How to Build a Real-Time Notification System in Web Applications? It’s a question buzzing in the minds of every developer aiming for that slick, modern user experience. Real-time notifications – those little bursts of info that keep users hooked – aren’t just a nice-to-have; they’re a must-have for engaging apps. This guide dives deep into building your own, exploring the tech behind the magic, from WebSockets to clever client-side handling, and even tackling the scaling challenges that come with a truly popular app. Get ready to level up your notification game.
We’ll unpack the core components, compare different technologies (WebSockets, Server-Sent Events, and polling), and walk you through a practical implementation using WebSockets. We’ll cover security, optimization, and best practices for displaying notifications without annoying your users. By the end, you’ll be equipped to craft a notification system that’s both powerful and user-friendly.
Introduction to Real-Time Notification Systems
Real-time notification systems are the unsung heroes of a smooth, engaging user experience in today’s web applications. They’re the reason you know instantly when you’ve received a new message, a friend has liked your post, or a crucial system update has been deployed. Think of them as the digital equivalent of a persistent, ever-vigilant messenger, keeping you in the loop without you having to constantly refresh your screen.
Real-time notifications enhance user engagement and streamline communication by delivering information instantly. This immediacy fosters a more dynamic and interactive user experience, ultimately leading to increased user satisfaction and loyalty. The key is delivering the right information, to the right user, at the right time.
Core Components of Real-Time Notification Systems
A robust real-time notification system typically consists of several interconnected parts. First, you need a client-side component, usually JavaScript running within the web browser, responsible for receiving and displaying notifications. Next, a server-side component handles notification management, including sending, queuing, and managing the notification queue. A crucial element is a communication channel, which facilitates real-time, bidirectional communication between the client and server. Finally, a database is essential for storing notification data and user preferences.
Benefits of Real-Time Notifications in Web Applications
Implementing real-time notifications offers significant advantages. Increased user engagement is a primary benefit – instant updates keep users actively involved. Improved communication allows for immediate feedback and streamlined interactions. Enhanced user experience results in higher satisfaction and loyalty. Finally, real-time notifications can drive conversions by promptly alerting users to relevant information, such as time-sensitive offers or important updates.
Types of Real-Time Notifications
Several types of real-time notifications cater to different needs. Push notifications, delivered even when the application isn’t actively open, are excellent for urgent alerts. In-app alerts, appearing within the application itself, are ideal for less critical updates. Toast notifications, brief messages that appear temporarily, provide quick feedback without disrupting the user’s workflow. The choice depends on the notification’s urgency and the context of the application.
Comparison of Notification Technologies
Choosing the right technology is crucial for building an efficient and scalable notification system. The table below compares popular options:
Technology | Pros | Cons | Use Cases |
---|---|---|---|
WebSockets | Full-duplex communication, low latency, efficient for high-volume updates | More complex to implement, requires persistent connection | Chat applications, collaborative tools, live dashboards |
Server-Sent Events (SSE) | Simple to implement, unidirectional communication, efficient for server-push updates | Limited to server-to-client communication, less efficient for bidirectional interactions | Live news feeds, stock tickers, real-time data streams |
Polling | Simple to implement, works with most browsers and servers | High latency, inefficient use of resources, increased server load | Applications with infrequent updates, where low latency isn’t critical |
Choosing the Right Technology
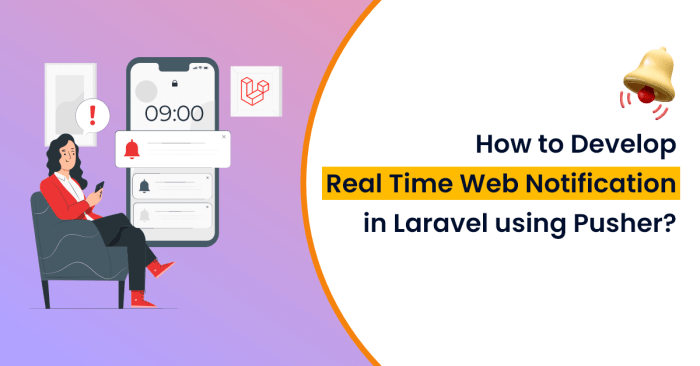
Source: oneclickitsolution.com
Building a real-time notification system for web apps requires finesse; think about the immediate feedback needed, much like understanding your healthcare costs. Knowing when a payment is due, for example, is crucial, and that’s why checking out this resource on The Importance of Understanding Health Insurance Co-pays and Deductibles is key. Applying this same urgency to your web app notifications ensures users stay informed and engaged.
Building a real-time notification system requires careful consideration of the underlying communication technology. The choice significantly impacts performance, scalability, and security. This section dives into the key players – WebSockets, Server-Sent Events (SSE), and polling – comparing their strengths and weaknesses to help you make the right decision for your application.
Comparison of WebSockets, Server-Sent Events, and Polling
Selecting the appropriate technology hinges on understanding the nuances of each approach. WebSockets provide a persistent, bidirectional connection between client and server, ideal for constant data streams. SSE, on the other hand, offers a unidirectional connection where the server pushes updates to the client. Polling, the simplest method, involves the client repeatedly requesting updates from the server. Each method presents a unique trade-off between complexity, efficiency, and features.
Feature | WebSockets | Server-Sent Events | Polling |
---|---|---|---|
Connection Type | Persistent, bidirectional | Persistent, unidirectional | Short-lived, repeated requests |
Data Transfer | Full-duplex communication | Server pushes data to client | Client requests data from server |
Overhead | Higher initial overhead | Lower overhead than WebSockets | High overhead due to repeated requests |
Latency | Low latency | Relatively low latency | High latency, depends on polling frequency |
Browser Compatibility | Widely supported in modern browsers | Widely supported in modern browsers | Universally supported |
Scalability | Can be challenging to scale to a massive number of connections | Generally easier to scale than WebSockets | Scales relatively well with appropriate caching and load balancing |
Factors to Consider When Selecting a Technology
The optimal technology depends on several factors, including the application’s requirements and constraints. Scalability refers to the system’s ability to handle a growing number of users and connections. Latency represents the delay between sending and receiving data. Browser compatibility ensures the system functions across various browsers and devices. Security concerns include protecting data transmitted between client and server.
Security Considerations for Each Technology
Security is paramount in real-time communication. WebSockets, due to their persistent nature, require robust security measures, such as TLS encryption to protect data in transit. SSE, while inherently simpler, still necessitates secure HTTPS to prevent eavesdropping. Polling, being stateless, is less susceptible to certain attacks but still requires secure endpoints to prevent data tampering or unauthorized access. Implementing proper authentication and authorization mechanisms is crucial for all technologies.
Decision-Making Flowchart for Technology Selection
The choice of technology involves a series of considerations. A simple flowchart can guide this process.
Imagine a flowchart with three decision points.
Decision Point 1: Does the application require bidirectional communication (e.g., chat application)?
* Yes: Proceed to Decision Point 2 (WebSockets)
* No: Proceed to Decision Point 3 (SSE or Polling)
Decision Point 2: Can the application handle the complexity and scalability challenges of WebSockets?
* Yes: Choose WebSockets
* No: Choose SSE
Decision Point 3: Is low latency critical?
* Yes: Choose SSE
* No: Choose Polling
This flowchart provides a simplified approach. The actual decision might involve a more nuanced evaluation of specific application requirements and constraints. For example, a simple notification system might favor SSE for its ease of implementation and scalability, while a high-frequency trading application might demand the low latency of WebSockets.
Implementing a Real-Time Notification System with WebSockets: How To Build A Real-Time Notification System In Web Applications
WebSockets offer a powerful way to build real-time applications, ditching the constant polling and embracing a persistent connection between client and server. This allows for instant updates, a crucial feature for notification systems. Let’s dive into building one using Node.js and Socket.IO, a popular library simplifying WebSocket development.
Setting up a WebSocket Server with Node.js and Socket.IO
Socket.IO handles the complexities of WebSockets, providing a higher-level API. First, install it: npm install socket.io
. Then, create a server:
“`javascript
const express = require(‘express’);
const http = require(‘http’);
const socketio = require(‘socket.io’);
const app = express();
const server = http.createServer(app);
const io = socketio(server);
io.on(‘connection’, (socket) =>
console.log(‘A user connected’);
socket.on(‘disconnect’, () =>
console.log(‘User disconnected’);
);
socket.on(‘chat message’, (msg) =>
io.emit(‘chat message’, msg); // Broadcast to all connected clients
);
);
server.listen(3000, () =>
console.log(‘listening on *:3000’);
);
“`
This code establishes a server, listens for connections, and broadcasts messages received on the ‘chat message’ event to all connected clients. Error handling is implicitly included through the standard Node.js error handling mechanisms; any uncaught exceptions will halt the server. More robust error handling could involve using a try-catch block within the event listeners.
Handling WebSocket Connections, Message Broadcasting, and Error Handling
The previous example demonstrated basic message broadcasting. For more sophisticated notification systems, you’d likely need to handle different event types and target specific users. For instance, you might have separate events for new messages, friend requests, and system alerts. Each event would be handled differently, possibly involving database lookups to determine recipients. A more robust approach might involve a message queue for asynchronous processing to prevent blocking the main thread. Error handling is critical. A well-structured system should gracefully handle connection failures, message parsing errors, and database errors, logging them appropriately and potentially notifying the user.
Implementing Authentication and Authorization for WebSocket Connections
Securing your WebSocket connections is paramount. You shouldn’t broadcast sensitive information to unauthorized users. A common approach involves using JWT (JSON Web Tokens) for authentication. The client sends a JWT upon connection, and the server verifies it. Authorization can be implemented by associating user roles with permissions to receive specific types of notifications. For example:
“`javascript
io.use((socket, next) =>
const token = socket.handshake.auth.token;
// Verify JWT here…
if (!isValidToken(token))
return next(new Error(‘Authentication failed’));
// Add user ID to socket object for later use
socket.userId = decodedToken.userId;
next();
);
“`
This middleware verifies the JWT before allowing the connection. The isValidToken
function would perform the actual token verification. Subsequently, you can use socket.userId
to target specific users with notifications.
Integrating WebSockets with a Front-End Framework (React Example)
Let’s illustrate integration with React using the socket.io-client
library. First, install it: npm install socket.io-client
. Then, in your React component:
“`javascript
import React, useEffect, useState from ‘react’;
import io from ‘socket.io-client’;
function App()
const [socket, setSocket] = useState(null);
const [messages, setMessages] = useState([]);
useEffect(() =>
const newSocket = io(‘http://localhost:3000’);
setSocket(newSocket);
newSocket.on(‘chat message’, (msg) =>
setMessages([…messages, msg]);
);
return () => newSocket.close();
, []);
return (
);
export default App;
“`
This code establishes a connection to the WebSocket server, listens for ‘chat message’ events, and updates the component state. Remember to handle potential errors, such as connection failures, gracefully. The cleanup function ensures the socket is closed when the component unmounts. Similar approaches can be used with Angular or Vue.js, utilizing their respective lifecycle methods and state management systems. The core concept remains the same: establishing a connection, listening for events, and updating the UI accordingly.
Handling Notifications on the Client-Side
Building a killer real-time notification system isn’t just about the backend magic; it’s about delivering those notifications gracefully to the user. A poorly implemented client-side handling can transform a helpful feature into an annoying distraction. Let’s explore best practices to ensure your notifications enhance, not hinder, the user experience.
Managing a steady stream of notifications requires finesse. Overwhelming users with a constant barrage of alerts is a surefire way to make them ignore your system entirely. We need strategies to control the flow and ensure important notifications get noticed while less critical ones don’t interrupt workflow.
Notification Display Techniques, How to Build a Real-Time Notification System in Web Applications
Different notification types call for different display methods. A simple “like” on a post deserves a subtle visual cue, while a critical system alert needs immediate attention. Choosing the right display method is crucial for user engagement and avoiding notification fatigue. Browser notifications, toast messages, and modals each offer distinct advantages and should be used strategically.
Browser notifications, for example, are perfect for less time-sensitive updates. They appear as a small pop-up outside the application window, ensuring the user is aware even if they aren’t actively using the app. Toast messages, on the other hand, are short, non-intrusive messages that appear briefly within the app itself, ideal for quick updates or confirmations. Modals, reserved for critical alerts or requiring immediate user action, demand attention by temporarily blocking interaction with the rest of the application.
Prioritizing Notifications Based on Importance
Not all notifications are created equal. A new message from a friend shouldn’t have the same visual impact as a system error. Prioritization is key. We can achieve this through a combination of visual cues (color-coding, icons, urgency labels) and display methods (using modals for high-priority alerts, toast messages for low-priority ones). A well-defined notification system should incorporate a clear hierarchy of importance, allowing users to focus on what matters most. For example, a banking app might prioritize security alerts over marketing promotions, visually differentiating them through color (red for security, blue for marketing) and placement (top for security, bottom for marketing).
User Interface Mockup: A Visual Guide
Imagine a simple social media application. The notification center is located in the top right corner, represented by a bell icon that changes color (grey when no new notifications, orange when new notifications exist). Clicking the bell icon reveals a dropdown menu.
Within the dropdown, notifications are displayed as cards. Each card contains:
- Icon: A small icon representing the notification type (e.g., a speech bubble for messages, a heart for likes).
- Sender/Source: The name or source of the notification (e.g., “John Doe” or “System Alert”).
- Message Preview: A short summary of the notification.
- Timestamp: The time the notification was received.
High-priority notifications (e.g., direct messages from important contacts, system errors) are displayed with a red background and a prominent “!” icon. Low-priority notifications (e.g., likes, comments) have a light grey background. The dropdown menu allows for marking notifications as read, dismissing them individually, or clearing all notifications. The unread count is prominently displayed next to the bell icon. This system ensures a clear visual hierarchy and easy management of notifications, preventing user overload.
Scaling and Optimizing the System
Building a real-time notification system that can handle a massive influx of users without crumbling under pressure is crucial for a smooth user experience. Think Twitter notifications during a major breaking news event – you want that system to stay up and deliver the messages, not crash and burn. Scaling and optimization aren’t just about adding more servers; it’s about smart design choices and efficient resource management.
The key to a robust real-time notification system lies in anticipating potential bottlenecks and implementing proactive solutions. Ignoring scalability from the outset can lead to a system that’s slow, unreliable, and ultimately, a frustrating experience for your users. This section dives into strategies for ensuring your notification system can handle the growth, no matter how explosive.
Load Balancing and Workload Distribution
Distributing the load across multiple servers is fundamental to scalability. Imagine a single server trying to handle millions of concurrent connections – it’s like trying to fit an elephant into a shoebox. Load balancing techniques, such as round-robin, least connections, or IP hash, distribute incoming requests evenly across a pool of servers. This prevents any single server from becoming overloaded and ensures consistent performance. For example, a round-robin approach would cycle through available servers, sending each new connection to the next server in the queue. This ensures a fairly even distribution of the workload, preventing any single server from being overwhelmed. More sophisticated algorithms consider server load and other factors to optimize distribution further.
Strategies for Scaling a Real-Time Notification System
Several strategies can be employed to ensure your system can handle a large number of concurrent users. These strategies often work in tandem to provide a comprehensive solution.
- Horizontal Scaling: Adding more servers to the system. This is generally the most cost-effective and efficient approach for handling increased load. Instead of upgrading a single, powerful server, you add multiple less powerful servers that work together. Think of it like having a team of workers instead of a single super-worker.
- Vertical Scaling: Upgrading the hardware of existing servers (more RAM, faster processors, etc.). This is a simpler approach but has limitations. There’s a point where you can’t upgrade a single server any further, whereas you can always add more servers.
- Database Optimization: Efficient database design and query optimization are vital. Using appropriate indexing, caching strategies (like Redis), and connection pooling can significantly improve database performance and reduce latency.
- Caching: Implementing caching mechanisms (like Redis or Memcached) to store frequently accessed data can dramatically reduce the load on the database and improve response times. Imagine having a readily available copy of frequently used data, eliminating the need to repeatedly fetch it from the main database.
- Message Queues: Utilizing message queues (like RabbitMQ or Kafka) can decouple the notification system from other parts of the application, improving resilience and scalability. This allows for asynchronous processing, preventing bottlenecks and ensuring notifications are delivered even under heavy load. Think of it as a post office: messages are stored and delivered at a pace the system can handle.
Potential Bottlenecks and Performance Optimization Techniques
Identifying and addressing potential bottlenecks is critical for a high-performing system. Common bottlenecks include database access, network latency, and inefficient code.
- Minimize Database Queries: Reduce the number of database queries by using efficient data structures and caching frequently accessed data. Each query adds overhead; minimizing them improves response time.
- Optimize Network Communication: Use efficient protocols (like WebSockets) and minimize the size of messages exchanged between the client and server. Smaller messages translate to faster transmission.
- Efficient Code Implementation: Write clean, optimized code to minimize resource consumption and improve overall performance. This includes using appropriate data structures and algorithms.
- Connection Pooling: Reuse database connections instead of creating new ones for each request. This significantly reduces the overhead associated with database connections.
- Asynchronous Processing: Process tasks asynchronously to prevent blocking operations from slowing down the system. This allows the system to handle multiple tasks concurrently without waiting for each one to finish before starting the next.
Security Best Practices
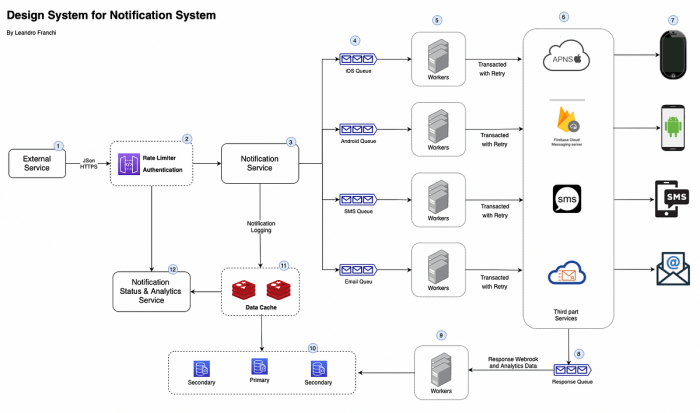
Source: medium.com
Building a real-time notification system is like building a high-speed highway – efficient, but also vulnerable if not properly secured. Ignoring security can lead to data breaches, system outages, and a seriously unhappy user base. Let’s make sure your notification system is as robust as it is speedy.
Real-time notification systems, by their nature, present unique security challenges. The constant flow of data between server and client creates opportunities for attackers to exploit vulnerabilities. Protecting against these threats requires a multi-layered approach, encompassing secure authentication, authorization, and robust protection against denial-of-service attacks. Ignoring these aspects can lead to significant consequences, from compromised user data to complete system failure.
Authentication and Authorization Mechanisms
Secure authentication ensures only authorized users can access the notification system. This typically involves verifying user credentials using strong passwords, multi-factor authentication (MFA), or other robust methods. Authorization, on the other hand, controls what actions authenticated users can perform within the system. For example, a regular user might only receive notifications, while an administrator could manage user settings and notification configurations. Implementing both is crucial; a system with strong authentication but weak authorization is still vulnerable. Consider using industry-standard protocols like OAuth 2.0 or OpenID Connect for secure authentication and authorization flows. Properly managing API keys and tokens is also essential to prevent unauthorized access. Regularly rotate these keys to minimize the impact of any compromise.
Protection Against Denial-of-Service (DoS) Attacks
Denial-of-service attacks aim to overwhelm the notification system, making it unavailable to legitimate users. These attacks can range from simple flooding attacks to more sophisticated techniques. Mitigation strategies include implementing rate limiting to restrict the number of requests from a single IP address or user within a specific timeframe. Using a content delivery network (CDN) can distribute the load across multiple servers, making it more difficult for attackers to overwhelm the system. Investing in robust infrastructure with sufficient capacity is also crucial. Regular security audits and penetration testing can identify vulnerabilities and help prepare for potential attacks. Think of it like having a robust fire suppression system in place – you hope you never need it, but it’s vital to have it when you do.
Security Best Practices Checklist
Prioritizing security from the outset is key. Here’s a checklist for developers to ensure a secure real-time notification system:
- Use HTTPS: Always encrypt communication between the client and server using HTTPS to protect data in transit.
- Implement robust authentication and authorization: Employ strong passwords, MFA, and granular access controls.
- Regularly update dependencies and libraries: Keep your software and its components up-to-date to patch known vulnerabilities.
- Input validation and sanitization: Thoroughly validate all user inputs to prevent injection attacks (SQL injection, XSS, etc.).
- Rate limiting and DDoS protection: Implement mechanisms to mitigate denial-of-service attacks.
- Regular security audits and penetration testing: Proactively identify and address security vulnerabilities.
- Secure storage of sensitive data: Encrypt sensitive data both in transit and at rest.
- Implement logging and monitoring: Track system activity to detect suspicious behavior.
- Follow secure coding practices: Adhere to secure coding guidelines to minimize vulnerabilities.
- Use a Web Application Firewall (WAF): A WAF can help filter malicious traffic and protect against common web attacks.
Outcome Summary
Building a real-time notification system is a journey, not a sprint. From choosing the right technology to meticulously handling notifications on the client-side and scaling for growth, every step matters. This guide has armed you with the knowledge and practical steps to build a robust and engaging system. Remember, user experience is key – notifications should enhance, not disrupt. So go forth, build something amazing, and watch your users stay connected and engaged!