How to Optimize Your SQL Queries for Better Performance? It’s the question every developer wrestles with at some point. Sluggish queries can cripple your application, leaving users frustrated and your servers groaning under the strain. This isn’t just about tweaking a few lines of code; it’s about understanding the inner workings of your database, mastering indexing techniques, and wielding the power of query optimization strategies. We’ll dive deep into the nitty-gritty, exploring everything from basic indexing to advanced techniques like query profiling, ensuring your SQL queries run like a well-oiled machine.
We’ll cover common pitfalls that lead to slow queries, showing you how to identify and fix them. Learn to choose the right indexes, master JOINs and subqueries, and even harness the power of database tuning. Real-world examples will illustrate how even seemingly small changes can dramatically improve performance. By the end, you’ll be equipped to tackle even the most challenging SQL optimization problems with confidence.
Understanding SQL Query Performance Issues
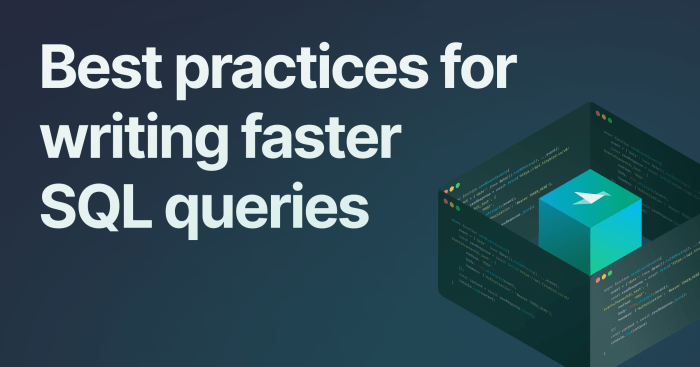
Source: ghost.io
Boosting your SQL query performance is all about efficiency; just like securing your family’s future requires a solid plan. Planning for the unexpected is key, and you can learn more about that crucial step by checking out this guide on The Importance of Protecting Your Family’s Future with Life Insurance. Similarly, optimizing indexes and avoiding unnecessary joins in your SQL queries ensures a smooth, responsive system – a kind of financial security for your database.
Slow SQL queries can be a major headache, bringing your database—and your entire application—to its knees. Imagine users staring at loading screens, transactions failing, and reports taking forever to generate. This isn’t just frustrating; it directly impacts user experience and can significantly hinder business operations. Understanding the root causes of slow queries is the first step towards building faster, more efficient applications.
Common Causes of Slow SQL Queries
Several factors contribute to sluggish SQL query performance. These range from poorly written queries to inadequate database indexing and even hardware limitations. A poorly designed database schema can also significantly impact performance. Let’s explore some of the most frequent culprits. Inefficient queries often involve unnecessary table scans, complex joins, and the lack of appropriate indexes.
Examples of Poorly Written SQL Queries and Their Performance Impact
Consider this example: a query that selects all columns from a large table without any filtering. This forces the database to read every single row, a process known as a full table scan. This is incredibly inefficient, especially with large datasets. A better approach would involve using a `WHERE` clause to filter the results and limit the number of rows processed.
Another common issue is using `SELECT *` instead of specifying the required columns. This forces the database to retrieve all columns, even if only a few are actually needed, significantly increasing the query’s execution time and the amount of data transferred.
For instance, imagine a query like this:
SELECT * FROM large_table WHERE customer_id = 123;
This query, while functional, is inefficient. A more optimized version would be:
SELECT customer_name, customer_email FROM large_table WHERE customer_id = 123;
This revised query only retrieves the necessary columns, significantly reducing the data processed and improving performance.
Strategies for Identifying Performance Bottlenecks in SQL Queries
Pinpointing the exact source of a performance problem requires a systematic approach. Database management systems (DBMS) offer various tools to help. Profiling tools, built into most DBMSs, analyze query execution plans, highlighting bottlenecks. These plans illustrate how the database intends to execute the query, revealing areas for improvement, such as missing indexes or inefficient join strategies. Analyzing query execution plans is crucial for effective optimization.
Methods for Measuring Query Execution Time
Most database systems provide built-in functions or tools to measure query execution time. For example, many systems allow you to use functions like `GETDATE()` or `NOW()` before and after query execution to calculate the elapsed time. Alternatively, many database monitoring tools provide detailed performance metrics, including query execution times, allowing you to track performance over time and identify trends. Some tools even offer real-time monitoring, giving you immediate insights into query performance.
Comparison of Query Optimization Techniques
Technique | Description | Effectiveness | Example |
---|---|---|---|
Adding Indexes | Creating indexes on frequently queried columns speeds up data retrieval. | High, significantly reduces query execution time for searches and joins. | CREATE INDEX idx_customer_id ON large_table (customer_id); |
Query Rewriting | Rewriting queries to use more efficient syntax or algorithms. | Moderate to High, depends on the specific query and rewrite. | Replacing nested queries with joins. |
Optimizing Joins | Choosing appropriate join types (e.g., inner join vs. outer join) and join order. | Moderate to High, impacts performance especially with large tables. | Using `INNER JOIN` instead of `LEFT JOIN` when appropriate. |
Database Normalization | Organizing data to reduce redundancy and improve data integrity. | High, long-term improvement for data consistency and query performance. | Applying normalization rules (1NF, 2NF, 3NF) to database design. |
Indexing Techniques for Enhanced Performance: How To Optimize Your SQL Queries For Better Performance
Database indexing is like having a super-powered index in the back of a textbook – it dramatically speeds up your search for specific information. Without it, your database has to painstakingly scan every single row, which can be incredibly slow, especially with large datasets. Indexing allows your database to quickly locate the data it needs, significantly boosting query performance and making your application feel snappy and responsive. Think of it as the difference between searching a library by browsing every single shelf versus using the card catalog (or its modern equivalent).
Efficient indexing is crucial for optimizing SQL queries. It’s not a one-size-fits-all solution, however, and choosing the right index type is key to maximizing performance. Let’s dive into the world of indexing techniques.
B-tree Indexes
B-tree indexes are the workhorses of many database systems. They’re tree-like data structures that efficiently organize data for both searching and sorting. A B-tree index is particularly effective for range queries (e.g., finding all customers with ages between 25 and 35) and equality queries (e.g., finding a specific customer by ID). The “B” in B-tree doesn’t stand for anything specific, but it’s commonly associated with “balanced tree,” hinting at its structure that ensures efficient searching. B-trees handle insertions and deletions relatively efficiently, making them a versatile choice for most applications. They’re optimized for disk access, minimizing the number of disk reads required to locate data. For example, imagine a B-tree index on a `customer_id` column. The database can quickly navigate the tree to locate the specific row containing the desired customer information.
Hash Indexes
Hash indexes utilize a hash function to map data values to their corresponding disk locations. This makes them incredibly fast for equality searches. If you need to find a specific record based on a unique identifier, a hash index can be a blazing-fast solution. However, hash indexes are not suitable for range queries or sorting operations because the data isn’t stored in any particular order. Consider a scenario where you’re managing product IDs; a hash index on the `product_id` column would be ideal for quickly retrieving specific product details.
Full-Text Indexes
Full-text indexes are specialized for searching within textual data. They go beyond simple matching, offering capabilities like stemming (reducing words to their root form) and phonetic matching (finding words that sound alike). This makes them invaluable for applications like search engines or document databases where finding relevant information based on textual content is paramount. Imagine an e-commerce site with product descriptions; a full-text index allows users to search for products using natural language phrases, finding matches even if the exact words aren’t present.
Creating and Managing Indexes
The process of creating an index typically involves specifying the table, column(s) to be indexed, and the index type. Most database systems provide SQL commands for this purpose. For example, in MySQL, you might use a command like `CREATE INDEX index_name ON table_name (column_name);`. Managing indexes involves monitoring their performance and removing or modifying them as needed. Over-indexing (creating too many indexes) can actually degrade performance, as it adds overhead to data modification operations. Therefore, a strategic approach to index creation and management is essential. Regularly analyzing query execution plans can help identify underperforming queries and pinpoint opportunities for index optimization.
Example Database Schema and Index Selection
Let’s consider a simple e-commerce database with tables for `products` and `orders`.
Table | Columns | Suggested Indexes |
---|---|---|
products | product_id (INT, PRIMARY KEY), product_name (VARCHAR), category_id (INT), price (DECIMAL) | product_id (PRIMARY KEY), category_id |
orders | order_id (INT, PRIMARY KEY), customer_id (INT), order_date (DATE), total_amount (DECIMAL) | order_id (PRIMARY KEY), customer_id, order_date |
In this example, primary keys are automatically indexed. Additional indexes on `category_id` in the `products` table and `customer_id` and `order_date` in the `orders` table would optimize queries filtering by category, customer, or order date. The choice of indexes depends on the most frequently executed queries. For instance, if you often need to retrieve products by category, indexing `category_id` is beneficial.
Query Optimization Strategies
So, you’ve tackled the *why* of slow SQL queries – now let’s dive into the *how* of fixing them. Query optimization isn’t just about slapping indexes everywhere; it’s a strategic process of rewriting and refining your queries for peak performance. Think of it as sculpting a masterpiece from a block of raw data – the right tools and techniques make all the difference.
Query Rewriting Techniques
Effective query rewriting is about transforming inefficient SQL statements into their optimized equivalents. This often involves identifying bottlenecks and applying specific techniques to eliminate them. For instance, a query with multiple nested loops might be significantly improved by restructuring it to utilize indexes more effectively or by employing set operations. The goal is to minimize the amount of data the database needs to process, leading to faster execution times. A simple example would be changing a query that uses `LIKE ‘%pattern%’` (which is notoriously slow) to one using a full-text search or a more specific pattern match, if possible.
Effective Use of JOINs, Subqueries, and CTEs
Choosing the right join type (INNER, LEFT, RIGHT, FULL) is crucial. INNER JOINs are generally faster than other join types because they only return matching rows. Subqueries, while useful for isolating specific data sets, can sometimes be slower than their equivalent CTE (Common Table Expression) counterparts. CTEs improve readability and, in many cases, performance by materializing the results of a subquery once, then reusing them multiple times within the main query, avoiding redundant calculations.
For example, an inefficient query using nested subqueries to find customers with orders totaling more than $1000 could be rewritten using a CTE to improve readability and potentially performance:
Instead of:
SELECT customer_id FROM customers WHERE customer_id IN (SELECT customer_id FROM orders GROUP BY customer_id HAVING SUM(order_total) > 1000);Use:
WITH high_value_customers AS (SELECT customer_id FROM orders GROUP BY customer_id HAVING SUM(order_total) > 1000) SELECT customer_id FROM high_value_customers;
The CTE approach often leads to a more efficient execution plan, especially in complex queries. Similarly, well-structured JOINs can dramatically reduce the amount of data processed compared to subqueries.
Utilizing Set Operations for Performance Gains
Set operations like UNION, INTERSECT, and EXCEPT offer elegant ways to combine or compare results from multiple queries. These operations can be significantly faster than using joins, especially when dealing with large datasets, because they often utilize optimized algorithms for set manipulation. For example, finding customers who placed orders both in January and February is far more efficient using INTERSECT than joining the January and February order tables.
Optimizing Queries with Window Functions
Window functions are a powerful tool for performing calculations across a set of rows related to the current row, without the need for self-joins or subqueries. This can lead to significant performance improvements, especially when dealing with ranking, aggregation, or running totals. For instance, calculating the running total of sales for each month can be efficiently done with a window function, avoiding the complexities and potential performance issues of a self-join approach.
Best Practices for Efficient SQL Queries
Efficient query writing is a skill honed over time. Here are some key practices:
- Use appropriate data types: Choosing the right data type for each column minimizes storage space and improves query performance.
- Avoid using functions in WHERE clauses: Functions applied to columns in the WHERE clause often prevent the use of indexes, slowing down query execution. For example, avoid `WHERE UPPER(column) = ‘VALUE’`, instead, use `WHERE column = ‘value’` if case-sensitivity isn’t crucial.
- Optimize table designs: Properly normalized tables reduce data redundancy and improve query efficiency.
- Use EXPLAIN PLAN: Utilize your database system’s query analyzer (like `EXPLAIN PLAN` in Oracle or similar tools in other systems) to understand how the database plans to execute your query. This reveals potential bottlenecks and guides optimization efforts.
- Index strategically: Indexes are your friends, but too many indexes can slow down write operations. Focus on frequently queried columns and those used in JOIN conditions.
By mastering these techniques, you can transform your SQL queries from lumbering behemoths into lean, mean, data-retrieval machines. Remember, optimization is an iterative process; continuous monitoring and refinement are key to maintaining peak performance.
Database Tuning and Configuration
Optimizing your SQL queries isn’t just about writing clever code; it’s also about making sure your database server is properly configured. Think of it like this: you can have the best race car in the world, but if the track is poorly maintained or the engine isn’t tuned correctly, you won’t win the race. Similarly, even the most efficient SQL queries will be hampered by a poorly configured database server. Proper configuration is the unsung hero of database performance.
Database server configuration plays a pivotal role in query performance. Factors like memory allocation, buffer pool size, and various other settings directly impact how quickly your database can retrieve and process data. Neglecting these aspects can lead to slow query execution, increased latency, and ultimately, a less responsive application. Let’s delve into some key areas.
Buffer Pool Management
The buffer pool is a crucial area of database configuration. It’s a cache in the database server’s memory that stores frequently accessed data pages. When a query needs data, the database first checks the buffer pool. If the data is present (a “cache hit”), the query is executed much faster. If the data isn’t in the buffer pool (a “cache miss”), the database has to read it from disk, which is significantly slower. Therefore, allocating sufficient memory to the buffer pool is paramount. A larger buffer pool generally leads to fewer cache misses and improved query performance. However, excessively large buffer pools can lead to reduced memory available for other operating system processes. Finding the optimal size often involves experimentation and monitoring. For instance, a database handling large transactional workloads might benefit from a larger buffer pool compared to a database primarily used for analytical queries.
Memory Allocation and Other Server Settings
Beyond the buffer pool, overall memory allocation significantly impacts performance. Insufficient memory can lead to excessive swapping (moving data between RAM and disk), drastically slowing down query execution. Other important settings include the number of worker threads, connection pool size, and the choice of storage engine. The number of worker threads determines how many concurrent queries the server can handle. Too few threads can lead to bottlenecks, while too many can lead to context switching overhead. Similarly, the connection pool size dictates how many concurrent connections the server can accept. A poorly sized connection pool can lead to connection timeouts or performance degradation under heavy load. Finally, the choice of storage engine (e.g., InnoDB, MyISAM) impacts performance characteristics; InnoDB generally offers better transactional support but might be slightly slower for read-only operations compared to MyISAM.
Configuration Changes for Performance Improvement
Consider a scenario where a database consistently experiences slow query performance due to frequent disk I/O. Increasing the buffer pool size by 50% (e.g., from 4GB to 6GB) could dramatically reduce disk reads and improve query response times. Another example involves optimizing the number of worker threads. If monitoring reveals high CPU utilization and long query wait times, increasing the number of worker threads (within reasonable limits) might alleviate the bottleneck. Similarly, adjusting the connection pool size to accommodate peak loads can prevent connection failures and improve application responsiveness. These adjustments should be made incrementally and carefully monitored to avoid negative consequences.
Monitoring Database Performance Metrics, How to Optimize Your SQL Queries for Better Performance
Effective database monitoring is essential for identifying performance bottlenecks and making informed configuration changes. Key metrics to track include CPU utilization, memory usage, disk I/O, query execution times, and the number of active connections. Database management systems (DBMS) typically offer built-in monitoring tools or integrate with third-party monitoring solutions. Regularly analyzing these metrics allows for proactive identification of issues and prevents performance degradation. For example, consistently high disk I/O could indicate a need for increased buffer pool size or faster storage. Similarly, consistently high CPU utilization might suggest a need for more worker threads or query optimization.
Checklist for Optimizing Database Server Configuration
Before making any changes, always back up your database!
- Assess Current Performance: Analyze existing performance metrics to identify bottlenecks.
- Buffer Pool Sizing: Determine the optimal buffer pool size based on workload and available memory. Start with a reasonable increase and monitor the impact.
- Memory Allocation: Ensure sufficient memory is allocated to the database server to avoid swapping.
- Worker Threads: Adjust the number of worker threads based on CPU utilization and concurrency needs.
- Connection Pool Size: Configure the connection pool size to handle peak loads without connection timeouts.
- Storage Engine Selection: Choose the appropriate storage engine based on the application’s requirements (e.g., transactional vs. analytical).
- Regular Monitoring: Implement a robust monitoring system to track key performance metrics and proactively identify issues.
Advanced Optimization Techniques
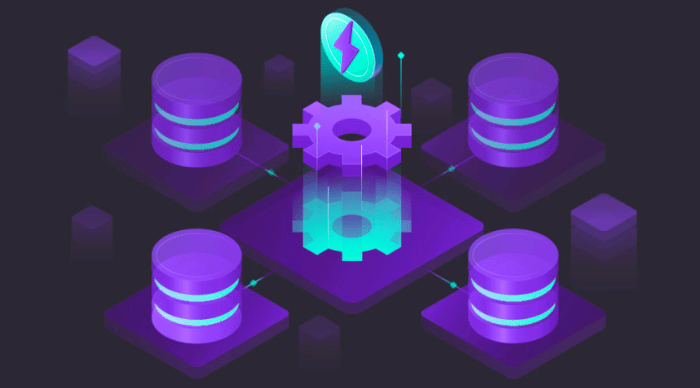
Source: medium.com
So you’ve tackled the basics of SQL optimization – indexing, query strategies, and database tuning. But what if your queries are still crawling? That’s where the advanced techniques come in, offering powerful tools to wrangle even the most stubborn performance bottlenecks. Think of this as moving from standard tools to precision instruments for your database surgery.
Query Profiling and Performance Issue Identification
Query profiling is like having a detective investigate your database’s performance. It pinpoints exactly where your queries are spending their time, revealing slowdowns caused by specific operations, table scans, or joins. Tools like SQL Server Profiler or MySQL’s slow query log record execution plans, durations, and resource consumption for each query. By analyzing this data, you can identify the culprits slowing down your database and focus your optimization efforts. For example, if a profile shows a query spending 90% of its time on a table scan instead of using an index, you know exactly where to concentrate your optimization work. This targeted approach is far more efficient than blindly trying different optimization strategies.
The Use of Query Hints and Their Potential Impact
Query hints are directives you give the database optimizer, guiding it to use specific execution plans. They can be powerful tools, but use them cautiously. While hints can sometimes override suboptimal choices by the optimizer, they can also lead to less efficient plans if misused. A well-placed hint can significantly improve query performance, but poorly chosen hints can hinder it. For instance, if the optimizer consistently chooses a less-than-ideal join order, a hint can force it to use a more efficient strategy. However, remember that the optimizer usually knows best; only use hints when you have a thorough understanding of your query and its execution plan. Improper use can lead to performance degradation and maintenance headaches down the road.
Optimizing Large Datasets and Complex Queries
Dealing with massive datasets and complex queries requires a multi-pronged approach. Partitioning large tables into smaller, manageable chunks can dramatically speed up queries that only need to access a subset of the data. Materialized views can pre-compute results for frequently accessed queries, eliminating the need for repeated calculations. Techniques like data warehousing and ETL (Extract, Transform, Load) processes become crucial for efficiently handling and transforming this volume of data. For instance, if you have a table with billions of rows, partitioning it by date or region allows queries to only scan the relevant partitions, reducing processing time substantially. Imagine searching for sales data from a specific quarter; partitioning by quarter immediately limits the scope of the search.
Handling Different Types of Database Locks to Improve Concurrency
Database locks are essential for maintaining data integrity, but they can also create bottlenecks. Understanding different lock types – shared locks, exclusive locks, row-level locks, and page-level locks – is crucial. Optimizing concurrency involves minimizing lock contention by using appropriate lock granularity and minimizing the duration of locks held. For example, switching from page-level locks to row-level locks can drastically reduce blocking when multiple users are accessing the same table concurrently. A well-designed application with careful consideration of lock usage can handle thousands of concurrent users without significant performance issues. Conversely, poor lock management can lead to significant slowdowns and deadlocks.
Application of Stored Procedures and Functions for Performance Enhancement
Stored procedures and functions pre-compile SQL code, reducing the overhead of parsing and optimizing queries every time they’re executed. They also enhance code reusability and maintainability. Moreover, stored procedures can encapsulate complex logic, improving performance by avoiding repeated execution of individual SQL statements. For example, a stored procedure performing a series of updates and inserts will generally outperform a series of individual SQL statements sent from the application, as the database only needs to parse and optimize the stored procedure once. This pre-compilation and reduced network traffic contribute to significant performance improvements, especially for frequently executed operations.
Analyzing Execution Plans
Understanding how your SQL queries are executed is crucial for optimization. SQL execution plans are detailed roadmaps showing the database’s strategy for retrieving data. Analyzing these plans reveals bottlenecks and allows for targeted improvements. Think of it as getting a behind-the-scenes look at your database’s problem-solving process.
Execution Plan Interpretation
SQL execution plans detail the steps the database takes to fulfill a query. They typically show the order of operations, the algorithms used (e.g., index scans, table scans, nested loops), and the estimated cost associated with each step. A high cost usually indicates a performance bottleneck. The plan might display information like the number of rows read, the amount of I/O, and the execution time for each operation. Different database systems (like MySQL, PostgreSQL, SQL Server) present execution plans slightly differently, but the core concepts remain the same. For example, a high “cost” value next to a “Table Scan” operation often signifies a performance issue that could be addressed by using an appropriate index.
Identifying Inefficiencies in Execution Plans
Inefficient execution plans often involve full table scans instead of index lookups, poorly chosen join methods (e.g., nested loop joins on large tables), or suboptimal sorting strategies. Look for operations with high costs, large row counts, or significant I/O. For instance, if a plan shows a full table scan on a large table followed by a filter operation, adding an index on the filtered column will likely drastically improve performance. Similarly, if a join operation involves a nested loop on large tables, consider using a hash join or merge join for better efficiency. The specific improvements will depend on the query and the database system.
Impact of Indexing Strategies on Execution Plans
Indexing significantly impacts execution plans. Without an index on a frequently filtered column, the database will resort to a full table scan, which is incredibly slow for large tables. Adding an appropriate index changes the plan dramatically, replacing the table scan with a much faster index lookup. For example, if you have a query filtering for customers in a specific city, an index on the “city” column will drastically reduce the execution time. The execution plan will clearly show the shift from a table scan to an index seek. Different index types (B-tree, hash, etc.) can also influence the execution plan and its efficiency. Careful index design is crucial for optimal query performance.
Visualizing Execution Plans
Most database management systems (DBMS) provide tools for visualizing execution plans. These tools typically present the plan as a tree or graph, clearly showing the sequence of operations and their costs. Some systems offer graphical representations, while others display textual representations. Understanding these visual representations is key to pinpointing bottlenecks. For example, a graphical representation might highlight operations with high costs using different colors or sizes, making it easier to identify areas for improvement. The specific visualization method varies across different DBMS.
Analyzing Execution Plans to Pinpoint Performance Bottlenecks
Analyzing an execution plan requires a systematic approach. Here’s a guide:
- Identify High-Cost Operations: Start by looking for operations with the highest costs. These are the primary candidates for optimization.
- Examine the Operations Sequence: Analyze the order of operations to identify potential inefficiencies. Are expensive operations performed unnecessarily early in the process?
- Check for Full Table Scans: Full table scans are often a major performance bottleneck. Determine if indexes can be used to avoid them.
- Analyze Join Methods: Review the join methods used (e.g., nested loop, hash join, merge join). Are the most efficient join methods being used for the data sizes involved?
- Assess Sorting and Grouping Strategies: Determine if the sorting and grouping methods are optimal for the data volume.
- Consider I/O Operations: Pay attention to the amount of I/O involved. High I/O often indicates a need for indexing or other optimizations.
- Iterative Refinement: Implement changes based on your analysis, and then re-examine the execution plan to verify the improvements.
Case Studies
Let’s dive into some real-world examples where optimizing SQL queries made a massive difference. These aren’t theoretical exercises; these are stories of battling slow databases and emerging victorious. We’ll see how seemingly small tweaks can lead to dramatic improvements in application performance.
Optimizing SQL queries isn’t just about writing cleaner code; it’s about understanding your data, your application, and how they interact. The following examples highlight the importance of a holistic approach to database performance.
E-commerce Website Catalog Search Optimization
This case study focuses on an e-commerce platform struggling with slow product searches. Customers experienced significant delays when browsing the catalog, leading to frustration and potentially lost sales. The initial query used a poorly structured `JOIN` across multiple tables without appropriate indexing. This resulted in full table scans, drastically slowing down the retrieval of product information.
The solution involved creating composite indexes on the relevant columns used in the `JOIN` conditions, optimizing the query to use these indexes, and rewriting parts of the query to leverage database features for better performance. This reduced the query execution time from an average of 15 seconds to under 0.2 seconds.
Metric | Before Optimization | After Optimization |
---|---|---|
Average Query Execution Time | 15 seconds | 0.2 seconds |
Number of Rows Scanned | Millions | Thousands |
Customer Satisfaction (Based on Survey) | 3.2 out of 5 | 4.5 out of 5 |
Financial Reporting System Performance Enhancement
A large financial institution faced challenges with its daily reporting system. Generating reports, especially those involving complex aggregations across large datasets, took hours, hindering timely decision-making. The existing queries lacked efficient use of window functions and were performing redundant calculations.
The solution involved refactoring queries to leverage window functions, reducing redundant calculations and improving the overall query structure. Additionally, materialized views were implemented to pre-calculate frequently accessed aggregated data. The result was a significant reduction in report generation time, from an average of 4 hours to under 15 minutes.
Metric | Before Optimization | After Optimization |
---|---|---|
Average Report Generation Time | 4 hours | 15 minutes |
Resource Consumption (CPU) | High (80% utilization) | Low (20% utilization) |
Data Analyst Productivity | Low | High |
Closing Notes
Optimizing your SQL queries isn’t a one-time fix; it’s an ongoing process of refinement and learning. By understanding the underlying principles and applying the techniques discussed here, you’ll not only improve the performance of your applications but also gain a deeper appreciation for the elegance and power of efficient database design. Remember, the journey to perfectly optimized queries is a marathon, not a sprint – but with the right knowledge and tools, you’ll be well on your way to building faster, more responsive, and ultimately, more successful applications. So buckle up and get ready to supercharge your SQL performance!