How to Use the Command Line for Programming and Development: Forget those clunky GUIs! Dive into the world of the command line interface (CLI) – the secret weapon of seasoned programmers. This isn’t your grandpappy’s DOS prompt; it’s a streamlined powerhouse for boosting your coding efficiency and unlocking a level of control you never thought possible. We’ll navigate the essential commands, master Git from the terminal, build applications with ease, and even conquer remote servers. Get ready to level up your dev game.
This guide will walk you through the fundamental commands, showing you how to navigate your file system, search for files, manipulate text, and manage your code with Git. We’ll then explore more advanced techniques, including using regular expressions, processing data with `awk`, and automating tasks with shell scripts. Finally, we’ll cover working with remote servers via SSH, troubleshooting common issues, and leveraging the CLI for debugging. By the end, you’ll be comfortable using the command line for a wide range of development tasks, making you a more efficient and powerful programmer.
Introduction to the Command Line Interface (CLI) for Developers
Forget the mouse clicks and endless menu navigation. For developers, the command line interface (CLI) – that text-based window where you type commands – is a secret weapon for boosting productivity and mastering your development workflow. It’s faster, more powerful, and once you get the hang of it, incredibly efficient.
The CLI offers a direct line of communication with your computer’s operating system. This allows for automation of repetitive tasks, streamlined workflows, and precise control over your development environment. Imagine scripting complex operations in minutes instead of hours spent clicking through GUIs. That’s the power of the CLI.
Basic CLI Navigation Commands
Navigating your file system is fundamental to using the CLI. These commands are your essential tools for moving around, creating, and deleting directories and files.
Here’s a quick rundown of the most frequently used commands:
Command | Description | Example | Output/Effect |
---|---|---|---|
pwd |
Print Working Directory – Shows your current location in the file system. | pwd |
Displays the current path, e.g., /home/user/projects |
ls |
List – Shows the contents of the current directory (files and folders). | ls -l |
Lists files and folders with details (permissions, size, etc.). |
cd |
Change Directory – Moves you to a different directory. | cd projects/myproject |
Changes the working directory to /home/user/projects/myproject (assuming pwd was /home/user/projects ). |
mkdir |
Make Directory – Creates a new directory. | mkdir newfolder |
Creates a new folder named “newfolder” in the current directory. |
rmdir |
Remove Directory – Deletes an empty directory. | rmdir emptyfolder |
Deletes the “emptyfolder” directory. Will fail if the directory is not empty. |
rm |
Remove – Deletes files or directories. Use with caution! | rm -rf myfile.txt |
Deletes the file “myfile.txt”. -r recursively deletes directories and their contents, -f forces deletion without confirmation. Use this with extreme caution! |
GUI vs. CLI for Software Development
Choosing between a GUI and a CLI often depends on the task at hand. Both have their strengths and weaknesses in the context of software development.
Feature | GUI | CLI |
---|---|---|
User Friendliness | Generally more intuitive for beginners, visual feedback. | Steeper learning curve, requires memorization of commands. |
Speed and Efficiency | Can be slower for repetitive tasks, many clicks needed. | Faster for automation and complex operations, fewer steps. |
Automation | Limited automation capabilities, usually requires third-party tools. | Powerful scripting capabilities, easily automate complex workflows. |
Control and Precision | Less precise control over system processes. | Provides granular control over system resources and processes. |
Essential CLI s for Programmers
Let’s face it: the command line interface (CLI) might seem intimidating at first, but mastering it is a game-changer for any programmer. It’s about speed, efficiency, and a deeper understanding of your system. Think of it as unlocking a secret level in your development journey. This section will cover some essential CLI commands that will significantly boost your productivity.
Beyond the basics, several powerful commands are indispensable for navigating, manipulating, and understanding your codebase. These tools allow you to perform complex operations with concise commands, saving you precious time and effort.
Finding Files and Text with `find` and `grep`
`find` and `grep` are your best friends when it comes to locating specific files and searching within them. `find` helps you locate files based on name, type, or other criteria, while `grep` lets you search for specific patterns within files. Imagine needing to find all `.java` files modified in the last week containing the string “database connection”. That’s where these commands shine.
For example, to find all `.txt` files in the current directory and its subdirectories, you would use:
find . -name "*.txt"
To search for lines containing “error” within all `.log` files in a directory, you’d use:
grep "error" *.log
Combining `find` and `grep` is even more powerful. To find all `.cpp` files containing “segmentation fault” within a project directory:
find . -name "*.cpp" -exec grep "segmentation fault" \;
Redirection and Piping: `>`, `>>`, `|`
Redirection and piping are fundamental for chaining commands together and managing output. The `>` operator redirects the output of a command to a file, overwriting the file if it exists. `>>` appends the output to a file. The pipe symbol `|` takes the output of one command and feeds it as input to another. This allows you to create powerful workflows.
For instance, to save the output of a `ls -l` command to a file named `file_list.txt`:
ls -l > file_list.txt
To append the output of a `date` command to an existing file:
date >> my_log.txt
To count the number of lines in all `.py` files and display only the total:
find . -name "*.py" -exec cat + | wc -l
File Manipulation with `wc`, `head`, and `tail`
`wc` (word count), `head`, and `tail` are invaluable for quick file inspection and manipulation. `wc` provides information about the number of lines, words, and characters in a file. `head` displays the first few lines of a file, and `tail` displays the last few lines. These are perfect for quickly reviewing log files or checking the status of a large data file.
To count the lines in a file named `data.csv`:
wc -l data.csv
To display the first 10 lines of a log file:
head -n 10 my_log.txt
To monitor the last 20 lines of a continuously updating log file:
tail -f -n 20 my_log.txt
Automating a Development Task: Compiling and Running Tests
Let’s say you’re working on a C++ project. A common task is compiling the code and then running unit tests. This can be easily automated using the CLI. Assuming you have a `Makefile` that handles compilation (`make`) and a test runner (e.g., `ctest`), you could create a simple script to automate this process. This script could be a simple shell script or even a batch file, depending on your operating system.
A basic example (in bash) could look like this:
make && ctest
This single line compiles the code using `make` and then runs the tests using `ctest`. If `make` fails, `ctest` won’t run, ensuring a clean and reliable workflow. This illustrates how chaining commands via `&&` (and) allows for conditional execution.
Version Control with Git via the Command Line
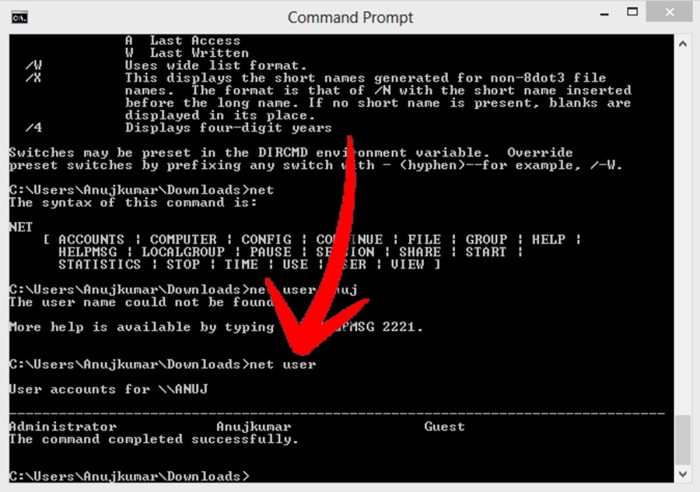
Source: wikihow.com
Git is your best friend when it comes to managing code changes, especially when collaborating with others. Using the command line gives you direct, granular control over your repository, and mastering it is a crucial skill for any developer. This section will walk you through the essential Git commands you’ll use daily.
The command line offers a level of precision and efficiency unmatched by graphical user interfaces. You can script complex workflows, automate tasks, and develop a deeper understanding of Git’s inner workings.
Initializing a Git Repository, Staging Changes, and Committing Them
Before you can track changes, you need a Git repository. This is where Git stores all your project’s files and their history. Once initialized, you can start staging changes (preparing them for a commit) and committing them (saving a snapshot of your project’s state).
To initialize a repository, navigate to your project directory in the terminal and type git init
. This creates a hidden .git
folder containing all the necessary Git metadata. To stage changes, use git add .
(to stage all changes) or git add
(to stage specific files). Finally, commit your changes with git commit -m "Your descriptive commit message"
. Always write clear, concise commit messages that explain *why* the changes were made, not just *what* was changed.
Using Git Branch, Git Merge, and Git Checkout for Branching and Merging
Branching allows you to work on new features or bug fixes in isolation without affecting the main codebase. git branch
creates a new branch. git checkout
switches to a different branch. After completing your work on a branch, you can merge it back into the main branch using git merge
. This integrates your changes into the main codeline.
Imagine you’re working on a new feature (a “login” page) while simultaneously fixing a bug in the existing code. Creating a branch called “feature/login” lets you develop the login page without interfering with the bug fix. Once the login page is ready, you can merge it into the main branch.
Pushing and Pulling Changes to a Remote Repository
To collaborate with others, you need a remote repository (usually hosted on platforms like GitHub, GitLab, or Bitbucket). git remote add origin
adds a remote repository. git push -u origin
pushes your local branch to the remote repository. git pull origin
downloads changes from the remote repository to your local branch. The -u
flag sets up tracking, making future pushes and pulls simpler.
This process ensures that your changes are synced with the central repository, allowing for seamless collaboration among team members. It also provides a backup of your code.
Mastering the command line is crucial for any programmer, especially when diving into frameworks like Ruby on Rails. You’ll be using it constantly for tasks like setting up your development environment and managing your Rails projects, so get comfy! Check out this awesome guide on getting started with Rails: An Introduction to Web Development with Ruby on Rails , then get back to conquering those command-line skills—they’re your secret weapon for coding efficiency.
Resolving Merge Conflicts
Merge conflicts occur when two branches have made changes to the same lines of code. Git will mark these conflicts in the affected files, indicating where the differences lie. You need to manually edit the files, resolving the conflicts by choosing the correct changes or combining them. After resolving the conflicts, stage the changes using git add
and then commit the merge with git commit
.
For example, if two developers modify the same function in different branches, Git will highlight the conflicting sections during the merge. You’ll need to open the file, review the changes from both branches, and decide which changes to keep or how to combine them. Once you’ve made the necessary edits, stage and commit the resolved changes.
Building and Running Applications from the CLI
The command line interface (CLI) isn’t just for navigating files; it’s a powerful tool for building and running applications. Mastering CLI commands for your chosen programming languages streamlines your workflow, offering a level of control and efficiency that graphical interfaces often lack. This section dives into how to leverage the CLI for building and running applications in various programming languages, exploring build systems and automation techniques.
Building applications from the command line involves using compilers, interpreters, and build systems to transform your source code into executable files. This process often includes steps like compiling source code, linking libraries, and packaging the final application. The specific commands and processes vary depending on the programming language and build system used.
Compilation and Execution in Different Languages
Building and running applications differs significantly across programming languages. Python, being interpreted, requires a simpler process than compiled languages like C++ or Java.
For Python, you typically just execute the script directly using the python
command (or python3
depending on your setup): python my_script.py
. For C++, you’ll need a compiler (like g++) to translate the code into machine-readable instructions. This usually involves two steps: compilation (creating an object file) and linking (combining object files and libraries to create an executable). For example: g++ my_program.cpp -o my_program
compiles and links my_program.cpp
, creating an executable named my_program
. Then, you run it using ./my_program
. Java requires compilation to bytecode (using javac
) and then execution using the Java Virtual Machine (JVM) via java
. The build process for larger Java projects often involves tools like Maven or Gradle (discussed below).
Build Systems for Different Programming Languages
Using a build system automates the compilation and linking process, especially crucial for larger projects. Several popular build systems cater to different needs and programming languages.
Language | Build System | Description | Example Command |
---|---|---|---|
C++, C | Make | A classic build system; uses a Makefile to define dependencies and build steps. | make |
Java | Maven | A powerful build system for Java projects; manages dependencies and builds using a pom.xml file. |
mvn clean install |
Java | Gradle | A flexible build system for Java (and other languages); uses Groovy or Kotlin for configuration. | ./gradlew build |
Python | Setuptools | Commonly used for managing Python packages and dependencies. | python setup.py install |
Using Environment Variables for Application Configuration
Environment variables provide a flexible way to configure applications without modifying the code directly. They allow you to set parameters like database connection strings, API keys, or paths to configuration files.
For example, you might set a database URL using the DATABASE_URL
environment variable. Your application can then access this value using the appropriate mechanism for your language (e.g., os.environ['DATABASE_URL']
in Python, getenv("DATABASE_URL")
in C++). This allows you to easily switch between different environments (development, testing, production) by simply changing the environment variables.
Automating the Build and Deployment Process
A simple bash script can automate the build and deployment of a small application. This example assumes a Python application:
Let’s say you have a Python script my_app.py
. A simple bash script (deploy.sh
) could look like this:
#!/bin/bash
# Build the application (in this case, just copying the script)
cp my_app.py /path/to/deployment/directory/# Run the application (assuming it runs in the background)
nohup python /path/to/deployment/directory/my_app.py &
echo "Application deployed and running."
This script copies the application to the deployment directory and runs it in the background using nohup
(ignoring hangup signals). More complex scenarios would involve more sophisticated build steps (e.g., using virtual environments, running tests, packaging the application). Remember to make the script executable: chmod +x deploy.sh
.
Advanced CLI Techniques for Developers
Level up your command-line prowess! We’ve covered the basics, but mastering advanced techniques is where true developer efficiency shines. This section dives into powerful tools that transform your workflow from merely functional to downright elegant. Prepare to unlock a new level of command-line mastery.
Beyond the fundamental commands, the command line offers a treasure trove of sophisticated tools for streamlining your development process. By mastering regular expressions, leveraging the power of `awk`, and understanding how to chain commands with `xargs`, you’ll significantly reduce development time and improve the accuracy of your tasks.
Regular Expressions with grep and sed
Regular expressions (regex) are patterns that match specific sequences of characters within text. `grep` uses regex to search for lines matching a pattern in files, while `sed` uses regex to perform substitutions and other edits on text. Mastering these tools is crucial for efficient text processing. Let’s explore some examples.
For instance, to find all lines containing an email address in a log file (assuming email addresses follow a simple pattern), you might use `grep ‘[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]2,’ logfile.txt`. This command uses a regular expression to identify email-like patterns within the `logfile.txt` file. `sed` allows you to replace specific patterns. For example, `sed ‘s/oldstring/newstring/g’ file.txt` replaces all occurrences of “oldstring” with “newstring” in `file.txt`. The `g` flag ensures global replacement.
Awk for Data Processing and Manipulation
Awk is a powerful text processing tool that’s particularly useful for manipulating structured data. It’s like a mini-programming language embedded within your terminal, allowing you to filter, sort, and transform data with ease. It excels at tasks that would otherwise require scripting in a full-fledged programming language.
Consider a comma-separated value (CSV) file containing user data. You can use `awk` to extract specific columns, calculate sums, or perform conditional operations. For example, to extract the username and email from a CSV file named `users.csv`, you might use a command like: `awk -F ‘,’ ‘print $1″,”$3’ users.csv`. This command uses a comma (`,`) as the field separator (`-F ‘,’`) and prints the first and third fields (username and email, assuming they are in those columns).
Xargs for Processing Command Output
Often, you’ll find yourself needing to process the output of one command as input to another. `xargs` is your friend here. It takes the output of a command (often a list of files or arguments) and uses it to construct and execute another command. This avoids complex piping and makes your commands more readable.
Imagine you need to compress a large number of `.txt` files. Instead of manually typing each filename into a `gzip` command, you can use `find . -name “*.txt” | xargs gzip`. This command finds all `.txt` files in the current directory and passes them to `gzip` for compression. This significantly streamlines the process, especially when dealing with many files.
Scenarios Where Advanced CLI Techniques Improve Developer Efficiency
Mastering these techniques significantly boosts developer productivity. Here are some key scenarios:
- Automated Code Cleanup: Using `sed` with regular expressions to automatically reformat code, ensuring consistent style and removing unnecessary whitespace.
- Log File Analysis: `grep` and `awk` allow for quick identification of errors or specific events within large log files, speeding up debugging.
- Data Transformation: `awk` simplifies the transformation of data between different formats (e.g., CSV to JSON), saving time and effort.
- Batch Processing: `xargs` enables efficient batch processing of files or data, automating repetitive tasks.
- Complex Search and Replace: `sed` with advanced regex allows for powerful search and replace operations, handling complex patterns beyond simple string replacements.
Working with Remote Servers via SSH
SSH, or Secure Shell, is your trusty sidekick for managing remote servers. It’s the go-to method for securely connecting to and interacting with servers hosted elsewhere, whether it’s a personal server, a cloud instance, or a development environment. Mastering SSH opens up a world of possibilities for programmers and developers.
Connecting to a remote server involves using the `ssh` command followed by the username and server address. This establishes a secure connection, allowing you to execute commands and manage files remotely. But remember, security is paramount – always use strong passwords and consider SSH keys for enhanced protection.
Connecting to a Remote Server, How to Use the Command Line for Programming and Development
To connect to a remote server, you’ll use the `ssh` command. The basic syntax is: ssh username@server_address
. For example, to connect to a server named `myServer` with the username `john.doe`, you’d type: ssh john.doe@myServer
. You’ll then be prompted for your password. Once authenticated, you’ll have a command-line interface on the remote server. You can then execute commands as if you were directly on the server itself.
Executing Commands on a Remote Server
Once connected via SSH, you can execute commands on the remote server just as you would on your local machine. For example, to list the files in the current directory on the remote server, you would type `ls`. To run a script named `myScript.sh`, you’d type `./myScript.sh`. The output of these commands will be displayed on your local terminal. Remember that the commands you execute affect the remote server, not your local machine. This is why careful command execution is crucial.
Transferring Files with scp and rsync
Transferring files between your local machine and a remote server is a common task. `scp` (secure copy) is a simple command for copying individual files, while `rsync` offers more advanced features, including resuming interrupted transfers and synchronizing directories.
Using scp
`scp` uses a syntax similar to `cp` but adds the remote server information. To copy a file named `myfile.txt` from your local machine to the remote server, you’d use: scp myfile.txt username@server_address:/path/on/server
. To copy a file from the remote server to your local machine, reverse the source and destination: scp username@server_address:/path/on/server/myfile.txt .
(The `.` represents the current directory on your local machine).
Using rsync
`rsync` provides more robust file transfer capabilities. It’s particularly useful for synchronizing directories, as it only transfers changes, making it efficient for large projects or frequent updates. A basic `rsync` command for copying a directory looks like this: rsync -avz /local/directory username@server_address:/path/on/server
. The `-a` flag ensures archive mode (preserves permissions, timestamps, etc.), `-v` provides verbose output, and `-z` enables compression.
Setting Up Secure SSH Keys
Using passwords for SSH authentication is convenient but less secure. SSH keys offer a more secure alternative, eliminating the need to enter passwords repeatedly. Here’s how to set up SSH keys:
First, generate a key pair on your local machine using the command: ssh-keygen
. You’ll be prompted to enter a file name (default is `id_rsa`), a passphrase (optional but recommended), and to confirm the passphrase. This creates two files: `id_rsa` (your private key, keep this secret!) and `id_rsa.pub` (your public key).
Next, copy the contents of your `id_rsa.pub` file to the `~/.ssh/authorized_keys` file on your remote server. You can do this using `scp`: scp ~/.ssh/id_rsa.pub username@server_address:~/.ssh/authorized_keys
. If the `.ssh` directory doesn’t exist on the remote server, you’ll need to create it first. After copying the public key, you should be able to connect to the server without entering a password. Remember to set appropriate permissions on the `.ssh` directory and its contents (chmod 700 ~/.ssh; chmod 600 ~/.ssh/authorized_keys
on both local and remote machines).
Troubleshooting and Debugging with the CLI: How To Use The Command Line For Programming And Development
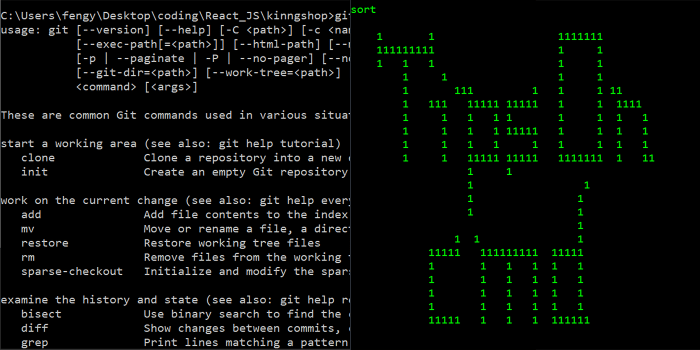
Source: medium.com
The command line isn’t just for executing commands; it’s a powerful tool for identifying and resolving issues in your code and system. Understanding common errors and utilizing the CLI’s debugging capabilities can significantly speed up your development workflow and save you countless hours of frustration. This section explores effective strategies for troubleshooting and debugging directly from the command line.
Effective debugging often involves a combination of careful error analysis, strategic use of logging, and leveraging the power of system tools accessible via the CLI. By mastering these techniques, you can transform the command line from a simple execution environment into a sophisticated debugging platform.
Common CLI Errors and Solutions
Many common CLI errors stem from simple typos, incorrect syntax, or permission issues. Let’s examine some frequent offenders and their solutions. For example, a “command not found” error usually indicates a misspelling or that the command isn’t installed. A “permission denied” error suggests a lack of appropriate access rights to the file or directory. Carefully reviewing the command syntax and ensuring the correct path and permissions are in place are key steps in resolving these issues. Using the `which` command (on Linux/macOS) or `where` (on Windows) can help verify if a command is installed and located correctly.
Using Logging Tools and Debugging Techniques from the CLI
Integrated debugging tools are often available within the command line itself. For instance, many programming languages offer command-line debuggers that allow you to step through code, inspect variables, and set breakpoints directly from your terminal. These debuggers are invaluable for identifying subtle logic errors that might be difficult to spot otherwise. Furthermore, incorporating robust logging into your applications allows you to track the execution flow, identify problematic areas, and gain insights into the application’s behavior. Analyzing log files via the CLI, using tools like `grep`, `awk`, and `sed`, enables efficient filtering and analysis of the log data.
Common Error Messages and Interpretations
Understanding the meaning of error messages is crucial for effective debugging. The following table Artikels some common error messages and their interpretations.
Error Message | Interpretation | Possible Solution | Example |
---|---|---|---|
Command not found | The specified command doesn’t exist or isn’t in your system’s PATH. | Verify spelling, install the command, or add its directory to your PATH. | bash: mycommand: command not found |
Permission denied | You lack the necessary permissions to access a file or directory. | Use `sudo` (Linux/macOS) or run the command as administrator (Windows). Check file permissions using `ls -l` (Linux/macOS) or `dir /a` (Windows). | Permission denied |
FileNotFoundError | The specified file or directory does not exist. | Verify the file path and ensure the file exists. | FileNotFoundError: [Errno 2] No such file or directory: 'myfile.txt' (Python example) |
Segmentation fault | A program has attempted to access memory it doesn’t have permission to access. This often indicates a serious bug in the program. | Debug the code carefully, looking for memory management issues like buffer overflows or pointer errors. | Segmentation fault (core dumped) |
Analyzing System Logs for Troubleshooting
System logs are a treasure trove of information, providing a detailed record of system events, including errors and warnings. The CLI offers powerful tools to efficiently analyze these logs. For example, on Linux systems, the `/var/log` directory often contains numerous log files. Commands like `grep` can be used to search for specific error messages or patterns within these logs, while `awk` and `sed` can be used for more sophisticated filtering and manipulation of the log data. For instance, `grep “error” /var/log/syslog` will display all lines containing the word “error” from the syslog file. Understanding the structure and content of your system’s logs is essential for effective troubleshooting. Knowing which log files to examine for specific types of problems is a valuable skill for any developer.
Ultimate Conclusion
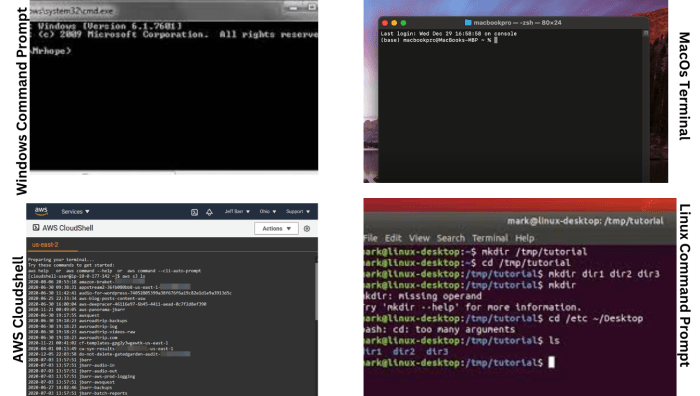
Source: freecodecamp.org
Mastering the command line isn’t just about knowing commands; it’s about gaining a deeper understanding of your system and workflow. By embracing the CLI, you’ll unlock a level of speed and efficiency that will transform your programming experience. From automating repetitive tasks to troubleshooting complex issues, the command line is your secret weapon for becoming a truly effective developer. So ditch the mouse, embrace the keyboard, and prepare to conquer the command line!