Building Cross-Platform Mobile Apps with Flutter: Forget the endless cycle of iOS and Android development. Dive into the world of streamlined app creation with Flutter, the revolutionary framework that lets you build stunning, native-quality apps for both platforms from a single codebase. This isn’t just about saving time; it’s about unlocking a whole new level of efficiency and creativity.
We’ll unpack everything from setting up your development environment to mastering state management and deploying your masterpiece to the app stores. Get ready to build beautiful, high-performing apps without the usual cross-platform headaches. We’ll cover the core concepts, compare it to other frameworks, and guide you through practical examples – because seeing is believing (and building is even better!).
Introduction to Flutter for Cross-Platform Mobile App Development
Flutter has emerged as a game-changer in the world of cross-platform mobile app development. Its ability to build beautiful, natively compiled applications for iOS and Android from a single codebase has attracted developers worldwide, offering a compelling alternative to traditional native development or other cross-platform solutions. This section dives into what makes Flutter tick, highlighting its advantages and comparing it to other popular frameworks.
Flutter’s Advantages in Cross-Platform Development
Flutter boasts several key advantages that make it a strong contender in the cross-platform arena. Firstly, its “hot reload” feature dramatically speeds up development, allowing developers to see changes reflected instantly in the app without restarting. This iterative development process significantly reduces debugging time and improves overall efficiency. Secondly, Flutter uses its own rendering engine, Skia, which ensures consistent performance and UI appearance across different devices and Android versions, eliminating many compatibility headaches. Finally, Flutter’s growing community provides ample resources, support, and readily available packages, simplifying development and accelerating project completion. This combination of speed, consistency, and community support makes Flutter a highly attractive option for businesses and developers alike.
Flutter’s Core Principles and Architecture
At its heart, Flutter is built on the Dart programming language, a client-optimized language developed by Google. Dart’s features, including its garbage collection and asynchronous programming capabilities, contribute to Flutter’s performance and ease of use. Flutter’s architecture is based on a layered approach. The foundation is the Skia graphics engine, responsible for rendering the UI. Above this sits the Flutter framework, providing widgets, which are reusable UI elements, and APIs for accessing platform features. The framework’s declarative approach simplifies UI development, allowing developers to describe the desired UI state, and Flutter handles the rendering and updates efficiently. This structure allows for customization and high performance, key ingredients for successful mobile applications.
Comparison of Flutter with React Native and Xamarin
Flutter, React Native, and Xamarin are popular cross-platform frameworks, each with its own strengths and weaknesses. React Native, built using JavaScript, leverages native components for UI rendering, resulting in a performance that can sometimes be less consistent than Flutter’s. Xamarin, on the other hand, uses C# and relies heavily on native platform APIs, which can lead to more complex codebases and slower development cycles. Flutter, with its own rendering engine and Dart language, offers a balance between performance, development speed, and ease of use. While each framework has its place, Flutter’s hot reload, consistent UI, and growing ecosystem have solidified its position as a strong competitor.
Key Feature Comparison: Flutter, React Native, and Xamarin
The following table summarizes key features and allows for a direct comparison of these three prominent cross-platform development frameworks.
Feature | Flutter | React Native | Xamarin |
---|---|---|---|
Programming Language | Dart | JavaScript | C# |
UI Rendering | Skia (Own Rendering Engine) | Native Components | Native Components |
Performance | High | Moderate | Moderate to High (depending on implementation) |
Development Speed | High (Hot Reload) | Moderate | Moderate |
Setting up the Development Environment
Getting started with Flutter app development requires setting up your development environment correctly. This involves installing the Flutter SDK, choosing an IDE, and configuring it to work seamlessly with Flutter. Don’t worry, it’s less daunting than it sounds – we’ll walk you through it step-by-step for Windows, macOS, and Linux.
Setting up your environment involves several key steps: installing the Flutter SDK, configuring your IDE (either Android Studio or VS Code), and setting up an emulator or connecting a physical device for testing. Each operating system has slight variations, but the core process remains consistent.
Flutter SDK Installation
First, download the Flutter SDK from the official Flutter website. You’ll find a zip file containing the SDK. Once downloaded, extract the contents to a preferred location on your system. For example, on Windows, you might extract it to `C:\src\flutter`. On macOS, `/Users/
After extraction, you’ll need to add the `bin` directory within the Flutter SDK directory to your system’s PATH environment variable. This allows your system to locate the Flutter command-line tools. The exact method for adding to the PATH variable varies depending on your operating system. For Windows, you’ll typically find this setting in the system environment variables. On macOS and Linux, you might need to edit your shell’s configuration file (e.g., `.bashrc`, `.zshrc`, or similar). Imagine a visual representation of the Windows environment variables settings window, showing the addition of the Flutter bin directory to the PATH variable. This would involve selecting the PATH variable, clicking “Edit,” and adding the path to the Flutter bin directory. The macOS equivalent would be opening the terminal and using the command `echo ‘export PATH=”$PATH:/Users/
IDE Setup: Android Studio
Android Studio, a fully-featured IDE, provides excellent Flutter support. After installing Android Studio, you need to install the Flutter and Dart plugins. This can be done through the Android Studio plugin manager (File > Settings > Plugins on Windows/Linux, Android Studio > Preferences > Plugins on macOS). Search for “Flutter” and “Dart,” click “Install,” and restart Android Studio once the installation is complete. A screenshot would show the Plugins window in Android Studio with the Flutter and Dart plugins highlighted, ready for installation. The installation process involves clicking an “Install” button and waiting for the download and installation to complete, followed by a prompt to restart the IDE.
IDE Setup: VS Code
VS Code is a lightweight yet powerful alternative. Install the Flutter extension from the VS Code marketplace. After installation, restart VS Code. The VS Code marketplace would be visually represented as a screen showing the Flutter extension, with its description and download button. The installation process is simple; it involves clicking the “Install” button and waiting for the download and installation to complete, followed by a prompt to restart the editor.
Emulator Setup or Physical Device Connection
Before running your Flutter app, you need an emulator or a physical device to test it on. For Android, you can use the Android Emulator included with Android Studio, or a third-party emulator like Genymotion. To connect a physical Android device, enable USB debugging in your device’s developer options. A visual representation of this would be a screenshot of an Android device’s settings menu, showing the “Developer options” section with the “USB debugging” toggle enabled.
For iOS, you’ll need a macOS machine and an Apple device connected via USB. You’ll also need to configure Xcode and the necessary iOS development tools. A visual would be a screenshot of Xcode, showing the device selection in the simulator or a physical device connected and ready for debugging. Remember to enable developer mode and any necessary settings on your iOS device.
After completing these steps, you are ready to start building your first Flutter app!
Core Flutter Widgets and Layouts
Building user interfaces in Flutter revolves entirely around widgets. Think of widgets as the fundamental building blocks of your app’s visual appearance. Every element you see—buttons, text, images, even the layout itself—is a widget. Understanding widgets and how they interact is crucial for creating effective and visually appealing mobile applications. This section dives into the core widgets and layout mechanisms that form the backbone of Flutter UI development.
Widgets in Flutter are arranged hierarchically, forming a widget tree. The root widget sits at the top, and all other widgets are nested beneath it. This tree structure dictates how the UI is rendered. Flutter’s declarative approach means you describe the UI you want, and Flutter handles the rendering and updates automatically. This makes building dynamic and responsive interfaces much easier.
Basic Widgets: Text and Image
The simplest widgets are those that display content directly. `Text` widgets render text strings, allowing for styling options like font size, color, and weight. `Image` widgets display images from various sources, including assets bundled with your app or fetched from a network. For example, a `Text` widget might be used to display a user’s name, while an `Image` widget could show their profile picture. These widgets form the foundation upon which more complex UIs are built. Consider the following code snippet illustrating the use of both:
Text(
'Hello, Flutter!',
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold),
),
Image.asset('assets/profile_picture.png'),
This code displays the text “Hello, Flutter!” in a bold, 24-point font, followed by an image loaded from the `assets` folder named `profile_picture.png`.
Layout Widgets: Row, Column, and Container
Flutter provides several widgets for arranging other widgets on the screen. `Row` widgets arrange children horizontally, while `Column` widgets arrange them vertically. `Container` widgets provide a way to add padding, margins, borders, and background colors to other widgets. They are essential for controlling the visual presentation and positioning of elements within your layout.
These layout widgets are fundamental for creating structured and organized UIs. Combining them strategically allows for creating complex layouts with ease.
- Row: Arranges widgets horizontally. Useful for creating horizontal lists or placing elements side-by-side.
- Column: Arranges widgets vertically. Ideal for creating lists, forms, or stacking elements on top of each other.
- Container: Provides styling and layout properties for a single child widget. Useful for adding padding, margins, background colors, and more.
Creating Responsive Layouts
Responsive layouts adapt to different screen sizes and orientations. Flutter’s flexible widget system makes this relatively straightforward. Using `Row` and `Column` widgets in combination with `Expanded` widgets (which allow widgets to take up available space) enables the creation of layouts that gracefully adjust to different screen sizes. For instance, a `Row` containing several `Expanded` widgets will distribute available space evenly among its children, ensuring elements remain appropriately sized regardless of screen width. Similarly, using `Column` with `Expanded` widgets can achieve the same effect for vertical layouts.
Consider a scenario where you have a list of items. On a larger screen, you might want to display them in a two-column grid using nested `Row` and `Column` widgets, while on a smaller screen, a single column layout would be more suitable. The `Expanded` widget allows for a seamless transition between these layouts based on available screen space. This dynamic adjustment ensures your app remains user-friendly across various devices.
State Management in Flutter
Flutter’s flexibility comes with a challenge: managing the ever-changing state of your application. As your app grows, keeping track of data and updating the UI efficiently becomes crucial. This is where state management solutions come in, providing structured ways to handle data flow and UI updates. Choosing the right approach depends on your app’s complexity and your team’s preferences.
Provider
Provider is a lightweight and relatively simple state management solution. It leverages Flutter’s built-in `InheritedWidget` to efficiently propagate changes throughout the widget tree. Its ease of use makes it a great choice for smaller to medium-sized projects. However, for very large and complex apps, its simplicity can become a limitation, leading to less organized code as the app scales. Provider’s strength lies in its intuitive approach and minimal boilerplate code.
BLoC (Business Logic Component)
BLoC is a more architectural approach to state management. It separates the business logic from the UI, using streams to manage data flow. This architectural separation promotes cleaner, more testable code. However, this separation also adds complexity, requiring a deeper understanding of streams and reactive programming. BLoC shines in complex apps where maintainability and testability are paramount, but it comes with a steeper learning curve.
Riverpod
Riverpod builds upon the Provider package, addressing some of its scalability limitations. It offers a more sophisticated approach to dependency injection and state management, making it suitable for larger projects. Riverpod provides improved performance and better error handling compared to Provider. While more powerful than Provider, it still retains a relatively straightforward learning curve compared to BLoC.
GetX
GetX is a relatively new but rapidly gaining popularity. It combines state management, routing, and dependency injection into a single package. This all-in-one approach simplifies development, but can lead to tight coupling within the application. While convenient for rapid prototyping and smaller projects, the tight coupling might become a hindrance in large-scale projects requiring greater flexibility and modularity.
Simple Counter App Implementation: Provider vs. BLoC, Building Cross-Platform Mobile Apps with Flutter
Let’s illustrate the difference by implementing a simple counter app using both Provider and BLoC.
Counter App with Provider
The Provider implementation would involve creating a `ChangeNotifier` class to manage the counter state and using `ChangeNotifierProvider` to make it accessible throughout the widget tree. UI updates would be triggered automatically whenever the counter value changes within the `ChangeNotifier`. The code would be relatively concise and easy to understand.
Counter App with BLoC
The BLoC implementation would involve creating a BLoC class with streams for the counter value and events to increment or decrement it. The UI would subscribe to these streams, updating automatically whenever a new value is emitted. This approach would involve more boilerplate code, but the separation of concerns would make the code more organized and testable.
State Management Solution Comparison
Feature | Provider | BLoC | Riverpod | GetX |
---|---|---|---|---|
Complexity | Low | High | Medium | Medium |
Scalability | Limited | Excellent | Excellent | Good |
Learning Curve | Easy | Steep | Moderate | Moderate |
Testability | Good | Excellent | Excellent | Good |
Working with APIs and Data Handling
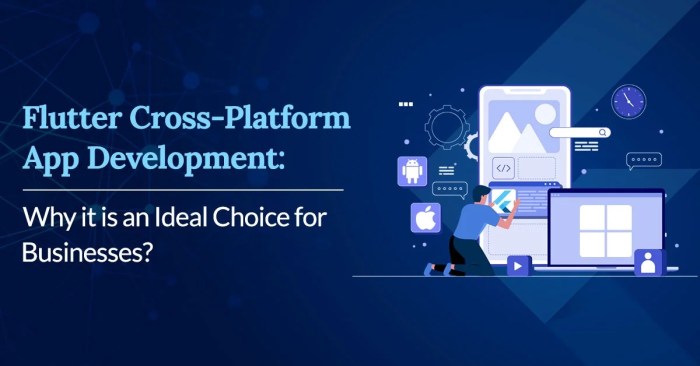
Source: eitbiz.com
Fetching and displaying data from external sources is a crucial aspect of building dynamic and engaging mobile applications. Flutter, with its rich ecosystem of packages, makes this process relatively straightforward. This section explores how to seamlessly integrate APIs into your Flutter projects, focusing on retrieving, parsing, and presenting data efficiently.
Interacting with APIs typically involves making HTTP requests to retrieve data in formats like JSON. Flutter provides the `http` package, a simple yet powerful tool for managing these requests. Once the data is fetched, it needs to be parsed (often from JSON) into Dart objects that can be used to populate your app’s UI. Error handling and asynchronous operations are key considerations to ensure a robust and user-friendly experience.
Fetching Data from REST APIs using the `http` package
The `http` package simplifies the process of making HTTP requests. To use it, you first need to add it as a dependency to your `pubspec.yaml` file and run `flutter pub get`. Then, you can use the `get` method to fetch data from a REST API endpoint. The response will be an instance of `Response` containing the HTTP status code and the body of the response. Successful requests (typically status codes in the 200 range) will have the data in the `body` property, usually as a JSON string.
For example, to fetch data from a hypothetical API endpoint `https://api.example.com/data`, you would use the following code:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future
final response = await http.get(Uri.parse('https://api.example.com/data'));
if (response.statusCode == 200)
return jsonDecode(response.body);
else
throw Exception('Failed to load data: $response.statusCode');
Parsing JSON Data
Once you’ve fetched data from an API, it’s usually in JSON format. Dart provides the `jsonDecode` function to parse this JSON string into a Dart object (usually a Map or List). This allows you to access the individual data points within the JSON response. You’ll need to understand the structure of the JSON response from your API to correctly access the necessary fields.
For instance, if the JSON response is a list of maps, each representing a product, you’d iterate through the list and access the properties of each product (e.g., name, price, description).
// Assuming 'data' is the decoded JSON from the fetchData function
List
for (var product in products)
String productName = product['name'];
double productPrice = product['price'];
// ... access other properties
Implementing a Simple Data Fetching Application
Let’s create a simple application that fetches a list of posts from a public API (e.g., JSONPlaceholder) and displays them in a ListView. This involves making an HTTP request, parsing the JSON response, and then using the data to populate the ListView’s items.
The application would consist of a `FutureBuilder` widget to handle the asynchronous operation of fetching data. The `FutureBuilder` will display a loading indicator while the data is being fetched, and then render the ListView once the data is available. Error handling would be implemented to gracefully handle network issues or API errors.
Best Practices for Handling Asynchronous Operations and Error Handling
Asynchronous operations, like API calls, are fundamental to building responsive apps. Using `async` and `await` s makes asynchronous code easier to read and maintain. Always wrap API calls in `try-catch` blocks to handle potential errors, such as network connectivity issues or API errors. Display informative error messages to the user to provide a better user experience. Consider using a state management solution (like Provider or BLoC) to manage the loading state and potential errors more effectively, separating data fetching logic from the UI.
Navigation and Routing
So you’ve built some awesome Flutter widgets, but how do you connect them? Navigation is the key to creating a fluid and engaging user experience in your mobile app. It’s about seamlessly moving users between different screens, providing a clear path through your application’s functionality. This section dives into the different techniques Flutter offers for managing navigation and routing, making your app feel polished and professional.
Flutter offers powerful tools to manage navigation between different screens or views within your application. The primary mechanism is the Navigator
widget, which manages a stack of routes. Each route represents a screen in your app. This stack-based approach allows for straightforward back-and-forth navigation, mirroring the natural flow of most mobile apps.
Using the Navigator Widget
The Navigator
widget is the core of Flutter’s navigation system. It manages a stack of routes, each representing a screen. Pushing a new route onto the stack displays the new screen, while popping a route removes it, returning the user to the previous screen. The simplest way to navigate is using Navigator.push
to push a new route and Navigator.pop
to return to the previous route.
For example, pushing a new route named ‘/secondScreen’ would look like this:
Navigator.pushNamed(context, '/secondScreen');
And popping the current route (returning to the previous screen):
Navigator.pop(context);
Named Routes
Instead of directly pushing routes using widgets, you can define named routes in your app’s routing table. This approach improves code readability and maintainability, especially in larger applications. Named routes allow you to reference screens by name, making your navigation logic more declarative. They’re defined in your app’s MaterialApp
widget using the routes
property.
Example of defining named routes:
MaterialApp(
title: 'My App',
routes:
'/': (context) => HomeScreen(),
'/secondScreen': (context) => SecondScreen(),
,
)
This defines two routes: ‘/’ for the home screen (HomeScreen
) and ‘/secondScreen’ for a second screen (SecondScreen
). You then navigate using Navigator.pushNamed
, referencing the route name.
Passing Data Between Screens Using Arguments
Often, you’ll need to pass data between screens. This is easily accomplished using the arguments
parameter in Navigator.pushNamed
. You pass data as an argument, and the receiving screen can access it through the ModalRoute.of(context)?.settings.arguments
property.
Example of passing data:
Navigator.pushNamed(context, '/secondScreen', arguments: 'name': 'John Doe');
And retrieving the data in the SecondScreen
:
final Map? arguments = ModalRoute.of(context)?.settings.arguments as Map?;
final String? name = arguments?['name'];
Creating a Multi-Screen Application
Let’s imagine a simple app with a home screen and a details screen. The home screen displays a list of items, and tapping an item navigates to the details screen showing that item’s information. The home screen would push the details screen using Navigator.pushNamed
, passing the item’s data as an argument. The details screen would receive the data and display it. The user could then navigate back to the home screen using the system back button or Navigator.pop
.
Bottom Navigation Bar Implementation
Bottom navigation bars are a common pattern for apps with a few main sections. Flutter’s BottomNavigationBar
widget makes implementing this straightforward. Each item in the BottomNavigationBar
represents a different screen, and tapping an item navigates to the corresponding screen. You would typically manage the currently selected screen using state management (like Provider or Riverpod) and conditionally render the appropriate screen based on the selected index.
A BottomNavigationBar
could be created with three items, each representing a different section of the app (e.g., Home, Profile, Settings). Switching between these sections would involve updating the state variable that controls which screen is displayed, triggering a rebuild of the UI to show the appropriate screen.
Building cross-platform mobile apps with Flutter offers speed and efficiency, letting developers reach wider audiences faster. But even with the best tech, life throws curveballs; securing your family’s future is key, especially if you’re a parent. That’s why understanding the importance of financial planning, like reading up on The Benefits of Life Insurance for Parents with Young Children , is crucial.
Back to Flutter, remember that a solid app strategy should also encompass financial stability for its creators.
Drawer Navigation Implementation
Drawer navigation provides a side menu for accessing different parts of your application. Flutter’s Scaffold
widget includes a drawer
property for easily implementing this. The drawer contains a list of menu items, each navigating to a different screen within the app. Similar to bottom navigation, you’d manage the selected screen using state management to control which screen is shown.
A typical drawer might contain options for Home, About, Settings, and perhaps a logout button. Tapping any of these options would navigate to the corresponding screen and close the drawer.
Testing Flutter Applications: Building Cross-Platform Mobile Apps With Flutter
Building robust and reliable Flutter apps isn’t just about pretty UIs and smooth animations; it demands a rigorous testing strategy. Thorough testing ensures your app functions correctly across different devices and handles various user interactions without crashing or exhibiting unexpected behavior. This section dives into the essential testing approaches in Flutter, equipping you with the knowledge to build high-quality, dependable mobile applications.
Unit Testing in Flutter
Unit tests focus on isolating the smallest testable parts of your code – individual functions, methods, or classes – and verifying their behavior in isolation. This approach allows for quick feedback and easy debugging, making it crucial for catching errors early in the development process. In Flutter, you can use the `flutter_test` package to write unit tests for your business logic and smaller widgets. A well-structured unit test should have clear setup, execution, and assertion phases. For example, a unit test for a function calculating the total price of items in a shopping cart would verify the correct calculation for various input scenarios, including empty carts, single items, and multiple items with different prices. This granular approach helps identify and fix bugs before they propagate to larger components of your application.
Widget Testing in Flutter
Widget tests are used to verify the UI behavior of your Flutter widgets. Unlike unit tests, widget tests render and interact with the actual widgets, allowing you to check if the UI behaves as expected. These tests are essential for ensuring the visual correctness and responsiveness of your app. For instance, a widget test for a button might verify that tapping the button triggers the expected action and that the button’s appearance changes appropriately when pressed or disabled. The `flutter_test` package provides tools to interact with widgets, such as finding widgets by their type or key, and simulating user interactions like taps and gestures.
Integration Testing in Flutter
Integration tests examine the interaction between different parts of your application. They go beyond testing individual units or widgets and verify the integrated behavior of multiple components working together. This is crucial for ensuring that different parts of your app communicate and interact correctly. For example, an integration test might verify the flow of data from a network request through your data layer and into the UI. These tests are more complex than unit or widget tests, but they are vital for ensuring the overall functionality and stability of your app. They often involve simulating user flows and checking for the expected outcomes across multiple screens or components.
Best Practices for Writing Effective Flutter Tests
Writing effective and maintainable tests requires careful planning and adherence to best practices. Well-structured tests are easier to understand, maintain, and debug. Consider these points:
- Keep tests concise and focused: Each test should verify a single aspect of your code’s behavior. Avoid creating tests that try to verify too many things at once.
- Use descriptive test names: Test names should clearly communicate what is being tested. This improves readability and maintainability.
- Use proper assertions: Use the appropriate assertion methods to verify the expected outcomes. The `expect()` method in `flutter_test` is commonly used.
- Write tests first (Test-Driven Development): Writing tests before writing the code helps clarify requirements and ensures that the code meets those requirements.
- Organize tests effectively: Group tests logically by functionality or component to improve maintainability.
Deployment and Publishing
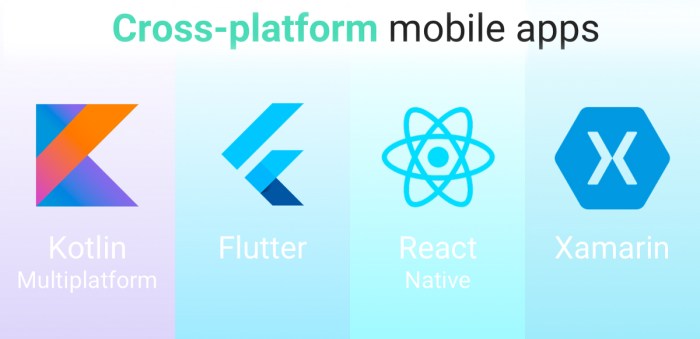
Source: premware.services
Getting your Flutter app ready for the world involves more than just writing the code. Deployment and publishing to the Apple App Store and Google Play Store require careful preparation, adherence to platform guidelines, and a methodical approach. This section Artikels the key steps involved in this crucial final phase of app development.
Preparing your app for release isn’t just about submitting the code; it’s about ensuring a polished and professional user experience. This includes optimizing the app’s performance, addressing potential bugs, and creating compelling marketing assets. A well-prepared app significantly increases the chances of successful adoption and positive user reviews.
App Store Connect and Google Play Console Setup
Before you can even think about uploading your app, you’ll need accounts on Apple’s App Store Connect and Google’s Play Console. These platforms handle the submission, review, and distribution of your app. You’ll need to register as a developer, provide necessary information about your app, and pay the relevant annual fees. The process involves creating developer profiles, agreeing to terms of service, and providing banking details for payment processing. Thoroughly review the requirements and guidelines for both platforms to ensure a smooth process.
Generating Release Builds
Once your app is thoroughly tested and ready for deployment, you need to generate release builds. These builds are optimized for size and performance, unlike debug builds used during development. Flutter provides tools to generate these builds for both iOS and Android. The process typically involves running specific commands in your terminal, selecting the appropriate build type (release), and specifying any necessary build configurations. The resulting `.ipa` file for iOS and `.apk` file for Android are ready for submission to their respective app stores.
Creating App Icons and Splash Screens
First impressions matter. Your app’s icon and splash screen are crucial for grabbing users’ attention. Both platforms have specific guidelines regarding icon sizes and formats. You’ll need to create multiple versions of your app icon, ranging from small sizes used in app lists to larger sizes for the app’s home screen. Similarly, the splash screen, the screen displayed while the app loads, should be visually appealing and consistent with your app’s branding. Many designers use vector graphics for icons to ensure they scale properly across various screen resolutions. For example, a well-designed icon might use a combination of sharp lines and vibrant colors to communicate the app’s core functionality instantly.
Preparing App Metadata
App store listings require comprehensive metadata, including descriptions, screenshots, s, and privacy policies. This metadata plays a critical role in app discovery. Write clear, concise, and engaging descriptions that highlight your app’s key features and benefits. Use relevant s to improve searchability. High-quality screenshots and videos showcasing your app’s functionality are essential to attract potential users. For instance, a detailed description might emphasize the app’s speed, user-friendliness, and unique selling points. Similarly, high-quality screenshots should visually demonstrate these features in action.
Deployment Checklist
A well-structured checklist ensures a smooth deployment process. Before submitting, verify that you’ve completed all the necessary steps. This includes creating and submitting your app’s metadata, generating the release builds for both platforms, and ensuring compliance with all platform guidelines. Testing the release builds on various devices is also critical to identify any last-minute issues. Regularly reviewing the guidelines and requirements of both the Apple App Store and Google Play Store is essential, as they are subject to change. A pre-submission checklist, carefully followed, dramatically reduces the risk of delays or rejections.
Closing Notes
Building cross-platform mobile apps shouldn’t feel like scaling Mount Everest. Flutter makes it surprisingly smooth, letting you focus on the innovation, not the infrastructure. From its intuitive widget system to robust state management solutions, Flutter empowers you to create truly amazing apps faster and more efficiently than ever before. So ditch the platform-specific struggles and embrace the future of mobile development – the Flutter way.