How to Build a Personal Finance Tracker App with Python? It sounds daunting, right? But imagine: a custom app, built by you, meticulously tracking your every penny. This isn’t some futuristic fantasy; it’s totally achievable with Python’s power and a dash of coding know-how. We’ll walk you through each step, from database design to deployment, turning your financial anxieties into a sleek, personalized solution.
This guide will equip you with the skills and knowledge to create a fully functional personal finance tracker. We’ll cover everything from planning and database design to building the user interface, implementing core logic, managing data persistence, and deploying your finished app. Get ready to take control of your finances, one line of code at a time!
Project Planning and Setup
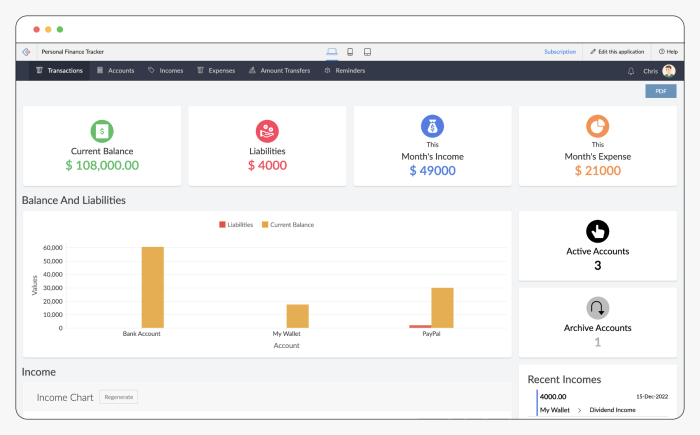
Source: zohowebstatic.com
Building a personal finance tracker app isn’t just about coding; it’s about meticulous planning. A well-defined scope ensures your project stays focused and manageable, preventing scope creep and ensuring a successful launch. This section Artikels the crucial steps in planning and setting up your personal finance tracker app development.
Defining Project Scope and Features
The first step is clearly defining what your app will and won’t do. For a personal finance tracker, this might include features like adding transactions, categorizing expenses, generating reports (monthly, yearly summaries), setting budgets, and visualizing spending habits through charts and graphs. Consider the minimum viable product (MVP) – the core features needed for a functional app – and prioritize them for initial development. Advanced features, like automated import from bank accounts or investment tracking, can be added later. Documenting these decisions helps prevent feature bloat and keeps the project on track. For example, your MVP might only include manual transaction entry, basic categorization, and simple summary reports.
Database Schema Design
A robust database is the backbone of your app. For storing financial transactions, a relational database is suitable. Here’s a sample schema:
Column Name | Data Type | Description |
---|---|---|
transaction_id | INTEGER (Primary Key) | Unique identifier for each transaction |
date | DATE | Date of the transaction |
description | VARCHAR(255) | Description of the transaction |
amount | DECIMAL(10,2) | Amount of the transaction (positive for income, negative for expense) |
category | VARCHAR(50) | Category of the transaction (e.g., Food, Rent, Entertainment) |
account_id | INTEGER (Foreign Key) | Reference to the account table |
An additional “accounts” table would store information about different accounts (e.g., checking, savings). This design allows for easy querying and reporting across different accounts and categories.
Project Plan
A well-structured project plan is essential. Break down development into phases: design, database setup, core functionality development, testing, and deployment. Allocate realistic timelines for each phase, considering potential delays. For resource allocation, consider the time commitment needed from developers and any external resources (e.g., cloud hosting). A Gantt chart can visually represent the project timeline and dependencies between tasks. For example, database setup should be completed before core functionality development can begin.
Python Libraries
Several Python libraries facilitate database interaction and app development. Here’s a comparison of four popular choices:
Library | Database Support | Pros | Cons |
---|---|---|---|
SQLAlchemy | PostgreSQL, MySQL, SQLite, and more | Object-Relational Mapper (ORM), flexible, powerful | Steeper learning curve |
psycopg2 | PostgreSQL | Fast, mature, widely used | Specific to PostgreSQL |
mysql.connector | MySQL | Official MySQL connector, well-documented | Specific to MySQL |
sqlite3 | SQLite | Built-in to Python, simple for small projects | Limited scalability |
Installation is typically done using pip: pip install sqlalchemy psycopg2 mysql-connector-python
(replace with the library you choose). Remember to install any additional libraries needed for your UI framework (e.g., Tkinter, PyQt, Kivy).
User Interface (UI) Design
Designing the user interface for your personal finance tracker app is crucial for its usability and overall success. A well-designed UI ensures users can easily input, view, and manage their financial data without frustration. This section will Artikel the design process, including the selection of a UI framework and the creation of visually appealing charts and graphs.
UI Framework Selection: Tkinter
For this project, we’ll use Tkinter. Tkinter is Python’s built-in GUI framework, making it readily accessible and requiring no additional installations. Its simplicity is ideal for a project of this scope, allowing us to focus on the core functionality of the finance tracker rather than getting bogged down in complex UI frameworks. While frameworks like PyQt offer more advanced features, Tkinter’s ease of use and readily available resources outweigh these advantages for this particular application. The learning curve is gentler, enabling quicker development and easier maintenance.
Screen Layouts and Navigation Flows
The application will feature three primary screens: a transaction input screen, a summary screen, and a settings screen. The transaction input screen will allow users to add new transactions, specifying the date, description, amount, and category (income or expense). The summary screen will display various charts and graphs summarizing the user’s financial data. A simple tabbed interface could be used to navigate between these screens, enhancing usability. Imagine a clean layout, with clear labels and intuitive input fields for the transaction input screen. The summary screen would feature prominently displayed charts, with clear titles and legends. Navigation between these screens will be achieved through easily identifiable buttons or tabs, placed strategically for ease of access.
Chart and Graph Creation
Visual representations of financial data are key to understanding financial trends. We’ll leverage Matplotlib, a powerful Python library for creating static, interactive, and animated visualizations.
Chart Types and Suitability
The application will utilize three primary chart types:
- Bar Chart: Ideal for comparing income and expenses across different categories (e.g., food, rent, entertainment). A bar chart clearly visualizes the relative proportions of each category, providing an immediate understanding of spending habits.
- Line Chart: Excellent for showing the net worth over time. A line chart effectively illustrates trends and fluctuations in net worth, allowing users to track their financial progress visually.
- Pie Chart: Useful for illustrating the proportion of income and expenses. A pie chart provides a quick overview of the distribution of funds, highlighting the largest contributors to income and expenses.
Style Guide
Maintaining a consistent visual style throughout the application is essential for user experience. We will employ a clean and minimalist design, using a neutral color palette (e.g., shades of gray and blue) to avoid visual clutter. Fonts will be legible and consistent across all screens. Clear and concise labels will be used throughout, ensuring that all elements are easily understood. Buttons and interactive elements will have a consistent size, shape, and style to maintain visual harmony. The overall look will be modern and uncluttered, prioritizing functionality and ease of use.
Core Application Logic: How To Build A Personal Finance Tracker App With Python
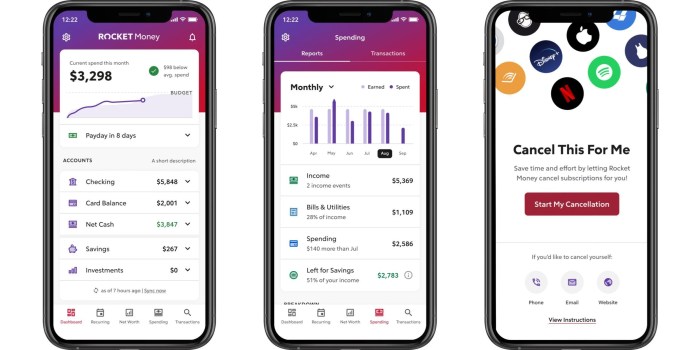
Source: pcmag.com
Building the brains of your personal finance tracker involves crafting the algorithms that make it all work. This section dives into the core logic, from calculating your net worth to generating insightful reports and ensuring your data remains accurate and safe. We’ll cover the key algorithms, data validation techniques, and methods for handling user input and data persistence, all with code examples to get you started.
The heart of any personal finance tracker lies in its ability to accurately reflect your financial standing. This requires robust algorithms for managing transactions, calculating balances, and generating comprehensive reports. Data validation is crucial to prevent errors and ensure the reliability of your application. Finally, efficient methods for handling user input and persistent storage are essential for a smooth and reliable user experience.
Transaction Categorization
Categorizing transactions is key to understanding your spending habits. This involves using a combination of techniques, including matching and machine learning (for more advanced applications). Simple matching can categorize transactions based on predefined s associated with specific categories (e.g., “Starbucks” for “Food and Beverage”). More sophisticated approaches might involve training a machine learning model on your transaction history to automatically categorize new transactions with higher accuracy.
Balance Calculation
Calculating balances involves iterating through all transactions and summing up income and expenses. The algorithm should differentiate between debit and credit transactions. A simple approach uses a running total, updated with each new transaction. For example, a positive value represents income, increasing the balance, while a negative value represents an expense, decreasing the balance.
def calculate_balance(transactions):
balance = 0
for transaction in transactions:
if transaction['type'] == 'income':
balance += transaction['amount']
else:
balance -= transaction['amount']
return balance
Report Generation
Generating reports involves aggregating and summarizing transaction data based on various criteria, such as time periods (daily, weekly, monthly, yearly) or categories. This can be achieved by grouping transactions based on the chosen criteria and then calculating summary statistics, like total income, total expenses, and net balance for each group.
def generate_monthly_report(transactions, year, month):
monthly_transactions = [t for t in transactions if t['date'].year == year and t['date'].month == month]
# ... further processing to calculate totals for income, expenses, and net balance ...
return report_data
Data Validation
Data validation is crucial to maintain data integrity. This includes checks for valid data types (e.g., ensuring amounts are numeric), reasonable values (e.g., preventing negative income), and consistent formats (e.g., date formats). Regular expressions can be used to validate input formats, and range checks can ensure values are within acceptable limits. Error handling mechanisms should be implemented to gracefully manage invalid inputs.
So you’re diving into the world of Python and building a killer personal finance tracker app? Smart move! Managing your money is key, but remember, protecting your assets is just as crucial. That’s where understanding home insurance comes in; check out this guide on How to Protect Your Home from Legal Liabilities with Home Insurance to ensure you’re financially secure.
Once you’ve got your home covered, you can focus on perfecting your Python app and automating those savings!
def validate_transaction(transaction):
if not isinstance(transaction['amount'], (int, float)):
raise ValueError("Invalid transaction amount")
if transaction['amount'] < 0 and transaction['type'] == 'income':
raise ValueError("Income amount cannot be negative")
# ... other validation checks ...
return True
User Input and Data Persistence
Efficient methods for handling user input and data persistence are essential. User input can be handled using graphical user interfaces (GUIs) or command-line interfaces (CLIs). Data persistence can be achieved using various methods, including saving data to files (e.g., CSV, JSON) or using databases (e.g., SQLite). Choosing the appropriate method depends on the complexity of the application and the expected data volume.
For example, using a simple text file to store transactions might suffice for a small-scale personal finance tracker, while a database would be more suitable for a more complex application with a large number of transactions and users.
Data Management and Persistence
Building a robust personal finance tracker hinges on effectively managing and storing your financial data. This section dives into the nitty-gritty of choosing the right storage solution, implementing backups, and ensuring your financial information remains safe and secure. We'll explore different options and show you how to integrate them seamlessly into your Python application.
Choosing the right data storage mechanism is crucial for your app's performance and scalability. Factors like data volume, security needs, and your technical expertise will guide your decision.
SQLite Database Integration
SQLite is a lightweight, file-based database ideal for personal finance trackers. Its simplicity makes it easy to integrate into your Python application using the `sqlite3` module. You can define tables to store transactions, budgets, and other relevant financial data. For example, a "transactions" table might have columns for date, description, amount, category, and account. The `sqlite3` module provides functions for creating tables, inserting data, querying data, and updating records. This approach keeps your data locally, offering good performance and privacy, but lacks the scalability of cloud solutions. Example code snippets would demonstrate creating the database, defining the tables, and performing basic CRUD (Create, Read, Update, Delete) operations.
Cloud-Based Solutions
Cloud solutions like Google Firebase or AWS DynamoDB offer scalability and accessibility from multiple devices. Firebase, for example, provides a NoSQL database and authentication services, simplifying data management and user authentication. Integrating Firebase requires setting up a project, configuring the database rules, and using the Firebase Python SDK to interact with the database. While offering enhanced scalability and accessibility, cloud solutions introduce dependence on external services and may raise concerns about data privacy and security, depending on the chosen provider and configuration.
Data Backup and Recovery
Regular data backups are paramount. For SQLite, a simple approach is to create a copy of the database file periodically. More sophisticated techniques could involve version control systems like Git or using database backup utilities. For cloud solutions, leverage the built-in backup and restore functionalities offered by the respective cloud providers. Recovery procedures should be well-documented and tested to ensure you can restore your data quickly and easily in case of data loss or corruption. A well-defined recovery plan should include steps for restoring the database from a backup, verifying data integrity, and informing the user about the recovery process.
Data Security and Privacy
Protecting your financial data is crucial. For local databases, store the database file in a secure location and use strong passwords or encryption if your chosen database system supports it. For cloud solutions, configure appropriate security rules to restrict access to authorized users only. Consider implementing encryption both in transit and at rest to protect your data from unauthorized access. Always adhere to relevant data privacy regulations (like GDPR or CCPA) when handling user financial information. This might involve obtaining explicit consent, anonymizing data where possible, and providing users with control over their data.
Importing Data from External Sources, How to Build a Personal Finance Tracker App with Python
Many personal finance apps allow users to import data from bank statements or other financial institutions. This typically involves parsing the data from files (CSV, OFX, QFX, etc.) or using APIs provided by the financial institutions. Libraries like `pandas` can be used for efficient CSV parsing. Parsing other formats might require dedicated libraries or custom parsing logic. API-based import requires authentication and careful handling of rate limits imposed by the APIs. Data validation is essential to ensure the accuracy and consistency of imported data. Error handling is also critical to gracefully handle potential issues like incorrect file formats or API errors.
Testing and Deployment
Building a robust personal finance tracker isn't just about slick visuals and clever algorithms; it's about ensuring the app works flawlessly and delivers reliable results. This stage focuses on rigorous testing and deploying your creation to the world. We'll cover everything from unit tests to getting your app into the hands of users.
Testing Strategy
A comprehensive testing strategy is crucial for building user trust and preventing costly bugs. This involves a multi-layered approach that checks every aspect of the application, from individual functions to the overall user experience. We'll focus on three key testing types: unit tests, integration tests, and user acceptance testing (UAT). These tests are not optional; they're the bedrock of a reliable application.
Unit Testing
Unit tests verify individual components (functions, modules) of your code operate correctly in isolation. For example, you'd write unit tests to ensure your calculation functions (e.g., calculating total expenses) produce accurate results given various inputs. A good unit test suite uses a testing framework like `unittest` or `pytest` in Python, allowing for automated testing and reporting. These frameworks provide tools for asserting expected outputs against actual outputs. Consider using mocking techniques to isolate units from external dependencies during testing. For instance, you might mock database interactions to ensure a function's core logic works correctly without a real database connection.
Integration Testing
Integration tests examine how different parts of your application work together. This is where you test the interactions between your UI, core logic, and data management components. For instance, you would test the flow of saving a new transaction: does the UI correctly capture the data, does the core logic process it appropriately, and is the data stored correctly in the database? Integration tests help identify issues stemming from unexpected interactions between different modules. They are crucial to uncover integration problems that are difficult to detect with unit tests alone.
User Acceptance Testing (UAT)
UAT involves real users testing the application in a realistic environment. This helps identify usability issues and ensures the app meets user expectations. Recruit a small group of representative users to test the application, providing feedback on its functionality, usability, and overall user experience. UAT is invaluable for catching problems that might be missed during development and testing. UAT often uses feedback forms or structured interviews to collect user insights. This is your final check before releasing the application.
Deployment to Different Platforms
Deploying your application involves making it accessible to users. The deployment process varies depending on the platform.
Desktop Deployment
For desktop deployment, you'll typically create an executable file (`.exe` for Windows, `.app` for macOS) using tools like PyInstaller or Nuitka. These tools bundle your Python code, libraries, and any necessary resources into a single package. The process involves configuring the packaging tool with your application's files and dependencies, then running the build process to generate the executable. Consider providing installers (e.g., Inno Setup for Windows) to simplify the installation process for users.
Web Deployment
Web deployment involves hosting your application on a server. This often involves using a web framework like Flask or Django, which provides tools for handling HTTP requests and managing web pages. You'll need a web server (like Apache or Nginx) and a database server (like PostgreSQL or MySQL) to handle user requests and store application data. Deployment involves uploading your application code and associated files to the server and configuring the web server to serve your application. Consider using platforms like Heroku or AWS for simplified web deployment.
Packaging for Distribution
Packaging is the final step before releasing your application. For desktop applications, this involves creating installers that guide users through the installation process. For web applications, packaging might involve creating a deployment archive containing your application code, configurations, and other necessary files. This packaged application is ready for distribution to users through various channels, such as app stores (for mobile apps) or your own website. Ensure clear and concise instructions are included in the package.
Error Handling Mechanisms
Robust error handling is vital for a reliable application. Your application should gracefully handle unexpected situations, preventing crashes and providing informative messages to the user.
Exception Handling
Use `try...except` blocks in your Python code to catch and handle potential errors. For example, you might handle `FileNotFoundError` if a data file is missing or `ValueError` if the user inputs invalid data. Provide informative error messages to the user, guiding them on how to resolve the issue.
Input Validation
Validate user inputs to prevent errors caused by unexpected data. Check data types, ranges, and formats to ensure data integrity. For instance, you might check if an amount entered is a valid number and within a reasonable range.
Logging
Implement logging to record application events and errors. This helps in debugging and monitoring the application's performance. Python's `logging` module provides tools for recording events to files or consoles. Detailed logs are invaluable for identifying and resolving issues after deployment.
Advanced Features (Optional)
Level up your personal finance tracker app beyond the basics! This section explores optional features that can transform your app from a simple expense recorder into a powerful tool for managing your financial future. Adding these advanced features will require more development effort, but the user experience and overall value will significantly increase.
Integrating advanced functionalities like budgeting, investment tracking, and predictive analytics can provide users with valuable insights and empower them to make smarter financial decisions. The possibilities are vast, and the choices you make will depend on your users' needs and your technical expertise.
Budgeting Tools
A robust budgeting feature is crucial for effective financial management. Users should be able to create and track budgets across various categories (e.g., housing, transportation, food). The app could visually represent budget allocation using charts and graphs, providing a clear overview of spending habits. For example, a pie chart could show the percentage of the budget allocated to each category, while a bar chart could illustrate spending over time. Users should also be able to set budget limits for each category and receive alerts when approaching or exceeding those limits. This functionality could be implemented using Python libraries like Matplotlib or Seaborn for data visualization.
Goal Setting
Allowing users to set financial goals is another valuable addition. This could include saving for a down payment on a house, paying off debt, or building an emergency fund. The app can then track progress towards these goals, providing motivation and a clear path to achieving them. For example, users could input a goal amount and a target date, and the app would calculate the required monthly savings amount. Progress tracking could be visually represented using progress bars or timelines.
Investment Tracking
For users interested in investing, the app could include features to track investment portfolios. This might involve integrating with financial APIs to fetch real-time data on stock prices, mutual funds, or other investments. The app could calculate portfolio performance, providing insights into returns and risks. Security and data privacy are paramount when integrating with financial APIs; robust authentication and authorization mechanisms are essential. Consider using established and reputable APIs that prioritize security.
Expense Forecasting
Predictive analytics can provide users with valuable insights into their future spending. By analyzing past spending patterns, the app can forecast future expenses. This could involve using machine learning algorithms to identify trends and seasonality in spending. For example, the app could predict higher spending during the holiday season or identify recurring expenses that could be optimized. Simple forecasting techniques like moving averages could be used initially, with more sophisticated machine learning models implemented later. For example, a simple linear regression model could be used to predict future spending based on past trends.
Third-Party API Integration
Connecting your app to third-party APIs significantly enhances its functionality. Integrating with bank APIs allows users to automatically import transaction data, eliminating manual entry. Payment gateway integration enables users to make payments directly through the app. However, careful consideration must be given to security and privacy when handling sensitive financial data. Always adhere to the API provider's guidelines and prioritize secure data transmission methods. Consider using OAuth 2.0 for secure authorization.
Personalized Financial Insights and Recommendations
Leveraging the data collected, the app can generate personalized financial insights and recommendations. This could include suggestions for reducing expenses, optimizing investments, or setting more realistic financial goals. For example, the app could identify areas where the user is overspending compared to their budget or recommend investment strategies based on their risk tolerance and financial goals. This feature can utilize basic statistical analysis or more advanced machine learning techniques for personalized recommendations.
Machine Learning Applications
Machine learning can significantly improve the app's capabilities. For instance, anomaly detection can identify unusual transactions that might warrant further investigation (potentially fraudulent activity). Predictive modeling can forecast future expenses, helping users better manage their finances. Clustering algorithms can group similar transactions, making it easier for users to analyze their spending patterns. These features require familiarity with machine learning libraries like scikit-learn. A simple example could be using a decision tree classifier to flag potentially fraudulent transactions based on transaction amount, location, and merchant.
Closing Summary
Building your own personal finance tracker app with Python is a rewarding journey. You've learned to design databases, craft user interfaces, manage data, and deploy your creation. This isn't just about managing money; it's about gaining a deeper understanding of your financial habits and empowering yourself with the tools to build a brighter financial future. So, dust off your Python skills, dive in, and start building!