The Basics of Web Security: Common Vulnerabilities and Fixes – sounds boring, right? Wrong! Think of your website as a fortress, constantly under siege from digital ninjas wielding SQL injection shurikens and XSS ninja stars. This isn’t some geeky tech mumbo-jumbo; it’s about protecting your online presence, your data, and your reputation. We’ll unravel the mysteries of common web vulnerabilities, from the sneaky SQL injection to the insidious Cross-Site Scripting (XSS), showing you how to patch those digital holes before the bad guys break in.
We’ll explore the most common attacks, explain how they work, and, most importantly, equip you with the knowledge to defend against them. Think of this as your ultimate guide to building an impenetrable digital fortress, one line of code at a time. Get ready to level up your web security game!
Introduction to Web Security
In today’s hyper-connected world, where nearly every aspect of our lives – from banking to social interactions – relies on the internet, web security is no longer a luxury but a fundamental necessity. The sheer volume of sensitive data transmitted and stored online makes robust security measures paramount, protecting individuals, businesses, and governments from the devastating consequences of cyberattacks. Ignoring web security is akin to leaving your front door unlocked – an open invitation for trouble.
Web security encompasses the practices and technologies designed to protect websites, web applications, and online data from unauthorized access, use, disclosure, disruption, modification, or destruction. Its key components include authentication (verifying user identity), authorization (controlling access to resources), data encryption (protecting data in transit and at rest), and intrusion detection/prevention (monitoring and responding to security threats). These components work in concert to create a layered defense against various cyber threats.
Real-World Web Security Breaches and Their Consequences
The consequences of inadequate web security can be catastrophic. Consider the 2017 Equifax data breach, where hackers stole the personal information of nearly 150 million people, including Social Security numbers, birth dates, and addresses. The fallout included massive financial losses for Equifax, legal battles, and a severe erosion of public trust. Similarly, the 2013 Target breach compromised millions of credit card numbers, resulting in significant financial losses for Target and lasting damage to its reputation. These examples highlight the tangible and intangible costs associated with poor web security – financial penalties, legal repercussions, reputational damage, and loss of customer trust. Even smaller-scale breaches can lead to significant disruption and financial hardship for businesses and individuals alike. The cost of inaction far outweighs the investment in robust security measures.
Common Web Vulnerabilities
Understanding common web vulnerabilities is crucial for building secure websites. Ignoring these weaknesses leaves your online presence vulnerable to attacks ranging from minor annoyances to catastrophic data breaches. This section details five of the most prevalent threats and how to mitigate them.
SQL Injection
SQL injection exploits vulnerabilities in how web applications handle user inputs. Attackers craft malicious SQL code within input fields (like search bars or login forms) to manipulate database queries. This allows them to access, modify, or delete sensitive data, potentially compromising entire databases. The mechanism involves injecting SQL commands that bypass intended functionality, executing unauthorized operations on the database server. For instance, an attacker might inject a string like `’ OR ‘1’=’1` into a login form, bypassing authentication checks.
Vulnerability Type | Description | Impact | Example |
SQL Injection | Malicious SQL code injected into user inputs to manipulate database queries. | Data breaches, unauthorized access, data modification or deletion. | Injecting `’ OR ‘1’=’1` into a login form to bypass authentication. |
Cross-Site Scripting (XSS)
Cross-site scripting (XSS) occurs when malicious scripts are injected into otherwise benign and trusted websites. These scripts can then be executed in the victim’s browser, allowing attackers to steal cookies, session tokens, or other sensitive information. The mechanism involves embedding JavaScript code within website content, often through user-submitted data. This code then executes within the victim’s browser context, granting the attacker access to the victim’s session and potentially their entire account. A common example is an attacker posting a malicious link on a forum; clicking this link might inject a script into the victim’s browser.
Vulnerability Type | Description | Impact | Example |
Cross-Site Scripting (XSS) | Malicious scripts injected into trusted websites, executed in the victim’s browser. | Session hijacking, data theft, redirecting users to malicious sites. | Malicious link on a forum injecting a script into the victim’s browser. |
Cross-Site Request Forgery (CSRF)
Cross-site request forgery (CSRF) tricks a user into performing unwanted actions on a website they’re already authenticated to. Attackers achieve this by crafting malicious links or forms that exploit the user’s existing session. The mechanism leverages the user’s authenticated session to perform actions without their knowledge or consent. Imagine an attacker creating a link that automatically transfers funds from a banking website – if a logged-in user clicks this link, the transaction might go through without their explicit authorization.
Vulnerability Type | Description | Impact | Example |
Cross-Site Request Forgery (CSRF) | Tricking a user into performing unwanted actions on a website they’re already authenticated to. | Unauthorized actions, financial loss, data manipulation. | Malicious link automatically transferring funds from a banking website. |
Broken Authentication and Session Management
Weak or improperly implemented authentication and session management mechanisms are a significant security risk. This can involve using predictable passwords, failing to properly handle session timeouts, or lacking robust password reset mechanisms. The mechanisms often involve inadequate password policies, lack of multi-factor authentication, and vulnerable session handling leading to session hijacking. A simple example is a website that only uses a username and password without any additional security measures.
Vulnerability Type | Description | Impact | Example |
Broken Authentication & Session Management | Weak or improperly implemented authentication and session management. | Account takeover, unauthorized access, data breaches. | Website using only username and password without additional security measures. |
Insecure Direct Object References
Insecure direct object references (IDOR) allow attackers to access resources they shouldn’t have access to by manipulating URLs or other input parameters. This often happens when a website directly exposes internal identifiers, such as database IDs, in URLs. The mechanism involves directly referencing objects (like files or database records) using predictable identifiers, allowing unauthorized access. For example, a URL like `/profile?id=123` might reveal information about user with ID 123, even if the attacker isn’t authorized to access that profile.
Vulnerability Type | Description | Impact | Example |
Insecure Direct Object References (IDOR) | Manipulating URLs or input parameters to access unauthorized resources. | Unauthorized access to sensitive data, data breaches, manipulation of resources. | Accessing a user profile via a URL like `/profile?id=123` without proper authorization. |
SQL Injection
SQL injection is a sneaky attack that exploits vulnerabilities in how web applications handle user inputs. Imagine a website that lets you log in using a username and password. A malicious user might try to inject malicious SQL code into the username field, manipulating the database query to gain unauthorized access or wreak havoc. It’s like slipping a secret message into a perfectly normal-looking envelope – the system processes it unknowingly, with potentially devastating consequences. This is a serious threat because databases hold sensitive information, and compromising them can lead to data breaches, financial losses, and reputational damage.
SQL injection attacks work by injecting malicious SQL code into the input fields of a web application. This code is then interpreted and executed by the database server, allowing the attacker to manipulate the database in various ways. Instead of simply retrieving data, the attacker can potentially read, modify, or delete data, bypass authentication mechanisms, or even execute arbitrary commands on the server. The consequences can range from minor inconvenience to a full-blown security disaster. The core issue lies in how the application handles and sanitizes user-provided data before sending it to the database.
Types of SQL Injection Vulnerabilities
There are several ways SQL injection can manifest. The vulnerability’s severity and the attacker’s potential impact depend heavily on the specific type and the application’s architecture. Improperly handled user inputs are the common denominator in all these variations.
- In-band SQL Injection: This is the most common type. The attacker injects malicious SQL code directly into the input field, and the results are returned within the application’s normal response. For example, an attacker might modify a login query to retrieve all usernames and passwords from the database.
- Blind SQL Injection: Here, the attacker cannot directly see the results of the injected SQL code. Instead, they infer information by observing changes in the application’s behavior or response time. This might involve using conditional statements in the injected code to check for the existence of specific data or database characteristics.
- Out-of-band SQL Injection: This sophisticated technique involves using the injected SQL code to send data outside the application’s normal communication channels, such as to a remote server controlled by the attacker. This often uses features like error messages or database functions to exfiltrate data.
Preventing SQL Injection Vulnerabilities
The best defense against SQL injection is proactive and multi-layered. It’s not enough to just hope for the best; a robust strategy is crucial.
The most effective method is to use parameterized queries or prepared statements. These techniques separate the data from the SQL code, preventing the injected code from being interpreted as part of the query. Instead of directly embedding user input into the SQL string, the data is treated as parameters. The database driver then handles the safe insertion of the parameters, neutralizing any malicious code.
Use parameterized queries or prepared statements. This is the gold standard for preventing SQL injection.
Another crucial step is input validation. Before accepting any user input, rigorously check its format, length, and data type. Reject or sanitize any input that doesn’t conform to the expected standards. This prevents unexpected characters or patterns from slipping through.
Regular security audits and penetration testing are also vital. These assessments can identify potential vulnerabilities before attackers find them. Keeping your database software and drivers updated is equally important, as patches often address known SQL injection vulnerabilities. Finally, employing a web application firewall (WAF) can provide an additional layer of protection by filtering out malicious traffic.
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) attacks are a sneaky way for hackers to inject malicious scripts into websites that unsuspecting users then visit. These scripts can steal sensitive information like passwords, credit card details, or even hijack user accounts. It’s like planting a hidden camera in a seemingly safe place – you wouldn’t know it’s there until it’s too late.
XSS attacks leverage the trust users place in websites. The attacker crafts malicious code, often hidden within seemingly harmless content, which is then executed by the user’s browser. This allows the attacker to perform actions on behalf of the victim, without them ever knowing. Think of it as a digital Trojan horse, hiding within a seemingly innocent package.
Reflected, Stored, and DOM-Based XSS Attacks
The three main types of XSS attacks – reflected, stored, and DOM-based – differ in how the malicious script reaches the victim’s browser. Understanding these differences is crucial for effective prevention.
Reflected XSS attacks occur when the malicious script is sent to the victim through a URL. The website then reflects this script back to the user’s browser without proper sanitization. Imagine a search engine where an attacker inserts a malicious script into the search query. If the engine displays the query without filtering, the script executes on the victim’s browser. The script is only temporary and disappears after the user leaves the page.
Stored XSS attacks, on the other hand, are more persistent. The malicious script is stored on the server, typically within a database. Every time a user accesses the affected page or content, the script executes. Think of a forum where an attacker posts a message containing malicious code. If the forum doesn’t sanitize user inputs, this code will be stored and executed whenever anyone views the post. This means the attack can affect many users over an extended period.
DOM-based XSS attacks are different. They target the Document Object Model (DOM) of the webpage, manipulating the client-side code directly within the user’s browser. This means the malicious script doesn’t necessarily need to be stored on the server or reflected from it. It can be injected through manipulation of existing website functionality, for instance, by exploiting a vulnerability in how the site handles JavaScript. This type of attack relies on manipulating the browser’s own interpretation of the code.
Examples of XSS Attacks and Their Impact
A reflected XSS attack might involve an attacker sending a link containing a malicious script to a victim via email. When the victim clicks the link, the script is reflected from the website and executed, potentially stealing their session cookie. This could grant the attacker access to the victim’s account on that website.
A stored XSS attack could be a malicious comment on a blog that, when viewed by other users, executes a script stealing their information. This could result in widespread data theft.
A DOM-based XSS attack could occur if a website uses user input to dynamically generate JavaScript code without proper validation. If the input contains malicious code, the browser will execute it, potentially compromising the user’s system.
The impact of XSS attacks can range from minor annoyances like pop-up ads to serious security breaches, leading to identity theft, financial loss, and data breaches. The severity depends on the nature of the injected script and the sensitivity of the data it targets. The consequences can be significant and long-lasting.
Cross-Site Request Forgery (CSRF): The Basics Of Web Security: Common Vulnerabilities And Fixes
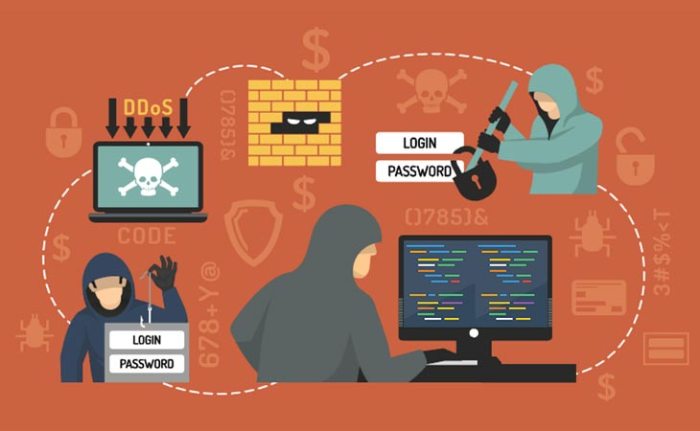
Source: sisainfosec.com
Cross-Site Request Forgery (CSRF), also known as a one-click attack, is a malicious exploit where an attacker tricks a victim into performing unwanted actions on a web application in which they’re currently authenticated. Unlike XSS, which injects malicious scripts, CSRF leverages the victim’s existing session to execute actions on their behalf, often without their knowledge or consent. This means the attacker doesn’t need to steal the victim’s credentials; they only need to trick them into clicking a link or visiting a compromised website.
CSRF attacks exploit the trust relationship between the user’s browser and the target website. The attacker crafts a malicious request, often hidden within an image or link on a seemingly innocuous website. When the victim, already logged into the target website, interacts with this malicious element, their browser automatically sends the request with their existing authentication cookies, allowing the attacker to perform actions like transferring funds, changing passwords, or posting unwanted content. The attack’s success relies on the victim’s already established session.
CSRF Prevention Techniques
Several effective techniques exist to mitigate the risk of CSRF attacks. Implementing these safeguards is crucial for protecting user accounts and data integrity. The most common and reliable methods focus on verifying that the request originates from the legitimate website and not from a malicious actor.
- Synchronizer Token Pattern: This involves generating a unique, unpredictable token for each form submission. The token is included in a hidden field within the form and validated on the server-side. If the token doesn’t match, the request is rejected. This ensures that only requests originating from the legitimate form are processed.
- HTTP Referer Header Check: While not entirely reliable, checking the HTTP Referer header can provide an additional layer of security. The Referer header indicates the origin of the request. However, it can be easily manipulated or omitted by the attacker, making it unsuitable as the sole prevention method.
- Double Submit Cookie: This method involves storing a randomly generated token in both a cookie and a hidden form field. The server compares the values of both upon submission. If they match, the request is considered legitimate.
Implementing CSRF Protection
Implementing CSRF protection requires careful consideration of both client-side and server-side measures. The most effective approach typically combines several techniques to provide robust protection. Here’s an example using the synchronizer token pattern in a simplified PHP scenario:
First, generate a unique token on the server-side and store it in a session variable:
Next, include the token in a hidden field within the form:
Finally, verify the token on the server-side before processing the form submission:
This example demonstrates a basic implementation; real-world applications often employ more sophisticated techniques and libraries for improved security. Remember that consistent updates and rigorous testing are essential for maintaining effective CSRF protection.
Session Management Vulnerabilities
Session management is a critical aspect of web security, responsible for tracking users’ interactions and maintaining their logged-in state. A poorly implemented session management system can leave your application vulnerable to a range of attacks, potentially compromising user data and control. Understanding these vulnerabilities and implementing robust security measures is paramount for any web application.
Session management vulnerabilities stem from weaknesses in how web applications identify and authenticate users, manage their sessions, and ultimately, control access to sensitive information. These weaknesses can be exploited by attackers to hijack sessions, impersonate users, or gain unauthorized access to restricted resources. The consequences can range from simple account takeovers to complete system compromise.
Session Hijacking
Session hijacking occurs when an attacker gains unauthorized access to a user’s session ID. This ID, often a randomly generated string, acts as a key to the user’s session data stored on the server. By obtaining this ID, an attacker can effectively impersonate the user, accessing their data and performing actions on their behalf. This can happen through various methods, including sniffing network traffic (if the session ID is transmitted insecurely, such as over HTTP), exploiting vulnerabilities in the application’s code to directly retrieve the session ID, or using cross-site scripting (XSS) to steal the ID from the victim’s browser. Imagine a scenario where an attacker intercepts a user’s session ID while the user is shopping online; the attacker could then use that ID to empty the user’s shopping cart and make purchases using the victim’s payment information.
Session Fixation
Session fixation is an attack where an attacker forces a user to use a specific session ID of their choosing. This pre-selected session ID might already be compromised or easily guessable. The attacker then waits for the victim to log in, using the provided session ID. Once the user is logged in with the manipulated session, the attacker can use the session ID to access the user’s account. This attack often relies on tricking the user into clicking a malicious link or visiting a compromised website. A real-world example could involve a phishing email containing a link to a fake login page that sets a pre-determined session ID. After the user logs in, the attacker has control over the session.
Predictable Session IDs
Using predictable session IDs is a significant security risk. If the session ID generation algorithm is weak or easily guessable (e.g., sequential numbers or easily reverse-engineered patterns), attackers can potentially guess valid session IDs and gain unauthorized access. This vulnerability can be exacerbated if the application doesn’t implement appropriate session timeout mechanisms. For instance, if an application generates session IDs incrementally, an attacker could potentially guess the next session ID after observing a few valid ones.
Best Practices for Secure Session Management
Secure session handling is crucial for protecting user accounts and sensitive data. Implementing the following best practices will significantly enhance the security of your web application.
- Use strong, unpredictable session IDs: Generate session IDs using cryptographically secure random number generators. Avoid predictable patterns or sequential numbering.
- Use HTTPS: Always transmit session IDs over HTTPS to prevent eavesdropping and session hijacking.
- Implement appropriate session timeouts: Set reasonable session timeout periods to automatically log users out after a period of inactivity. This limits the window of opportunity for attackers.
- Regularly rotate session IDs: Periodically regenerate session IDs to minimize the impact of compromised IDs.
- Use HTTPOnly cookies: This flag prevents client-side scripts (like JavaScript) from accessing session cookies, mitigating XSS attacks.
- Use Secure cookies: This flag ensures that session cookies are only transmitted over HTTPS.
- Validate session IDs on the server-side: Always verify the validity and integrity of session IDs on the server before granting access to resources.
- Implement robust input validation: Prevent session fixation attacks by thoroughly validating all user inputs related to session management.
- Avoid storing sensitive data in the session: Minimize the amount of sensitive data stored in the session to reduce the impact of a session compromise.
Authentication and Authorization Vulnerabilities
Securing user access is paramount for any web application. Authentication verifies the user’s identity, while authorization determines what actions they’re permitted to perform. Weaknesses in either process can lead to significant security breaches, ranging from unauthorized data access to complete system compromise. Let’s delve into the common vulnerabilities and best practices.
Authentication and authorization vulnerabilities stem from weak implementations or outright neglect of security best practices. These vulnerabilities often exploit flaws in password management, authentication protocols, or the way access permissions are handled. The consequences can be devastating, leading to data theft, account hijacking, and even complete application takeover. A robust security strategy needs to address both aspects holistically.
Understanding web security basics, like patching vulnerabilities and using strong passwords, is crucial for online safety. Just like regularly checking your web security, you should also review your financial protection; it’s smart to check in on things like your auto insurance annually, as highlighted in this helpful article: The Importance of Reviewing Your Auto Insurance Annually.
Proactive maintenance, whether it’s online or offline, keeps you covered and minimizes risks down the line.
Strong Password Policies
Strong password policies are the first line of defense against unauthorized access. Simply requiring a password isn’t enough; a robust policy mandates minimum length, complexity requirements (including uppercase, lowercase, numbers, and symbols), and regular password changes. Furthermore, password reuse across multiple accounts should be strictly prohibited. Implementing multi-factor authentication (MFA) adds an extra layer of security, requiring users to provide multiple forms of verification before granting access, even if their password is compromised. For example, a strong password policy might dictate a minimum length of 12 characters, with at least one uppercase letter, one lowercase letter, one number, and one special character, and require a password change every 90 days. Failing to enforce such a policy leaves the system vulnerable to brute-force attacks or easily guessable passwords.
Authentication Methods and Their Security Implications
Several authentication methods exist, each with its own security strengths and weaknesses. Password-based authentication, while widely used, is susceptible to brute-force attacks and phishing scams. Other methods offer enhanced security.
Here’s a comparison of common authentication methods:
Authentication Method | Security Implications |
---|---|
Password-based | Vulnerable to brute-force attacks, phishing, and credential stuffing. Requires strong password policies and MFA for better security. |
Multi-Factor Authentication (MFA) | Significantly enhances security by requiring multiple verification factors (e.g., password, one-time code, biometric scan). Reduces the risk of unauthorized access even if one factor is compromised. |
OAuth 2.0 | Allows users to grant access to third-party applications without sharing their credentials directly. Reduces the risk of credential theft but relies on the security of the authorization server. |
OpenID Connect (OIDC) | Builds upon OAuth 2.0 to provide an identity layer, allowing applications to verify the user’s identity and obtain user profile information. Offers improved security and user experience compared to traditional password-based authentication. |
Biometric Authentication | Uses unique biological characteristics (e.g., fingerprints, facial recognition) for authentication. Offers strong security but can be vulnerable to spoofing attacks if not implemented properly. |
Fixing Web Vulnerabilities
So, you’ve identified some nasty vulnerabilities lurking in your web application. Don’t panic! Fixing these issues is crucial for maintaining the security and integrity of your site, preventing data breaches, and protecting your users. This section provides a practical guide to tackling common vulnerabilities, from identifying the problem to implementing effective solutions. Remember, a proactive approach is key – regular security checks are your best defense.
Identifying and Fixing Common Web Vulnerabilities
Identifying and patching vulnerabilities requires a systematic approach. First, you need to perform a thorough security audit, possibly involving penetration testing. This helps uncover hidden weaknesses. Once vulnerabilities are identified, the remediation process begins. This usually involves updating software, patching code, implementing secure coding practices, and configuring security settings correctly. For example, if a SQL injection vulnerability is discovered, the solution might involve parameterized queries or input sanitization. Similarly, fixing a cross-site scripting (XSS) vulnerability might require output encoding or input validation. Addressing CSRF often involves using tokens or implementing the double-submit cookie pattern. Each vulnerability demands a specific solution, and understanding the root cause is crucial for effective remediation.
Regular Security Audits and Penetration Testing
Regular security audits and penetration testing are not just good practice; they are essential for maintaining a robust security posture. Think of them as your website’s annual health check-up. Security audits involve systematic reviews of your code, infrastructure, and security configurations to identify potential weaknesses. Penetration testing, on the other hand, simulates real-world attacks to expose vulnerabilities that might have been missed during an audit. These tests can reveal critical flaws before malicious actors do, saving you significant time, money, and potential reputational damage. Scheduling regular audits and penetration tests (ideally, at least annually, and more frequently for high-risk applications) is vital. Consider involving external security experts for a more objective assessment. For example, a company like OWASP can provide resources and guidance for performing these assessments.
Web Application Firewalls (WAFs) Comparison
Web Application Firewalls (WAFs) act as a crucial layer of defense, filtering malicious traffic before it reaches your web application. Choosing the right WAF depends on your specific needs and budget. Below is a comparison of some popular WAFs. Note that features and pricing can change, so always refer to the vendor’s website for the most up-to-date information.
WAF | Features | Pricing Model | Strengths | Weaknesses |
---|---|---|---|---|
Cloudflare WAF | Extensive rule sets, DDoS protection, bot management | Pay-as-you-go, tiered plans | Ease of use, robust features, global network | Can be complex to configure for advanced users |
AWS WAF | Integration with AWS services, customizable rules, rate limiting | Pay-as-you-go | Seamless integration with AWS ecosystem, scalability | Steeper learning curve compared to some competitors |
Akamai Kona Site Defender | Advanced DDoS protection, bot management, web application security | Subscription-based | Excellent performance, strong DDoS mitigation | Can be expensive, complex configuration |
Imperva WAF | Machine learning-based threat detection, comprehensive security features | Subscription-based | Advanced threat detection capabilities | Can be expensive |
Secure Coding Practices
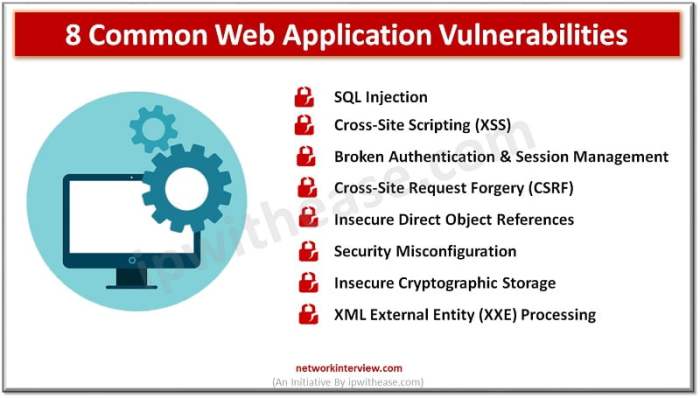
Source: networkinterview.com
Building secure web applications isn’t just about slapping on security patches after the fact; it’s about baking security into every line of code. Secure coding practices are the foundation of a robust and resilient web application, preventing vulnerabilities before they even have a chance to emerge. This involves a proactive approach, embedding security considerations throughout the entire software development lifecycle.
This section delves into the essential secure coding practices that developers should adopt to minimize the risk of common web vulnerabilities. We’ll explore how these practices directly address the vulnerabilities discussed earlier, like SQL injection and XSS, by preventing them from ever taking hold.
Input Validation and Sanitization
Input validation and sanitization are critical for preventing many common vulnerabilities. Input validation ensures that data received from users conforms to predefined rules and formats. Sanitization, on the other hand, removes or neutralizes potentially harmful characters from user input before it’s used in the application. Failing to perform these steps leaves your application wide open to attacks. For example, without input validation, a user could submit malicious SQL code within a search field, leading to an SQL injection attack. Similarly, unsanitized user input can allow attackers to inject malicious JavaScript code, leading to a cross-site scripting (XSS) attack.
Secure Coding Guidelines for Developers, The Basics of Web Security: Common Vulnerabilities and Fixes
Effective secure coding guidelines provide a framework for developers to follow. These guidelines should be integrated into the development process, acting as a checklist for developers to follow during every stage of development. They should cover various aspects, from secure coding standards to secure design patterns and best practices. For instance, guidelines should explicitly mandate input validation and sanitization for all user inputs, regardless of the source (web forms, APIs, etc.). They should also emphasize the use of parameterized queries to prevent SQL injection and the proper encoding of user data to prevent XSS. Regular security training and code reviews are crucial to ensure adherence to these guidelines. Furthermore, adopting a secure development lifecycle (SDLC) methodology, which incorporates security testing at each phase, is essential. This might include static analysis, dynamic analysis, and penetration testing to identify and address vulnerabilities early in the development process. Examples of specific secure coding practices include:
- Always validate and sanitize user inputs before using them in database queries or displaying them on web pages.
- Use parameterized queries or prepared statements to prevent SQL injection vulnerabilities.
- Encode all user-supplied data before displaying it on web pages to prevent cross-site scripting (XSS) vulnerabilities.
- Implement robust session management techniques to prevent session hijacking and other session-related vulnerabilities.
- Use strong cryptography for authentication and authorization, and store passwords securely using one-way hashing techniques.
- Regularly update software and libraries to patch known security vulnerabilities.
- Perform thorough security testing throughout the software development lifecycle.
Implementing these guidelines not only prevents vulnerabilities but also reduces the risk of data breaches, improves application security, and enhances the overall user experience.
Importance of Regular Updates and Patches
Think of your website’s software as a house. A well-maintained house requires regular upkeep – painting, repairs, and pest control. Similarly, your website’s software needs regular updates and patches to stay secure and function optimally. Ignoring these updates leaves your digital dwelling vulnerable to intruders and malfunctions.
Outdated software is a breeding ground for security vulnerabilities. Hackers constantly scan the internet for weaknesses, and known vulnerabilities in older versions of software are prime targets. These vulnerabilities can be exploited to gain unauthorized access, steal data, inject malicious code, or even completely cripple your website. The longer you delay updates, the greater the risk becomes, essentially making your website a sitting duck for cyberattacks. This not only poses a threat to your data and reputation but also carries significant financial and legal repercussions.
Risks Associated with Outdated Software
Failing to update software exposes your website to a wide range of threats. These include malware infections, data breaches, denial-of-service attacks, and unauthorized access. For example, an outdated CMS (Content Management System) might have a known vulnerability that allows hackers to upload malicious files, potentially leading to a complete compromise of your website. Furthermore, neglecting security patches can lead to hefty fines and legal action if you handle sensitive user data, as regulations like GDPR require organizations to maintain a certain level of security. The financial costs associated with a data breach, including legal fees, remediation efforts, and reputational damage, can far outweigh the time and effort required to keep your software updated.
Resources for Checking Software Updates
Staying informed about software updates is crucial for maintaining a secure online presence. Several resources can help you track updates and ensure your software is always up-to-date. Many software providers offer automatic update features; however, it’s good practice to manually check for updates periodically. You can typically find update notifications on the software vendor’s website, often in a dedicated support or download section. Additionally, subscribing to security newsletters and following industry blogs can provide valuable insights into emerging threats and relevant patches. Finally, consider utilizing vulnerability scanning tools, which automatically check your systems for known vulnerabilities and suggest necessary updates. These tools can greatly simplify the update process and ensure that your website remains protected against the latest threats.
Concluding Remarks
So, you’ve journeyed through the wild west of web security, dodged digital bullets, and learned how to fortify your online kingdom. Remember, web security isn’t a one-time fix; it’s an ongoing battle. Staying updated, practicing secure coding, and regularly auditing your systems are crucial. Think of it like this: you wouldn’t leave your front door unlocked, right? The same principle applies to your website. Stay vigilant, stay secure, and keep those digital ninjas at bay!