How to Build a RESTful API with Django? Think sleek, scalable backends powering your killer apps. This isn’t your grandpappy’s web development; we’re diving deep into the world of Django REST framework, building APIs that are not only functional but downright elegant. Get ready to master the art of crafting robust, efficient, and maintainable RESTful APIs, one line of code at a time.
We’ll cover everything from setting up your Django project and defining your models, to crafting serializers that handle data transformations seamlessly. We’ll explore the power of Viewsets, master the art of API routing, and even tackle authentication and authorization – ensuring your API is secure and ready for prime time. By the end, you’ll be equipped to build APIs that can handle anything you throw at them.
Setting up the Django Project and Environment: How To Build A RESTful API With Django
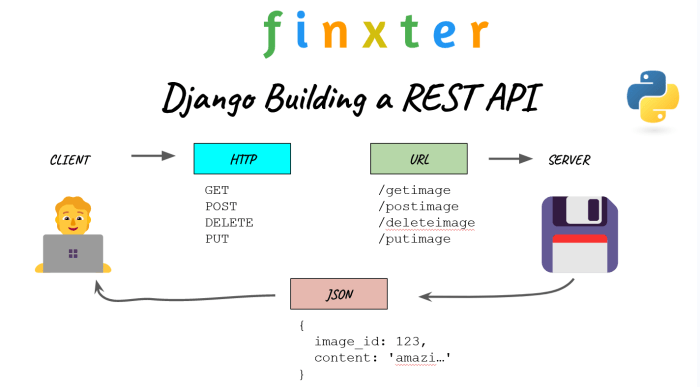
Source: finxter.com
Building a RESTful API with Django is like assembling a really cool Lego castle – you need the right bricks (packages) and a solid foundation (project setup). This section guides you through the crucial first steps: setting up your Django project and installing the necessary tools. We’ll make sure you’ve got everything you need before diving into the exciting world of API creation.
Creating a New Django Project
Creating a Django project is straightforward. We’ll use the command line (your terminal or command prompt) to initiate the process. This creates the basic structure for your entire application, including settings, URLs, and other essential files. Think of it as laying the groundwork for your magnificent API castle.
Installing Necessary Packages, How to Build a RESTful API with Django
Once your project’s foundation is laid, it’s time to add the essential packages. This involves using pip, Python’s package installer, to bring in Django REST framework and any other dependencies your API requires. Django REST framework is your go-to tool for building and managing APIs in Django. It provides tools for serialization, authentication, and more. Adding these packages is like adding the cool features to your Lego castle – towers, drawbridges, and secret passages!
Configuring settings.py
The `settings.py` file is the control center of your Django project. Here, you configure various aspects, including database settings, installed apps, static files, and more. For your API, you’ll need to ensure that the Django REST framework is included in the `INSTALLED_APPS` setting. This tells Django to use the framework’s functionalities within your project. Think of this as configuring the castle’s inner workings, making sure everything runs smoothly.
Project Setup and Package Installation Example
Let’s illustrate the process with a code example and a handy table. The following steps will guide you through setting up your project and installing the necessary packages. Remember to replace `myapi` with your desired project name.
Step | Command | Explanation | Output/Result |
---|---|---|---|
1. Create a virtual environment (recommended) | python3 -m venv .venv |
Creates an isolated environment for your project’s dependencies. | A new `.venv` directory containing the virtual environment. |
2. Activate the virtual environment | source .venv/bin/activate (Linux/macOS) .venv\Scripts\activate (Windows) |
Activates the virtual environment, ensuring your project uses its own dependencies. | Your terminal prompt will change, indicating the active environment. |
3. Create a Django project | django-admin startproject myapi |
Creates the basic structure for your Django project. | A new `myapi` directory containing project files. |
4. Create a Django app | cd myapi && python manage.py startapp api |
Creates a Django app specifically for your API. | A new `api` directory within `myapi`. |
5. Install Django REST framework | pip install djangorestframework |
Installs the necessary package for building your REST API. | The package is installed and ready to use. |
6. Add DRF to INSTALLED_APPS in settings.py | Add 'rest_framework', to the INSTALLED_APPS list in myapi/settings.py |
Registers the framework with your Django project. | The `settings.py` file is updated. |
Creating Serializers
Okay, so you’ve got your Django project humming along, and your models are all set. Now comes the crucial step of making your data talk to the outside world – that’s where serializers step in. Think of them as the friendly translators between your Python objects and the JSON that your API needs to send and receive. They’re the unsung heroes of any successful RESTful API.
Serializers in Django REST Framework (DRF) are classes that convert Python objects (like your Django models) into JSON (or other formats) and vice versa. This is essential for interacting with your API using HTTP requests. Without them, your beautifully crafted models would be trapped in their Python world, unable to share their wisdom with the rest of the internet.
Serializer Creation and Usage
Creating a serializer involves defining a class that inherits from `serializers.ModelSerializer` or `serializers.Serializer`. `ModelSerializer` is a shortcut if you’re working directly with Django models; it automatically creates fields based on your model’s fields. `Serializer` gives you more granular control if you need it. Let’s illustrate with a simple example: suppose we have a `BlogPost` model with `title` and `content` fields.
“`python
from rest_framework import serializers
from .models import BlogPost
class BlogPostSerializer(serializers.ModelSerializer):
class Meta:
model = BlogPost
fields = [‘id’, ‘title’, ‘content’, ‘created_at’] #Specify which fields to include
“`
This concise code defines a serializer for our `BlogPost` model. The `Meta` class specifies the model and which fields to serialize. Now, you can use this serializer to convert `BlogPost` instances to JSON:
“`python
post = BlogPost.objects.get(pk=1)
serializer = BlogPostSerializer(post)
json_data = serializer.data
print(json_data)
“`
This will print a JSON representation of the `BlogPost` object. To create a new `BlogPost` from JSON data, you would use the serializer’s `create()` method:
“`python
json_data = ‘title’: ‘My New Post’, ‘content’: ‘This is some amazing content!’
serializer = BlogPostSerializer(data=json_data)
if serializer.is_valid():
serializer.save()
“`
This code snippet attempts to create a new `BlogPost` instance from the provided JSON data. `is_valid()` checks for validation errors, and `save()` persists the new object to the database. Remember that validation is crucial for data integrity.
Serializer Field Options
Configuring serializer fields offers fine-grained control over how your data is represented. You can customize field types, add validators, and control read-only behavior. Let’s look at some key options:
Field Option | Description |
---|---|
read_only=True |
Makes the field read-only; it will be included in the serialized output but cannot be modified during deserialization (creation or updates). Useful for fields like IDs or automatically generated timestamps. |
required=False |
Allows the field to be omitted during deserialization. Useful for optional fields. |
default |
Specifies a default value for the field if it’s not provided during deserialization. |
validators |
Allows you to specify custom validation functions to enforce specific constraints on the field’s value. |
source |
Specifies the source of the field’s data. Useful for calculated fields or fields derived from related models. |
many=True |
Used with `ListSerializer` to serialize a list of objects. For example, if a `BlogPost` model has a `many-to-many` relationship with `Comment` model, you can use `many=True` to serialize all comments related to a specific post. |
Remember, mastering serializers is key to building robust and maintainable REST APIs. They handle the complex task of data transformation, leaving you free to focus on the bigger picture of your application’s logic.
Defining API Viewsets
Django REST framework’s Viewsets are a powerful tool for building clean, efficient, and maintainable APIs. They act as a more organized and streamlined approach compared to defining individual views for each HTTP method (GET, POST, PUT, DELETE) for each resource. Think of them as blueprints for your API endpoints, handling the common logic across different HTTP requests. This approach dramatically reduces code duplication and improves overall API design.
Viewsets provide a higher-level abstraction, allowing you to focus on the business logic of your API rather than getting bogged down in the intricacies of individual HTTP request handling. This makes your code more readable, easier to test, and simpler to maintain, especially as your API grows in complexity.
Defining API Viewsets for CRUD Operations
Viewsets in Django REST framework are classes that handle all the CRUD (Create, Read, Update, Delete) operations for a given resource. They achieve this by leveraging generic views which provide pre-built functionality for common HTTP methods. By inheriting from these generic views, you can easily create a Viewset that manages all CRUD operations without writing extensive code for each method individually.
Using Generic Views for Simplified API Development
The Django REST framework provides a suite of generic views (ListAPIView, RetrieveAPIView, CreateAPIView, UpdateAPIView, DestroyAPIView) that handle the most common HTTP methods for RESTful APIs. These generic views handle tasks like serialization, deserialization, and database interactions, making it easier to create complex APIs with minimal code. They also enforce consistent behavior across your API endpoints, improving maintainability and predictability.
Example: A Viewset for a Simple Blog Post Resource
Let’s illustrate how to create a Viewset for a “BlogPost” resource, handling all CRUD operations. We’ll use the generic views to minimize code duplication.
“`python
from rest_framework import viewsets
from .serializers import BlogPostSerializer
from .models import BlogPost
class BlogPostViewSet(viewsets.ModelViewSet):
queryset = BlogPost.objects.all()
serializer_class = BlogPostSerializer
“`
This concise code snippet defines a `BlogPostViewSet` that inherits from `viewsets.ModelViewSet`. `ModelViewSet` combines the functionality of `ListAPIView`, `RetrieveAPIView`, `CreateAPIView`, `UpdateAPIView`, and `DestroyAPIView`, providing all CRUD operations out of the box. We simply specify the `queryset` (the database query to retrieve objects) and the `serializer_class` (the serializer to use for data transformation).
* HTTP Methods Handled:
* GET: Retrieves a list of blog posts (using `ListAPIView` functionality). Retrieves a single blog post using the primary key (using `RetrieveAPIView` functionality).
* POST: Creates a new blog post (using `CreateAPIView` functionality).
* PUT: Updates an existing blog post (using `UpdateAPIView` functionality).
* DELETE: Deletes a blog post (using `DestroyAPIView` functionality).
This approach drastically simplifies API development, allowing developers to focus on the core logic of their application rather than the repetitive tasks of handling individual HTTP requests. The use of generic views ensures consistency and maintainability across all API endpoints, resulting in a more robust and scalable application.
Implementing API Endpoints and Routing
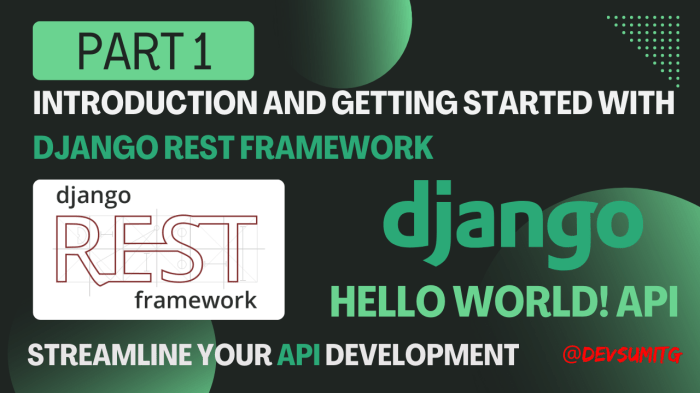
Source: medium.com
Building a robust RESTful API with Django requires careful planning, much like choosing the right insurance provider. Before you dive into serializers and views, consider the crucial aspects of your API’s design, just as you would check the claims process and customer service of a potential insurer by reading How to Evaluate the Quality of Your Insurance Provider.
A well-structured API, like a reliable insurance policy, offers peace of mind and long-term benefits. So, prioritize clean code and thorough testing from the get-go.
So you’ve got your Django project humming, your serializers are spitting out JSON like a champion, and your ViewSets are standing by, ready for action. Now it’s time to connect the dots – to actually make your API accessible. This involves defining the URLs that users will hit to interact with your data, and that’s where Django’s URL routing system comes in. We’ll explore how to elegantly map URLs to your ViewSets, making your API both powerful and user-friendly.
Defining API endpoints involves mapping specific URLs to the functions (your ViewSets) that handle requests to those URLs. Django’s URL dispatcher acts as the central traffic controller, directing incoming requests to the appropriate handler based on the requested URL. This process is crucial for building a well-structured and maintainable API. Think of it like a meticulously organized restaurant menu – each dish (API endpoint) has its designated section (URL path) and method of preparation (ViewSets handling GET, POST, etc.).
URL Routing in Django
Django’s URL routing system relies on regular expressions to match incoming URLs to specific views. You define URL patterns in a URLconf (URL configuration) file, typically located in your app’s `urls.py`. These patterns specify the URL structure and the associated view function. The dispatcher then uses these patterns to determine which view should handle a particular request. For example, a URL pattern like `r’^api/products/(?P
Using Routers in Django REST Framework
Django REST Framework provides a powerful feature called routers. Routers automate the process of generating URLs for your ViewSets. Instead of manually defining URL patterns for each action (list, create, retrieve, update, delete) of each ViewSet, you can use a router to handle this automatically. This significantly reduces boilerplate code and makes your URL configuration much cleaner and easier to manage.
The `DefaultRouter` is a commonly used router that automatically creates URL patterns for standard ViewSet actions. You simply register your ViewSets with the router, and it generates the necessary URL patterns.
Here’s how you might use a router:
“`python
from rest_framework.routers import DefaultRouter
from .views import ProductViewSet
router = DefaultRouter()
router.register(r’products’, ProductViewSet)
urlpatterns = [
path(”, include(router.urls)),
]
“`
This code snippet registers the `ProductViewSet` with the router for the URL path `/products`. The router automatically generates the URLs for listing products (`/products/`), retrieving a single product (`/products/pk/`), creating a product (`/products/`), updating a product (`/products/pk/`), and deleting a product (`/products/pk/`).
Using routers offers significant advantages: It reduces boilerplate code, improves code maintainability, and ensures consistency in URL structure across your API. This promotes a cleaner, more organized, and ultimately more efficient development workflow.
Configuring URL Patterns to Connect URLs to Viewsets
To connect your URLs to your ViewSets, you need to include the generated URLs from the router in your main URLconf (`urls.py`). This typically involves using `include()` to incorporate the router’s URLs into your project’s overall URL structure. The example above demonstrates this integration by including `router.urls` within the main URL patterns. This approach effectively maps URLs to the actions defined in your ViewSets, allowing your API to handle incoming requests efficiently. If you were not using a router, you would need to manually define each URL pattern, mapping each URL to the correct ViewSet method. This would be more complex and error-prone.
Implementing Authentication and Authorization
Securing your Django REST API is paramount. Without proper authentication and authorization, your API becomes vulnerable to unauthorized access and data breaches. This section details how to implement robust security measures, ensuring only authorized users can access specific resources. We’ll explore different authentication methods and demonstrate their implementation using Django’s built-in features.
Authentication verifies the identity of a user, while authorization determines what actions a user is permitted to perform. Think of it this way: authentication is like showing your ID to get into a building, while authorization is like getting a specific key to access a particular room within that building. Both are crucial for maintaining the integrity and security of your API.
Token-Based Authentication
Token-based authentication is a popular and widely used method for REST APIs. Instead of relying on session cookies, it uses tokens—unique strings—to authenticate users. These tokens are typically generated upon successful login and included in subsequent API requests as headers (usually `Authorization: Bearer
Implementing token-based authentication involves creating a view that handles user login, generating a token upon successful authentication, and then verifying this token in subsequent API requests. The token itself can be stored securely on the client-side (e.g., in local storage) and passed along with each request.
Session-Based Authentication
Session-based authentication leverages browser cookies to maintain user sessions. Upon successful login, the server creates a session and assigns a unique session ID to the user, storing it in a cookie on the client’s browser. Subsequent requests from the browser include this session ID, allowing the server to identify the user. While simpler to implement, session-based authentication is less scalable than token-based authentication and can be less secure in certain scenarios.
Django’s built-in session framework handles the complexities of session management, including session expiry and security. Using session authentication with a Django REST API generally involves configuring the appropriate authentication backend and potentially customizing session settings for enhanced security.
Django’s Authentication Backend and Permission Classes
Django’s authentication backend is responsible for verifying user credentials and authenticating users. Django REST Framework integrates seamlessly with Django’s authentication system. You can leverage Django’s existing user model and authentication mechanisms, or you can create custom authentication backends for specific needs.
Permission classes control authorization. They determine whether a user has permission to access a specific resource or perform a specific action. Django REST Framework allows you to define custom permission classes to implement fine-grained access control. For instance, you could create a permission class that only allows users with specific roles or permissions to access certain API endpoints.
Code Example: Token-Based Authentication with SimpleJWT
This example Artikels the basic steps for implementing token-based authentication using `djangorestframework-simplejwt`. Remember to install the package: `pip install djangorestframework-simplejwt`.
You’ll need to configure `SIMPLE_JWT` settings in your `settings.py` and potentially create custom views and serializers. The core concept involves generating an access token upon successful login and using this token for subsequent requests. Error handling and token refresh mechanisms are crucial for a robust implementation. Detailed configuration and usage examples can be found in the SimpleJWT documentation.
Common Authentication and Authorization Techniques
Choosing the right authentication and authorization method depends on your specific needs and security requirements. Here are some common techniques:
- Token-Based Authentication (JWT): Offers statelessness, scalability, and improved security compared to session-based authentication.
- Session-Based Authentication: Simpler to implement but less scalable and potentially less secure than token-based authentication.
- OAuth 2.0: A widely used authorization framework that allows third-party applications to access resources on behalf of a user without sharing their credentials.
- Basic Authentication: Simple but insecure method involving sending username and password in the request header.
- API Keys: Simple approach where clients use API keys for authentication, often combined with IP address restrictions for added security.
Testing the API
Building a rock-solid RESTful API isn’t just about crafting elegant endpoints; it’s about ensuring they behave predictably and reliably under pressure. Thorough testing is your secret weapon in achieving this, preventing embarrassing bugs and ensuring a smooth user experience. This section dives into the crucial world of API testing within the Django framework.
Testing Strategies for REST APIs
Testing your Django REST API involves employing a multi-pronged approach, combining different testing strategies to cover all bases. Unit tests focus on individual components, ensuring each piece works correctly in isolation. Integration tests, on the other hand, examine how these components interact, verifying the entire system functions as intended. Think of unit tests as checking individual LEGO bricks, and integration tests as making sure the entire LEGO castle stands strong.
Writing Tests for API Endpoints using Django’s Testing Framework
Django’s built-in testing framework provides a powerful and efficient way to test your API endpoints. You can use its `TestCase` class to create test cases, leveraging Django’s client to make requests to your API and assert expected responses. This allows you to verify that your endpoints return the correct data, handle errors gracefully, and adhere to expected HTTP status codes. Remember, the goal is to cover various scenarios, including successful requests, error conditions, and edge cases.
Testing API Functionality: Data Validation and Error Handling
Testing isn’t just about confirming correct responses; it’s about ensuring robustness. This involves rigorously testing data validation – confirming that your API rejects invalid input and handles it appropriately. Furthermore, robust error handling is crucial. Your tests should cover how the API reacts to various errors, ensuring informative and user-friendly error messages are returned. This builds a resilient API that can handle unexpected situations gracefully.
Test Cases with Django’s Testing Framework
Let’s illustrate with a concrete example. Suppose we have a simple API endpoint for creating blog posts. We’ll use Django’s `TestCase` and its client to test different scenarios: valid input, missing required fields, and invalid data types.
Test Case | Expected Result | Actual Result |
---|---|---|
Create a blog post with valid title and content | HTTP 201 Created, new blog post created in the database | HTTP 201 Created, blog post found in the database |
Attempt to create a blog post with a missing title | HTTP 400 Bad Request, error message indicating missing title | HTTP 400 Bad Request, error message indicating missing title |
Attempt to create a blog post with an excessively long title (exceeding character limits) | HTTP 400 Bad Request, error message indicating title length exceeded | HTTP 400 Bad Request, error message indicating title length exceeded |
Deploying the API
Getting your shiny new Django REST API into the hands of users (or other services) is the final, crucial step. Deployment might seem daunting, but with a structured approach, it’s manageable. We’ll explore several popular methods, focusing on practicality and scalability. Remember, the best choice depends on your project’s size, complexity, and infrastructure.
Deployment options for Django REST APIs offer a spectrum of choices, from simple setups for small projects to robust, scalable solutions for larger applications. Each option presents a unique set of trade-offs regarding ease of setup, scalability, and maintenance. Let’s dive into some of the most common approaches.
Deployment Options: Gunicorn, uWSGI, and Docker
Gunicorn (Green Unicorn) and uWSGI are WSGI HTTP servers that act as intermediaries between your Django application and a web server like Nginx or Apache. They handle requests efficiently, managing multiple worker processes to handle concurrent connections. Docker, on the other hand, provides containerization, packaging your application and its dependencies into a single, portable unit. This simplifies deployment across different environments (development, staging, production) and ensures consistency.
Deploying to a Production Environment
Deploying to production requires a careful, step-by-step process to minimize downtime and ensure a smooth transition. A well-defined deployment strategy is crucial for maintaining the API’s stability and reliability.
- Prepare your environment: This involves setting up a production-ready server (cloud instance, dedicated server, etc.) with the necessary dependencies (Python, Django, your API’s requirements). Ensure sufficient resources (CPU, RAM, storage) are allocated to handle the expected load.
- Configure your WSGI server (Gunicorn or uWSGI): This involves specifying the number of worker processes, binding address and port, and other settings relevant to your application’s needs. For example, a Gunicorn configuration file might specify the number of worker processes to handle concurrent requests efficiently, preventing bottlenecks. A sample configuration could be:
workers = 3, bind = '0.0.0.0:8000'
- Configure a reverse proxy server (Nginx or Apache): A reverse proxy sits in front of your WSGI server, handling tasks like load balancing, SSL termination, and static file serving. This improves security and performance. Nginx configuration would involve defining server blocks, setting up SSL certificates, and routing traffic to your Gunicorn/uWSGI server. For example, a simple Nginx configuration might direct all traffic on port 80 to your Gunicorn server listening on port 8000.
- Deploy your code: Use a version control system (Git) to manage your codebase. Implement a deployment strategy (e.g., using tools like Fabric or Ansible) to automate the process of transferring your code to the production server, restarting the WSGI server, and verifying the deployment.
- Monitor and log: Set up monitoring tools to track your API’s performance (response times, error rates, resource usage). Implement robust logging to help diagnose and troubleshoot issues. Tools like Prometheus and Grafana are commonly used for monitoring, providing valuable insights into your API’s health and performance.
Web Server Configuration
The web server (Nginx or Apache) acts as a gatekeeper, managing incoming requests and routing them to the appropriate backend server (Gunicorn or uWSGI). Proper configuration is essential for security, performance, and scalability. Key aspects include SSL configuration for secure HTTPS connections, load balancing to distribute traffic across multiple instances of your API, and caching to improve response times. For example, Nginx can be configured to use a load balancing algorithm to distribute requests across multiple Gunicorn worker processes, ensuring no single process becomes overloaded. The specific configuration will depend on the chosen web server and deployment setup.
Final Review
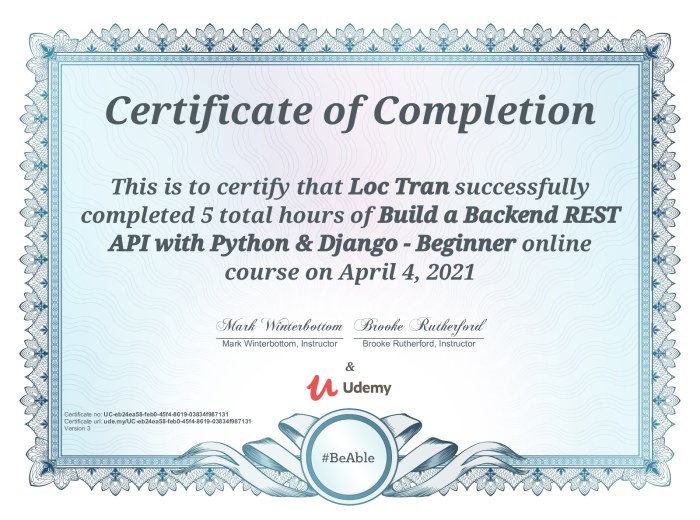
Source: com.au
Building a RESTful API with Django doesn’t have to be a Herculean task. By following the steps Artikeld here, you’ve armed yourself with the knowledge and skills to create robust, scalable, and secure APIs. Remember, practice makes perfect – so get coding, experiment, and watch your API skills soar. The world of web development awaits your beautifully crafted RESTful creations. Now go forth and build!