The Basics of Swift for iOS Development: Dive into the world of app creation! Forget cryptic code; we’re breaking down Swift’s fundamentals, from setting up Xcode to crafting killer UIs. This isn’t your grandpappy’s programming – we’re talking sleek syntax, powerful tools, and the potential to build the next big iOS hit. Get ready to unleash your inner app developer.
This guide walks you through the essential building blocks of Swift programming for iOS. We’ll cover everything from basic data types and control flow to object-oriented programming and designing user interfaces with UIKit. We’ll even tackle common pitfalls and offer tips to streamline your coding journey. By the end, you’ll have a solid foundation to build upon, whether you’re a complete newbie or looking to brush up on your skills.
Introduction to Swift and iOS Development
So, you want to build the next killer app? Great! But before you start dreaming of App Store riches, you need to learn Swift, the language that powers iOS, macOS, watchOS, and tvOS. This isn’t just another coding language; it’s your key to unlocking the potential of Apple’s ecosystem. Let’s dive into the world of Swift and iOS development.
Swift’s History and Evolution
Swift’s journey began in 2010, as a secret project within Apple. It emerged from the ashes of Objective-C, Apple’s previous go-to language, aiming to address its complexities and limitations. Officially unveiled in 2014, Swift quickly gained popularity due to its cleaner syntax, improved performance, and modern features. Over the years, it’s undergone significant updates, each iteration bringing enhancements in safety, speed, and expressiveness. The transition from Objective-C to Swift marked a significant leap forward in iOS development, making it more accessible to a broader range of developers.
Advantages of Swift for iOS Development
Compared to other languages like Objective-C or Java, Swift offers several key advantages for iOS development. Its concise syntax reduces development time and improves code readability, making it easier to maintain and collaborate on projects. Swift’s built-in safety features help prevent common coding errors, leading to more robust and reliable apps. Furthermore, its performance is exceptional, resulting in faster and more responsive applications. The strong community support and extensive documentation further solidify Swift’s position as a leading choice for iOS development.
Setting Up the Development Environment
Building your first iOS app requires a few essential tools. First, you’ll need a Mac computer running macOS. Next, download and install Xcode, Apple’s integrated development environment (IDE). Xcode provides everything you need – a code editor, compiler, debugger, simulator, and more – all in one package. The installation process is straightforward; simply download the Xcode installer from the Mac App Store and follow the on-screen instructions. Once Xcode is installed, you’re ready to start coding!
Creating a “Hello, World!” Program
Let’s create the quintessential first program – “Hello, World!” Open Xcode and create a new project. Select the “App” template under iOS. Give your project a name (e.g., “HelloWorld”) and choose Swift as the language. Xcode will generate a basic project structure. Locate the `ContentView.swift` file and replace its contents with the following code:
“`swift
import SwiftUIstruct ContentView: View
var body: some View
Text(“Hello, World!”)struct ContentView_Previews: PreviewProvider
static var previews: some View
ContentView()“`
Let’s break it down:
* `import SwiftUI`: This line imports the SwiftUI framework, which provides tools for building user interfaces.
* `struct ContentView: View`: This declares a structure named `ContentView` that conforms to the `View` protocol, making it a view in SwiftUI.
* `var body: some View`: This is a computed property that returns the view’s content.
* `Text(“Hello, World!”):` This creates a text view displaying “Hello, World!”.
* `struct ContentView_Previews`: This is a preview provider for the view, allowing you to see a preview in Xcode.
Run the program using the play button in Xcode. You should see “Hello, World!” displayed in the simulator. Congratulations, you’ve just written your first Swift program!
Basic Swift Syntax and Data Types
So, you’ve dipped your toes into the world of Swift and iOS development. Awesome! Now let’s get down to the nitty-gritty: the fundamental building blocks of any Swift program – variables, constants, and data types. Think of them as the LEGO bricks of your app; you’ll need to master them to build anything truly amazing.
Swift is a strongly-typed language, meaning you need to explicitly or implicitly declare the type of data a variable or constant holds. This helps prevent common programming errors and makes your code easier to read and understand. Let’s explore the core concepts.
Variables and Constants
Variables and constants are used to store data within your program. The key difference lies in their mutability: variables can be changed after they’re declared, while constants remain fixed. Think of a variable as a sticky note you can rewrite, and a constant as a permanent marker inscription. You declare a variable using the var
and a constant using let
.
Example:
var myVariable = "Hello" // This is a variable; its value can change
myVariable = "World"
let myConstant = 42 // This is a constant; its value cannot change
Data Types
Swift offers a variety of built-in data types to handle different kinds of information. Here are some of the most common ones:
Int
: Represents whole numbers (e.g., 10, -5, 0).Double
: Represents 64-bit floating-point numbers (e.g., 3.14159, -2.5).Float
: Represents 32-bit floating-point numbers (generally less precise than Double).String
: Represents text (e.g., “Hello, Swift!”, “This is a string”).Bool
: Represents Boolean values (true
orfalse
).
Operators
Operators are symbols that perform operations on values. Swift supports various types of operators:
Arithmetic Operators
These operators perform basic mathematical calculations:
+
(addition)-
(subtraction)*
(multiplication)/
(division)%
(modulo – remainder after division)
Example:
let sum = 10 + 5 // sum will be 15
let difference = 10 - 5 // difference will be 5
let product = 10 * 5 // product will be 50
let quotient = 10 / 5 // quotient will be 2
let remainder = 10 % 3 // remainder will be 1
Comparison Operators
These operators compare two values and return a Boolean result:
==
(equal to)!=
(not equal to)>
(greater than)<
(less than)>=
(greater than or equal to)<=
(less than or equal to)
Example:
let isEqual = 10 == 10 // isEqual will be true
let isNotEqual = 10 != 5 // isNotEqual will be true
Logical Operators, The Basics of Swift for iOS Development
These operators combine or modify Boolean values:
&&
(logical AND – true only if both operands are true)||
(logical OR – true if at least one operand is true)!
(logical NOT – inverts the Boolean value)
Example:
let isTrue = true && true // isTrue will be true
let isFalse = true && false // isFalse will be false
let isAlsoTrue = true || false // isAlsoTrue will be true
let isNotTrue = !true // isNotTrue will be false
Type Inference and Type Casting
Swift's type inference system automatically determines the type of a variable or constant based on its value. This reduces the amount of code you need to write. Type casting allows you to convert a value from one type to another.
Example (Type Inference):
let myNumber = 42 // Swift infers that myNumber is an Int
let myString = "Hello" // Swift infers that myString is a String
Example (Type Casting):
let integerValue: Int = 10
let doubleValue: Double = Double(integerValue) // Cast Int to Double
let stringValue: String = String(integerValue) // Cast Int to String
Naming Conventions and Code Style
Following consistent naming conventions and code style makes your Swift code more readable and maintainable. Use descriptive names for variables and constants (e.g., userName
instead of u
), and follow the standard camel case convention (e.g., myVariableName
).
Swift also encourages the use of consistent indentation and spacing to improve code readability. Leverage Xcode's built-in code formatting features to maintain a clean and organized codebase.
Control Flow and Loops
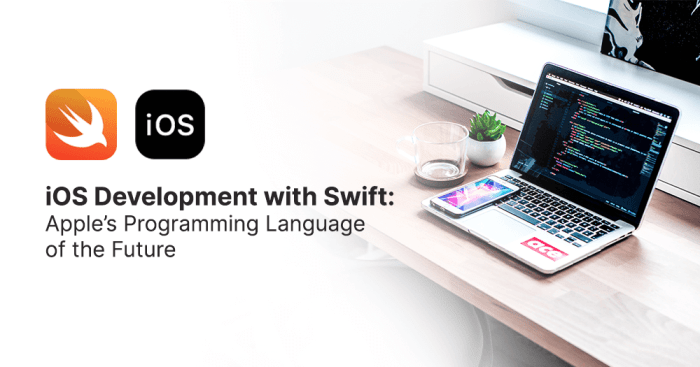
Source: mobisoftinfotech.com
Swift, like most programming languages, lets you control the order in which your code executes. This is crucial for creating dynamic and responsive apps. Control flow dictates whether a section of code runs or is skipped, and loops allow you to repeat code blocks efficiently. Mastering these concepts is fundamental to building robust iOS applications.
Imagine building a game where a character's actions depend on their current health or score. Or picture an app that displays different content based on user preferences. Control flow and loops are the tools that bring these functionalities to life. Let's dive into the specifics.
Conditional Statements
Conditional statements allow your code to make decisions based on different conditions. The primary structure uses `if`, `else if`, and `else` s. The `if` statement evaluates a Boolean expression (true or false). If true, the code block within the `if` statement executes. If false, the code proceeds to the `else if` statement (if present), and so on, finally reaching the `else` block if all preceding conditions are false.
Here’s a simple example: Let's say we want to determine if a user is eligible for a discount based on their age:
let age = 25
if age < 18
print("You are not eligible for a discount.")
else if age >= 18 && age < 65
print("You are eligible for a 10% discount.")
else
print("You are eligible for a 20% senior discount.")
This code snippet checks the `age` variable. If it's below 18, a specific message is printed. If it's between 18 and 64, a different message is shown, and if it's 65 or older, another message indicating a senior discount is displayed. The `&&` operator represents a logical AND, ensuring both conditions must be true for the `else if` block to execute.
Loop Structures
Loops are used to execute a block of code repeatedly. Swift offers several types of loops: `for`, `while`, and `repeat-while`.
Choosing the right loop depends on your specific needs. Understanding their differences is key to writing efficient and readable code.
For Loop
The `for` loop is ideal for iterating over a sequence, like an array or a range of numbers.
let names = ["Alice", "Bob", "Charlie"]
for name in names
print("Hello, \(name)!")
for i in 1...5 //Closed range (includes 5)
print("Number: \(i)")
for i in 1..<5 //Half-open range (excludes 5) print("Number: \(i)")
The first example iterates through the `names` array, printing a greeting for each name. The second and third examples demonstrate the use of ranges to iterate through numbers. The `...` operator creates a closed range (inclusive), while `..<` creates a half-open range (exclusive of the upper bound).
While Loop
The `while` loop repeats a block of code as long as a given condition is true. It's useful when you don't know the exact number of iterations in advance.
var counter = 0
while counter < 3
print("Counter: \(counter)")
counter += 1
This code prints the value of `counter` until it reaches 3. The loop continues as long as `counter` is less than 3.
Repeat-While Loop
The `repeat-while` loop is similar to `while`, but it guarantees at least one execution of the code block before checking the condition.
var counter = 0
repeat
print("Counter: \(counter)")
counter += 1
while counter < 3
This loop functions identically to the `while` loop example above in terms of output, but the code inside the `repeat` block will always run at least once, even if the condition `counter < 3` is initially false.
Nested Loops and Pattern Creation
Nested loops involve placing one loop inside another. This is powerful for creating patterns or processing multi-dimensional data. Let's create a simple multiplication table using nested loops:
for i in 1...5
for j in 1...5
print("\(i) x \(j) = \(i * j)", terminator: "\t")
print()
This code will produce a 5x5 multiplication table. The outer loop iterates through rows, and the inner loop iterates through columns. `terminator: "\t"` adds a tab after each calculation, creating a neat table format, and `print()` adds a new line after each row.
So you're diving into the basics of Swift for iOS development? Awesome! Building your app is like building a life, you need the right protection. That's where understanding how to choose the right insurance broker comes in, check out this guide: How to Choose the Right Insurance Broker for Your Needs. Once you've got your coverage sorted, you can focus on mastering those Swift closures and building that killer app!
Loop Structure Comparison
The choice between `for`, `while`, and `repeat-while` depends on the specific task. `for` is best for iterating over known sequences. `while` and `repeat-while` are suitable for situations where the number of iterations isn't predetermined, with `repeat-while` ensuring at least one iteration. Choosing the right loop enhances code readability and efficiency.
Working with Functions: The Basics Of Swift For IOS Development
Functions are the building blocks of reusable code in Swift. Think of them as mini-programs within your larger program, allowing you to organize your code, avoid repetition, and make it easier to understand and maintain. Using functions improves code readability and makes debugging a breeze.
Functions in Swift are defined using the `func` , followed by the function name, parameters (if any), a return type (if any), and the function body enclosed in curly braces ``. They're incredibly versatile and essential for efficient iOS development.
Function Syntax and Definition
The basic syntax for defining a function involves specifying its name, parameters (input values), and return type (output value). Let's break it down:
```swift
func functionName(parameter1: ParameterType, parameter2: ParameterType) -> ReturnType
// Function body: code to be executed
return returnValue
```
For example, a function to add two integers:
```swift
func addTwoNumbers(num1: Int, num2: Int) -> Int
return num1 + num2
```
Calling this function is straightforward:
```swift
let sum = addTwoNumbers(num1: 5, num2: 10) // sum will be 15
```
Function Parameters: In-Out and Optional
Swift offers flexibility in handling function parameters. In-out parameters (`inout`) allow a function to modify the original value of a variable passed to it. Optional parameters (`?`) can be omitted when calling the function.
Here's an example demonstrating both:
```swift
func modifyValue(value: inout Int, optionalValue: Int? = 0)
value += 10
if let optional = optionalValue
value += optional
var myNumber = 5
modifyValue(value: &myNumber, optionalValue: 5) // myNumber will now be 20
modifyValue(value: &myNumber) // myNumber will now be 30
```
Notice the `&` before `myNumber` when passing it as an `inout` parameter. The `optionalValue` parameter has a default value of 0, so it's optional.
Example: Calculating the Area of a Circle
Let's create a function to calculate the area of a circle given its radius:
```swift
func calculateCircleArea(radius: Double) -> Double
return Double.pi * radius * radius
let area = calculateCircleArea(radius: 5.0) // area will be approximately 78.5398
```
This function takes the radius as a `Double` and returns the calculated area, also as a `Double`. It uses the built-in `Double.pi` constant for the value of π. This showcases a practical application of functions – encapsulating a specific calculation into a reusable piece of code.
Data Structures in Swift
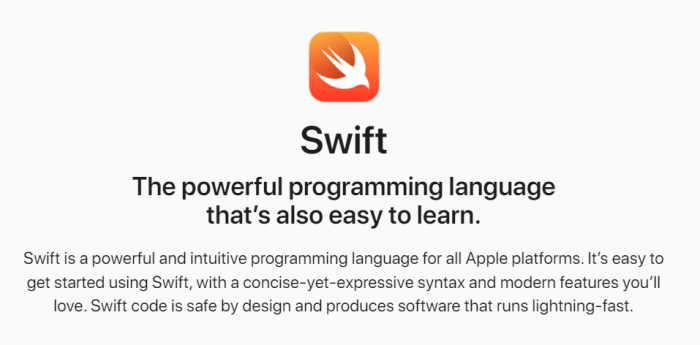
Source: hoisolutions.com
Swift offers several built-in data structures to organize and manage collections of data efficiently. Understanding these structures—arrays, dictionaries, and sets—is crucial for building robust and scalable iOS applications. Choosing the right data structure depends heavily on how you intend to access and manipulate your data.
Arrays in Swift
Arrays are ordered collections of values of the same type. Think of them like numbered lists; each item has a specific index starting from zero. This makes accessing elements by their position straightforward. Arrays are perfect when the order of your data matters and you need quick access to elements based on their index.
Creating an array is simple: let numbers = [1, 2, 3, 4, 5]
. You can access elements using their index: print(numbers[0]) // Output: 1
. Modifying an array is equally easy; you can append elements using numbers.append(6)
or insert elements at specific indices using numbers.insert(0, at: 0)
. Remember that arrays are value types, meaning assigning one array to another creates a copy.
Dictionaries in Swift
Dictionaries, unlike arrays, store data in key-value pairs. Each value is associated with a unique key, allowing for fast lookups based on the key. This is ideal when you need to access data based on a specific identifier rather than its position in a sequence. Imagine a contact list where each contact (value) is associated with a phone number (key).
Creating a dictionary: let contact = ["name": "Alice", "phone": "555-1212"]
. Accessing values is done using the key: print(contact["name"]!) // Output: Alice
(Note the `!` – force unwrapping is used here because we're certain the key exists. Consider using optional binding for safer access). Adding or modifying entries is straightforward: contact["email"] = "[email protected]"
. Dictionaries, like arrays, are value types.
Sets in Swift
Sets are unordered collections of unique values. They are particularly useful when you need to ensure that you don't have duplicate entries and the order doesn't matter. Imagine tracking unique user IDs or managing a list of distinct items in a shopping cart.
Creating a set: let uniqueNumbers: Set = [1, 2, 3, 2, 1] // Results in [1, 2, 3]
. Notice how duplicate values are automatically removed. You can add elements using uniqueNumbers.insert(4)
and check for membership using uniqueNumbers.contains(3) // Output: true
. Sets are value types.
Comparison of Data Structures
Characteristic | Array | Dictionary | Set |
---|---|---|---|
Order | Ordered | Unordered | Unordered |
Uniqueness | Allows duplicates | Allows duplicates (for values, not keys) | Unique values only |
Access | By index | By key | Membership check |
Use Cases | Lists, sequences | Key-value data, lookups | Unique identifiers, removing duplicates |
Object-Oriented Programming (OOP) Concepts in Swift
Swift, Apple's powerful programming language, embraces object-oriented programming (OOP) principles to create robust and maintainable iOS applications. Understanding OOP concepts is crucial for building complex and scalable apps. This section dives into the core elements of OOP in Swift, focusing on classes, structures, protocols, and the principles of inheritance, polymorphism, and encapsulation.
Classes and Structures
Classes and structures are fundamental building blocks in Swift's OOP model. Both define blueprints for creating instances (objects) with properties (data) and methods (functions). However, they differ significantly in their behavior. Classes support inheritance, allowing one class to inherit properties and methods from another, while structures do not. Structures are value types, meaning copies are created when passed around, ensuring data integrity. Classes, on the other hand, are reference types, sharing a single instance across multiple references.
Inheritance
Inheritance is a powerful mechanism that allows a class (subclass or derived class) to inherit properties and methods from another class (superclass or base class). This promotes code reusability and reduces redundancy. For example, a `SportsCar` class could inherit from a `Car` class, inheriting properties like `model` and `color` and adding its own specific properties like `horsepower` and `turbocharged`. The subclass can also override or extend methods inherited from the superclass.
Polymorphism
Polymorphism, meaning "many forms," allows objects of different classes to be treated as objects of a common type. This is particularly useful when working with collections of objects where you need to perform the same operation on objects of different classes. In Swift, polymorphism is achieved through protocols and inheritance. A method defined in a protocol can be implemented differently by different classes conforming to that protocol.
Encapsulation
Encapsulation involves bundling data (properties) and methods that operate on that data within a class or structure, protecting the internal state from direct external access. This is achieved using access control modifiers like `public`, `private`, and `internal`. Encapsulation enhances code organization, maintainability, and prevents unintended modifications to an object's internal state.
Creating and Using Classes and Structures
Let's illustrate with a simple example: a `Car` class.
```swift
class Car
var model: String
var color: String
var speed: Int = 0
init(model: String, color: String)
self.model = model
self.color = color
func accelerate()
speed += 10
print("Accelerating... Current speed: \(speed) mph")
let myCar = Car(model: "Toyota Camry", color: "Silver")
myCar.accelerate() // Output: Accelerating... Current speed: 10 mph
```
This code defines a `Car` class with properties for `model`, `color`, and `speed`, and a method `accelerate()`. We create an instance of the `Car` class and call the `accelerate()` method. A similar structure could be created using a `struct` instead of a `class`, but without inheritance capabilities. The choice between class and struct depends on the specific needs of your application.
Introduction to iOS UI Design with UIKit
Building amazing iOS apps isn't just about coding logic; it's about crafting intuitive and visually appealing user interfaces. UIKit, Apple's powerful framework, is your key to unlocking this world of design. It provides a comprehensive set of tools and components to create interactive and engaging experiences for your users. Let's dive into the basics.
UIKit offers a vast library of pre-built UI elements, allowing you to quickly assemble complex interfaces. Mastering these elements is the foundation of effective iOS app design.
Basic UI Elements in UIKit
UIKit provides a range of essential UI elements, each serving a specific purpose in the user interface. Understanding their functionality and appropriate usage is crucial for creating effective and user-friendly apps. These elements work together to form the visual structure and interactive components of your app.
- UILabel: Displays static text to the user. Think of it as a simple text label, perfect for headings, descriptions, or any other non-editable text information.
- UIButton: A clickable button that triggers an action when tapped. Buttons are vital for user interaction, allowing them to navigate, submit information, or initiate various app functions.
- UITextField: Allows users to input text. This is essential for forms, search bars, and any situation where you need user-provided text data.
- UIImageView: Displays images within your app. This is essential for incorporating visual elements, such as logos, icons, or photographs.
- UISwitch: A toggle switch allowing users to turn features on or off. This provides a simple and intuitive way for users to control binary settings.
- UISlider: Lets users select a value within a range. Useful for controlling volume, brightness, or any setting requiring a continuous value selection.
Creating a Simple iOS App with a Basic User Interface
Let's imagine a simple "Hello, World!" app. We'll use a single view controller, placing a label displaying "Hello, World!" at the center of the screen. This illustrates the fundamental process of adding UI elements to a view. The code would involve creating a UILabel object, setting its text property to "Hello, World!", and then adding it to the view hierarchy of the view controller. The precise code would depend on the chosen development environment (Xcode) and the specific implementation details.
Designing a User Interface for a Simple Calculator App
A simple calculator provides a perfect example of utilizing multiple UIKit elements to create a functional and user-friendly interface. The design should prioritize clarity and ease of use.
- Display: A large `UILabel` at the top to show the current calculation and result. This should be prominently positioned, easily visible to the user.
- Number Buttons (0-9): Nine `UIButton` objects for digits 0-9, arranged in a 3x3 grid. These buttons are the core of the calculator's input mechanism.
- Operator Buttons (+, -, *, /): Four `UIButton` objects for basic arithmetic operations. These buttons should be clearly distinguishable from the number buttons.
- Equals Button (=): A `UIButton` to trigger the calculation. This button should be visually distinct and easily accessible.
- Clear Button (C): A `UIButton` to clear the display and reset the calculator. This button should be easily identifiable and placed strategically.
The layout should be clean and organized, with buttons evenly spaced and clearly labeled. Consider using a grid layout to ensure consistent spacing and visual appeal. The display label should be large enough to easily accommodate numbers and operators. The overall design should be intuitive and easy to navigate, minimizing cognitive load for the user.
Handling User Input and Events
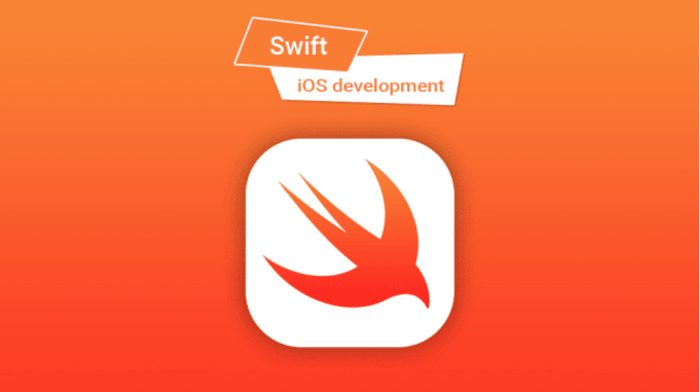
Source: com.ng
Building interactive iOS apps means making them responsive to user actions. This involves capturing events like button taps, text entry, and gestures, then using that information to update the app's state and provide feedback. Mastering user input handling is key to creating engaging and intuitive user experiences.
Swift provides several powerful mechanisms for handling user interactions. The most common methods involve using target-action patterns for simple events and delegates or closures for more complex scenarios, particularly when dealing with multiple interacting components. Understanding these techniques is crucial for building robust and responsive applications.
Target-Action Pattern
The target-action pattern is a simple and straightforward way to connect UI elements to actions. You assign a target (an object, usually a view controller) and an action (a method) to a control. When the control is activated (e.g., a button is tapped), the specified action method is invoked on the target object. This pattern is ideal for simple interactions where you don't need complex event handling logic. For instance, a button's `addTarget(_:action:for:)` method establishes this connection.
Imagine a button labeled "Submit." When the user taps it, you want to collect the text from a text field and process it. The target would be your view controller, and the action would be a method within that controller that handles the submission logic. The method might validate the input, send it to a server, or update the UI.
Delegates
Delegates provide a more sophisticated approach to handling events, particularly when dealing with multiple components. A delegate is an object that acts on behalf of another object (the delegating object). The delegating object notifies its delegate of significant events, allowing the delegate to respond accordingly. This design pattern promotes loose coupling and better code organization.
Consider a `UITableView`. The table view itself doesn't know how to handle the selection of a row; that logic resides in its delegate. When a row is selected, the table view informs its delegate through a delegate method. The delegate then handles the selection, perhaps navigating to a detail view or updating other parts of the UI.
Closures
Closures offer a concise and flexible way to handle events. A closure is a self-contained block of code that can be passed around and executed later. Many UI elements allow you to assign closures directly to handle specific events. This eliminates the need for separate delegate methods, making the code more compact and readable. For example, you might use a closure to handle a button tap, directly defining the actions within the closure's body.
Imagine a button with an action to update the UI based on the button's state. A closure would encapsulate this logic, directly updating the button's appearance and potentially other UI elements based on whether it's currently selected or not. This approach simplifies the code compared to using a delegate.
Error Handling and User Experience
Robust error handling is critical for a positive user experience. Unexpected errors can lead to app crashes or confusing behavior. Swift's error handling mechanisms, using `do-catch` blocks, allow you to gracefully handle errors and provide informative feedback to the user. For example, if a network request fails, you might display a message indicating the problem, rather than letting the app crash. Effective error handling is about preventing unexpected app behavior and providing users with helpful messages to guide them.
A well-designed user interface is crucial for providing clear feedback and guiding the user through the app. Visual cues, such as progress indicators, loading spinners, and confirmation messages, can significantly improve the user experience. For example, a progress bar during a lengthy operation keeps the user informed of the app's progress, preventing them from thinking the app has frozen. This proactive communication builds trust and makes the app feel more responsive.
Final Wrap-Up
Building iOS apps with Swift doesn't have to be a daunting task. By mastering the basics—from understanding data structures to designing intuitive user interfaces—you’ll be well on your way to creating your own amazing applications. So, ditch the intimidation and embrace the power of Swift. The app-building world awaits!