An Introduction to Web Development with Ruby on Rails: Dive into the elegant world of Ruby on Rails, where building dynamic web apps feels less like coding and more like crafting. We’ll unpack the magic behind this popular framework, from its MVC architecture to deploying your creations to the wild. Get ready to build, learn, and maybe even fall in love with the Rails way.
This guide serves as your compass, navigating you through the core concepts, practical examples, and essential techniques needed to build functional web applications using Ruby on Rails. We’ll cover everything from setting up your development environment to deploying your finished product, ensuring you’re equipped to tackle any challenge along the way. Think of it as your fast-track ticket to becoming a Rails rockstar.
What is Ruby on Rails?: An Introduction To Web Development With Ruby On Rails
Ruby on Rails, often shortened to Rails, is a popular open-source web application framework written in Ruby. It’s known for its elegant design, convention-over-configuration approach, and emphasis on developer productivity. Essentially, Rails provides a structured environment and a set of tools that significantly speed up the process of building web applications, allowing developers to focus on the application’s logic and features rather than getting bogged down in repetitive coding tasks.
Fundamental Concepts of Ruby on Rails
Rails follows the Model-View-Controller (MVC) architectural pattern. This pattern separates an application into three interconnected parts: Models, Views, and Controllers. Models represent the data (e.g., database tables), Views handle the user interface (what the user sees), and Controllers manage the logic that connects the Models and Views, handling user input and updating the data. This separation promotes code organization, maintainability, and testability. Rails also utilizes concepts like Active Record (an Object-Relational Mapper, or ORM, that simplifies database interactions), scaffolding (automatic generation of basic CRUD operations), and RESTful routing (a standardized way to structure web application URLs). These features streamline development and adhere to best practices.
Model-View-Controller (MVC) Architecture in Rails
The MVC architecture in Rails facilitates a clean separation of concerns. The Model interacts directly with the database, handling data persistence and validation. The View is responsible for rendering the user interface, using templates (often written in ERB, Embedded Ruby) to display data received from the Controller. The Controller acts as an intermediary, receiving requests from the user, interacting with the Model to retrieve or manipulate data, and then selecting the appropriate View to render the response. For example, a user might submit a form (View). The Controller receives this data, updates the database via the Model, and then redirects the user to a confirmation page (another View).
Advantages and Disadvantages of Using Ruby on Rails
Rails offers several advantages, including rapid development, a large and active community providing ample support and resources, and a vast ecosystem of gems (libraries) that extend its functionality. However, it might not be the ideal choice for projects requiring extremely high performance or those with complex, highly customized needs. The “magic” of Rails, while convenient, can sometimes make debugging more challenging for beginners. Performance can also become a concern in very large applications if not carefully optimized.
Comparison of Ruby on Rails with Other Popular Web Frameworks
Compared to Node.js, Rails offers a more structured and opinionated approach, leading to faster development for certain types of applications. Node.js, with its asynchronous nature, excels in real-time applications and handling high concurrency, but can lead to more complex codebases for less experienced developers. Django, a Python-based framework, shares similarities with Rails in its structure and emphasis on rapid development but employs a different programming language and has its own set of strengths and weaknesses. The best choice depends on project requirements, team expertise, and the specific needs of the application.
Key Features of Ruby on Rails
Feature | Description | Advantage | Example |
---|---|---|---|
MVC Architecture | Separates application logic into Models, Views, and Controllers. | Improved code organization and maintainability. | A user submits a form (View), the Controller processes it, updates the database (Model), and displays a confirmation page (View). |
Active Record | An ORM that simplifies database interactions. | Reduces boilerplate code and speeds up development. | Easily create, read, update, and delete database records using Ruby objects. |
Scaffolding | Automatic generation of basic CRUD operations. | Quickly creates basic functionality for rapid prototyping. | Generate basic controllers, views, and routes for a model with a single command. |
RESTful Routing | Standardized way to structure web application URLs. | Improved URL consistency and ease of use. | URLs follow predictable patterns, e.g., /users, /users/1, /users/new. |
Setting up a Development Environment
So, you’re ready to dive into the world of Ruby on Rails? Awesome! Before you can build the next Instagram killer, you’ll need to set up your development environment. Think of this as prepping your kitchen before you start baking that delicious cake – you need the right ingredients and tools. This section walks you through the essential steps.
Installing Ruby and Rails
Getting Ruby and Rails up and running is surprisingly straightforward. We’ll use Ruby Version Manager (rbenv) for managing multiple Ruby versions, a best practice for keeping your projects organized and avoiding conflicts. rbenv allows you to install and switch between different Ruby versions effortlessly. This is crucial because different Rails versions might require specific Ruby versions.
- Install rbenv: The exact commands will depend on your operating system (OS). For macOS using Homebrew, it’s a simple `brew install rbenv ruby-build`. Consult the rbenv documentation for instructions specific to your OS.
- Install Ruby: Once rbenv is installed, you can install a specific Ruby version. For example, to install Ruby 3.2.2, you’d use `rbenv install 3.2.2`. Check the official Ruby website for the latest stable version.
- Set the global Ruby version: After installation, set the global Ruby version using `rbenv global 3.2.2` (replace with your installed version). This makes the chosen Ruby version the default for all your projects.
- Install Rails: With Ruby installed, installing Rails is a breeze. Open your terminal and type `gem install rails`. This command uses the RubyGems package manager to download and install the latest version of Rails.
Setting up a Local Development Server
Once Rails is installed, creating and running a local development server is incredibly simple. This server lets you test your application locally before deploying it to the internet.
Rails uses a built-in web server called `webrick`. To start the server, navigate to your Rails application directory in your terminal and type `rails server`. This will start the server on a default port (usually 3000). You can then access your application by opening your web browser and going to `http://localhost:3000`.
Creating a New Rails Application
Creating a new Rails application is as easy as typing a single command in your terminal. This command generates the basic file structure and configuration for your project.
To create a new app named “my_app,” navigate to your desired directory in the terminal and type `rails new my_app`. Rails will then generate a directory named “my_app” containing all the necessary files and folders.
Organizing a Rails Project’s File Structure
Rails follows a well-defined directory structure to keep your project organized and maintainable. Understanding this structure is key to navigating your project efficiently.
The `app` directory houses the core application logic, including models (database interactions), controllers (handling requests), views (user interface), helpers (reusable code snippets), and assets (stylesheets, JavaScript, images). The `config` directory contains settings and configurations, while `db` holds your database schema and migrations. `lib` is for custom libraries and reusable code, and `test` contains your test suites. This structured approach promotes clean code and collaboration.
Diagram Illustrating the Flow of Information within a Rails Application
Imagine a simplified diagram: A user’s request (e.g., visiting a page) enters the system. The Rails router determines which controller should handle the request. The controller interacts with the model to access and manipulate data from the database. The model then returns the data to the controller. The controller prepares the data and selects the appropriate view. The view renders the HTML response, which is sent back to the user’s browser. This cycle represents the fundamental flow in a Rails application. The diagram would visually represent this sequence with arrows indicating the data flow between these components (Router -> Controller -> Model -> Controller -> View -> Response). This illustrates the Model-View-Controller (MVC) architectural pattern that Rails employs.
Core Rails Concepts
So, you’ve got your Rails environment set up – congrats! Now let’s dive into the heart of the framework: the core concepts that make Rails tick. Understanding these building blocks will unlock the power to build robust and scalable web applications. We’ll explore ActiveRecord, controllers, views, migrations, and some handy helpers.
ActiveRecord: Your Database BFF
ActiveRecord is the magic behind Rails’ seamless database interaction. Think of it as a translator, effortlessly converting your Ruby code into database queries and vice-versa. Instead of writing raw SQL, you define Ruby classes that represent your database tables. ActiveRecord handles the heavy lifting of creating, reading, updating, and deleting records, abstracting away the complexities of database management. For instance, if you have a `User` model, you can easily create a new user with `User.create(name: “Hipwee Dev”, email: “[email protected]”)` – no SQL needed! This approach promotes cleaner, more maintainable code and reduces the risk of SQL injection vulnerabilities.
Controllers: Orchestrating the Web Symphony
Controllers are the brains of your Rails application, acting as the intermediary between models and views. They receive requests from users, interact with models to fetch or manipulate data, and then select the appropriate view to render the response. Imagine a controller as a conductor of an orchestra, directing the flow of information between the different parts of your application. A typical controller action might fetch data from a model (e.g., retrieve a list of blog posts), process it (e.g., paginate the results), and then pass it to a view for rendering on the webpage.
Database Migrations: Version Control for Your Data
Database migrations are essential for managing changes to your database schema over time. They allow you to define changes in a structured and version-controlled manner, making it easy to track and revert modifications. Instead of manually altering your database, you write Ruby code that describes the changes (e.g., adding a new column to a table, creating a new table). Rails then uses these migrations to apply the changes to your database, ensuring consistency across different environments (development, testing, production). This is crucial for collaboration and prevents accidental data loss.
Rails Helpers: Boosting Your View Productivity
Rails helpers are reusable pieces of code that simplify the process of generating HTML within your views. They provide shortcuts for common tasks, making your views cleaner and more maintainable. For example, `link_to` generates HTML links, `form_with` simplifies the creation of HTML forms, and `image_tag` creates `img` tags. Using helpers significantly reduces boilerplate code and improves readability.
Common Rails Gems and Their Functionalities
Rails’ power is amplified by its extensive ecosystem of gems (pre-built libraries). Here are a few commonly used gems and what they do:
- Devise: Authentication and authorization – handles user login, registration, and access control.
- Rspec: Testing framework – allows you to write tests to ensure the quality and reliability of your code.
- Paperclip/CarrierWave: File uploads – simplifies the process of uploading and managing files (images, documents, etc.).
- Sidekiq: Background job processing – handles time-consuming tasks asynchronously, improving application responsiveness.
- Bootstrap: Front-end framework – provides pre-built CSS and JavaScript components for creating responsive and visually appealing web interfaces.
Building a Simple Web Application
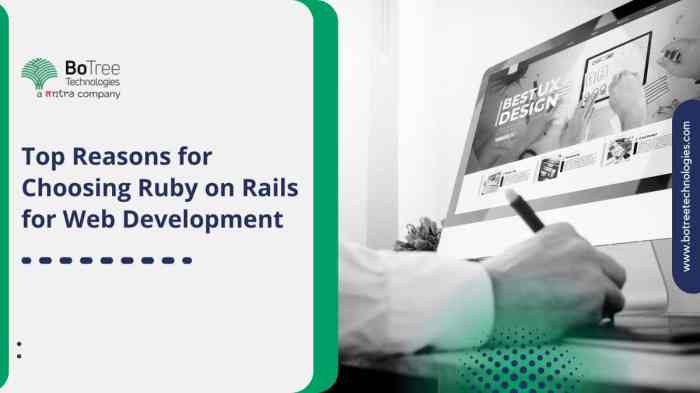
Source: botreetechnologies.com
So you’re diving into the world of web development with Ruby on Rails? It’s a thrilling ride, building robust and scalable apps. But just like building a solid web application requires careful planning, safeguarding your real-world assets is equally important; check out this guide on How to Protect Your Home from Wildfires with the Right Insurance for some serious risk mitigation.
Then, armed with both digital and physical security strategies, you can confidently conquer your coding challenges and life’s unexpected curveballs.
So, you’ve grasped the fundamentals of Ruby on Rails. Now it’s time to get your hands dirty and build something! We’ll start with the classic “Hello, world!” and then ramp up to a more substantial blog application. This will solidify your understanding of Rails’ core concepts and give you a tangible project to showcase your skills.
Creating a “Hello, World!” Application
The simplest Rails application demonstrates the framework’s basic functionality. Generating this application involves using the Rails command-line interface. First, ensure you have a Rails project directory set up. Then, navigate to this directory in your terminal and execute the command `rails new hello_world`. This command creates a new Rails application named “hello_world”. Next, navigate into the newly created directory using `cd hello_world`. After that, start the Rails server with `rails server`. You can then access the application in your web browser at `http://localhost:3000`. You should see a welcome page generated by the Rails framework. This provides a foundation upon which to build more complex applications. Modifying the default views and controllers allows for quick and easy experimentation with the framework’s functionality.
Building a Simple Blog Application
Let’s build a basic blog. This will involve creating models (representing database tables), controllers (managing application logic), and views (displaying information to the user). We’ll use scaffolding to quickly generate the necessary files.
Database Tables and Models
A blog needs to store posts. We’ll create a `Post` model with attributes like `title` and `body`. Rails uses ActiveRecord to interact with the database. The command `rails generate model Post title:string body:text` creates the `Post` model and associated migration file. This migration file defines the database table structure. Running `rails db:migrate` creates the table in your database. The `title` field is a string, and the `body` field is a text field, capable of holding longer text content. These data types are essential for effectively storing the relevant information for each blog post.
Creating, Reading, Updating, and Deleting Posts (CRUD)
Rails provides generators to expedite the creation of controllers and views for CRUD operations. The command `rails generate scaffold Post title:string body:text` creates the necessary files for creating, reading, updating, and deleting posts. This includes controllers for handling requests, views for displaying data, and routes to map URLs to actions. These actions are implemented using standard Rails conventions. The generated views use Rails’ built-in helpers to simplify the creation of forms and data display.
HTML Forms
Rails provides helpers to simplify the creation of HTML forms. For example, the `form_with` helper generates a form with the appropriate attributes and inputs based on the model. Within the form, you would typically include input fields for the `title` and `body` of the post. These fields will be bound to the corresponding attributes in the `Post` model.
User Authentication
Securing your blog requires a user authentication system. Gems like Devise simplify this process. Installing Devise is as simple as running `gem install devise` in your Gemfile and then running `bundle install`. After that, you’ll run the generator `rails generate devise:install` followed by `rails generate devise User name:string email:string` to generate user models. This sets up user accounts with a secure login process. Devise handles user registration, login, password resets, and other authentication-related tasks.
Routing and URL Structure
Rails uses a routing system to map URLs to controller actions. Routes are defined in `config/routes.rb`. For example, `resources :posts` defines routes for creating, reading, updating, and deleting posts. This results in URLs like `/posts`, `/posts/new`, `/posts/1`, `/posts/1/edit`, and `/posts/1/update`. A well-structured routing system improves the organization and maintainability of your application.
User Interface (UI) Design Best Practices
Designing a user-friendly interface is crucial. Rails integrates well with front-end frameworks like Bootstrap or Tailwind CSS to enhance the visual appeal and user experience. Consider using a consistent layout, clear navigation, and intuitive form design. Prioritize mobile responsiveness to ensure your blog is accessible on various devices. Testing your UI on different browsers and devices is essential to ensure cross-browser compatibility and a consistent user experience.
Advanced Topics in Rails Development
So, you’ve built your first Rails app – congrats! But the world of Rails is vast, and there’s a whole universe of power and efficiency waiting to be unlocked. Let’s dive into some advanced techniques that will transform you from a Rails novice to a seasoned pro. We’ll explore extending functionality, mastering testing and debugging, deploying your app, and securing it against common threats.
Gems and Plugins: Extending Rails Functionality
Rails’ strength lies partly in its vibrant ecosystem of gems and plugins. These pre-built packages offer ready-made solutions for common development tasks, from authentication and payment processing to complex data visualizations. By leveraging gems, you drastically reduce development time and avoid reinventing the wheel. For example, `devise` simplifies user authentication, while `activeadmin` provides a powerful admin interface. Careful selection and integration of gems are key to maintaining a clean and efficient application architecture. Understanding gem dependencies and managing conflicts is a crucial skill for any experienced Rails developer.
Testing and Debugging in Rails
Thorough testing is non-negotiable in Rails development. It’s not just about finding bugs; it’s about ensuring your application’s robustness and maintainability. Rails encourages a test-driven development (TDD) approach, where tests are written *before* the code. Common testing frameworks include RSpec and Minitest. Debugging, inevitably a part of the process, involves using tools like the Rails console, logs, and debuggers to pinpoint and resolve issues. Effective debugging strategies significantly reduce development time and frustration. Understanding how to interpret stack traces and leverage debugging tools is essential for efficient problem-solving.
Deploying a Rails Application to a Production Server, An Introduction to Web Development with Ruby on Rails
Deploying your shiny new application to a production server marks a significant milestone. This process involves transferring your code, configuring the server environment, and ensuring the application runs smoothly and efficiently. Popular deployment methods include Capistrano, which automates the deployment process, and platforms like Heroku and AWS, which provide managed infrastructure. Choosing the right deployment strategy depends on factors like application size, scalability requirements, and budget. Understanding server configuration, database management, and potential deployment pitfalls is crucial for a successful launch.
Deployment Strategies: A Comparison
Several strategies exist for deploying Rails applications, each with its strengths and weaknesses. Capistrano, a popular choice, offers fine-grained control and automation but requires more technical expertise. Platform-as-a-Service (PaaS) solutions like Heroku simplify deployment but may offer less customization. Infrastructure-as-a-Service (IaaS) providers like AWS give maximum control but demand significant infrastructure management skills. The optimal strategy depends on the specific needs of the project and the team’s technical capabilities. For instance, a small startup might favor Heroku’s ease of use, while a large enterprise might opt for the scalability and customization offered by AWS.
Common Rails Security Vulnerabilities and Mitigation
Security is paramount in any web application, and Rails applications are no exception. Common vulnerabilities include SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Mitigating these risks involves using parameterized queries to prevent SQL injection, escaping user input to prevent XSS, and implementing CSRF protection mechanisms. Regular security audits and penetration testing are also crucial to identify and address potential weaknesses. Staying updated with the latest security best practices and leveraging security gems is essential for maintaining a secure application. For example, using strong passwords and implementing proper authentication mechanisms are critical to prevent unauthorized access.
Front-End Integration
Building a killer web application with Ruby on Rails isn’t just about the back-end magic; it’s about creating a seamless and engaging user experience. This means mastering the art of front-end integration – blending the power of Rails with the beauty and responsiveness of HTML, CSS, and JavaScript. Think of it as marrying brains (Rails) with brawn (front-end).
Front-end technologies like HTML, CSS, and JavaScript are essential for creating the user interface (UI) and user experience (UX) of your Rails application. HTML structures the content, CSS styles its appearance, and JavaScript adds interactivity and dynamic behavior. Integrating these effectively ensures your app looks great and works flawlessly.
Integrating HTML, CSS, and JavaScript
Rails provides several ways to integrate these core front-end technologies. You can embed them directly within your Rails views (`.erb` files), using ERB templating to seamlessly combine Ruby logic with HTML, CSS, and JavaScript. Alternatively, you can use asset pipelines (like Sprockets) to manage and compile your front-end assets efficiently, ensuring optimal performance and organization. This keeps your code clean and easy to maintain. Consider using a CSS preprocessor like Sass or Less to improve your CSS workflow.
Using JavaScript Frameworks
Modern web development often leverages JavaScript frameworks to build complex and interactive user interfaces. Popular choices include React, Vue.js, and Angular. These frameworks provide structure, components, and tools that simplify the development process and enhance the performance of your application. Within a Rails application, these frameworks are often integrated using asset pipelines or by employing JavaScript bundlers like Webpack or Parcel. For example, a React component might fetch data from a Rails API endpoint via AJAX, updating the UI dynamically without requiring a full page reload.
AJAX and Dynamic Web Applications
AJAX (Asynchronous JavaScript and XML) is a cornerstone of creating dynamic web applications. It allows you to update parts of a web page without reloading the entire page. This results in a smoother, more responsive user experience. In a Rails context, AJAX is typically used to send requests to controllers and receive JSON responses, which are then used to update the DOM (Document Object Model) using JavaScript. Imagine a search bar that updates results in real-time as you type – that’s the power of AJAX.
Responsive Web Design with CSS Frameworks
Creating a responsive web design is crucial for ensuring your application looks great on all devices, from desktops to smartphones and tablets. CSS frameworks like Bootstrap and Tailwind CSS provide pre-built components and utility classes that significantly simplify the process of building responsive layouts. These frameworks handle the complexities of adapting your layout to different screen sizes, allowing you to focus on the application’s functionality and content. They offer responsive grids, pre-styled components, and helpful utilities for quick and consistent styling.
Front-End and Back-End Communication
Imagine a diagram: On the left, you have the user’s browser interacting with the front-end (HTML, CSS, JavaScript, possibly a framework like React). The front-end makes requests (often via AJAX) to the back-end (your Rails application). The back-end processes these requests, interacts with the database, and sends back responses (typically in JSON format). The front-end then uses this data to update the UI, creating a dynamic and interactive experience. This communication is often asynchronous, meaning the front-end doesn’t have to wait for the back-end to fully process a request before continuing.
Final Thoughts
So, you’ve journeyed through the exciting world of Ruby on Rails. From setting up your environment to deploying your first app, you’ve gained a solid foundation. Remember, the beauty of Rails lies in its convention over configuration, making development efficient and enjoyable. Now go forth, build amazing things, and don’t be afraid to experiment – the Rails community is always there to support you.