A Guide to Building APIs with Flask in Python: Dive into the world of Python API development with this comprehensive guide. We’ll walk you through setting up your environment, crafting robust endpoints, handling data with JSON, integrating databases, and implementing secure authentication. Get ready to build powerful and scalable APIs with Flask, from the basics to advanced techniques like asynchronous tasks and API versioning. This isn’t just another tutorial; it’s your roadmap to mastering Flask API development.
This guide covers everything from setting up your Flask development environment and designing your first “Hello, World!” API to implementing advanced features like authentication, database integration, and robust error handling. We’ll explore different HTTP methods, JSON handling, and various database options. You’ll also learn about testing strategies, deployment options, and best practices for building production-ready APIs. Whether you’re a beginner or have some experience, this guide will equip you with the knowledge and skills to build impressive Flask APIs.
Introduction to Flask and API Development
Flask, a micro web framework written in Python, is a fantastic choice for building APIs. Its lightweight nature and flexibility make it ideal for both small-scale projects and larger, more complex applications. Unlike heavier frameworks, Flask doesn’t force you into a specific project structure or impose unnecessary dependencies, giving you the freedom to tailor your API to your exact needs. This translates to faster development times and easier maintenance.
Flask’s simplicity doesn’t mean a lack of power; it offers robust features and a thriving community, providing ample resources and support. This makes it an excellent option for developers of all skill levels, from beginners taking their first steps in API development to seasoned professionals building sophisticated systems.
Setting up a Flask Development Environment
Setting up your Flask development environment is straightforward. First, ensure you have Python installed. Then, use pip, Python’s package installer, to install Flask: pip install Flask
. That’s it! You can then create a simple Python file (e.g., `app.py`) and start coding your API. For a more structured approach, consider using a virtual environment (recommended) to isolate your project’s dependencies. You can create one using python3 -m venv .venv
and activate it with source .venv/bin/activate
(on Linux/macOS) or .venv\Scripts\activate
(on Windows). This keeps your project’s dependencies separate from your system’s Python installation, preventing conflicts.
Flask API Project Structure Best Practices
A well-structured project is key to maintainability and scalability. Consider organizing your project with separate folders for your API logic, models (if using a database), tests, and configuration files. This approach promotes clarity and makes it easier for you and others to understand and navigate the codebase. For example, you might have a `models` folder for database interaction code, an `api` folder for your Flask routes and controllers, a `tests` folder for unit and integration tests, and a `config` folder for settings like database credentials. This modular design also facilitates collaboration and future expansion.
Designing a Simple “Hello, World!” Flask API, A Guide to Building APIs with Flask in Python
Let’s build a basic “Hello, World!” API to demonstrate Flask’s core functionality. Create a file named `app.py` and add the following code:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(“/”)
def hello_world():
return “Hello, World!”
if __name__ == “__main__”:
app.run(debug=True)
“`
This code creates a Flask application, defines a route (“/”) that responds with “Hello, World!”, and then runs the application in debug mode (helpful for development). To run this, navigate to the directory containing `app.py` in your terminal and execute `python app.py`. You can then access your API by visiting `http://127.0.0.1:5000/` in your web browser. The debug mode automatically reloads the server whenever you make changes to your code, making the development process much smoother. Remember to disable debug mode in a production environment.
Defining API Endpoints and HTTP Methods
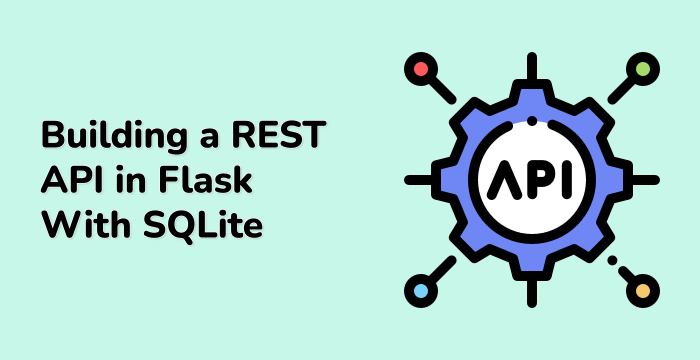
Source: appbot.io
Building APIs with Flask involves defining endpoints—specific URLs that your API responds to—and associating them with HTTP methods that dictate how the client interacts with those endpoints. Think of it like a restaurant menu: the endpoints are the dishes, and the HTTP methods are how you order (GET a menu, POST an order, PUT in a special request, DELETE an item). Understanding this is crucial for creating a well-structured and functional API.
HTTP Methods and Their Uses
HTTP methods define the type of operation a client wants to perform on a specific resource. They provide a standardized way for clients and servers to communicate. The most common methods are GET, POST, PUT, and DELETE, each serving a distinct purpose. Using the right method is vital for API clarity and maintainability.
Method | Purpose | Typical Use Cases | Example |
---|---|---|---|
GET | Retrieve data from the server. This is an idempotent operation, meaning multiple requests have the same effect as one. | Fetching a list of users, getting details of a specific product. | Retrieving a user’s profile: GET /users/123 |
POST | Send data to the server to create or update a resource. Not idempotent; each request creates a new resource or updates existing one. | Creating a new user account, submitting a form, sending a message. | Creating a new user: POST /users (with user data in the request body) |
PUT | Update an existing resource on the server. Idempotent; multiple requests result in the same final state. | Updating user information, modifying a product’s details. | Updating a user’s profile: PUT /users/123 (with updated user data) |
DELETE | Delete a resource from the server. Idempotent; multiple requests have the same effect as one. | Deleting a user account, removing a product from a catalog. | Deleting a user: DELETE /users/123 |
Defining API Endpoints with Flask
Flask’s routing mechanism uses the `@app.route` decorator to map URLs to specific functions. This decorator allows you to define which HTTP methods each endpoint supports. For instance, you can specify that a particular endpoint only accepts GET requests or handle both GET and POST requests differently.
Handling Requests and Returning Responses
When a client sends a request to an endpoint, Flask’s routing system directs it to the corresponding function. This function then processes the request, performs necessary actions (e.g., database queries), and constructs a response to send back to the client. The response typically includes data in a format like JSON.
Flask Code Examples
Let’s illustrate this with some simple Flask code snippets. Assume we’re building a basic API for managing to-do items.
“`python
from flask import Flask, request, jsonify
app = Flask(__name__)
todos = []
@app.route(‘/todos’, methods=[‘GET’, ‘POST’])
def handle_todos():
if request.method == ‘GET’:
return jsonify(todos)
elif request.method == ‘POST’:
new_todo = request.get_json()
todos.append(new_todo)
return jsonify(‘message’: ‘Todo added’), 201
@app.route(‘/todos/
def handle_todo(todo_id):
todo = next((todo for todo in todos if todo.get(‘id’) == todo_id), None)
if request.method == ‘GET’:
if todo:
return jsonify(todo)
else:
return jsonify(‘message’: ‘Todo not found’), 404
elif request.method == ‘PUT’:
updated_todo = request.get_json()
todos[todos.index(todo)] = updated_todo
return jsonify(‘message’: ‘Todo updated’)
elif request.method == ‘DELETE’:
todos.remove(todo)
return jsonify(‘message’: ‘Todo deleted’)
if __name__ == ‘__main__’:
app.run(debug=True)
“`
This example shows how to handle GET, POST, PUT, and DELETE requests using different endpoints and methods. Remember to install Flask using `pip install Flask`. This provides a functional, albeit simple, API foundation. More sophisticated error handling and data validation would be needed in a production environment.
Handling Data with Flask and JSON
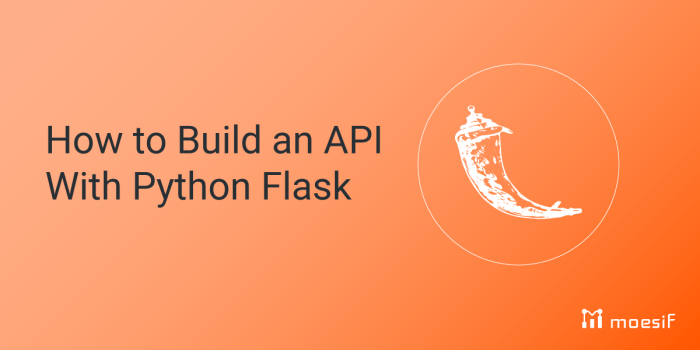
Source: moesif.com
So, you’ve built your awesome Flask API, but now you need to deal with the nitty-gritty of data – specifically, JSON. JSON (JavaScript Object Notation) is the lingua franca of the web API world, a lightweight format perfect for exchanging data between your Flask application and other systems. This section dives into the essential techniques for mastering JSON within your Flask projects.
JSON’s human-readable nature makes it a breeze to work with, but understanding how to efficiently parse incoming requests and craft outgoing responses is crucial for building robust and scalable APIs. We’ll cover everything from basic parsing to advanced validation techniques, even throwing in some handy Flask extensions to streamline the process.
Parsing JSON Requests
Flask makes receiving JSON data surprisingly straightforward. Incoming requests often contain JSON payloads, and Flask provides tools to easily access this data. The `request.get_json()` method is your go-to function for extracting JSON data from an incoming request. Let’s imagine a scenario where a client sends a JSON payload containing user details: `”name”: “Alice”, “age”: 30`. Here’s how you’d handle it in your Flask route:
“`python
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(‘/users’, methods=[‘POST’])
def create_user():
user_data = request.get_json()
if user_data and ‘name’ in user_data and ‘age’ in user_data:
name = user_data[‘name’]
age = user_data[‘age’]
# Process user data (e.g., save to database)
return jsonify(“message”: f”User name created successfully!”), 201
else:
return jsonify(“error”: “Invalid JSON payload”), 400
if __name__ == ‘__main__’:
app.run(debug=True)
“`
This snippet checks if the request contains valid JSON and the necessary keys (‘name’ and ‘age’) before processing the data. The `jsonify` function conveniently converts Python dictionaries into JSON responses.
Generating JSON Responses
Creating JSON responses is equally simple. The `jsonify` function, as shown above, handles the conversion from a Python dictionary to a JSON string. This ensures your API returns data in a format easily consumed by other applications. Remember to include the appropriate HTTP status code to indicate the success or failure of the request (e.g., 200 OK, 201 Created, 400 Bad Request, etc.).
Validating JSON Data
Validating JSON data is paramount for security and data integrity. While `request.get_json()` handles basic parsing, you’ll likely need more robust validation. This can involve checking data types, lengths, formats, and ensuring the presence of required fields. Python’s built-in `jsonschema` library provides a powerful way to define and validate JSON schemas.
For instance, you might define a schema like this:
“`json
“type”: “object”,
“properties”:
“name”: “type”: “string”,
“age”: “type”: “integer”, “minimum”: 0
,
“required”: [“name”, “age”]
“`
Then, you can use `jsonschema` to validate your incoming JSON against this schema before processing it.
Using Flask Extensions for JSON Handling
While you can handle JSON manually, Flask extensions like `marshmallow` can significantly simplify the process. Marshmallow allows you to define schemas that map between Python objects and JSON, providing validation and serialization/deserialization capabilities. This reduces boilerplate code and enhances the maintainability of your API. Using Marshmallow often results in cleaner, more readable, and less error-prone code. It handles the intricacies of data transformation and validation, freeing you to focus on the core logic of your API.
Database Integration with Flask APIs
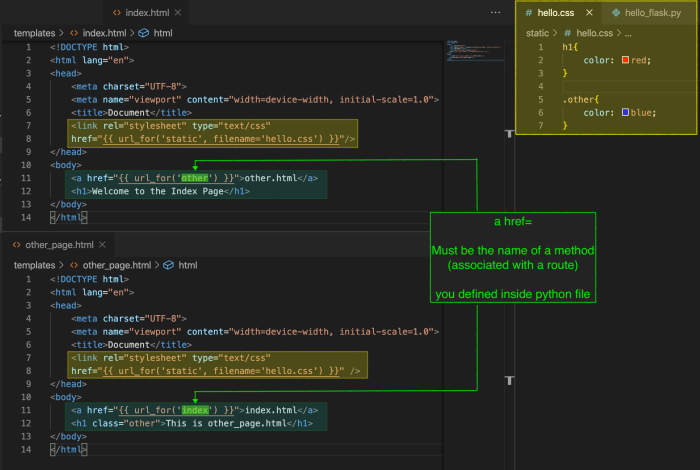
Source: medium.com
So you’re diving into the world of building APIs with Flask in Python – awesome! It’s all about clean, efficient code, right? Think about the meticulous process involved, much like the detailed work of an insurance adjuster investigating a claim, as described in this helpful guide: What is the Role of an Insurance Adjuster in Claims?.
Back to Flask, remember to handle errors gracefully – just as a good adjuster handles a complex claim. Efficient API design is key!
So you’ve built your awesome Flask API, but it’s currently living a pretty lonely existence, confined to RAM. Time to give it a home – a database! Connecting your API to a database lets you persist data, making your app truly dynamic and scalable. This section dives into how to seamlessly integrate databases into your Flask projects, turning them from ephemeral wonders into robust, data-driven powerhouses.
Choosing the right database is crucial, and it depends heavily on your project’s needs and scale. We’ll explore some popular choices and guide you through the integration process.
Database Options for Flask APIs
Several database management systems (DBMS) are readily compatible with Flask. The best choice depends on factors like project size, complexity, and scalability requirements. Here’s a breakdown of some common options:
- SQLite: A lightweight, file-based database ideal for smaller projects or prototypes. It’s incredibly easy to set up and integrate, making it perfect for learning and quick development. However, it’s not suitable for large-scale applications or concurrent access from multiple users.
- PostgreSQL: A powerful, open-source relational database known for its robustness, scalability, and advanced features. It’s a great choice for complex applications requiring high performance and data integrity. It’s more involved to set up than SQLite but offers significant advantages for larger projects.
- MySQL: Another popular open-source relational database known for its speed and ease of use. It’s a good middle ground between SQLite and PostgreSQL, offering a balance between simplicity and scalability. It’s widely used and well-documented.
Integrating a Database with a Flask API (using SQLAlchemy and SQLite)
We’ll use SQLAlchemy, a powerful Object-Relational Mapper (ORM), to simplify database interactions. SQLAlchemy acts as an intermediary, allowing you to interact with your database using Python objects instead of writing raw SQL queries. This makes your code cleaner, more maintainable, and less prone to errors. Let’s use SQLite for simplicity.
First, install SQLAlchemy: pip install SQLAlchemy
Next, let’s create a simple Flask app that manages blog posts. We’ll define a model for a blog post, create a database, and add some endpoints to interact with it.
“`python
from flask import Flask, jsonify, request
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config[‘SQLALCHEMY_DATABASE_URI’] = ‘sqlite:///blog.db’ # Connects to a SQLite database file named ‘blog.db’
db = SQLAlchemy(app)
class BlogPost(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
content = db.Column(db.Text, nullable=False)
def to_dict(self):
return
‘id’: self.id,
‘title’: self.title,
‘content’: self.content
with app.app_context():
db.create_all() # Creates the database tables
@app.route(‘/posts’, methods=[‘GET’])
def get_posts():
posts = BlogPost.query.all()
return jsonify([post.to_dict() for post in posts])
@app.route(‘/posts’, methods=[‘POST’])
def create_post():
data = request.get_json()
new_post = BlogPost(title=data[‘title’], content=data[‘content’])
db.session.add(new_post)
db.session.commit()
return jsonify(‘message’: ‘Post created’), 201
if __name__ == ‘__main__’:
app.run(debug=True)
“`
This code defines a `BlogPost` model, creates the database table, and provides endpoints to retrieve and create blog posts. Remember to replace ‘sqlite:///blog.db’ with your database URI if you are using a different database.
Best Practices for Database Interaction
Efficient and secure database interaction is paramount for API performance and data integrity. Here are some key best practices:
- Use an ORM: ORMs like SQLAlchemy abstract away the complexities of SQL, making your code more readable and maintainable.
- Parameterize Queries: Always use parameterized queries to prevent SQL injection vulnerabilities. This is crucial for security.
- Transactions: Wrap database operations within transactions to ensure data consistency. If any part of a transaction fails, the entire operation is rolled back.
- Connection Pooling: Use connection pooling to reuse database connections, reducing the overhead of establishing new connections for each request.
- Optimize Queries: Write efficient SQL queries to minimize database load and improve API response times. Use indexes appropriately.
Implementing API Authentication and Authorization
Building a robust API isn’t just about crafting elegant endpoints; it’s about safeguarding your data. Authentication and authorization are the cornerstones of secure API design, ensuring only authorized users access specific resources. This section dives into the practical implementation of these crucial security measures within your Flask application.
API Key Authentication
API keys are a simple yet effective authentication method. They involve generating unique keys for each user or application, which are then included in the request headers to verify identity. This approach is suitable for situations where a high level of security isn’t strictly required, such as internal APIs or applications with less sensitive data. Implementing API key authentication in Flask involves verifying the provided key against a database or a list of valid keys. A successful verification grants access to the requested resources. For example, you might store API keys in a database along with user details and use them to identify users accessing the API.
OAuth 2.0 Authentication
OAuth 2.0 is a widely used authorization framework that allows applications to access user resources without directly handling user credentials. Unlike API keys, which are tied to a specific application, OAuth 2.0 leverages access tokens, providing more granular control over access rights and facilitating the management of user permissions across multiple applications. This approach is ideal for APIs that need to interact with external services or handle sensitive user data, providing a more robust security posture than API key authentication. Flask extensions such as `flask-oauthlib` simplify the integration of OAuth 2.0.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a powerful authorization mechanism that defines permissions based on user roles. Instead of assigning permissions directly to individual users, RBAC groups users into roles (e.g., “admin,” “editor,” “viewer”), each with a specific set of permissions. This simplifies user management and improves security by centralizing permission control. In a Flask API, you can implement RBAC by associating roles with users in your database and then checking the user’s role before granting access to specific endpoints. For example, an “admin” role might have full access, while a “viewer” role might only be able to read data. This can be enforced using decorators or custom middleware.
Designing a Secure Flask API
Designing a secure Flask API involves a layered approach, combining authentication and authorization mechanisms. A typical implementation might use API keys for less sensitive operations and OAuth 2.0 for more critical ones, supplemented by RBAC for granular permission management. Consider using HTTPS to encrypt communication, input validation to prevent injection attacks, and robust error handling to prevent information leakage. Regular security audits and updates are essential to maintain a secure API environment. Remember to choose authentication and authorization methods appropriate for the sensitivity of your data and the needs of your application. Over-engineering security can lead to unnecessary complexity, while under-engineering it can leave your API vulnerable.
Testing and Debugging Flask APIs: A Guide To Building APIs With Flask In Python
Building robust and reliable APIs requires a solid testing strategy. Ignoring testing can lead to frustrating debugging sessions and ultimately, a less-than-stellar user experience. This section dives into various testing methodologies and debugging techniques crucial for ensuring your Flask APIs are production-ready. We’ll cover both unit and integration tests, offering practical examples to guide you through the process.
Unit Tests for API Endpoints
Unit tests focus on individual components of your application, ensuring each part functions correctly in isolation. For Flask APIs, this means testing individual endpoints to verify they handle requests and return the expected responses. The `unittest` module in Python, or a more advanced framework like `pytest`, provides the tools for creating these tests.
Here’s a simple example using `unittest` to test a Flask endpoint that returns a “Hello, World!” message:
“`python
import unittest
from your_app import app # Replace your_app with your app’s name
class TestAPI(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
def test_hello_world(self):
response = self.app.get(‘/hello’)
self.assertEqual(response.status_code, 200)
self.assertEqual(response.data.decode(), ‘Hello, World!’)
if __name__ == ‘__main__’:
unittest.main()
“`
This test creates a test client, makes a GET request to the `/hello` endpoint, and asserts that the status code is 200 (OK) and the response data matches the expected string. Remember to replace `your_app` with the actual name of your Flask application file. More complex endpoints might involve testing different HTTP methods (POST, PUT, DELETE), handling various input parameters, and verifying the accuracy of the returned JSON data.
Integration Tests for Flask APIs
Integration tests go a step further than unit tests by testing the interaction between different parts of your application. For Flask APIs, this might involve testing the interaction between your API endpoints and your database, or between your API and external services. These tests ensure that different components work together seamlessly. Again, `unittest` or `pytest` can be used for these tests, but you might need to set up a test database or mock external services.
Consider an endpoint that retrieves data from a database. An integration test would verify that the endpoint correctly connects to the database, retrieves the expected data, and returns it in the correct format. This test would involve setting up a test database, populating it with sample data, and then making requests to the endpoint to verify the results.
Debugging Common Issues in Flask APIs
Debugging Flask APIs often involves carefully examining logs, using a debugger, and understanding HTTP status codes. Flask’s built-in logging capabilities are invaluable for tracking errors and tracing the flow of execution. Using a debugger like pdb (Python Debugger) allows you to step through your code line by line, inspect variables, and identify the root cause of issues. Furthermore, understanding HTTP status codes is crucial for interpreting responses from your API and identifying potential problems. A 500 Internal Server Error, for example, indicates a server-side problem, while a 404 Not Found indicates a missing resource.
Example of Testing a Simple Flask API Endpoint
Let’s consider a simple Flask API endpoint that adds two numbers:
“`python
from flask import Flask, jsonify, request
app = Flask(__name__)
@app.route(‘/add’, methods=[‘POST’])
def add_numbers():
data = request.get_json()
try:
num1 = data[‘num1’]
num2 = data[‘num2’]
result = num1 + num2
return jsonify(‘result’: result)
except KeyError:
return jsonify(‘error’: ‘Missing parameters’), 400
except TypeError:
return jsonify(‘error’: ‘Invalid parameters’), 400
if __name__ == ‘__main__’:
app.run(debug=True)
“`
A comprehensive test for this endpoint might look like this (using `pytest` this time):
“`python
import pytest
import requests
def test_add_numbers_success():
url = ‘http://127.0.0.1:5000/add’ # Replace with your app’s URL
data = ‘num1’: 10, ‘num2’: 5
response = requests.post(url, json=data)
assert response.status_code == 200
assert response.json() == ‘result’: 15
def test_add_numbers_missing_param():
url = ‘http://127.0.0.1:5000/add’
data = ‘num1’: 10
response = requests.post(url, json=data)
assert response.status_code == 400
assert response.json() == ‘error’: ‘Missing parameters’
def test_add_numbers_invalid_param():
url = ‘http://127.0.0.1:5000/add’
data = ‘num1’: ‘abc’, ‘num2’: 5
response = requests.post(url, json=data)
assert response.status_code == 400
assert response.json() == ‘error’: ‘Invalid parameters’
“`
This example demonstrates testing different scenarios: successful addition, missing parameters, and invalid parameters. Remember to run your Flask app before executing these tests.
Deploying Flask APIs
Getting your Flask API live and accessible to the world is the ultimate goal. Deployment takes your meticulously crafted code from your local machine to a server where users can interact with it. This involves choosing the right platform, configuring your environment, and ensuring smooth operation. Let’s explore the options and best practices.
Deployment Platforms
Choosing a deployment platform depends on your project’s needs and your technical expertise. Popular choices include Heroku, Amazon Web Services (AWS), and Google Cloud Platform (GCP). Each offers different strengths in terms of cost, ease of use, and scalability.
Deploying to Heroku: A Step-by-Step Guide
Heroku is a popular Platform as a Service (PaaS) known for its ease of use, especially for beginners. It abstracts away much of the server management complexity.
- Create a Heroku Account: Sign up for a free Heroku account if you don’t already have one.
- Install the Heroku CLI: Download and install the Heroku Command Line Interface (CLI) to interact with Heroku from your terminal.
- Create a Procfile: This file tells Heroku how to run your application. Create a file named `Procfile` (no extension) in your project’s root directory with the following content:
web: gunicorn app:app
(assuming your Flask app is named `app` and is in a file named `app.py`). - Create a Requirements.txt: List all your project’s dependencies in a `requirements.txt` file using
pip freeze > requirements.txt
. - Initialize a Git Repository: If you haven’t already, initialize a Git repository in your project directory using
git init
. - Commit and Push to Heroku: Create a Heroku app using
heroku create
. Then, push your code to Heroku:git add .
,git commit -m "Deploying to Heroku"
, andgit push heroku main
(or your main branch name). - Open Your App: Once the deployment is complete, Heroku will provide a URL to access your deployed API. You can open it in your browser.
Best Practices for Production Deployment
Deploying to production is more than just getting your code online. Several best practices ensure stability, security, and scalability.
- Use a Virtual Environment: Isolate your project’s dependencies to prevent conflicts.
- Implement Proper Logging: Track errors and events for debugging and monitoring.
- Use a Process Manager: Gunicorn or uWSGI manage multiple worker processes for better performance and resilience.
- Implement Error Handling: Gracefully handle exceptions and return informative error messages.
- Use a Reverse Proxy: Nginx or Apache can act as a reverse proxy, handling static assets and improving security.
- Monitor Your Application: Regularly monitor performance and resource usage to identify and address issues proactively. Tools like Datadog or New Relic can help.
Comparison of Deployment Platforms
The choice of deployment platform is crucial. Here’s a comparison:
Platform | Cost | Ease of Use | Scalability |
---|---|---|---|
Heroku | Free tier available, pay-as-you-go for larger applications | Easy to use, especially for beginners | Good scalability options, but can become expensive at higher scales |
AWS (e.g., EC2, Elastic Beanstalk) | Variable, depending on resource usage | More complex than Heroku, requires more technical expertise | Highly scalable and flexible |
Google Cloud Platform (GCP) (e.g., Compute Engine, App Engine) | Variable, depending on resource usage | Similar complexity to AWS, requires technical expertise | Highly scalable and flexible, offers strong integration with other Google services |
Advanced Flask API Techniques
Level up your Flask API game! Building a robust and scalable API requires more than just the basics. This section dives into advanced techniques that will transform your Flask projects from simple scripts to sophisticated, production-ready applications. We’ll explore powerful extensions, smart versioning strategies, and the magic of asynchronous tasks.
Flask Extensions: Supercharging Your API
Flask’s extensibility is a key strength. Extensions provide pre-built functionality, saving you time and effort. Marshmallow, for instance, is a game-changer for serialization and deserialization of data. It allows you to define schemas that map your Python objects to JSON, handling data validation and transformation effortlessly. This simplifies data exchange between your API and clients, ensuring consistency and preventing errors. Using Marshmallow means cleaner, more maintainable code and less headache debugging data inconsistencies. Imagine defining a User schema with fields like `id`, `username`, and `email`. Marshmallow will handle converting a User object to a JSON representation and vice-versa, validating data types along the way.
API Versioning Strategies
As your API evolves, managing different versions becomes crucial. Inconsistent API versions can lead to compatibility issues and break existing integrations. Several strategies exist, including URI versioning (e.g., `/v1/users`, `/v2/users`), header-based versioning (using a custom header like `X-API-Version`), and content negotiation (using the `Accept` header). Choosing the right strategy depends on your specific needs and how you anticipate your API evolving. URI versioning is often preferred for its simplicity and clarity, while header-based versioning offers more flexibility. Consider the long-term implications of your choice; a well-planned versioning strategy prevents future headaches.
Asynchronous Tasks with Flask and Celery
Long-running tasks, such as sending emails or processing large datasets, can block your API’s responsiveness. Celery, a distributed task queue, offers a solution. It allows you to offload these tasks to background workers, keeping your API fast and responsive. Imagine a scenario where your API needs to process a large image upload. Instead of blocking the main thread, you can use Celery to enqueue the image processing task. The API immediately responds to the user, and the task is processed asynchronously in the background. This significantly improves the user experience and overall API performance. Celery integrates seamlessly with Flask, providing a robust and scalable solution for handling asynchronous operations.
Example: A Flask API with Marshmallow and API Versioning
Let’s build a simple API for managing blog posts. We’ll use Marshmallow for data serialization and URI versioning for managing different API versions. Version 1 might only include title and content, while version 2 adds features like author information and publication date. The API would include endpoints like `/v1/posts` and `/v2/posts`, each returning data according to its respective schema defined using Marshmallow. This approach ensures backward compatibility while allowing for the introduction of new features in later versions. The code would involve defining Marshmallow schemas for each version, implementing appropriate routing in Flask, and handling requests accordingly. Error handling and input validation are crucial for a robust implementation. Detailed code examples would showcase the integration of Marshmallow and versioning strategies, highlighting best practices for error handling and data validation.
Error Handling and Logging
Building robust and reliable APIs requires more than just crafting elegant endpoints; it demands a thoughtful approach to error handling and logging. These two pillars ensure your API gracefully handles unexpected situations, provides informative feedback to clients, and aids in debugging and maintenance. Ignoring them can lead to frustrating user experiences and difficult-to-diagnose issues.
Error handling involves anticipating potential problems – from invalid user input to database connection failures – and implementing mechanisms to manage them effectively. This prevents crashes and provides meaningful responses to clients, improving the overall API experience. Meanwhile, comprehensive logging offers a detailed record of API activity, invaluable for tracking down bugs, monitoring performance, and gaining insights into usage patterns. Without it, troubleshooting becomes a nightmare.
Custom Error Handlers in Flask
Flask provides a flexible mechanism for handling exceptions. Instead of letting exceptions bubble up and cause a server-side crash, you can define custom error handlers to intercept specific exception types and return tailored responses. This allows you to present user-friendly error messages, maintain a consistent API response format, and log the error for later analysis.
For instance, a `404 Not Found` error handler could return a JSON response indicating the resource wasn’t found, while a `500 Internal Server Error` handler might return a generic error message while simultaneously logging detailed stack traces. Here’s an example:
“`python
from flask import Flask, jsonify
app = Flask(__name__)
@app.errorhandler(404)
def not_found(error):
return jsonify(‘error’: ‘Not found’), 404
@app.errorhandler(500)
def internal_server_error(error):
return jsonify(‘error’: ‘Internal server error’), 500
# … rest of your app code …
“`
This code defines custom handlers for 404 and 500 errors. Instead of Flask’s default error pages, the API now returns JSON responses containing error messages, making it easier for client applications to handle these situations.
Logging API Events
Effective logging is crucial for monitoring API health and troubleshooting issues. Logs should record significant events, including successful requests, errors, and warnings. They should provide enough context to quickly identify the root cause of problems. This includes timestamps, request details (method, URL, parameters), response status codes, and any relevant error messages.
Consider using a structured logging approach, such as JSON logging, to facilitate easier parsing and analysis of log data. This makes it much simpler to automate log processing and analysis, especially when dealing with high volumes of log entries.
Robust Error Handling and Logging Example
Let’s combine custom error handling and logging into a comprehensive example:
“`python
import logging
from flask import Flask, jsonify, request
app = Flask(__name__)
# Configure logging
logging.basicConfig(filename=’api.log’, level=logging.DEBUG,
format=’%(asctime)s – %(levelname)s – %(message)s’)
@app.route(‘/users/
def get_user(user_id):
try:
# Simulate database interaction
if user_id == 1:
user = ‘id’: 1, ‘name’: ‘John Doe’
return jsonify(user)
else:
raise ValueError(“User not found”)
except ValueError as e:
logging.exception(f”Error fetching user user_id: e”)
return jsonify(‘error’: ‘User not found’), 404
except Exception as e:
logging.exception(f”An unexpected error occurred: e”)
return jsonify(‘error’: ‘Internal server error’), 500
if __name__ == ‘__main__’:
app.run(debug=True)
“`
This example demonstrates how to log exceptions using `logging.exception()`, which automatically includes the stack trace. It also shows how to handle different exception types with custom error handlers.
Best practices for log message formatting generally recommend including a timestamp, log level, a concise message describing the event, and relevant context such as user ID, request ID, or error details. The format should be consistent across all log entries for easier parsing and analysis. For example: `[2024-10-27 10:30:00] – ERROR – User authentication failed for user ID 123 – Invalid password.`
Summary
Building APIs with Flask in Python doesn’t have to be daunting. By following the steps Artikeld in this guide, you’ll gain a solid understanding of the core concepts and best practices needed to create robust, scalable, and secure APIs. From designing efficient endpoints and handling data effectively to implementing secure authentication and deploying your application, you’ll be well-equipped to tackle any API development challenge. So, go forth and build amazing things!