treating all user input as potentially malicious is a fundamental principle of secure coding. By consistently applying these techniques, you significantly reduce the risk of vulnerabilities in your JavaScript applications.
Preventing Cross-Site Scripting (XSS) Attacks
Cross-site scripting (XSS) attacks are a serious threat to web applications, allowing attackers to inject malicious scripts into otherwise trusted websites. These scripts can then steal user data, hijack sessions, redirect users to phishing sites, or even deface the website itself. Understanding how XSS works and implementing robust prevention strategies is crucial for building secure JavaScript applications.
XSS attacks exploit vulnerabilities in how websites handle user-supplied data. Attackers inject malicious code into input fields, comments, or other data entry points. When this tainted data is rendered on the page without proper sanitization, the malicious script executes in the context of the victim’s browser, granting the attacker significant control. The impact can range from minor annoyance to complete account takeover, depending on the sophistication of the attack and the sensitivity of the data involved. For example, an attacker might inject a script that steals a user’s session cookie, allowing them to impersonate the user and access their account.
Escaping User Input to Prevent XSS
Preventing XSS hinges on consistently escaping user-supplied data before it’s rendered on the page. This means converting special characters that have meaning in HTML, JavaScript, or URLs into their corresponding HTML entities. For instance, the less-than symbol (<) should be converted to `<`, and the greater-than symbol (>) should be converted to `>`. Failure to escape these characters allows attackers to inject HTML tags and JavaScript code.
Consider a simple example: a comment field on a blog. If a user enters ``, and this is directly rendered on the page without escaping, the alert box will pop up, demonstrating a successful XSS attack. However, if the input is properly escaped, the code will be rendered as plain text, preventing execution. Most modern JavaScript frameworks offer built-in functions for escaping user input, making this process significantly easier. For instance, many frameworks provide methods to automatically escape HTML entities when rendering data to the DOM.
Using Content Security Policy (CSP) to Mitigate XSS Attacks
Content Security Policy (CSP) is a powerful mechanism that allows you to control the resources the browser is allowed to load, reducing the risk of XSS attacks. CSP works by specifying allowed sources for scripts, stylesheets, images, and other resources using HTTP response headers. This prevents the browser from loading resources from untrusted domains, even if an attacker manages to inject a malicious script. A well-configured CSP can significantly limit the impact of a successful XSS attack, even if some input sanitization fails. For example, a CSP directive might restrict script execution to only the application’s origin, preventing the execution of scripts injected by an attacker.
Encoding Techniques for Preventing XSS
Different encoding techniques are required for HTML, JavaScript, and URL parameters. HTML encoding converts special characters into HTML entities, preventing the browser from interpreting them as HTML tags. JavaScript encoding converts special characters into their Unicode escape sequences, preventing them from being interpreted as JavaScript code. URL encoding converts special characters into a format suitable for URLs. It’s crucial to use the correct encoding method for the context in which the data is used. Using the wrong encoding can leave vulnerabilities open. For example, HTML encoding is ineffective against JavaScript injection, and vice-versa. A comprehensive approach requires careful attention to context-specific encoding.
Protecting Against Cross-Site Request Forgery (CSRF) Attacks
Cross-Site Request Forgery (CSRF) attacks are sneaky villains in the world of web security. They exploit the trust a website has in its users’ browsers, tricking users into unknowingly submitting malicious requests. Unlike XSS attacks that inject malicious scripts, CSRF attacks leverage the user’s already-established session to perform unwanted actions. Imagine a malicious website embedding a hidden form that submits a request to your banking site – if you’re logged into your bank at the same time, the attack could transfer funds without your knowledge or consent. Understanding how CSRF works is crucial for building secure JavaScript applications.
CSRF attacks succeed because they rely on the user’s authenticated session. The attacker doesn’t need to know the user’s credentials; they just need to craft a request that looks legitimate to the server. This request could be anything from changing a password to making a purchase. The attack often involves embedding a hidden image or form within a seemingly innocuous webpage, which then automatically submits a request to the vulnerable application. The browser, trusting the user’s session, executes the request without any explicit user interaction beyond visiting the malicious site.
CSRF Protection Mechanisms: Tokens and Synchronization Checks
A robust defense against CSRF attacks involves implementing a double-layered security system: using unique, unpredictable tokens and incorporating synchronization checks. The core principle is to ensure that only requests originating from the legitimate application are processed. This is achieved by embedding a unique, secret token within each form that needs protection. This token is then checked on the server-side before processing the request. Any request lacking the correct token is rejected. Furthermore, synchronization checks involve verifying that the request data hasn’t been tampered with during transmission. This often involves comparing timestamps or other metadata. If inconsistencies are found, the request is considered suspicious and rejected.
The Role of HTTP Methods in CSRF Vulnerability
The HTTP method used (GET or POST) plays a significant role in CSRF vulnerability. GET requests are inherently more susceptible because they can be easily triggered without the user’s direct knowledge, often through embedded images or links within a malicious site. POST requests, while not entirely immune, require a more deliberate action, usually the submission of a form, making them slightly more resistant. However, it’s crucial to remember that even POST requests can be vulnerable if not properly protected with tokens and synchronization checks. Relying solely on the HTTP method for CSRF protection is insufficient.
Implementing CSRF Protection in JavaScript Applications
Implementing CSRF protection in JavaScript applications requires a coordinated effort on both the client-side (JavaScript) and server-side. On the server-side, the application needs to generate and manage unique, unpredictable tokens. These tokens are often stored in the user’s session. On the client-side, JavaScript is used to embed these tokens within forms or AJAX requests. For instance, a hidden input field within a form can contain the token. When the form is submitted, the token is sent along with the other data. The server then verifies the token against the one stored in the user’s session. If they match, the request is considered legitimate; otherwise, it’s rejected. This process is typically handled by server-side frameworks and libraries, but JavaScript plays a vital role in ensuring that the token is properly included in outgoing requests. Additionally, using frameworks and libraries that provide built-in CSRF protection is highly recommended, as they often incorporate best practices and handle many complexities. Failure to implement proper CSRF protection can lead to significant security breaches.
Secure Handling of Sensitive Data
Protecting sensitive data in JavaScript applications is crucial for maintaining user trust and complying with regulations like GDPR and CCPA. Failing to do so can lead to significant breaches and reputational damage. This section Artikels best practices for handling sensitive information, focusing on encryption, secure storage, and transmission.
Client-side encryption in JavaScript, while offering some level of protection, should be considered a supplementary security measure, not a primary defense. The primary security should always be server-side encryption and secure backend systems. Client-side encryption can add an extra layer of protection if the data is compromised before reaching the server, but it’s crucial to remember that determined attackers can still potentially bypass client-side encryption.
Client-Side Encryption Techniques
While JavaScript offers libraries for encryption, it’s essential to understand that client-side encryption is easily reversed if the attacker has access to the client’s machine or the JavaScript code itself. Therefore, focus should always be on robust server-side encryption and security measures. However, for enhancing the security posture, libraries like CryptoJS can be used to perform basic encryption tasks. This involves encrypting sensitive data before it’s transmitted to the server or stored locally. Remember, the encryption key should never be stored alongside the encrypted data in the client. It should be managed securely on the server.
Example using CryptoJS (remember, this is just for illustration and should not be considered a robust security solution):
//Encrypting data using AES
var ciphertext = CryptoJS.AES.encrypt("mysecret", "secretkey").toString();
//Decrypting data
var bytes = CryptoJS.AES.decrypt(ciphertext, "secretkey");
var plaintext = bytes.toString(CryptoJS.enc.Utf8);
Secure Storage and Transmission of Sensitive Data
Storing and transmitting sensitive data requires a multi-layered approach. Simply encrypting data isn’t enough; the entire process, from storage to transmission, must be secure.
For transmission, HTTPS is non-negotiable. HTTPS encrypts the communication between the client and the server, protecting data in transit from eavesdropping. Using secure cookies (with the `Secure` and `HttpOnly` flags) further protects data stored on the client-side. The `Secure` flag ensures cookies are only transmitted over HTTPS, while the `HttpOnly` flag prevents access to the cookie via JavaScript, mitigating XSS vulnerabilities.
Risks of Storing Sensitive Data in Local Storage or Cookies
Browser storage mechanisms like localStorage and cookies offer convenience but pose security risks. If a malicious script gains access to the browser, it can potentially read data from localStorage or cookies, compromising sensitive information. Therefore, avoid storing sensitive data directly in these locations unless absolutely necessary and only after employing strong encryption. Even then, it is highly discouraged.
Using HTTPS and Secure Cookies
HTTPS should be implemented across the entire application. This ensures that all communication between the client and the server is encrypted. Secure cookies should be used for any sensitive data that needs to be stored on the client-side. The `Secure` attribute prevents cookies from being sent over HTTP, and the `HttpOnly` attribute prevents JavaScript from accessing the cookie, reducing the risk of XSS attacks.
Example of setting a secure cookie (server-side implementation is required):
Set-Cookie: sessionid=some_secure_value; Secure; HttpOnly; SameSite=Strict;
The `SameSite=Strict` attribute further enhances security by preventing the cookie from being sent with cross-site requests, mitigating CSRF attacks.
Secure Use of Third-Party Libraries and APIs
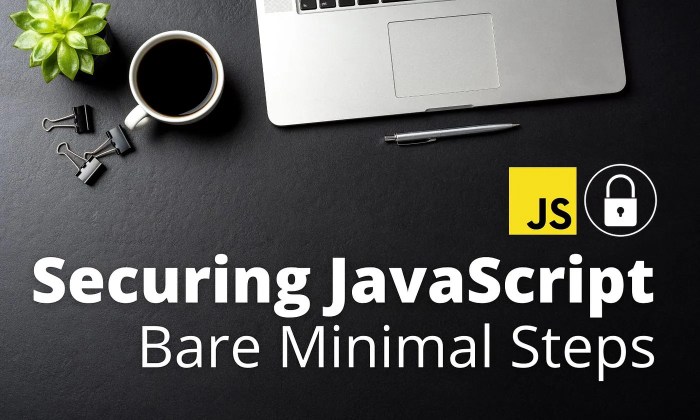
Source: ezitech.org
Modern JavaScript development heavily relies on third-party libraries and APIs to accelerate development and access functionalities. However, this reliance introduces significant security risks if not handled carefully. Integrating insecure or poorly maintained external components can compromise the security of your entire application, potentially exposing sensitive user data or opening vulnerabilities to attacks. Understanding how to vet and securely integrate these external resources is crucial for building robust and secure JavaScript applications.
Securely integrating third-party libraries and APIs involves a multi-faceted approach. It’s not just about grabbing the latest package; it’s about a thorough evaluation of its security posture, responsible integration into your codebase, and ongoing monitoring for updates and potential vulnerabilities. Failing to do so can lead to serious consequences, including data breaches, application crashes, and reputational damage.
Vetting Third-Party Libraries
Before incorporating any third-party library, a thorough vetting process is essential. This involves examining the library’s reputation, security practices, and maintenance status. Consider factors such as the library’s popularity (a widely used library often has more scrutiny and bug fixes), its license (ensuring compatibility with your project’s licensing requirements), and the community’s activity (active maintenance suggests ongoing security updates and support). A library with a large, active community is more likely to have identified and addressed security vulnerabilities promptly. Look for libraries with clear security policies and a documented process for handling reported vulnerabilities. Don’t rely solely on the number of stars on platforms like GitHub; delve deeper into the project’s documentation and history.
Secure Integration of External APIs
Integrating external APIs requires careful consideration of authentication, authorization, and data handling. Always use secure communication protocols like HTTPS to protect data in transit. Implement robust authentication mechanisms to verify the identity of your application when interacting with the API. Never expose API keys or sensitive credentials directly in your client-side code. Instead, utilize server-side proxies or backend services to handle API interactions and protect your secrets. Validate all data received from APIs before using it in your application to prevent injection attacks. Thoroughly review the API’s documentation for security best practices and potential vulnerabilities. Consider rate limiting and error handling to prevent denial-of-service attacks and gracefully handle unexpected responses.
Risks of Outdated or Unmaintained Libraries
Using outdated or unmaintained libraries presents significant security risks. These libraries may contain known vulnerabilities that have not been patched, leaving your application susceptible to attacks. Outdated libraries might also lack compatibility with modern browsers and security standards, increasing the likelihood of exploits. Regularly check for updates and promptly update your libraries to benefit from security patches and bug fixes. Set up automated dependency updates to streamline the process and minimize the window of vulnerability. Monitor security advisories and vulnerability databases for known issues related to your libraries. If a library is no longer maintained, consider replacing it with a well-maintained alternative to mitigate risks.
Checklist for Evaluating Third-Party JavaScript Libraries
Prioritizing security when selecting third-party libraries is crucial. Here’s a checklist to help you evaluate their security:
This checklist provides a structured approach to evaluating the security of third-party JavaScript libraries. Remember that even with careful evaluation, continuous monitoring and updates are necessary to maintain a secure application.
- Reputation and Community: Is the library widely used and well-regarded? Does it have an active and responsive community?
- Maintenance and Updates: Is the library actively maintained? How frequently are updates released? Are security updates promptly addressed?
- Security Practices: Does the library have a documented security policy? Has it undergone security audits?
- License Compatibility: Is the library’s license compatible with your project’s requirements?
- Vulnerability History: Check for any known vulnerabilities or security advisories related to the library.
- Code Quality: Is the code well-written, documented, and easy to understand? This can help identify potential security flaws.
- Dependencies: Review the library’s dependencies to ensure they are also secure and well-maintained.
Secure Authentication and Authorization
Securing your JavaScript application involves more than just preventing bad actors from accessing your code; it’s about controlling who can do what within your application. This means implementing robust authentication and authorization mechanisms to verify user identity and manage access permissions. This section delves into the specifics of these crucial security components.
Authentication verifies the identity of a user, while authorization determines what actions a user is permitted to perform. These are distinct but interconnected processes. A user might authenticate successfully, but still lack the authorization to access specific resources or functionality. We’ll explore various methods for implementing both, focusing on their strengths and weaknesses in a JavaScript context.
OAuth 2.0 and JWT for Secure Authentication
OAuth 2.0 is an authorization framework, not an authentication protocol itself. It delegates authentication to an authorization server, allowing your application to access protected resources on behalf of a user without directly handling their credentials. This reduces the risk of credential compromise. JSON Web Tokens (JWTs) are commonly used to represent the authorization granted by OAuth 2.0. A JWT is a compact, URL-safe method for representing claims securely between two parties. These claims often include user information and access permissions. The typical OAuth 2.0 flow involves a user authenticating with an authorization server (like Google or Facebook), receiving an authorization code, and then exchanging that code for an access token (often a JWT) which your application uses to access protected resources. This architecture minimizes the risk of your application needing to directly manage user passwords.
Authorization Mechanisms in JavaScript Applications
Implementing authorization effectively involves carefully controlling access to resources based on a user’s identity and roles. This is often achieved through role-based access control (RBAC). In RBAC, users are assigned to roles (e.g., “admin,” “editor,” “viewer”), and each role has associated permissions. Your application then checks if a user has the necessary permissions before allowing access to a specific resource or function. This can be implemented using various techniques, including server-side checks (for enhanced security) and client-side checks (for a better user experience, but requiring careful consideration to avoid bypassing security). Client-side checks should always be complemented by robust server-side validation. Another approach is attribute-based access control (ABAC), which allows for more granular control based on various attributes, like user location, device, or time of day.
Secure Authentication Flow using Passport.js
Passport.js is a popular Node.js authentication middleware. A secure authentication flow using Passport.js might involve these steps: 1. The user attempts to log in, providing their credentials. 2. Passport.js uses a strategy (e.g., a local strategy for username/password, or an OAuth 2.0 strategy for third-party authentication) to verify the credentials. 3. Upon successful authentication, Passport.js serializes the user’s information and stores it in a session. 4. Subsequent requests include a session identifier, allowing Passport.js to deserialize the user’s information and grant access to authorized resources. The crucial element is that sensitive information like passwords is never directly handled by the frontend JavaScript code. The entire authentication process occurs server-side. This minimizes the risk of credential theft through client-side attacks.
Comparison of Authentication and Authorization Mechanisms
The choice of authentication and authorization mechanisms depends on the specific needs of your application. OAuth 2.0 with JWTs offers strong security and scalability, but can be more complex to implement than simpler username/password authentication. RBAC is widely used for its simplicity and ease of management, while ABAC provides greater flexibility but might require more complex configuration. A well-designed system might leverage a combination of these approaches. For instance, you might use OAuth 2.0 for authentication and RBAC for authorization. The key is to choose a system that balances security needs with development effort and maintainability. Overly complex systems can introduce vulnerabilities if not properly implemented and maintained.
Secure Session Management
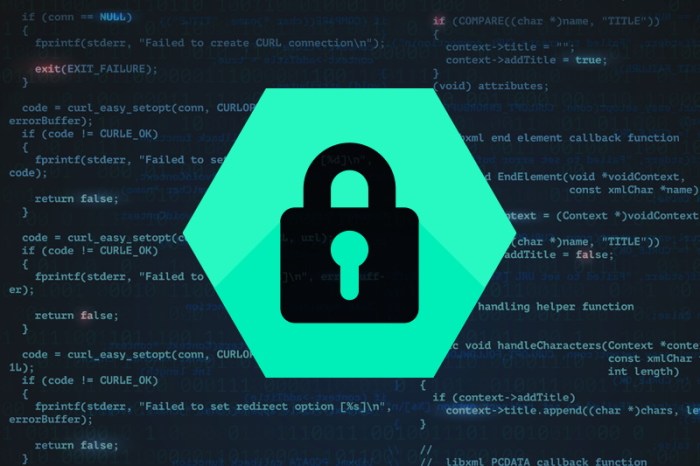
Source: skillreactor.io
Securing your JavaScript code is crucial, especially when handling sensitive data. Just like choosing the right insurance plan requires careful consideration, selecting the best security practices is vital. Understanding your options is key; for instance, exploring different insurance providers can help you make an informed decision, much like researching various security libraries. Learn more about finding the perfect fit by checking out The Benefits of Using an Independent Insurance Agent , then return to focusing on those essential JavaScript security measures.
In the wild world of web applications, secure session management is like Fort Knox for your user data. A poorly managed session is an open invitation for hackers to wreak havoc, potentially exposing sensitive information and compromising user accounts. This section dives into the crucial best practices for keeping your JavaScript application’s sessions safe and sound.
Protecting user sessions requires a multi-layered approach. It’s not just about generating a unique identifier; it’s about how you handle, store, and ultimately, destroy that identifier. We’ll cover techniques to mitigate common attacks and ensure a robust, secure session experience for your users.
Secure Cookie Implementation
Using HTTP-only and Secure flags for your session cookies is non-negotiable. The `HttpOnly` flag prevents client-side JavaScript from accessing the cookie, significantly reducing the risk of XSS attacks that could steal the session ID. The `Secure` flag ensures the cookie is only transmitted over HTTPS, preventing eavesdropping on insecure connections. These flags are essential building blocks for a secure session management strategy. Failing to implement them leaves your application vulnerable to various attacks. For example, without the `HttpOnly` flag, a malicious script could easily read the session cookie and impersonate the user.
Session Timeouts and Automatic Logout
Implementing session timeouts and automatic logout functionality is crucial for limiting the impact of compromised sessions. Setting a reasonable timeout period, say 30 minutes or an hour of inactivity, forces users to re-authenticate, minimizing the window of opportunity for attackers. The automatic logout feature should gracefully terminate the session, clearing any sensitive data stored client-side and redirecting the user to a login page. Consider allowing users to configure their preferred timeout period to improve the user experience while maintaining security. For instance, a banking application might have shorter timeouts than a social media platform.
Session Hijacking Mitigation
Session hijacking is a serious threat where an attacker gains unauthorized access to a user’s session. Robust strategies are needed to defend against this. Using strong, unpredictable session IDs is paramount; avoid predictable patterns or sequential numbering. Regularly rotating session IDs adds another layer of protection. Furthermore, implementing mechanisms to detect unusual activity, such as login attempts from unexpected locations or a sudden surge in requests from a single session, can help identify and mitigate hijacking attempts. Alerting users to suspicious activity, perhaps through a secondary verification step, can also greatly improve security. For example, flagging login attempts from a new IP address and prompting the user to verify the login via email or SMS.
Regular Security Audits and Updates: How To Write Secure Code In JavaScript
Building secure JavaScript applications isn’t a one-time fix; it’s an ongoing process. Think of it like regular check-ups for your health – neglecting them can lead to serious problems down the line. Regular security audits and updates are crucial for identifying and patching vulnerabilities before they can be exploited by malicious actors. Ignoring this aspect leaves your application vulnerable to attacks, potentially leading to data breaches, financial losses, and reputational damage.
Regular security audits and code reviews are essential for maintaining the security posture of your JavaScript applications. These processes allow for the proactive identification and mitigation of vulnerabilities, preventing potential security breaches before they occur. Essentially, you’re catching problems before they become major headaches.
Security Audit Tools and Techniques
Effective security audits involve a combination of automated tools and manual code reviews. Automated tools can scan your codebase for common vulnerabilities, while manual reviews provide a deeper level of analysis and can uncover more subtle issues. This two-pronged approach offers a comprehensive security assessment.
Examples of automated tools include static analysis tools like ESLint with security plugins, and dynamic analysis tools like penetration testing frameworks. These tools can flag potential issues such as insecure coding practices, SQL injection vulnerabilities, and cross-site scripting (XSS) flaws. Manual code reviews, often performed by experienced security professionals, involve a careful examination of the codebase to identify vulnerabilities that might be missed by automated tools. This process frequently involves code walkthroughs and discussions about potential security implications.
JavaScript Library and Dependency Updates
Keeping your JavaScript libraries and dependencies up-to-date is paramount. Outdated libraries often contain known security vulnerabilities that have been patched in newer versions. Failing to update exposes your application to potential exploits. Think of it like leaving your front door unlocked – you’re inviting trouble.
A robust update strategy involves regularly checking for updates using tools like npm outdated or yarn outdated. These commands list all outdated packages and their latest versions, enabling you to prioritize updates based on the severity of the vulnerabilities. Integrating automated dependency update tools into your CI/CD pipeline helps to streamline the process and ensure that updates are applied consistently and efficiently.
Incorporating Security Updates into the Development Workflow, How to Write Secure Code in JavaScript
Integrating security updates into your development workflow requires a well-defined plan. This involves establishing clear processes for identifying, testing, and deploying security updates. Regular security assessments, automated testing, and a robust update management system are key components of this plan.
A practical approach involves scheduling regular security assessments as part of the sprint cycle. Automated tests, including unit tests, integration tests, and security-specific tests, should be incorporated into the CI/CD pipeline to verify the security of each update before deployment. Implementing a well-defined rollback plan is also crucial to mitigate any unforeseen issues arising from security updates. This systematic approach minimizes disruption and ensures the security of your application throughout its lifecycle.
Summary
Writing secure JavaScript code isn’t about adding a few extra lines of defense; it’s about building security into the very fabric of your application. By understanding common vulnerabilities and implementing robust mitigation strategies, you can create applications that are resistant to attacks and protect your users’ data. Remember, vigilance is key – stay updated on the latest threats and best practices, and continuously review your code. Secure coding isn’t a destination, it’s a journey. Let’s build a safer internet, one line of code at a time.