How to Create a Data-Driven Website with JavaScript and Node.js? Sounds daunting, right? But it’s not as scary as it seems. This guide breaks down building a dynamic, data-powered website using these powerful tools, from setting up your environment to deploying your finished product. We’ll cover everything from choosing the right database to handling user interactions, ensuring you build a website that’s not only functional but also engaging and user-friendly.
We’ll walk you through the entire process, from understanding core concepts like data fetching and manipulation to mastering backend development with Node.js and Express.js. Get ready to ditch static content and embrace the exciting world of dynamic websites!
Understanding the Fundamentals
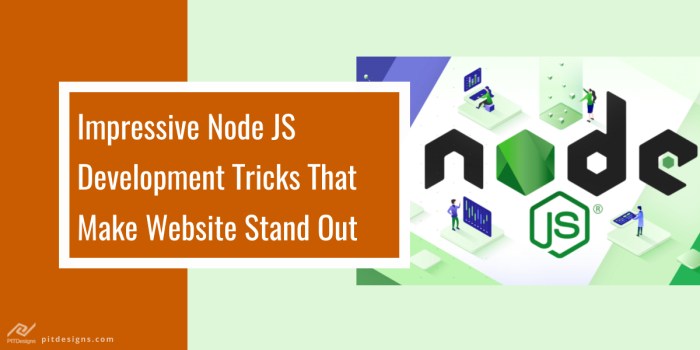
Source: pitdesigns.com
So, you want to build a data-driven website using JavaScript and Node.js? Awesome! This means you’re ready to move beyond static pages and create dynamic, engaging experiences tailored to your users. Let’s break down the core concepts and get you started.
Data-driven websites are all about leveraging data to personalize and enhance the user experience. Instead of fixed content, these sites adapt and respond based on user interactions, preferences, and even real-time information. Imagine a personalized news feed, a dynamic e-commerce product recommendation engine, or a website that adjusts its layout based on the device being used – that’s the power of data-driven design.
The Roles of JavaScript and Node.js
JavaScript is the undisputed king of front-end web development, handling all the client-side interactions that make your website feel responsive and interactive. Node.js, on the other hand, is a server-side JavaScript runtime environment. This means you can use the same language (JavaScript) for both the front-end and back-end, streamlining development and simplifying the process of handling data. Node.js excels at handling real-time data streams and building scalable applications. In a data-driven website, JavaScript handles the dynamic display of data to the user, while Node.js manages the fetching, processing, and serving of that data.
Data Sources for Integration
The beauty of data-driven websites lies in their ability to connect to various data sources. The possibilities are practically endless. Consider these examples:
- Databases: Relational databases like MySQL, PostgreSQL, or NoSQL databases like MongoDB are common choices for storing and managing website data. Think user profiles, product catalogs, blog posts, and more.
- APIs: Application Programming Interfaces (APIs) allow you to access data from external services. For instance, you might use a weather API to display current conditions on your site, a payment gateway API to process transactions, or a social media API to integrate user logins.
- CSV Files: Simple comma-separated value files are a great option for smaller datasets, especially during the initial development phases. They’re easy to import and manipulate.
- Spreadsheets: Similar to CSV files, spreadsheets can be a quick way to get started with a small amount of data. Google Sheets, for example, offers APIs for accessing and updating data.
Basic Architecture of a Data-Driven Website
A typical architecture for a data-driven website using JavaScript and Node.js involves several key components:
- Frontend (Client-Side): This is the part of the website users interact with directly. It’s built using HTML, CSS, and JavaScript. JavaScript handles fetching data from the server, updating the user interface, and managing user interactions.
- Backend (Server-Side): Powered by Node.js, this is where the magic happens. It’s responsible for connecting to the data source, processing requests from the frontend, and sending the appropriate data back. This might involve using a framework like Express.js to handle routing and requests.
- Database: This is where your website’s data is stored. The choice of database depends on the type and volume of data you’re handling.
A simple example: Imagine a blog. The frontend displays blog posts. When a user requests a post, the frontend sends a request to the Node.js backend. The backend fetches the post from the database and sends it back to the frontend for display.
Setting up the Development Environment
Building a data-driven website with JavaScript and Node.js requires a solid foundation. This section guides you through setting up your development environment, ensuring a smooth coding journey. We’ll cover installing the necessary tools, choosing a JavaScript framework, structuring your project, and connecting to a database. Let’s dive in!
Node.js and npm Installation
Node.js is the JavaScript runtime environment that powers our backend, while npm (Node Package Manager) manages our project’s dependencies. The installation process is straightforward and typically involves downloading the appropriate installer from the official Node.js website. Once installed, verify the installation by opening your terminal and typing `node -v` and `npm -v`. You should see the versions of Node.js and npm printed to the console. This confirms that everything is set up correctly. Remember to restart your terminal after installation to ensure the changes take effect.
Choosing and Installing a JavaScript Framework
Several excellent JavaScript frameworks are available for building user interfaces, each with its strengths and weaknesses. React, Vue, and Angular are popular choices. React, known for its component-based architecture and virtual DOM, offers flexibility and a large community. Vue, praised for its ease of learning and progressive adoption, is a great choice for smaller projects or those needing a gentle learning curve. Angular, a comprehensive framework, provides a structured approach suitable for large-scale applications. The choice depends on project requirements and developer preference. Installation usually involves using npm: `npm install -g
Sample Project Structure
A well-organized project structure is crucial for maintainability and scalability. Here’s a suggested structure for a data-driven website:
my-website/
├── client/ // Frontend code (React, Vue, or Angular)
│ ├── src/
│ │ ├── components/
│ │ ├── App.js (or equivalent)
│ │ └── ...
│ └── package.json
├── server/ // Backend code (Node.js)
│ ├── routes/
│ ├── models/
│ ├── controllers/
│ └── app.js
└── database/ // Database scripts and configurations
└── ...
This structure separates the frontend and backend code, promoting better organization and collaboration. The `client` directory houses the frontend code, while the `server` directory contains the backend logic. The `database` directory holds database-related scripts and configurations. This structure can be adapted based on project complexity.
Connecting to a Database
Connecting your application to a database is essential for managing and retrieving data. Popular choices include MongoDB (NoSQL) and PostgreSQL (SQL). For MongoDB, you’ll need the `mongodb` driver: `npm install mongodb`. For PostgreSQL, you might use the `pg` driver: `npm install pg`. Connecting typically involves establishing a connection string with your database credentials (hostname, port, database name, username, password). The specific code for connecting will depend on the chosen database and driver. For example, a simple MongoDB connection might look like this:
const MongoClient = require('mongodb');
const uri = "mongodb://: @ : / "; // Replace with your credentials
const client = new MongoClient(uri);async function run()
try
await client.connect();
console.log("Connected successfully to server");
finally
await client.close();run().catch(console.dir);
Remember to replace the placeholder values with your actual database credentials. Proper error handling and security measures are crucial when working with databases.
Data Fetching and Handling
Building a dynamic, data-driven website with JavaScript and Node.js hinges on efficiently fetching and managing data. This involves selecting the right tools, understanding asynchronous operations, and gracefully handling potential errors. Let’s dive into the nitty-gritty of getting your data where it needs to be.
Data fetching is the process of retrieving data from various sources – be it a local JSON file, a remote API, or a database. In JavaScript, we have several powerful tools at our disposal to achieve this. Choosing the right method depends on factors like the data source, the complexity of the data, and your project’s overall architecture.
JavaScript Fetch API and Axios
The JavaScript Fetch API and Axios are popular libraries for fetching data. The Fetch API is a built-in browser feature, providing a clean and modern approach to making network requests. Axios, on the other hand, is a third-party library offering additional features like automatic JSON transformation and better error handling. Both are excellent choices, and the best one for you will often depend on project-specific needs and preferences. Axios tends to be preferred for its extra features and ease of use in complex situations.
Here’s a simple example using the Fetch API to retrieve data from a JSONPlaceholder API:
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
And here’s the equivalent using Axios:
axios.get('https://jsonplaceholder.typicode.com/todos/1')
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
Synchronous vs. Asynchronous Data Fetching
Synchronous operations block the execution of subsequent code until they are completed. Asynchronous operations, in contrast, don’t block execution. They allow the program to continue running while waiting for the data to arrive. In web development, asynchronous data fetching is crucial for maintaining a responsive user interface. Imagine a website freezing while waiting for a large dataset to load – not a good user experience! Asynchronous fetching ensures that the user interface remains interactive while the data is being retrieved in the background.
Error Handling During Data Retrieval
Network requests can fail for various reasons – network connectivity issues, server errors, or even incorrect API endpoints. Robust error handling is paramount. Both the Fetch API and Axios provide mechanisms for handling errors gracefully. The `.catch()` block in the examples above demonstrates a basic approach. More sophisticated error handling might involve displaying user-friendly error messages, retrying the request after a delay, or implementing fallback mechanisms. For instance, you could display a “Loading…” message while fetching data and then an error message if the fetch fails.
Data Transformation and Manipulation
Once data is fetched, it often needs transformation or manipulation before it can be used effectively. JavaScript provides a wealth of tools for this, including array methods like `map`, `filter`, `reduce`, and object manipulation techniques.
Method | Description | Example | Use Case |
---|---|---|---|
map() |
Transforms each element of an array into a new element. | const doubled = numbers.map(number => number * 2); |
Creating a new array with modified values. |
filter() |
Creates a new array with elements that pass a certain condition. | const evenNumbers = numbers.filter(number => number % 2 === 0); |
Filtering out unwanted data. |
reduce() |
Reduces an array to a single value. | const sum = numbers.reduce((total, number) => total + number, 0); |
Calculating totals, averages, etc. |
Object.keys() , Object.values() , Object.entries() |
Methods to access keys, values, or key-value pairs of an object. | const keys = Object.keys(myObject); |
Iterating through object properties. |
Data Display and User Interaction
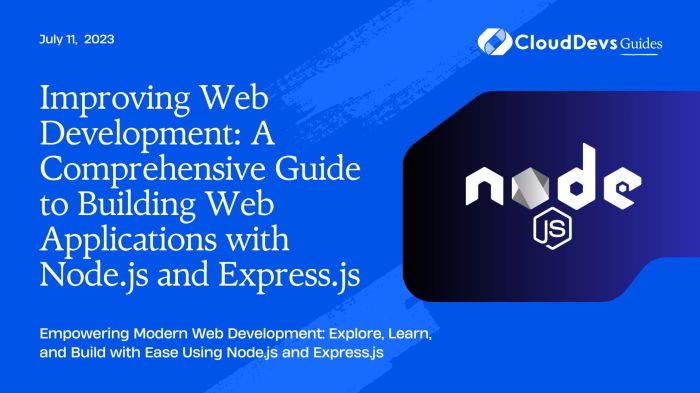
Source: cloudfront.net
Bringing your data to life on a website involves more than just fetching it; it’s about presenting it in a clear, engaging, and interactive way. Users need to easily understand the information, and they should be able to interact with it to gain further insights. This section dives into the crucial aspects of displaying data and creating a dynamic user experience. We’ll explore techniques to visualize data, handle user input, and ensure data integrity.
Dynamically updating the user interface (UI) based on user interactions is key to creating a responsive and engaging web application. This allows for a more intuitive and personalized experience, improving overall user satisfaction. By combining data fetching with UI manipulation, we can build powerful applications that adapt to user needs in real-time.
Interactive Data Visualization, How to Create a Data-Driven Website with JavaScript and Node.js
Interactive data visualization is all about presenting your data in a visually appealing and understandable manner, often using charts, graphs, and tables. Libraries like Chart.js, D3.js, and ApexCharts simplify the process. For instance, Chart.js allows you to easily create bar charts, line graphs, pie charts, and more, directly within your JavaScript code. Imagine a website displaying sales figures over time; a line chart would instantly show trends and patterns far more effectively than a simple table of numbers. Consider using appropriate chart types based on the nature of your data and the insights you want to convey. For example, a bar chart is excellent for comparing different categories, while a scatter plot is ideal for showing correlations between two variables. The choice of visualization tool should depend on your data and the story you want to tell.
Dynamic UI Updates
Updating the UI dynamically is essential for creating a responsive user experience. This involves using JavaScript to modify the HTML content of your webpage based on user actions or new data received. For example, imagine a search bar on an e-commerce website. As the user types, JavaScript can filter the displayed product list in real-time, providing immediate feedback. This is achieved by using JavaScript’s DOM manipulation capabilities to update the HTML elements displaying the product list. The process typically involves fetching new data based on the user’s input, then updating the relevant parts of the HTML to reflect the changes. Libraries like React, Vue, or Angular can simplify this process significantly by providing efficient mechanisms for managing the UI and updating it reactively.
User Input Forms and Data Submission
Creating user input forms allows users to interact directly with your data. These forms can collect various types of data, from simple text input to complex file uploads. Consider a simple form for collecting user feedback; this would likely involve text fields for name, email, and a larger text area for comments. JavaScript plays a crucial role in handling form submissions. It can validate the input data before submission, preventing errors and ensuring data integrity. Upon successful validation, JavaScript can send the data to your Node.js backend using AJAX or Fetch API for processing and storage. This ensures a seamless user experience without requiring a full page reload. Remember to implement client-side validation to provide immediate feedback to the user, and server-side validation to further ensure data security and consistency.
Data Validation
Data validation is crucial for maintaining data integrity. It involves checking user input to ensure it conforms to expected formats and constraints. For example, an email field should only accept valid email addresses, and a numerical field should only accept numbers within a specific range. JavaScript provides several methods for validating data, including regular expressions for pattern matching and built-in functions for type checking. Implementing robust validation prevents errors and improves the overall reliability of your application. Client-side validation provides immediate feedback to the user, while server-side validation adds an extra layer of security. Consider implementing both for comprehensive data validation. For instance, a form might check if a required field is filled using JavaScript, and the server might further validate the data against a database schema.
Backend Development with Node.js
Building the brains behind your data-driven website requires a robust backend. This is where Node.js, with its non-blocking, event-driven architecture, shines. We’ll leverage its power to create a RESTful API, the crucial link between your frontend JavaScript and your data source. Think of it as the silent, efficient worker behind the scenes, making sure everything runs smoothly.
Node.js, combined with the Express.js framework, provides a streamlined approach to building efficient and scalable APIs. Express.js simplifies the process of creating routes, handling requests, and sending responses, allowing us to focus on the core logic of our application rather than getting bogged down in low-level details. This section will guide you through creating and securing your API.
Creating RESTful APIs with Node.js and Express.js
Creating a RESTful API involves defining endpoints that allow for the standard CRUD (Create, Read, Update, Delete) operations on your data. Express.js provides a clean and intuitive way to define these routes. Each route maps an HTTP request method (GET, POST, PUT, DELETE) to a specific function that handles the request and sends a response. This allows for a clear and organized structure for managing your data interactions. We’ll use clear examples to illustrate the process.
Organizing API Endpoints for Data Management
A well-structured API is essential for maintainability and scalability. Consider grouping related endpoints logically. For example, if you’re managing blog posts, you might have endpoints like `/posts`, `/posts/:id`, `/posts/:id/comments`. This clear structure makes it easy to understand the API’s functionality and to add new features later. Consistency in naming conventions is also key.
Let’s consider a simple example of managing blog posts. Each post will have an `id`, `title`, `content`, and `author` field. We’ll use Express.js to handle requests for different operations on these posts. The following illustrates the structure:
GET /posts
: Retrieves a list of all blog posts.GET /posts/:id
: Retrieves a single blog post with the given ID.POST /posts
: Creates a new blog post.PUT /posts/:id
: Updates an existing blog post with the given ID.DELETE /posts/:id
: Deletes a blog post with the given ID.
Examples of API Responses in JSON Format
RESTful APIs typically return data in JSON (JavaScript Object Notation) format. This is a lightweight and widely used data-interchange format that’s easy for both servers and clients to parse. Here are examples of responses for the blog post API endpoints:
Example response for GET /posts
:
[
"id": 1, "title": "My First Post", "content": "This is the content of my first post.", "author": "John Doe",
"id": 2, "title": "Second Post", "content": "This is the content of my second post.", "author": "Jane Doe"
]
Example response for GET /posts/1
:
"id": 1,
"title": "My First Post",
"content": "This is the content of my first post.",
"author": "John Doe"
Example response for a successful POST /posts
(creating a new post):
"message": "Post created successfully",
"postId": 3
API Security and Authentication
Security is paramount when building APIs that handle sensitive data. Several strategies can be employed to secure your API. Implementing authentication mechanisms, such as using JSON Web Tokens (JWTs), is crucial to verify the identity of users accessing the API. Input validation and sanitization help prevent vulnerabilities like SQL injection and cross-site scripting (XSS). Using HTTPS ensures data is transmitted securely. Regular security audits and updates are essential to maintain a secure API. Failure to implement robust security measures can lead to data breaches and compromise user privacy.
Database Interaction
So, you’ve got your snazzy Node.js website fetching and displaying data like a champ. But where’s that data actually *coming from*? That’s where database interaction comes in – the secret sauce that makes your website truly dynamic and data-driven. We’ll explore how to connect your Node.js application to a database, perform crucial operations, and optimize your queries for peak performance. Get ready to dive deep into the heart of your data!
Connecting your Node.js application to a database involves choosing a database system (like PostgreSQL, MySQL, MongoDB, etc.), installing the appropriate database driver, and establishing a connection using your database credentials. The specific steps will vary depending on your chosen database, but the general process involves importing the driver, creating a connection pool, and then using that connection to execute queries. This allows your Node.js server to interact with the database, retrieving, inserting, updating, and deleting data as needed. Efficient database management is crucial for the performance and scalability of your website.
Performing Database Queries
Using a database driver (like `pg` for PostgreSQL or `mysql2` for MySQL), you can execute SQL queries to retrieve, insert, update, and delete data. For example, using the `pg` library for PostgreSQL, you’d execute a query like this:
const Pool = require('pg');
const pool = new Pool( /* connection details */ );
pool.query('SELECT * FROM users WHERE id = $1', [1], (err, res) =>
if (err)
console.error(err);
else
console.log(res.rows); // Access the retrieved data
);
pool.end();
This code snippet shows a basic query using parameterized queries to prevent SQL injection vulnerabilities. Remember to always sanitize user inputs to prevent security risks. Different drivers will have slightly different APIs, but the fundamental principle remains the same: you send a query to the database and receive a result.
Data Manipulation Operations
Beyond querying, you need to manage your data. Let’s look at the core operations:
* Insertion: Adding new data. For example, adding a new user to a `users` table might look like this (using parameterized queries for security):
pool.query('INSERT INTO users (name, email) VALUES ($1, $2)', ['New User', '[email protected]'], (err, res) =>
// Handle error or success
);
* Update: Modifying existing data. Updating a user’s email address:
pool.query('UPDATE users SET email = $1 WHERE id = $2', ['[email protected]', 1], (err, res) =>
// Handle error or success
);
* Deletion: Removing data. Deleting a user:
pool.query('DELETE FROM users WHERE id = $1', [1], (err, res) =>
// Handle error or success
);
Database Query Optimization
Efficient database queries are vital for a responsive website. Here are some key optimization strategies:
Optimizing database queries involves several techniques to ensure your application retrieves data quickly and efficiently. These optimizations can significantly impact the performance and responsiveness of your website, especially as your data volume grows.
Building a data-driven website with JavaScript and Node.js is all about smooth operations, but remember, even the slickest online booking system needs a solid foundation. That’s why, before you even think about launching your vacation rental site, check out this essential guide on Why You Should Never Skip Insurance for Your Vacation Rental Property to protect your investment.
Then, get back to crafting that killer user experience with your Node.js backend!
- Use indexes appropriately: Indexes speed up data retrieval by creating a searchable structure for specific columns. However, over-indexing can slow down write operations. Strategically index frequently queried columns.
- Avoid using `SELECT *`: Only select the columns you actually need. Fetching unnecessary data wastes resources.
- Optimize your `WHERE` clause: Use appropriate conditions and data types to ensure efficient filtering. Avoid using functions within the `WHERE` clause if possible.
- Use joins effectively: When working with multiple tables, choose the most efficient join type for your query.
- Analyze query execution plans: Use database tools to understand how your queries are being executed and identify potential bottlenecks.
Database Security Best Practices
Security is paramount when dealing with databases. Neglecting security can lead to data breaches and other serious consequences. Follow these best practices:
- Use parameterized queries (or prepared statements): This prevents SQL injection attacks, a common vulnerability.
- Validate and sanitize all user inputs: Never trust user-provided data. Always validate and sanitize it before using it in queries.
- Use strong passwords and access control: Restrict database access to authorized users only, using strong, unique passwords.
- Regularly back up your data: This ensures data recovery in case of failures or attacks.
- Keep your database software updated: Patches often address security vulnerabilities.
- Employ a robust firewall: Protect your database server from unauthorized access.
Deployment and Hosting: How To Create A Data-Driven Website With JavaScript And Node.js
So, you’ve built an awesome data-driven website using JavaScript and Node.js. Congratulations! Now, the real fun begins: getting your creation out into the world. Deployment and hosting might seem daunting, but with the right approach, it’s a smooth process. This section will guide you through the essential steps, from choosing a platform to ensuring your site scales effectively.
Deploying your Node.js application involves transferring your code and its dependencies to a server where it can run continuously. Popular platforms like Heroku and Netlify simplify this process, offering user-friendly interfaces and automated deployment tools. The choice of platform often depends on factors such as project size, budget, and desired level of control.
Choosing a Hosting Platform
Selecting the right hosting platform is crucial. Heroku, known for its ease of use and scalability, is a great option for many Node.js projects. It handles server management, allowing developers to focus on their code. Netlify, on the other hand, excels in static site hosting and serverless functions, making it ideal for projects with a strong front-end focus. Other platforms like AWS, Google Cloud Platform, and DigitalOcean offer more granular control and customization but require a deeper understanding of server administration. The best platform for you will depend on your project’s specific needs and your comfort level with server management.
Scaling a Data-Driven Website
As your website grows in popularity, you’ll need to ensure it can handle the increased traffic and data load. Scaling involves adding more resources to your application to maintain performance and responsiveness. This can include scaling vertically (upgrading your server’s hardware) or horizontally (adding more servers to distribute the load). For data-driven websites, database scaling is particularly important. Techniques like database sharding (splitting the database across multiple servers) and caching (storing frequently accessed data in memory) are essential for handling large datasets efficiently. Consider using load balancers to distribute traffic evenly across multiple servers, preventing any single server from becoming overloaded. Netflix, for example, famously uses a microservices architecture and sophisticated load balancing to handle millions of concurrent users.
Monitoring and Logging Tools
Monitoring your website’s performance is vital for identifying and resolving issues promptly. Tools like Datadog, New Relic, and Prometheus provide comprehensive monitoring capabilities, tracking metrics such as response times, error rates, and resource utilization. Logging tools, such as Winston or Bunyan for Node.js, help you track events and errors within your application, providing valuable insights for debugging and performance optimization. These tools are indispensable for proactively identifying and addressing potential bottlenecks before they impact your users. Imagine a scenario where a spike in database queries causes slowdowns; a monitoring tool would alert you to this, allowing for timely intervention.
Deployment Checklist
Before deploying your website, a thorough checklist is essential to ensure a smooth process.
- Code Review: Ensure your code is well-tested and optimized.
- Environment Configuration: Double-check your environment variables and configurations on the hosting platform.
- Database Setup: Confirm your database is correctly configured and populated with data.
- Deployment Script: Use a deployment script to automate the process and minimize errors.
- Testing: Thoroughly test your website on the staging environment before deploying to production.
- Monitoring Setup: Configure your monitoring and logging tools to track website performance.
Following this checklist reduces the risk of deployment issues and ensures a stable launch. A well-planned deployment process saves time and frustration in the long run.
Advanced Techniques
So, you’ve built your data-driven website—congrats! But the journey doesn’t end there. To truly elevate your creation from “functional” to “fantastic,” you need to delve into some advanced techniques. Think of this as leveling up your website game, adding features that not only improve the user experience but also ensure your site can handle growth and complexity.
This section explores crucial techniques that will transform your website from a static display of information into a dynamic, responsive, and scalable powerhouse.
Real-time Data Updates with WebSockets
WebSockets provide a persistent connection between the client (your website’s front-end) and the server (your Node.js backend). This allows for real-time, bidirectional communication, unlike traditional HTTP requests which are request-response based. Imagine a stock ticker constantly updating, a live chat application, or a collaborative document editor—these are all powered by the magic of WebSockets. Using libraries like Socket.IO on both the client and server simplifies the process significantly. Data changes on the server are instantly reflected on the client, creating a truly dynamic and engaging user experience. For example, a social media feed could update with new posts in real-time without the user needing to refresh the page.
Handling Large Datasets Efficiently
Dealing with massive datasets can bring your website to its knees if not handled properly. Pagination is your best friend here—breaking down the data into smaller, manageable chunks presented to the user one page at a time. Efficient database queries are also essential; using appropriate indexing and optimizing your database schema are critical steps. Consider techniques like lazy loading, where data is fetched only when needed, instead of loading everything upfront. For instance, an e-commerce site with millions of products would benefit greatly from pagination and lazy loading of product images, preventing overwhelming the user’s browser.
Improving Website Performance and Scalability
Performance and scalability go hand in hand. A fast website is a happy website. Caching is your secret weapon here; caching frequently accessed data reduces the load on your server and speeds up response times. Content Delivery Networks (CDNs) distribute your website’s content across multiple servers globally, ensuring users experience faster load times regardless of their location. Load balancing distributes traffic across multiple servers, preventing overload on a single server. For example, a news website with millions of daily visitors would need a robust CDN and load balancing strategy to handle the traffic efficiently. Profiling your code to identify bottlenecks is also crucial for optimization.
User Authentication and Authorization
Security is paramount. A robust authentication and authorization system protects your users’ data and prevents unauthorized access. JSON Web Tokens (JWTs) are a popular choice for authentication, offering a secure and efficient way to verify user identity. Authorization determines what actions a user is permitted to perform. For instance, an admin user might have access to all features, while a regular user might only have access to certain sections of the website. Implementing role-based access control (RBAC) provides a structured way to manage user permissions. A well-designed system prevents unauthorized users from accessing sensitive information or performing actions they shouldn’t. This is critical for applications dealing with personal data or financial transactions.
Summary
Building a data-driven website with JavaScript and Node.js is a journey, not a sprint. But by following the steps Artikeld in this guide, you’ll have the skills and knowledge to create powerful, interactive websites that adapt to user needs. Remember, practice makes perfect. So start building, experiment, and don’t be afraid to break things – that’s how you learn! The possibilities are endless; go create something amazing.