Introduction to API Documentation and Best Practices: Dive into the world of APIs – the unseen powerhouses connecting applications. We’ll unravel the mysteries of REST, SOAP, and GraphQL, showing you how to design, document, and secure your APIs like a pro. Get ready to master the art of API interaction and build seamless, robust applications.
This guide covers everything from understanding the fundamental concepts of APIs to implementing best practices in design, documentation, and security. We’ll explore various API documentation styles and tools, helping you create clear, concise, and developer-friendly documentation. We’ll also delve into crucial aspects like API versioning, testing, and debugging, ensuring your APIs are not only functional but also maintainable and scalable.
What is an API?
Imagine you’re at a restaurant. You don’t go into the kitchen to cook your own food, right? You use a menu (the interface) to order what you want from the waiter (the intermediary), who then communicates your request to the chef (the server) in the kitchen. The chef prepares your meal and the waiter brings it back to you. An API is similar – it’s the menu and waiter combined, acting as an intermediary between different software applications. It defines how different software components can talk to each other and exchange information without needing to know the inner workings of each other.
An Application Programming Interface (API) is essentially a set of rules and specifications that software programs can follow to communicate and exchange data. It acts as a messenger, translating requests from one application (the client) into a format understandable by another application (the server), and vice-versa. This allows different systems, built perhaps by different teams or even different companies, to seamlessly interact. Think of it as a standardized way for different software pieces to “speak” the same language.
Types of APIs
Several different architectural styles exist for APIs, each with its own strengths and weaknesses. Choosing the right API style depends heavily on the specific needs of the application.
- REST (Representational State Transfer): This is the most common type of API. It uses standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. Think of it as a very user-friendly way for applications to communicate, using familiar web protocols. For example, a social media app might use a REST API to fetch user profiles or post updates.
- SOAP (Simple Object Access Protocol): SOAP APIs are more complex and structured than REST APIs. They use XML for data exchange and often rely on more heavyweight protocols like HTTP or SMTP. While powerful, SOAP APIs can be less flexible and harder to implement than REST APIs. A classic example is a banking system using SOAP to securely transfer financial data.
- GraphQL: This newer API type allows clients to request only the data they need, reducing the amount of data transferred. This improves efficiency and performance, especially in complex applications with many interconnected data points. A mobile app with many data points to retrieve might benefit greatly from the efficiency of a GraphQL API.
Benefits of Using APIs
APIs offer significant advantages in software development, fostering collaboration and innovation.
- Improved Efficiency and Productivity: Developers can reuse existing functionality instead of building everything from scratch, saving time and resources.
- Enhanced Collaboration: APIs enable different teams and organizations to integrate their systems easily, fostering collaboration and data sharing.
- Increased Innovation: By making data and functionality readily accessible, APIs allow developers to build new and innovative applications on top of existing platforms.
- Faster Development Cycles: Leveraging existing APIs speeds up development, allowing for quicker releases and faster iterations.
API Client-Server Interaction
Imagine a simple diagram: A box labeled “API Client” (e.g., a mobile app) sends a request to a box labeled “API Server” (e.g., a database). The server processes the request, accesses the necessary data, and sends a response back to the client. Arrows indicate the flow of data between the client and server. The API acts as the intermediary, ensuring both parties understand each other’s “language” and format. This interaction might involve multiple steps, including authentication and error handling, but the core concept remains the same: a request, processing, and a response.
Understanding API Documentation
So, you’ve grasped the basic concept of APIs – the backstage pass to data and functionality. But a well-functioning API is nothing without clear, concise documentation. Think of it as the instruction manual for developers; without it, even the most brilliant API will gather digital dust. Good documentation is the key to unlocking its full potential and ensuring developers can use it effectively.
Comprehensive API documentation acts as a bridge between the API creators and the developers who will use it. It’s the lifeline for anyone trying to integrate the API into their own projects. Without it, developers are left to guess, leading to frustration, errors, and wasted time. Good documentation, on the other hand, fosters collaboration, streamlines development, and ultimately leads to better applications.
Key Components of API Documentation
Effective API documentation typically includes several crucial elements. These components ensure that developers have all the information they need to understand and use the API correctly. Missing even one key piece can lead to significant hurdles in the development process.
These key components usually include a detailed description of each endpoint (the specific URLs that handle requests), a clear explanation of the request methods (like GET, POST, PUT, DELETE), a precise definition of all parameters (the data sent with the request), a complete description of the responses (the data returned by the API), examples of requests and responses (in various formats like JSON or XML), authentication methods, error codes and their meanings, and rate limits to prevent abuse.
Importance of Clear and Concise Documentation
Imagine trying to assemble IKEA furniture without instructions. Frustrating, right? Poorly written API documentation has a similar effect on developers. Clear and concise documentation is paramount because it directly impacts developer productivity and reduces integration time. Ambiguous or incomplete documentation leads to errors, delays, and increased development costs. Conversely, well-written documentation empowers developers to quickly understand how to use the API, allowing them to focus on building their applications rather than deciphering cryptic instructions.
Think of it this way: well-documented APIs are like well-oiled machines, running smoothly and efficiently. Poorly documented APIs are like rusty, creaky contraptions, prone to breaking down and causing headaches.
Challenges of Poorly Documented APIs
Working with poorly documented APIs is a developer’s nightmare. The challenges are numerous and can significantly impact a project’s timeline and success. Common problems include difficulty in understanding the API’s functionality, inability to correctly format requests, troubleshooting issues due to unclear error messages, and wasted time spent reverse-engineering the API to figure out how it works. This often leads to inefficient code, increased debugging time, and ultimately, a less robust and reliable application.
Consider the scenario of a mobile app developer integrating a payment gateway API. Without clear documentation, they might struggle to understand how to handle different payment types, leading to errors in processing transactions and potentially impacting user experience and revenue.
Mastering API documentation is crucial, especially when diving into complex projects. Understanding how to effectively navigate and utilize APIs is key, and this becomes even more apparent when you start building games. For instance, if you’re exploring the exciting world of game development, check out this awesome resource on Exploring the World of Game Development with Unity to see how APIs are used in practice.
Returning to APIs, robust documentation ensures smoother development and reduces frustrating debugging sessions.
Example API Endpoint Parameters
Here’s a simple table illustrating how endpoint parameters and their descriptions should be presented in good API documentation:
Parameter | Type | Description | Example |
---|---|---|---|
userId |
integer |
The unique identifier for the user. | 12345 |
productName |
string |
The name of the product. | "Awesome Widget" |
quantity |
integer |
The number of products. | 3 |
date |
date |
The date of the transaction (YYYY-MM-DD). | 2024-10-27 |
Best Practices for API Design
Crafting a stellar API isn’t just about making it work; it’s about making it a joy to use. A well-designed API is intuitive, efficient, and robust, leading to happier developers and ultimately, a more successful application. This section dives into the key principles and practices that elevate an API from merely functional to truly exceptional.
Think of your API as a digital handshake. It needs to be clear, consistent, and reliable to foster a strong connection between your application and those who integrate with it. Ignoring best practices can lead to frustration, errors, and ultimately, a poorly performing application. Let’s explore how to avoid these pitfalls.
RESTful API Design Principles
REST, or Representational State Transfer, is the architectural style that forms the foundation for many successful APIs. Adhering to RESTful principles ensures consistency, scalability, and ease of use. Key aspects include using standard HTTP methods (GET, POST, PUT, DELETE) to represent actions on resources, utilizing a uniform interface, and maintaining statelessness. For example, a GET request typically retrieves data, a POST creates new data, a PUT updates existing data, and a DELETE removes data. This predictable structure makes the API easier to understand and use. A well-structured URL, such as `/users/userId/orders`, clearly indicates the resource and its identifier.
API Authentication Methods: OAuth 2.0 and API Keys
Securely authorizing access to your API is crucial. Two common methods are OAuth 2.0 and API keys. OAuth 2.0 is a more robust solution, offering granular control over access permissions. It’s particularly useful for scenarios where third-party applications need to access user data on behalf of the user, such as social media logins. In contrast, API keys provide a simpler, less granular approach, typically involving a single key for authentication. API keys are suitable for applications where security requirements are less stringent or where managing access tokens is overly complex. The choice depends on the specific security needs and complexity of your application. For high-security applications, OAuth 2.0 is generally preferred.
Error and Exception Handling in API Responses
Handling errors gracefully is critical for a positive user experience. Consistent error handling across your API is key. A standardized response format, including a clear error code, a descriptive error message, and potentially a developer-friendly error detail, is essential. For instance, a 404 (Not Found) status code should always indicate a missing resource, while a 400 (Bad Request) signifies an issue with the client’s request. Avoid vague error messages; instead, provide specific information to help developers quickly diagnose and resolve problems. Consider including a field with suggestions on how to resolve the error.
Structuring API Responses with JSON or XML
Choosing the right data format significantly impacts API readability and usability. JSON (JavaScript Object Notation) is generally preferred for its lightweight nature and ease of parsing by most programming languages. Its hierarchical structure using key-value pairs makes it simple to understand and use. XML (Extensible Markup Language) offers a more verbose and structured approach, which can be beneficial in certain situations. However, JSON’s simplicity and efficiency often make it the better choice for most APIs. A well-structured JSON response, for example, might include a status code, a message, and the requested data, all clearly organized within a JSON object.
API Documentation Styles and Tools
So, you’ve grasped the basics of APIs and their documentation. Now let’s dive into the nitty-gritty: the different ways you can document your APIs and the tools that make the process smoother. Choosing the right style and tool can significantly impact the usability and maintainability of your API documentation.
API documentation isn’t just about throwing together a bunch of endpoints and their parameters. It’s about creating a clear, concise, and comprehensive guide that developers can easily understand and use. This involves selecting a suitable documentation style and leveraging powerful tools to streamline the process.
API Documentation Styles
Several styles exist for structuring API documentation, each with its own strengths and weaknesses. The choice often depends on factors like project complexity, team preference, and the need for automation.
- OpenAPI/Swagger: This is arguably the most popular style, using a standardized, machine-readable format (YAML or JSON). It allows for automatic generation of interactive documentation, client SDKs, and server stubs. Imagine a beautifully interactive webpage that lets developers test API calls directly from the documentation – that’s the power of Swagger.
- RAML (RESTful API Modeling Language): RAML provides a more concise and human-readable way to define APIs. It focuses on the design and structure, emphasizing simplicity and ease of understanding. Think of it as a more streamlined approach, perfect for smaller projects or when clarity is paramount.
- API Blueprint: This style uses Markdown, making it highly accessible and easy to collaborate on. While not as feature-rich as OpenAPI, its simplicity makes it a great choice for quick documentation or smaller projects where ease of writing and readability take precedence.
Popular API Documentation Tools
Several tools exist to assist in creating and managing API documentation. These tools often support various documentation styles and offer features like interactive testing, version control, and collaborative editing.
Tool | Supported Styles | Key Features | Pros | Cons |
---|---|---|---|---|
SwaggerHub | OpenAPI/Swagger | Collaboration, version control, interactive documentation, mocking | Powerful features, strong community support | Can be complex for smaller projects, paid plans for advanced features |
Postman | OpenAPI, RAML, and others | API testing, collaboration, documentation generation, collections | Excellent for testing and collaboration, user-friendly interface | Documentation features might not be as comprehensive as dedicated tools |
Redoc | OpenAPI/Swagger | Static site generation, customization options, supports various themes | Fast, lightweight, produces beautiful and easily deployable documentation | Fewer collaboration features compared to SwaggerHub |
Generating API Documentation from Code with Swagger
One of the most significant advantages of using OpenAPI/Swagger is the ability to generate documentation directly from your code. This ensures that your documentation always stays in sync with your API implementation. Tools like Swagger Codegen allow you to generate client SDKs and server stubs, further streamlining the development process.
The process typically involves adding annotations to your code (using Swagger annotations or similar) that describe your API endpoints, parameters, and responses. Then, you run a code generation tool to automatically produce the documentation in the chosen format (HTML, Markdown, etc.). This automation ensures consistency and reduces the risk of documentation becoming outdated.
For example, using a Java framework like Spring Boot with Springdoc OpenAPI, you can add annotations to your controllers to automatically generate Swagger documentation. This eliminates the manual work of writing the documentation separately, keeping it always in sync with the codebase.
Versioning and Maintenance of APIs: Introduction To API Documentation And Best Practices
API versioning and maintenance are crucial for the long-term success and stability of any API. Without a robust versioning strategy, updates can break existing integrations, leading to frustrated developers and a damaged reputation. Proper maintenance ensures your API remains reliable, secure, and relevant, fostering a healthy ecosystem of users.
Managing multiple API versions effectively requires a well-defined strategy and a commitment to clear communication. This involves not only technical considerations like backward compatibility but also a proactive approach to documentation updates and deprecation announcements. Ignoring these aspects can lead to a chaotic API landscape, difficult to navigate and maintain.
API Versioning Strategies, Introduction to API Documentation and Best Practices
Choosing the right versioning strategy is key to managing multiple API versions smoothly. Several approaches exist, each with its own advantages and disadvantages. The most common strategies are semantic versioning (SemVer) and URL-based versioning.
- Semantic Versioning (SemVer): This approach uses a three-part version number (MAJOR.MINOR.PATCH) to indicate the type of changes made. A MAJOR version bump signifies breaking changes, MINOR versions introduce new features, and PATCH versions are for bug fixes. For example, transitioning from v1.0.0 to v2.0.0 indicates significant changes that might require adjustments from users.
- URL-based Versioning: This method incorporates the version number directly into the API endpoint URL (e.g., `/v1/users`, `/v2/users`). This makes it clear which version of the API is being accessed. This strategy offers simplicity and clarity, especially for developers.
Deprecating Older API Versions
Deprecation is a necessary process for maintaining a healthy API ecosystem. It allows you to remove outdated functionality while giving developers sufficient time to migrate to newer versions. A well-defined deprecation policy is crucial for this process.
- Clear Communication: Announce deprecation plans well in advance through release notes, documentation updates, and possibly email notifications to registered users. Provide a clear timeline for deprecation and suggest migration paths.
- Grace Period: Allow ample time for developers to update their integrations. A grace period of several months or even a year is often recommended, depending on the complexity of the changes.
- Deprecation Warnings: Implement warnings in the deprecated API endpoints to alert developers that they are using outdated functionality and encourage migration.
- Example: A company might announce that API version 1.0 will be deprecated in six months, providing detailed migration guides and supporting users during the transition period. After six months, version 1.0 is removed.
Ensuring Backward Compatibility
Backward compatibility ensures that newer versions of your API continue to function with existing client applications. This minimizes disruption and maintains a smooth transition for users.
- Thorough Testing: Rigorous testing of each update is essential. This includes unit tests, integration tests, and end-to-end tests to ensure that existing functionalities remain unchanged.
- Versioned Responses: Return consistent response formats across versions, even if the underlying data structures have changed. This can involve using a common data structure or providing mapping guides between different versions.
- API Contracts: Define and maintain a strict API contract, specifying the expected input and output formats. Changes to the contract should be clearly communicated and documented.
Maintaining API Documentation
Keeping your API documentation up-to-date is essential for maintaining user satisfaction and successful API adoption. A well-defined workflow can ensure this process remains efficient and reliable.
- Versioned Documentation: Maintain separate documentation for each API version. This ensures that developers always have access to the relevant documentation for their specific version.
- Automated Documentation Generation: Tools can automatically generate API documentation from code comments, saving time and reducing manual effort. This helps to ensure consistency and accuracy.
- Regular Reviews and Updates: Establish a regular review process to ensure that the documentation remains accurate and up-to-date. This might involve assigning responsibility to a specific team member or incorporating documentation updates into the API development lifecycle.
Security Considerations for APIs
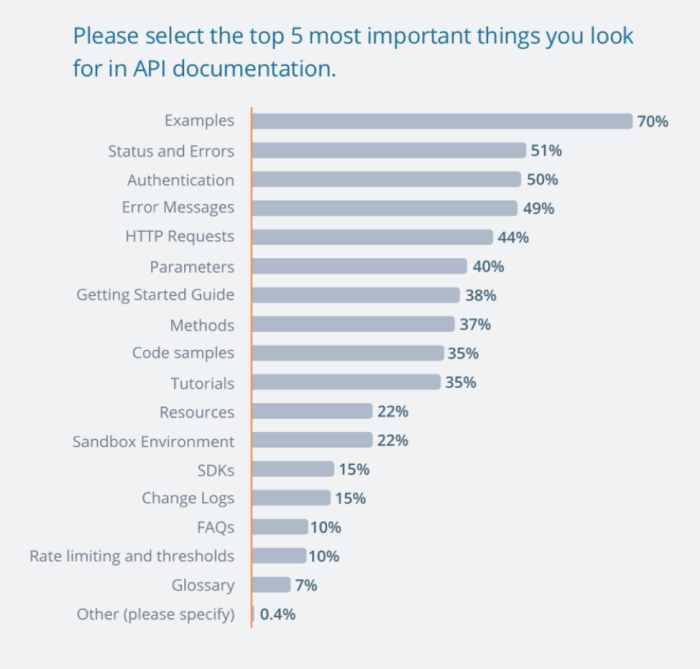
Source: altexsoft.com
APIs are the backbone of modern applications, silently orchestrating data exchange between different systems. But this powerful functionality comes with responsibility. Ignoring API security can expose your application and its users to serious vulnerabilities, leading to data breaches, financial losses, and reputational damage. Robust security measures are not an afterthought; they’re integral to the design and development process.
Building secure APIs requires a multi-faceted approach, encompassing everything from authentication and authorization to input validation and the prevention of common attack vectors. Failing to address these aspects can leave your API vulnerable to exploitation, potentially leading to significant consequences.
Common API Vulnerabilities and Mitigation Strategies
Several common vulnerabilities plague APIs. Understanding these vulnerabilities is the first step towards building robust defenses. Proper mitigation strategies are crucial for ensuring the security and integrity of your API.
- SQL Injection: Malicious code injected into API inputs can manipulate database queries, potentially allowing attackers to steal or modify data. Mitigation: Use parameterized queries or prepared statements to prevent direct SQL string concatenation.
- Cross-Site Request Forgery (CSRF): Attackers can trick users into performing unwanted actions on a web application they’re already authenticated to. Mitigation: Implement CSRF tokens to verify that requests originate from the legitimate web application.
- Cross-Site Scripting (XSS): Attackers inject malicious scripts into API responses, which are then executed in the user’s browser. Mitigation: Properly sanitize all user-supplied data before displaying it in the API response. Use output encoding to escape special characters.
- Broken Authentication and Session Management: Weak or improperly implemented authentication mechanisms can allow attackers to gain unauthorized access. Mitigation: Use strong password policies, multi-factor authentication (MFA), and secure session management techniques.
- Exposure of Sensitive Data: APIs might inadvertently expose sensitive data such as API keys, database credentials, or user information. Mitigation: Employ robust access control mechanisms, and never expose sensitive data in API responses unless absolutely necessary. Encrypt sensitive data both in transit and at rest.
Input Validation and Sanitization
Input validation and sanitization are critical for preventing many common API vulnerabilities. Failing to validate and sanitize inputs can leave your API vulnerable to attacks such as SQL injection, XSS, and command injection.
Input validation involves checking that the data received by the API conforms to the expected format and constraints. Sanitization involves cleaning the data to remove any potentially harmful characters or elements. For example, validating an email address to ensure it follows the correct format, and then sanitizing it to remove any potentially malicious code embedded within the address.
Securing API Keys and Authentication Tokens
API keys and authentication tokens are crucial for authorizing access to your API. However, they are also prime targets for attackers. Strong security measures are essential to protect these credentials.
- Use strong, randomly generated keys: Avoid predictable patterns or easily guessable keys.
- Implement key rotation: Regularly rotate API keys to minimize the impact of compromised keys.
- Use HTTPS: Encrypt all communication between the client and the API to prevent eavesdropping.
- Limit key permissions: Grant only the necessary permissions to each API key.
- Implement token revocation: Have a mechanism to revoke compromised tokens.
Secure API Design Patterns
Employing secure design patterns can significantly enhance the security posture of your APIs. These patterns provide a structured approach to building secure and resilient systems.
- OAuth 2.0: A widely used authorization framework that allows third-party applications to access user data without sharing their credentials.
- JWT (JSON Web Tokens): A compact and self-contained way to transmit information securely between parties as a JSON object.
- API Gateways: Act as a reverse proxy, providing a central point for managing and securing API traffic. They can enforce authentication, authorization, and rate limiting policies.
Testing and Debugging APIs
Building robust and reliable APIs requires a rigorous testing strategy. Without proper testing, even the most meticulously designed API can suffer from unexpected errors and inconsistencies, leading to frustrating user experiences and potentially serious business consequences. Thorough testing ensures your API functions as intended, handles edge cases gracefully, and performs optimally under various conditions. This section explores different testing approaches and best practices for debugging API issues.
API Testing Approaches
Effective API testing involves a combination of techniques to cover different aspects of functionality and performance. Two primary approaches are unit testing and integration testing. These methods, when used strategically, ensure comprehensive coverage of your API’s capabilities.
- Unit Testing: This focuses on individual components or functions within your API. Each unit is tested in isolation to verify its correctness. For example, a unit test might check if a specific function correctly validates user input or performs a calculation accurately. This granular approach helps pinpoint the source of errors quickly.
- Integration Testing: This tests the interaction between different components of your API. It ensures that these components work together seamlessly and that data flows correctly between them. For instance, an integration test might verify that a user authentication module correctly interacts with a database to retrieve user information and grant access.
Debugging API Issues
Debugging APIs can be challenging, requiring systematic approaches and the right tools. Effective debugging involves careful analysis of error messages, logs, and network traffic. A well-structured debugging process can significantly reduce troubleshooting time.
- Analyze Error Messages: API responses often include error codes and messages that provide clues about the problem. Understanding the meaning of these messages is crucial for efficient debugging. For example, a “404 Not Found” error indicates that the requested resource doesn’t exist, while a “500 Internal Server Error” suggests a problem on the server side.
- Utilize Logging: Implement comprehensive logging throughout your API to track the flow of execution and identify potential issues. Logs should include timestamps, request parameters, responses, and any relevant error information. Effective logging provides a detailed audit trail, simplifying the debugging process.
- Inspect Network Traffic: Tools like browser developer tools or dedicated API testing tools allow you to inspect the network traffic between your client and the API. This helps to identify issues related to request headers, data formatting, or network connectivity.
Common API Error Messages and Solutions
Several common API error messages and their solutions are presented below. Understanding these common issues can significantly improve debugging efficiency.
Error Message | Possible Cause | Solution |
---|---|---|
400 Bad Request | Invalid request parameters or data format | Check request parameters for correctness and ensure data conforms to the API specification. |
401 Unauthorized | Missing or invalid authentication credentials | Verify authentication credentials and ensure proper authorization headers are included in the request. |
404 Not Found | Requested resource does not exist | Double-check the URL and ensure the resource exists. |
500 Internal Server Error | Unhandled exception or error on the server | Examine server logs for detailed error information and address the underlying issue. |
Example API Test Plan: A Simple To-Do List API
Let’s consider a hypothetical API for a to-do list application. This API allows users to create, read, update, and delete to-do items.
A simple test plan might include:
- Unit Tests: Verify individual functions, such as creating a new to-do item, validating input data (e.g., ensuring the description field is not empty), and updating the status of an existing item.
- Integration Tests: Test the interaction between different API endpoints. For example, verify that creating a new item correctly updates the list of items, or that deleting an item removes it from the database and returns the correct response.
- Performance Tests: Evaluate the API’s response time under various load conditions. This ensures that the API can handle a large number of requests without significant performance degradation.
- Security Tests: Check for vulnerabilities such as SQL injection or cross-site scripting (XSS).
Real-world API Examples
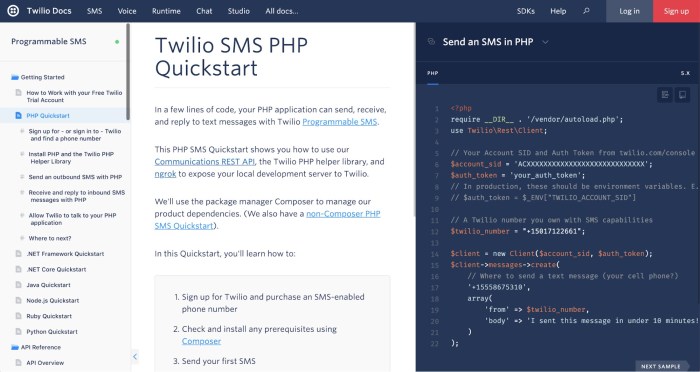
Source: website-files.com
Let’s dive into the practical world of APIs with some real-world examples. Understanding how popular APIs work and how to interact with them is key to becoming a proficient developer. We’ll explore the Twitter API and show you how to make API calls using Python and JavaScript, highlighting best practices along the way.
APIs are the backbone of countless applications, enabling seamless communication and data exchange between different systems. Mastering their use opens doors to building powerful and interconnected applications.
The Twitter API
The Twitter API is a powerful tool for accessing and interacting with Twitter data. Its comprehensive documentation makes it relatively easy to use, even for beginners. The API allows developers to retrieve tweets, user information, trends, and more. Access is granted through API keys and access tokens, which are managed through the Twitter Developer portal. Rate limits are in place to prevent abuse and ensure fair access for all developers.
Making API Calls with Python
Python’s rich ecosystem of libraries makes interacting with APIs a breeze. The `requests` library is a popular choice for making HTTP requests. Here’s a simple example of fetching recent tweets using the Twitter API (Note: This example requires you to have a Twitter Developer account and obtain the necessary API keys and tokens. Replace placeholders with your actual keys.):
import requests
# Replace with your actual API keys
bearer_token = "YOUR_BEARER_TOKEN"
headers = "Authorization": "Bearer ".format(bearer_token)
url = "https://api.twitter.com/2/tweets/search/recent?query=python"
response = requests.get(url, headers=headers)
data = response.json()
print(data)
Making API Calls with JavaScript
JavaScript, particularly within a browser environment, often utilizes the `fetch` API for making network requests. The following example demonstrates fetching data from a public API (not Twitter, for simplicity and avoiding sensitive keys):
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then(response => response.json())
.then(data => console.log(data));
Best Practices for Consuming External APIs
When working with external APIs, several best practices can significantly improve your application’s reliability and efficiency.
Proper error handling is crucial. Always check for HTTP status codes and handle potential errors gracefully. Rate limiting should be respected to avoid being blocked. Caching frequently accessed data can improve performance and reduce load on the API. Consider using asynchronous operations (like promises or async/await) to avoid blocking the main thread, ensuring a responsive user experience. Security is paramount; never expose API keys directly in your client-side code.
API Learning Resources
Numerous resources are available for those looking to deepen their understanding of APIs and API development.
Websites like the developer portals of popular API providers (e.g., Twitter, Google, Stripe) offer comprehensive documentation, tutorials, and code samples. Online courses and tutorials on platforms such as Coursera, Udemy, and freeCodeCamp provide structured learning paths. Books on RESTful APIs and API design principles offer in-depth knowledge. Stack Overflow and other developer communities are invaluable for troubleshooting and finding solutions to common problems.
Final Thoughts
Mastering API documentation and best practices isn’t just about writing good code; it’s about building a bridge between developers and applications. By following the strategies Artikeld here, you can create APIs that are not only efficient and secure but also easy to use and maintain. So, go forth and build amazing things – with well-documented, robust APIs at your core!