How to Integrate Third-Party Services into Your Web Application? Level up your web app game! This isn’t just about slapping on a payment gateway; it’s about strategically choosing the right tools to boost functionality, security, and user experience. We’re diving deep into the nitty-gritty, from selecting the perfect services to mastering API integration and securing your data. Get ready to transform your web app from basic to brilliant.
We’ll cover everything from understanding different API integration methods (REST, GraphQL, SOAP) and secure authentication (OAuth 2.0, OpenID Connect, JWT) to handling errors gracefully and building a robust, scalable application. We’ll even tackle the often-overlooked aspects like data transformation, thorough testing, and ongoing monitoring. Think of this as your ultimate guide to building a web app that’s not just functional, but future-proof.
Choosing the Right Third-Party Service
Integrating third-party services can supercharge your web application, adding features and functionality without reinventing the wheel. But choosing the wrong service can lead to headaches, security vulnerabilities, and ultimately, a less-than-stellar user experience. So, picking the right partner is crucial. Think of it like choosing a bandmate – you want someone reliable, skilled, and who fits seamlessly into your overall sound.
Selecting a third-party service requires careful consideration of several key factors. Ignoring these factors can lead to integration nightmares and potentially compromise your application’s security and performance. It’s all about finding the perfect fit for your specific needs and budget.
Factors to Consider When Selecting a Third-Party Service
Before diving into specific services, let’s Artikel the essential criteria for evaluation. This framework will help you navigate the plethora of options available and make an informed decision.
- Functionality and Features: Does the service offer the exact features you need? Consider future scalability and whether the service can grow with your application.
- Security: What security measures does the service employ? Are their security practices transparent and auditable? How do they handle data privacy and compliance with regulations like GDPR or CCPA?
- Scalability and Reliability: Can the service handle your current and projected user load? What’s their uptime guarantee and how do they handle outages?
- Pricing and Support: What’s the pricing model (subscription, pay-as-you-go, etc.)? What level of support do they offer? Is it readily accessible and responsive?
- Integration Complexity: How easy is it to integrate the service into your existing application? Is there comprehensive documentation and readily available support?
- API Documentation and Support: Thorough API documentation is essential for smooth integration. The service provider should offer robust documentation, tutorials, and readily available support channels.
Comparison of Popular Third-Party Services
Let’s compare some popular services across three key areas: authentication, payment processing, and analytics. This table provides a snapshot; thorough research is still essential before making a decision.
Service Name | Key Features | Pricing Model | Integration Complexity |
---|---|---|---|
Auth0 (Authentication) | Multi-factor authentication, social logins, customizability | Subscription-based, tiered pricing | Moderate (well-documented APIs) |
Firebase Authentication (Authentication) | Email/password, phone auth, social logins, easy integration with other Firebase services | Pay-as-you-go, based on usage | Easy (excellent integration with other Firebase services) |
Stripe (Payment Processing) | Wide range of payment methods, fraud prevention tools, recurring billing | Percentage-based fees per transaction | Moderate (well-documented APIs) |
PayPal (Payment Processing) | Widely recognized, supports various payment methods, buyer protection | Percentage-based fees per transaction | Easy (various SDKs and plugins available) |
Google Analytics (Analytics) | Comprehensive website analytics, real-time reporting, custom dashboards | Free (with limitations), paid version for advanced features | Easy (JavaScript tracking code) |
Mixpanel (Analytics) | Event tracking, user segmentation, A/B testing, cohort analysis | Subscription-based, tiered pricing | Moderate (APIs and SDKs available) |
Security Implications of Integrating Third-Party Services
Integrating third-party services introduces security considerations that require careful attention. A breach in a third-party service could potentially expose your application’s data or compromise user accounts. Proactive mitigation strategies are essential.
- Thorough Vendor Due Diligence: Carefully vet potential vendors, examining their security practices, certifications (e.g., SOC 2), and incident response plans.
- Secure API Integration: Use secure communication protocols (HTTPS) and implement robust authentication and authorization mechanisms to protect your API keys and sensitive data.
- Input Validation and Sanitization: Always validate and sanitize user inputs before passing them to third-party services to prevent injection attacks.
- Regular Security Audits: Conduct regular security audits of your integrated services to identify and address potential vulnerabilities.
- Monitoring and Alerting: Implement monitoring and alerting systems to detect suspicious activity and promptly respond to security incidents.
API Integration Methods
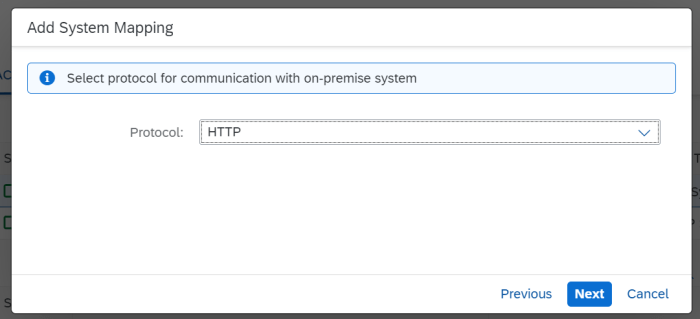
Source: sap.com
So, you’ve chosen your third-party service. Now comes the fun (and sometimes frustrating) part: integrating it into your web application. This involves using Application Programming Interfaces, or APIs, to connect your application with the external service. Think of it like building a bridge – you need the right tools and techniques to ensure a smooth and stable connection. This section will explore some common API integration methods and guide you through the process.
APIs act as intermediaries, allowing your application to communicate and exchange data with the third-party service without needing to know the internal workings. Several methods exist, each with its strengths and weaknesses. Choosing the right method depends on factors like the complexity of your application, the type of data being exchanged, and the capabilities of the third-party service.
RESTful APIs
REST (Representational State Transfer) is the most popular API architecture. It uses standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. This simplicity and widespread adoption make it a go-to choice for many developers. REST APIs typically return data in formats like JSON or XML, which are easy to parse and use in web applications. A major advantage is its ease of use and broad tooling support. However, over-fetching data (retrieving more than needed) can be a drawback, and complex queries might require multiple requests. Think of it as ordering a la carte – you get exactly what you ask for, but might need multiple orders for a complete meal.
GraphQL APIs
GraphQL offers a more flexible approach. Unlike REST, which defines endpoints for specific resources, GraphQL allows you to request precisely the data you need in a single query. This reduces over-fetching and under-fetching, optimizing data transfer. GraphQL uses a schema to define the available data and its relationships, providing strong typing and improved developer experience. Its power lies in its efficiency and precision; however, it requires a more complex setup and might have a steeper learning curve than REST. Imagine it as a buffet – you select exactly the dishes you want, avoiding unnecessary items.
SOAP APIs
SOAP (Simple Object Access Protocol) is an older, more formal approach to API integration. It relies on XML for both data exchange and messaging, using standards like WSDL (Web Services Description Language) for defining the API’s interface. SOAP is known for its robustness and security features, making it suitable for critical applications. However, its verbosity and complexity can make it less efficient and harder to implement compared to REST or GraphQL. It’s like a formal, multi-course dinner – everything is meticulously planned and presented, but it can be quite elaborate.
Integrating a Payment Gateway using a RESTful API: A Step-by-Step Guide
Let’s walk through integrating a payment gateway using a RESTful API. This example uses a hypothetical payment gateway for illustrative purposes. Remember to always consult the specific documentation of your chosen payment gateway.
- Obtain API Credentials: Sign up for an account with your chosen payment gateway and obtain your API keys and other necessary credentials. These are essential for authentication and authorization.
- Understand the API Documentation: Thoroughly review the payment gateway’s API documentation. This will detail the available endpoints, request formats (e.g., JSON), and response structures.
- Set up the Development Environment: Choose a suitable programming language (like Python or JavaScript) and set up your development environment. You’ll likely need libraries or SDKs for making HTTP requests.
- Create an API Request: Construct an HTTP POST request to the payment gateway’s API endpoint for processing payments. The request body should include details such as the amount, currency, customer information, and other relevant data, formatted according to the API documentation. Example using Python’s `requests` library:
import requestsurl = "https://api.examplepaymentgateway.com/payments"
payload =
"amount": 100.00,
"currency": "USD",
"customer_name": "John Doe",
# ... other relevant dataheaders =
"Authorization": "Bearer YOUR_API_KEY"response = requests.post(url, json=payload, headers=headers)
print(response.json())
- Handle the API Response: Process the response from the payment gateway. A successful transaction will typically return a confirmation code or transaction ID. Handle errors appropriately, providing user-friendly messages.
- Implement Security Measures: Never expose your API keys directly in your client-side code. Use server-side processing to protect sensitive information.
- Testing and Deployment: Thoroughly test your integration in a staging environment before deploying to production. This will help prevent errors and ensure a smooth user experience.
Authentication and Authorization: How To Integrate Third-Party Services Into Your Web Application
Integrating third-party services seamlessly into your web application is crucial for expanding functionality and enhancing user experience. However, this integration often involves sensitive user data, making robust authentication and authorization mechanisms absolutely essential. Failing to properly secure these aspects can lead to devastating security breaches and compromise your users’ trust. Let’s dive into the critical elements of securing your application.
Authentication verifies the identity of a user or service, while authorization determines what actions that authenticated entity is permitted to perform. Think of it like this: authentication is like showing your ID to get into a building; authorization is like having the right keycard to access specific floors or rooms. Both are crucial for maintaining security.
OAuth 2.0
OAuth 2.0 is a widely adopted authorization framework that allows users to grant third-party applications access to their resources without sharing their credentials. Instead of directly handing over passwords, users authorize the application to access specific data on their behalf. This is particularly useful for services like Google, Facebook, and Twitter, where you might want to log in using your existing accounts without revealing your password to the new application. Different grant types exist within OAuth 2.0, each tailored to different use cases, like authorization codes for web applications or client credentials for server-to-server communication.
OpenID Connect
OpenID Connect (OIDC) builds upon OAuth 2.0 to provide an authentication layer. While OAuth 2.0 focuses on authorization, OIDC adds the ability to verify a user’s identity and obtain basic profile information. This means that after successfully authenticating with an identity provider (like Google or Auth0), the application can receive a token containing verified user information, simplifying the authentication process. OIDC significantly streamlines user logins and improves security by relying on established identity providers to handle the complex authentication tasks.
JSON Web Tokens (JWT)
JWTs are a compact and self-contained way to securely transmit information between parties as a JSON object. This information is digitally signed, ensuring its integrity and authenticity. A JWT typically consists of three parts: a header, a payload (containing claims about the user), and a signature. The signature verifies that the token hasn’t been tampered with. JWTs are often used to represent the user’s session after successful authentication, enabling stateless authentication—meaning the server doesn’t need to store session data, improving scalability and security.
Secure Authorization Practices
Implementing secure authorization practices is paramount to safeguarding user data. The principle of least privilege should be strictly adhered to: grant users only the necessary permissions to perform their tasks. Regularly review and update access control lists to ensure they remain aligned with the evolving needs of the application. Input validation is crucial to prevent malicious code injection. Robust logging and monitoring systems can help detect and respond to unauthorized access attempts promptly. Furthermore, employing strong encryption techniques to protect sensitive data both in transit and at rest is essential.
Secure Token Handling with JWT
The following code snippet demonstrates a simplified example of secure JWT handling in a Node.js application using the `jsonwebtoken` library. Remember to replace `”your-secret-key”` with a strong, randomly generated secret key.
“`javascript
const jwt = require(‘jsonwebtoken’);// Generate a JWT
const token = jwt.sign( userId: 123, username: ‘john.doe’ , ‘your-secret-key’);// Verify a JWT
jwt.verify(token, ‘your-secret-key’, (err, decoded) =>
if (err)
console.error(“Token verification failed:”, err);
else
console.log(“Token verified successfully:”, decoded););
“`
This example shows basic JWT creation and verification. In a real-world application, you’d integrate this with your authentication system and handle potential errors more robustly. Remember that storing the secret key securely is crucial. Never hardcode it directly in your application code; use environment variables or a secure secrets management service.
Handling Errors and Exceptions
Integrating third-party services is rarely a smooth, uninterrupted journey. Expect hiccups. The reality is that APIs can throw errors, networks can fail, and unexpected data can wreak havoc. Robust error handling isn’t just a nice-to-have; it’s the backbone of a resilient application. This section explores how to gracefully manage these inevitable issues, ensuring a positive user experience even when things go wrong.
Effective error handling goes beyond simply catching exceptions. It involves anticipating potential problems, designing strategies for recovery, and providing users with clear, helpful messages. A well-designed error handling mechanism should improve the overall stability and reliability of your application, minimizing disruptions and improving the overall user experience. Ignoring error handling can lead to crashes, data loss, and frustrated users—definitely not a recipe for success.
Common Error Scenarios
Several common scenarios can cause problems when integrating third-party services. Understanding these potential pitfalls allows you to proactively build in safeguards.
Mastering third-party service integration in your web app is key to scalability and functionality. But remember, security breaches can be costly, especially if you’re handling sensitive data – that’s why understanding the importance of robust insurance is vital, as highlighted in this article: Why Insurance for High-Risk Businesses Is Crucial. Proper insurance safeguards your business against potential liabilities, allowing you to focus on seamless third-party integration and overall app development.
- Network Errors: These are among the most frequent issues. A temporary network outage, slow connection, or DNS resolution problems can prevent your application from reaching the third-party service.
- API Errors: The third-party API itself might return error codes indicating problems like invalid requests, rate limiting, or server-side issues. These often come with detailed error messages that can help in debugging.
- Data Errors: The data received from the API might be malformed, incomplete, or contain unexpected values. Your application needs to be able to handle such inconsistencies without crashing.
- Authentication and Authorization Failures: Problems with API keys, tokens, or user permissions can prevent access to the third-party service. These often require specific handling to guide the user toward resolution.
- Rate Limiting: Exceeding the allowed number of requests to the API within a specific time frame can result in temporary blocks. Your application needs mechanisms to handle these limitations gracefully, perhaps by queuing requests or implementing exponential backoff.
Strategies for Graceful Error Handling and User Feedback
Providing informative and helpful feedback to the user is crucial when errors occur. Avoid cryptic technical messages; instead, translate errors into user-friendly language.
- Clear Error Messages: Instead of “API request failed,” use something like “We’re having trouble connecting to the service. Please try again later.”
- Contextual Information: If possible, provide the user with context about the error. For example, if a payment failed, specify the reason (“Insufficient funds” or “Invalid card details”).
- Retry Mechanisms: For transient errors (like network hiccups), implement automatic retry logic with exponential backoff. This avoids overwhelming the user with repeated failure messages.
- Fallback Mechanisms: If the third-party service is unavailable, provide a fallback mechanism. This might involve using cached data, displaying a default message, or offering a degraded version of the functionality.
Robust Error Handling Mechanism: Logging, Retry Logic, and Fallbacks
A robust error handling mechanism combines several techniques to ensure resilience and maintainability.
Logging: Thorough logging is essential for debugging and monitoring. Log both successful and failed API calls, including timestamps, request parameters, response codes, and error messages. This data is invaluable for identifying patterns, diagnosing problems, and improving the system’s reliability.
Retry Logic: Implement a retry mechanism that attempts to repeat failed requests after a short delay. Use exponential backoff to avoid overwhelming the third-party service. For example, the first retry might wait 1 second, the second 2 seconds, the third 4 seconds, and so on. This strategy is especially helpful for transient network errors.
Fallback Mechanisms: Design fallback mechanisms to handle situations where the third-party service is unavailable. This might involve using cached data, displaying a default message, or providing a degraded version of the functionality. This ensures that the application remains functional even when external dependencies are down. For example, if a map service is unavailable, you might display a simple text address instead of an interactive map.
Data Mapping and Transformation
Integrating third-party services often involves a crucial step: aligning your application’s data structure with that of the external service. This isn’t always a straightforward process, as different services use varying data formats and schemas. Effective data mapping and transformation ensure seamless data exchange and prevent integration headaches down the line.
Data mapping involves identifying corresponding fields between your application’s data model and the third-party service’s API. This requires a careful comparison of both structures to establish clear relationships. Transformation, on the other hand, focuses on converting data from one format to another – for instance, restructuring JSON objects or converting XML to a more suitable format for your application. This often involves data type conversions, data cleaning, and potentially even data enrichment.
JSON and XML Data Transformation Examples
Let’s illustrate data transformation with examples using JSON and XML. Imagine you’re integrating with a payment gateway that expects data in a specific JSON format. Your application, however, might store the same information in a different structure. Consider the following scenario:
Your application stores customer data like this (JSON):
"customerID": 123,
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"address":
"street": "123 Main St",
"city": "Anytown",
"state": "CA",
"zip": "90210"
The payment gateway, however, requires this format:
"id": 123,
"name": "John Doe",
"email": "[email protected]",
"billingAddress": "123 Main St, Anytown, CA 90210"
To integrate, you’d need to transform your application’s JSON into the gateway’s required format. This would involve concatenating the address fields, renaming fields (“customerID” to “id”, “firstName” and “lastName” to “name”), and potentially using a different field name for the address.
Similarly, with XML, you might need to parse an XML response from a service and extract relevant information, potentially converting it to a JSON object or a custom data structure for easier handling within your application. This would involve using XML parsing libraries to navigate the XML tree and extract the needed data points.
Data Flow Illustration
A visual representation of the data flow can be helpful. Imagine a flowchart with three main boxes:
Box 1: Your Application. This box represents your application’s data structure and processes.
Box 2: Transformation Logic. This is the central part of the process, showing the conversion from your application’s data format to the third-party service’s format. This could involve various transformations as described earlier.
Box 3: Third-Party Service. This box represents the third-party service and its expected data format. Arrows connecting the boxes illustrate the data flow from your application, through the transformation logic, and finally to the third-party service. The arrows could be labeled to indicate the data format at each stage. This visual helps clarify the steps involved and simplifies the understanding of the data transformation process.
Testing and Deployment
Integrating third-party services is like adding a new ingredient to a complex recipe – it needs careful testing to ensure it doesn’t spoil the whole dish. Thorough testing prevents unexpected behavior in production and ensures a smooth user experience. This phase is crucial for preventing costly downtime and maintaining a positive user perception of your application.
Deploying your application after successful testing is the final step, and just as critical as the integration itself. A well-planned deployment minimizes disruption and ensures a seamless transition to a live environment.
Testing Strategies
Effective testing involves a multi-pronged approach. You need to verify the functionality of the integrated service, the reliability of the connection, and the security of the data exchange. Unit tests focus on individual components, while integration tests assess the interaction between different parts of your system, including the third-party service. End-to-end tests simulate real-world scenarios to ensure everything works as expected from a user’s perspective. Load testing is also crucial to assess the system’s performance under stress, identifying potential bottlenecks.
Pre-Production Testing Checklist
Before releasing your application to the public, a rigorous pre-production testing phase is essential. This involves a series of checks to ensure stability and functionality.
- Unit Tests: Verify individual components of the integration work correctly in isolation.
- Integration Tests: Test the interaction between your application and the third-party service.
- End-to-End Tests: Simulate real-world user scenarios to ensure the entire system functions as expected.
- Security Tests: Check for vulnerabilities in the integration, ensuring data is transmitted securely.
- Performance Tests: Assess response times and identify potential bottlenecks under various load conditions.
- Regression Tests: Verify that new features or bug fixes haven’t introduced new problems into existing functionality.
Post-Production Testing Checklist
Even after deployment, monitoring and testing continue. This phase focuses on identifying issues that may only surface under real-world conditions.
- Monitoring: Continuously monitor key metrics such as response times, error rates, and resource utilization.
- User Feedback: Gather user feedback to identify potential issues or areas for improvement.
- A/B Testing: Compare different versions of the integration to determine which performs better.
- Performance Tuning: Optimize the integration based on monitoring data and user feedback.
Deployment Process
Deploying your application to production involves a structured approach to minimize disruption and ensure a smooth transition. This often involves a staged rollout, starting with a small subset of users before expanding to the entire user base. Continuous integration and continuous delivery (CI/CD) pipelines automate the build, testing, and deployment process, improving efficiency and reducing the risk of errors.
A robust CI/CD pipeline is key to efficient and reliable deployment.
For example, a company like Netflix uses a sophisticated CI/CD pipeline to deploy thousands of code changes daily. Their system allows them to roll back changes quickly if problems arise, minimizing downtime and ensuring a smooth user experience. This is a prime example of how a well-structured deployment process can significantly impact the success of an application.
Monitoring and Maintenance
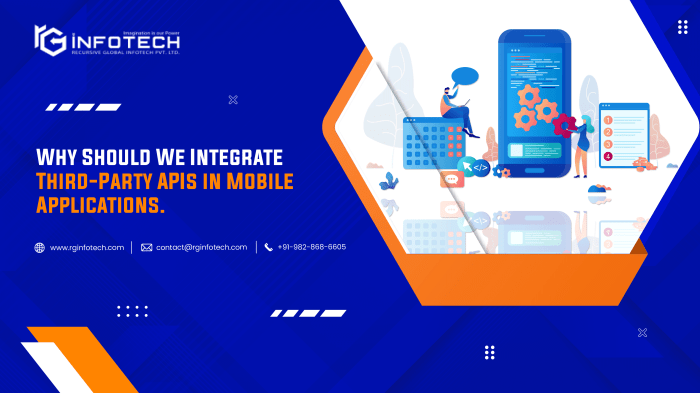
Source: rginfotech.com
Integrating third-party services boosts your web app’s functionality, but it also introduces new points of potential failure. Ignoring the health and performance of these integrations is like ignoring a ticking time bomb – eventually, it’ll explode, impacting your users and your bottom line. Proactive monitoring is key to preventing these disasters and ensuring a smooth, reliable user experience.
Think of it like this: you wouldn’t drive a car without checking the oil and tire pressure, right? Similarly, you can’t just assume your third-party integrations are running flawlessly. Regular monitoring gives you the visibility you need to identify and address problems *before* they escalate.
Key Performance Indicators (KPIs) for Integrated Services
Tracking the right metrics is crucial for understanding the health of your integrations. Ignoring important indicators is like flying blind – you might reach your destination, but the journey will be significantly riskier. Here are some essential KPIs to monitor:
- API Response Times: How long does it take for the third-party service to respond to your requests? Slow response times directly impact user experience, leading to frustration and potentially lost customers. Aim for response times under 200 milliseconds for optimal performance; anything over a second can be noticeable to users.
- Error Rates: How often do API calls fail? High error rates indicate problems with the integration, the third-party service, or even your own code. Tracking error types helps pinpoint the root cause.
- Success Rates: Conversely, monitor the percentage of successful API calls. This metric provides a direct measure of the reliability of your integration.
- Data Volume: How much data is being exchanged between your application and the third-party service? Sudden spikes or drops in data volume can signal issues. This is especially important for services handling user data or transactions.
- Service Availability: Is the third-party service itself up and running? Many services provide status pages or APIs to check their uptime. Integrate this into your monitoring system to be alerted to potential outages.
Designing a Monitoring Dashboard
A well-designed monitoring dashboard provides a centralized view of your integrated services’ health. Imagine it as your control panel, providing real-time insights into the performance of your entire system. A good dashboard should:
- Visualize Key Metrics: Use charts and graphs to display API response times, error rates, and other KPIs. Color-coding can highlight critical issues immediately.
- Provide Real-time Updates: The dashboard should refresh frequently to give you an up-to-the-minute view of your integrations’ performance. A refresh rate of every 5-15 seconds is a good starting point.
- Offer Alerting Capabilities: Set thresholds for critical metrics (e.g., if API response time exceeds 500ms, send an alert). This ensures you’re notified of problems immediately.
- Include Service Status Indicators: Clearly display the status of each integrated service (e.g., green for operational, yellow for degraded performance, red for outage).
- Enable Drill-Down Capabilities: Allow users to click on specific metrics to see more detailed information, such as error logs or individual API call performance.
For example, a dashboard might display a graph showing API response times over the past 24 hours, with color-coded alerts triggered if response times exceed a predefined threshold. Another section might show a table summarizing the error rates for each integrated service, with links to detailed error logs. This allows for rapid identification and resolution of issues. A simple example might be a dashboard displaying a green light for a payment gateway with an average response time of 150ms and an error rate of 0.1%, and a red light for a mapping service with an average response time of 1000ms and an error rate of 5%, immediately signaling the need for attention.
Security Best Practices
Integrating third-party services boosts functionality, but it also expands your attack surface. Ignoring security best practices can lead to data breaches, compromised user accounts, and reputational damage – basically, a total buzzkill for your web app. Let’s dive into how to keep your app safe and sound.
Third-party integrations introduce several potential security vulnerabilities. These vulnerabilities can range from insecure API keys exposed in your code to vulnerabilities within the third-party service itself. A common attack vector is exploiting weaknesses in the authentication and authorization mechanisms used to communicate with these services. Another concern is the potential for data leakage if sensitive information isn’t handled correctly during transmission and storage. Failing to properly validate and sanitize data received from third-party APIs can also lead to injection attacks.
API Key Management
Protecting your API keys is paramount. Think of them as the keys to your kingdom; losing them is a major security breach. Hardcoding API keys directly into your code is a big no-no. Instead, leverage environment variables or dedicated secrets management services. These services encrypt and securely store your keys, providing access only through secure mechanisms. Regularly rotate your API keys to minimize the impact of any potential compromise. Consider using short-lived access tokens instead of long-lived API keys whenever possible. This limits the damage if a token is compromised.
Sensitive Data Handling, How to Integrate Third-Party Services into Your Web Application
Handling sensitive user data, such as credit card information or personally identifiable information (PII), requires extra vigilance. Always encrypt data both in transit (using HTTPS) and at rest (using database encryption). Comply with relevant data privacy regulations like GDPR and CCPA. Minimize the amount of sensitive data you collect and store, only retaining what’s absolutely necessary for your application’s functionality. Implement robust access controls to limit who can access and modify sensitive data.
Security Considerations During Development and Deployment
Before launching your application, a comprehensive security review is crucial. This involves code analysis to identify potential vulnerabilities, penetration testing to simulate real-world attacks, and security audits to assess your overall security posture. Consider using a Web Application Firewall (WAF) to protect your application from common web attacks. Regularly update your dependencies to patch known vulnerabilities in third-party libraries and frameworks. Implement robust logging and monitoring to detect and respond quickly to security incidents. A well-defined incident response plan is also essential to minimize the impact of any security breaches. Employ code review practices, including peer reviews and automated static analysis, to detect and mitigate potential security flaws.
Final Review
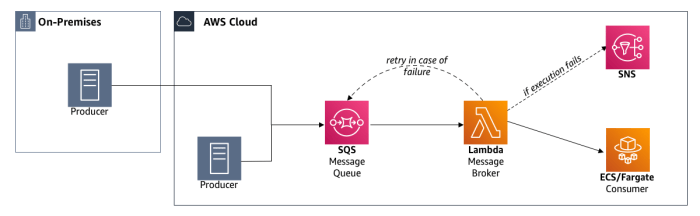
Source: cloudfront.net
Integrating third-party services is more than just adding features; it’s about building a smarter, more efficient, and ultimately more successful web application. By carefully selecting services, mastering API integration, prioritizing security, and implementing robust error handling, you can unlock a world of possibilities. Remember, continuous monitoring and adaptation are key to long-term success. So go forth, and build something amazing!